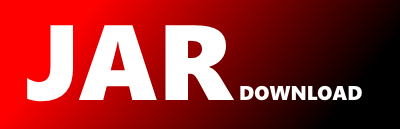
com.google.gerrit.acceptance.AutoValue_GerritServer_Description Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance;
import com.google.gerrit.acceptance.config.GerritConfig;
import com.google.gerrit.acceptance.config.GerritConfigs;
import com.google.gerrit.acceptance.config.GerritSystemProperties;
import com.google.gerrit.acceptance.config.GerritSystemProperty;
import com.google.gerrit.acceptance.config.GlobalPluginConfig;
import com.google.gerrit.acceptance.config.GlobalPluginConfigs;
import com.google.gerrit.common.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_GerritServer_Description extends GerritServer.Description {
private final org.junit.runner.Description testDescription;
@Nullable
private final String configName;
private final boolean memory;
private final boolean httpd;
private final boolean sandboxed;
private final boolean skipProjectClone;
private final boolean useSshAnnotation;
private final boolean verifyNoPiiInChangeNotes;
private final boolean useSystemTime;
@Nullable
private final UseClockStep useClockStep;
@Nullable
private final UseTimezone useTimezone;
@Nullable
private final GerritSystemProperty systemProperty;
@Nullable
private final GerritSystemProperties systemProperties;
@Nullable
private final GerritConfig config;
@Nullable
private final GerritConfigs configs;
@Nullable
private final GlobalPluginConfig pluginConfig;
@Nullable
private final GlobalPluginConfigs pluginConfigs;
AutoValue_GerritServer_Description(
org.junit.runner.Description testDescription,
@Nullable String configName,
boolean memory,
boolean httpd,
boolean sandboxed,
boolean skipProjectClone,
boolean useSshAnnotation,
boolean verifyNoPiiInChangeNotes,
boolean useSystemTime,
@Nullable UseClockStep useClockStep,
@Nullable UseTimezone useTimezone,
@Nullable GerritSystemProperty systemProperty,
@Nullable GerritSystemProperties systemProperties,
@Nullable GerritConfig config,
@Nullable GerritConfigs configs,
@Nullable GlobalPluginConfig pluginConfig,
@Nullable GlobalPluginConfigs pluginConfigs) {
if (testDescription == null) {
throw new NullPointerException("Null testDescription");
}
this.testDescription = testDescription;
this.configName = configName;
this.memory = memory;
this.httpd = httpd;
this.sandboxed = sandboxed;
this.skipProjectClone = skipProjectClone;
this.useSshAnnotation = useSshAnnotation;
this.verifyNoPiiInChangeNotes = verifyNoPiiInChangeNotes;
this.useSystemTime = useSystemTime;
this.useClockStep = useClockStep;
this.useTimezone = useTimezone;
this.systemProperty = systemProperty;
this.systemProperties = systemProperties;
this.config = config;
this.configs = configs;
this.pluginConfig = pluginConfig;
this.pluginConfigs = pluginConfigs;
}
@Override
org.junit.runner.Description testDescription() {
return testDescription;
}
@Nullable
@Override
String configName() {
return configName;
}
@Override
boolean memory() {
return memory;
}
@Override
boolean httpd() {
return httpd;
}
@Override
boolean sandboxed() {
return sandboxed;
}
@Override
public boolean skipProjectClone() {
return skipProjectClone;
}
@Override
boolean useSshAnnotation() {
return useSshAnnotation;
}
@Override
boolean verifyNoPiiInChangeNotes() {
return verifyNoPiiInChangeNotes;
}
@Override
boolean useSystemTime() {
return useSystemTime;
}
@Nullable
@Override
UseClockStep useClockStep() {
return useClockStep;
}
@Nullable
@Override
UseTimezone useTimezone() {
return useTimezone;
}
@Nullable
@Override
GerritSystemProperty systemProperty() {
return systemProperty;
}
@Nullable
@Override
GerritSystemProperties systemProperties() {
return systemProperties;
}
@Nullable
@Override
GerritConfig config() {
return config;
}
@Nullable
@Override
GerritConfigs configs() {
return configs;
}
@Nullable
@Override
GlobalPluginConfig pluginConfig() {
return pluginConfig;
}
@Nullable
@Override
GlobalPluginConfigs pluginConfigs() {
return pluginConfigs;
}
@Override
public String toString() {
return "Description{"
+ "testDescription=" + testDescription + ", "
+ "configName=" + configName + ", "
+ "memory=" + memory + ", "
+ "httpd=" + httpd + ", "
+ "sandboxed=" + sandboxed + ", "
+ "skipProjectClone=" + skipProjectClone + ", "
+ "useSshAnnotation=" + useSshAnnotation + ", "
+ "verifyNoPiiInChangeNotes=" + verifyNoPiiInChangeNotes + ", "
+ "useSystemTime=" + useSystemTime + ", "
+ "useClockStep=" + useClockStep + ", "
+ "useTimezone=" + useTimezone + ", "
+ "systemProperty=" + systemProperty + ", "
+ "systemProperties=" + systemProperties + ", "
+ "config=" + config + ", "
+ "configs=" + configs + ", "
+ "pluginConfig=" + pluginConfig + ", "
+ "pluginConfigs=" + pluginConfigs
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof GerritServer.Description) {
GerritServer.Description that = (GerritServer.Description) o;
return this.testDescription.equals(that.testDescription())
&& (this.configName == null ? that.configName() == null : this.configName.equals(that.configName()))
&& this.memory == that.memory()
&& this.httpd == that.httpd()
&& this.sandboxed == that.sandboxed()
&& this.skipProjectClone == that.skipProjectClone()
&& this.useSshAnnotation == that.useSshAnnotation()
&& this.verifyNoPiiInChangeNotes == that.verifyNoPiiInChangeNotes()
&& this.useSystemTime == that.useSystemTime()
&& (this.useClockStep == null ? that.useClockStep() == null : this.useClockStep.equals(that.useClockStep()))
&& (this.useTimezone == null ? that.useTimezone() == null : this.useTimezone.equals(that.useTimezone()))
&& (this.systemProperty == null ? that.systemProperty() == null : this.systemProperty.equals(that.systemProperty()))
&& (this.systemProperties == null ? that.systemProperties() == null : this.systemProperties.equals(that.systemProperties()))
&& (this.config == null ? that.config() == null : this.config.equals(that.config()))
&& (this.configs == null ? that.configs() == null : this.configs.equals(that.configs()))
&& (this.pluginConfig == null ? that.pluginConfig() == null : this.pluginConfig.equals(that.pluginConfig()))
&& (this.pluginConfigs == null ? that.pluginConfigs() == null : this.pluginConfigs.equals(that.pluginConfigs()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= testDescription.hashCode();
h$ *= 1000003;
h$ ^= (configName == null) ? 0 : configName.hashCode();
h$ *= 1000003;
h$ ^= memory ? 1231 : 1237;
h$ *= 1000003;
h$ ^= httpd ? 1231 : 1237;
h$ *= 1000003;
h$ ^= sandboxed ? 1231 : 1237;
h$ *= 1000003;
h$ ^= skipProjectClone ? 1231 : 1237;
h$ *= 1000003;
h$ ^= useSshAnnotation ? 1231 : 1237;
h$ *= 1000003;
h$ ^= verifyNoPiiInChangeNotes ? 1231 : 1237;
h$ *= 1000003;
h$ ^= useSystemTime ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (useClockStep == null) ? 0 : useClockStep.hashCode();
h$ *= 1000003;
h$ ^= (useTimezone == null) ? 0 : useTimezone.hashCode();
h$ *= 1000003;
h$ ^= (systemProperty == null) ? 0 : systemProperty.hashCode();
h$ *= 1000003;
h$ ^= (systemProperties == null) ? 0 : systemProperties.hashCode();
h$ *= 1000003;
h$ ^= (config == null) ? 0 : config.hashCode();
h$ *= 1000003;
h$ ^= (configs == null) ? 0 : configs.hashCode();
h$ *= 1000003;
h$ ^= (pluginConfig == null) ? 0 : pluginConfig.hashCode();
h$ *= 1000003;
h$ ^= (pluginConfigs == null) ? 0 : pluginConfigs.hashCode();
return h$;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy