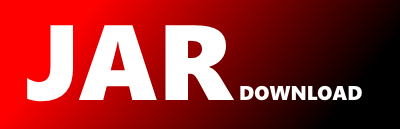
com.google.gcloud.resourcemanager.spi.ResourceManagerRpc Maven / Gradle / Ivy
/*
* Copyright 2015 Google Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.gcloud.resourcemanager.spi;
import com.google.api.services.cloudresourcemanager.model.Policy;
import com.google.api.services.cloudresourcemanager.model.Project;
import com.google.gcloud.resourcemanager.ResourceManagerException;
import java.util.List;
import java.util.Map;
public interface ResourceManagerRpc {
enum Option {
FILTER("filter"),
FIELDS("fields"),
PAGE_SIZE("pageSize"),
PAGE_TOKEN("pageToken");
private final String value;
Option(String value) {
this.value = value;
}
public String value() {
return value;
}
@SuppressWarnings("unchecked")
T get(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy