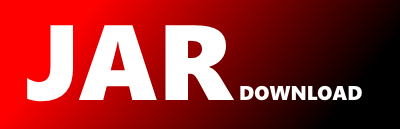
com.google.gerrit.acceptance.testsuite.change.AutoValue_TestPatchsetCreation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gerrit-acceptance-framework Show documentation
Show all versions of gerrit-acceptance-framework Show documentation
Framework for Gerrit's acceptance tests
package com.google.gerrit.acceptance.testsuite.change;
import com.google.common.collect.ImmutableList;
import com.google.gerrit.acceptance.testsuite.ThrowingFunction;
import com.google.gerrit.entities.Account;
import com.google.gerrit.entities.PatchSet;
import com.google.gerrit.server.edit.tree.TreeModification;
import java.util.Optional;
import javax.annotation.processing.Generated;
import org.eclipse.jgit.lib.PersonIdent;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TestPatchsetCreation extends TestPatchsetCreation {
private final Optional uploader;
private final Optional author;
private final Optional authorIdent;
private final Optional committer;
private final Optional committerIdent;
private final Optional commitMessage;
private final ImmutableList treeModifications;
private final Optional> parents;
private final ThrowingFunction patchsetCreator;
private AutoValue_TestPatchsetCreation(
Optional uploader,
Optional author,
Optional authorIdent,
Optional committer,
Optional committerIdent,
Optional commitMessage,
ImmutableList treeModifications,
Optional> parents,
ThrowingFunction patchsetCreator) {
this.uploader = uploader;
this.author = author;
this.authorIdent = authorIdent;
this.committer = committer;
this.committerIdent = committerIdent;
this.commitMessage = commitMessage;
this.treeModifications = treeModifications;
this.parents = parents;
this.patchsetCreator = patchsetCreator;
}
@Override
public Optional uploader() {
return uploader;
}
@Override
public Optional author() {
return author;
}
@Override
public Optional authorIdent() {
return authorIdent;
}
@Override
public Optional committer() {
return committer;
}
@Override
public Optional committerIdent() {
return committerIdent;
}
@Override
public Optional commitMessage() {
return commitMessage;
}
@Override
public ImmutableList treeModifications() {
return treeModifications;
}
@Override
public Optional> parents() {
return parents;
}
@Override
ThrowingFunction patchsetCreator() {
return patchsetCreator;
}
@Override
public String toString() {
return "TestPatchsetCreation{"
+ "uploader=" + uploader + ", "
+ "author=" + author + ", "
+ "authorIdent=" + authorIdent + ", "
+ "committer=" + committer + ", "
+ "committerIdent=" + committerIdent + ", "
+ "commitMessage=" + commitMessage + ", "
+ "treeModifications=" + treeModifications + ", "
+ "parents=" + parents + ", "
+ "patchsetCreator=" + patchsetCreator
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TestPatchsetCreation) {
TestPatchsetCreation that = (TestPatchsetCreation) o;
return this.uploader.equals(that.uploader())
&& this.author.equals(that.author())
&& this.authorIdent.equals(that.authorIdent())
&& this.committer.equals(that.committer())
&& this.committerIdent.equals(that.committerIdent())
&& this.commitMessage.equals(that.commitMessage())
&& this.treeModifications.equals(that.treeModifications())
&& this.parents.equals(that.parents())
&& this.patchsetCreator.equals(that.patchsetCreator());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= uploader.hashCode();
h$ *= 1000003;
h$ ^= author.hashCode();
h$ *= 1000003;
h$ ^= authorIdent.hashCode();
h$ *= 1000003;
h$ ^= committer.hashCode();
h$ *= 1000003;
h$ ^= committerIdent.hashCode();
h$ *= 1000003;
h$ ^= commitMessage.hashCode();
h$ *= 1000003;
h$ ^= treeModifications.hashCode();
h$ *= 1000003;
h$ ^= parents.hashCode();
h$ *= 1000003;
h$ ^= patchsetCreator.hashCode();
return h$;
}
static final class Builder extends TestPatchsetCreation.Builder {
private Optional uploader = Optional.empty();
private Optional author = Optional.empty();
private Optional authorIdent = Optional.empty();
private Optional committer = Optional.empty();
private Optional committerIdent = Optional.empty();
private Optional commitMessage = Optional.empty();
private ImmutableList.Builder treeModificationsBuilder$;
private ImmutableList treeModifications;
private Optional> parents = Optional.empty();
private ThrowingFunction patchsetCreator;
Builder() {
}
@Override
public TestPatchsetCreation.Builder uploader(Account.Id uploader) {
this.uploader = Optional.of(uploader);
return this;
}
@Override
public TestPatchsetCreation.Builder author(Account.Id author) {
this.author = Optional.of(author);
return this;
}
@Override
public Optional author() {
return author;
}
@Override
public TestPatchsetCreation.Builder authorIdent(PersonIdent authorIdent) {
this.authorIdent = Optional.of(authorIdent);
return this;
}
@Override
public Optional authorIdent() {
return authorIdent;
}
@Override
public TestPatchsetCreation.Builder committer(Account.Id committer) {
this.committer = Optional.of(committer);
return this;
}
@Override
public Optional committer() {
return committer;
}
@Override
public TestPatchsetCreation.Builder committerIdent(PersonIdent committerIdent) {
this.committerIdent = Optional.of(committerIdent);
return this;
}
@Override
public Optional committerIdent() {
return committerIdent;
}
@Override
public TestPatchsetCreation.Builder commitMessage(String commitMessage) {
this.commitMessage = Optional.of(commitMessage);
return this;
}
@Override
ImmutableList.Builder treeModificationsBuilder() {
if (treeModificationsBuilder$ == null) {
treeModificationsBuilder$ = ImmutableList.builder();
}
return treeModificationsBuilder$;
}
@Override
TestPatchsetCreation.Builder parents(ImmutableList parents) {
this.parents = Optional.of(parents);
return this;
}
@Override
TestPatchsetCreation.Builder patchsetCreator(ThrowingFunction patchsetCreator) {
if (patchsetCreator == null) {
throw new NullPointerException("Null patchsetCreator");
}
this.patchsetCreator = patchsetCreator;
return this;
}
@Override
TestPatchsetCreation autoBuild() {
if (treeModificationsBuilder$ != null) {
this.treeModifications = treeModificationsBuilder$.build();
} else if (this.treeModifications == null) {
this.treeModifications = ImmutableList.of();
}
if (this.patchsetCreator == null) {
String missing = " patchsetCreator";
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TestPatchsetCreation(
this.uploader,
this.author,
this.authorIdent,
this.committer,
this.committerIdent,
this.commitMessage,
this.treeModifications,
this.parents,
this.patchsetCreator);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy