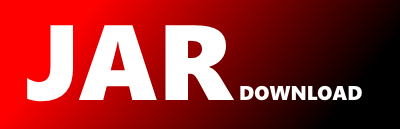
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tink Show documentation
Show all versions of tink Show documentation
Tink is a small cryptographic library that provides a safe, simple, agile and fast way to accomplish some common cryptographic tasks.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: proto/rsa_ssa_pkcs1.proto
package com.google.crypto.tink.proto;
/**
*
* key_type: type.googleapis.com/google.crypto.tink.RsaSsaPkcs1PrivateKey
*
*
* Protobuf type {@code google.crypto.tink.RsaSsaPkcs1PrivateKey}
*/
public final class RsaSsaPkcs1PrivateKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.crypto.tink.RsaSsaPkcs1PrivateKey)
RsaSsaPkcs1PrivateKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use RsaSsaPkcs1PrivateKey.newBuilder() to construct.
private RsaSsaPkcs1PrivateKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RsaSsaPkcs1PrivateKey() {
d_ = com.google.protobuf.ByteString.EMPTY;
p_ = com.google.protobuf.ByteString.EMPTY;
q_ = com.google.protobuf.ByteString.EMPTY;
dp_ = com.google.protobuf.ByteString.EMPTY;
dq_ = com.google.protobuf.ByteString.EMPTY;
crt_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RsaSsaPkcs1PrivateKey();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RsaSsaPkcs1PrivateKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
version_ = input.readUInt32();
break;
}
case 18: {
com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.Builder subBuilder = null;
if (publicKey_ != null) {
subBuilder = publicKey_.toBuilder();
}
publicKey_ = input.readMessage(com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(publicKey_);
publicKey_ = subBuilder.buildPartial();
}
break;
}
case 26: {
d_ = input.readBytes();
break;
}
case 34: {
p_ = input.readBytes();
break;
}
case 42: {
q_ = input.readBytes();
break;
}
case 50: {
dp_ = input.readBytes();
break;
}
case 58: {
dq_ = input.readBytes();
break;
}
case 66: {
crt_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.RsaSsaPkcs1.internal_static_google_crypto_tink_RsaSsaPkcs1PrivateKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.RsaSsaPkcs1.internal_static_google_crypto_tink_RsaSsaPkcs1PrivateKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey.class, com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey.Builder.class);
}
public static final int VERSION_FIELD_NUMBER = 1;
private int version_;
/**
*
* Required.
*
*
* uint32 version = 1;
*/
public int getVersion() {
return version_;
}
public static final int PUBLIC_KEY_FIELD_NUMBER = 2;
private com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey publicKey_;
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public boolean hasPublicKey() {
return publicKey_ != null;
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey getPublicKey() {
return publicKey_ == null ? com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.getDefaultInstance() : publicKey_;
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public com.google.crypto.tink.proto.RsaSsaPkcs1PublicKeyOrBuilder getPublicKeyOrBuilder() {
return getPublicKey();
}
public static final int D_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString d_;
/**
*
* Private exponent.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes d = 3;
*/
public com.google.protobuf.ByteString getD() {
return d_;
}
public static final int P_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString p_;
/**
*
* The following parameters are used to optimize RSA signature computation.
* The prime factor p of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes p = 4;
*/
public com.google.protobuf.ByteString getP() {
return p_;
}
public static final int Q_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString q_;
/**
*
* The prime factor q of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes q = 5;
*/
public com.google.protobuf.ByteString getQ() {
return q_;
}
public static final int DP_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString dp_;
/**
*
* d mod (p - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dp = 6;
*/
public com.google.protobuf.ByteString getDp() {
return dp_;
}
public static final int DQ_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString dq_;
/**
*
* d mod (q - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dq = 7;
*/
public com.google.protobuf.ByteString getDq() {
return dq_;
}
public static final int CRT_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString crt_;
/**
*
* Chinese Remainder Theorem coefficient q^(-1) mod p.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes crt = 8;
*/
public com.google.protobuf.ByteString getCrt() {
return crt_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (version_ != 0) {
output.writeUInt32(1, version_);
}
if (publicKey_ != null) {
output.writeMessage(2, getPublicKey());
}
if (!d_.isEmpty()) {
output.writeBytes(3, d_);
}
if (!p_.isEmpty()) {
output.writeBytes(4, p_);
}
if (!q_.isEmpty()) {
output.writeBytes(5, q_);
}
if (!dp_.isEmpty()) {
output.writeBytes(6, dp_);
}
if (!dq_.isEmpty()) {
output.writeBytes(7, dq_);
}
if (!crt_.isEmpty()) {
output.writeBytes(8, crt_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (version_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, version_);
}
if (publicKey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPublicKey());
}
if (!d_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, d_);
}
if (!p_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, p_);
}
if (!q_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, q_);
}
if (!dp_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, dp_);
}
if (!dq_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, dq_);
}
if (!crt_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, crt_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey)) {
return super.equals(obj);
}
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey other = (com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey) obj;
if (getVersion()
!= other.getVersion()) return false;
if (hasPublicKey() != other.hasPublicKey()) return false;
if (hasPublicKey()) {
if (!getPublicKey()
.equals(other.getPublicKey())) return false;
}
if (!getD()
.equals(other.getD())) return false;
if (!getP()
.equals(other.getP())) return false;
if (!getQ()
.equals(other.getQ())) return false;
if (!getDp()
.equals(other.getDp())) return false;
if (!getDq()
.equals(other.getDq())) return false;
if (!getCrt()
.equals(other.getCrt())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
if (hasPublicKey()) {
hash = (37 * hash) + PUBLIC_KEY_FIELD_NUMBER;
hash = (53 * hash) + getPublicKey().hashCode();
}
hash = (37 * hash) + D_FIELD_NUMBER;
hash = (53 * hash) + getD().hashCode();
hash = (37 * hash) + P_FIELD_NUMBER;
hash = (53 * hash) + getP().hashCode();
hash = (37 * hash) + Q_FIELD_NUMBER;
hash = (53 * hash) + getQ().hashCode();
hash = (37 * hash) + DP_FIELD_NUMBER;
hash = (53 * hash) + getDp().hashCode();
hash = (37 * hash) + DQ_FIELD_NUMBER;
hash = (53 * hash) + getDq().hashCode();
hash = (37 * hash) + CRT_FIELD_NUMBER;
hash = (53 * hash) + getCrt().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* key_type: type.googleapis.com/google.crypto.tink.RsaSsaPkcs1PrivateKey
*
*
* Protobuf type {@code google.crypto.tink.RsaSsaPkcs1PrivateKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.crypto.tink.RsaSsaPkcs1PrivateKey)
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.RsaSsaPkcs1.internal_static_google_crypto_tink_RsaSsaPkcs1PrivateKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.RsaSsaPkcs1.internal_static_google_crypto_tink_RsaSsaPkcs1PrivateKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey.class, com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey.Builder.class);
}
// Construct using com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
version_ = 0;
if (publicKeyBuilder_ == null) {
publicKey_ = null;
} else {
publicKey_ = null;
publicKeyBuilder_ = null;
}
d_ = com.google.protobuf.ByteString.EMPTY;
p_ = com.google.protobuf.ByteString.EMPTY;
q_ = com.google.protobuf.ByteString.EMPTY;
dp_ = com.google.protobuf.ByteString.EMPTY;
dq_ = com.google.protobuf.ByteString.EMPTY;
crt_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.crypto.tink.proto.RsaSsaPkcs1.internal_static_google_crypto_tink_RsaSsaPkcs1PrivateKey_descriptor;
}
@java.lang.Override
public com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey getDefaultInstanceForType() {
return com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey.getDefaultInstance();
}
@java.lang.Override
public com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey build() {
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey buildPartial() {
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey result = new com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey(this);
result.version_ = version_;
if (publicKeyBuilder_ == null) {
result.publicKey_ = publicKey_;
} else {
result.publicKey_ = publicKeyBuilder_.build();
}
result.d_ = d_;
result.p_ = p_;
result.q_ = q_;
result.dp_ = dp_;
result.dq_ = dq_;
result.crt_ = crt_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey) {
return mergeFrom((com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey other) {
if (other == com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey.getDefaultInstance()) return this;
if (other.getVersion() != 0) {
setVersion(other.getVersion());
}
if (other.hasPublicKey()) {
mergePublicKey(other.getPublicKey());
}
if (other.getD() != com.google.protobuf.ByteString.EMPTY) {
setD(other.getD());
}
if (other.getP() != com.google.protobuf.ByteString.EMPTY) {
setP(other.getP());
}
if (other.getQ() != com.google.protobuf.ByteString.EMPTY) {
setQ(other.getQ());
}
if (other.getDp() != com.google.protobuf.ByteString.EMPTY) {
setDp(other.getDp());
}
if (other.getDq() != com.google.protobuf.ByteString.EMPTY) {
setDq(other.getDq());
}
if (other.getCrt() != com.google.protobuf.ByteString.EMPTY) {
setCrt(other.getCrt());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int version_ ;
/**
*
* Required.
*
*
* uint32 version = 1;
*/
public int getVersion() {
return version_;
}
/**
*
* Required.
*
*
* uint32 version = 1;
*/
public Builder setVersion(int value) {
version_ = value;
onChanged();
return this;
}
/**
*
* Required.
*
*
* uint32 version = 1;
*/
public Builder clearVersion() {
version_ = 0;
onChanged();
return this;
}
private com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey publicKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey, com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.Builder, com.google.crypto.tink.proto.RsaSsaPkcs1PublicKeyOrBuilder> publicKeyBuilder_;
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public boolean hasPublicKey() {
return publicKeyBuilder_ != null || publicKey_ != null;
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey getPublicKey() {
if (publicKeyBuilder_ == null) {
return publicKey_ == null ? com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.getDefaultInstance() : publicKey_;
} else {
return publicKeyBuilder_.getMessage();
}
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public Builder setPublicKey(com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey value) {
if (publicKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
publicKey_ = value;
onChanged();
} else {
publicKeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public Builder setPublicKey(
com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.Builder builderForValue) {
if (publicKeyBuilder_ == null) {
publicKey_ = builderForValue.build();
onChanged();
} else {
publicKeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public Builder mergePublicKey(com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey value) {
if (publicKeyBuilder_ == null) {
if (publicKey_ != null) {
publicKey_ =
com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.newBuilder(publicKey_).mergeFrom(value).buildPartial();
} else {
publicKey_ = value;
}
onChanged();
} else {
publicKeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public Builder clearPublicKey() {
if (publicKeyBuilder_ == null) {
publicKey_ = null;
onChanged();
} else {
publicKey_ = null;
publicKeyBuilder_ = null;
}
return this;
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.Builder getPublicKeyBuilder() {
onChanged();
return getPublicKeyFieldBuilder().getBuilder();
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
public com.google.crypto.tink.proto.RsaSsaPkcs1PublicKeyOrBuilder getPublicKeyOrBuilder() {
if (publicKeyBuilder_ != null) {
return publicKeyBuilder_.getMessageOrBuilder();
} else {
return publicKey_ == null ?
com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.getDefaultInstance() : publicKey_;
}
}
/**
*
* Required.
*
*
* .google.crypto.tink.RsaSsaPkcs1PublicKey public_key = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey, com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.Builder, com.google.crypto.tink.proto.RsaSsaPkcs1PublicKeyOrBuilder>
getPublicKeyFieldBuilder() {
if (publicKeyBuilder_ == null) {
publicKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey, com.google.crypto.tink.proto.RsaSsaPkcs1PublicKey.Builder, com.google.crypto.tink.proto.RsaSsaPkcs1PublicKeyOrBuilder>(
getPublicKey(),
getParentForChildren(),
isClean());
publicKey_ = null;
}
return publicKeyBuilder_;
}
private com.google.protobuf.ByteString d_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Private exponent.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes d = 3;
*/
public com.google.protobuf.ByteString getD() {
return d_;
}
/**
*
* Private exponent.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes d = 3;
*/
public Builder setD(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
d_ = value;
onChanged();
return this;
}
/**
*
* Private exponent.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes d = 3;
*/
public Builder clearD() {
d_ = getDefaultInstance().getD();
onChanged();
return this;
}
private com.google.protobuf.ByteString p_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The following parameters are used to optimize RSA signature computation.
* The prime factor p of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes p = 4;
*/
public com.google.protobuf.ByteString getP() {
return p_;
}
/**
*
* The following parameters are used to optimize RSA signature computation.
* The prime factor p of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes p = 4;
*/
public Builder setP(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
p_ = value;
onChanged();
return this;
}
/**
*
* The following parameters are used to optimize RSA signature computation.
* The prime factor p of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes p = 4;
*/
public Builder clearP() {
p_ = getDefaultInstance().getP();
onChanged();
return this;
}
private com.google.protobuf.ByteString q_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The prime factor q of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes q = 5;
*/
public com.google.protobuf.ByteString getQ() {
return q_;
}
/**
*
* The prime factor q of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes q = 5;
*/
public Builder setQ(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
q_ = value;
onChanged();
return this;
}
/**
*
* The prime factor q of n.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes q = 5;
*/
public Builder clearQ() {
q_ = getDefaultInstance().getQ();
onChanged();
return this;
}
private com.google.protobuf.ByteString dp_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* d mod (p - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dp = 6;
*/
public com.google.protobuf.ByteString getDp() {
return dp_;
}
/**
*
* d mod (p - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dp = 6;
*/
public Builder setDp(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
dp_ = value;
onChanged();
return this;
}
/**
*
* d mod (p - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dp = 6;
*/
public Builder clearDp() {
dp_ = getDefaultInstance().getDp();
onChanged();
return this;
}
private com.google.protobuf.ByteString dq_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* d mod (q - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dq = 7;
*/
public com.google.protobuf.ByteString getDq() {
return dq_;
}
/**
*
* d mod (q - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dq = 7;
*/
public Builder setDq(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
dq_ = value;
onChanged();
return this;
}
/**
*
* d mod (q - 1).
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes dq = 7;
*/
public Builder clearDq() {
dq_ = getDefaultInstance().getDq();
onChanged();
return this;
}
private com.google.protobuf.ByteString crt_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Chinese Remainder Theorem coefficient q^(-1) mod p.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes crt = 8;
*/
public com.google.protobuf.ByteString getCrt() {
return crt_;
}
/**
*
* Chinese Remainder Theorem coefficient q^(-1) mod p.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes crt = 8;
*/
public Builder setCrt(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
crt_ = value;
onChanged();
return this;
}
/**
*
* Chinese Remainder Theorem coefficient q^(-1) mod p.
* Unsigned big integer in bigendian representation.
* Required.
*
*
* bytes crt = 8;
*/
public Builder clearCrt() {
crt_ = getDefaultInstance().getCrt();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.crypto.tink.RsaSsaPkcs1PrivateKey)
}
// @@protoc_insertion_point(class_scope:google.crypto.tink.RsaSsaPkcs1PrivateKey)
private static final com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey();
}
public static com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RsaSsaPkcs1PrivateKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RsaSsaPkcs1PrivateKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.crypto.tink.proto.RsaSsaPkcs1PrivateKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy