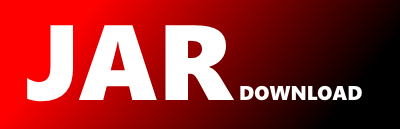
com.google.errorprone.BugPatternIndexWriter Maven / Gradle / Ivy
/*
* Copyright 2014 Google Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.errorprone;
import com.github.mustachejava.DefaultMustacheFactory;
import com.github.mustachejava.Mustache;
import com.github.mustachejava.MustacheFactory;
import com.google.auto.value.AutoValue;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Multimaps;
import com.google.common.collect.SortedSetMultimap;
import com.google.common.collect.TreeMultimap;
import com.google.errorprone.BugPattern.SeverityLevel;
import com.google.errorprone.DocGenTool.Target;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import org.yaml.snakeyaml.DumperOptions;
import org.yaml.snakeyaml.Yaml;
/** @author [email protected] (Alex Eagle) */
public class BugPatternIndexWriter {
@AutoValue
abstract static class IndexEntry {
abstract boolean onByDefault();
abstract SeverityLevel severity();
static IndexEntry create(boolean onByDefault, SeverityLevel severity) {
return new AutoValue_BugPatternIndexWriter_IndexEntry(onByDefault, severity);
}
String asCategoryHeader() {
return (onByDefault() ? "On by default" : "Experimental") + " : " + severity();
}
}
@AutoValue
abstract static class MiniDescription {
abstract String name();
abstract String summary();
static MiniDescription create(BugPatternInstance bugPattern) {
return new AutoValue_BugPatternIndexWriter_MiniDescription(
bugPattern.name, bugPattern.summary);
}
}
void dump(
Collection patterns, Writer w, Target target, Set enabledChecks)
throws IOException {
// (Default, Severity) -> [Pattern...]
SortedSetMultimap sorted =
TreeMultimap.create(
Comparator.comparing(IndexEntry::onByDefault)
.reversed()
.thenComparing(IndexEntry::severity),
Comparator.comparing(MiniDescription::name));
for (BugPatternInstance pattern : patterns) {
sorted.put(
IndexEntry.create(enabledChecks.contains(pattern.name), pattern.severity),
MiniDescription.create(pattern));
}
Map templateData = new HashMap<>();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy