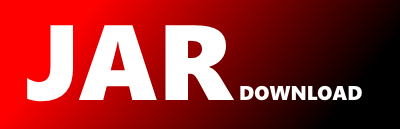
com.google.gerrit.server.account.AutoValue_Preferences_Edit Maven / Gradle / Ivy
package com.google.gerrit.server.account;
import com.google.gerrit.common.Nullable;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Preferences_Edit extends Preferences.Edit {
private final Optional tabSize;
private final Optional lineLength;
private final Optional indentUnit;
private final Optional cursorBlinkRate;
private final Optional hideTopMenu;
private final Optional showTabs;
private final Optional showWhitespaceErrors;
private final Optional syntaxHighlighting;
private final Optional hideLineNumbers;
private final Optional matchBrackets;
private final Optional lineWrapping;
private final Optional indentWithTabs;
private final Optional autoCloseBrackets;
private final Optional showBase;
private AutoValue_Preferences_Edit(
Optional tabSize,
Optional lineLength,
Optional indentUnit,
Optional cursorBlinkRate,
Optional hideTopMenu,
Optional showTabs,
Optional showWhitespaceErrors,
Optional syntaxHighlighting,
Optional hideLineNumbers,
Optional matchBrackets,
Optional lineWrapping,
Optional indentWithTabs,
Optional autoCloseBrackets,
Optional showBase) {
this.tabSize = tabSize;
this.lineLength = lineLength;
this.indentUnit = indentUnit;
this.cursorBlinkRate = cursorBlinkRate;
this.hideTopMenu = hideTopMenu;
this.showTabs = showTabs;
this.showWhitespaceErrors = showWhitespaceErrors;
this.syntaxHighlighting = syntaxHighlighting;
this.hideLineNumbers = hideLineNumbers;
this.matchBrackets = matchBrackets;
this.lineWrapping = lineWrapping;
this.indentWithTabs = indentWithTabs;
this.autoCloseBrackets = autoCloseBrackets;
this.showBase = showBase;
}
@Override
public Optional tabSize() {
return tabSize;
}
@Override
public Optional lineLength() {
return lineLength;
}
@Override
public Optional indentUnit() {
return indentUnit;
}
@Override
public Optional cursorBlinkRate() {
return cursorBlinkRate;
}
@Override
public Optional hideTopMenu() {
return hideTopMenu;
}
@Override
public Optional showTabs() {
return showTabs;
}
@Override
public Optional showWhitespaceErrors() {
return showWhitespaceErrors;
}
@Override
public Optional syntaxHighlighting() {
return syntaxHighlighting;
}
@Override
public Optional hideLineNumbers() {
return hideLineNumbers;
}
@Override
public Optional matchBrackets() {
return matchBrackets;
}
@Override
public Optional lineWrapping() {
return lineWrapping;
}
@Override
public Optional indentWithTabs() {
return indentWithTabs;
}
@Override
public Optional autoCloseBrackets() {
return autoCloseBrackets;
}
@Override
public Optional showBase() {
return showBase;
}
@Override
public String toString() {
return "Edit{"
+ "tabSize=" + tabSize + ", "
+ "lineLength=" + lineLength + ", "
+ "indentUnit=" + indentUnit + ", "
+ "cursorBlinkRate=" + cursorBlinkRate + ", "
+ "hideTopMenu=" + hideTopMenu + ", "
+ "showTabs=" + showTabs + ", "
+ "showWhitespaceErrors=" + showWhitespaceErrors + ", "
+ "syntaxHighlighting=" + syntaxHighlighting + ", "
+ "hideLineNumbers=" + hideLineNumbers + ", "
+ "matchBrackets=" + matchBrackets + ", "
+ "lineWrapping=" + lineWrapping + ", "
+ "indentWithTabs=" + indentWithTabs + ", "
+ "autoCloseBrackets=" + autoCloseBrackets + ", "
+ "showBase=" + showBase
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Preferences.Edit) {
Preferences.Edit that = (Preferences.Edit) o;
return this.tabSize.equals(that.tabSize())
&& this.lineLength.equals(that.lineLength())
&& this.indentUnit.equals(that.indentUnit())
&& this.cursorBlinkRate.equals(that.cursorBlinkRate())
&& this.hideTopMenu.equals(that.hideTopMenu())
&& this.showTabs.equals(that.showTabs())
&& this.showWhitespaceErrors.equals(that.showWhitespaceErrors())
&& this.syntaxHighlighting.equals(that.syntaxHighlighting())
&& this.hideLineNumbers.equals(that.hideLineNumbers())
&& this.matchBrackets.equals(that.matchBrackets())
&& this.lineWrapping.equals(that.lineWrapping())
&& this.indentWithTabs.equals(that.indentWithTabs())
&& this.autoCloseBrackets.equals(that.autoCloseBrackets())
&& this.showBase.equals(that.showBase());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= tabSize.hashCode();
h$ *= 1000003;
h$ ^= lineLength.hashCode();
h$ *= 1000003;
h$ ^= indentUnit.hashCode();
h$ *= 1000003;
h$ ^= cursorBlinkRate.hashCode();
h$ *= 1000003;
h$ ^= hideTopMenu.hashCode();
h$ *= 1000003;
h$ ^= showTabs.hashCode();
h$ *= 1000003;
h$ ^= showWhitespaceErrors.hashCode();
h$ *= 1000003;
h$ ^= syntaxHighlighting.hashCode();
h$ *= 1000003;
h$ ^= hideLineNumbers.hashCode();
h$ *= 1000003;
h$ ^= matchBrackets.hashCode();
h$ *= 1000003;
h$ ^= lineWrapping.hashCode();
h$ *= 1000003;
h$ ^= indentWithTabs.hashCode();
h$ *= 1000003;
h$ ^= autoCloseBrackets.hashCode();
h$ *= 1000003;
h$ ^= showBase.hashCode();
return h$;
}
static final class Builder extends Preferences.Edit.Builder {
private Optional tabSize = Optional.empty();
private Optional lineLength = Optional.empty();
private Optional indentUnit = Optional.empty();
private Optional cursorBlinkRate = Optional.empty();
private Optional hideTopMenu = Optional.empty();
private Optional showTabs = Optional.empty();
private Optional showWhitespaceErrors = Optional.empty();
private Optional syntaxHighlighting = Optional.empty();
private Optional hideLineNumbers = Optional.empty();
private Optional matchBrackets = Optional.empty();
private Optional lineWrapping = Optional.empty();
private Optional indentWithTabs = Optional.empty();
private Optional autoCloseBrackets = Optional.empty();
private Optional showBase = Optional.empty();
Builder() {
}
@Override
Preferences.Edit.Builder tabSize(@Nullable Integer tabSize) {
this.tabSize = Optional.ofNullable(tabSize);
return this;
}
@Override
Preferences.Edit.Builder lineLength(@Nullable Integer lineLength) {
this.lineLength = Optional.ofNullable(lineLength);
return this;
}
@Override
Preferences.Edit.Builder indentUnit(@Nullable Integer indentUnit) {
this.indentUnit = Optional.ofNullable(indentUnit);
return this;
}
@Override
Preferences.Edit.Builder cursorBlinkRate(@Nullable Integer cursorBlinkRate) {
this.cursorBlinkRate = Optional.ofNullable(cursorBlinkRate);
return this;
}
@Override
Preferences.Edit.Builder hideTopMenu(@Nullable Boolean hideTopMenu) {
this.hideTopMenu = Optional.ofNullable(hideTopMenu);
return this;
}
@Override
Preferences.Edit.Builder showTabs(@Nullable Boolean showTabs) {
this.showTabs = Optional.ofNullable(showTabs);
return this;
}
@Override
Preferences.Edit.Builder showWhitespaceErrors(@Nullable Boolean showWhitespaceErrors) {
this.showWhitespaceErrors = Optional.ofNullable(showWhitespaceErrors);
return this;
}
@Override
Preferences.Edit.Builder syntaxHighlighting(@Nullable Boolean syntaxHighlighting) {
this.syntaxHighlighting = Optional.ofNullable(syntaxHighlighting);
return this;
}
@Override
Preferences.Edit.Builder hideLineNumbers(@Nullable Boolean hideLineNumbers) {
this.hideLineNumbers = Optional.ofNullable(hideLineNumbers);
return this;
}
@Override
Preferences.Edit.Builder matchBrackets(@Nullable Boolean matchBrackets) {
this.matchBrackets = Optional.ofNullable(matchBrackets);
return this;
}
@Override
Preferences.Edit.Builder lineWrapping(@Nullable Boolean lineWrapping) {
this.lineWrapping = Optional.ofNullable(lineWrapping);
return this;
}
@Override
Preferences.Edit.Builder indentWithTabs(@Nullable Boolean indentWithTabs) {
this.indentWithTabs = Optional.ofNullable(indentWithTabs);
return this;
}
@Override
Preferences.Edit.Builder autoCloseBrackets(@Nullable Boolean autoCloseBrackets) {
this.autoCloseBrackets = Optional.ofNullable(autoCloseBrackets);
return this;
}
@Override
Preferences.Edit.Builder showBase(@Nullable Boolean showBase) {
this.showBase = Optional.ofNullable(showBase);
return this;
}
@Override
Preferences.Edit build() {
return new AutoValue_Preferences_Edit(
this.tabSize,
this.lineLength,
this.indentUnit,
this.cursorBlinkRate,
this.hideTopMenu,
this.showTabs,
this.showWhitespaceErrors,
this.syntaxHighlighting,
this.hideLineNumbers,
this.matchBrackets,
this.lineWrapping,
this.indentWithTabs,
this.autoCloseBrackets,
this.showBase);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy