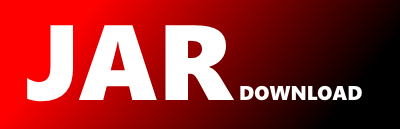
com.google.gerrit.acceptance.testsuite.change.AutoValue_TestPatchset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.change;
import com.google.gerrit.entities.PatchSet;
import javax.annotation.processing.Generated;
import org.eclipse.jgit.lib.ObjectId;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestPatchset extends TestPatchset {
private final PatchSet.Id patchsetId;
private final ObjectId commitId;
private AutoValue_TestPatchset(
PatchSet.Id patchsetId,
ObjectId commitId) {
this.patchsetId = patchsetId;
this.commitId = commitId;
}
@Override
public PatchSet.Id patchsetId() {
return patchsetId;
}
@Override
public ObjectId commitId() {
return commitId;
}
@Override
public String toString() {
return "TestPatchset{"
+ "patchsetId=" + patchsetId + ", "
+ "commitId=" + commitId
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestPatchset) {
TestPatchset that = (TestPatchset) o;
return this.patchsetId.equals(that.patchsetId())
&& this.commitId.equals(that.commitId());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= patchsetId.hashCode();
h$ *= 1000003;
h$ ^= commitId.hashCode();
return h$;
}
static final class Builder extends TestPatchset.Builder {
private PatchSet.Id patchsetId;
private ObjectId commitId;
Builder() {
}
@Override
TestPatchset.Builder patchsetId(PatchSet.Id patchsetId) {
if (patchsetId == null) {
throw new NullPointerException("Null patchsetId");
}
this.patchsetId = patchsetId;
return this;
}
@Override
TestPatchset.Builder commitId(ObjectId commitId) {
if (commitId == null) {
throw new NullPointerException("Null commitId");
}
this.commitId = commitId;
return this;
}
@Override
TestPatchset build() {
if (this.patchsetId == null
|| this.commitId == null) {
StringBuilder missing = new StringBuilder();
if (this.patchsetId == null) {
missing.append(" patchsetId");
}
if (this.commitId == null) {
missing.append(" commitId");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestPatchset(
this.patchsetId,
this.commitId);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy