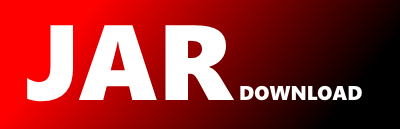
com.google.gerrit.acceptance.testsuite.change.AutoOneOf_TestCommitIdentifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.change;
import com.google.gerrit.entities.Change;
import com.google.gerrit.entities.PatchSet;
import javax.annotation.processing.Generated;
import org.eclipse.jgit.lib.ObjectId;
@Generated("com.google.auto.value.processor.AutoOneOfProcessor")
final class AutoOneOf_TestCommitIdentifier {
private AutoOneOf_TestCommitIdentifier() {} // There are no instances of this type.
static TestCommitIdentifier commitSha1(ObjectId commitSha1) {
if (commitSha1 == null) {
throw new NullPointerException();
}
return new Impl_commitSha1(commitSha1);
}
static TestCommitIdentifier branch(String branch) {
if (branch == null) {
throw new NullPointerException();
}
return new Impl_branch(branch);
}
static TestCommitIdentifier changeId(Change.Id changeId) {
if (changeId == null) {
throw new NullPointerException();
}
return new Impl_changeId(changeId);
}
static TestCommitIdentifier patchsetId(PatchSet.Id patchsetId) {
if (patchsetId == null) {
throw new NullPointerException();
}
return new Impl_patchsetId(patchsetId);
}
// Parent class that each implementation will inherit from.
private abstract static class Parent_ extends TestCommitIdentifier {
@Override
public ObjectId commitSha1() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public String branch() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public Change.Id changeId() {
throw new UnsupportedOperationException(getKind().toString());
}
@Override
public PatchSet.Id patchsetId() {
throw new UnsupportedOperationException(getKind().toString());
}
}
// Implementation when the contained property is "commitSha1".
private static final class Impl_commitSha1 extends Parent_ {
private final ObjectId commitSha1;
Impl_commitSha1(ObjectId commitSha1) {
this.commitSha1 = commitSha1;
}
@Override
public ObjectId commitSha1() {
return commitSha1;
}
@Override
public String toString() {
return "TestCommitIdentifier{commitSha1=" + this.commitSha1 + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof TestCommitIdentifier) {
TestCommitIdentifier that = (TestCommitIdentifier) x;
return this.getKind() == that.getKind()
&& this.commitSha1.equals(that.commitSha1());
} else {
return false;
}
}
@Override
public int hashCode() {
return commitSha1.hashCode();
}
@Override
public TestCommitIdentifier.Kind getKind() {
return TestCommitIdentifier.Kind.COMMIT_SHA_1;
}
}
// Implementation when the contained property is "branch".
private static final class Impl_branch extends Parent_ {
private final String branch;
Impl_branch(String branch) {
this.branch = branch;
}
@Override
public String branch() {
return branch;
}
@Override
public String toString() {
return "TestCommitIdentifier{branch=" + this.branch + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof TestCommitIdentifier) {
TestCommitIdentifier that = (TestCommitIdentifier) x;
return this.getKind() == that.getKind()
&& this.branch.equals(that.branch());
} else {
return false;
}
}
@Override
public int hashCode() {
return branch.hashCode();
}
@Override
public TestCommitIdentifier.Kind getKind() {
return TestCommitIdentifier.Kind.BRANCH;
}
}
// Implementation when the contained property is "changeId".
private static final class Impl_changeId extends Parent_ {
private final Change.Id changeId;
Impl_changeId(Change.Id changeId) {
this.changeId = changeId;
}
@Override
public Change.Id changeId() {
return changeId;
}
@Override
public String toString() {
return "TestCommitIdentifier{changeId=" + this.changeId + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof TestCommitIdentifier) {
TestCommitIdentifier that = (TestCommitIdentifier) x;
return this.getKind() == that.getKind()
&& this.changeId.equals(that.changeId());
} else {
return false;
}
}
@Override
public int hashCode() {
return changeId.hashCode();
}
@Override
public TestCommitIdentifier.Kind getKind() {
return TestCommitIdentifier.Kind.CHANGE_ID;
}
}
// Implementation when the contained property is "patchsetId".
private static final class Impl_patchsetId extends Parent_ {
private final PatchSet.Id patchsetId;
Impl_patchsetId(PatchSet.Id patchsetId) {
this.patchsetId = patchsetId;
}
@Override
public PatchSet.Id patchsetId() {
return patchsetId;
}
@Override
public String toString() {
return "TestCommitIdentifier{patchsetId=" + this.patchsetId + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof TestCommitIdentifier) {
TestCommitIdentifier that = (TestCommitIdentifier) x;
return this.getKind() == that.getKind()
&& this.patchsetId.equals(that.patchsetId());
} else {
return false;
}
}
@Override
public int hashCode() {
return patchsetId.hashCode();
}
@Override
public TestCommitIdentifier.Kind getKind() {
return TestCommitIdentifier.Kind.PATCHSET_ID;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy