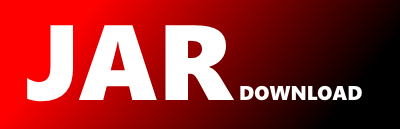
com.google.crypto.tink.proto.Keyset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tink Show documentation
Show all versions of tink Show documentation
Tink is a small cryptographic library that provides a safe, simple, agile and fast way to accomplish some common cryptographic tasks.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: proto/tink.proto
// Protobuf Java Version: 4.28.2
package com.google.crypto.tink.proto;
/**
*
* A Tink user works usually not with single keys, but with keysets,
* to enable key rotation. The keys in a keyset can belong to different
* implementations/key types, but must all implement the same primitive.
* Any given keyset (and any given key) can be used for one primitive only.
*
*
* Protobuf type {@code google.crypto.tink.Keyset}
*/
public final class Keyset extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:google.crypto.tink.Keyset)
KeysetOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Keyset.class.getName());
}
// Use Keyset.newBuilder() to construct.
private Keyset(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Keyset() {
key_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.Keyset.class, com.google.crypto.tink.proto.Keyset.Builder.class);
}
public interface KeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.crypto.tink.Keyset.Key)
com.google.protobuf.MessageOrBuilder {
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
* @return Whether the keyData field is set.
*/
boolean hasKeyData();
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
* @return The keyData.
*/
com.google.crypto.tink.proto.KeyData getKeyData();
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
com.google.crypto.tink.proto.KeyDataOrBuilder getKeyDataOrBuilder();
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @return The status.
*/
com.google.crypto.tink.proto.KeyStatusType getStatus();
/**
*
* Identifies a key within a keyset, is a part of metadata
* of a ciphertext/signature.
*
*
* uint32 key_id = 3;
* @return The keyId.
*/
int getKeyId();
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The enum numeric value on the wire for outputPrefixType.
*/
int getOutputPrefixTypeValue();
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The outputPrefixType.
*/
com.google.crypto.tink.proto.OutputPrefixType getOutputPrefixType();
}
/**
* Protobuf type {@code google.crypto.tink.Keyset.Key}
*/
public static final class Key extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:google.crypto.tink.Keyset.Key)
KeyOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Key.class.getName());
}
// Use Key.newBuilder() to construct.
private Key(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Key() {
status_ = 0;
outputPrefixType_ = 0;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_Key_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_Key_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.Keyset.Key.class, com.google.crypto.tink.proto.Keyset.Key.Builder.class);
}
private int bitField0_;
public static final int KEY_DATA_FIELD_NUMBER = 1;
private com.google.crypto.tink.proto.KeyData keyData_;
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
* @return Whether the keyData field is set.
*/
@java.lang.Override
public boolean hasKeyData() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
* @return The keyData.
*/
@java.lang.Override
public com.google.crypto.tink.proto.KeyData getKeyData() {
return keyData_ == null ? com.google.crypto.tink.proto.KeyData.getDefaultInstance() : keyData_;
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
@java.lang.Override
public com.google.crypto.tink.proto.KeyDataOrBuilder getKeyDataOrBuilder() {
return keyData_ == null ? com.google.crypto.tink.proto.KeyData.getDefaultInstance() : keyData_;
}
public static final int STATUS_FIELD_NUMBER = 2;
private int status_ = 0;
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @return The status.
*/
@java.lang.Override public com.google.crypto.tink.proto.KeyStatusType getStatus() {
com.google.crypto.tink.proto.KeyStatusType result = com.google.crypto.tink.proto.KeyStatusType.forNumber(status_);
return result == null ? com.google.crypto.tink.proto.KeyStatusType.UNRECOGNIZED : result;
}
public static final int KEY_ID_FIELD_NUMBER = 3;
private int keyId_ = 0;
/**
*
* Identifies a key within a keyset, is a part of metadata
* of a ciphertext/signature.
*
*
* uint32 key_id = 3;
* @return The keyId.
*/
@java.lang.Override
public int getKeyId() {
return keyId_;
}
public static final int OUTPUT_PREFIX_TYPE_FIELD_NUMBER = 4;
private int outputPrefixType_ = 0;
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The enum numeric value on the wire for outputPrefixType.
*/
@java.lang.Override public int getOutputPrefixTypeValue() {
return outputPrefixType_;
}
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The outputPrefixType.
*/
@java.lang.Override public com.google.crypto.tink.proto.OutputPrefixType getOutputPrefixType() {
com.google.crypto.tink.proto.OutputPrefixType result = com.google.crypto.tink.proto.OutputPrefixType.forNumber(outputPrefixType_);
return result == null ? com.google.crypto.tink.proto.OutputPrefixType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getKeyData());
}
if (status_ != com.google.crypto.tink.proto.KeyStatusType.UNKNOWN_STATUS.getNumber()) {
output.writeEnum(2, status_);
}
if (keyId_ != 0) {
output.writeUInt32(3, keyId_);
}
if (outputPrefixType_ != com.google.crypto.tink.proto.OutputPrefixType.UNKNOWN_PREFIX.getNumber()) {
output.writeEnum(4, outputPrefixType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getKeyData());
}
if (status_ != com.google.crypto.tink.proto.KeyStatusType.UNKNOWN_STATUS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_);
}
if (keyId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, keyId_);
}
if (outputPrefixType_ != com.google.crypto.tink.proto.OutputPrefixType.UNKNOWN_PREFIX.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, outputPrefixType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.crypto.tink.proto.Keyset.Key)) {
return super.equals(obj);
}
com.google.crypto.tink.proto.Keyset.Key other = (com.google.crypto.tink.proto.Keyset.Key) obj;
if (hasKeyData() != other.hasKeyData()) return false;
if (hasKeyData()) {
if (!getKeyData()
.equals(other.getKeyData())) return false;
}
if (status_ != other.status_) return false;
if (getKeyId()
!= other.getKeyId()) return false;
if (outputPrefixType_ != other.outputPrefixType_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKeyData()) {
hash = (37 * hash) + KEY_DATA_FIELD_NUMBER;
hash = (53 * hash) + getKeyData().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + getKeyId();
hash = (37 * hash) + OUTPUT_PREFIX_TYPE_FIELD_NUMBER;
hash = (53 * hash) + outputPrefixType_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset.Key parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.Keyset.Key parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.Keyset.Key parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.crypto.tink.proto.Keyset.Key prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code google.crypto.tink.Keyset.Key}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:google.crypto.tink.Keyset.Key)
com.google.crypto.tink.proto.Keyset.KeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_Key_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_Key_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.Keyset.Key.class, com.google.crypto.tink.proto.Keyset.Key.Builder.class);
}
// Construct using com.google.crypto.tink.proto.Keyset.Key.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getKeyDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
keyData_ = null;
if (keyDataBuilder_ != null) {
keyDataBuilder_.dispose();
keyDataBuilder_ = null;
}
status_ = 0;
keyId_ = 0;
outputPrefixType_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_Key_descriptor;
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset.Key getDefaultInstanceForType() {
return com.google.crypto.tink.proto.Keyset.Key.getDefaultInstance();
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset.Key build() {
com.google.crypto.tink.proto.Keyset.Key result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset.Key buildPartial() {
com.google.crypto.tink.proto.Keyset.Key result = new com.google.crypto.tink.proto.Keyset.Key(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.crypto.tink.proto.Keyset.Key result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.keyData_ = keyDataBuilder_ == null
? keyData_
: keyDataBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.status_ = status_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.keyId_ = keyId_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.outputPrefixType_ = outputPrefixType_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.crypto.tink.proto.Keyset.Key) {
return mergeFrom((com.google.crypto.tink.proto.Keyset.Key)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.crypto.tink.proto.Keyset.Key other) {
if (other == com.google.crypto.tink.proto.Keyset.Key.getDefaultInstance()) return this;
if (other.hasKeyData()) {
mergeKeyData(other.getKeyData());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.getKeyId() != 0) {
setKeyId(other.getKeyId());
}
if (other.outputPrefixType_ != 0) {
setOutputPrefixTypeValue(other.getOutputPrefixTypeValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getKeyDataFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
status_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
keyId_ = input.readUInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
outputPrefixType_ = input.readEnum();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.crypto.tink.proto.KeyData keyData_;
private com.google.protobuf.SingleFieldBuilder<
com.google.crypto.tink.proto.KeyData, com.google.crypto.tink.proto.KeyData.Builder, com.google.crypto.tink.proto.KeyDataOrBuilder> keyDataBuilder_;
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
* @return Whether the keyData field is set.
*/
public boolean hasKeyData() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
* @return The keyData.
*/
public com.google.crypto.tink.proto.KeyData getKeyData() {
if (keyDataBuilder_ == null) {
return keyData_ == null ? com.google.crypto.tink.proto.KeyData.getDefaultInstance() : keyData_;
} else {
return keyDataBuilder_.getMessage();
}
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
public Builder setKeyData(com.google.crypto.tink.proto.KeyData value) {
if (keyDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
keyData_ = value;
} else {
keyDataBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
public Builder setKeyData(
com.google.crypto.tink.proto.KeyData.Builder builderForValue) {
if (keyDataBuilder_ == null) {
keyData_ = builderForValue.build();
} else {
keyDataBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
public Builder mergeKeyData(com.google.crypto.tink.proto.KeyData value) {
if (keyDataBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
keyData_ != null &&
keyData_ != com.google.crypto.tink.proto.KeyData.getDefaultInstance()) {
getKeyDataBuilder().mergeFrom(value);
} else {
keyData_ = value;
}
} else {
keyDataBuilder_.mergeFrom(value);
}
if (keyData_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
public Builder clearKeyData() {
bitField0_ = (bitField0_ & ~0x00000001);
keyData_ = null;
if (keyDataBuilder_ != null) {
keyDataBuilder_.dispose();
keyDataBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
public com.google.crypto.tink.proto.KeyData.Builder getKeyDataBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getKeyDataFieldBuilder().getBuilder();
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
public com.google.crypto.tink.proto.KeyDataOrBuilder getKeyDataOrBuilder() {
if (keyDataBuilder_ != null) {
return keyDataBuilder_.getMessageOrBuilder();
} else {
return keyData_ == null ?
com.google.crypto.tink.proto.KeyData.getDefaultInstance() : keyData_;
}
}
/**
*
* Contains the actual, instantiation specific key proto.
* By convention, each key proto contains a version field.
*
*
* .google.crypto.tink.KeyData key_data = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.crypto.tink.proto.KeyData, com.google.crypto.tink.proto.KeyData.Builder, com.google.crypto.tink.proto.KeyDataOrBuilder>
getKeyDataFieldBuilder() {
if (keyDataBuilder_ == null) {
keyDataBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.crypto.tink.proto.KeyData, com.google.crypto.tink.proto.KeyData.Builder, com.google.crypto.tink.proto.KeyDataOrBuilder>(
getKeyData(),
getParentForChildren(),
isClean());
keyData_ = null;
}
return keyDataBuilder_;
}
private int status_ = 0;
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @return The status.
*/
@java.lang.Override
public com.google.crypto.tink.proto.KeyStatusType getStatus() {
com.google.crypto.tink.proto.KeyStatusType result = com.google.crypto.tink.proto.KeyStatusType.forNumber(status_);
return result == null ? com.google.crypto.tink.proto.KeyStatusType.UNRECOGNIZED : result;
}
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.crypto.tink.proto.KeyStatusType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* .google.crypto.tink.KeyStatusType status = 2;
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000002);
status_ = 0;
onChanged();
return this;
}
private int keyId_ ;
/**
*
* Identifies a key within a keyset, is a part of metadata
* of a ciphertext/signature.
*
*
* uint32 key_id = 3;
* @return The keyId.
*/
@java.lang.Override
public int getKeyId() {
return keyId_;
}
/**
*
* Identifies a key within a keyset, is a part of metadata
* of a ciphertext/signature.
*
*
* uint32 key_id = 3;
* @param value The keyId to set.
* @return This builder for chaining.
*/
public Builder setKeyId(int value) {
keyId_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Identifies a key within a keyset, is a part of metadata
* of a ciphertext/signature.
*
*
* uint32 key_id = 3;
* @return This builder for chaining.
*/
public Builder clearKeyId() {
bitField0_ = (bitField0_ & ~0x00000004);
keyId_ = 0;
onChanged();
return this;
}
private int outputPrefixType_ = 0;
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The enum numeric value on the wire for outputPrefixType.
*/
@java.lang.Override public int getOutputPrefixTypeValue() {
return outputPrefixType_;
}
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @param value The enum numeric value on the wire for outputPrefixType to set.
* @return This builder for chaining.
*/
public Builder setOutputPrefixTypeValue(int value) {
outputPrefixType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The outputPrefixType.
*/
@java.lang.Override
public com.google.crypto.tink.proto.OutputPrefixType getOutputPrefixType() {
com.google.crypto.tink.proto.OutputPrefixType result = com.google.crypto.tink.proto.OutputPrefixType.forNumber(outputPrefixType_);
return result == null ? com.google.crypto.tink.proto.OutputPrefixType.UNRECOGNIZED : result;
}
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @param value The outputPrefixType to set.
* @return This builder for chaining.
*/
public Builder setOutputPrefixType(com.google.crypto.tink.proto.OutputPrefixType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
outputPrefixType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Determines the prefix of the ciphertexts/signatures produced by this key.
* This value is copied verbatim from the key template.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return This builder for chaining.
*/
public Builder clearOutputPrefixType() {
bitField0_ = (bitField0_ & ~0x00000008);
outputPrefixType_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:google.crypto.tink.Keyset.Key)
}
// @@protoc_insertion_point(class_scope:google.crypto.tink.Keyset.Key)
private static final com.google.crypto.tink.proto.Keyset.Key DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.crypto.tink.proto.Keyset.Key();
}
public static com.google.crypto.tink.proto.Keyset.Key getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Key parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset.Key getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int PRIMARY_KEY_ID_FIELD_NUMBER = 1;
private int primaryKeyId_ = 0;
/**
*
* Identifies key used to generate new crypto data (encrypt, sign).
* Required.
*
*
* uint32 primary_key_id = 1;
* @return The primaryKeyId.
*/
@java.lang.Override
public int getPrimaryKeyId() {
return primaryKeyId_;
}
public static final int KEY_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List key_;
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
@java.lang.Override
public java.util.List getKeyList() {
return key_;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
@java.lang.Override
public java.util.List extends com.google.crypto.tink.proto.Keyset.KeyOrBuilder>
getKeyOrBuilderList() {
return key_;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
@java.lang.Override
public int getKeyCount() {
return key_.size();
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
@java.lang.Override
public com.google.crypto.tink.proto.Keyset.Key getKey(int index) {
return key_.get(index);
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
@java.lang.Override
public com.google.crypto.tink.proto.Keyset.KeyOrBuilder getKeyOrBuilder(
int index) {
return key_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (primaryKeyId_ != 0) {
output.writeUInt32(1, primaryKeyId_);
}
for (int i = 0; i < key_.size(); i++) {
output.writeMessage(2, key_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (primaryKeyId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, primaryKeyId_);
}
for (int i = 0; i < key_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, key_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.crypto.tink.proto.Keyset)) {
return super.equals(obj);
}
com.google.crypto.tink.proto.Keyset other = (com.google.crypto.tink.proto.Keyset) obj;
if (getPrimaryKeyId()
!= other.getPrimaryKeyId()) return false;
if (!getKeyList()
.equals(other.getKeyList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PRIMARY_KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + getPrimaryKeyId();
if (getKeyCount() > 0) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKeyList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.Keyset parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.Keyset parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.crypto.tink.proto.Keyset prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A Tink user works usually not with single keys, but with keysets,
* to enable key rotation. The keys in a keyset can belong to different
* implementations/key types, but must all implement the same primitive.
* Any given keyset (and any given key) can be used for one primitive only.
*
*
* Protobuf type {@code google.crypto.tink.Keyset}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:google.crypto.tink.Keyset)
com.google.crypto.tink.proto.KeysetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.Keyset.class, com.google.crypto.tink.proto.Keyset.Builder.class);
}
// Construct using com.google.crypto.tink.proto.Keyset.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
primaryKeyId_ = 0;
if (keyBuilder_ == null) {
key_ = java.util.Collections.emptyList();
} else {
key_ = null;
keyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_Keyset_descriptor;
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset getDefaultInstanceForType() {
return com.google.crypto.tink.proto.Keyset.getDefaultInstance();
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset build() {
com.google.crypto.tink.proto.Keyset result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset buildPartial() {
com.google.crypto.tink.proto.Keyset result = new com.google.crypto.tink.proto.Keyset(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.crypto.tink.proto.Keyset result) {
if (keyBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
key_ = java.util.Collections.unmodifiableList(key_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
}
private void buildPartial0(com.google.crypto.tink.proto.Keyset result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.primaryKeyId_ = primaryKeyId_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.crypto.tink.proto.Keyset) {
return mergeFrom((com.google.crypto.tink.proto.Keyset)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.crypto.tink.proto.Keyset other) {
if (other == com.google.crypto.tink.proto.Keyset.getDefaultInstance()) return this;
if (other.getPrimaryKeyId() != 0) {
setPrimaryKeyId(other.getPrimaryKeyId());
}
if (keyBuilder_ == null) {
if (!other.key_.isEmpty()) {
if (key_.isEmpty()) {
key_ = other.key_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureKeyIsMutable();
key_.addAll(other.key_);
}
onChanged();
}
} else {
if (!other.key_.isEmpty()) {
if (keyBuilder_.isEmpty()) {
keyBuilder_.dispose();
keyBuilder_ = null;
key_ = other.key_;
bitField0_ = (bitField0_ & ~0x00000002);
keyBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getKeyFieldBuilder() : null;
} else {
keyBuilder_.addAllMessages(other.key_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
primaryKeyId_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
com.google.crypto.tink.proto.Keyset.Key m =
input.readMessage(
com.google.crypto.tink.proto.Keyset.Key.parser(),
extensionRegistry);
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.add(m);
} else {
keyBuilder_.addMessage(m);
}
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int primaryKeyId_ ;
/**
*
* Identifies key used to generate new crypto data (encrypt, sign).
* Required.
*
*
* uint32 primary_key_id = 1;
* @return The primaryKeyId.
*/
@java.lang.Override
public int getPrimaryKeyId() {
return primaryKeyId_;
}
/**
*
* Identifies key used to generate new crypto data (encrypt, sign).
* Required.
*
*
* uint32 primary_key_id = 1;
* @param value The primaryKeyId to set.
* @return This builder for chaining.
*/
public Builder setPrimaryKeyId(int value) {
primaryKeyId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Identifies key used to generate new crypto data (encrypt, sign).
* Required.
*
*
* uint32 primary_key_id = 1;
* @return This builder for chaining.
*/
public Builder clearPrimaryKeyId() {
bitField0_ = (bitField0_ & ~0x00000001);
primaryKeyId_ = 0;
onChanged();
return this;
}
private java.util.List key_ =
java.util.Collections.emptyList();
private void ensureKeyIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
key_ = new java.util.ArrayList(key_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.crypto.tink.proto.Keyset.Key, com.google.crypto.tink.proto.Keyset.Key.Builder, com.google.crypto.tink.proto.Keyset.KeyOrBuilder> keyBuilder_;
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public java.util.List getKeyList() {
if (keyBuilder_ == null) {
return java.util.Collections.unmodifiableList(key_);
} else {
return keyBuilder_.getMessageList();
}
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public int getKeyCount() {
if (keyBuilder_ == null) {
return key_.size();
} else {
return keyBuilder_.getCount();
}
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public com.google.crypto.tink.proto.Keyset.Key getKey(int index) {
if (keyBuilder_ == null) {
return key_.get(index);
} else {
return keyBuilder_.getMessage(index);
}
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder setKey(
int index, com.google.crypto.tink.proto.Keyset.Key value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.set(index, value);
onChanged();
} else {
keyBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder setKey(
int index, com.google.crypto.tink.proto.Keyset.Key.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.set(index, builderForValue.build());
onChanged();
} else {
keyBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder addKey(com.google.crypto.tink.proto.Keyset.Key value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.add(value);
onChanged();
} else {
keyBuilder_.addMessage(value);
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder addKey(
int index, com.google.crypto.tink.proto.Keyset.Key value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.add(index, value);
onChanged();
} else {
keyBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder addKey(
com.google.crypto.tink.proto.Keyset.Key.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.add(builderForValue.build());
onChanged();
} else {
keyBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder addKey(
int index, com.google.crypto.tink.proto.Keyset.Key.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.add(index, builderForValue.build());
onChanged();
} else {
keyBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder addAllKey(
java.lang.Iterable extends com.google.crypto.tink.proto.Keyset.Key> values) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, key_);
onChanged();
} else {
keyBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
keyBuilder_.clear();
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public Builder removeKey(int index) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.remove(index);
onChanged();
} else {
keyBuilder_.remove(index);
}
return this;
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public com.google.crypto.tink.proto.Keyset.Key.Builder getKeyBuilder(
int index) {
return getKeyFieldBuilder().getBuilder(index);
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public com.google.crypto.tink.proto.Keyset.KeyOrBuilder getKeyOrBuilder(
int index) {
if (keyBuilder_ == null) {
return key_.get(index); } else {
return keyBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public java.util.List extends com.google.crypto.tink.proto.Keyset.KeyOrBuilder>
getKeyOrBuilderList() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(key_);
}
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public com.google.crypto.tink.proto.Keyset.Key.Builder addKeyBuilder() {
return getKeyFieldBuilder().addBuilder(
com.google.crypto.tink.proto.Keyset.Key.getDefaultInstance());
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public com.google.crypto.tink.proto.Keyset.Key.Builder addKeyBuilder(
int index) {
return getKeyFieldBuilder().addBuilder(
index, com.google.crypto.tink.proto.Keyset.Key.getDefaultInstance());
}
/**
*
* Actual keys in the Keyset.
* Required.
*
*
* repeated .google.crypto.tink.Keyset.Key key = 2;
*/
public java.util.List
getKeyBuilderList() {
return getKeyFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.crypto.tink.proto.Keyset.Key, com.google.crypto.tink.proto.Keyset.Key.Builder, com.google.crypto.tink.proto.Keyset.KeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.google.crypto.tink.proto.Keyset.Key, com.google.crypto.tink.proto.Keyset.Key.Builder, com.google.crypto.tink.proto.Keyset.KeyOrBuilder>(
key_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
// @@protoc_insertion_point(builder_scope:google.crypto.tink.Keyset)
}
// @@protoc_insertion_point(class_scope:google.crypto.tink.Keyset)
private static final com.google.crypto.tink.proto.Keyset DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.crypto.tink.proto.Keyset();
}
public static com.google.crypto.tink.proto.Keyset getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Keyset parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.crypto.tink.proto.Keyset getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy