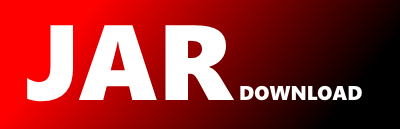
com.google.gerrit.acceptance.testsuite.account.AutoValue_TestAccountUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.account;
import com.google.common.collect.ImmutableSet;
import com.google.gerrit.acceptance.testsuite.ThrowingConsumer;
import java.util.Optional;
import java.util.Set;
import java.util.function.Function;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestAccountUpdate extends TestAccountUpdate {
private final Optional fullname;
private final Optional httpPassword;
private final Optional preferredEmail;
private final Optional username;
private final Optional status;
private final Optional active;
private final Function, Set> secondaryEmailsModification;
private final ThrowingConsumer accountUpdater;
private AutoValue_TestAccountUpdate(
Optional fullname,
Optional httpPassword,
Optional preferredEmail,
Optional username,
Optional status,
Optional active,
Function, Set> secondaryEmailsModification,
ThrowingConsumer accountUpdater) {
this.fullname = fullname;
this.httpPassword = httpPassword;
this.preferredEmail = preferredEmail;
this.username = username;
this.status = status;
this.active = active;
this.secondaryEmailsModification = secondaryEmailsModification;
this.accountUpdater = accountUpdater;
}
@Override
public Optional fullname() {
return fullname;
}
@Override
public Optional httpPassword() {
return httpPassword;
}
@Override
public Optional preferredEmail() {
return preferredEmail;
}
@Override
public Optional username() {
return username;
}
@Override
public Optional status() {
return status;
}
@Override
public Optional active() {
return active;
}
@Override
public Function, Set> secondaryEmailsModification() {
return secondaryEmailsModification;
}
@Override
ThrowingConsumer accountUpdater() {
return accountUpdater;
}
@Override
public String toString() {
return "TestAccountUpdate{"
+ "fullname=" + fullname + ", "
+ "httpPassword=" + httpPassword + ", "
+ "preferredEmail=" + preferredEmail + ", "
+ "username=" + username + ", "
+ "status=" + status + ", "
+ "active=" + active + ", "
+ "secondaryEmailsModification=" + secondaryEmailsModification + ", "
+ "accountUpdater=" + accountUpdater
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestAccountUpdate) {
TestAccountUpdate that = (TestAccountUpdate) o;
return this.fullname.equals(that.fullname())
&& this.httpPassword.equals(that.httpPassword())
&& this.preferredEmail.equals(that.preferredEmail())
&& this.username.equals(that.username())
&& this.status.equals(that.status())
&& this.active.equals(that.active())
&& this.secondaryEmailsModification.equals(that.secondaryEmailsModification())
&& this.accountUpdater.equals(that.accountUpdater());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= fullname.hashCode();
h$ *= 1000003;
h$ ^= httpPassword.hashCode();
h$ *= 1000003;
h$ ^= preferredEmail.hashCode();
h$ *= 1000003;
h$ ^= username.hashCode();
h$ *= 1000003;
h$ ^= status.hashCode();
h$ *= 1000003;
h$ ^= active.hashCode();
h$ *= 1000003;
h$ ^= secondaryEmailsModification.hashCode();
h$ *= 1000003;
h$ ^= accountUpdater.hashCode();
return h$;
}
static final class Builder extends TestAccountUpdate.Builder {
private Optional fullname = Optional.empty();
private Optional httpPassword = Optional.empty();
private Optional preferredEmail = Optional.empty();
private Optional username = Optional.empty();
private Optional status = Optional.empty();
private Optional active = Optional.empty();
private Function, Set> secondaryEmailsModification;
private ThrowingConsumer accountUpdater;
Builder() {
}
@Override
public TestAccountUpdate.Builder fullname(String fullname) {
this.fullname = Optional.of(fullname);
return this;
}
@Override
public TestAccountUpdate.Builder httpPassword(String httpPassword) {
this.httpPassword = Optional.of(httpPassword);
return this;
}
@Override
public TestAccountUpdate.Builder preferredEmail(String preferredEmail) {
this.preferredEmail = Optional.of(preferredEmail);
return this;
}
@Override
public TestAccountUpdate.Builder username(String username) {
this.username = Optional.of(username);
return this;
}
@Override
public TestAccountUpdate.Builder status(String status) {
this.status = Optional.of(status);
return this;
}
@Override
TestAccountUpdate.Builder active(boolean active) {
this.active = Optional.of(active);
return this;
}
@Override
TestAccountUpdate.Builder secondaryEmailsModification(Function, Set> secondaryEmailsModification) {
if (secondaryEmailsModification == null) {
throw new NullPointerException("Null secondaryEmailsModification");
}
this.secondaryEmailsModification = secondaryEmailsModification;
return this;
}
@Override
Function, Set> secondaryEmailsModification() {
if (this.secondaryEmailsModification == null) {
throw new IllegalStateException("Property \"secondaryEmailsModification\" has not been set");
}
return secondaryEmailsModification;
}
@Override
TestAccountUpdate.Builder accountUpdater(ThrowingConsumer accountUpdater) {
if (accountUpdater == null) {
throw new NullPointerException("Null accountUpdater");
}
this.accountUpdater = accountUpdater;
return this;
}
@Override
TestAccountUpdate autoBuild() {
if (this.secondaryEmailsModification == null
|| this.accountUpdater == null) {
StringBuilder missing = new StringBuilder();
if (this.secondaryEmailsModification == null) {
missing.append(" secondaryEmailsModification");
}
if (this.accountUpdater == null) {
missing.append(" accountUpdater");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestAccountUpdate(
this.fullname,
this.httpPassword,
this.preferredEmail,
this.username,
this.status,
this.active,
this.secondaryEmailsModification,
this.accountUpdater);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy