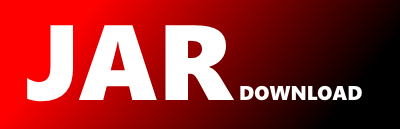
com.google.crypto.tink.proto.KeysetInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tink Show documentation
Show all versions of tink Show documentation
Tink is a small cryptographic library that provides a safe, simple, agile and fast way to accomplish some common cryptographic tasks.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: proto/tink.proto
package com.google.crypto.tink.proto;
/**
*
* Represents a "safe" Keyset that doesn't contain any actual key material,
* thus can be used for logging or monitoring. Most fields are copied from
* Keyset.
*
*
* Protobuf type {@code google.crypto.tink.KeysetInfo}
*/
public final class KeysetInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.crypto.tink.KeysetInfo)
KeysetInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeysetInfo.newBuilder() to construct.
private KeysetInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeysetInfo() {
keyInfo_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new KeysetInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KeysetInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
primaryKeyId_ = input.readUInt32();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
keyInfo_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
keyInfo_.add(
input.readMessage(com.google.crypto.tink.proto.KeysetInfo.KeyInfo.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
keyInfo_ = java.util.Collections.unmodifiableList(keyInfo_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.KeysetInfo.class, com.google.crypto.tink.proto.KeysetInfo.Builder.class);
}
public interface KeyInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.crypto.tink.KeysetInfo.KeyInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @return The typeUrl.
*/
java.lang.String getTypeUrl();
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @return The bytes for typeUrl.
*/
com.google.protobuf.ByteString
getTypeUrlBytes();
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @return The status.
*/
com.google.crypto.tink.proto.KeyStatusType getStatus();
/**
*
* See Keyset.Key.key_id.
*
*
* uint32 key_id = 3;
* @return The keyId.
*/
int getKeyId();
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The enum numeric value on the wire for outputPrefixType.
*/
int getOutputPrefixTypeValue();
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The outputPrefixType.
*/
com.google.crypto.tink.proto.OutputPrefixType getOutputPrefixType();
}
/**
* Protobuf type {@code google.crypto.tink.KeysetInfo.KeyInfo}
*/
public static final class KeyInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.crypto.tink.KeysetInfo.KeyInfo)
KeyInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeyInfo.newBuilder() to construct.
private KeyInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeyInfo() {
typeUrl_ = "";
status_ = 0;
outputPrefixType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new KeyInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KeyInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
typeUrl_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 24: {
keyId_ = input.readUInt32();
break;
}
case 32: {
int rawValue = input.readEnum();
outputPrefixType_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_KeyInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_KeyInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.KeysetInfo.KeyInfo.class, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder.class);
}
public static final int TYPE_URL_FIELD_NUMBER = 1;
private volatile java.lang.Object typeUrl_;
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @return The typeUrl.
*/
public java.lang.String getTypeUrl() {
java.lang.Object ref = typeUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
typeUrl_ = s;
return s;
}
}
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @return The bytes for typeUrl.
*/
public com.google.protobuf.ByteString
getTypeUrlBytes() {
java.lang.Object ref = typeUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
typeUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 2;
private int status_;
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @return The enum numeric value on the wire for status.
*/
public int getStatusValue() {
return status_;
}
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @return The status.
*/
public com.google.crypto.tink.proto.KeyStatusType getStatus() {
@SuppressWarnings("deprecation")
com.google.crypto.tink.proto.KeyStatusType result = com.google.crypto.tink.proto.KeyStatusType.valueOf(status_);
return result == null ? com.google.crypto.tink.proto.KeyStatusType.UNRECOGNIZED : result;
}
public static final int KEY_ID_FIELD_NUMBER = 3;
private int keyId_;
/**
*
* See Keyset.Key.key_id.
*
*
* uint32 key_id = 3;
* @return The keyId.
*/
public int getKeyId() {
return keyId_;
}
public static final int OUTPUT_PREFIX_TYPE_FIELD_NUMBER = 4;
private int outputPrefixType_;
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The enum numeric value on the wire for outputPrefixType.
*/
public int getOutputPrefixTypeValue() {
return outputPrefixType_;
}
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The outputPrefixType.
*/
public com.google.crypto.tink.proto.OutputPrefixType getOutputPrefixType() {
@SuppressWarnings("deprecation")
com.google.crypto.tink.proto.OutputPrefixType result = com.google.crypto.tink.proto.OutputPrefixType.valueOf(outputPrefixType_);
return result == null ? com.google.crypto.tink.proto.OutputPrefixType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTypeUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, typeUrl_);
}
if (status_ != com.google.crypto.tink.proto.KeyStatusType.UNKNOWN_STATUS.getNumber()) {
output.writeEnum(2, status_);
}
if (keyId_ != 0) {
output.writeUInt32(3, keyId_);
}
if (outputPrefixType_ != com.google.crypto.tink.proto.OutputPrefixType.UNKNOWN_PREFIX.getNumber()) {
output.writeEnum(4, outputPrefixType_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTypeUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, typeUrl_);
}
if (status_ != com.google.crypto.tink.proto.KeyStatusType.UNKNOWN_STATUS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_);
}
if (keyId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, keyId_);
}
if (outputPrefixType_ != com.google.crypto.tink.proto.OutputPrefixType.UNKNOWN_PREFIX.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, outputPrefixType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.crypto.tink.proto.KeysetInfo.KeyInfo)) {
return super.equals(obj);
}
com.google.crypto.tink.proto.KeysetInfo.KeyInfo other = (com.google.crypto.tink.proto.KeysetInfo.KeyInfo) obj;
if (!getTypeUrl()
.equals(other.getTypeUrl())) return false;
if (status_ != other.status_) return false;
if (getKeyId()
!= other.getKeyId()) return false;
if (outputPrefixType_ != other.outputPrefixType_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_URL_FIELD_NUMBER;
hash = (53 * hash) + getTypeUrl().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + getKeyId();
hash = (37 * hash) + OUTPUT_PREFIX_TYPE_FIELD_NUMBER;
hash = (53 * hash) + outputPrefixType_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.crypto.tink.proto.KeysetInfo.KeyInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code google.crypto.tink.KeysetInfo.KeyInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.crypto.tink.KeysetInfo.KeyInfo)
com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_KeyInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_KeyInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.KeysetInfo.KeyInfo.class, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder.class);
}
// Construct using com.google.crypto.tink.proto.KeysetInfo.KeyInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
typeUrl_ = "";
status_ = 0;
keyId_ = 0;
outputPrefixType_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_KeyInfo_descriptor;
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo getDefaultInstanceForType() {
return com.google.crypto.tink.proto.KeysetInfo.KeyInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo build() {
com.google.crypto.tink.proto.KeysetInfo.KeyInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo buildPartial() {
com.google.crypto.tink.proto.KeysetInfo.KeyInfo result = new com.google.crypto.tink.proto.KeysetInfo.KeyInfo(this);
result.typeUrl_ = typeUrl_;
result.status_ = status_;
result.keyId_ = keyId_;
result.outputPrefixType_ = outputPrefixType_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.crypto.tink.proto.KeysetInfo.KeyInfo) {
return mergeFrom((com.google.crypto.tink.proto.KeysetInfo.KeyInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.crypto.tink.proto.KeysetInfo.KeyInfo other) {
if (other == com.google.crypto.tink.proto.KeysetInfo.KeyInfo.getDefaultInstance()) return this;
if (!other.getTypeUrl().isEmpty()) {
typeUrl_ = other.typeUrl_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.getKeyId() != 0) {
setKeyId(other.getKeyId());
}
if (other.outputPrefixType_ != 0) {
setOutputPrefixTypeValue(other.getOutputPrefixTypeValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.crypto.tink.proto.KeysetInfo.KeyInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.crypto.tink.proto.KeysetInfo.KeyInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object typeUrl_ = "";
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @return The typeUrl.
*/
public java.lang.String getTypeUrl() {
java.lang.Object ref = typeUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
typeUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @return The bytes for typeUrl.
*/
public com.google.protobuf.ByteString
getTypeUrlBytes() {
java.lang.Object ref = typeUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
typeUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @param value The typeUrl to set.
* @return This builder for chaining.
*/
public Builder setTypeUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
typeUrl_ = value;
onChanged();
return this;
}
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @return This builder for chaining.
*/
public Builder clearTypeUrl() {
typeUrl_ = getDefaultInstance().getTypeUrl();
onChanged();
return this;
}
/**
*
* the type url of this key,
* e.g., type.googleapis.com/google.crypto.tink.HmacKey.
*
*
* string type_url = 1;
* @param value The bytes for typeUrl to set.
* @return This builder for chaining.
*/
public Builder setTypeUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
typeUrl_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @return The enum numeric value on the wire for status.
*/
public int getStatusValue() {
return status_;
}
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @return The status.
*/
public com.google.crypto.tink.proto.KeyStatusType getStatus() {
@SuppressWarnings("deprecation")
com.google.crypto.tink.proto.KeyStatusType result = com.google.crypto.tink.proto.KeyStatusType.valueOf(status_);
return result == null ? com.google.crypto.tink.proto.KeyStatusType.UNRECOGNIZED : result;
}
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.crypto.tink.proto.KeyStatusType value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* See Keyset.Key.status.
*
*
* .google.crypto.tink.KeyStatusType status = 2;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private int keyId_ ;
/**
*
* See Keyset.Key.key_id.
*
*
* uint32 key_id = 3;
* @return The keyId.
*/
public int getKeyId() {
return keyId_;
}
/**
*
* See Keyset.Key.key_id.
*
*
* uint32 key_id = 3;
* @param value The keyId to set.
* @return This builder for chaining.
*/
public Builder setKeyId(int value) {
keyId_ = value;
onChanged();
return this;
}
/**
*
* See Keyset.Key.key_id.
*
*
* uint32 key_id = 3;
* @return This builder for chaining.
*/
public Builder clearKeyId() {
keyId_ = 0;
onChanged();
return this;
}
private int outputPrefixType_ = 0;
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The enum numeric value on the wire for outputPrefixType.
*/
public int getOutputPrefixTypeValue() {
return outputPrefixType_;
}
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @param value The enum numeric value on the wire for outputPrefixType to set.
* @return This builder for chaining.
*/
public Builder setOutputPrefixTypeValue(int value) {
outputPrefixType_ = value;
onChanged();
return this;
}
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return The outputPrefixType.
*/
public com.google.crypto.tink.proto.OutputPrefixType getOutputPrefixType() {
@SuppressWarnings("deprecation")
com.google.crypto.tink.proto.OutputPrefixType result = com.google.crypto.tink.proto.OutputPrefixType.valueOf(outputPrefixType_);
return result == null ? com.google.crypto.tink.proto.OutputPrefixType.UNRECOGNIZED : result;
}
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @param value The outputPrefixType to set.
* @return This builder for chaining.
*/
public Builder setOutputPrefixType(com.google.crypto.tink.proto.OutputPrefixType value) {
if (value == null) {
throw new NullPointerException();
}
outputPrefixType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* See Keyset.Key.output_prefix_type.
*
*
* .google.crypto.tink.OutputPrefixType output_prefix_type = 4;
* @return This builder for chaining.
*/
public Builder clearOutputPrefixType() {
outputPrefixType_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.crypto.tink.KeysetInfo.KeyInfo)
}
// @@protoc_insertion_point(class_scope:google.crypto.tink.KeysetInfo.KeyInfo)
private static final com.google.crypto.tink.proto.KeysetInfo.KeyInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.crypto.tink.proto.KeysetInfo.KeyInfo();
}
public static com.google.crypto.tink.proto.KeysetInfo.KeyInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KeyInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KeyInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int PRIMARY_KEY_ID_FIELD_NUMBER = 1;
private int primaryKeyId_;
/**
*
* See Keyset.primary_key_id.
*
*
* uint32 primary_key_id = 1;
* @return The primaryKeyId.
*/
public int getPrimaryKeyId() {
return primaryKeyId_;
}
public static final int KEY_INFO_FIELD_NUMBER = 2;
private java.util.List keyInfo_;
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public java.util.List getKeyInfoList() {
return keyInfo_;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public java.util.List extends com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder>
getKeyInfoOrBuilderList() {
return keyInfo_;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public int getKeyInfoCount() {
return keyInfo_.size();
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo getKeyInfo(int index) {
return keyInfo_.get(index);
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder getKeyInfoOrBuilder(
int index) {
return keyInfo_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (primaryKeyId_ != 0) {
output.writeUInt32(1, primaryKeyId_);
}
for (int i = 0; i < keyInfo_.size(); i++) {
output.writeMessage(2, keyInfo_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (primaryKeyId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, primaryKeyId_);
}
for (int i = 0; i < keyInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, keyInfo_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.crypto.tink.proto.KeysetInfo)) {
return super.equals(obj);
}
com.google.crypto.tink.proto.KeysetInfo other = (com.google.crypto.tink.proto.KeysetInfo) obj;
if (getPrimaryKeyId()
!= other.getPrimaryKeyId()) return false;
if (!getKeyInfoList()
.equals(other.getKeyInfoList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PRIMARY_KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + getPrimaryKeyId();
if (getKeyInfoCount() > 0) {
hash = (37 * hash) + KEY_INFO_FIELD_NUMBER;
hash = (53 * hash) + getKeyInfoList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.KeysetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.KeysetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.crypto.tink.proto.KeysetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents a "safe" Keyset that doesn't contain any actual key material,
* thus can be used for logging or monitoring. Most fields are copied from
* Keyset.
*
*
* Protobuf type {@code google.crypto.tink.KeysetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.crypto.tink.KeysetInfo)
com.google.crypto.tink.proto.KeysetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.KeysetInfo.class, com.google.crypto.tink.proto.KeysetInfo.Builder.class);
}
// Construct using com.google.crypto.tink.proto.KeysetInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getKeyInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
primaryKeyId_ = 0;
if (keyInfoBuilder_ == null) {
keyInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
keyInfoBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.crypto.tink.proto.Tink.internal_static_google_crypto_tink_KeysetInfo_descriptor;
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo getDefaultInstanceForType() {
return com.google.crypto.tink.proto.KeysetInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo build() {
com.google.crypto.tink.proto.KeysetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo buildPartial() {
com.google.crypto.tink.proto.KeysetInfo result = new com.google.crypto.tink.proto.KeysetInfo(this);
int from_bitField0_ = bitField0_;
result.primaryKeyId_ = primaryKeyId_;
if (keyInfoBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
keyInfo_ = java.util.Collections.unmodifiableList(keyInfo_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.keyInfo_ = keyInfo_;
} else {
result.keyInfo_ = keyInfoBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.crypto.tink.proto.KeysetInfo) {
return mergeFrom((com.google.crypto.tink.proto.KeysetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.crypto.tink.proto.KeysetInfo other) {
if (other == com.google.crypto.tink.proto.KeysetInfo.getDefaultInstance()) return this;
if (other.getPrimaryKeyId() != 0) {
setPrimaryKeyId(other.getPrimaryKeyId());
}
if (keyInfoBuilder_ == null) {
if (!other.keyInfo_.isEmpty()) {
if (keyInfo_.isEmpty()) {
keyInfo_ = other.keyInfo_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeyInfoIsMutable();
keyInfo_.addAll(other.keyInfo_);
}
onChanged();
}
} else {
if (!other.keyInfo_.isEmpty()) {
if (keyInfoBuilder_.isEmpty()) {
keyInfoBuilder_.dispose();
keyInfoBuilder_ = null;
keyInfo_ = other.keyInfo_;
bitField0_ = (bitField0_ & ~0x00000001);
keyInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getKeyInfoFieldBuilder() : null;
} else {
keyInfoBuilder_.addAllMessages(other.keyInfo_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.crypto.tink.proto.KeysetInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.crypto.tink.proto.KeysetInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int primaryKeyId_ ;
/**
*
* See Keyset.primary_key_id.
*
*
* uint32 primary_key_id = 1;
* @return The primaryKeyId.
*/
public int getPrimaryKeyId() {
return primaryKeyId_;
}
/**
*
* See Keyset.primary_key_id.
*
*
* uint32 primary_key_id = 1;
* @param value The primaryKeyId to set.
* @return This builder for chaining.
*/
public Builder setPrimaryKeyId(int value) {
primaryKeyId_ = value;
onChanged();
return this;
}
/**
*
* See Keyset.primary_key_id.
*
*
* uint32 primary_key_id = 1;
* @return This builder for chaining.
*/
public Builder clearPrimaryKeyId() {
primaryKeyId_ = 0;
onChanged();
return this;
}
private java.util.List keyInfo_ =
java.util.Collections.emptyList();
private void ensureKeyInfoIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
keyInfo_ = new java.util.ArrayList(keyInfo_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.crypto.tink.proto.KeysetInfo.KeyInfo, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder, com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder> keyInfoBuilder_;
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public java.util.List getKeyInfoList() {
if (keyInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(keyInfo_);
} else {
return keyInfoBuilder_.getMessageList();
}
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public int getKeyInfoCount() {
if (keyInfoBuilder_ == null) {
return keyInfo_.size();
} else {
return keyInfoBuilder_.getCount();
}
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo getKeyInfo(int index) {
if (keyInfoBuilder_ == null) {
return keyInfo_.get(index);
} else {
return keyInfoBuilder_.getMessage(index);
}
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder setKeyInfo(
int index, com.google.crypto.tink.proto.KeysetInfo.KeyInfo value) {
if (keyInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyInfoIsMutable();
keyInfo_.set(index, value);
onChanged();
} else {
keyInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder setKeyInfo(
int index, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder builderForValue) {
if (keyInfoBuilder_ == null) {
ensureKeyInfoIsMutable();
keyInfo_.set(index, builderForValue.build());
onChanged();
} else {
keyInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder addKeyInfo(com.google.crypto.tink.proto.KeysetInfo.KeyInfo value) {
if (keyInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyInfoIsMutable();
keyInfo_.add(value);
onChanged();
} else {
keyInfoBuilder_.addMessage(value);
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder addKeyInfo(
int index, com.google.crypto.tink.proto.KeysetInfo.KeyInfo value) {
if (keyInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyInfoIsMutable();
keyInfo_.add(index, value);
onChanged();
} else {
keyInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder addKeyInfo(
com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder builderForValue) {
if (keyInfoBuilder_ == null) {
ensureKeyInfoIsMutable();
keyInfo_.add(builderForValue.build());
onChanged();
} else {
keyInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder addKeyInfo(
int index, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder builderForValue) {
if (keyInfoBuilder_ == null) {
ensureKeyInfoIsMutable();
keyInfo_.add(index, builderForValue.build());
onChanged();
} else {
keyInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder addAllKeyInfo(
java.lang.Iterable extends com.google.crypto.tink.proto.KeysetInfo.KeyInfo> values) {
if (keyInfoBuilder_ == null) {
ensureKeyInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keyInfo_);
onChanged();
} else {
keyInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder clearKeyInfo() {
if (keyInfoBuilder_ == null) {
keyInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
keyInfoBuilder_.clear();
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public Builder removeKeyInfo(int index) {
if (keyInfoBuilder_ == null) {
ensureKeyInfoIsMutable();
keyInfo_.remove(index);
onChanged();
} else {
keyInfoBuilder_.remove(index);
}
return this;
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder getKeyInfoBuilder(
int index) {
return getKeyInfoFieldBuilder().getBuilder(index);
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder getKeyInfoOrBuilder(
int index) {
if (keyInfoBuilder_ == null) {
return keyInfo_.get(index); } else {
return keyInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public java.util.List extends com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder>
getKeyInfoOrBuilderList() {
if (keyInfoBuilder_ != null) {
return keyInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(keyInfo_);
}
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder addKeyInfoBuilder() {
return getKeyInfoFieldBuilder().addBuilder(
com.google.crypto.tink.proto.KeysetInfo.KeyInfo.getDefaultInstance());
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder addKeyInfoBuilder(
int index) {
return getKeyInfoFieldBuilder().addBuilder(
index, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.getDefaultInstance());
}
/**
*
* KeyInfos in the KeysetInfo.
* Each KeyInfo is corresponding to a Key in the corresponding Keyset.
*
*
* repeated .google.crypto.tink.KeysetInfo.KeyInfo key_info = 2;
*/
public java.util.List
getKeyInfoBuilderList() {
return getKeyInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.crypto.tink.proto.KeysetInfo.KeyInfo, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder, com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder>
getKeyInfoFieldBuilder() {
if (keyInfoBuilder_ == null) {
keyInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.crypto.tink.proto.KeysetInfo.KeyInfo, com.google.crypto.tink.proto.KeysetInfo.KeyInfo.Builder, com.google.crypto.tink.proto.KeysetInfo.KeyInfoOrBuilder>(
keyInfo_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
keyInfo_ = null;
}
return keyInfoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.crypto.tink.KeysetInfo)
}
// @@protoc_insertion_point(class_scope:google.crypto.tink.KeysetInfo)
private static final com.google.crypto.tink.proto.KeysetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.crypto.tink.proto.KeysetInfo();
}
public static com.google.crypto.tink.proto.KeysetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KeysetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KeysetInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.crypto.tink.proto.KeysetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy