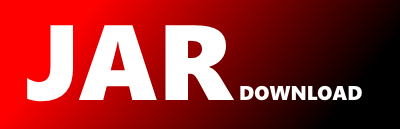
com.google.gerrit.acceptance.testsuite.account.AutoValue_TestAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.account;
import com.google.common.collect.ImmutableSet;
import com.google.gerrit.entities.Account;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestAccount extends TestAccount {
private final Account.Id accountId;
private final Optional fullname;
private final Optional preferredEmail;
private final Optional username;
private final boolean active;
private final ImmutableSet emails;
private AutoValue_TestAccount(
Account.Id accountId,
Optional fullname,
Optional preferredEmail,
Optional username,
boolean active,
ImmutableSet emails) {
this.accountId = accountId;
this.fullname = fullname;
this.preferredEmail = preferredEmail;
this.username = username;
this.active = active;
this.emails = emails;
}
@Override
public Account.Id accountId() {
return accountId;
}
@Override
public Optional fullname() {
return fullname;
}
@Override
public Optional preferredEmail() {
return preferredEmail;
}
@Override
public Optional username() {
return username;
}
@Override
public boolean active() {
return active;
}
@Override
public ImmutableSet emails() {
return emails;
}
@Override
public String toString() {
return "TestAccount{"
+ "accountId=" + accountId + ", "
+ "fullname=" + fullname + ", "
+ "preferredEmail=" + preferredEmail + ", "
+ "username=" + username + ", "
+ "active=" + active + ", "
+ "emails=" + emails
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestAccount) {
TestAccount that = (TestAccount) o;
return this.accountId.equals(that.accountId())
&& this.fullname.equals(that.fullname())
&& this.preferredEmail.equals(that.preferredEmail())
&& this.username.equals(that.username())
&& this.active == that.active()
&& this.emails.equals(that.emails());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= accountId.hashCode();
h$ *= 1000003;
h$ ^= fullname.hashCode();
h$ *= 1000003;
h$ ^= preferredEmail.hashCode();
h$ *= 1000003;
h$ ^= username.hashCode();
h$ *= 1000003;
h$ ^= active ? 1231 : 1237;
h$ *= 1000003;
h$ ^= emails.hashCode();
return h$;
}
static final class Builder extends TestAccount.Builder {
private Account.Id accountId;
private Optional fullname = Optional.empty();
private Optional preferredEmail = Optional.empty();
private Optional username = Optional.empty();
private boolean active;
private ImmutableSet emails;
private byte set$0;
Builder() {
}
@Override
TestAccount.Builder accountId(Account.Id accountId) {
if (accountId == null) {
throw new NullPointerException("Null accountId");
}
this.accountId = accountId;
return this;
}
@Override
TestAccount.Builder fullname(Optional fullname) {
if (fullname == null) {
throw new NullPointerException("Null fullname");
}
this.fullname = fullname;
return this;
}
@Override
TestAccount.Builder preferredEmail(Optional preferredEmail) {
if (preferredEmail == null) {
throw new NullPointerException("Null preferredEmail");
}
this.preferredEmail = preferredEmail;
return this;
}
@Override
TestAccount.Builder username(Optional username) {
if (username == null) {
throw new NullPointerException("Null username");
}
this.username = username;
return this;
}
@Override
TestAccount.Builder active(boolean active) {
this.active = active;
set$0 |= (byte) 1;
return this;
}
@Override
TestAccount.Builder emails(ImmutableSet emails) {
if (emails == null) {
throw new NullPointerException("Null emails");
}
this.emails = emails;
return this;
}
@Override
TestAccount build() {
if (set$0 != 1
|| this.accountId == null
|| this.emails == null) {
StringBuilder missing = new StringBuilder();
if (this.accountId == null) {
missing.append(" accountId");
}
if ((set$0 & 1) == 0) {
missing.append(" active");
}
if (this.emails == null) {
missing.append(" emails");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestAccount(
this.accountId,
this.fullname,
this.preferredEmail,
this.username,
this.active,
this.emails);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy