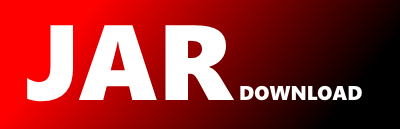
com.google.gerrit.acceptance.testsuite.account.AutoValue_TestAccountCreation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.account;
import com.google.common.collect.ImmutableSet;
import com.google.gerrit.acceptance.testsuite.ThrowingFunction;
import com.google.gerrit.entities.Account;
import java.util.Optional;
import java.util.Set;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestAccountCreation extends TestAccountCreation {
private final Optional fullname;
private final Optional httpPassword;
private final Optional preferredEmail;
private final Optional username;
private final Optional status;
private final Optional active;
private final ImmutableSet secondaryEmails;
private final ThrowingFunction accountCreator;
private AutoValue_TestAccountCreation(
Optional fullname,
Optional httpPassword,
Optional preferredEmail,
Optional username,
Optional status,
Optional active,
ImmutableSet secondaryEmails,
ThrowingFunction accountCreator) {
this.fullname = fullname;
this.httpPassword = httpPassword;
this.preferredEmail = preferredEmail;
this.username = username;
this.status = status;
this.active = active;
this.secondaryEmails = secondaryEmails;
this.accountCreator = accountCreator;
}
@Override
public Optional fullname() {
return fullname;
}
@Override
public Optional httpPassword() {
return httpPassword;
}
@Override
public Optional preferredEmail() {
return preferredEmail;
}
@Override
public Optional username() {
return username;
}
@Override
public Optional status() {
return status;
}
@Override
public Optional active() {
return active;
}
@Override
public ImmutableSet secondaryEmails() {
return secondaryEmails;
}
@Override
ThrowingFunction accountCreator() {
return accountCreator;
}
@Override
public String toString() {
return "TestAccountCreation{"
+ "fullname=" + fullname + ", "
+ "httpPassword=" + httpPassword + ", "
+ "preferredEmail=" + preferredEmail + ", "
+ "username=" + username + ", "
+ "status=" + status + ", "
+ "active=" + active + ", "
+ "secondaryEmails=" + secondaryEmails + ", "
+ "accountCreator=" + accountCreator
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestAccountCreation) {
TestAccountCreation that = (TestAccountCreation) o;
return this.fullname.equals(that.fullname())
&& this.httpPassword.equals(that.httpPassword())
&& this.preferredEmail.equals(that.preferredEmail())
&& this.username.equals(that.username())
&& this.status.equals(that.status())
&& this.active.equals(that.active())
&& this.secondaryEmails.equals(that.secondaryEmails())
&& this.accountCreator.equals(that.accountCreator());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= fullname.hashCode();
h$ *= 1000003;
h$ ^= httpPassword.hashCode();
h$ *= 1000003;
h$ ^= preferredEmail.hashCode();
h$ *= 1000003;
h$ ^= username.hashCode();
h$ *= 1000003;
h$ ^= status.hashCode();
h$ *= 1000003;
h$ ^= active.hashCode();
h$ *= 1000003;
h$ ^= secondaryEmails.hashCode();
h$ *= 1000003;
h$ ^= accountCreator.hashCode();
return h$;
}
static final class Builder extends TestAccountCreation.Builder {
private Optional fullname = Optional.empty();
private Optional httpPassword = Optional.empty();
private Optional preferredEmail = Optional.empty();
private Optional username = Optional.empty();
private Optional status = Optional.empty();
private Optional active = Optional.empty();
private ImmutableSet.Builder secondaryEmailsBuilder$;
private ImmutableSet secondaryEmails;
private ThrowingFunction accountCreator;
Builder() {
}
@Override
public TestAccountCreation.Builder fullname(String fullname) {
this.fullname = Optional.of(fullname);
return this;
}
@Override
public TestAccountCreation.Builder httpPassword(String httpPassword) {
this.httpPassword = Optional.of(httpPassword);
return this;
}
@Override
public TestAccountCreation.Builder preferredEmail(String preferredEmail) {
this.preferredEmail = Optional.of(preferredEmail);
return this;
}
@Override
public TestAccountCreation.Builder username(String username) {
this.username = Optional.of(username);
return this;
}
@Override
public TestAccountCreation.Builder status(String status) {
this.status = Optional.of(status);
return this;
}
@Override
TestAccountCreation.Builder active(boolean active) {
this.active = Optional.of(active);
return this;
}
@Override
public TestAccountCreation.Builder secondaryEmails(Set secondaryEmails) {
if (secondaryEmailsBuilder$ != null) {
throw new IllegalStateException("Cannot set secondaryEmails after calling secondaryEmailsBuilder()");
}
this.secondaryEmails = ImmutableSet.copyOf(secondaryEmails);
return this;
}
@Override
ImmutableSet.Builder secondaryEmailsBuilder() {
if (secondaryEmailsBuilder$ == null) {
if (secondaryEmails == null) {
secondaryEmailsBuilder$ = ImmutableSet.builder();
} else {
secondaryEmailsBuilder$ = ImmutableSet.builder();
secondaryEmailsBuilder$.addAll(secondaryEmails);
secondaryEmails = null;
}
}
return secondaryEmailsBuilder$;
}
@Override
TestAccountCreation.Builder accountCreator(ThrowingFunction accountCreator) {
if (accountCreator == null) {
throw new NullPointerException("Null accountCreator");
}
this.accountCreator = accountCreator;
return this;
}
@Override
TestAccountCreation autoBuild() {
if (secondaryEmailsBuilder$ != null) {
this.secondaryEmails = secondaryEmailsBuilder$.build();
} else if (this.secondaryEmails == null) {
this.secondaryEmails = ImmutableSet.of();
}
if (this.accountCreator == null) {
String missing = " accountCreator";
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestAccountCreation(
this.fullname,
this.httpPassword,
this.preferredEmail,
this.username,
this.status,
this.active,
this.secondaryEmails,
this.accountCreator);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy