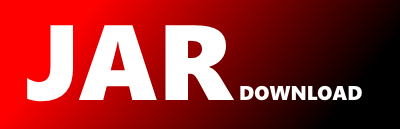
elemental2.webgl.WebGLRenderingContext__Constants Maven / Gradle / Ivy
/*
* Copyright 2018 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package elemental2.webgl;
import jsinterop.annotations.JsPackage;
import jsinterop.annotations.JsType;
@JsType(isNative = true, name = "WebGLRenderingContext", namespace = JsPackage.GLOBAL)
class WebGLRenderingContext__Constants {
static int ACTIVE_ATTRIBUTES;
static int ACTIVE_TEXTURE;
static int ACTIVE_UNIFORMS;
static int ALIASED_LINE_WIDTH_RANGE;
static int ALIASED_POINT_SIZE_RANGE;
static int ALPHA;
static int ALPHA_BITS;
static int ALWAYS;
static int ARRAY_BUFFER;
static int ARRAY_BUFFER_BINDING;
static int ATTACHED_SHADERS;
static int BACK;
static int BLEND;
static int BLEND_COLOR;
static int BLEND_DST_ALPHA;
static int BLEND_DST_RGB;
static int BLEND_EQUATION;
static int BLEND_EQUATION_ALPHA;
static int BLEND_EQUATION_RGB;
static int BLEND_SRC_ALPHA;
static int BLEND_SRC_RGB;
static int BLUE_BITS;
static int BOOL;
static int BOOL_VEC2;
static int BOOL_VEC3;
static int BOOL_VEC4;
static int BROWSER_DEFAULT_WEBGL;
static int BUFFER_SIZE;
static int BUFFER_USAGE;
static int BYTE;
static int CCW;
static int CLAMP_TO_EDGE;
static int COLOR_ATTACHMENT0;
static int COLOR_BUFFER_BIT;
static int COLOR_CLEAR_VALUE;
static int COLOR_WRITEMASK;
static int COMPILE_STATUS;
static int COMPRESSED_TEXTURE_FORMATS;
static int CONSTANT_ALPHA;
static int CONSTANT_COLOR;
static int CONTEXT_LOST_WEBGL;
static int CULL_FACE;
static int CULL_FACE_MODE;
static int CURRENT_PROGRAM;
static int CURRENT_VERTEX_ATTRIB;
static int CW;
static int DECR;
static int DECR_WRAP;
static int DELETE_STATUS;
static int DEPTH_ATTACHMENT;
static int DEPTH_BITS;
static int DEPTH_BUFFER_BIT;
static int DEPTH_CLEAR_VALUE;
static int DEPTH_COMPONENT;
static int DEPTH_COMPONENT16;
static int DEPTH_FUNC;
static int DEPTH_RANGE;
static int DEPTH_STENCIL;
static int DEPTH_STENCIL_ATTACHMENT;
static int DEPTH_TEST;
static int DEPTH_WRITEMASK;
static int DITHER;
static int DONT_CARE;
static int DST_ALPHA;
static int DST_COLOR;
static int DYNAMIC_DRAW;
static int ELEMENT_ARRAY_BUFFER;
static int ELEMENT_ARRAY_BUFFER_BINDING;
static int EQUAL;
static int FASTEST;
static int FLOAT;
static int FLOAT_MAT2;
static int FLOAT_MAT3;
static int FLOAT_MAT4;
static int FLOAT_VEC2;
static int FLOAT_VEC3;
static int FLOAT_VEC4;
static int FRAGMENT_SHADER;
static int FRAMEBUFFER;
static int FRAMEBUFFER_ATTACHMENT_OBJECT_NAME;
static int FRAMEBUFFER_ATTACHMENT_OBJECT_TYPE;
static int FRAMEBUFFER_ATTACHMENT_TEXTURE_CUBE_MAP_FACE;
static int FRAMEBUFFER_ATTACHMENT_TEXTURE_LEVEL;
static int FRAMEBUFFER_BINDING;
static int FRAMEBUFFER_COMPLETE;
static int FRAMEBUFFER_INCOMPLETE_ATTACHMENT;
static int FRAMEBUFFER_INCOMPLETE_DIMENSIONS;
static int FRAMEBUFFER_INCOMPLETE_MISSING_ATTACHMENT;
static int FRAMEBUFFER_UNSUPPORTED;
static int FRONT;
static int FRONT_AND_BACK;
static int FRONT_FACE;
static int FUNC_ADD;
static int FUNC_REVERSE_SUBTRACT;
static int FUNC_SUBTRACT;
static int GENERATE_MIPMAP_HINT;
static int GEQUAL;
static int GREATER;
static int GREEN_BITS;
static int HIGH_FLOAT;
static int HIGH_INT;
static int IMPLEMENTATION_COLOR_READ_FORMAT;
static int IMPLEMENTATION_COLOR_READ_TYPE;
static int INCR;
static int INCR_WRAP;
static int INT;
static int INT_VEC2;
static int INT_VEC3;
static int INT_VEC4;
static int INVALID_ENUM;
static int INVALID_FRAMEBUFFER_OPERATION;
static int INVALID_OPERATION;
static int INVALID_VALUE;
static int INVERT;
static int KEEP;
static int LEQUAL;
static int LESS;
static int LINEAR;
static int LINEAR_MIPMAP_LINEAR;
static int LINEAR_MIPMAP_NEAREST;
static int LINES;
static int LINE_LOOP;
static int LINE_STRIP;
static int LINE_WIDTH;
static int LINK_STATUS;
static int LOW_FLOAT;
static int LOW_INT;
static int LUMINANCE;
static int LUMINANCE_ALPHA;
static int MAX_COMBINED_TEXTURE_IMAGE_UNITS;
static int MAX_CUBE_MAP_TEXTURE_SIZE;
static int MAX_FRAGMENT_UNIFORM_VECTORS;
static int MAX_RENDERBUFFER_SIZE;
static int MAX_TEXTURE_IMAGE_UNITS;
static int MAX_TEXTURE_SIZE;
static int MAX_VARYING_VECTORS;
static int MAX_VERTEX_ATTRIBS;
static int MAX_VERTEX_TEXTURE_IMAGE_UNITS;
static int MAX_VERTEX_UNIFORM_VECTORS;
static int MAX_VIEWPORT_DIMS;
static int MEDIUM_FLOAT;
static int MEDIUM_INT;
static int MIRRORED_REPEAT;
static int NEAREST;
static int NEAREST_MIPMAP_LINEAR;
static int NEAREST_MIPMAP_NEAREST;
static int NEVER;
static int NICEST;
static int NONE;
static int NOTEQUAL;
static int NO_ERROR;
static int ONE;
static int ONE_MINUS_CONSTANT_ALPHA;
static int ONE_MINUS_CONSTANT_COLOR;
static int ONE_MINUS_DST_ALPHA;
static int ONE_MINUS_DST_COLOR;
static int ONE_MINUS_SRC_ALPHA;
static int ONE_MINUS_SRC_COLOR;
static int OUT_OF_MEMORY;
static int PACK_ALIGNMENT;
static int POINTS;
static int POLYGON_OFFSET_FACTOR;
static int POLYGON_OFFSET_FILL;
static int POLYGON_OFFSET_UNITS;
static int RED_BITS;
static int RENDERBUFFER;
static int RENDERBUFFER_ALPHA_SIZE;
static int RENDERBUFFER_BINDING;
static int RENDERBUFFER_BLUE_SIZE;
static int RENDERBUFFER_DEPTH_SIZE;
static int RENDERBUFFER_GREEN_SIZE;
static int RENDERBUFFER_HEIGHT;
static int RENDERBUFFER_INTERNAL_FORMAT;
static int RENDERBUFFER_RED_SIZE;
static int RENDERBUFFER_STENCIL_SIZE;
static int RENDERBUFFER_WIDTH;
static int RENDERER;
static int REPEAT;
static int REPLACE;
static int RGB;
static int RGB565;
static int RGB5_A1;
static int RGBA;
static int RGBA4;
static int SAMPLER_2D;
static int SAMPLER_CUBE;
static int SAMPLES;
static int SAMPLE_ALPHA_TO_COVERAGE;
static int SAMPLE_BUFFERS;
static int SAMPLE_COVERAGE;
static int SAMPLE_COVERAGE_INVERT;
static int SAMPLE_COVERAGE_VALUE;
static int SCISSOR_BOX;
static int SCISSOR_TEST;
static int SHADER_TYPE;
static int SHADING_LANGUAGE_VERSION;
static int SHORT;
static int SRC_ALPHA;
static int SRC_ALPHA_SATURATE;
static int SRC_COLOR;
static int STATIC_DRAW;
static int STENCIL_ATTACHMENT;
static int STENCIL_BACK_FAIL;
static int STENCIL_BACK_FUNC;
static int STENCIL_BACK_PASS_DEPTH_FAIL;
static int STENCIL_BACK_PASS_DEPTH_PASS;
static int STENCIL_BACK_REF;
static int STENCIL_BACK_VALUE_MASK;
static int STENCIL_BACK_WRITEMASK;
static int STENCIL_BITS;
static int STENCIL_BUFFER_BIT;
static int STENCIL_CLEAR_VALUE;
static int STENCIL_FAIL;
static int STENCIL_FUNC;
static double STENCIL_INDEX;
static int STENCIL_INDEX8;
static int STENCIL_PASS_DEPTH_FAIL;
static int STENCIL_PASS_DEPTH_PASS;
static int STENCIL_REF;
static int STENCIL_TEST;
static int STENCIL_VALUE_MASK;
static int STENCIL_WRITEMASK;
static int STREAM_DRAW;
static int SUBPIXEL_BITS;
static int TEXTURE;
static int TEXTURE0;
static int TEXTURE1;
static int TEXTURE10;
static int TEXTURE11;
static int TEXTURE12;
static int TEXTURE13;
static int TEXTURE14;
static int TEXTURE15;
static int TEXTURE16;
static int TEXTURE17;
static int TEXTURE18;
static int TEXTURE19;
static int TEXTURE2;
static int TEXTURE20;
static int TEXTURE21;
static int TEXTURE22;
static int TEXTURE23;
static int TEXTURE24;
static int TEXTURE25;
static int TEXTURE26;
static int TEXTURE27;
static int TEXTURE28;
static int TEXTURE29;
static int TEXTURE3;
static int TEXTURE30;
static int TEXTURE31;
static int TEXTURE4;
static int TEXTURE5;
static int TEXTURE6;
static int TEXTURE7;
static int TEXTURE8;
static int TEXTURE9;
static int TEXTURE_2D;
static int TEXTURE_BINDING_2D;
static int TEXTURE_BINDING_CUBE_MAP;
static int TEXTURE_CUBE_MAP;
static int TEXTURE_CUBE_MAP_NEGATIVE_X;
static int TEXTURE_CUBE_MAP_NEGATIVE_Y;
static int TEXTURE_CUBE_MAP_NEGATIVE_Z;
static int TEXTURE_CUBE_MAP_POSITIVE_X;
static int TEXTURE_CUBE_MAP_POSITIVE_Y;
static int TEXTURE_CUBE_MAP_POSITIVE_Z;
static int TEXTURE_MAG_FILTER;
static int TEXTURE_MIN_FILTER;
static int TEXTURE_WRAP_S;
static int TEXTURE_WRAP_T;
static int TRIANGLES;
static int TRIANGLE_FAN;
static int TRIANGLE_STRIP;
static int UNPACK_ALIGNMENT;
static int UNPACK_COLORSPACE_CONVERSION_WEBGL;
static int UNPACK_FLIP_Y_WEBGL;
static int UNPACK_PREMULTIPLY_ALPHA_WEBGL;
static int UNSIGNED_BYTE;
static int UNSIGNED_INT;
static int UNSIGNED_SHORT;
static int UNSIGNED_SHORT_4_4_4_4;
static int UNSIGNED_SHORT_5_5_5_1;
static int UNSIGNED_SHORT_5_6_5;
static int VALIDATE_STATUS;
static int VENDOR;
static int VERSION;
static int VERTEX_ATTRIB_ARRAY_BUFFER_BINDING;
static int VERTEX_ATTRIB_ARRAY_ENABLED;
static int VERTEX_ATTRIB_ARRAY_NORMALIZED;
static int VERTEX_ATTRIB_ARRAY_POINTER;
static int VERTEX_ATTRIB_ARRAY_SIZE;
static int VERTEX_ATTRIB_ARRAY_STRIDE;
static int VERTEX_ATTRIB_ARRAY_TYPE;
static int VERTEX_SHADER;
static int VIEWPORT;
static int ZERO;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy