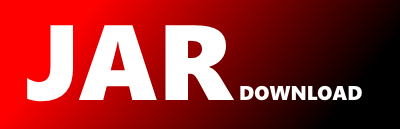
com.google.gerrit.acceptance.testsuite.change.AutoValue_TestCommentCreation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.change;
import com.google.gerrit.acceptance.testsuite.ThrowingFunction;
import com.google.gerrit.common.Nullable;
import com.google.gerrit.entities.Account;
import com.google.gerrit.entities.Comment;
import java.time.Instant;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestCommentCreation extends TestCommentCreation {
private final Optional message;
private final Optional file;
private final Optional line;
private final Optional range;
private final Optional side;
private final Optional unresolved;
private final Optional parentUuid;
private final Optional tag;
private final Optional author;
private final Optional createdOn;
private final Comment.Status status;
private final ThrowingFunction commentCreator;
private AutoValue_TestCommentCreation(
Optional message,
Optional file,
Optional line,
Optional range,
Optional side,
Optional unresolved,
Optional parentUuid,
Optional tag,
Optional author,
Optional createdOn,
Comment.Status status,
ThrowingFunction commentCreator) {
this.message = message;
this.file = file;
this.line = line;
this.range = range;
this.side = side;
this.unresolved = unresolved;
this.parentUuid = parentUuid;
this.tag = tag;
this.author = author;
this.createdOn = createdOn;
this.status = status;
this.commentCreator = commentCreator;
}
@Override
public Optional message() {
return message;
}
@Override
public Optional file() {
return file;
}
@Override
public Optional line() {
return line;
}
@Override
public Optional range() {
return range;
}
@Override
public Optional side() {
return side;
}
@Override
public Optional unresolved() {
return unresolved;
}
@Override
public Optional parentUuid() {
return parentUuid;
}
@Override
public Optional tag() {
return tag;
}
@Override
public Optional author() {
return author;
}
@Override
public Optional createdOn() {
return createdOn;
}
@Override
Comment.Status status() {
return status;
}
@Override
ThrowingFunction commentCreator() {
return commentCreator;
}
@Override
public String toString() {
return "TestCommentCreation{"
+ "message=" + message + ", "
+ "file=" + file + ", "
+ "line=" + line + ", "
+ "range=" + range + ", "
+ "side=" + side + ", "
+ "unresolved=" + unresolved + ", "
+ "parentUuid=" + parentUuid + ", "
+ "tag=" + tag + ", "
+ "author=" + author + ", "
+ "createdOn=" + createdOn + ", "
+ "status=" + status + ", "
+ "commentCreator=" + commentCreator
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestCommentCreation) {
TestCommentCreation that = (TestCommentCreation) o;
return this.message.equals(that.message())
&& this.file.equals(that.file())
&& this.line.equals(that.line())
&& this.range.equals(that.range())
&& this.side.equals(that.side())
&& this.unresolved.equals(that.unresolved())
&& this.parentUuid.equals(that.parentUuid())
&& this.tag.equals(that.tag())
&& this.author.equals(that.author())
&& this.createdOn.equals(that.createdOn())
&& this.status.equals(that.status())
&& this.commentCreator.equals(that.commentCreator());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= message.hashCode();
h$ *= 1000003;
h$ ^= file.hashCode();
h$ *= 1000003;
h$ ^= line.hashCode();
h$ *= 1000003;
h$ ^= range.hashCode();
h$ *= 1000003;
h$ ^= side.hashCode();
h$ *= 1000003;
h$ ^= unresolved.hashCode();
h$ *= 1000003;
h$ ^= parentUuid.hashCode();
h$ *= 1000003;
h$ ^= tag.hashCode();
h$ *= 1000003;
h$ ^= author.hashCode();
h$ *= 1000003;
h$ ^= createdOn.hashCode();
h$ *= 1000003;
h$ ^= status.hashCode();
h$ *= 1000003;
h$ ^= commentCreator.hashCode();
return h$;
}
static final class Builder extends TestCommentCreation.Builder {
private Optional message = Optional.empty();
private Optional file = Optional.empty();
private Optional line = Optional.empty();
private Optional range = Optional.empty();
private Optional side = Optional.empty();
private Optional unresolved = Optional.empty();
private Optional parentUuid = Optional.empty();
private Optional tag = Optional.empty();
private Optional author = Optional.empty();
private Optional createdOn = Optional.empty();
private Comment.Status status;
private ThrowingFunction commentCreator;
Builder() {
}
@Override
public TestCommentCreation.Builder message(String message) {
this.message = Optional.of(message);
return this;
}
@Override
TestCommentCreation.Builder file(String file) {
this.file = Optional.of(file);
return this;
}
@Override
TestCommentCreation.Builder line(@Nullable Integer line) {
this.line = Optional.ofNullable(line);
return this;
}
@Override
TestCommentCreation.Builder range(@Nullable TestRange range) {
this.range = Optional.ofNullable(range);
return this;
}
@Override
TestCommentCreation.Builder side(CommentSide side) {
this.side = Optional.of(side);
return this;
}
@Override
TestCommentCreation.Builder unresolved(boolean unresolved) {
this.unresolved = Optional.of(unresolved);
return this;
}
@Override
public TestCommentCreation.Builder parentUuid(String parentUuid) {
this.parentUuid = Optional.of(parentUuid);
return this;
}
@Override
public TestCommentCreation.Builder tag(String tag) {
this.tag = Optional.of(tag);
return this;
}
@Override
public TestCommentCreation.Builder author(Account.Id author) {
this.author = Optional.of(author);
return this;
}
@Override
public TestCommentCreation.Builder createdOn(Instant createdOn) {
this.createdOn = Optional.of(createdOn);
return this;
}
@Override
TestCommentCreation.Builder status(Comment.Status status) {
if (status == null) {
throw new NullPointerException("Null status");
}
this.status = status;
return this;
}
@Override
TestCommentCreation.Builder commentCreator(ThrowingFunction commentCreator) {
if (commentCreator == null) {
throw new NullPointerException("Null commentCreator");
}
this.commentCreator = commentCreator;
return this;
}
@Override
TestCommentCreation autoBuild() {
if (this.status == null
|| this.commentCreator == null) {
StringBuilder missing = new StringBuilder();
if (this.status == null) {
missing.append(" status");
}
if (this.commentCreator == null) {
missing.append(" commentCreator");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestCommentCreation(
this.message,
this.file,
this.line,
this.range,
this.side,
this.unresolved,
this.parentUuid,
this.tag,
this.author,
this.createdOn,
this.status,
this.commentCreator);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy