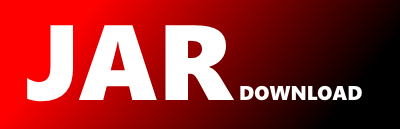
com.google.maps.routeoptimization.v1.ShipmentRouteOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-maps-routeoptimization-v1 Show documentation
Show all versions of proto-google-maps-routeoptimization-v1 Show documentation
Proto library for google-maps-routeoptimization
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/maps/routeoptimization/v1/route_optimization_service.proto
// Protobuf Java Version: 3.25.5
package com.google.maps.routeoptimization.v1;
public interface ShipmentRouteOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.ShipmentRoute)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Vehicle performing the route, identified by its index in the source
* `ShipmentModel`.
*
*
* int32 vehicle_index = 1;
*
* @return The vehicleIndex.
*/
int getVehicleIndex();
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @return The vehicleLabel.
*/
java.lang.String getVehicleLabel();
/**
*
*
*
* Label of the vehicle performing this route, equal to
* `ShipmentModel.vehicles(vehicle_index).label`, if specified.
*
*
* string vehicle_label = 2;
*
* @return The bytes for vehicleLabel.
*/
com.google.protobuf.ByteString getVehicleLabelBytes();
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*
* @return Whether the vehicleStartTime field is set.
*/
boolean hasVehicleStartTime();
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*
* @return The vehicleStartTime.
*/
com.google.protobuf.Timestamp getVehicleStartTime();
/**
*
*
*
* Time at which the vehicle starts its route.
*
*
* .google.protobuf.Timestamp vehicle_start_time = 5;
*/
com.google.protobuf.TimestampOrBuilder getVehicleStartTimeOrBuilder();
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*
* @return Whether the vehicleEndTime field is set.
*/
boolean hasVehicleEndTime();
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*
* @return The vehicleEndTime.
*/
com.google.protobuf.Timestamp getVehicleEndTime();
/**
*
*
*
* Time at which the vehicle finishes its route.
*
*
* .google.protobuf.Timestamp vehicle_end_time = 6;
*/
com.google.protobuf.TimestampOrBuilder getVehicleEndTimeOrBuilder();
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
java.util.List getVisitsList();
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.Visit getVisits(int index);
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
int getVisitsCount();
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
java.util.List extends com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder>
getVisitsOrBuilderList();
/**
*
*
*
* Ordered sequence of visits representing a route.
* visits[i] is the i-th visit in the route.
* If this field is empty, the vehicle is considered as unused.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Visit visits = 7;
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.VisitOrBuilder getVisitsOrBuilder(int index);
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
java.util.List
getTransitionsList();
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.Transition getTransitions(int index);
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
int getTransitionsCount();
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
java.util.List extends com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder>
getTransitionsOrBuilderList();
/**
*
*
*
* Ordered list of transitions for the route.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Transition transitions = 8;
*
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.TransitionOrBuilder getTransitionsOrBuilder(
int index);
/**
*
*
*
* When
* [OptimizeToursRequest.consider_road_traffic][google.maps.routeoptimization.v1.OptimizeToursRequest.consider_road_traffic],
* is set to true, this field indicates that inconsistencies in route timings
* are predicted using traffic-based travel duration estimates. There may be
* insufficient time to complete traffic-adjusted travel, delays, and breaks
* between visits, before the first visit, or after the last visit, while
* still satisfying the visit and vehicle time windows. For example,
*
* ```
* start_time(previous_visit) + duration(previous_visit) +
* travel_duration(previous_visit, next_visit) > start_time(next_visit)
* ```
*
* Arrival at next_visit will likely happen later than its current
* time window due the increased estimate of travel time
* `travel_duration(previous_visit, next_visit)` due to traffic. Also, a break
* may be forced to overlap with a visit due to an increase in travel time
* estimates and visit or break time window restrictions.
*
*
* bool has_traffic_infeasibilities = 9;
*
* @return The hasTrafficInfeasibilities.
*/
boolean getHasTrafficInfeasibilities();
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*
* @return Whether the routePolyline field is set.
*/
boolean hasRoutePolyline();
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*
* @return The routePolyline.
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline getRoutePolyline();
/**
*
*
*
* The encoded polyline representation of the route.
* This field is only populated if
* [OptimizeToursRequest.populate_polylines][google.maps.routeoptimization.v1.OptimizeToursRequest.populate_polylines]
* is set to true.
*
*
* .google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolyline route_polyline = 10;
*
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.EncodedPolylineOrBuilder
getRoutePolylineOrBuilder();
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
java.util.List getBreaksList();
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.Break getBreaks(int index);
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
int getBreaksCount();
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
java.util.List extends com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder>
getBreaksOrBuilderList();
/**
*
*
*
* Breaks scheduled for the vehicle performing this route.
* The `breaks` sequence represents time intervals, each starting at the
* corresponding `start_time` and lasting `duration` seconds.
*
*
* repeated .google.maps.routeoptimization.v1.ShipmentRoute.Break breaks = 11;
*/
com.google.maps.routeoptimization.v1.ShipmentRoute.BreakOrBuilder getBreaksOrBuilder(int index);
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*
* @return Whether the metrics field is set.
*/
boolean hasMetrics();
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*
* @return The metrics.
*/
com.google.maps.routeoptimization.v1.AggregatedMetrics getMetrics();
/**
*
*
*
* Duration, distance and load metrics for this route. The fields of
* [AggregatedMetrics][google.maps.routeoptimization.v1.AggregatedMetrics] are
* summed over all
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* or
* [ShipmentRoute.visits][google.maps.routeoptimization.v1.ShipmentRoute.visits],
* depending on the context.
*
*
* .google.maps.routeoptimization.v1.AggregatedMetrics metrics = 12;
*/
com.google.maps.routeoptimization.v1.AggregatedMetricsOrBuilder getMetricsOrBuilder();
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
int getRouteCostsCount();
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
boolean containsRouteCosts(java.lang.String key);
/** Use {@link #getRouteCostsMap()} instead. */
@java.lang.Deprecated
java.util.Map getRouteCosts();
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
java.util.Map getRouteCostsMap();
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
double getRouteCostsOrDefault(java.lang.String key, double defaultValue);
/**
*
*
*
* Cost of the route, broken down by cost-related request fields.
* The keys are proto paths, relative to the input OptimizeToursRequest, e.g.
* "model.shipments.pickups.cost", and the values are the total cost
* generated by the corresponding cost field, aggregated over the whole route.
* In other words, costs["model.shipments.pickups.cost"] is the sum of all
* pickup costs over the route. All costs defined in the model are reported in
* detail here with the exception of costs related to TransitionAttributes
* that are only reported in an aggregated way as of 2022/01.
*
*
* map<string, double> route_costs = 17;
*/
double getRouteCostsOrThrow(java.lang.String key);
/**
*
*
*
* Total cost of the route. The sum of all costs in the cost map.
*
*
* double route_total_cost = 18;
*
* @return The routeTotalCost.
*/
double getRouteTotalCost();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy