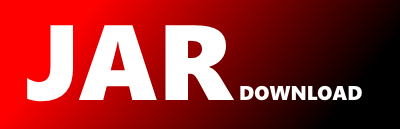
com.google.gerrit.acceptance.testsuite.change.AutoValue_TestRobotCommentCreation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.change;
import com.google.common.collect.ImmutableMap;
import com.google.gerrit.acceptance.testsuite.ThrowingFunction;
import com.google.gerrit.common.Nullable;
import com.google.gerrit.entities.Account;
import java.util.Map;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestRobotCommentCreation extends TestRobotCommentCreation {
private final Optional message;
private final Optional file;
private final Optional line;
private final Optional range;
private final Optional side;
private final Optional parentUuid;
private final Optional tag;
private final Optional author;
private final Optional robotId;
private final Optional robotRunId;
private final Optional url;
private final ImmutableMap properties;
private final ThrowingFunction commentCreator;
private AutoValue_TestRobotCommentCreation(
Optional message,
Optional file,
Optional line,
Optional range,
Optional side,
Optional parentUuid,
Optional tag,
Optional author,
Optional robotId,
Optional robotRunId,
Optional url,
ImmutableMap properties,
ThrowingFunction commentCreator) {
this.message = message;
this.file = file;
this.line = line;
this.range = range;
this.side = side;
this.parentUuid = parentUuid;
this.tag = tag;
this.author = author;
this.robotId = robotId;
this.robotRunId = robotRunId;
this.url = url;
this.properties = properties;
this.commentCreator = commentCreator;
}
@Override
public Optional message() {
return message;
}
@Override
public Optional file() {
return file;
}
@Override
public Optional line() {
return line;
}
@Override
public Optional range() {
return range;
}
@Override
public Optional side() {
return side;
}
@Override
public Optional parentUuid() {
return parentUuid;
}
@Override
public Optional tag() {
return tag;
}
@Override
public Optional author() {
return author;
}
@Override
public Optional robotId() {
return robotId;
}
@Override
public Optional robotRunId() {
return robotRunId;
}
@Override
public Optional url() {
return url;
}
@Override
public ImmutableMap properties() {
return properties;
}
@Override
ThrowingFunction commentCreator() {
return commentCreator;
}
@Override
public String toString() {
return "TestRobotCommentCreation{"
+ "message=" + message + ", "
+ "file=" + file + ", "
+ "line=" + line + ", "
+ "range=" + range + ", "
+ "side=" + side + ", "
+ "parentUuid=" + parentUuid + ", "
+ "tag=" + tag + ", "
+ "author=" + author + ", "
+ "robotId=" + robotId + ", "
+ "robotRunId=" + robotRunId + ", "
+ "url=" + url + ", "
+ "properties=" + properties + ", "
+ "commentCreator=" + commentCreator
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestRobotCommentCreation) {
TestRobotCommentCreation that = (TestRobotCommentCreation) o;
return this.message.equals(that.message())
&& this.file.equals(that.file())
&& this.line.equals(that.line())
&& this.range.equals(that.range())
&& this.side.equals(that.side())
&& this.parentUuid.equals(that.parentUuid())
&& this.tag.equals(that.tag())
&& this.author.equals(that.author())
&& this.robotId.equals(that.robotId())
&& this.robotRunId.equals(that.robotRunId())
&& this.url.equals(that.url())
&& this.properties.equals(that.properties())
&& this.commentCreator.equals(that.commentCreator());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= message.hashCode();
h$ *= 1000003;
h$ ^= file.hashCode();
h$ *= 1000003;
h$ ^= line.hashCode();
h$ *= 1000003;
h$ ^= range.hashCode();
h$ *= 1000003;
h$ ^= side.hashCode();
h$ *= 1000003;
h$ ^= parentUuid.hashCode();
h$ *= 1000003;
h$ ^= tag.hashCode();
h$ *= 1000003;
h$ ^= author.hashCode();
h$ *= 1000003;
h$ ^= robotId.hashCode();
h$ *= 1000003;
h$ ^= robotRunId.hashCode();
h$ *= 1000003;
h$ ^= url.hashCode();
h$ *= 1000003;
h$ ^= properties.hashCode();
h$ *= 1000003;
h$ ^= commentCreator.hashCode();
return h$;
}
static final class Builder extends TestRobotCommentCreation.Builder {
private Optional message = Optional.empty();
private Optional file = Optional.empty();
private Optional line = Optional.empty();
private Optional range = Optional.empty();
private Optional side = Optional.empty();
private Optional parentUuid = Optional.empty();
private Optional tag = Optional.empty();
private Optional author = Optional.empty();
private Optional robotId = Optional.empty();
private Optional robotRunId = Optional.empty();
private Optional url = Optional.empty();
private ImmutableMap.Builder propertiesBuilder$;
private ImmutableMap properties;
private ThrowingFunction commentCreator;
Builder() {
}
@Override
public TestRobotCommentCreation.Builder message(String message) {
this.message = Optional.of(message);
return this;
}
@Override
TestRobotCommentCreation.Builder file(String file) {
this.file = Optional.of(file);
return this;
}
@Override
TestRobotCommentCreation.Builder line(@Nullable Integer line) {
this.line = Optional.ofNullable(line);
return this;
}
@Override
TestRobotCommentCreation.Builder range(@Nullable TestRange range) {
this.range = Optional.ofNullable(range);
return this;
}
@Override
TestRobotCommentCreation.Builder side(CommentSide side) {
this.side = Optional.of(side);
return this;
}
@Override
public TestRobotCommentCreation.Builder parentUuid(String parentUuid) {
this.parentUuid = Optional.of(parentUuid);
return this;
}
@Override
public TestRobotCommentCreation.Builder tag(String tag) {
this.tag = Optional.of(tag);
return this;
}
@Override
public TestRobotCommentCreation.Builder author(Account.Id author) {
this.author = Optional.of(author);
return this;
}
@Override
public TestRobotCommentCreation.Builder robotId(String robotId) {
this.robotId = Optional.of(robotId);
return this;
}
@Override
public TestRobotCommentCreation.Builder robotRunId(String robotRunId) {
this.robotRunId = Optional.of(robotRunId);
return this;
}
@Override
public TestRobotCommentCreation.Builder url(String url) {
this.url = Optional.of(url);
return this;
}
@Override
public TestRobotCommentCreation.Builder properties(Map properties) {
if (propertiesBuilder$ != null) {
throw new IllegalStateException("Cannot set properties after calling propertiesBuilder()");
}
this.properties = ImmutableMap.copyOf(properties);
return this;
}
@Override
ImmutableMap.Builder propertiesBuilder() {
if (propertiesBuilder$ == null) {
if (properties == null) {
propertiesBuilder$ = ImmutableMap.builder();
} else {
propertiesBuilder$ = ImmutableMap.builder();
propertiesBuilder$.putAll(properties);
properties = null;
}
}
return propertiesBuilder$;
}
@Override
TestRobotCommentCreation.Builder commentCreator(ThrowingFunction commentCreator) {
if (commentCreator == null) {
throw new NullPointerException("Null commentCreator");
}
this.commentCreator = commentCreator;
return this;
}
@Override
TestRobotCommentCreation autoBuild() {
if (propertiesBuilder$ != null) {
this.properties = propertiesBuilder$.buildOrThrow();
} else if (this.properties == null) {
this.properties = ImmutableMap.of();
}
if (this.commentCreator == null) {
String missing = " commentCreator";
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestRobotCommentCreation(
this.message,
this.file,
this.line,
this.range,
this.side,
this.parentUuid,
this.tag,
this.author,
this.robotId,
this.robotRunId,
this.url,
this.properties,
this.commentCreator);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy