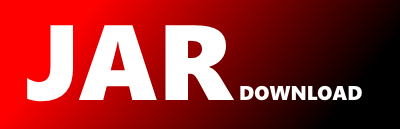
com.google.gerrit.server.index.account.AccountField Maven / Gradle / Ivy
// Copyright (C) 2016 The Android Open Source Project
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.google.gerrit.server.index.account;
import com.google.common.base.Function;
import com.google.common.base.Predicates;
import com.google.common.base.Strings;
import com.google.common.collect.FluentIterable;
import com.google.common.collect.Iterables;
import com.google.gerrit.reviewdb.client.AccountExternalId;
import com.google.gerrit.server.account.AccountState;
import com.google.gerrit.server.account.WatchConfig.ProjectWatchKey;
import com.google.gerrit.server.index.FieldDef;
import com.google.gerrit.server.index.FieldType;
import com.google.gerrit.server.index.SchemaUtil;
import java.sql.Timestamp;
import java.util.Collections;
import java.util.Set;
/** Secondary index schemas for accounts. */
public class AccountField {
public static final FieldDef ID =
new FieldDef.Single(
"id", FieldType.INTEGER, true) {
@Override
public Integer get(AccountState input, FillArgs args) {
return input.getAccount().getId().get();
}
};
public static final FieldDef> EXTERNAL_ID =
new FieldDef.Repeatable(
"external_id", FieldType.EXACT, false) {
@Override
public Iterable get(AccountState input, FillArgs args) {
return Iterables.transform(
input.getExternalIds(),
new Function() {
@Override
public String apply(AccountExternalId in) {
return in.getKey().get();
}
});
}
};
/** Fuzzy prefix match on name and email parts. */
public static final FieldDef> NAME_PART =
new FieldDef.Repeatable(
"name", FieldType.PREFIX, false) {
@Override
public Iterable get(AccountState input, FillArgs args) {
String fullName = input.getAccount().getFullName();
Set parts = SchemaUtil.getPersonParts(
fullName,
Iterables.transform(
input.getExternalIds(),
new Function() {
@Override
public String apply(AccountExternalId in) {
return in.getEmailAddress();
}
}));
// Additional values not currently added by getPersonParts.
// TODO(dborowitz): Move to getPersonParts and remove this hack.
if (fullName != null) {
parts.add(fullName.toLowerCase());
}
return parts;
}
};
public static final FieldDef FULL_NAME =
new FieldDef.Single("full_name", FieldType.EXACT,
false) {
@Override
public String get(AccountState input, FillArgs args) {
return input.getAccount().getFullName();
}
};
public static final FieldDef ACTIVE =
new FieldDef.Single(
"inactive", FieldType.EXACT, false) {
@Override
public String get(AccountState input, FillArgs args) {
return input.getAccount().isActive() ? "1" : "0";
}
};
public static final FieldDef> EMAIL =
new FieldDef.Repeatable(
"email", FieldType.PREFIX, false) {
@Override
public Iterable get(AccountState input, FillArgs args) {
return FluentIterable.from(input.getExternalIds())
.transform(
new Function() {
@Override
public String apply(AccountExternalId in) {
return in.getEmailAddress();
}
})
.append(
Collections.singleton(input.getAccount().getPreferredEmail()))
.filter(Predicates.notNull())
.transform(
new Function() {
@Override
public String apply(String in) {
return in.toLowerCase();
}
})
.toSet();
}
};
public static final FieldDef REGISTERED =
new FieldDef.Single(
"registered", FieldType.TIMESTAMP, false) {
@Override
public Timestamp get(AccountState input, FillArgs args) {
return input.getAccount().getRegisteredOn();
}
};
public static final FieldDef USERNAME =
new FieldDef.Single(
"username", FieldType.EXACT, false) {
@Override
public String get(AccountState input, FillArgs args) {
return Strings.nullToEmpty(input.getUserName()).toLowerCase();
}
};
public static final FieldDef> WATCHED_PROJECT =
new FieldDef.Repeatable(
"watchedproject", FieldType.EXACT, false) {
@Override
public Iterable get(AccountState input, FillArgs args) {
return FluentIterable.from(input.getProjectWatches().keySet())
.transform(new Function() {
@Override
public String apply(ProjectWatchKey in) {
return in.project().get();
}
}).toSet();
}
};
private AccountField() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy