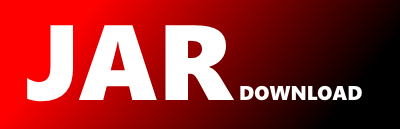
com.google.gerrit.acceptance.testsuite.change.AutoValue_TestChangeCreation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.change;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.gerrit.acceptance.testsuite.ThrowingFunction;
import com.google.gerrit.common.UsedAt;
import com.google.gerrit.entities.Account;
import com.google.gerrit.entities.Change;
import com.google.gerrit.entities.Project;
import com.google.gerrit.server.edit.tree.TreeModification;
import java.util.Optional;
import javax.annotation.processing.Generated;
import org.eclipse.jgit.lib.PersonIdent;
import org.eclipse.jgit.merge.MergeStrategy;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestChangeCreation extends TestChangeCreation {
private final Optional host;
private final Optional project;
private final String branch;
private final Optional owner;
private final Optional author;
private final Optional authorIdent;
private final Optional committer;
private final Optional committerIdent;
private final Optional topic;
private final ImmutableMap approvals;
private final String commitMessage;
private final ImmutableList treeModifications;
private final Optional> parents;
private final MergeStrategy mergeStrategy;
private final ThrowingFunction changeCreator;
private AutoValue_TestChangeCreation(
Optional host,
Optional project,
String branch,
Optional owner,
Optional author,
Optional authorIdent,
Optional committer,
Optional committerIdent,
Optional topic,
ImmutableMap approvals,
String commitMessage,
ImmutableList treeModifications,
Optional> parents,
MergeStrategy mergeStrategy,
ThrowingFunction changeCreator) {
this.host = host;
this.project = project;
this.branch = branch;
this.owner = owner;
this.author = author;
this.authorIdent = authorIdent;
this.committer = committer;
this.committerIdent = committerIdent;
this.topic = topic;
this.approvals = approvals;
this.commitMessage = commitMessage;
this.treeModifications = treeModifications;
this.parents = parents;
this.mergeStrategy = mergeStrategy;
this.changeCreator = changeCreator;
}
@UsedAt(UsedAt.Project.GOOGLE)
@Override
public Optional host() {
return host;
}
@Override
public Optional project() {
return project;
}
@Override
public String branch() {
return branch;
}
@Override
public Optional owner() {
return owner;
}
@Override
public Optional author() {
return author;
}
@Override
public Optional authorIdent() {
return authorIdent;
}
@Override
public Optional committer() {
return committer;
}
@Override
public Optional committerIdent() {
return committerIdent;
}
@Override
public Optional topic() {
return topic;
}
@Override
public ImmutableMap approvals() {
return approvals;
}
@Override
public String commitMessage() {
return commitMessage;
}
@Override
public ImmutableList treeModifications() {
return treeModifications;
}
@Override
public Optional> parents() {
return parents;
}
@Override
public MergeStrategy mergeStrategy() {
return mergeStrategy;
}
@Override
ThrowingFunction changeCreator() {
return changeCreator;
}
@Override
public String toString() {
return "TestChangeCreation{"
+ "host=" + host + ", "
+ "project=" + project + ", "
+ "branch=" + branch + ", "
+ "owner=" + owner + ", "
+ "author=" + author + ", "
+ "authorIdent=" + authorIdent + ", "
+ "committer=" + committer + ", "
+ "committerIdent=" + committerIdent + ", "
+ "topic=" + topic + ", "
+ "approvals=" + approvals + ", "
+ "commitMessage=" + commitMessage + ", "
+ "treeModifications=" + treeModifications + ", "
+ "parents=" + parents + ", "
+ "mergeStrategy=" + mergeStrategy + ", "
+ "changeCreator=" + changeCreator
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestChangeCreation) {
TestChangeCreation that = (TestChangeCreation) o;
return this.host.equals(that.host())
&& this.project.equals(that.project())
&& this.branch.equals(that.branch())
&& this.owner.equals(that.owner())
&& this.author.equals(that.author())
&& this.authorIdent.equals(that.authorIdent())
&& this.committer.equals(that.committer())
&& this.committerIdent.equals(that.committerIdent())
&& this.topic.equals(that.topic())
&& this.approvals.equals(that.approvals())
&& this.commitMessage.equals(that.commitMessage())
&& this.treeModifications.equals(that.treeModifications())
&& this.parents.equals(that.parents())
&& this.mergeStrategy.equals(that.mergeStrategy())
&& this.changeCreator.equals(that.changeCreator());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= host.hashCode();
h$ *= 1000003;
h$ ^= project.hashCode();
h$ *= 1000003;
h$ ^= branch.hashCode();
h$ *= 1000003;
h$ ^= owner.hashCode();
h$ *= 1000003;
h$ ^= author.hashCode();
h$ *= 1000003;
h$ ^= authorIdent.hashCode();
h$ *= 1000003;
h$ ^= committer.hashCode();
h$ *= 1000003;
h$ ^= committerIdent.hashCode();
h$ *= 1000003;
h$ ^= topic.hashCode();
h$ *= 1000003;
h$ ^= approvals.hashCode();
h$ *= 1000003;
h$ ^= commitMessage.hashCode();
h$ *= 1000003;
h$ ^= treeModifications.hashCode();
h$ *= 1000003;
h$ ^= parents.hashCode();
h$ *= 1000003;
h$ ^= mergeStrategy.hashCode();
h$ *= 1000003;
h$ ^= changeCreator.hashCode();
return h$;
}
static final class Builder extends TestChangeCreation.Builder {
private Optional host = Optional.empty();
private Optional project = Optional.empty();
private String branch;
private Optional owner = Optional.empty();
private Optional author = Optional.empty();
private Optional authorIdent = Optional.empty();
private Optional committer = Optional.empty();
private Optional committerIdent = Optional.empty();
private Optional topic = Optional.empty();
private ImmutableMap approvals;
private String commitMessage;
private ImmutableList.Builder treeModificationsBuilder$;
private ImmutableList treeModifications;
private Optional> parents = Optional.empty();
private MergeStrategy mergeStrategy;
private ThrowingFunction changeCreator;
Builder() {
}
@Override
public TestChangeCreation.Builder host(String host) {
this.host = Optional.of(host);
return this;
}
@Override
public TestChangeCreation.Builder project(Project.NameKey project) {
this.project = Optional.of(project);
return this;
}
@Override
public TestChangeCreation.Builder branch(String branch) {
if (branch == null) {
throw new NullPointerException("Null branch");
}
this.branch = branch;
return this;
}
@Override
public TestChangeCreation.Builder owner(Account.Id owner) {
this.owner = Optional.of(owner);
return this;
}
@Override
public TestChangeCreation.Builder author(Account.Id author) {
this.author = Optional.of(author);
return this;
}
@Override
public Optional author() {
return author;
}
@Override
public TestChangeCreation.Builder authorIdent(PersonIdent authorIdent) {
this.authorIdent = Optional.of(authorIdent);
return this;
}
@Override
public Optional authorIdent() {
return authorIdent;
}
@Override
public TestChangeCreation.Builder committer(Account.Id committer) {
this.committer = Optional.of(committer);
return this;
}
@Override
public Optional committer() {
return committer;
}
@Override
public TestChangeCreation.Builder committerIdent(PersonIdent committerIdent) {
this.committerIdent = Optional.of(committerIdent);
return this;
}
@Override
public Optional committerIdent() {
return committerIdent;
}
@Override
public TestChangeCreation.Builder topic(String topic) {
this.topic = Optional.of(topic);
return this;
}
@Override
public TestChangeCreation.Builder approvals(ImmutableMap approvals) {
if (approvals == null) {
throw new NullPointerException("Null approvals");
}
this.approvals = approvals;
return this;
}
@Override
public TestChangeCreation.Builder commitMessage(String commitMessage) {
if (commitMessage == null) {
throw new NullPointerException("Null commitMessage");
}
this.commitMessage = commitMessage;
return this;
}
@Override
ImmutableList.Builder treeModificationsBuilder() {
if (treeModificationsBuilder$ == null) {
treeModificationsBuilder$ = ImmutableList.builder();
}
return treeModificationsBuilder$;
}
@Override
TestChangeCreation.Builder parents(ImmutableList parents) {
this.parents = Optional.of(parents);
return this;
}
@Override
TestChangeCreation.Builder mergeStrategy(MergeStrategy mergeStrategy) {
if (mergeStrategy == null) {
throw new NullPointerException("Null mergeStrategy");
}
this.mergeStrategy = mergeStrategy;
return this;
}
@Override
TestChangeCreation.Builder changeCreator(ThrowingFunction changeCreator) {
if (changeCreator == null) {
throw new NullPointerException("Null changeCreator");
}
this.changeCreator = changeCreator;
return this;
}
@Override
TestChangeCreation autoBuild() {
if (treeModificationsBuilder$ != null) {
this.treeModifications = treeModificationsBuilder$.build();
} else if (this.treeModifications == null) {
this.treeModifications = ImmutableList.of();
}
if (this.branch == null
|| this.approvals == null
|| this.commitMessage == null
|| this.mergeStrategy == null
|| this.changeCreator == null) {
StringBuilder missing = new StringBuilder();
if (this.branch == null) {
missing.append(" branch");
}
if (this.approvals == null) {
missing.append(" approvals");
}
if (this.commitMessage == null) {
missing.append(" commitMessage");
}
if (this.mergeStrategy == null) {
missing.append(" mergeStrategy");
}
if (this.changeCreator == null) {
missing.append(" changeCreator");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestChangeCreation(
this.host,
this.project,
this.branch,
this.owner,
this.author,
this.authorIdent,
this.committer,
this.committerIdent,
this.topic,
this.approvals,
this.commitMessage,
this.treeModifications,
this.parents,
this.mergeStrategy,
this.changeCreator);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy