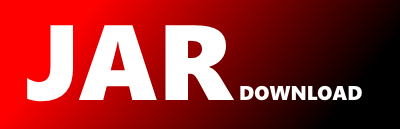
com.google.maps.routeoptimization.v1.ShipmentRoute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-maps-routeoptimization-v1 Show documentation
Show all versions of proto-google-maps-routeoptimization-v1 Show documentation
Proto library for google-maps-routeoptimization
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/maps/routeoptimization/v1/route_optimization_service.proto
// Protobuf Java Version: 3.25.5
package com.google.maps.routeoptimization.v1;
/**
*
*
*
* A vehicle's route can be decomposed, along the time axis, like this (we
* assume there are n visits):
* ```
* | | | | | T[2], | | |
* | Transition | Visit #0 | | | V[2], | | |
* | #0 | aka | T[1] | V[1] | ... | V[n-1] | T[n] |
* | aka T[0] | V[0] | | | V[n-2],| | |
* | | | | | T[n-1] | | |
* ^ ^ ^ ^ ^ ^ ^ ^
* vehicle V[0].start V[0].end V[1]. V[1]. V[n]. V[n]. vehicle
* start (arrival) (departure) start end start end end
* ```
* Note that we make a difference between:
*
* * "punctual events", such as the vehicle start and end and each visit's start
* and end (aka arrival and departure). They happen at a given second.
* * "time intervals", such as the visits themselves, and the transition between
* visits. Though time intervals can sometimes have zero duration, i.e. start
* and end at the same second, they often have a positive duration.
*
* Invariants:
*
* * If there are n visits, there are n+1 transitions.
* * A visit is always surrounded by a transition before it (same index) and a
* transition after it (index + 1).
* * The vehicle start is always followed by transition #0.
* * The vehicle end is always preceded by transition #n.
*
* Zooming in, here is what happens during a `Transition` and a `Visit`:
* ```
* ---+-------------------------------------+-----------------------------+-->
* | TRANSITION[i] | VISIT[i] |
* | | |
* | * TRAVEL: the vehicle moves from | PERFORM the visit: |
* | VISIT[i-1].departure_location to | |
* | VISIT[i].arrival_location, which | * Spend some time: |
* | takes a given travel duration | the "visit duration". |
* | and distance | |
* | | * Load or unload |
* | * BREAKS: the driver may have | some quantities from the |
* | breaks (e.g. lunch break). | vehicle: the "demand". |
* | | |
* | * WAIT: the driver/vehicle does | |
* | nothing. This can happen for | |
* | many reasons, for example when | |
* | the vehicle reaches the next | |
* | event's destination before the | |
* | start of its time window | |
* | | |
* | * DELAY: *right before* the next | |
* | arrival. E.g. the vehicle and/or | |
* | driver spends time unloading. | |
* | | |
* ---+-------------------------------------+-----------------------------+-->
* ^ ^ ^
* V[i-1].end V[i].start V[i].end
* ```
* Lastly, here is how the TRAVEL, BREAKS, DELAY and WAIT can be arranged
* during a transition.
*
* * They don't overlap.
* * The DELAY is unique and *must* be a contiguous period of time right
* before the next visit (or vehicle end). Thus, it suffice to know the
* delay duration to know its start and end time.
* * The BREAKS are contiguous, non-overlapping periods of time. The
* response specifies the start time and duration of each break.
* * TRAVEL and WAIT are "preemptable": they can be interrupted several times
* during this transition. Clients can assume that travel happens "as soon as
* possible" and that "wait" fills the remaining time.
*
* A (complex) example:
* ```
* TRANSITION[i]
* --++-----+-----------------------------------------------------------++-->
* || | | | | | | ||
* || T | B | T | | B | | D ||
* || r | r | r | W | r | W | e ||
* || a | e | a | a | e | a | l ||
* || v | a | v | i | a | i | a ||
* || e | k | e | t | k | t | y ||
* || l | | l | | | | ||
* || | | | | | | ||
* --++-----------------------------------------------------------------++-->
* ```
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute}
*/
public final class ShipmentRoute extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.ShipmentRoute)
ShipmentRouteOrBuilder {
private static final long serialVersionUID = 0L;
// Use ShipmentRoute.newBuilder() to construct.
private ShipmentRoute(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ShipmentRoute() {
vehicleLabel_ = "";
visits_ = java.util.Collections.emptyList();
transitions_ = java.util.Collections.emptyList();
breaks_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new ShipmentRoute();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 17:
return internalGetRouteCosts();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Builder.class);
}
public interface VisitOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.ShipmentRoute.Visit)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Index of the `shipments` field in the source
* [ShipmentModel][google.maps.routeoptimization.v1.ShipmentModel].
*
*
* int32 shipment_index = 1;
*
* @return The shipmentIndex.
*/
int getShipmentIndex();
/**
*
*
*
* If true the visit corresponds to a pickup of a `Shipment`. Otherwise, it
* corresponds to a delivery.
*
*
* bool is_pickup = 2;
*
* @return The isPickup.
*/
boolean getIsPickup();
/**
*
*
*
* Index of `VisitRequest` in either the pickup or delivery field of the
* `Shipment` (see `is_pickup`).
*
*
* int32 visit_request_index = 3;
*
* @return The visitRequestIndex.
*/
int getVisitRequestIndex();
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*
* @return Whether the startTime field is set.
*/
boolean hasStartTime();
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*
* @return The startTime.
*/
com.google.protobuf.Timestamp getStartTime();
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder();
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
int getLoadDemandsCount();
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
boolean containsLoadDemands(java.lang.String key);
/** Use {@link #getLoadDemandsMap()} instead. */
@java.lang.Deprecated
java.util.Map
getLoadDemands();
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
java.util.Map
getLoadDemandsMap();
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
/* nullable */
com.google.maps.routeoptimization.v1.Shipment.Load getLoadDemandsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.Shipment.Load defaultValue);
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
com.google.maps.routeoptimization.v1.Shipment.Load getLoadDemandsOrThrow(java.lang.String key);
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*
* @return Whether the detour field is set.
*/
boolean hasDetour();
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*
* @return The detour.
*/
com.google.protobuf.Duration getDetour();
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
com.google.protobuf.DurationOrBuilder getDetourOrBuilder();
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @return The shipmentLabel.
*/
java.lang.String getShipmentLabel();
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @return The bytes for shipmentLabel.
*/
com.google.protobuf.ByteString getShipmentLabelBytes();
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @return The visitLabel.
*/
java.lang.String getVisitLabel();
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @return The bytes for visitLabel.
*/
com.google.protobuf.ByteString getVisitLabelBytes();
}
/**
*
*
*
* A visit performed during a route. This visit corresponds to a pickup or a
* delivery of a `Shipment`.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.Visit}
*/
public static final class Visit extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.ShipmentRoute.Visit)
VisitOrBuilder {
private static final long serialVersionUID = 0L;
// Use Visit.newBuilder() to construct.
private Visit(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Visit() {
shipmentLabel_ = "";
visitLabel_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Visit();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Visit_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 11:
return internalGetLoadDemands();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Visit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder.class);
}
private int bitField0_;
public static final int SHIPMENT_INDEX_FIELD_NUMBER = 1;
private int shipmentIndex_ = 0;
/**
*
*
*
* Index of the `shipments` field in the source
* [ShipmentModel][google.maps.routeoptimization.v1.ShipmentModel].
*
*
* int32 shipment_index = 1;
*
* @return The shipmentIndex.
*/
@java.lang.Override
public int getShipmentIndex() {
return shipmentIndex_;
}
public static final int IS_PICKUP_FIELD_NUMBER = 2;
private boolean isPickup_ = false;
/**
*
*
*
* If true the visit corresponds to a pickup of a `Shipment`. Otherwise, it
* corresponds to a delivery.
*
*
* bool is_pickup = 2;
*
* @return The isPickup.
*/
@java.lang.Override
public boolean getIsPickup() {
return isPickup_;
}
public static final int VISIT_REQUEST_INDEX_FIELD_NUMBER = 3;
private int visitRequestIndex_ = 0;
/**
*
*
*
* Index of `VisitRequest` in either the pickup or delivery field of the
* `Shipment` (see `is_pickup`).
*
*
* int32 visit_request_index = 3;
*
* @return The visitRequestIndex.
*/
@java.lang.Override
public int getVisitRequestIndex() {
return visitRequestIndex_;
}
public static final int START_TIME_FIELD_NUMBER = 4;
private com.google.protobuf.Timestamp startTime_;
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*
* @return Whether the startTime field is set.
*/
@java.lang.Override
public boolean hasStartTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*
* @return The startTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getStartTime() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
public static final int LOAD_DEMANDS_FIELD_NUMBER = 11;
private static final class LoadDemandsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Shipment.Load>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Visit_LoadDemandsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.maps.routeoptimization.v1.Shipment.Load.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.maps.routeoptimization.v1.Shipment.Load>
loadDemands_;
private com.google.protobuf.MapField<
java.lang.String, com.google.maps.routeoptimization.v1.Shipment.Load>
internalGetLoadDemands() {
if (loadDemands_ == null) {
return com.google.protobuf.MapField.emptyMapField(
LoadDemandsDefaultEntryHolder.defaultEntry);
}
return loadDemands_;
}
public int getLoadDemandsCount() {
return internalGetLoadDemands().getMap().size();
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public boolean containsLoadDemands(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLoadDemands().getMap().containsKey(key);
}
/** Use {@link #getLoadDemandsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getLoadDemands() {
return getLoadDemandsMap();
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public java.util.Map
getLoadDemandsMap() {
return internalGetLoadDemands().getMap();
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public /* nullable */ com.google.maps.routeoptimization.v1.Shipment.Load
getLoadDemandsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.Shipment.Load defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetLoadDemands().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Shipment.Load getLoadDemandsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetLoadDemands().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int DETOUR_FIELD_NUMBER = 6;
private com.google.protobuf.Duration detour_;
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*
* @return Whether the detour field is set.
*/
@java.lang.Override
public boolean hasDetour() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*
* @return The detour.
*/
@java.lang.Override
public com.google.protobuf.Duration getDetour() {
return detour_ == null ? com.google.protobuf.Duration.getDefaultInstance() : detour_;
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getDetourOrBuilder() {
return detour_ == null ? com.google.protobuf.Duration.getDefaultInstance() : detour_;
}
public static final int SHIPMENT_LABEL_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object shipmentLabel_ = "";
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @return The shipmentLabel.
*/
@java.lang.Override
public java.lang.String getShipmentLabel() {
java.lang.Object ref = shipmentLabel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
shipmentLabel_ = s;
return s;
}
}
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @return The bytes for shipmentLabel.
*/
@java.lang.Override
public com.google.protobuf.ByteString getShipmentLabelBytes() {
java.lang.Object ref = shipmentLabel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
shipmentLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VISIT_LABEL_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object visitLabel_ = "";
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @return The visitLabel.
*/
@java.lang.Override
public java.lang.String getVisitLabel() {
java.lang.Object ref = visitLabel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
visitLabel_ = s;
return s;
}
}
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @return The bytes for visitLabel.
*/
@java.lang.Override
public com.google.protobuf.ByteString getVisitLabelBytes() {
java.lang.Object ref = visitLabel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
visitLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (shipmentIndex_ != 0) {
output.writeInt32(1, shipmentIndex_);
}
if (isPickup_ != false) {
output.writeBool(2, isPickup_);
}
if (visitRequestIndex_ != 0) {
output.writeInt32(3, visitRequestIndex_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(4, getStartTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(6, getDetour());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(shipmentLabel_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, shipmentLabel_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(visitLabel_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, visitLabel_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetLoadDemands(), LoadDemandsDefaultEntryHolder.defaultEntry, 11);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (shipmentIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(1, shipmentIndex_);
}
if (isPickup_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(2, isPickup_);
}
if (visitRequestIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(3, visitRequestIndex_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getStartTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getDetour());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(shipmentLabel_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, shipmentLabel_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(visitLabel_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, visitLabel_);
}
for (java.util.Map.Entry
entry : internalGetLoadDemands().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Shipment.Load>
loadDemands__ =
LoadDemandsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(11, loadDemands__);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.Visit)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit other =
(com.google.maps.routeoptimization.v1.ShipmentRoute.Visit) obj;
if (getShipmentIndex() != other.getShipmentIndex()) return false;
if (getIsPickup() != other.getIsPickup()) return false;
if (getVisitRequestIndex() != other.getVisitRequestIndex()) return false;
if (hasStartTime() != other.hasStartTime()) return false;
if (hasStartTime()) {
if (!getStartTime().equals(other.getStartTime())) return false;
}
if (!internalGetLoadDemands().equals(other.internalGetLoadDemands())) return false;
if (hasDetour() != other.hasDetour()) return false;
if (hasDetour()) {
if (!getDetour().equals(other.getDetour())) return false;
}
if (!getShipmentLabel().equals(other.getShipmentLabel())) return false;
if (!getVisitLabel().equals(other.getVisitLabel())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SHIPMENT_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getShipmentIndex();
hash = (37 * hash) + IS_PICKUP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getIsPickup());
hash = (37 * hash) + VISIT_REQUEST_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getVisitRequestIndex();
if (hasStartTime()) {
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + getStartTime().hashCode();
}
if (!internalGetLoadDemands().getMap().isEmpty()) {
hash = (37 * hash) + LOAD_DEMANDS_FIELD_NUMBER;
hash = (53 * hash) + internalGetLoadDemands().hashCode();
}
if (hasDetour()) {
hash = (37 * hash) + DETOUR_FIELD_NUMBER;
hash = (53 * hash) + getDetour().hashCode();
}
hash = (37 * hash) + SHIPMENT_LABEL_FIELD_NUMBER;
hash = (53 * hash) + getShipmentLabel().hashCode();
hash = (37 * hash) + VISIT_LABEL_FIELD_NUMBER;
hash = (53 * hash) + getVisitLabel().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A visit performed during a route. This visit corresponds to a pickup or a
* delivery of a `Shipment`.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.Visit}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.ShipmentRoute.Visit)
com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Visit_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 11:
return internalGetLoadDemands();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 11:
return internalGetMutableLoadDemands();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Visit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getStartTimeFieldBuilder();
getDetourFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
shipmentIndex_ = 0;
isPickup_ = false;
visitRequestIndex_ = 0;
startTime_ = null;
if (startTimeBuilder_ != null) {
startTimeBuilder_.dispose();
startTimeBuilder_ = null;
}
internalGetMutableLoadDemands().clear();
detour_ = null;
if (detourBuilder_ != null) {
detourBuilder_.dispose();
detourBuilder_ = null;
}
shipmentLabel_ = "";
visitLabel_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Visit_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit build() {
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit buildPartial() {
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit result =
new com.google.maps.routeoptimization.v1.ShipmentRoute.Visit(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.maps.routeoptimization.v1.ShipmentRoute.Visit result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.shipmentIndex_ = shipmentIndex_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.isPickup_ = isPickup_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.visitRequestIndex_ = visitRequestIndex_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.startTime_ = startTimeBuilder_ == null ? startTime_ : startTimeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.loadDemands_ =
internalGetLoadDemands().build(LoadDemandsDefaultEntryHolder.defaultEntry);
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.detour_ = detourBuilder_ == null ? detour_ : detourBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.shipmentLabel_ = shipmentLabel_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.visitLabel_ = visitLabel_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.Visit) {
return mergeFrom((com.google.maps.routeoptimization.v1.ShipmentRoute.Visit) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.maps.routeoptimization.v1.ShipmentRoute.Visit other) {
if (other == com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.getDefaultInstance())
return this;
if (other.getShipmentIndex() != 0) {
setShipmentIndex(other.getShipmentIndex());
}
if (other.getIsPickup() != false) {
setIsPickup(other.getIsPickup());
}
if (other.getVisitRequestIndex() != 0) {
setVisitRequestIndex(other.getVisitRequestIndex());
}
if (other.hasStartTime()) {
mergeStartTime(other.getStartTime());
}
internalGetMutableLoadDemands().mergeFrom(other.internalGetLoadDemands());
bitField0_ |= 0x00000010;
if (other.hasDetour()) {
mergeDetour(other.getDetour());
}
if (!other.getShipmentLabel().isEmpty()) {
shipmentLabel_ = other.shipmentLabel_;
bitField0_ |= 0x00000040;
onChanged();
}
if (!other.getVisitLabel().isEmpty()) {
visitLabel_ = other.visitLabel_;
bitField0_ |= 0x00000080;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
shipmentIndex_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16:
{
isPickup_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24:
{
visitRequestIndex_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34:
{
input.readMessage(getStartTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 50:
{
input.readMessage(getDetourFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 58:
{
shipmentLabel_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 58
case 66:
{
visitLabel_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 66
case 90:
{
com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Shipment.Load>
loadDemands__ =
input.readMessage(
LoadDemandsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableLoadDemands()
.ensureBuilderMap()
.put(loadDemands__.getKey(), loadDemands__.getValue());
bitField0_ |= 0x00000010;
break;
} // case 90
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int shipmentIndex_;
/**
*
*
*
* Index of the `shipments` field in the source
* [ShipmentModel][google.maps.routeoptimization.v1.ShipmentModel].
*
*
* int32 shipment_index = 1;
*
* @return The shipmentIndex.
*/
@java.lang.Override
public int getShipmentIndex() {
return shipmentIndex_;
}
/**
*
*
*
* Index of the `shipments` field in the source
* [ShipmentModel][google.maps.routeoptimization.v1.ShipmentModel].
*
*
* int32 shipment_index = 1;
*
* @param value The shipmentIndex to set.
* @return This builder for chaining.
*/
public Builder setShipmentIndex(int value) {
shipmentIndex_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Index of the `shipments` field in the source
* [ShipmentModel][google.maps.routeoptimization.v1.ShipmentModel].
*
*
* int32 shipment_index = 1;
*
* @return This builder for chaining.
*/
public Builder clearShipmentIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
shipmentIndex_ = 0;
onChanged();
return this;
}
private boolean isPickup_;
/**
*
*
*
* If true the visit corresponds to a pickup of a `Shipment`. Otherwise, it
* corresponds to a delivery.
*
*
* bool is_pickup = 2;
*
* @return The isPickup.
*/
@java.lang.Override
public boolean getIsPickup() {
return isPickup_;
}
/**
*
*
*
* If true the visit corresponds to a pickup of a `Shipment`. Otherwise, it
* corresponds to a delivery.
*
*
* bool is_pickup = 2;
*
* @param value The isPickup to set.
* @return This builder for chaining.
*/
public Builder setIsPickup(boolean value) {
isPickup_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* If true the visit corresponds to a pickup of a `Shipment`. Otherwise, it
* corresponds to a delivery.
*
*
* bool is_pickup = 2;
*
* @return This builder for chaining.
*/
public Builder clearIsPickup() {
bitField0_ = (bitField0_ & ~0x00000002);
isPickup_ = false;
onChanged();
return this;
}
private int visitRequestIndex_;
/**
*
*
*
* Index of `VisitRequest` in either the pickup or delivery field of the
* `Shipment` (see `is_pickup`).
*
*
* int32 visit_request_index = 3;
*
* @return The visitRequestIndex.
*/
@java.lang.Override
public int getVisitRequestIndex() {
return visitRequestIndex_;
}
/**
*
*
*
* Index of `VisitRequest` in either the pickup or delivery field of the
* `Shipment` (see `is_pickup`).
*
*
* int32 visit_request_index = 3;
*
* @param value The visitRequestIndex to set.
* @return This builder for chaining.
*/
public Builder setVisitRequestIndex(int value) {
visitRequestIndex_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Index of `VisitRequest` in either the pickup or delivery field of the
* `Shipment` (see `is_pickup`).
*
*
* int32 visit_request_index = 3;
*
* @return This builder for chaining.
*/
public Builder clearVisitRequestIndex() {
bitField0_ = (bitField0_ & ~0x00000004);
visitRequestIndex_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp startTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
startTimeBuilder_;
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*
* @return Whether the startTime field is set.
*/
public boolean hasStartTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*
* @return The startTime.
*/
public com.google.protobuf.Timestamp getStartTime() {
if (startTimeBuilder_ == null) {
return startTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: startTime_;
} else {
return startTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
public Builder setStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startTime_ = value;
} else {
startTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
public Builder setStartTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (startTimeBuilder_ == null) {
startTime_ = builderForValue.build();
} else {
startTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
public Builder mergeStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& startTime_ != null
&& startTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getStartTimeBuilder().mergeFrom(value);
} else {
startTime_ = value;
}
} else {
startTimeBuilder_.mergeFrom(value);
}
if (startTime_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
public Builder clearStartTime() {
bitField0_ = (bitField0_ & ~0x00000008);
startTime_ = null;
if (startTimeBuilder_ != null) {
startTimeBuilder_.dispose();
startTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
public com.google.protobuf.Timestamp.Builder getStartTimeBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getStartTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
if (startTimeBuilder_ != null) {
return startTimeBuilder_.getMessageOrBuilder();
} else {
return startTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: startTime_;
}
}
/**
*
*
*
* Time at which the visit starts. Note that the vehicle may arrive earlier
* than this at the visit location. Times are consistent with the
* `ShipmentModel`.
*
*
* .google.protobuf.Timestamp start_time = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getStartTimeFieldBuilder() {
if (startTimeBuilder_ == null) {
startTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getStartTime(), getParentForChildren(), isClean());
startTime_ = null;
}
return startTimeBuilder_;
}
private static final class LoadDemandsConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.maps.routeoptimization.v1.Shipment.LoadOrBuilder,
com.google.maps.routeoptimization.v1.Shipment.Load> {
@java.lang.Override
public com.google.maps.routeoptimization.v1.Shipment.Load build(
com.google.maps.routeoptimization.v1.Shipment.LoadOrBuilder val) {
if (val instanceof com.google.maps.routeoptimization.v1.Shipment.Load) {
return (com.google.maps.routeoptimization.v1.Shipment.Load) val;
}
return ((com.google.maps.routeoptimization.v1.Shipment.Load.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Shipment.Load>
defaultEntry() {
return LoadDemandsDefaultEntryHolder.defaultEntry;
}
};
private static final LoadDemandsConverter loadDemandsConverter = new LoadDemandsConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.Shipment.LoadOrBuilder,
com.google.maps.routeoptimization.v1.Shipment.Load,
com.google.maps.routeoptimization.v1.Shipment.Load.Builder>
loadDemands_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.Shipment.LoadOrBuilder,
com.google.maps.routeoptimization.v1.Shipment.Load,
com.google.maps.routeoptimization.v1.Shipment.Load.Builder>
internalGetLoadDemands() {
if (loadDemands_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(loadDemandsConverter);
}
return loadDemands_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.Shipment.LoadOrBuilder,
com.google.maps.routeoptimization.v1.Shipment.Load,
com.google.maps.routeoptimization.v1.Shipment.Load.Builder>
internalGetMutableLoadDemands() {
if (loadDemands_ == null) {
loadDemands_ = new com.google.protobuf.MapFieldBuilder<>(loadDemandsConverter);
}
bitField0_ |= 0x00000010;
onChanged();
return loadDemands_;
}
public int getLoadDemandsCount() {
return internalGetLoadDemands().ensureBuilderMap().size();
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public boolean containsLoadDemands(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLoadDemands().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getLoadDemandsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getLoadDemands() {
return getLoadDemandsMap();
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public java.util.Map
getLoadDemandsMap() {
return internalGetLoadDemands().getImmutableMap();
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public /* nullable */ com.google.maps.routeoptimization.v1.Shipment.Load
getLoadDemandsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.Shipment.Load defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableLoadDemands().ensureBuilderMap();
return map.containsKey(key) ? loadDemandsConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Shipment.Load getLoadDemandsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableLoadDemands().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return loadDemandsConverter.build(map.get(key));
}
public Builder clearLoadDemands() {
bitField0_ = (bitField0_ & ~0x00000010);
internalGetMutableLoadDemands().clear();
return this;
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
public Builder removeLoadDemands(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableLoadDemands().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map
getMutableLoadDemands() {
bitField0_ |= 0x00000010;
return internalGetMutableLoadDemands().ensureMessageMap();
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
public Builder putLoadDemands(
java.lang.String key, com.google.maps.routeoptimization.v1.Shipment.Load value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableLoadDemands().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00000010;
return this;
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
public Builder putAllLoadDemands(
java.util.Map
values) {
for (java.util.Map.Entry<
java.lang.String, com.google.maps.routeoptimization.v1.Shipment.Load>
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableLoadDemands().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00000010;
return this;
}
/**
*
*
*
* Total visit load demand as the sum of the shipment and the visit request
* `load_demands`. The values are negative if the visit is a delivery.
* Demands are reported for the same types as the
* [Transition.loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition]
* (see this field).
*
*
* map<string, .google.maps.routeoptimization.v1.Shipment.Load> load_demands = 11;
*
*/
public com.google.maps.routeoptimization.v1.Shipment.Load.Builder
putLoadDemandsBuilderIfAbsent(java.lang.String key) {
java.util.Map
builderMap = internalGetMutableLoadDemands().ensureBuilderMap();
com.google.maps.routeoptimization.v1.Shipment.LoadOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.maps.routeoptimization.v1.Shipment.Load.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.maps.routeoptimization.v1.Shipment.Load) {
entry = ((com.google.maps.routeoptimization.v1.Shipment.Load) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.maps.routeoptimization.v1.Shipment.Load.Builder) entry;
}
private com.google.protobuf.Duration detour_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
detourBuilder_;
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*
* @return Whether the detour field is set.
*/
public boolean hasDetour() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*
* @return The detour.
*/
public com.google.protobuf.Duration getDetour() {
if (detourBuilder_ == null) {
return detour_ == null ? com.google.protobuf.Duration.getDefaultInstance() : detour_;
} else {
return detourBuilder_.getMessage();
}
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
public Builder setDetour(com.google.protobuf.Duration value) {
if (detourBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detour_ = value;
} else {
detourBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
public Builder setDetour(com.google.protobuf.Duration.Builder builderForValue) {
if (detourBuilder_ == null) {
detour_ = builderForValue.build();
} else {
detourBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
public Builder mergeDetour(com.google.protobuf.Duration value) {
if (detourBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& detour_ != null
&& detour_ != com.google.protobuf.Duration.getDefaultInstance()) {
getDetourBuilder().mergeFrom(value);
} else {
detour_ = value;
}
} else {
detourBuilder_.mergeFrom(value);
}
if (detour_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
public Builder clearDetour() {
bitField0_ = (bitField0_ & ~0x00000020);
detour_ = null;
if (detourBuilder_ != null) {
detourBuilder_.dispose();
detourBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
public com.google.protobuf.Duration.Builder getDetourBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getDetourFieldBuilder().getBuilder();
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
public com.google.protobuf.DurationOrBuilder getDetourOrBuilder() {
if (detourBuilder_ != null) {
return detourBuilder_.getMessageOrBuilder();
} else {
return detour_ == null ? com.google.protobuf.Duration.getDefaultInstance() : detour_;
}
}
/**
*
*
*
* Extra detour time due to the shipments visited on the route before the
* visit and to the potential waiting time induced by time windows.
* If the visit is a delivery, the detour is computed from the corresponding
* pickup visit and is equal to:
* ```
* start_time(delivery) - start_time(pickup)
* - (duration(pickup) + travel duration from the pickup location
* to the delivery location).
* ```
* Otherwise, it is computed from the vehicle `start_location` and is equal
* to:
* ```
* start_time - vehicle_start_time - travel duration from
* the vehicle's `start_location` to the visit.
* ```
*
*
* .google.protobuf.Duration detour = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getDetourFieldBuilder() {
if (detourBuilder_ == null) {
detourBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getDetour(), getParentForChildren(), isClean());
detour_ = null;
}
return detourBuilder_;
}
private java.lang.Object shipmentLabel_ = "";
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @return The shipmentLabel.
*/
public java.lang.String getShipmentLabel() {
java.lang.Object ref = shipmentLabel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
shipmentLabel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @return The bytes for shipmentLabel.
*/
public com.google.protobuf.ByteString getShipmentLabelBytes() {
java.lang.Object ref = shipmentLabel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
shipmentLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @param value The shipmentLabel to set.
* @return This builder for chaining.
*/
public Builder setShipmentLabel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
shipmentLabel_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @return This builder for chaining.
*/
public Builder clearShipmentLabel() {
shipmentLabel_ = getDefaultInstance().getShipmentLabel();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* Copy of the corresponding `Shipment.label`, if specified in the
* `Shipment`.
*
*
* string shipment_label = 7;
*
* @param value The bytes for shipmentLabel to set.
* @return This builder for chaining.
*/
public Builder setShipmentLabelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
shipmentLabel_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object visitLabel_ = "";
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @return The visitLabel.
*/
public java.lang.String getVisitLabel() {
java.lang.Object ref = visitLabel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
visitLabel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @return The bytes for visitLabel.
*/
public com.google.protobuf.ByteString getVisitLabelBytes() {
java.lang.Object ref = visitLabel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
visitLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @param value The visitLabel to set.
* @return This builder for chaining.
*/
public Builder setVisitLabel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
visitLabel_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @return This builder for chaining.
*/
public Builder clearVisitLabel() {
visitLabel_ = getDefaultInstance().getVisitLabel();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* Copy of the corresponding
* [VisitRequest.label][google.maps.routeoptimization.v1.Shipment.VisitRequest.label],
* if specified in the `VisitRequest`.
*
*
* string visit_label = 8;
*
* @param value The bytes for visitLabel to set.
* @return This builder for chaining.
*/
public Builder setVisitLabelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
visitLabel_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.ShipmentRoute.Visit)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.ShipmentRoute.Visit)
private static final com.google.maps.routeoptimization.v1.ShipmentRoute.Visit DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.ShipmentRoute.Visit();
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Visit getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Visit parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TransitionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.ShipmentRoute.Transition)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*
* @return Whether the travelDuration field is set.
*/
boolean hasTravelDuration();
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*
* @return The travelDuration.
*/
com.google.protobuf.Duration getTravelDuration();
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
com.google.protobuf.DurationOrBuilder getTravelDurationOrBuilder();
/**
*
*
*
* Distance traveled during the transition.
*
*
* double travel_distance_meters = 2;
*
* @return The travelDistanceMeters.
*/
double getTravelDistanceMeters();
/**
*
*
*
* When traffic is requested via
* [OptimizeToursRequest.consider_road_traffic]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* and the traffic info couldn't be retrieved for a `Transition`, this
* boolean is set to true. This may be temporary (rare hiccup in the
* realtime traffic servers) or permanent (no data for this location).
*
*
* bool traffic_info_unavailable = 3;
*
* @return The trafficInfoUnavailable.
*/
boolean getTrafficInfoUnavailable();
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*
* @return Whether the delayDuration field is set.
*/
boolean hasDelayDuration();
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*
* @return The delayDuration.
*/
com.google.protobuf.Duration getDelayDuration();
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
com.google.protobuf.DurationOrBuilder getDelayDurationOrBuilder();
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*
* @return Whether the breakDuration field is set.
*/
boolean hasBreakDuration();
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*
* @return The breakDuration.
*/
com.google.protobuf.Duration getBreakDuration();
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
com.google.protobuf.DurationOrBuilder getBreakDurationOrBuilder();
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*
* @return Whether the waitDuration field is set.
*/
boolean hasWaitDuration();
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*
* @return The waitDuration.
*/
com.google.protobuf.Duration getWaitDuration();
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
com.google.protobuf.DurationOrBuilder getWaitDurationOrBuilder();
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*
* @return Whether the totalDuration field is set.
*/
boolean hasTotalDuration();
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*
* @return The totalDuration.
*/
com.google.protobuf.Duration getTotalDuration();
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
com.google.protobuf.DurationOrBuilder getTotalDurationOrBuilder();
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*
* @return Whether the startTime field is set.
*/
boolean hasStartTime();
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*
* @return The startTime.
*/
com.google.protobuf.Timestamp getStartTime();
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder();
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*
* @return Whether the routePolyline field is set.
*/
boolean hasRoutePolyline();
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*
* @return The routePolyline.
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline getRoutePolyline();
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder
getRoutePolylineOrBuilder();
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The routeToken.
*/
java.lang.String getRouteToken();
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for routeToken.
*/
com.google.protobuf.ByteString getRouteTokenBytes();
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
int getVehicleLoadsCount();
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
boolean containsVehicleLoads(java.lang.String key);
/** Use {@link #getVehicleLoadsMap()} instead. */
@java.lang.Deprecated
java.util.Map
getVehicleLoads();
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
java.util.Map
getVehicleLoadsMap();
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
/* nullable */
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad getVehicleLoadsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad defaultValue);
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad getVehicleLoadsOrThrow(
java.lang.String key);
}
/**
*
*
*
* Transition between two events on the route. See the description of
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
* If the vehicle does not have a `start_location` and/or `end_location`, the
* corresponding travel metrics are 0.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.Transition}
*/
public static final class Transition extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.ShipmentRoute.Transition)
TransitionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Transition.newBuilder() to construct.
private Transition(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Transition() {
routeToken_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Transition();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Transition_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 11:
return internalGetVehicleLoads();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Transition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder.class);
}
private int bitField0_;
public static final int TRAVEL_DURATION_FIELD_NUMBER = 1;
private com.google.protobuf.Duration travelDuration_;
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*
* @return Whether the travelDuration field is set.
*/
@java.lang.Override
public boolean hasTravelDuration() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*
* @return The travelDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getTravelDuration() {
return travelDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: travelDuration_;
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getTravelDurationOrBuilder() {
return travelDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: travelDuration_;
}
public static final int TRAVEL_DISTANCE_METERS_FIELD_NUMBER = 2;
private double travelDistanceMeters_ = 0D;
/**
*
*
*
* Distance traveled during the transition.
*
*
* double travel_distance_meters = 2;
*
* @return The travelDistanceMeters.
*/
@java.lang.Override
public double getTravelDistanceMeters() {
return travelDistanceMeters_;
}
public static final int TRAFFIC_INFO_UNAVAILABLE_FIELD_NUMBER = 3;
private boolean trafficInfoUnavailable_ = false;
/**
*
*
*
* When traffic is requested via
* [OptimizeToursRequest.consider_road_traffic]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* and the traffic info couldn't be retrieved for a `Transition`, this
* boolean is set to true. This may be temporary (rare hiccup in the
* realtime traffic servers) or permanent (no data for this location).
*
*
* bool traffic_info_unavailable = 3;
*
* @return The trafficInfoUnavailable.
*/
@java.lang.Override
public boolean getTrafficInfoUnavailable() {
return trafficInfoUnavailable_;
}
public static final int DELAY_DURATION_FIELD_NUMBER = 4;
private com.google.protobuf.Duration delayDuration_;
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*
* @return Whether the delayDuration field is set.
*/
@java.lang.Override
public boolean hasDelayDuration() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*
* @return The delayDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getDelayDuration() {
return delayDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: delayDuration_;
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getDelayDurationOrBuilder() {
return delayDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: delayDuration_;
}
public static final int BREAK_DURATION_FIELD_NUMBER = 5;
private com.google.protobuf.Duration breakDuration_;
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*
* @return Whether the breakDuration field is set.
*/
@java.lang.Override
public boolean hasBreakDuration() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*
* @return The breakDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getBreakDuration() {
return breakDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: breakDuration_;
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getBreakDurationOrBuilder() {
return breakDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: breakDuration_;
}
public static final int WAIT_DURATION_FIELD_NUMBER = 6;
private com.google.protobuf.Duration waitDuration_;
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*
* @return Whether the waitDuration field is set.
*/
@java.lang.Override
public boolean hasWaitDuration() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*
* @return The waitDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getWaitDuration() {
return waitDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: waitDuration_;
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getWaitDurationOrBuilder() {
return waitDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: waitDuration_;
}
public static final int TOTAL_DURATION_FIELD_NUMBER = 7;
private com.google.protobuf.Duration totalDuration_;
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*
* @return Whether the totalDuration field is set.
*/
@java.lang.Override
public boolean hasTotalDuration() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*
* @return The totalDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getTotalDuration() {
return totalDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: totalDuration_;
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getTotalDurationOrBuilder() {
return totalDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: totalDuration_;
}
public static final int START_TIME_FIELD_NUMBER = 8;
private com.google.protobuf.Timestamp startTime_;
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*
* @return Whether the startTime field is set.
*/
@java.lang.Override
public boolean hasStartTime() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*
* @return The startTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getStartTime() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
public static final int ROUTE_POLYLINE_FIELD_NUMBER = 9;
private com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline routePolyline_;
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*
* @return Whether the routePolyline field is set.
*/
@java.lang.Override
public boolean hasRoutePolyline() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*
* @return The routePolyline.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline getRoutePolyline() {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.getDefaultInstance()
: routePolyline_;
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder
getRoutePolylineOrBuilder() {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.getDefaultInstance()
: routePolyline_;
}
public static final int ROUTE_TOKEN_FIELD_NUMBER = 12;
@SuppressWarnings("serial")
private volatile java.lang.Object routeToken_ = "";
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The routeToken.
*/
@java.lang.Override
public java.lang.String getRouteToken() {
java.lang.Object ref = routeToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
routeToken_ = s;
return s;
}
}
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for routeToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRouteTokenBytes() {
java.lang.Object ref = routeToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
routeToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VEHICLE_LOADS_FIELD_NUMBER = 11;
private static final class VehicleLoadsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Transition_VehicleLoadsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad
.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
vehicleLoads_;
private com.google.protobuf.MapField<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
internalGetVehicleLoads() {
if (vehicleLoads_ == null) {
return com.google.protobuf.MapField.emptyMapField(
VehicleLoadsDefaultEntryHolder.defaultEntry);
}
return vehicleLoads_;
}
public int getVehicleLoadsCount() {
return internalGetVehicleLoads().getMap().size();
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public boolean containsVehicleLoads(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetVehicleLoads().getMap().containsKey(key);
}
/** Use {@link #getVehicleLoadsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
getVehicleLoads() {
return getVehicleLoadsMap();
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
getVehicleLoadsMap() {
return internalGetVehicleLoads().getMap();
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public /* nullable */ com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad
getVehicleLoadsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
map = internalGetVehicleLoads().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad getVehicleLoadsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
map = internalGetVehicleLoads().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getTravelDuration());
}
if (java.lang.Double.doubleToRawLongBits(travelDistanceMeters_) != 0) {
output.writeDouble(2, travelDistanceMeters_);
}
if (trafficInfoUnavailable_ != false) {
output.writeBool(3, trafficInfoUnavailable_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(4, getDelayDuration());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(5, getBreakDuration());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(6, getWaitDuration());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(7, getTotalDuration());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(8, getStartTime());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(9, getRoutePolyline());
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetVehicleLoads(), VehicleLoadsDefaultEntryHolder.defaultEntry, 11);
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(routeToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, routeToken_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getTravelDuration());
}
if (java.lang.Double.doubleToRawLongBits(travelDistanceMeters_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(2, travelDistanceMeters_);
}
if (trafficInfoUnavailable_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(3, trafficInfoUnavailable_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getDelayDuration());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(5, getBreakDuration());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getWaitDuration());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(7, getTotalDuration());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(8, getStartTime());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(9, getRoutePolyline());
}
for (java.util.Map.Entry<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
entry : internalGetVehicleLoads().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
vehicleLoads__ =
VehicleLoadsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(11, vehicleLoads__);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(routeToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, routeToken_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.Transition)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition other =
(com.google.maps.routeoptimization.v1.ShipmentRoute.Transition) obj;
if (hasTravelDuration() != other.hasTravelDuration()) return false;
if (hasTravelDuration()) {
if (!getTravelDuration().equals(other.getTravelDuration())) return false;
}
if (java.lang.Double.doubleToLongBits(getTravelDistanceMeters())
!= java.lang.Double.doubleToLongBits(other.getTravelDistanceMeters())) return false;
if (getTrafficInfoUnavailable() != other.getTrafficInfoUnavailable()) return false;
if (hasDelayDuration() != other.hasDelayDuration()) return false;
if (hasDelayDuration()) {
if (!getDelayDuration().equals(other.getDelayDuration())) return false;
}
if (hasBreakDuration() != other.hasBreakDuration()) return false;
if (hasBreakDuration()) {
if (!getBreakDuration().equals(other.getBreakDuration())) return false;
}
if (hasWaitDuration() != other.hasWaitDuration()) return false;
if (hasWaitDuration()) {
if (!getWaitDuration().equals(other.getWaitDuration())) return false;
}
if (hasTotalDuration() != other.hasTotalDuration()) return false;
if (hasTotalDuration()) {
if (!getTotalDuration().equals(other.getTotalDuration())) return false;
}
if (hasStartTime() != other.hasStartTime()) return false;
if (hasStartTime()) {
if (!getStartTime().equals(other.getStartTime())) return false;
}
if (hasRoutePolyline() != other.hasRoutePolyline()) return false;
if (hasRoutePolyline()) {
if (!getRoutePolyline().equals(other.getRoutePolyline())) return false;
}
if (!getRouteToken().equals(other.getRouteToken())) return false;
if (!internalGetVehicleLoads().equals(other.internalGetVehicleLoads())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTravelDuration()) {
hash = (37 * hash) + TRAVEL_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getTravelDuration().hashCode();
}
hash = (37 * hash) + TRAVEL_DISTANCE_METERS_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTravelDistanceMeters()));
hash = (37 * hash) + TRAFFIC_INFO_UNAVAILABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getTrafficInfoUnavailable());
if (hasDelayDuration()) {
hash = (37 * hash) + DELAY_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getDelayDuration().hashCode();
}
if (hasBreakDuration()) {
hash = (37 * hash) + BREAK_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getBreakDuration().hashCode();
}
if (hasWaitDuration()) {
hash = (37 * hash) + WAIT_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getWaitDuration().hashCode();
}
if (hasTotalDuration()) {
hash = (37 * hash) + TOTAL_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getTotalDuration().hashCode();
}
if (hasStartTime()) {
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + getStartTime().hashCode();
}
if (hasRoutePolyline()) {
hash = (37 * hash) + ROUTE_POLYLINE_FIELD_NUMBER;
hash = (53 * hash) + getRoutePolyline().hashCode();
}
hash = (37 * hash) + ROUTE_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getRouteToken().hashCode();
if (!internalGetVehicleLoads().getMap().isEmpty()) {
hash = (37 * hash) + VEHICLE_LOADS_FIELD_NUMBER;
hash = (53 * hash) + internalGetVehicleLoads().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Transition between two events on the route. See the description of
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
* If the vehicle does not have a `start_location` and/or `end_location`, the
* corresponding travel metrics are 0.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.Transition}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.ShipmentRoute.Transition)
com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Transition_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 11:
return internalGetVehicleLoads();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 11:
return internalGetMutableVehicleLoads();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Transition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getTravelDurationFieldBuilder();
getDelayDurationFieldBuilder();
getBreakDurationFieldBuilder();
getWaitDurationFieldBuilder();
getTotalDurationFieldBuilder();
getStartTimeFieldBuilder();
getRoutePolylineFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
travelDuration_ = null;
if (travelDurationBuilder_ != null) {
travelDurationBuilder_.dispose();
travelDurationBuilder_ = null;
}
travelDistanceMeters_ = 0D;
trafficInfoUnavailable_ = false;
delayDuration_ = null;
if (delayDurationBuilder_ != null) {
delayDurationBuilder_.dispose();
delayDurationBuilder_ = null;
}
breakDuration_ = null;
if (breakDurationBuilder_ != null) {
breakDurationBuilder_.dispose();
breakDurationBuilder_ = null;
}
waitDuration_ = null;
if (waitDurationBuilder_ != null) {
waitDurationBuilder_.dispose();
waitDurationBuilder_ = null;
}
totalDuration_ = null;
if (totalDurationBuilder_ != null) {
totalDurationBuilder_.dispose();
totalDurationBuilder_ = null;
}
startTime_ = null;
if (startTimeBuilder_ != null) {
startTimeBuilder_.dispose();
startTimeBuilder_ = null;
}
routePolyline_ = null;
if (routePolylineBuilder_ != null) {
routePolylineBuilder_.dispose();
routePolylineBuilder_ = null;
}
routeToken_ = "";
internalGetMutableVehicleLoads().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Transition_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition
getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition build() {
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition buildPartial() {
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition result =
new com.google.maps.routeoptimization.v1.ShipmentRoute.Transition(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.travelDuration_ =
travelDurationBuilder_ == null ? travelDuration_ : travelDurationBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.travelDistanceMeters_ = travelDistanceMeters_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.trafficInfoUnavailable_ = trafficInfoUnavailable_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.delayDuration_ =
delayDurationBuilder_ == null ? delayDuration_ : delayDurationBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.breakDuration_ =
breakDurationBuilder_ == null ? breakDuration_ : breakDurationBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.waitDuration_ =
waitDurationBuilder_ == null ? waitDuration_ : waitDurationBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.totalDuration_ =
totalDurationBuilder_ == null ? totalDuration_ : totalDurationBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.startTime_ = startTimeBuilder_ == null ? startTime_ : startTimeBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.routePolyline_ =
routePolylineBuilder_ == null ? routePolyline_ : routePolylineBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.routeToken_ = routeToken_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.vehicleLoads_ =
internalGetVehicleLoads().build(VehicleLoadsDefaultEntryHolder.defaultEntry);
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.Transition) {
return mergeFrom((com.google.maps.routeoptimization.v1.ShipmentRoute.Transition) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition other) {
if (other
== com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.getDefaultInstance())
return this;
if (other.hasTravelDuration()) {
mergeTravelDuration(other.getTravelDuration());
}
if (other.getTravelDistanceMeters() != 0D) {
setTravelDistanceMeters(other.getTravelDistanceMeters());
}
if (other.getTrafficInfoUnavailable() != false) {
setTrafficInfoUnavailable(other.getTrafficInfoUnavailable());
}
if (other.hasDelayDuration()) {
mergeDelayDuration(other.getDelayDuration());
}
if (other.hasBreakDuration()) {
mergeBreakDuration(other.getBreakDuration());
}
if (other.hasWaitDuration()) {
mergeWaitDuration(other.getWaitDuration());
}
if (other.hasTotalDuration()) {
mergeTotalDuration(other.getTotalDuration());
}
if (other.hasStartTime()) {
mergeStartTime(other.getStartTime());
}
if (other.hasRoutePolyline()) {
mergeRoutePolyline(other.getRoutePolyline());
}
if (!other.getRouteToken().isEmpty()) {
routeToken_ = other.routeToken_;
bitField0_ |= 0x00000200;
onChanged();
}
internalGetMutableVehicleLoads().mergeFrom(other.internalGetVehicleLoads());
bitField0_ |= 0x00000400;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(
getTravelDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 17:
{
travelDistanceMeters_ = input.readDouble();
bitField0_ |= 0x00000002;
break;
} // case 17
case 24:
{
trafficInfoUnavailable_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34:
{
input.readMessage(getDelayDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42:
{
input.readMessage(getBreakDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
case 50:
{
input.readMessage(getWaitDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 58:
{
input.readMessage(getTotalDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 58
case 66:
{
input.readMessage(getStartTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 74:
{
input.readMessage(getRoutePolylineFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 74
case 90:
{
com.google.protobuf.MapEntry<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
vehicleLoads__ =
input.readMessage(
VehicleLoadsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableVehicleLoads()
.ensureBuilderMap()
.put(vehicleLoads__.getKey(), vehicleLoads__.getValue());
bitField0_ |= 0x00000400;
break;
} // case 90
case 98:
{
routeToken_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 98
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.Duration travelDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
travelDurationBuilder_;
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*
* @return Whether the travelDuration field is set.
*/
public boolean hasTravelDuration() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*
* @return The travelDuration.
*/
public com.google.protobuf.Duration getTravelDuration() {
if (travelDurationBuilder_ == null) {
return travelDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: travelDuration_;
} else {
return travelDurationBuilder_.getMessage();
}
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
public Builder setTravelDuration(com.google.protobuf.Duration value) {
if (travelDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
travelDuration_ = value;
} else {
travelDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
public Builder setTravelDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (travelDurationBuilder_ == null) {
travelDuration_ = builderForValue.build();
} else {
travelDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
public Builder mergeTravelDuration(com.google.protobuf.Duration value) {
if (travelDurationBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& travelDuration_ != null
&& travelDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getTravelDurationBuilder().mergeFrom(value);
} else {
travelDuration_ = value;
}
} else {
travelDurationBuilder_.mergeFrom(value);
}
if (travelDuration_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
public Builder clearTravelDuration() {
bitField0_ = (bitField0_ & ~0x00000001);
travelDuration_ = null;
if (travelDurationBuilder_ != null) {
travelDurationBuilder_.dispose();
travelDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
public com.google.protobuf.Duration.Builder getTravelDurationBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getTravelDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
public com.google.protobuf.DurationOrBuilder getTravelDurationOrBuilder() {
if (travelDurationBuilder_ != null) {
return travelDurationBuilder_.getMessageOrBuilder();
} else {
return travelDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: travelDuration_;
}
}
/**
*
*
*
* Travel duration during this transition.
*
*
* .google.protobuf.Duration travel_duration = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getTravelDurationFieldBuilder() {
if (travelDurationBuilder_ == null) {
travelDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getTravelDuration(), getParentForChildren(), isClean());
travelDuration_ = null;
}
return travelDurationBuilder_;
}
private double travelDistanceMeters_;
/**
*
*
*
* Distance traveled during the transition.
*
*
* double travel_distance_meters = 2;
*
* @return The travelDistanceMeters.
*/
@java.lang.Override
public double getTravelDistanceMeters() {
return travelDistanceMeters_;
}
/**
*
*
*
* Distance traveled during the transition.
*
*
* double travel_distance_meters = 2;
*
* @param value The travelDistanceMeters to set.
* @return This builder for chaining.
*/
public Builder setTravelDistanceMeters(double value) {
travelDistanceMeters_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Distance traveled during the transition.
*
*
* double travel_distance_meters = 2;
*
* @return This builder for chaining.
*/
public Builder clearTravelDistanceMeters() {
bitField0_ = (bitField0_ & ~0x00000002);
travelDistanceMeters_ = 0D;
onChanged();
return this;
}
private boolean trafficInfoUnavailable_;
/**
*
*
*
* When traffic is requested via
* [OptimizeToursRequest.consider_road_traffic]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* and the traffic info couldn't be retrieved for a `Transition`, this
* boolean is set to true. This may be temporary (rare hiccup in the
* realtime traffic servers) or permanent (no data for this location).
*
*
* bool traffic_info_unavailable = 3;
*
* @return The trafficInfoUnavailable.
*/
@java.lang.Override
public boolean getTrafficInfoUnavailable() {
return trafficInfoUnavailable_;
}
/**
*
*
*
* When traffic is requested via
* [OptimizeToursRequest.consider_road_traffic]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* and the traffic info couldn't be retrieved for a `Transition`, this
* boolean is set to true. This may be temporary (rare hiccup in the
* realtime traffic servers) or permanent (no data for this location).
*
*
* bool traffic_info_unavailable = 3;
*
* @param value The trafficInfoUnavailable to set.
* @return This builder for chaining.
*/
public Builder setTrafficInfoUnavailable(boolean value) {
trafficInfoUnavailable_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* When traffic is requested via
* [OptimizeToursRequest.consider_road_traffic]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* and the traffic info couldn't be retrieved for a `Transition`, this
* boolean is set to true. This may be temporary (rare hiccup in the
* realtime traffic servers) or permanent (no data for this location).
*
*
* bool traffic_info_unavailable = 3;
*
* @return This builder for chaining.
*/
public Builder clearTrafficInfoUnavailable() {
bitField0_ = (bitField0_ & ~0x00000004);
trafficInfoUnavailable_ = false;
onChanged();
return this;
}
private com.google.protobuf.Duration delayDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
delayDurationBuilder_;
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*
* @return Whether the delayDuration field is set.
*/
public boolean hasDelayDuration() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*
* @return The delayDuration.
*/
public com.google.protobuf.Duration getDelayDuration() {
if (delayDurationBuilder_ == null) {
return delayDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: delayDuration_;
} else {
return delayDurationBuilder_.getMessage();
}
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
public Builder setDelayDuration(com.google.protobuf.Duration value) {
if (delayDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
delayDuration_ = value;
} else {
delayDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
public Builder setDelayDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (delayDurationBuilder_ == null) {
delayDuration_ = builderForValue.build();
} else {
delayDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
public Builder mergeDelayDuration(com.google.protobuf.Duration value) {
if (delayDurationBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& delayDuration_ != null
&& delayDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getDelayDurationBuilder().mergeFrom(value);
} else {
delayDuration_ = value;
}
} else {
delayDurationBuilder_.mergeFrom(value);
}
if (delayDuration_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
public Builder clearDelayDuration() {
bitField0_ = (bitField0_ & ~0x00000008);
delayDuration_ = null;
if (delayDurationBuilder_ != null) {
delayDurationBuilder_.dispose();
delayDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
public com.google.protobuf.Duration.Builder getDelayDurationBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getDelayDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
public com.google.protobuf.DurationOrBuilder getDelayDurationOrBuilder() {
if (delayDurationBuilder_ != null) {
return delayDurationBuilder_.getMessageOrBuilder();
} else {
return delayDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: delayDuration_;
}
}
/**
*
*
*
* Sum of the delay durations applied to this transition. If any, the delay
* starts exactly `delay_duration` seconds before the next event (visit or
* vehicle end). See
* [TransitionAttributes.delay][google.maps.routeoptimization.v1.TransitionAttributes.delay].
*
*
* .google.protobuf.Duration delay_duration = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getDelayDurationFieldBuilder() {
if (delayDurationBuilder_ == null) {
delayDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getDelayDuration(), getParentForChildren(), isClean());
delayDuration_ = null;
}
return delayDurationBuilder_;
}
private com.google.protobuf.Duration breakDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
breakDurationBuilder_;
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*
* @return Whether the breakDuration field is set.
*/
public boolean hasBreakDuration() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*
* @return The breakDuration.
*/
public com.google.protobuf.Duration getBreakDuration() {
if (breakDurationBuilder_ == null) {
return breakDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: breakDuration_;
} else {
return breakDurationBuilder_.getMessage();
}
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
public Builder setBreakDuration(com.google.protobuf.Duration value) {
if (breakDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
breakDuration_ = value;
} else {
breakDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
public Builder setBreakDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (breakDurationBuilder_ == null) {
breakDuration_ = builderForValue.build();
} else {
breakDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
public Builder mergeBreakDuration(com.google.protobuf.Duration value) {
if (breakDurationBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)
&& breakDuration_ != null
&& breakDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getBreakDurationBuilder().mergeFrom(value);
} else {
breakDuration_ = value;
}
} else {
breakDurationBuilder_.mergeFrom(value);
}
if (breakDuration_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
public Builder clearBreakDuration() {
bitField0_ = (bitField0_ & ~0x00000010);
breakDuration_ = null;
if (breakDurationBuilder_ != null) {
breakDurationBuilder_.dispose();
breakDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
public com.google.protobuf.Duration.Builder getBreakDurationBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getBreakDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
public com.google.protobuf.DurationOrBuilder getBreakDurationOrBuilder() {
if (breakDurationBuilder_ != null) {
return breakDurationBuilder_.getMessageOrBuilder();
} else {
return breakDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: breakDuration_;
}
}
/**
*
*
*
* Sum of the duration of the breaks occurring during this transition, if
* any. Details about each break's start time and duration are stored in
* [ShipmentRoute.breaks][google.maps.routeoptimization.v1.ShipmentRoute.breaks].
*
*
* .google.protobuf.Duration break_duration = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getBreakDurationFieldBuilder() {
if (breakDurationBuilder_ == null) {
breakDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getBreakDuration(), getParentForChildren(), isClean());
breakDuration_ = null;
}
return breakDurationBuilder_;
}
private com.google.protobuf.Duration waitDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
waitDurationBuilder_;
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*
* @return Whether the waitDuration field is set.
*/
public boolean hasWaitDuration() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*
* @return The waitDuration.
*/
public com.google.protobuf.Duration getWaitDuration() {
if (waitDurationBuilder_ == null) {
return waitDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: waitDuration_;
} else {
return waitDurationBuilder_.getMessage();
}
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
public Builder setWaitDuration(com.google.protobuf.Duration value) {
if (waitDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
waitDuration_ = value;
} else {
waitDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
public Builder setWaitDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (waitDurationBuilder_ == null) {
waitDuration_ = builderForValue.build();
} else {
waitDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
public Builder mergeWaitDuration(com.google.protobuf.Duration value) {
if (waitDurationBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& waitDuration_ != null
&& waitDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getWaitDurationBuilder().mergeFrom(value);
} else {
waitDuration_ = value;
}
} else {
waitDurationBuilder_.mergeFrom(value);
}
if (waitDuration_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
public Builder clearWaitDuration() {
bitField0_ = (bitField0_ & ~0x00000020);
waitDuration_ = null;
if (waitDurationBuilder_ != null) {
waitDurationBuilder_.dispose();
waitDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
public com.google.protobuf.Duration.Builder getWaitDurationBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getWaitDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
public com.google.protobuf.DurationOrBuilder getWaitDurationOrBuilder() {
if (waitDurationBuilder_ != null) {
return waitDurationBuilder_.getMessageOrBuilder();
} else {
return waitDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: waitDuration_;
}
}
/**
*
*
*
* Time spent waiting during this transition. Wait duration corresponds to
* idle time and does not include break time. Also note that this wait time
* may be split into several non-contiguous intervals.
*
*
* .google.protobuf.Duration wait_duration = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getWaitDurationFieldBuilder() {
if (waitDurationBuilder_ == null) {
waitDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getWaitDuration(), getParentForChildren(), isClean());
waitDuration_ = null;
}
return waitDurationBuilder_;
}
private com.google.protobuf.Duration totalDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
totalDurationBuilder_;
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*
* @return Whether the totalDuration field is set.
*/
public boolean hasTotalDuration() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*
* @return The totalDuration.
*/
public com.google.protobuf.Duration getTotalDuration() {
if (totalDurationBuilder_ == null) {
return totalDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: totalDuration_;
} else {
return totalDurationBuilder_.getMessage();
}
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
public Builder setTotalDuration(com.google.protobuf.Duration value) {
if (totalDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
totalDuration_ = value;
} else {
totalDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
public Builder setTotalDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (totalDurationBuilder_ == null) {
totalDuration_ = builderForValue.build();
} else {
totalDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
public Builder mergeTotalDuration(com.google.protobuf.Duration value) {
if (totalDurationBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)
&& totalDuration_ != null
&& totalDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getTotalDurationBuilder().mergeFrom(value);
} else {
totalDuration_ = value;
}
} else {
totalDurationBuilder_.mergeFrom(value);
}
if (totalDuration_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
public Builder clearTotalDuration() {
bitField0_ = (bitField0_ & ~0x00000040);
totalDuration_ = null;
if (totalDurationBuilder_ != null) {
totalDurationBuilder_.dispose();
totalDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
public com.google.protobuf.Duration.Builder getTotalDurationBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getTotalDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
public com.google.protobuf.DurationOrBuilder getTotalDurationOrBuilder() {
if (totalDurationBuilder_ != null) {
return totalDurationBuilder_.getMessageOrBuilder();
} else {
return totalDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: totalDuration_;
}
}
/**
*
*
*
* Total duration of the transition, provided for convenience. It is equal
* to:
*
* * next visit `start_time` (or `vehicle_end_time` if this is the last
* transition) - this transition's `start_time`;
* * if `ShipmentRoute.has_traffic_infeasibilities` is false, the following
* additionally holds: `total_duration = travel_duration + delay_duration
* + break_duration + wait_duration`.
*
*
* .google.protobuf.Duration total_duration = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getTotalDurationFieldBuilder() {
if (totalDurationBuilder_ == null) {
totalDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getTotalDuration(), getParentForChildren(), isClean());
totalDuration_ = null;
}
return totalDurationBuilder_;
}
private com.google.protobuf.Timestamp startTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
startTimeBuilder_;
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*
* @return Whether the startTime field is set.
*/
public boolean hasStartTime() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*
* @return The startTime.
*/
public com.google.protobuf.Timestamp getStartTime() {
if (startTimeBuilder_ == null) {
return startTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: startTime_;
} else {
return startTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
public Builder setStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startTime_ = value;
} else {
startTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
public Builder setStartTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (startTimeBuilder_ == null) {
startTime_ = builderForValue.build();
} else {
startTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
public Builder mergeStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& startTime_ != null
&& startTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getStartTimeBuilder().mergeFrom(value);
} else {
startTime_ = value;
}
} else {
startTimeBuilder_.mergeFrom(value);
}
if (startTime_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
public Builder clearStartTime() {
bitField0_ = (bitField0_ & ~0x00000080);
startTime_ = null;
if (startTimeBuilder_ != null) {
startTimeBuilder_.dispose();
startTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
public com.google.protobuf.Timestamp.Builder getStartTimeBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getStartTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
if (startTimeBuilder_ != null) {
return startTimeBuilder_.getMessageOrBuilder();
} else {
return startTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: startTime_;
}
}
/**
*
*
*
* Start time of this transition.
*
*
* .google.protobuf.Timestamp start_time = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getStartTimeFieldBuilder() {
if (startTimeBuilder_ == null) {
startTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getStartTime(), getParentForChildren(), isClean());
startTime_ = null;
}
return startTimeBuilder_;
}
private com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline routePolyline_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder>
routePolylineBuilder_;
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*
* @return Whether the routePolyline field is set.
*/
public boolean hasRoutePolyline() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*
* @return The routePolyline.
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline getRoutePolyline() {
if (routePolylineBuilder_ == null) {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance()
: routePolyline_;
} else {
return routePolylineBuilder_.getMessage();
}
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
public Builder setRoutePolyline(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline value) {
if (routePolylineBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
routePolyline_ = value;
} else {
routePolylineBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
public Builder setRoutePolyline(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder
builderForValue) {
if (routePolylineBuilder_ == null) {
routePolyline_ = builderForValue.build();
} else {
routePolylineBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
public Builder mergeRoutePolyline(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline value) {
if (routePolylineBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)
&& routePolyline_ != null
&& routePolyline_
!= com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance()) {
getRoutePolylineBuilder().mergeFrom(value);
} else {
routePolyline_ = value;
}
} else {
routePolylineBuilder_.mergeFrom(value);
}
if (routePolyline_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
public Builder clearRoutePolyline() {
bitField0_ = (bitField0_ & ~0x00000100);
routePolyline_ = null;
if (routePolylineBuilder_ != null) {
routePolylineBuilder_.dispose();
routePolylineBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder
getRoutePolylineBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getRoutePolylineFieldBuilder().getBuilder();
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder
getRoutePolylineOrBuilder() {
if (routePolylineBuilder_ != null) {
return routePolylineBuilder_.getMessageOrBuilder();
} else {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance()
: routePolyline_;
}
}
/**
*
*
*
* The encoded polyline representation of the route followed during the
* transition.
* This field is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 9;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder>
getRoutePolylineFieldBuilder() {
if (routePolylineBuilder_ == null) {
routePolylineBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder>(
getRoutePolyline(), getParentForChildren(), isClean());
routePolyline_ = null;
}
return routePolylineBuilder_;
}
private java.lang.Object routeToken_ = "";
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The routeToken.
*/
public java.lang.String getRouteToken() {
java.lang.Object ref = routeToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
routeToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for routeToken.
*/
public com.google.protobuf.ByteString getRouteTokenBytes() {
java.lang.Object ref = routeToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
routeToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The routeToken to set.
* @return This builder for chaining.
*/
public Builder setRouteToken(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
routeToken_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearRouteToken() {
routeToken_ = getDefaultInstance().getRouteToken();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* Output only. An opaque token that can be passed to [Navigation
* SDK](https://developers.google.com/maps/documentation/navigation) to
* reconstruct the route during navigation, and, in the event of rerouting,
* honor the original intention when the route was created. Treat this token
* as an opaque blob. Don't compare its value across requests as its value
* may change even if the service returns the exact same route. This field
* is only populated if [populate_transition_polylines]
* [google.maps.routeoptimization.v1.OptimizeToursRequest.populate_transition_polylines]
* is set to true.
*
*
* string route_token = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for routeToken to set.
* @return This builder for chaining.
*/
public Builder setRouteTokenBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
routeToken_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private static final class VehicleLoadsConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> {
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad build(
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder val) {
if (val instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad) {
return (com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad) val;
}
return ((com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder) val)
.build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
defaultEntry() {
return VehicleLoadsDefaultEntryHolder.defaultEntry;
}
};
private static final VehicleLoadsConverter vehicleLoadsConverter =
new VehicleLoadsConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder>
vehicleLoads_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder>
internalGetVehicleLoads() {
if (vehicleLoads_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(vehicleLoadsConverter);
}
return vehicleLoads_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder>
internalGetMutableVehicleLoads() {
if (vehicleLoads_ == null) {
vehicleLoads_ = new com.google.protobuf.MapFieldBuilder<>(vehicleLoadsConverter);
}
bitField0_ |= 0x00000400;
onChanged();
return vehicleLoads_;
}
public int getVehicleLoadsCount() {
return internalGetVehicleLoads().ensureBuilderMap().size();
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public boolean containsVehicleLoads(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetVehicleLoads().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getVehicleLoadsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
getVehicleLoads() {
return getVehicleLoadsMap();
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
getVehicleLoadsMap() {
return internalGetVehicleLoads().getImmutableMap();
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public /* nullable */ com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad
getVehicleLoadsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder>
map = internalGetMutableVehicleLoads().ensureBuilderMap();
return map.containsKey(key) ? vehicleLoadsConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad getVehicleLoadsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder>
map = internalGetMutableVehicleLoads().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return vehicleLoadsConverter.build(map.get(key));
}
public Builder clearVehicleLoads() {
bitField0_ = (bitField0_ & ~0x00000400);
internalGetMutableVehicleLoads().clear();
return this;
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
public Builder removeVehicleLoads(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableVehicleLoads().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
getMutableVehicleLoads() {
bitField0_ |= 0x00000400;
return internalGetMutableVehicleLoads().ensureMessageMap();
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
public Builder putVehicleLoads(
java.lang.String key,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableVehicleLoads().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00000400;
return this;
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
public Builder putAllVehicleLoads(
java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
values) {
for (java.util.Map.Entry<
java.lang.String, com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad>
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableVehicleLoads().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00000400;
return this;
}
/**
*
*
*
* Vehicle loads during this transition, for each type that either appears
* in this vehicle's
* [Vehicle.load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits],
* or that have non-zero
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* on some shipment performed on this route.
*
* The loads during the first transition are the starting loads of the
* vehicle route. Then, after each visit, the visit's `load_demands` are
* either added or subtracted to get the next transition's loads, depending
* on whether the visit was a pickup or a delivery.
*
*
*
* map<string, .google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad> vehicle_loads = 11;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder
putVehicleLoadsBuilderIfAbsent(java.lang.String key) {
java.util.Map<
java.lang.String,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder>
builderMap = internalGetMutableVehicleLoads().ensureBuilderMap();
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder entry =
builderMap.get(key);
if (entry == null) {
entry = com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad) {
entry =
((com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder) entry;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.ShipmentRoute.Transition)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.ShipmentRoute.Transition)
private static final com.google.maps.routeoptimization.v1.ShipmentRoute.Transition
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.ShipmentRoute.Transition();
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Transition
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Transition parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VehicleLoadOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The amount of load on the vehicle, for the given type. The unit of load
* is usually indicated by the type. See
* [Transition.vehicle_loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition.vehicle_loads].
*
*
* int64 amount = 1;
*
* @return The amount.
*/
long getAmount();
}
/**
*
*
*
* Reports the actual load of the vehicle at some point along the route,
* for a given type (see
* [Transition.vehicle_loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition.vehicle_loads]).
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad}
*/
public static final class VehicleLoad extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad)
VehicleLoadOrBuilder {
private static final long serialVersionUID = 0L;
// Use VehicleLoad.newBuilder() to construct.
private VehicleLoad(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VehicleLoad() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new VehicleLoad();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_VehicleLoad_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_VehicleLoad_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder.class);
}
public static final int AMOUNT_FIELD_NUMBER = 1;
private long amount_ = 0L;
/**
*
*
*
* The amount of load on the vehicle, for the given type. The unit of load
* is usually indicated by the type. See
* [Transition.vehicle_loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition.vehicle_loads].
*
*
* int64 amount = 1;
*
* @return The amount.
*/
@java.lang.Override
public long getAmount() {
return amount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (amount_ != 0L) {
output.writeInt64(1, amount_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (amount_ != 0L) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(1, amount_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad other =
(com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad) obj;
if (getAmount() != other.getAmount()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getAmount());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Reports the actual load of the vehicle at some point along the route,
* for a given type (see
* [Transition.vehicle_loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition.vehicle_loads]).
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad)
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_VehicleLoad_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_VehicleLoad_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
amount_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_VehicleLoad_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad
getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad build() {
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad buildPartial() {
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad result =
new com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.amount_ = amount_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad) {
return mergeFrom((com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad other) {
if (other
== com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad.getDefaultInstance())
return this;
if (other.getAmount() != 0L) {
setAmount(other.getAmount());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
amount_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long amount_;
/**
*
*
*
* The amount of load on the vehicle, for the given type. The unit of load
* is usually indicated by the type. See
* [Transition.vehicle_loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition.vehicle_loads].
*
*
* int64 amount = 1;
*
* @return The amount.
*/
@java.lang.Override
public long getAmount() {
return amount_;
}
/**
*
*
*
* The amount of load on the vehicle, for the given type. The unit of load
* is usually indicated by the type. See
* [Transition.vehicle_loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition.vehicle_loads].
*
*
* int64 amount = 1;
*
* @param value The amount to set.
* @return This builder for chaining.
*/
public Builder setAmount(long value) {
amount_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The amount of load on the vehicle, for the given type. The unit of load
* is usually indicated by the type. See
* [Transition.vehicle_loads][google.maps.routeoptimization.v1.ShipmentRoute.Transition.vehicle_loads].
*
*
* int64 amount = 1;
*
* @return This builder for chaining.
*/
public Builder clearAmount() {
bitField0_ = (bitField0_ & ~0x00000001);
amount_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad)
private static final com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad();
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VehicleLoad parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VehicleLoad
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EncodedPolylineOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @return The points.
*/
java.lang.String getPoints();
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @return The bytes for points.
*/
com.google.protobuf.ByteString getPointsBytes();
}
/**
*
*
*
* The encoded representation of a polyline. More information on polyline
* encoding can be found here:
* https://developers.google.com/maps/documentation/utilities/polylinealgorithm
* https://developers.google.com/maps/documentation/javascript/reference/geometry#encoding.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline}
*/
public static final class EncodedPolyline extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline)
EncodedPolylineOrBuilder {
private static final long serialVersionUID = 0L;
// Use EncodedPolyline.newBuilder() to construct.
private EncodedPolyline(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EncodedPolyline() {
points_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new EncodedPolyline();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_EncodedPolyline_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_EncodedPolyline_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder.class);
}
public static final int POINTS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object points_ = "";
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @return The points.
*/
@java.lang.Override
public java.lang.String getPoints() {
java.lang.Object ref = points_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
points_ = s;
return s;
}
}
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @return The bytes for points.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPointsBytes() {
java.lang.Object ref = points_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
points_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(points_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, points_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(points_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, points_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline other =
(com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline) obj;
if (!getPoints().equals(other.getPoints())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + POINTS_FIELD_NUMBER;
hash = (53 * hash) + getPoints().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* The encoded representation of a polyline. More information on polyline
* encoding can be found here:
* https://developers.google.com/maps/documentation/utilities/polylinealgorithm
* https://developers.google.com/maps/documentation/javascript/reference/geometry#encoding.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline)
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_EncodedPolyline_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_EncodedPolyline_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder.class);
}
// Construct using
// com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
points_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_EncodedPolyline_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline build() {
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline buildPartial() {
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline result =
new com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.points_ = points_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline) {
return mergeFrom(
(com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline other) {
if (other
== com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance()) return this;
if (!other.getPoints().isEmpty()) {
points_ = other.points_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
points_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object points_ = "";
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @return The points.
*/
public java.lang.String getPoints() {
java.lang.Object ref = points_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
points_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @return The bytes for points.
*/
public com.google.protobuf.ByteString getPointsBytes() {
java.lang.Object ref = points_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
points_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @param value The points to set.
* @return This builder for chaining.
*/
public Builder setPoints(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
points_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @return This builder for chaining.
*/
public Builder clearPoints() {
points_ = getDefaultInstance().getPoints();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* String representing encoded points of the polyline.
*
*
* string points = 1;
*
* @param value The bytes for points to set.
* @return This builder for chaining.
*/
public Builder setPointsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
points_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline)
private static final com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline();
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EncodedPolyline parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BreakOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.ShipmentRoute.Break)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*
* @return Whether the startTime field is set.
*/
boolean hasStartTime();
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*
* @return The startTime.
*/
com.google.protobuf.Timestamp getStartTime();
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder();
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return Whether the duration field is set.
*/
boolean hasDuration();
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return The duration.
*/
com.google.protobuf.Duration getDuration();
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
com.google.protobuf.DurationOrBuilder getDurationOrBuilder();
}
/**
*
*
*
* Data representing the execution of a break.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.Break}
*/
public static final class Break extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.ShipmentRoute.Break)
BreakOrBuilder {
private static final long serialVersionUID = 0L;
// Use Break.newBuilder() to construct.
private Break(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Break() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Break();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Break_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Break_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder.class);
}
private int bitField0_;
public static final int START_TIME_FIELD_NUMBER = 1;
private com.google.protobuf.Timestamp startTime_;
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*
* @return Whether the startTime field is set.
*/
@java.lang.Override
public boolean hasStartTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*
* @return The startTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getStartTime() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
public static final int DURATION_FIELD_NUMBER = 2;
private com.google.protobuf.Duration duration_;
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return Whether the duration field is set.
*/
@java.lang.Override
public boolean hasDuration() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return The duration.
*/
@java.lang.Override
public com.google.protobuf.Duration getDuration() {
return duration_ == null ? com.google.protobuf.Duration.getDefaultInstance() : duration_;
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getDurationOrBuilder() {
return duration_ == null ? com.google.protobuf.Duration.getDefaultInstance() : duration_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getStartTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getDuration());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getStartTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(2, getDuration());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.Break)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.ShipmentRoute.Break other =
(com.google.maps.routeoptimization.v1.ShipmentRoute.Break) obj;
if (hasStartTime() != other.hasStartTime()) return false;
if (hasStartTime()) {
if (!getStartTime().equals(other.getStartTime())) return false;
}
if (hasDuration() != other.hasDuration()) return false;
if (hasDuration()) {
if (!getDuration().equals(other.getDuration())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStartTime()) {
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + getStartTime().hashCode();
}
if (hasDuration()) {
hash = (37 * hash) + DURATION_FIELD_NUMBER;
hash = (53 * hash) + getDuration().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.Break prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Data representing the execution of a break.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute.Break}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.ShipmentRoute.Break)
com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Break_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Break_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.ShipmentRoute.Break.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getStartTimeFieldBuilder();
getDurationFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
startTime_ = null;
if (startTimeBuilder_ != null) {
startTimeBuilder_.dispose();
startTimeBuilder_ = null;
}
duration_ = null;
if (durationBuilder_ != null) {
durationBuilder_.dispose();
durationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_Break_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.ShipmentRoute.Break.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break build() {
com.google.maps.routeoptimization.v1.ShipmentRoute.Break result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break buildPartial() {
com.google.maps.routeoptimization.v1.ShipmentRoute.Break result =
new com.google.maps.routeoptimization.v1.ShipmentRoute.Break(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.maps.routeoptimization.v1.ShipmentRoute.Break result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.startTime_ = startTimeBuilder_ == null ? startTime_ : startTimeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.duration_ = durationBuilder_ == null ? duration_ : durationBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.ShipmentRoute.Break) {
return mergeFrom((com.google.maps.routeoptimization.v1.ShipmentRoute.Break) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.maps.routeoptimization.v1.ShipmentRoute.Break other) {
if (other == com.google.maps.routeoptimization.v1.ShipmentRoute.Break.getDefaultInstance())
return this;
if (other.hasStartTime()) {
mergeStartTime(other.getStartTime());
}
if (other.hasDuration()) {
mergeDuration(other.getDuration());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(getStartTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
input.readMessage(getDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.Timestamp startTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
startTimeBuilder_;
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*
* @return Whether the startTime field is set.
*/
public boolean hasStartTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*
* @return The startTime.
*/
public com.google.protobuf.Timestamp getStartTime() {
if (startTimeBuilder_ == null) {
return startTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: startTime_;
} else {
return startTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
public Builder setStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startTime_ = value;
} else {
startTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
public Builder setStartTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (startTimeBuilder_ == null) {
startTime_ = builderForValue.build();
} else {
startTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
public Builder mergeStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& startTime_ != null
&& startTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getStartTimeBuilder().mergeFrom(value);
} else {
startTime_ = value;
}
} else {
startTimeBuilder_.mergeFrom(value);
}
if (startTime_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
public Builder clearStartTime() {
bitField0_ = (bitField0_ & ~0x00000001);
startTime_ = null;
if (startTimeBuilder_ != null) {
startTimeBuilder_.dispose();
startTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
public com.google.protobuf.Timestamp.Builder getStartTimeBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getStartTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
if (startTimeBuilder_ != null) {
return startTimeBuilder_.getMessageOrBuilder();
} else {
return startTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: startTime_;
}
}
/**
*
*
*
* Start time of a break.
*
*
* .google.protobuf.Timestamp start_time = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getStartTimeFieldBuilder() {
if (startTimeBuilder_ == null) {
startTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getStartTime(), getParentForChildren(), isClean());
startTime_ = null;
}
return startTimeBuilder_;
}
private com.google.protobuf.Duration duration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
durationBuilder_;
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return Whether the duration field is set.
*/
public boolean hasDuration() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return The duration.
*/
public com.google.protobuf.Duration getDuration() {
if (durationBuilder_ == null) {
return duration_ == null ? com.google.protobuf.Duration.getDefaultInstance() : duration_;
} else {
return durationBuilder_.getMessage();
}
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
public Builder setDuration(com.google.protobuf.Duration value) {
if (durationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
duration_ = value;
} else {
durationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
public Builder setDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (durationBuilder_ == null) {
duration_ = builderForValue.build();
} else {
durationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
public Builder mergeDuration(com.google.protobuf.Duration value) {
if (durationBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)
&& duration_ != null
&& duration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getDurationBuilder().mergeFrom(value);
} else {
duration_ = value;
}
} else {
durationBuilder_.mergeFrom(value);
}
if (duration_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
public Builder clearDuration() {
bitField0_ = (bitField0_ & ~0x00000002);
duration_ = null;
if (durationBuilder_ != null) {
durationBuilder_.dispose();
durationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
public com.google.protobuf.Duration.Builder getDurationBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
public com.google.protobuf.DurationOrBuilder getDurationOrBuilder() {
if (durationBuilder_ != null) {
return durationBuilder_.getMessageOrBuilder();
} else {
return duration_ == null ? com.google.protobuf.Duration.getDefaultInstance() : duration_;
}
}
/**
*
*
*
* Duration of a break.
*
*
* .google.protobuf.Duration duration = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getDurationFieldBuilder() {
if (durationBuilder_ == null) {
durationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getDuration(), getParentForChildren(), isClean());
duration_ = null;
}
return durationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.ShipmentRoute.Break)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.ShipmentRoute.Break)
private static final com.google.maps.routeoptimization.v1.ShipmentRoute.Break DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.ShipmentRoute.Break();
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute.Break getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Break parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int VEHICLE_INDEX_FIELD_NUMBER = 1;
private int vehicleIndex_ = 0;
/**
*
*
*
* Vehicle performing the route, identified by its index in the source
* `ShipmentModel`.
*
*
* int32 vehicle_index = 1;
*
* @return The vehicleIndex.
*/
@java.lang.Override
public int getVehicleIndex() {
return vehicleIndex_;
}
public static final int VEHICLE_LABEL_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object vehicleLabel_ = "";
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @return The vehicleLabel.
*/
@java.lang.Override
public java.lang.String getVehicleLabel() {
java.lang.Object ref = vehicleLabel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vehicleLabel_ = s;
return s;
}
}
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @return The bytes for vehicleLabel.
*/
@java.lang.Override
public com.google.protobuf.ByteString getVehicleLabelBytes() {
java.lang.Object ref = vehicleLabel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
vehicleLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VEHICLE_START_TIME_FIELD_NUMBER = 5;
private com.google.protobuf.Timestamp vehicleStartTime_;
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*
* @return Whether the vehicleStartTime field is set.
*/
@java.lang.Override
public boolean hasVehicleStartTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*
* @return The vehicleStartTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getVehicleStartTime() {
return vehicleStartTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleStartTime_;
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getVehicleStartTimeOrBuilder() {
return vehicleStartTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleStartTime_;
}
public static final int VEHICLE_END_TIME_FIELD_NUMBER = 6;
private com.google.protobuf.Timestamp vehicleEndTime_;
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*
* @return Whether the vehicleEndTime field is set.
*/
@java.lang.Override
public boolean hasVehicleEndTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*
* @return The vehicleEndTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getVehicleEndTime() {
return vehicleEndTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleEndTime_;
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getVehicleEndTimeOrBuilder() {
return vehicleEndTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleEndTime_;
}
public static final int VISITS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List visits_;
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
@java.lang.Override
public java.util.List getVisitsList() {
return visits_;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
@java.lang.Override
public java.util.List extends com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder>
getVisitsOrBuilderList() {
return visits_;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
@java.lang.Override
public int getVisitsCount() {
return visits_.size();
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit getVisits(int index) {
return visits_.get(index);
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder getVisitsOrBuilder(
int index) {
return visits_.get(index);
}
public static final int TRANSITIONS_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private java.util.List
transitions_;
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
@java.lang.Override
public java.util.List
getTransitionsList() {
return transitions_;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
@java.lang.Override
public java.util.List<
? extends com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder>
getTransitionsOrBuilderList() {
return transitions_;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
@java.lang.Override
public int getTransitionsCount() {
return transitions_.size();
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition getTransitions(int index) {
return transitions_.get(index);
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder
getTransitionsOrBuilder(int index) {
return transitions_.get(index);
}
public static final int HAS_TRAFFIC_INFEASIBILITIES_FIELD_NUMBER = 9;
private boolean hasTrafficInfeasibilities_ = false;
/**
*
*
*
* When
* [OptimizeToursRequest.consider_road_traffic][google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* is set to true, this field indicates that inconsistencies in route timings
* are predicted using traffic-based travel duration estimates. There may be
* insufficient time to complete traffic-adjusted travel, delays, and breaks
* between visits, before the first visit, or after the last visit, while
* still satisfying the visit and vehicle time windows. For example,
*
* ```
* start_time(previous_visit) + duration(previous_visit) +
* travel_duration(previous_visit, next_visit) > start_time(next_visit)
* ```
*
* Arrival at next_visit will likely happen later than its current
* time window due the increased estimate of travel time
* `travel_duration(previous_visit, next_visit)` due to traffic. Also, a break
* may be forced to overlap with a visit due to an increase in travel time
* estimates and visit or break time window restrictions.
*
*
* bool has_traffic_infeasibilities = 9;
*
* @return The hasTrafficInfeasibilities.
*/
@java.lang.Override
public boolean getHasTrafficInfeasibilities() {
return hasTrafficInfeasibilities_;
}
public static final int ROUTE_POLYLINE_FIELD_NUMBER = 10;
private com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline routePolyline_;
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*
* @return Whether the routePolyline field is set.
*/
@java.lang.Override
public boolean hasRoutePolyline() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*
* @return The routePolyline.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline getRoutePolyline() {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.getDefaultInstance()
: routePolyline_;
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder
getRoutePolylineOrBuilder() {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.getDefaultInstance()
: routePolyline_;
}
public static final int BREAKS_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private java.util.List breaks_;
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
@java.lang.Override
public java.util.List getBreaksList() {
return breaks_;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
@java.lang.Override
public java.util.List extends com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder>
getBreaksOrBuilderList() {
return breaks_;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
@java.lang.Override
public int getBreaksCount() {
return breaks_.size();
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break getBreaks(int index) {
return breaks_.get(index);
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder getBreaksOrBuilder(
int index) {
return breaks_.get(index);
}
public static final int METRICS_FIELD_NUMBER = 12;
private com.google.maps.routeoptimization.v1.AggregatedMetrics metrics_;
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*
* @return Whether the metrics field is set.
*/
@java.lang.Override
public boolean hasMetrics() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*
* @return The metrics.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.AggregatedMetrics getMetrics() {
return metrics_ == null
? com.google.maps.routeoptimization.v1.AggregatedMetrics.getDefaultInstance()
: metrics_;
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.AggregatedMetricsOrBuilder getMetricsOrBuilder() {
return metrics_ == null
? com.google.maps.routeoptimization.v1.AggregatedMetrics.getDefaultInstance()
: metrics_;
}
public static final int ROUTE_COSTS_FIELD_NUMBER = 17;
private static final class RouteCostsDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_RouteCostsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.DOUBLE,
0D);
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField routeCosts_;
private com.google.protobuf.MapField internalGetRouteCosts() {
if (routeCosts_ == null) {
return com.google.protobuf.MapField.emptyMapField(RouteCostsDefaultEntryHolder.defaultEntry);
}
return routeCosts_;
}
public int getRouteCostsCount() {
return internalGetRouteCosts().getMap().size();
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public boolean containsRouteCosts(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetRouteCosts().getMap().containsKey(key);
}
/** Use {@link #getRouteCostsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getRouteCosts() {
return getRouteCostsMap();
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public java.util.Map getRouteCostsMap() {
return internalGetRouteCosts().getMap();
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public double getRouteCostsOrDefault(java.lang.String key, double defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetRouteCosts().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public double getRouteCostsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetRouteCosts().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int ROUTE_TOTAL_COST_FIELD_NUMBER = 18;
private double routeTotalCost_ = 0D;
/**
*
*
*
* Total cost of the route. The sum of all costs in the cost map.
*
*
* double route_total_cost = 18;
*
* @return The routeTotalCost.
*/
@java.lang.Override
public double getRouteTotalCost() {
return routeTotalCost_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (vehicleIndex_ != 0) {
output.writeInt32(1, vehicleIndex_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(vehicleLabel_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, vehicleLabel_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(5, getVehicleStartTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(6, getVehicleEndTime());
}
for (int i = 0; i < visits_.size(); i++) {
output.writeMessage(7, visits_.get(i));
}
for (int i = 0; i < transitions_.size(); i++) {
output.writeMessage(8, transitions_.get(i));
}
if (hasTrafficInfeasibilities_ != false) {
output.writeBool(9, hasTrafficInfeasibilities_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(10, getRoutePolyline());
}
for (int i = 0; i < breaks_.size(); i++) {
output.writeMessage(11, breaks_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(12, getMetrics());
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetRouteCosts(), RouteCostsDefaultEntryHolder.defaultEntry, 17);
if (java.lang.Double.doubleToRawLongBits(routeTotalCost_) != 0) {
output.writeDouble(18, routeTotalCost_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (vehicleIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(1, vehicleIndex_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(vehicleLabel_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, vehicleLabel_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(5, getVehicleStartTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getVehicleEndTime());
}
for (int i = 0; i < visits_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(7, visits_.get(i));
}
for (int i = 0; i < transitions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(8, transitions_.get(i));
}
if (hasTrafficInfeasibilities_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(9, hasTrafficInfeasibilities_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(10, getRoutePolyline());
}
for (int i = 0; i < breaks_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(11, breaks_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(12, getMetrics());
}
for (java.util.Map.Entry entry :
internalGetRouteCosts().getMap().entrySet()) {
com.google.protobuf.MapEntry routeCosts__ =
RouteCostsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(17, routeCosts__);
}
if (java.lang.Double.doubleToRawLongBits(routeTotalCost_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(18, routeTotalCost_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.ShipmentRoute)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.ShipmentRoute other =
(com.google.maps.routeoptimization.v1.ShipmentRoute) obj;
if (getVehicleIndex() != other.getVehicleIndex()) return false;
if (!getVehicleLabel().equals(other.getVehicleLabel())) return false;
if (hasVehicleStartTime() != other.hasVehicleStartTime()) return false;
if (hasVehicleStartTime()) {
if (!getVehicleStartTime().equals(other.getVehicleStartTime())) return false;
}
if (hasVehicleEndTime() != other.hasVehicleEndTime()) return false;
if (hasVehicleEndTime()) {
if (!getVehicleEndTime().equals(other.getVehicleEndTime())) return false;
}
if (!getVisitsList().equals(other.getVisitsList())) return false;
if (!getTransitionsList().equals(other.getTransitionsList())) return false;
if (getHasTrafficInfeasibilities() != other.getHasTrafficInfeasibilities()) return false;
if (hasRoutePolyline() != other.hasRoutePolyline()) return false;
if (hasRoutePolyline()) {
if (!getRoutePolyline().equals(other.getRoutePolyline())) return false;
}
if (!getBreaksList().equals(other.getBreaksList())) return false;
if (hasMetrics() != other.hasMetrics()) return false;
if (hasMetrics()) {
if (!getMetrics().equals(other.getMetrics())) return false;
}
if (!internalGetRouteCosts().equals(other.internalGetRouteCosts())) return false;
if (java.lang.Double.doubleToLongBits(getRouteTotalCost())
!= java.lang.Double.doubleToLongBits(other.getRouteTotalCost())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VEHICLE_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getVehicleIndex();
hash = (37 * hash) + VEHICLE_LABEL_FIELD_NUMBER;
hash = (53 * hash) + getVehicleLabel().hashCode();
if (hasVehicleStartTime()) {
hash = (37 * hash) + VEHICLE_START_TIME_FIELD_NUMBER;
hash = (53 * hash) + getVehicleStartTime().hashCode();
}
if (hasVehicleEndTime()) {
hash = (37 * hash) + VEHICLE_END_TIME_FIELD_NUMBER;
hash = (53 * hash) + getVehicleEndTime().hashCode();
}
if (getVisitsCount() > 0) {
hash = (37 * hash) + VISITS_FIELD_NUMBER;
hash = (53 * hash) + getVisitsList().hashCode();
}
if (getTransitionsCount() > 0) {
hash = (37 * hash) + TRANSITIONS_FIELD_NUMBER;
hash = (53 * hash) + getTransitionsList().hashCode();
}
hash = (37 * hash) + HAS_TRAFFIC_INFEASIBILITIES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getHasTrafficInfeasibilities());
if (hasRoutePolyline()) {
hash = (37 * hash) + ROUTE_POLYLINE_FIELD_NUMBER;
hash = (53 * hash) + getRoutePolyline().hashCode();
}
if (getBreaksCount() > 0) {
hash = (37 * hash) + BREAKS_FIELD_NUMBER;
hash = (53 * hash) + getBreaksList().hashCode();
}
if (hasMetrics()) {
hash = (37 * hash) + METRICS_FIELD_NUMBER;
hash = (53 * hash) + getMetrics().hashCode();
}
if (!internalGetRouteCosts().getMap().isEmpty()) {
hash = (37 * hash) + ROUTE_COSTS_FIELD_NUMBER;
hash = (53 * hash) + internalGetRouteCosts().hashCode();
}
hash = (37 * hash) + ROUTE_TOTAL_COST_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getRouteTotalCost()));
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.maps.routeoptimization.v1.ShipmentRoute prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A vehicle's route can be decomposed, along the time axis, like this (we
* assume there are n visits):
* ```
* | | | | | T[2], | | |
* | Transition | Visit #0 | | | V[2], | | |
* | #0 | aka | T[1] | V[1] | ... | V[n-1] | T[n] |
* | aka T[0] | V[0] | | | V[n-2],| | |
* | | | | | T[n-1] | | |
* ^ ^ ^ ^ ^ ^ ^ ^
* vehicle V[0].start V[0].end V[1]. V[1]. V[n]. V[n]. vehicle
* start (arrival) (departure) start end start end end
* ```
* Note that we make a difference between:
*
* * "punctual events", such as the vehicle start and end and each visit's start
* and end (aka arrival and departure). They happen at a given second.
* * "time intervals", such as the visits themselves, and the transition between
* visits. Though time intervals can sometimes have zero duration, i.e. start
* and end at the same second, they often have a positive duration.
*
* Invariants:
*
* * If there are n visits, there are n+1 transitions.
* * A visit is always surrounded by a transition before it (same index) and a
* transition after it (index + 1).
* * The vehicle start is always followed by transition #0.
* * The vehicle end is always preceded by transition #n.
*
* Zooming in, here is what happens during a `Transition` and a `Visit`:
* ```
* ---+-------------------------------------+-----------------------------+-->
* | TRANSITION[i] | VISIT[i] |
* | | |
* | * TRAVEL: the vehicle moves from | PERFORM the visit: |
* | VISIT[i-1].departure_location to | |
* | VISIT[i].arrival_location, which | * Spend some time: |
* | takes a given travel duration | the "visit duration". |
* | and distance | |
* | | * Load or unload |
* | * BREAKS: the driver may have | some quantities from the |
* | breaks (e.g. lunch break). | vehicle: the "demand". |
* | | |
* | * WAIT: the driver/vehicle does | |
* | nothing. This can happen for | |
* | many reasons, for example when | |
* | the vehicle reaches the next | |
* | event's destination before the | |
* | start of its time window | |
* | | |
* | * DELAY: *right before* the next | |
* | arrival. E.g. the vehicle and/or | |
* | driver spends time unloading. | |
* | | |
* ---+-------------------------------------+-----------------------------+-->
* ^ ^ ^
* V[i-1].end V[i].start V[i].end
* ```
* Lastly, here is how the TRAVEL, BREAKS, DELAY and WAIT can be arranged
* during a transition.
*
* * They don't overlap.
* * The DELAY is unique and *must* be a contiguous period of time right
* before the next visit (or vehicle end). Thus, it suffice to know the
* delay duration to know its start and end time.
* * The BREAKS are contiguous, non-overlapping periods of time. The
* response specifies the start time and duration of each break.
* * TRAVEL and WAIT are "preemptable": they can be interrupted several times
* during this transition. Clients can assume that travel happens "as soon as
* possible" and that "wait" fills the remaining time.
*
* A (complex) example:
* ```
* TRANSITION[i]
* --++-----+-----------------------------------------------------------++-->
* || | | | | | | ||
* || T | B | T | | B | | D ||
* || r | r | r | W | r | W | e ||
* || a | e | a | a | e | a | l ||
* || v | a | v | i | a | i | a ||
* || e | k | e | t | k | t | y ||
* || l | | l | | | | ||
* || | | | | | | ||
* --++-----------------------------------------------------------------++-->
* ```
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.ShipmentRoute}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.ShipmentRoute)
com.google.maps.routeoptimization.v1.ShipmentRouteOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 17:
return internalGetRouteCosts();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 17:
return internalGetMutableRouteCosts();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.ShipmentRoute.class,
com.google.maps.routeoptimization.v1.ShipmentRoute.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.ShipmentRoute.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getVehicleStartTimeFieldBuilder();
getVehicleEndTimeFieldBuilder();
getVisitsFieldBuilder();
getTransitionsFieldBuilder();
getRoutePolylineFieldBuilder();
getBreaksFieldBuilder();
getMetricsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
vehicleIndex_ = 0;
vehicleLabel_ = "";
vehicleStartTime_ = null;
if (vehicleStartTimeBuilder_ != null) {
vehicleStartTimeBuilder_.dispose();
vehicleStartTimeBuilder_ = null;
}
vehicleEndTime_ = null;
if (vehicleEndTimeBuilder_ != null) {
vehicleEndTimeBuilder_.dispose();
vehicleEndTimeBuilder_ = null;
}
if (visitsBuilder_ == null) {
visits_ = java.util.Collections.emptyList();
} else {
visits_ = null;
visitsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (transitionsBuilder_ == null) {
transitions_ = java.util.Collections.emptyList();
} else {
transitions_ = null;
transitionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
hasTrafficInfeasibilities_ = false;
routePolyline_ = null;
if (routePolylineBuilder_ != null) {
routePolylineBuilder_.dispose();
routePolylineBuilder_ = null;
}
if (breaksBuilder_ == null) {
breaks_ = java.util.Collections.emptyList();
} else {
breaks_ = null;
breaksBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
metrics_ = null;
if (metricsBuilder_ != null) {
metricsBuilder_.dispose();
metricsBuilder_ = null;
}
internalGetMutableRouteCosts().clear();
routeTotalCost_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_ShipmentRoute_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.ShipmentRoute.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute build() {
com.google.maps.routeoptimization.v1.ShipmentRoute result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute buildPartial() {
com.google.maps.routeoptimization.v1.ShipmentRoute result =
new com.google.maps.routeoptimization.v1.ShipmentRoute(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.maps.routeoptimization.v1.ShipmentRoute result) {
if (visitsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
visits_ = java.util.Collections.unmodifiableList(visits_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.visits_ = visits_;
} else {
result.visits_ = visitsBuilder_.build();
}
if (transitionsBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
transitions_ = java.util.Collections.unmodifiableList(transitions_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.transitions_ = transitions_;
} else {
result.transitions_ = transitionsBuilder_.build();
}
if (breaksBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
breaks_ = java.util.Collections.unmodifiableList(breaks_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.breaks_ = breaks_;
} else {
result.breaks_ = breaksBuilder_.build();
}
}
private void buildPartial0(com.google.maps.routeoptimization.v1.ShipmentRoute result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.vehicleIndex_ = vehicleIndex_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.vehicleLabel_ = vehicleLabel_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.vehicleStartTime_ =
vehicleStartTimeBuilder_ == null ? vehicleStartTime_ : vehicleStartTimeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.vehicleEndTime_ =
vehicleEndTimeBuilder_ == null ? vehicleEndTime_ : vehicleEndTimeBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.hasTrafficInfeasibilities_ = hasTrafficInfeasibilities_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.routePolyline_ =
routePolylineBuilder_ == null ? routePolyline_ : routePolylineBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.metrics_ = metricsBuilder_ == null ? metrics_ : metricsBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.routeCosts_ = internalGetRouteCosts();
result.routeCosts_.makeImmutable();
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.routeTotalCost_ = routeTotalCost_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.ShipmentRoute) {
return mergeFrom((com.google.maps.routeoptimization.v1.ShipmentRoute) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.maps.routeoptimization.v1.ShipmentRoute other) {
if (other == com.google.maps.routeoptimization.v1.ShipmentRoute.getDefaultInstance())
return this;
if (other.getVehicleIndex() != 0) {
setVehicleIndex(other.getVehicleIndex());
}
if (!other.getVehicleLabel().isEmpty()) {
vehicleLabel_ = other.vehicleLabel_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasVehicleStartTime()) {
mergeVehicleStartTime(other.getVehicleStartTime());
}
if (other.hasVehicleEndTime()) {
mergeVehicleEndTime(other.getVehicleEndTime());
}
if (visitsBuilder_ == null) {
if (!other.visits_.isEmpty()) {
if (visits_.isEmpty()) {
visits_ = other.visits_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureVisitsIsMutable();
visits_.addAll(other.visits_);
}
onChanged();
}
} else {
if (!other.visits_.isEmpty()) {
if (visitsBuilder_.isEmpty()) {
visitsBuilder_.dispose();
visitsBuilder_ = null;
visits_ = other.visits_;
bitField0_ = (bitField0_ & ~0x00000010);
visitsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getVisitsFieldBuilder()
: null;
} else {
visitsBuilder_.addAllMessages(other.visits_);
}
}
}
if (transitionsBuilder_ == null) {
if (!other.transitions_.isEmpty()) {
if (transitions_.isEmpty()) {
transitions_ = other.transitions_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureTransitionsIsMutable();
transitions_.addAll(other.transitions_);
}
onChanged();
}
} else {
if (!other.transitions_.isEmpty()) {
if (transitionsBuilder_.isEmpty()) {
transitionsBuilder_.dispose();
transitionsBuilder_ = null;
transitions_ = other.transitions_;
bitField0_ = (bitField0_ & ~0x00000020);
transitionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getTransitionsFieldBuilder()
: null;
} else {
transitionsBuilder_.addAllMessages(other.transitions_);
}
}
}
if (other.getHasTrafficInfeasibilities() != false) {
setHasTrafficInfeasibilities(other.getHasTrafficInfeasibilities());
}
if (other.hasRoutePolyline()) {
mergeRoutePolyline(other.getRoutePolyline());
}
if (breaksBuilder_ == null) {
if (!other.breaks_.isEmpty()) {
if (breaks_.isEmpty()) {
breaks_ = other.breaks_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureBreaksIsMutable();
breaks_.addAll(other.breaks_);
}
onChanged();
}
} else {
if (!other.breaks_.isEmpty()) {
if (breaksBuilder_.isEmpty()) {
breaksBuilder_.dispose();
breaksBuilder_ = null;
breaks_ = other.breaks_;
bitField0_ = (bitField0_ & ~0x00000100);
breaksBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getBreaksFieldBuilder()
: null;
} else {
breaksBuilder_.addAllMessages(other.breaks_);
}
}
}
if (other.hasMetrics()) {
mergeMetrics(other.getMetrics());
}
internalGetMutableRouteCosts().mergeFrom(other.internalGetRouteCosts());
bitField0_ |= 0x00000400;
if (other.getRouteTotalCost() != 0D) {
setRouteTotalCost(other.getRouteTotalCost());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
vehicleIndex_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18:
{
vehicleLabel_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 42:
{
input.readMessage(
getVehicleStartTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 42
case 50:
{
input.readMessage(getVehicleEndTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 50
case 58:
{
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit m =
input.readMessage(
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.parser(),
extensionRegistry);
if (visitsBuilder_ == null) {
ensureVisitsIsMutable();
visits_.add(m);
} else {
visitsBuilder_.addMessage(m);
}
break;
} // case 58
case 66:
{
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition m =
input.readMessage(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.parser(),
extensionRegistry);
if (transitionsBuilder_ == null) {
ensureTransitionsIsMutable();
transitions_.add(m);
} else {
transitionsBuilder_.addMessage(m);
}
break;
} // case 66
case 72:
{
hasTrafficInfeasibilities_ = input.readBool();
bitField0_ |= 0x00000040;
break;
} // case 72
case 82:
{
input.readMessage(getRoutePolylineFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 82
case 90:
{
com.google.maps.routeoptimization.v1.ShipmentRoute.Break m =
input.readMessage(
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.parser(),
extensionRegistry);
if (breaksBuilder_ == null) {
ensureBreaksIsMutable();
breaks_.add(m);
} else {
breaksBuilder_.addMessage(m);
}
break;
} // case 90
case 98:
{
input.readMessage(getMetricsFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 98
case 138:
{
com.google.protobuf.MapEntry routeCosts__ =
input.readMessage(
RouteCostsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableRouteCosts()
.getMutableMap()
.put(routeCosts__.getKey(), routeCosts__.getValue());
bitField0_ |= 0x00000400;
break;
} // case 138
case 145:
{
routeTotalCost_ = input.readDouble();
bitField0_ |= 0x00000800;
break;
} // case 145
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int vehicleIndex_;
/**
*
*
*
* Vehicle performing the route, identified by its index in the source
* `ShipmentModel`.
*
*
* int32 vehicle_index = 1;
*
* @return The vehicleIndex.
*/
@java.lang.Override
public int getVehicleIndex() {
return vehicleIndex_;
}
/**
*
*
*
* Vehicle performing the route, identified by its index in the source
* `ShipmentModel`.
*
*
* int32 vehicle_index = 1;
*
* @param value The vehicleIndex to set.
* @return This builder for chaining.
*/
public Builder setVehicleIndex(int value) {
vehicleIndex_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Vehicle performing the route, identified by its index in the source
* `ShipmentModel`.
*
*
* int32 vehicle_index = 1;
*
* @return This builder for chaining.
*/
public Builder clearVehicleIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
vehicleIndex_ = 0;
onChanged();
return this;
}
private java.lang.Object vehicleLabel_ = "";
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @return The vehicleLabel.
*/
public java.lang.String getVehicleLabel() {
java.lang.Object ref = vehicleLabel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vehicleLabel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @return The bytes for vehicleLabel.
*/
public com.google.protobuf.ByteString getVehicleLabelBytes() {
java.lang.Object ref = vehicleLabel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
vehicleLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @param value The vehicleLabel to set.
* @return This builder for chaining.
*/
public Builder setVehicleLabel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
vehicleLabel_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @return This builder for chaining.
*/
public Builder clearVehicleLabel() {
vehicleLabel_ = getDefaultInstance().getVehicleLabel();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @param value The bytes for vehicleLabel to set.
* @return This builder for chaining.
*/
public Builder setVehicleLabelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
vehicleLabel_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.Timestamp vehicleStartTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
vehicleStartTimeBuilder_;
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*
* @return Whether the vehicleStartTime field is set.
*/
public boolean hasVehicleStartTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*
* @return The vehicleStartTime.
*/
public com.google.protobuf.Timestamp getVehicleStartTime() {
if (vehicleStartTimeBuilder_ == null) {
return vehicleStartTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleStartTime_;
} else {
return vehicleStartTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
public Builder setVehicleStartTime(com.google.protobuf.Timestamp value) {
if (vehicleStartTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
vehicleStartTime_ = value;
} else {
vehicleStartTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
public Builder setVehicleStartTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (vehicleStartTimeBuilder_ == null) {
vehicleStartTime_ = builderForValue.build();
} else {
vehicleStartTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
public Builder mergeVehicleStartTime(com.google.protobuf.Timestamp value) {
if (vehicleStartTimeBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& vehicleStartTime_ != null
&& vehicleStartTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getVehicleStartTimeBuilder().mergeFrom(value);
} else {
vehicleStartTime_ = value;
}
} else {
vehicleStartTimeBuilder_.mergeFrom(value);
}
if (vehicleStartTime_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
public Builder clearVehicleStartTime() {
bitField0_ = (bitField0_ & ~0x00000004);
vehicleStartTime_ = null;
if (vehicleStartTimeBuilder_ != null) {
vehicleStartTimeBuilder_.dispose();
vehicleStartTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
public com.google.protobuf.Timestamp.Builder getVehicleStartTimeBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getVehicleStartTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
public com.google.protobuf.TimestampOrBuilder getVehicleStartTimeOrBuilder() {
if (vehicleStartTimeBuilder_ != null) {
return vehicleStartTimeBuilder_.getMessageOrBuilder();
} else {
return vehicleStartTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleStartTime_;
}
}
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getVehicleStartTimeFieldBuilder() {
if (vehicleStartTimeBuilder_ == null) {
vehicleStartTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getVehicleStartTime(), getParentForChildren(), isClean());
vehicleStartTime_ = null;
}
return vehicleStartTimeBuilder_;
}
private com.google.protobuf.Timestamp vehicleEndTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
vehicleEndTimeBuilder_;
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*
* @return Whether the vehicleEndTime field is set.
*/
public boolean hasVehicleEndTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*
* @return The vehicleEndTime.
*/
public com.google.protobuf.Timestamp getVehicleEndTime() {
if (vehicleEndTimeBuilder_ == null) {
return vehicleEndTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleEndTime_;
} else {
return vehicleEndTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
public Builder setVehicleEndTime(com.google.protobuf.Timestamp value) {
if (vehicleEndTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
vehicleEndTime_ = value;
} else {
vehicleEndTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
public Builder setVehicleEndTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (vehicleEndTimeBuilder_ == null) {
vehicleEndTime_ = builderForValue.build();
} else {
vehicleEndTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
public Builder mergeVehicleEndTime(com.google.protobuf.Timestamp value) {
if (vehicleEndTimeBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& vehicleEndTime_ != null
&& vehicleEndTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getVehicleEndTimeBuilder().mergeFrom(value);
} else {
vehicleEndTime_ = value;
}
} else {
vehicleEndTimeBuilder_.mergeFrom(value);
}
if (vehicleEndTime_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
public Builder clearVehicleEndTime() {
bitField0_ = (bitField0_ & ~0x00000008);
vehicleEndTime_ = null;
if (vehicleEndTimeBuilder_ != null) {
vehicleEndTimeBuilder_.dispose();
vehicleEndTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
public com.google.protobuf.Timestamp.Builder getVehicleEndTimeBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getVehicleEndTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
public com.google.protobuf.TimestampOrBuilder getVehicleEndTimeOrBuilder() {
if (vehicleEndTimeBuilder_ != null) {
return vehicleEndTimeBuilder_.getMessageOrBuilder();
} else {
return vehicleEndTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: vehicleEndTime_;
}
}
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getVehicleEndTimeFieldBuilder() {
if (vehicleEndTimeBuilder_ == null) {
vehicleEndTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getVehicleEndTime(), getParentForChildren(), isClean());
vehicleEndTime_ = null;
}
return vehicleEndTimeBuilder_;
}
private java.util.List visits_ =
java.util.Collections.emptyList();
private void ensureVisitsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
visits_ =
new java.util.ArrayList(
visits_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit,
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder>
visitsBuilder_;
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public java.util.List
getVisitsList() {
if (visitsBuilder_ == null) {
return java.util.Collections.unmodifiableList(visits_);
} else {
return visitsBuilder_.getMessageList();
}
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public int getVisitsCount() {
if (visitsBuilder_ == null) {
return visits_.size();
} else {
return visitsBuilder_.getCount();
}
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit getVisits(int index) {
if (visitsBuilder_ == null) {
return visits_.get(index);
} else {
return visitsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder setVisits(
int index, com.google.maps.routeoptimization.v1.ShipmentRoute.Visit value) {
if (visitsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVisitsIsMutable();
visits_.set(index, value);
onChanged();
} else {
visitsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder setVisits(
int index,
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder builderForValue) {
if (visitsBuilder_ == null) {
ensureVisitsIsMutable();
visits_.set(index, builderForValue.build());
onChanged();
} else {
visitsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder addVisits(com.google.maps.routeoptimization.v1.ShipmentRoute.Visit value) {
if (visitsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVisitsIsMutable();
visits_.add(value);
onChanged();
} else {
visitsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder addVisits(
int index, com.google.maps.routeoptimization.v1.ShipmentRoute.Visit value) {
if (visitsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVisitsIsMutable();
visits_.add(index, value);
onChanged();
} else {
visitsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder addVisits(
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder builderForValue) {
if (visitsBuilder_ == null) {
ensureVisitsIsMutable();
visits_.add(builderForValue.build());
onChanged();
} else {
visitsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder addVisits(
int index,
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder builderForValue) {
if (visitsBuilder_ == null) {
ensureVisitsIsMutable();
visits_.add(index, builderForValue.build());
onChanged();
} else {
visitsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder addAllVisits(
java.lang.Iterable extends com.google.maps.routeoptimization.v1.ShipmentRoute.Visit>
values) {
if (visitsBuilder_ == null) {
ensureVisitsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, visits_);
onChanged();
} else {
visitsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder clearVisits() {
if (visitsBuilder_ == null) {
visits_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
visitsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public Builder removeVisits(int index) {
if (visitsBuilder_ == null) {
ensureVisitsIsMutable();
visits_.remove(index);
onChanged();
} else {
visitsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder getVisitsBuilder(
int index) {
return getVisitsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder getVisitsOrBuilder(
int index) {
if (visitsBuilder_ == null) {
return visits_.get(index);
} else {
return visitsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public java.util.List<
? extends com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder>
getVisitsOrBuilderList() {
if (visitsBuilder_ != null) {
return visitsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(visits_);
}
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder addVisitsBuilder() {
return getVisitsFieldBuilder()
.addBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.getDefaultInstance());
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder addVisitsBuilder(
int index) {
return getVisitsFieldBuilder()
.addBuilder(
index, com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.getDefaultInstance());
}
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
public java.util.List
getVisitsBuilderList() {
return getVisitsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit,
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder>
getVisitsFieldBuilder() {
if (visitsBuilder_ == null) {
visitsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit,
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder>(
visits_, ((bitField0_ & 0x00000010) != 0), getParentForChildren(), isClean());
visits_ = null;
}
return visitsBuilder_;
}
private java.util.List
transitions_ = java.util.Collections.emptyList();
private void ensureTransitionsIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
transitions_ =
new java.util.ArrayList(
transitions_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder>
transitionsBuilder_;
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public java.util.List
getTransitionsList() {
if (transitionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(transitions_);
} else {
return transitionsBuilder_.getMessageList();
}
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public int getTransitionsCount() {
if (transitionsBuilder_ == null) {
return transitions_.size();
} else {
return transitionsBuilder_.getCount();
}
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition getTransitions(int index) {
if (transitionsBuilder_ == null) {
return transitions_.get(index);
} else {
return transitionsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder setTransitions(
int index, com.google.maps.routeoptimization.v1.ShipmentRoute.Transition value) {
if (transitionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransitionsIsMutable();
transitions_.set(index, value);
onChanged();
} else {
transitionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder setTransitions(
int index,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder builderForValue) {
if (transitionsBuilder_ == null) {
ensureTransitionsIsMutable();
transitions_.set(index, builderForValue.build());
onChanged();
} else {
transitionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder addTransitions(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition value) {
if (transitionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransitionsIsMutable();
transitions_.add(value);
onChanged();
} else {
transitionsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder addTransitions(
int index, com.google.maps.routeoptimization.v1.ShipmentRoute.Transition value) {
if (transitionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransitionsIsMutable();
transitions_.add(index, value);
onChanged();
} else {
transitionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder addTransitions(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder builderForValue) {
if (transitionsBuilder_ == null) {
ensureTransitionsIsMutable();
transitions_.add(builderForValue.build());
onChanged();
} else {
transitionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder addTransitions(
int index,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder builderForValue) {
if (transitionsBuilder_ == null) {
ensureTransitionsIsMutable();
transitions_.add(index, builderForValue.build());
onChanged();
} else {
transitionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder addAllTransitions(
java.lang.Iterable extends com.google.maps.routeoptimization.v1.ShipmentRoute.Transition>
values) {
if (transitionsBuilder_ == null) {
ensureTransitionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, transitions_);
onChanged();
} else {
transitionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder clearTransitions() {
if (transitionsBuilder_ == null) {
transitions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
transitionsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public Builder removeTransitions(int index) {
if (transitionsBuilder_ == null) {
ensureTransitionsIsMutable();
transitions_.remove(index);
onChanged();
} else {
transitionsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder
getTransitionsBuilder(int index) {
return getTransitionsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder
getTransitionsOrBuilder(int index) {
if (transitionsBuilder_ == null) {
return transitions_.get(index);
} else {
return transitionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public java.util.List<
? extends com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder>
getTransitionsOrBuilderList() {
if (transitionsBuilder_ != null) {
return transitionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(transitions_);
}
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder
addTransitionsBuilder() {
return getTransitionsFieldBuilder()
.addBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.getDefaultInstance());
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder
addTransitionsBuilder(int index) {
return getTransitionsFieldBuilder()
.addBuilder(
index,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.getDefaultInstance());
}
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
public java.util.List
getTransitionsBuilderList() {
return getTransitionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder>
getTransitionsFieldBuilder() {
if (transitionsBuilder_ == null) {
transitionsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition,
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder>(
transitions_, ((bitField0_ & 0x00000020) != 0), getParentForChildren(), isClean());
transitions_ = null;
}
return transitionsBuilder_;
}
private boolean hasTrafficInfeasibilities_;
/**
*
*
*
* When
* [OptimizeToursRequest.consider_road_traffic][google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* is set to true, this field indicates that inconsistencies in route timings
* are predicted using traffic-based travel duration estimates. There may be
* insufficient time to complete traffic-adjusted travel, delays, and breaks
* between visits, before the first visit, or after the last visit, while
* still satisfying the visit and vehicle time windows. For example,
*
* ```
* start_time(previous_visit) + duration(previous_visit) +
* travel_duration(previous_visit, next_visit) > start_time(next_visit)
* ```
*
* Arrival at next_visit will likely happen later than its current
* time window due the increased estimate of travel time
* `travel_duration(previous_visit, next_visit)` due to traffic. Also, a break
* may be forced to overlap with a visit due to an increase in travel time
* estimates and visit or break time window restrictions.
*
*
* bool has_traffic_infeasibilities = 9;
*
* @return The hasTrafficInfeasibilities.
*/
@java.lang.Override
public boolean getHasTrafficInfeasibilities() {
return hasTrafficInfeasibilities_;
}
/**
*
*
*
* When
* [OptimizeToursRequest.consider_road_traffic][google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* is set to true, this field indicates that inconsistencies in route timings
* are predicted using traffic-based travel duration estimates. There may be
* insufficient time to complete traffic-adjusted travel, delays, and breaks
* between visits, before the first visit, or after the last visit, while
* still satisfying the visit and vehicle time windows. For example,
*
* ```
* start_time(previous_visit) + duration(previous_visit) +
* travel_duration(previous_visit, next_visit) > start_time(next_visit)
* ```
*
* Arrival at next_visit will likely happen later than its current
* time window due the increased estimate of travel time
* `travel_duration(previous_visit, next_visit)` due to traffic. Also, a break
* may be forced to overlap with a visit due to an increase in travel time
* estimates and visit or break time window restrictions.
*
*
* bool has_traffic_infeasibilities = 9;
*
* @param value The hasTrafficInfeasibilities to set.
* @return This builder for chaining.
*/
public Builder setHasTrafficInfeasibilities(boolean value) {
hasTrafficInfeasibilities_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* When
* [OptimizeToursRequest.consider_road_traffic][google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* is set to true, this field indicates that inconsistencies in route timings
* are predicted using traffic-based travel duration estimates. There may be
* insufficient time to complete traffic-adjusted travel, delays, and breaks
* between visits, before the first visit, or after the last visit, while
* still satisfying the visit and vehicle time windows. For example,
*
* ```
* start_time(previous_visit) + duration(previous_visit) +
* travel_duration(previous_visit, next_visit) > start_time(next_visit)
* ```
*
* Arrival at next_visit will likely happen later than its current
* time window due the increased estimate of travel time
* `travel_duration(previous_visit, next_visit)` due to traffic. Also, a break
* may be forced to overlap with a visit due to an increase in travel time
* estimates and visit or break time window restrictions.
*
*
* bool has_traffic_infeasibilities = 9;
*
* @return This builder for chaining.
*/
public Builder clearHasTrafficInfeasibilities() {
bitField0_ = (bitField0_ & ~0x00000040);
hasTrafficInfeasibilities_ = false;
onChanged();
return this;
}
private com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline routePolyline_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder>
routePolylineBuilder_;
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*
* @return Whether the routePolyline field is set.
*/
public boolean hasRoutePolyline() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*
* @return The routePolyline.
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline getRoutePolyline() {
if (routePolylineBuilder_ == null) {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance()
: routePolyline_;
} else {
return routePolylineBuilder_.getMessage();
}
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
public Builder setRoutePolyline(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline value) {
if (routePolylineBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
routePolyline_ = value;
} else {
routePolylineBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
public Builder setRoutePolyline(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder
builderForValue) {
if (routePolylineBuilder_ == null) {
routePolyline_ = builderForValue.build();
} else {
routePolylineBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
public Builder mergeRoutePolyline(
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline value) {
if (routePolylineBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& routePolyline_ != null
&& routePolyline_
!= com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance()) {
getRoutePolylineBuilder().mergeFrom(value);
} else {
routePolyline_ = value;
}
} else {
routePolylineBuilder_.mergeFrom(value);
}
if (routePolyline_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
public Builder clearRoutePolyline() {
bitField0_ = (bitField0_ & ~0x00000080);
routePolyline_ = null;
if (routePolylineBuilder_ != null) {
routePolylineBuilder_.dispose();
routePolylineBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder
getRoutePolylineBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getRoutePolylineFieldBuilder().getBuilder();
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder
getRoutePolylineOrBuilder() {
if (routePolylineBuilder_ != null) {
return routePolylineBuilder_.getMessageOrBuilder();
} else {
return routePolyline_ == null
? com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline
.getDefaultInstance()
: routePolyline_;
}
}
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder>
getRoutePolylineFieldBuilder() {
if (routePolylineBuilder_ == null) {
routePolylineBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder>(
getRoutePolyline(), getParentForChildren(), isClean());
routePolyline_ = null;
}
return routePolylineBuilder_;
}
private java.util.List breaks_ =
java.util.Collections.emptyList();
private void ensureBreaksIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
breaks_ =
new java.util.ArrayList(
breaks_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Break,
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder>
breaksBuilder_;
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public java.util.List
getBreaksList() {
if (breaksBuilder_ == null) {
return java.util.Collections.unmodifiableList(breaks_);
} else {
return breaksBuilder_.getMessageList();
}
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public int getBreaksCount() {
if (breaksBuilder_ == null) {
return breaks_.size();
} else {
return breaksBuilder_.getCount();
}
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break getBreaks(int index) {
if (breaksBuilder_ == null) {
return breaks_.get(index);
} else {
return breaksBuilder_.getMessage(index);
}
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder setBreaks(
int index, com.google.maps.routeoptimization.v1.ShipmentRoute.Break value) {
if (breaksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBreaksIsMutable();
breaks_.set(index, value);
onChanged();
} else {
breaksBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder setBreaks(
int index,
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder builderForValue) {
if (breaksBuilder_ == null) {
ensureBreaksIsMutable();
breaks_.set(index, builderForValue.build());
onChanged();
} else {
breaksBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder addBreaks(com.google.maps.routeoptimization.v1.ShipmentRoute.Break value) {
if (breaksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBreaksIsMutable();
breaks_.add(value);
onChanged();
} else {
breaksBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder addBreaks(
int index, com.google.maps.routeoptimization.v1.ShipmentRoute.Break value) {
if (breaksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBreaksIsMutable();
breaks_.add(index, value);
onChanged();
} else {
breaksBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder addBreaks(
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder builderForValue) {
if (breaksBuilder_ == null) {
ensureBreaksIsMutable();
breaks_.add(builderForValue.build());
onChanged();
} else {
breaksBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder addBreaks(
int index,
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder builderForValue) {
if (breaksBuilder_ == null) {
ensureBreaksIsMutable();
breaks_.add(index, builderForValue.build());
onChanged();
} else {
breaksBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder addAllBreaks(
java.lang.Iterable extends com.google.maps.routeoptimization.v1.ShipmentRoute.Break>
values) {
if (breaksBuilder_ == null) {
ensureBreaksIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, breaks_);
onChanged();
} else {
breaksBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder clearBreaks() {
if (breaksBuilder_ == null) {
breaks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
breaksBuilder_.clear();
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public Builder removeBreaks(int index) {
if (breaksBuilder_ == null) {
ensureBreaksIsMutable();
breaks_.remove(index);
onChanged();
} else {
breaksBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder getBreaksBuilder(
int index) {
return getBreaksFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder getBreaksOrBuilder(
int index) {
if (breaksBuilder_ == null) {
return breaks_.get(index);
} else {
return breaksBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public java.util.List<
? extends com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder>
getBreaksOrBuilderList() {
if (breaksBuilder_ != null) {
return breaksBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(breaks_);
}
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder addBreaksBuilder() {
return getBreaksFieldBuilder()
.addBuilder(
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.getDefaultInstance());
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder addBreaksBuilder(
int index) {
return getBreaksFieldBuilder()
.addBuilder(
index, com.google.maps.routeoptimization.v1.ShipmentRoute.Break.getDefaultInstance());
}
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
public java.util.List
getBreaksBuilderList() {
return getBreaksFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Break,
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder>
getBreaksFieldBuilder() {
if (breaksBuilder_ == null) {
breaksBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.ShipmentRoute.Break,
com.google.maps.routeoptimization.v1.ShipmentRoute.Break.Builder,
com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder>(
breaks_, ((bitField0_ & 0x00000100) != 0), getParentForChildren(), isClean());
breaks_ = null;
}
return breaksBuilder_;
}
private com.google.maps.routeoptimization.v1.AggregatedMetrics metrics_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.AggregatedMetrics,
com.google.maps.routeoptimization.v1.AggregatedMetrics.Builder,
com.google.maps.routeoptimization.v1.AggregatedMetricsOrBuilder>
metricsBuilder_;
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*
* @return Whether the metrics field is set.
*/
public boolean hasMetrics() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*
* @return The metrics.
*/
public com.google.maps.routeoptimization.v1.AggregatedMetrics getMetrics() {
if (metricsBuilder_ == null) {
return metrics_ == null
? com.google.maps.routeoptimization.v1.AggregatedMetrics.getDefaultInstance()
: metrics_;
} else {
return metricsBuilder_.getMessage();
}
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
public Builder setMetrics(com.google.maps.routeoptimization.v1.AggregatedMetrics value) {
if (metricsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
metrics_ = value;
} else {
metricsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
public Builder setMetrics(
com.google.maps.routeoptimization.v1.AggregatedMetrics.Builder builderForValue) {
if (metricsBuilder_ == null) {
metrics_ = builderForValue.build();
} else {
metricsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
public Builder mergeMetrics(com.google.maps.routeoptimization.v1.AggregatedMetrics value) {
if (metricsBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)
&& metrics_ != null
&& metrics_
!= com.google.maps.routeoptimization.v1.AggregatedMetrics.getDefaultInstance()) {
getMetricsBuilder().mergeFrom(value);
} else {
metrics_ = value;
}
} else {
metricsBuilder_.mergeFrom(value);
}
if (metrics_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
public Builder clearMetrics() {
bitField0_ = (bitField0_ & ~0x00000200);
metrics_ = null;
if (metricsBuilder_ != null) {
metricsBuilder_.dispose();
metricsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
public com.google.maps.routeoptimization.v1.AggregatedMetrics.Builder getMetricsBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getMetricsFieldBuilder().getBuilder();
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
public com.google.maps.routeoptimization.v1.AggregatedMetricsOrBuilder getMetricsOrBuilder() {
if (metricsBuilder_ != null) {
return metricsBuilder_.getMessageOrBuilder();
} else {
return metrics_ == null
? com.google.maps.routeoptimization.v1.AggregatedMetrics.getDefaultInstance()
: metrics_;
}
}
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.AggregatedMetrics,
com.google.maps.routeoptimization.v1.AggregatedMetrics.Builder,
com.google.maps.routeoptimization.v1.AggregatedMetricsOrBuilder>
getMetricsFieldBuilder() {
if (metricsBuilder_ == null) {
metricsBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.AggregatedMetrics,
com.google.maps.routeoptimization.v1.AggregatedMetrics.Builder,
com.google.maps.routeoptimization.v1.AggregatedMetricsOrBuilder>(
getMetrics(), getParentForChildren(), isClean());
metrics_ = null;
}
return metricsBuilder_;
}
private com.google.protobuf.MapField routeCosts_;
private com.google.protobuf.MapField
internalGetRouteCosts() {
if (routeCosts_ == null) {
return com.google.protobuf.MapField.emptyMapField(
RouteCostsDefaultEntryHolder.defaultEntry);
}
return routeCosts_;
}
private com.google.protobuf.MapField
internalGetMutableRouteCosts() {
if (routeCosts_ == null) {
routeCosts_ =
com.google.protobuf.MapField.newMapField(RouteCostsDefaultEntryHolder.defaultEntry);
}
if (!routeCosts_.isMutable()) {
routeCosts_ = routeCosts_.copy();
}
bitField0_ |= 0x00000400;
onChanged();
return routeCosts_;
}
public int getRouteCostsCount() {
return internalGetRouteCosts().getMap().size();
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public boolean containsRouteCosts(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetRouteCosts().getMap().containsKey(key);
}
/** Use {@link #getRouteCostsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getRouteCosts() {
return getRouteCostsMap();
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public java.util.Map getRouteCostsMap() {
return internalGetRouteCosts().getMap();
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public double getRouteCostsOrDefault(java.lang.String key, double defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetRouteCosts().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
@java.lang.Override
public double getRouteCostsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetRouteCosts().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearRouteCosts() {
bitField0_ = (bitField0_ & ~0x00000400);
internalGetMutableRouteCosts().getMutableMap().clear();
return this;
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
public Builder removeRouteCosts(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableRouteCosts().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableRouteCosts() {
bitField0_ |= 0x00000400;
return internalGetMutableRouteCosts().getMutableMap();
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
public Builder putRouteCosts(java.lang.String key, double value) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableRouteCosts().getMutableMap().put(key, value);
bitField0_ |= 0x00000400;
return this;
}
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
public Builder putAllRouteCosts(java.util.Map values) {
internalGetMutableRouteCosts().getMutableMap().putAll(values);
bitField0_ |= 0x00000400;
return this;
}
private double routeTotalCost_;
/**
*
*
*
* Total cost of the route. The sum of all costs in the cost map.
*
*
* double route_total_cost = 18;
*
* @return The routeTotalCost.
*/
@java.lang.Override
public double getRouteTotalCost() {
return routeTotalCost_;
}
/**
*
*
*
* Total cost of the route. The sum of all costs in the cost map.
*
*
* double route_total_cost = 18;
*
* @param value The routeTotalCost to set.
* @return This builder for chaining.
*/
public Builder setRouteTotalCost(double value) {
routeTotalCost_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* Total cost of the route. The sum of all costs in the cost map.
*
*
* double route_total_cost = 18;
*
* @return This builder for chaining.
*/
public Builder clearRouteTotalCost() {
bitField0_ = (bitField0_ & ~0x00000800);
routeTotalCost_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.ShipmentRoute)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.ShipmentRoute)
private static final com.google.maps.routeoptimization.v1.ShipmentRoute DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.ShipmentRoute();
}
public static com.google.maps.routeoptimization.v1.ShipmentRoute getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ShipmentRoute parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.ShipmentRoute getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy