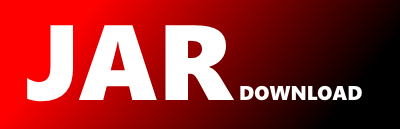
com.google.gerrit.server.mail.receive.AutoValue_MailMessage Maven / Gradle / Ivy
package com.google.gerrit.server.mail.receive;
import com.google.common.collect.ImmutableList;
import com.google.gerrit.common.Nullable;
import com.google.gerrit.server.mail.Address;
import javax.annotation.Generated;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_MailMessage extends MailMessage {
private final String id;
private final Address from;
private final ImmutableList to;
private final ImmutableList cc;
private final DateTime dateReceived;
private final ImmutableList additionalHeaders;
private final String subject;
private final String textContent;
private final String htmlContent;
private final ImmutableList rawContent;
private final String rawContentUTF;
private AutoValue_MailMessage(
String id,
Address from,
ImmutableList to,
ImmutableList cc,
DateTime dateReceived,
ImmutableList additionalHeaders,
String subject,
@Nullable String textContent,
@Nullable String htmlContent,
@Nullable ImmutableList rawContent,
@Nullable String rawContentUTF) {
this.id = id;
this.from = from;
this.to = to;
this.cc = cc;
this.dateReceived = dateReceived;
this.additionalHeaders = additionalHeaders;
this.subject = subject;
this.textContent = textContent;
this.htmlContent = htmlContent;
this.rawContent = rawContent;
this.rawContentUTF = rawContentUTF;
}
@Override
public String id() {
return id;
}
@Override
public Address from() {
return from;
}
@Override
public ImmutableList to() {
return to;
}
@Override
public ImmutableList cc() {
return cc;
}
@Override
public DateTime dateReceived() {
return dateReceived;
}
@Override
public ImmutableList additionalHeaders() {
return additionalHeaders;
}
@Override
public String subject() {
return subject;
}
@Nullable
@Override
public String textContent() {
return textContent;
}
@Nullable
@Override
public String htmlContent() {
return htmlContent;
}
@Nullable
@Override
public ImmutableList rawContent() {
return rawContent;
}
@Nullable
@Override
public String rawContentUTF() {
return rawContentUTF;
}
@Override
public String toString() {
return "MailMessage{"
+ "id=" + id + ", "
+ "from=" + from + ", "
+ "to=" + to + ", "
+ "cc=" + cc + ", "
+ "dateReceived=" + dateReceived + ", "
+ "additionalHeaders=" + additionalHeaders + ", "
+ "subject=" + subject + ", "
+ "textContent=" + textContent + ", "
+ "htmlContent=" + htmlContent + ", "
+ "rawContent=" + rawContent + ", "
+ "rawContentUTF=" + rawContentUTF
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof MailMessage) {
MailMessage that = (MailMessage) o;
return (this.id.equals(that.id()))
&& (this.from.equals(that.from()))
&& (this.to.equals(that.to()))
&& (this.cc.equals(that.cc()))
&& (this.dateReceived.equals(that.dateReceived()))
&& (this.additionalHeaders.equals(that.additionalHeaders()))
&& (this.subject.equals(that.subject()))
&& ((this.textContent == null) ? (that.textContent() == null) : this.textContent.equals(that.textContent()))
&& ((this.htmlContent == null) ? (that.htmlContent() == null) : this.htmlContent.equals(that.htmlContent()))
&& ((this.rawContent == null) ? (that.rawContent() == null) : this.rawContent.equals(that.rawContent()))
&& ((this.rawContentUTF == null) ? (that.rawContentUTF() == null) : this.rawContentUTF.equals(that.rawContentUTF()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= from.hashCode();
h$ *= 1000003;
h$ ^= to.hashCode();
h$ *= 1000003;
h$ ^= cc.hashCode();
h$ *= 1000003;
h$ ^= dateReceived.hashCode();
h$ *= 1000003;
h$ ^= additionalHeaders.hashCode();
h$ *= 1000003;
h$ ^= subject.hashCode();
h$ *= 1000003;
h$ ^= (textContent == null) ? 0 : textContent.hashCode();
h$ *= 1000003;
h$ ^= (htmlContent == null) ? 0 : htmlContent.hashCode();
h$ *= 1000003;
h$ ^= (rawContent == null) ? 0 : rawContent.hashCode();
h$ *= 1000003;
h$ ^= (rawContentUTF == null) ? 0 : rawContentUTF.hashCode();
return h$;
}
@Override
public MailMessage.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends MailMessage.Builder {
private String id;
private Address from;
private ImmutableList.Builder toBuilder$;
private ImmutableList to;
private ImmutableList.Builder ccBuilder$;
private ImmutableList cc;
private DateTime dateReceived;
private ImmutableList.Builder additionalHeadersBuilder$;
private ImmutableList additionalHeaders;
private String subject;
private String textContent;
private String htmlContent;
private ImmutableList rawContent;
private String rawContentUTF;
Builder() {
}
private Builder(MailMessage source) {
this.id = source.id();
this.from = source.from();
this.to = source.to();
this.cc = source.cc();
this.dateReceived = source.dateReceived();
this.additionalHeaders = source.additionalHeaders();
this.subject = source.subject();
this.textContent = source.textContent();
this.htmlContent = source.htmlContent();
this.rawContent = source.rawContent();
this.rawContentUTF = source.rawContentUTF();
}
@Override
public MailMessage.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public MailMessage.Builder from(Address from) {
if (from == null) {
throw new NullPointerException("Null from");
}
this.from = from;
return this;
}
@Override
public ImmutableList.Builder toBuilder() {
if (toBuilder$ == null) {
if (to == null) {
toBuilder$ = ImmutableList.builder();
} else {
toBuilder$ = ImmutableList.builder();
toBuilder$.addAll(to);
to = null;
}
}
return toBuilder$;
}
@Override
public ImmutableList.Builder ccBuilder() {
if (ccBuilder$ == null) {
if (cc == null) {
ccBuilder$ = ImmutableList.builder();
} else {
ccBuilder$ = ImmutableList.builder();
ccBuilder$.addAll(cc);
cc = null;
}
}
return ccBuilder$;
}
@Override
public MailMessage.Builder dateReceived(DateTime dateReceived) {
if (dateReceived == null) {
throw new NullPointerException("Null dateReceived");
}
this.dateReceived = dateReceived;
return this;
}
@Override
public ImmutableList.Builder additionalHeadersBuilder() {
if (additionalHeadersBuilder$ == null) {
if (additionalHeaders == null) {
additionalHeadersBuilder$ = ImmutableList.builder();
} else {
additionalHeadersBuilder$ = ImmutableList.builder();
additionalHeadersBuilder$.addAll(additionalHeaders);
additionalHeaders = null;
}
}
return additionalHeadersBuilder$;
}
@Override
public MailMessage.Builder subject(String subject) {
if (subject == null) {
throw new NullPointerException("Null subject");
}
this.subject = subject;
return this;
}
@Override
public MailMessage.Builder textContent(String textContent) {
this.textContent = textContent;
return this;
}
@Override
public MailMessage.Builder htmlContent(String htmlContent) {
this.htmlContent = htmlContent;
return this;
}
@Override
public MailMessage.Builder rawContent(ImmutableList rawContent) {
this.rawContent = rawContent;
return this;
}
@Override
public MailMessage.Builder rawContentUTF(String rawContentUTF) {
this.rawContentUTF = rawContentUTF;
return this;
}
@Override
public MailMessage build() {
if (toBuilder$ != null) {
this.to = toBuilder$.build();
} else if (this.to == null) {
this.to = ImmutableList.of();
}
if (ccBuilder$ != null) {
this.cc = ccBuilder$.build();
} else if (this.cc == null) {
this.cc = ImmutableList.of();
}
if (additionalHeadersBuilder$ != null) {
this.additionalHeaders = additionalHeadersBuilder$.build();
} else if (this.additionalHeaders == null) {
this.additionalHeaders = ImmutableList.of();
}
String missing = "";
if (this.id == null) {
missing += " id";
}
if (this.from == null) {
missing += " from";
}
if (this.dateReceived == null) {
missing += " dateReceived";
}
if (this.subject == null) {
missing += " subject";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_MailMessage(
this.id,
this.from,
this.to,
this.cc,
this.dateReceived,
this.additionalHeaders,
this.subject,
this.textContent,
this.htmlContent,
this.rawContent,
this.rawContentUTF);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy