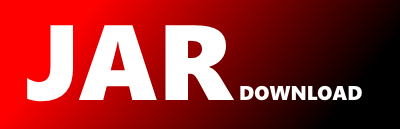
elemental2.media.BaseAudioContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elemental2-media Show documentation
Show all versions of elemental2-media Show documentation
Thin Java abstractions for the native media APIs provided by the browser.
The newest version!
/*
* Copyright 2018 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package elemental2.media;
import elemental2.core.ArrayBuffer;
import elemental2.core.Float32Array;
import elemental2.dom.Event;
import elemental2.dom.EventListener;
import elemental2.dom.EventTarget;
import elemental2.promise.Promise;
import jsinterop.annotations.JsFunction;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsPackage;
import jsinterop.annotations.JsType;
import jsinterop.base.Js;
import jsinterop.base.JsArrayLike;
@JsType(isNative = true, namespace = JsPackage.GLOBAL)
public class BaseAudioContext implements EventTarget {
@JsFunction
public interface DecodeAudioDataErrorCallbackFn {
Object onInvoke(Object p0);
}
@JsFunction
public interface DecodeAudioDataSuccessCallbackFn {
Object onInvoke(AudioBuffer p0);
}
@JsFunction
public interface OnstatechangeFn {
Object onInvoke(Event p0);
}
public AudioWorklet audioWorklet;
public double currentTime;
public AudioDestinationNode destination;
public AudioListener listener;
public BaseAudioContext.OnstatechangeFn onstatechange;
public double sampleRate;
public String state;
public native void addEventListener(
String type, EventListener listener, EventTarget.AddEventListenerOptionsUnionType options);
public native void addEventListener(String type, EventListener listener);
public native Promise close();
public native AnalyserNode createAnalyser();
public native Promise createAudioWorker(String scriptURL);
public native BiquadFilterNode createBiquadFilter();
public native AudioBuffer createBuffer(int numberOfChannels, int length, double sampleRate);
public native AudioBufferSourceNode createBufferSource();
public native ChannelMergerNode createChannelMerger();
public native ChannelMergerNode createChannelMerger(int numberOfInputs);
public native ChannelSplitterNode createChannelSplitter();
public native ChannelSplitterNode createChannelSplitter(int numberOfOutputs);
public native ConstantSourceNode createConstantSource();
public native ConvolverNode createConvolver();
public native DelayNode createDelay();
public native DelayNode createDelay(double maxDelayTime);
public native DynamicsCompressorNode createDynamicsCompressor();
public native GainNode createGain();
public native IIRFilterNode createIIRFilter(
JsArrayLike feedforward, JsArrayLike feedback);
@JsOverlay
public final IIRFilterNode createIIRFilter(double[] feedforward, double[] feedback) {
return createIIRFilter(
Js.>uncheckedCast(feedforward),
Js.>uncheckedCast(feedback));
}
public native OscillatorNode createOscillator();
public native PannerNode createPanner();
public native PeriodicWave createPeriodicWave(Float32Array real, Float32Array imag);
@Deprecated
public native ScriptProcessorNode createScriptProcessor();
@Deprecated
public native ScriptProcessorNode createScriptProcessor(
int bufferSize, int numberOfInputChannels_opt, int numberOfOutputChannels_opt);
@Deprecated
public native ScriptProcessorNode createScriptProcessor(
int bufferSize, int numberOfInputChannels_opt);
@Deprecated
public native ScriptProcessorNode createScriptProcessor(int bufferSize);
public native SpatialPannerNode createSpatialPanner();
public native StereoPannerNode createStereoPanner();
public native WaveShaperNode createWaveShaper();
public native Promise decodeAudioData(
ArrayBuffer audioData,
BaseAudioContext.DecodeAudioDataSuccessCallbackFn successCallback,
BaseAudioContext.DecodeAudioDataErrorCallbackFn errorCallback);
public native Promise decodeAudioData(
ArrayBuffer audioData, BaseAudioContext.DecodeAudioDataSuccessCallbackFn successCallback);
public native Promise decodeAudioData(ArrayBuffer audioData);
public native boolean dispatchEvent(Event evt);
public native void removeEventListener(
String type, EventListener listener, EventTarget.RemoveEventListenerOptionsUnionType options);
public native void removeEventListener(String type, EventListener listener);
public native Promise resume();
public native Promise suspend();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy