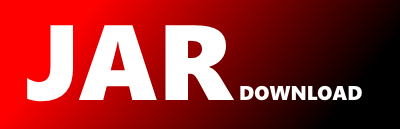
com.google.maps.routeoptimization.v1.Vehicle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-maps-routeoptimization-v1 Show documentation
Show all versions of proto-google-maps-routeoptimization-v1 Show documentation
Proto library for google-maps-routeoptimization
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/maps/routeoptimization/v1/route_optimization_service.proto
// Protobuf Java Version: 3.25.5
package com.google.maps.routeoptimization.v1;
/**
*
*
*
* Models a vehicle in a shipment problem. Solving a shipment problem will
* build a route starting from `start_location` and ending at `end_location`
* for this vehicle. A route is a sequence of visits (see `ShipmentRoute`).
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle}
*/
public final class Vehicle extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.Vehicle)
VehicleOrBuilder {
private static final long serialVersionUID = 0L;
// Use Vehicle.newBuilder() to construct.
private Vehicle(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Vehicle() {
displayName_ = "";
travelMode_ = 0;
startTags_ = com.google.protobuf.LazyStringArrayList.emptyList();
endTags_ = com.google.protobuf.LazyStringArrayList.emptyList();
startTimeWindows_ = java.util.Collections.emptyList();
endTimeWindows_ = java.util.Collections.emptyList();
unloadingPolicy_ = 0;
label_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Vehicle();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 30:
return internalGetLoadLimits();
case 24:
return internalGetExtraVisitDurationForVisitType();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.class,
com.google.maps.routeoptimization.v1.Vehicle.Builder.class);
}
/**
*
*
*
* Travel modes which can be used by vehicles.
*
* These should be a subset of the Google Maps Platform Routes Preferred API
* travel modes, see:
* https://developers.google.com/maps/documentation/routes_preferred/reference/rest/Shared.Types/RouteTravelMode.
*
*
* Protobuf enum {@code google.maps.routeoptimization.v1.Vehicle.TravelMode}
*/
public enum TravelMode implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* Unspecified travel mode, equivalent to `DRIVING`.
*
*
* TRAVEL_MODE_UNSPECIFIED = 0;
*/
TRAVEL_MODE_UNSPECIFIED(0),
/**
*
*
*
* Travel mode corresponding to driving directions (car, ...).
*
*
* DRIVING = 1;
*/
DRIVING(1),
/**
*
*
*
* Travel mode corresponding to walking directions.
*
*
* WALKING = 2;
*/
WALKING(2),
UNRECOGNIZED(-1),
;
/**
*
*
*
* Unspecified travel mode, equivalent to `DRIVING`.
*
*
* TRAVEL_MODE_UNSPECIFIED = 0;
*/
public static final int TRAVEL_MODE_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* Travel mode corresponding to driving directions (car, ...).
*
*
* DRIVING = 1;
*/
public static final int DRIVING_VALUE = 1;
/**
*
*
*
* Travel mode corresponding to walking directions.
*
*
* WALKING = 2;
*/
public static final int WALKING_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TravelMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static TravelMode forNumber(int value) {
switch (value) {
case 0:
return TRAVEL_MODE_UNSPECIFIED;
case 1:
return DRIVING;
case 2:
return WALKING;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TravelMode findValueByNumber(int number) {
return TravelMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.Vehicle.getDescriptor().getEnumTypes().get(0);
}
private static final TravelMode[] VALUES = values();
public static TravelMode valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private TravelMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.maps.routeoptimization.v1.Vehicle.TravelMode)
}
/**
*
*
*
* Policy on how a vehicle can be unloaded. Applies only to shipments having
* both a pickup and a delivery.
*
* Other shipments are free to occur anywhere on the route independent of
* `unloading_policy`.
*
*
* Protobuf enum {@code google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy}
*/
public enum UnloadingPolicy implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* Unspecified unloading policy; deliveries must just occur after their
* corresponding pickups.
*
*
* UNLOADING_POLICY_UNSPECIFIED = 0;
*/
UNLOADING_POLICY_UNSPECIFIED(0),
/**
*
*
*
* Deliveries must occur in reverse order of pickups
*
*
* LAST_IN_FIRST_OUT = 1;
*/
LAST_IN_FIRST_OUT(1),
/**
*
*
*
* Deliveries must occur in the same order as pickups
*
*
* FIRST_IN_FIRST_OUT = 2;
*/
FIRST_IN_FIRST_OUT(2),
UNRECOGNIZED(-1),
;
/**
*
*
*
* Unspecified unloading policy; deliveries must just occur after their
* corresponding pickups.
*
*
* UNLOADING_POLICY_UNSPECIFIED = 0;
*/
public static final int UNLOADING_POLICY_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* Deliveries must occur in reverse order of pickups
*
*
* LAST_IN_FIRST_OUT = 1;
*/
public static final int LAST_IN_FIRST_OUT_VALUE = 1;
/**
*
*
*
* Deliveries must occur in the same order as pickups
*
*
* FIRST_IN_FIRST_OUT = 2;
*/
public static final int FIRST_IN_FIRST_OUT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static UnloadingPolicy valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static UnloadingPolicy forNumber(int value) {
switch (value) {
case 0:
return UNLOADING_POLICY_UNSPECIFIED;
case 1:
return LAST_IN_FIRST_OUT;
case 2:
return FIRST_IN_FIRST_OUT;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public UnloadingPolicy findValueByNumber(int number) {
return UnloadingPolicy.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.Vehicle.getDescriptor().getEnumTypes().get(1);
}
private static final UnloadingPolicy[] VALUES = values();
public static UnloadingPolicy valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private UnloadingPolicy(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy)
}
public interface LoadLimitOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.Vehicle.LoadLimit)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @return Whether the maxLoad field is set.
*/
boolean hasMaxLoad();
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @return The maxLoad.
*/
long getMaxLoad();
/**
*
*
*
* A soft limit of the load. See
* [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max].
*
*
* int64 soft_max_load = 2;
*
* @return The softMaxLoad.
*/
long getSoftMaxLoad();
/**
*
*
*
* If the load ever exceeds
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load]
* along this vehicle's route, the following cost penalty applies (only once
* per vehicle): (load -
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load])
* * [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max]. All costs
* add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
*
* double cost_per_unit_above_soft_max = 3;
*
* @return The costPerUnitAboveSoftMax.
*/
double getCostPerUnitAboveSoftMax();
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*
* @return Whether the startLoadInterval field is set.
*/
boolean hasStartLoadInterval();
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*
* @return The startLoadInterval.
*/
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval getStartLoadInterval();
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder
getStartLoadIntervalOrBuilder();
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*
* @return Whether the endLoadInterval field is set.
*/
boolean hasEndLoadInterval();
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*
* @return The endLoadInterval.
*/
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval getEndLoadInterval();
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder
getEndLoadIntervalOrBuilder();
}
/**
*
*
*
* Defines a load limit applying to a vehicle, e.g. "this truck may only
* carry up to 3500 kg". See
* [load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits].
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle.LoadLimit}
*/
public static final class LoadLimit extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.Vehicle.LoadLimit)
LoadLimitOrBuilder {
private static final long serialVersionUID = 0L;
// Use LoadLimit.newBuilder() to construct.
private LoadLimit(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LoadLimit() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new LoadLimit();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.class,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder.class);
}
public interface IntervalOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* A minimum acceptable load. Must be ≥ 0.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* int64 min = 1;
*
* @return The min.
*/
long getMin();
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @return Whether the max field is set.
*/
boolean hasMax();
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @return The max.
*/
long getMax();
}
/**
*
*
*
* Interval of acceptable load amounts.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval}
*/
public static final class Interval extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval)
IntervalOrBuilder {
private static final long serialVersionUID = 0L;
// Use Interval.newBuilder() to construct.
private Interval(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Interval() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Interval();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_Interval_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_Interval_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.class,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder.class);
}
private int bitField0_;
public static final int MIN_FIELD_NUMBER = 1;
private long min_ = 0L;
/**
*
*
*
* A minimum acceptable load. Must be ≥ 0.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* int64 min = 1;
*
* @return The min.
*/
@java.lang.Override
public long getMin() {
return min_;
}
public static final int MAX_FIELD_NUMBER = 2;
private long max_ = 0L;
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @return Whether the max field is set.
*/
@java.lang.Override
public boolean hasMax() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @return The max.
*/
@java.lang.Override
public long getMax() {
return max_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (min_ != 0L) {
output.writeInt64(1, min_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(2, max_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (min_ != 0L) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(1, min_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(2, max_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval other =
(com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval) obj;
if (getMin() != other.getMin()) return false;
if (hasMax() != other.hasMax()) return false;
if (hasMax()) {
if (getMax() != other.getMax()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MIN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getMin());
if (hasMax()) {
hash = (37 * hash) + MAX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getMax());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Interval of acceptable load amounts.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval)
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_Interval_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_Interval_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.class,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder.class);
}
// Construct using
// com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
min_ = 0L;
max_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_Interval_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval build() {
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval buildPartial() {
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval result =
new com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.min_ = min_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.max_ = max_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval) {
return mergeFrom(
(com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval other) {
if (other
== com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
.getDefaultInstance()) return this;
if (other.getMin() != 0L) {
setMin(other.getMin());
}
if (other.hasMax()) {
setMax(other.getMax());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
min_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16:
{
max_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long min_;
/**
*
*
*
* A minimum acceptable load. Must be ≥ 0.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* int64 min = 1;
*
* @return The min.
*/
@java.lang.Override
public long getMin() {
return min_;
}
/**
*
*
*
* A minimum acceptable load. Must be ≥ 0.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* int64 min = 1;
*
* @param value The min to set.
* @return This builder for chaining.
*/
public Builder setMin(long value) {
min_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* A minimum acceptable load. Must be ≥ 0.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* int64 min = 1;
*
* @return This builder for chaining.
*/
public Builder clearMin() {
bitField0_ = (bitField0_ & ~0x00000001);
min_ = 0L;
onChanged();
return this;
}
private long max_;
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @return Whether the max field is set.
*/
@java.lang.Override
public boolean hasMax() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @return The max.
*/
@java.lang.Override
public long getMax() {
return max_;
}
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @param value The max to set.
* @return This builder for chaining.
*/
public Builder setMax(long value) {
max_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* A maximum acceptable load. Must be ≥ 0. If unspecified, the maximum
* load is unrestricted by this message.
* If they're both specified,
* [min][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.min]
* must be ≤
* [max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.max].
*
*
* optional int64 max = 2;
*
* @return This builder for chaining.
*/
public Builder clearMax() {
bitField0_ = (bitField0_ & ~0x00000002);
max_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval)
private static final com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval();
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Interval parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int MAX_LOAD_FIELD_NUMBER = 1;
private long maxLoad_ = 0L;
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @return Whether the maxLoad field is set.
*/
@java.lang.Override
public boolean hasMaxLoad() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @return The maxLoad.
*/
@java.lang.Override
public long getMaxLoad() {
return maxLoad_;
}
public static final int SOFT_MAX_LOAD_FIELD_NUMBER = 2;
private long softMaxLoad_ = 0L;
/**
*
*
*
* A soft limit of the load. See
* [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max].
*
*
* int64 soft_max_load = 2;
*
* @return The softMaxLoad.
*/
@java.lang.Override
public long getSoftMaxLoad() {
return softMaxLoad_;
}
public static final int COST_PER_UNIT_ABOVE_SOFT_MAX_FIELD_NUMBER = 3;
private double costPerUnitAboveSoftMax_ = 0D;
/**
*
*
*
* If the load ever exceeds
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load]
* along this vehicle's route, the following cost penalty applies (only once
* per vehicle): (load -
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load])
* * [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max]. All costs
* add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
*
* double cost_per_unit_above_soft_max = 3;
*
* @return The costPerUnitAboveSoftMax.
*/
@java.lang.Override
public double getCostPerUnitAboveSoftMax() {
return costPerUnitAboveSoftMax_;
}
public static final int START_LOAD_INTERVAL_FIELD_NUMBER = 4;
private com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval startLoadInterval_;
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*
* @return Whether the startLoadInterval field is set.
*/
@java.lang.Override
public boolean hasStartLoadInterval() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*
* @return The startLoadInterval.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval getStartLoadInterval() {
return startLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: startLoadInterval_;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder
getStartLoadIntervalOrBuilder() {
return startLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: startLoadInterval_;
}
public static final int END_LOAD_INTERVAL_FIELD_NUMBER = 5;
private com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval endLoadInterval_;
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*
* @return Whether the endLoadInterval field is set.
*/
@java.lang.Override
public boolean hasEndLoadInterval() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*
* @return The endLoadInterval.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval getEndLoadInterval() {
return endLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: endLoadInterval_;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder
getEndLoadIntervalOrBuilder() {
return endLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: endLoadInterval_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, maxLoad_);
}
if (softMaxLoad_ != 0L) {
output.writeInt64(2, softMaxLoad_);
}
if (java.lang.Double.doubleToRawLongBits(costPerUnitAboveSoftMax_) != 0) {
output.writeDouble(3, costPerUnitAboveSoftMax_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(4, getStartLoadInterval());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(5, getEndLoadInterval());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(1, maxLoad_);
}
if (softMaxLoad_ != 0L) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(2, softMaxLoad_);
}
if (java.lang.Double.doubleToRawLongBits(costPerUnitAboveSoftMax_) != 0) {
size +=
com.google.protobuf.CodedOutputStream.computeDoubleSize(3, costPerUnitAboveSoftMax_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getStartLoadInterval());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(5, getEndLoadInterval());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.Vehicle.LoadLimit)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit other =
(com.google.maps.routeoptimization.v1.Vehicle.LoadLimit) obj;
if (hasMaxLoad() != other.hasMaxLoad()) return false;
if (hasMaxLoad()) {
if (getMaxLoad() != other.getMaxLoad()) return false;
}
if (getSoftMaxLoad() != other.getSoftMaxLoad()) return false;
if (java.lang.Double.doubleToLongBits(getCostPerUnitAboveSoftMax())
!= java.lang.Double.doubleToLongBits(other.getCostPerUnitAboveSoftMax())) return false;
if (hasStartLoadInterval() != other.hasStartLoadInterval()) return false;
if (hasStartLoadInterval()) {
if (!getStartLoadInterval().equals(other.getStartLoadInterval())) return false;
}
if (hasEndLoadInterval() != other.hasEndLoadInterval()) return false;
if (hasEndLoadInterval()) {
if (!getEndLoadInterval().equals(other.getEndLoadInterval())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMaxLoad()) {
hash = (37 * hash) + MAX_LOAD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getMaxLoad());
}
hash = (37 * hash) + SOFT_MAX_LOAD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getSoftMaxLoad());
hash = (37 * hash) + COST_PER_UNIT_ABOVE_SOFT_MAX_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCostPerUnitAboveSoftMax()));
if (hasStartLoadInterval()) {
hash = (37 * hash) + START_LOAD_INTERVAL_FIELD_NUMBER;
hash = (53 * hash) + getStartLoadInterval().hashCode();
}
if (hasEndLoadInterval()) {
hash = (37 * hash) + END_LOAD_INTERVAL_FIELD_NUMBER;
hash = (53 * hash) + getEndLoadInterval().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Defines a load limit applying to a vehicle, e.g. "this truck may only
* carry up to 3500 kg". See
* [load_limits][google.maps.routeoptimization.v1.Vehicle.load_limits].
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle.LoadLimit}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.Vehicle.LoadLimit)
com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.class,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getStartLoadIntervalFieldBuilder();
getEndLoadIntervalFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
maxLoad_ = 0L;
softMaxLoad_ = 0L;
costPerUnitAboveSoftMax_ = 0D;
startLoadInterval_ = null;
if (startLoadIntervalBuilder_ != null) {
startLoadIntervalBuilder_.dispose();
startLoadIntervalBuilder_ = null;
}
endLoadInterval_ = null;
if (endLoadIntervalBuilder_ != null) {
endLoadIntervalBuilder_.dispose();
endLoadIntervalBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimit_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit build() {
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit buildPartial() {
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit result =
new com.google.maps.routeoptimization.v1.Vehicle.LoadLimit(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.maps.routeoptimization.v1.Vehicle.LoadLimit result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.maxLoad_ = maxLoad_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.softMaxLoad_ = softMaxLoad_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.costPerUnitAboveSoftMax_ = costPerUnitAboveSoftMax_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.startLoadInterval_ =
startLoadIntervalBuilder_ == null
? startLoadInterval_
: startLoadIntervalBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.endLoadInterval_ =
endLoadIntervalBuilder_ == null ? endLoadInterval_ : endLoadIntervalBuilder_.build();
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.Vehicle.LoadLimit) {
return mergeFrom((com.google.maps.routeoptimization.v1.Vehicle.LoadLimit) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.maps.routeoptimization.v1.Vehicle.LoadLimit other) {
if (other == com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.getDefaultInstance())
return this;
if (other.hasMaxLoad()) {
setMaxLoad(other.getMaxLoad());
}
if (other.getSoftMaxLoad() != 0L) {
setSoftMaxLoad(other.getSoftMaxLoad());
}
if (other.getCostPerUnitAboveSoftMax() != 0D) {
setCostPerUnitAboveSoftMax(other.getCostPerUnitAboveSoftMax());
}
if (other.hasStartLoadInterval()) {
mergeStartLoadInterval(other.getStartLoadInterval());
}
if (other.hasEndLoadInterval()) {
mergeEndLoadInterval(other.getEndLoadInterval());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
maxLoad_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16:
{
softMaxLoad_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 25:
{
costPerUnitAboveSoftMax_ = input.readDouble();
bitField0_ |= 0x00000004;
break;
} // case 25
case 34:
{
input.readMessage(
getStartLoadIntervalFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42:
{
input.readMessage(
getEndLoadIntervalFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long maxLoad_;
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @return Whether the maxLoad field is set.
*/
@java.lang.Override
public boolean hasMaxLoad() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @return The maxLoad.
*/
@java.lang.Override
public long getMaxLoad() {
return maxLoad_;
}
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @param value The maxLoad to set.
* @return This builder for chaining.
*/
public Builder setMaxLoad(long value) {
maxLoad_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The maximum acceptable amount of load.
*
*
* optional int64 max_load = 1;
*
* @return This builder for chaining.
*/
public Builder clearMaxLoad() {
bitField0_ = (bitField0_ & ~0x00000001);
maxLoad_ = 0L;
onChanged();
return this;
}
private long softMaxLoad_;
/**
*
*
*
* A soft limit of the load. See
* [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max].
*
*
* int64 soft_max_load = 2;
*
* @return The softMaxLoad.
*/
@java.lang.Override
public long getSoftMaxLoad() {
return softMaxLoad_;
}
/**
*
*
*
* A soft limit of the load. See
* [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max].
*
*
* int64 soft_max_load = 2;
*
* @param value The softMaxLoad to set.
* @return This builder for chaining.
*/
public Builder setSoftMaxLoad(long value) {
softMaxLoad_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* A soft limit of the load. See
* [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max].
*
*
* int64 soft_max_load = 2;
*
* @return This builder for chaining.
*/
public Builder clearSoftMaxLoad() {
bitField0_ = (bitField0_ & ~0x00000002);
softMaxLoad_ = 0L;
onChanged();
return this;
}
private double costPerUnitAboveSoftMax_;
/**
*
*
*
* If the load ever exceeds
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load]
* along this vehicle's route, the following cost penalty applies (only once
* per vehicle): (load -
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load])
* * [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max]. All costs
* add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
*
* double cost_per_unit_above_soft_max = 3;
*
* @return The costPerUnitAboveSoftMax.
*/
@java.lang.Override
public double getCostPerUnitAboveSoftMax() {
return costPerUnitAboveSoftMax_;
}
/**
*
*
*
* If the load ever exceeds
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load]
* along this vehicle's route, the following cost penalty applies (only once
* per vehicle): (load -
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load])
* * [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max]. All costs
* add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
*
* double cost_per_unit_above_soft_max = 3;
*
* @param value The costPerUnitAboveSoftMax to set.
* @return This builder for chaining.
*/
public Builder setCostPerUnitAboveSoftMax(double value) {
costPerUnitAboveSoftMax_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* If the load ever exceeds
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load]
* along this vehicle's route, the following cost penalty applies (only once
* per vehicle): (load -
* [soft_max_load][google.maps.routeoptimization.v1.Vehicle.LoadLimit.soft_max_load])
* * [cost_per_unit_above_soft_max][google.maps.routeoptimization.v1.Vehicle.LoadLimit.cost_per_unit_above_soft_max]. All costs
* add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
*
* double cost_per_unit_above_soft_max = 3;
*
* @return This builder for chaining.
*/
public Builder clearCostPerUnitAboveSoftMax() {
bitField0_ = (bitField0_ & ~0x00000004);
costPerUnitAboveSoftMax_ = 0D;
onChanged();
return this;
}
private com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval startLoadInterval_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder>
startLoadIntervalBuilder_;
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*
* @return Whether the startLoadInterval field is set.
*/
public boolean hasStartLoadInterval() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*
* @return The startLoadInterval.
*/
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
getStartLoadInterval() {
if (startLoadIntervalBuilder_ == null) {
return startLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: startLoadInterval_;
} else {
return startLoadIntervalBuilder_.getMessage();
}
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
public Builder setStartLoadInterval(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval value) {
if (startLoadIntervalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startLoadInterval_ = value;
} else {
startLoadIntervalBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
public Builder setStartLoadInterval(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder builderForValue) {
if (startLoadIntervalBuilder_ == null) {
startLoadInterval_ = builderForValue.build();
} else {
startLoadIntervalBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
public Builder mergeStartLoadInterval(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval value) {
if (startLoadIntervalBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& startLoadInterval_ != null
&& startLoadInterval_
!= com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
.getDefaultInstance()) {
getStartLoadIntervalBuilder().mergeFrom(value);
} else {
startLoadInterval_ = value;
}
} else {
startLoadIntervalBuilder_.mergeFrom(value);
}
if (startLoadInterval_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
public Builder clearStartLoadInterval() {
bitField0_ = (bitField0_ & ~0x00000008);
startLoadInterval_ = null;
if (startLoadIntervalBuilder_ != null) {
startLoadIntervalBuilder_.dispose();
startLoadIntervalBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder
getStartLoadIntervalBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getStartLoadIntervalFieldBuilder().getBuilder();
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder
getStartLoadIntervalOrBuilder() {
if (startLoadIntervalBuilder_ != null) {
return startLoadIntervalBuilder_.getMessageOrBuilder();
} else {
return startLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: startLoadInterval_;
}
}
/**
*
*
*
* The acceptable load interval of the vehicle at the start of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval start_load_interval = 4;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder>
getStartLoadIntervalFieldBuilder() {
if (startLoadIntervalBuilder_ == null) {
startLoadIntervalBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder>(
getStartLoadInterval(), getParentForChildren(), isClean());
startLoadInterval_ = null;
}
return startLoadIntervalBuilder_;
}
private com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval endLoadInterval_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder>
endLoadIntervalBuilder_;
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*
* @return Whether the endLoadInterval field is set.
*/
public boolean hasEndLoadInterval() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*
* @return The endLoadInterval.
*/
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval getEndLoadInterval() {
if (endLoadIntervalBuilder_ == null) {
return endLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: endLoadInterval_;
} else {
return endLoadIntervalBuilder_.getMessage();
}
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
public Builder setEndLoadInterval(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval value) {
if (endLoadIntervalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endLoadInterval_ = value;
} else {
endLoadIntervalBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
public Builder setEndLoadInterval(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder builderForValue) {
if (endLoadIntervalBuilder_ == null) {
endLoadInterval_ = builderForValue.build();
} else {
endLoadIntervalBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
public Builder mergeEndLoadInterval(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval value) {
if (endLoadIntervalBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)
&& endLoadInterval_ != null
&& endLoadInterval_
!= com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval
.getDefaultInstance()) {
getEndLoadIntervalBuilder().mergeFrom(value);
} else {
endLoadInterval_ = value;
}
} else {
endLoadIntervalBuilder_.mergeFrom(value);
}
if (endLoadInterval_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
public Builder clearEndLoadInterval() {
bitField0_ = (bitField0_ & ~0x00000010);
endLoadInterval_ = null;
if (endLoadIntervalBuilder_ != null) {
endLoadIntervalBuilder_.dispose();
endLoadIntervalBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder
getEndLoadIntervalBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getEndLoadIntervalFieldBuilder().getBuilder();
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder
getEndLoadIntervalOrBuilder() {
if (endLoadIntervalBuilder_ != null) {
return endLoadIntervalBuilder_.getMessageOrBuilder();
} else {
return endLoadInterval_ == null
? com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.getDefaultInstance()
: endLoadInterval_;
}
}
/**
*
*
*
* The acceptable load interval of the vehicle at the end of the route.
*
*
* .google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval end_load_interval = 5;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder>
getEndLoadIntervalFieldBuilder() {
if (endLoadIntervalBuilder_ == null) {
endLoadIntervalBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Interval.Builder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.IntervalOrBuilder>(
getEndLoadInterval(), getParentForChildren(), isClean());
endLoadInterval_ = null;
}
return endLoadIntervalBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.Vehicle.LoadLimit)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.Vehicle.LoadLimit)
private static final com.google.maps.routeoptimization.v1.Vehicle.LoadLimit DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.Vehicle.LoadLimit();
}
public static com.google.maps.routeoptimization.v1.Vehicle.LoadLimit getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LoadLimit parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DurationLimitOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routeoptimization.v1.Vehicle.DurationLimit)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*
* @return Whether the maxDuration field is set.
*/
boolean hasMaxDuration();
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*
* @return The maxDuration.
*/
com.google.protobuf.Duration getMaxDuration();
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
com.google.protobuf.DurationOrBuilder getMaxDurationOrBuilder();
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*
* @return Whether the softMaxDuration field is set.
*/
boolean hasSoftMaxDuration();
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*
* @return The softMaxDuration.
*/
com.google.protobuf.Duration getSoftMaxDuration();
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
com.google.protobuf.DurationOrBuilder getSoftMaxDurationOrBuilder();
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @return Whether the costPerHourAfterSoftMax field is set.
*/
boolean hasCostPerHourAfterSoftMax();
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @return The costPerHourAfterSoftMax.
*/
double getCostPerHourAfterSoftMax();
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*
* @return Whether the quadraticSoftMaxDuration field is set.
*/
boolean hasQuadraticSoftMaxDuration();
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*
* @return The quadraticSoftMaxDuration.
*/
com.google.protobuf.Duration getQuadraticSoftMaxDuration();
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
com.google.protobuf.DurationOrBuilder getQuadraticSoftMaxDurationOrBuilder();
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @return Whether the costPerSquareHourAfterQuadraticSoftMax field is set.
*/
boolean hasCostPerSquareHourAfterQuadraticSoftMax();
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @return The costPerSquareHourAfterQuadraticSoftMax.
*/
double getCostPerSquareHourAfterQuadraticSoftMax();
}
/**
*
*
*
* A limit defining a maximum duration of the route of a vehicle. It can be
* either hard or soft.
*
* When a soft limit field is defined, both the soft max threshold and its
* associated cost must be defined together.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle.DurationLimit}
*/
public static final class DurationLimit extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.maps.routeoptimization.v1.Vehicle.DurationLimit)
DurationLimitOrBuilder {
private static final long serialVersionUID = 0L;
// Use DurationLimit.newBuilder() to construct.
private DurationLimit(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DurationLimit() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new DurationLimit();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_DurationLimit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_DurationLimit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.class,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder.class);
}
private int bitField0_;
public static final int MAX_DURATION_FIELD_NUMBER = 1;
private com.google.protobuf.Duration maxDuration_;
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*
* @return Whether the maxDuration field is set.
*/
@java.lang.Override
public boolean hasMaxDuration() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*
* @return The maxDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getMaxDuration() {
return maxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: maxDuration_;
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getMaxDurationOrBuilder() {
return maxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: maxDuration_;
}
public static final int SOFT_MAX_DURATION_FIELD_NUMBER = 2;
private com.google.protobuf.Duration softMaxDuration_;
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*
* @return Whether the softMaxDuration field is set.
*/
@java.lang.Override
public boolean hasSoftMaxDuration() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*
* @return The softMaxDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getSoftMaxDuration() {
return softMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: softMaxDuration_;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getSoftMaxDurationOrBuilder() {
return softMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: softMaxDuration_;
}
public static final int COST_PER_HOUR_AFTER_SOFT_MAX_FIELD_NUMBER = 3;
private double costPerHourAfterSoftMax_ = 0D;
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @return Whether the costPerHourAfterSoftMax field is set.
*/
@java.lang.Override
public boolean hasCostPerHourAfterSoftMax() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @return The costPerHourAfterSoftMax.
*/
@java.lang.Override
public double getCostPerHourAfterSoftMax() {
return costPerHourAfterSoftMax_;
}
public static final int QUADRATIC_SOFT_MAX_DURATION_FIELD_NUMBER = 4;
private com.google.protobuf.Duration quadraticSoftMaxDuration_;
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*
* @return Whether the quadraticSoftMaxDuration field is set.
*/
@java.lang.Override
public boolean hasQuadraticSoftMaxDuration() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*
* @return The quadraticSoftMaxDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getQuadraticSoftMaxDuration() {
return quadraticSoftMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: quadraticSoftMaxDuration_;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getQuadraticSoftMaxDurationOrBuilder() {
return quadraticSoftMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: quadraticSoftMaxDuration_;
}
public static final int COST_PER_SQUARE_HOUR_AFTER_QUADRATIC_SOFT_MAX_FIELD_NUMBER = 5;
private double costPerSquareHourAfterQuadraticSoftMax_ = 0D;
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @return Whether the costPerSquareHourAfterQuadraticSoftMax field is set.
*/
@java.lang.Override
public boolean hasCostPerSquareHourAfterQuadraticSoftMax() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @return The costPerSquareHourAfterQuadraticSoftMax.
*/
@java.lang.Override
public double getCostPerSquareHourAfterQuadraticSoftMax() {
return costPerSquareHourAfterQuadraticSoftMax_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getMaxDuration());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getSoftMaxDuration());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeDouble(3, costPerHourAfterSoftMax_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(4, getQuadraticSoftMaxDuration());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeDouble(5, costPerSquareHourAfterQuadraticSoftMax_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getMaxDuration());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(2, getSoftMaxDuration());
}
if (((bitField0_ & 0x00000004) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeDoubleSize(3, costPerHourAfterSoftMax_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
4, getQuadraticSoftMaxDuration());
}
if (((bitField0_ & 0x00000010) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeDoubleSize(
5, costPerSquareHourAfterQuadraticSoftMax_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.Vehicle.DurationLimit)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit other =
(com.google.maps.routeoptimization.v1.Vehicle.DurationLimit) obj;
if (hasMaxDuration() != other.hasMaxDuration()) return false;
if (hasMaxDuration()) {
if (!getMaxDuration().equals(other.getMaxDuration())) return false;
}
if (hasSoftMaxDuration() != other.hasSoftMaxDuration()) return false;
if (hasSoftMaxDuration()) {
if (!getSoftMaxDuration().equals(other.getSoftMaxDuration())) return false;
}
if (hasCostPerHourAfterSoftMax() != other.hasCostPerHourAfterSoftMax()) return false;
if (hasCostPerHourAfterSoftMax()) {
if (java.lang.Double.doubleToLongBits(getCostPerHourAfterSoftMax())
!= java.lang.Double.doubleToLongBits(other.getCostPerHourAfterSoftMax())) return false;
}
if (hasQuadraticSoftMaxDuration() != other.hasQuadraticSoftMaxDuration()) return false;
if (hasQuadraticSoftMaxDuration()) {
if (!getQuadraticSoftMaxDuration().equals(other.getQuadraticSoftMaxDuration()))
return false;
}
if (hasCostPerSquareHourAfterQuadraticSoftMax()
!= other.hasCostPerSquareHourAfterQuadraticSoftMax()) return false;
if (hasCostPerSquareHourAfterQuadraticSoftMax()) {
if (java.lang.Double.doubleToLongBits(getCostPerSquareHourAfterQuadraticSoftMax())
!= java.lang.Double.doubleToLongBits(other.getCostPerSquareHourAfterQuadraticSoftMax()))
return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMaxDuration()) {
hash = (37 * hash) + MAX_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getMaxDuration().hashCode();
}
if (hasSoftMaxDuration()) {
hash = (37 * hash) + SOFT_MAX_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getSoftMaxDuration().hashCode();
}
if (hasCostPerHourAfterSoftMax()) {
hash = (37 * hash) + COST_PER_HOUR_AFTER_SOFT_MAX_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCostPerHourAfterSoftMax()));
}
if (hasQuadraticSoftMaxDuration()) {
hash = (37 * hash) + QUADRATIC_SOFT_MAX_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getQuadraticSoftMaxDuration().hashCode();
}
if (hasCostPerSquareHourAfterQuadraticSoftMax()) {
hash = (37 * hash) + COST_PER_SQUARE_HOUR_AFTER_QUADRATIC_SOFT_MAX_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCostPerSquareHourAfterQuadraticSoftMax()));
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A limit defining a maximum duration of the route of a vehicle. It can be
* either hard or soft.
*
* When a soft limit field is defined, both the soft max threshold and its
* associated cost must be defined together.
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle.DurationLimit}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.Vehicle.DurationLimit)
com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_DurationLimit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_DurationLimit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.class,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getMaxDurationFieldBuilder();
getSoftMaxDurationFieldBuilder();
getQuadraticSoftMaxDurationFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
maxDuration_ = null;
if (maxDurationBuilder_ != null) {
maxDurationBuilder_.dispose();
maxDurationBuilder_ = null;
}
softMaxDuration_ = null;
if (softMaxDurationBuilder_ != null) {
softMaxDurationBuilder_.dispose();
softMaxDurationBuilder_ = null;
}
costPerHourAfterSoftMax_ = 0D;
quadraticSoftMaxDuration_ = null;
if (quadraticSoftMaxDurationBuilder_ != null) {
quadraticSoftMaxDurationBuilder_.dispose();
quadraticSoftMaxDurationBuilder_ = null;
}
costPerSquareHourAfterQuadraticSoftMax_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_DurationLimit_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit
getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit build() {
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit buildPartial() {
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit result =
new com.google.maps.routeoptimization.v1.Vehicle.DurationLimit(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.maxDuration_ =
maxDurationBuilder_ == null ? maxDuration_ : maxDurationBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.softMaxDuration_ =
softMaxDurationBuilder_ == null ? softMaxDuration_ : softMaxDurationBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.costPerHourAfterSoftMax_ = costPerHourAfterSoftMax_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.quadraticSoftMaxDuration_ =
quadraticSoftMaxDurationBuilder_ == null
? quadraticSoftMaxDuration_
: quadraticSoftMaxDurationBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.costPerSquareHourAfterQuadraticSoftMax_ = costPerSquareHourAfterQuadraticSoftMax_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.Vehicle.DurationLimit) {
return mergeFrom((com.google.maps.routeoptimization.v1.Vehicle.DurationLimit) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.maps.routeoptimization.v1.Vehicle.DurationLimit other) {
if (other
== com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance())
return this;
if (other.hasMaxDuration()) {
mergeMaxDuration(other.getMaxDuration());
}
if (other.hasSoftMaxDuration()) {
mergeSoftMaxDuration(other.getSoftMaxDuration());
}
if (other.hasCostPerHourAfterSoftMax()) {
setCostPerHourAfterSoftMax(other.getCostPerHourAfterSoftMax());
}
if (other.hasQuadraticSoftMaxDuration()) {
mergeQuadraticSoftMaxDuration(other.getQuadraticSoftMaxDuration());
}
if (other.hasCostPerSquareHourAfterQuadraticSoftMax()) {
setCostPerSquareHourAfterQuadraticSoftMax(
other.getCostPerSquareHourAfterQuadraticSoftMax());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(getMaxDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
input.readMessage(
getSoftMaxDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 25:
{
costPerHourAfterSoftMax_ = input.readDouble();
bitField0_ |= 0x00000004;
break;
} // case 25
case 34:
{
input.readMessage(
getQuadraticSoftMaxDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 41:
{
costPerSquareHourAfterQuadraticSoftMax_ = input.readDouble();
bitField0_ |= 0x00000010;
break;
} // case 41
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.Duration maxDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
maxDurationBuilder_;
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*
* @return Whether the maxDuration field is set.
*/
public boolean hasMaxDuration() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*
* @return The maxDuration.
*/
public com.google.protobuf.Duration getMaxDuration() {
if (maxDurationBuilder_ == null) {
return maxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: maxDuration_;
} else {
return maxDurationBuilder_.getMessage();
}
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
public Builder setMaxDuration(com.google.protobuf.Duration value) {
if (maxDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxDuration_ = value;
} else {
maxDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
public Builder setMaxDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (maxDurationBuilder_ == null) {
maxDuration_ = builderForValue.build();
} else {
maxDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
public Builder mergeMaxDuration(com.google.protobuf.Duration value) {
if (maxDurationBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& maxDuration_ != null
&& maxDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getMaxDurationBuilder().mergeFrom(value);
} else {
maxDuration_ = value;
}
} else {
maxDurationBuilder_.mergeFrom(value);
}
if (maxDuration_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
public Builder clearMaxDuration() {
bitField0_ = (bitField0_ & ~0x00000001);
maxDuration_ = null;
if (maxDurationBuilder_ != null) {
maxDurationBuilder_.dispose();
maxDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
public com.google.protobuf.Duration.Builder getMaxDurationBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getMaxDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
public com.google.protobuf.DurationOrBuilder getMaxDurationOrBuilder() {
if (maxDurationBuilder_ != null) {
return maxDurationBuilder_.getMessageOrBuilder();
} else {
return maxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: maxDuration_;
}
}
/**
*
*
*
* A hard limit constraining the duration to be at most max_duration.
*
*
* .google.protobuf.Duration max_duration = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getMaxDurationFieldBuilder() {
if (maxDurationBuilder_ == null) {
maxDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getMaxDuration(), getParentForChildren(), isClean());
maxDuration_ = null;
}
return maxDurationBuilder_;
}
private com.google.protobuf.Duration softMaxDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
softMaxDurationBuilder_;
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*
* @return Whether the softMaxDuration field is set.
*/
public boolean hasSoftMaxDuration() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*
* @return The softMaxDuration.
*/
public com.google.protobuf.Duration getSoftMaxDuration() {
if (softMaxDurationBuilder_ == null) {
return softMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: softMaxDuration_;
} else {
return softMaxDurationBuilder_.getMessage();
}
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
public Builder setSoftMaxDuration(com.google.protobuf.Duration value) {
if (softMaxDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
softMaxDuration_ = value;
} else {
softMaxDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
public Builder setSoftMaxDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (softMaxDurationBuilder_ == null) {
softMaxDuration_ = builderForValue.build();
} else {
softMaxDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
public Builder mergeSoftMaxDuration(com.google.protobuf.Duration value) {
if (softMaxDurationBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)
&& softMaxDuration_ != null
&& softMaxDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getSoftMaxDurationBuilder().mergeFrom(value);
} else {
softMaxDuration_ = value;
}
} else {
softMaxDurationBuilder_.mergeFrom(value);
}
if (softMaxDuration_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
public Builder clearSoftMaxDuration() {
bitField0_ = (bitField0_ & ~0x00000002);
softMaxDuration_ = null;
if (softMaxDurationBuilder_ != null) {
softMaxDurationBuilder_.dispose();
softMaxDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
public com.google.protobuf.Duration.Builder getSoftMaxDurationBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getSoftMaxDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
public com.google.protobuf.DurationOrBuilder getSoftMaxDurationOrBuilder() {
if (softMaxDurationBuilder_ != null) {
return softMaxDurationBuilder_.getMessageOrBuilder();
} else {
return softMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: softMaxDuration_;
}
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost. This cost adds up to other costs defined in
* the model, with the same unit.
*
* If defined, `soft_max_duration` must be nonnegative. If max_duration is
* also defined, `soft_max_duration` must be less than max_duration.
*
*
* .google.protobuf.Duration soft_max_duration = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getSoftMaxDurationFieldBuilder() {
if (softMaxDurationBuilder_ == null) {
softMaxDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getSoftMaxDuration(), getParentForChildren(), isClean());
softMaxDuration_ = null;
}
return softMaxDurationBuilder_;
}
private double costPerHourAfterSoftMax_;
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @return Whether the costPerHourAfterSoftMax field is set.
*/
@java.lang.Override
public boolean hasCostPerHourAfterSoftMax() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @return The costPerHourAfterSoftMax.
*/
@java.lang.Override
public double getCostPerHourAfterSoftMax() {
return costPerHourAfterSoftMax_;
}
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @param value The costPerHourAfterSoftMax to set.
* @return This builder for chaining.
*/
public Builder setCostPerHourAfterSoftMax(double value) {
costPerHourAfterSoftMax_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Cost per hour incurred if the `soft_max_duration` threshold is violated.
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
* ```
* cost_per_hour_after_soft_max * (duration - soft_max_duration)
* ```
* The cost must be nonnegative.
*
*
* optional double cost_per_hour_after_soft_max = 3;
*
* @return This builder for chaining.
*/
public Builder clearCostPerHourAfterSoftMax() {
bitField0_ = (bitField0_ & ~0x00000004);
costPerHourAfterSoftMax_ = 0D;
onChanged();
return this;
}
private com.google.protobuf.Duration quadraticSoftMaxDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
quadraticSoftMaxDurationBuilder_;
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*
* @return Whether the quadraticSoftMaxDuration field is set.
*/
public boolean hasQuadraticSoftMaxDuration() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*
* @return The quadraticSoftMaxDuration.
*/
public com.google.protobuf.Duration getQuadraticSoftMaxDuration() {
if (quadraticSoftMaxDurationBuilder_ == null) {
return quadraticSoftMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: quadraticSoftMaxDuration_;
} else {
return quadraticSoftMaxDurationBuilder_.getMessage();
}
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
public Builder setQuadraticSoftMaxDuration(com.google.protobuf.Duration value) {
if (quadraticSoftMaxDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
quadraticSoftMaxDuration_ = value;
} else {
quadraticSoftMaxDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
public Builder setQuadraticSoftMaxDuration(
com.google.protobuf.Duration.Builder builderForValue) {
if (quadraticSoftMaxDurationBuilder_ == null) {
quadraticSoftMaxDuration_ = builderForValue.build();
} else {
quadraticSoftMaxDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
public Builder mergeQuadraticSoftMaxDuration(com.google.protobuf.Duration value) {
if (quadraticSoftMaxDurationBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& quadraticSoftMaxDuration_ != null
&& quadraticSoftMaxDuration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getQuadraticSoftMaxDurationBuilder().mergeFrom(value);
} else {
quadraticSoftMaxDuration_ = value;
}
} else {
quadraticSoftMaxDurationBuilder_.mergeFrom(value);
}
if (quadraticSoftMaxDuration_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
public Builder clearQuadraticSoftMaxDuration() {
bitField0_ = (bitField0_ & ~0x00000008);
quadraticSoftMaxDuration_ = null;
if (quadraticSoftMaxDurationBuilder_ != null) {
quadraticSoftMaxDurationBuilder_.dispose();
quadraticSoftMaxDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
public com.google.protobuf.Duration.Builder getQuadraticSoftMaxDurationBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getQuadraticSoftMaxDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
public com.google.protobuf.DurationOrBuilder getQuadraticSoftMaxDurationOrBuilder() {
if (quadraticSoftMaxDurationBuilder_ != null) {
return quadraticSoftMaxDurationBuilder_.getMessageOrBuilder();
} else {
return quadraticSoftMaxDuration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: quadraticSoftMaxDuration_;
}
}
/**
*
*
*
* A soft limit not enforcing a maximum duration limit, but when violated
* makes the route incur a cost, quadratic in the duration. This cost adds
* up to other costs defined in the model, with the same unit.
*
* If defined, `quadratic_soft_max_duration` must be nonnegative. If
* `max_duration` is also defined, `quadratic_soft_max_duration` must be
* less than `max_duration`, and the difference must be no larger than one
* day:
*
* `max_duration - quadratic_soft_max_duration <= 86400 seconds`
*
*
* .google.protobuf.Duration quadratic_soft_max_duration = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getQuadraticSoftMaxDurationFieldBuilder() {
if (quadraticSoftMaxDurationBuilder_ == null) {
quadraticSoftMaxDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getQuadraticSoftMaxDuration(), getParentForChildren(), isClean());
quadraticSoftMaxDuration_ = null;
}
return quadraticSoftMaxDurationBuilder_;
}
private double costPerSquareHourAfterQuadraticSoftMax_;
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @return Whether the costPerSquareHourAfterQuadraticSoftMax field is set.
*/
@java.lang.Override
public boolean hasCostPerSquareHourAfterQuadraticSoftMax() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @return The costPerSquareHourAfterQuadraticSoftMax.
*/
@java.lang.Override
public double getCostPerSquareHourAfterQuadraticSoftMax() {
return costPerSquareHourAfterQuadraticSoftMax_;
}
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @param value The costPerSquareHourAfterQuadraticSoftMax to set.
* @return This builder for chaining.
*/
public Builder setCostPerSquareHourAfterQuadraticSoftMax(double value) {
costPerSquareHourAfterQuadraticSoftMax_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Cost per square hour incurred if the
* `quadratic_soft_max_duration` threshold is violated.
*
* The additional cost is 0 if the duration is under the threshold,
* otherwise the cost depends on the duration as follows:
*
* ```
* cost_per_square_hour_after_quadratic_soft_max *
* (duration - quadratic_soft_max_duration)^2
* ```
*
* The cost must be nonnegative.
*
*
* optional double cost_per_square_hour_after_quadratic_soft_max = 5;
*
* @return This builder for chaining.
*/
public Builder clearCostPerSquareHourAfterQuadraticSoftMax() {
bitField0_ = (bitField0_ & ~0x00000010);
costPerSquareHourAfterQuadraticSoftMax_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.Vehicle.DurationLimit)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.Vehicle.DurationLimit)
private static final com.google.maps.routeoptimization.v1.Vehicle.DurationLimit
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.Vehicle.DurationLimit();
}
public static com.google.maps.routeoptimization.v1.Vehicle.DurationLimit getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DurationLimit parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int DISPLAY_NAME_FIELD_NUMBER = 32;
@SuppressWarnings("serial")
private volatile java.lang.Object displayName_ = "";
/**
*
*
*
* The user-defined display name of the vehicle.
* It can be up to 63 characters long and may use UTF-8 characters.
*
*
* string display_name = 32;
*
* @return The displayName.
*/
@java.lang.Override
public java.lang.String getDisplayName() {
java.lang.Object ref = displayName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
displayName_ = s;
return s;
}
}
/**
*
*
*
* The user-defined display name of the vehicle.
* It can be up to 63 characters long and may use UTF-8 characters.
*
*
* string display_name = 32;
*
* @return The bytes for displayName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDisplayNameBytes() {
java.lang.Object ref = displayName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
displayName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRAVEL_MODE_FIELD_NUMBER = 1;
private int travelMode_ = 0;
/**
*
*
*
* The travel mode which affects the roads usable by the vehicle and its
* speed. See also `travel_duration_multiple`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.TravelMode travel_mode = 1;
*
* @return The enum numeric value on the wire for travelMode.
*/
@java.lang.Override
public int getTravelModeValue() {
return travelMode_;
}
/**
*
*
*
* The travel mode which affects the roads usable by the vehicle and its
* speed. See also `travel_duration_multiple`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.TravelMode travel_mode = 1;
*
* @return The travelMode.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.TravelMode getTravelMode() {
com.google.maps.routeoptimization.v1.Vehicle.TravelMode result =
com.google.maps.routeoptimization.v1.Vehicle.TravelMode.forNumber(travelMode_);
return result == null
? com.google.maps.routeoptimization.v1.Vehicle.TravelMode.UNRECOGNIZED
: result;
}
public static final int ROUTE_MODIFIERS_FIELD_NUMBER = 2;
private com.google.maps.routeoptimization.v1.RouteModifiers routeModifiers_;
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*
* @return Whether the routeModifiers field is set.
*/
@java.lang.Override
public boolean hasRouteModifiers() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*
* @return The routeModifiers.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.RouteModifiers getRouteModifiers() {
return routeModifiers_ == null
? com.google.maps.routeoptimization.v1.RouteModifiers.getDefaultInstance()
: routeModifiers_;
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.RouteModifiersOrBuilder getRouteModifiersOrBuilder() {
return routeModifiers_ == null
? com.google.maps.routeoptimization.v1.RouteModifiers.getDefaultInstance()
: routeModifiers_;
}
public static final int START_LOCATION_FIELD_NUMBER = 3;
private com.google.type.LatLng startLocation_;
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*
* @return Whether the startLocation field is set.
*/
@java.lang.Override
public boolean hasStartLocation() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*
* @return The startLocation.
*/
@java.lang.Override
public com.google.type.LatLng getStartLocation() {
return startLocation_ == null ? com.google.type.LatLng.getDefaultInstance() : startLocation_;
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
@java.lang.Override
public com.google.type.LatLngOrBuilder getStartLocationOrBuilder() {
return startLocation_ == null ? com.google.type.LatLng.getDefaultInstance() : startLocation_;
}
public static final int START_WAYPOINT_FIELD_NUMBER = 4;
private com.google.maps.routeoptimization.v1.Waypoint startWaypoint_;
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*
* @return Whether the startWaypoint field is set.
*/
@java.lang.Override
public boolean hasStartWaypoint() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*
* @return The startWaypoint.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Waypoint getStartWaypoint() {
return startWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: startWaypoint_;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.WaypointOrBuilder getStartWaypointOrBuilder() {
return startWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: startWaypoint_;
}
public static final int END_LOCATION_FIELD_NUMBER = 5;
private com.google.type.LatLng endLocation_;
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*
* @return Whether the endLocation field is set.
*/
@java.lang.Override
public boolean hasEndLocation() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*
* @return The endLocation.
*/
@java.lang.Override
public com.google.type.LatLng getEndLocation() {
return endLocation_ == null ? com.google.type.LatLng.getDefaultInstance() : endLocation_;
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
@java.lang.Override
public com.google.type.LatLngOrBuilder getEndLocationOrBuilder() {
return endLocation_ == null ? com.google.type.LatLng.getDefaultInstance() : endLocation_;
}
public static final int END_WAYPOINT_FIELD_NUMBER = 6;
private com.google.maps.routeoptimization.v1.Waypoint endWaypoint_;
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*
* @return Whether the endWaypoint field is set.
*/
@java.lang.Override
public boolean hasEndWaypoint() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*
* @return The endWaypoint.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Waypoint getEndWaypoint() {
return endWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: endWaypoint_;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.WaypointOrBuilder getEndWaypointOrBuilder() {
return endWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: endWaypoint_;
}
public static final int START_TAGS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList startTags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @return A list containing the startTags.
*/
public com.google.protobuf.ProtocolStringList getStartTagsList() {
return startTags_;
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @return The count of startTags.
*/
public int getStartTagsCount() {
return startTags_.size();
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param index The index of the element to return.
* @return The startTags at the given index.
*/
public java.lang.String getStartTags(int index) {
return startTags_.get(index);
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param index The index of the value to return.
* @return The bytes of the startTags at the given index.
*/
public com.google.protobuf.ByteString getStartTagsBytes(int index) {
return startTags_.getByteString(index);
}
public static final int END_TAGS_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList endTags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @return A list containing the endTags.
*/
public com.google.protobuf.ProtocolStringList getEndTagsList() {
return endTags_;
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @return The count of endTags.
*/
public int getEndTagsCount() {
return endTags_.size();
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param index The index of the element to return.
* @return The endTags at the given index.
*/
public java.lang.String getEndTags(int index) {
return endTags_.get(index);
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param index The index of the value to return.
* @return The bytes of the endTags at the given index.
*/
public com.google.protobuf.ByteString getEndTagsBytes(int index) {
return endTags_.getByteString(index);
}
public static final int START_TIME_WINDOWS_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private java.util.List startTimeWindows_;
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
@java.lang.Override
public java.util.List getStartTimeWindowsList() {
return startTimeWindows_;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
@java.lang.Override
public java.util.List extends com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
getStartTimeWindowsOrBuilderList() {
return startTimeWindows_;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
@java.lang.Override
public int getStartTimeWindowsCount() {
return startTimeWindows_.size();
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.TimeWindow getStartTimeWindows(int index) {
return startTimeWindows_.get(index);
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.TimeWindowOrBuilder getStartTimeWindowsOrBuilder(
int index) {
return startTimeWindows_.get(index);
}
public static final int END_TIME_WINDOWS_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private java.util.List endTimeWindows_;
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
@java.lang.Override
public java.util.List getEndTimeWindowsList() {
return endTimeWindows_;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
@java.lang.Override
public java.util.List extends com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
getEndTimeWindowsOrBuilderList() {
return endTimeWindows_;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
@java.lang.Override
public int getEndTimeWindowsCount() {
return endTimeWindows_.size();
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.TimeWindow getEndTimeWindows(int index) {
return endTimeWindows_.get(index);
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.TimeWindowOrBuilder getEndTimeWindowsOrBuilder(
int index) {
return endTimeWindows_.get(index);
}
public static final int TRAVEL_DURATION_MULTIPLE_FIELD_NUMBER = 11;
private double travelDurationMultiple_ = 0D;
/**
*
*
*
* Specifies a multiplicative factor that can be used to increase or decrease
* travel times of this vehicle. For example, setting this to 2.0 means
* that this vehicle is slower and has travel times that are twice what they
* are for standard vehicles. This multiple does not affect visit durations.
* It does affect cost if `cost_per_hour` or `cost_per_traveled_hour` are
* specified. This must be in the range [0.001, 1000.0]. If unset, the vehicle
* is standard, and this multiple is considered 1.0.
*
* WARNING: Travel times will be rounded to the nearest second after this
* multiple is applied but before performing any numerical operations, thus,
* a small multiple may result in a loss of precision.
*
* See also `extra_visit_duration_for_visit_type` below.
*
*
* optional double travel_duration_multiple = 11;
*
* @return Whether the travelDurationMultiple field is set.
*/
@java.lang.Override
public boolean hasTravelDurationMultiple() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Specifies a multiplicative factor that can be used to increase or decrease
* travel times of this vehicle. For example, setting this to 2.0 means
* that this vehicle is slower and has travel times that are twice what they
* are for standard vehicles. This multiple does not affect visit durations.
* It does affect cost if `cost_per_hour` or `cost_per_traveled_hour` are
* specified. This must be in the range [0.001, 1000.0]. If unset, the vehicle
* is standard, and this multiple is considered 1.0.
*
* WARNING: Travel times will be rounded to the nearest second after this
* multiple is applied but before performing any numerical operations, thus,
* a small multiple may result in a loss of precision.
*
* See also `extra_visit_duration_for_visit_type` below.
*
*
* optional double travel_duration_multiple = 11;
*
* @return The travelDurationMultiple.
*/
@java.lang.Override
public double getTravelDurationMultiple() {
return travelDurationMultiple_;
}
public static final int UNLOADING_POLICY_FIELD_NUMBER = 12;
private int unloadingPolicy_ = 0;
/**
*
*
*
* Unloading policy enforced on the vehicle.
*
*
* .google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy unloading_policy = 12;
*
* @return The enum numeric value on the wire for unloadingPolicy.
*/
@java.lang.Override
public int getUnloadingPolicyValue() {
return unloadingPolicy_;
}
/**
*
*
*
* Unloading policy enforced on the vehicle.
*
*
* .google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy unloading_policy = 12;
*
* @return The unloadingPolicy.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy getUnloadingPolicy() {
com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy result =
com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy.forNumber(unloadingPolicy_);
return result == null
? com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy.UNRECOGNIZED
: result;
}
public static final int LOAD_LIMITS_FIELD_NUMBER = 30;
private static final class LoadLimitsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_LoadLimitsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit
.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
loadLimits_;
private com.google.protobuf.MapField<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
internalGetLoadLimits() {
if (loadLimits_ == null) {
return com.google.protobuf.MapField.emptyMapField(LoadLimitsDefaultEntryHolder.defaultEntry);
}
return loadLimits_;
}
public int getLoadLimitsCount() {
return internalGetLoadLimits().getMap().size();
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public boolean containsLoadLimits(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLoadLimits().getMap().containsKey(key);
}
/** Use {@link #getLoadLimitsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getLoadLimits() {
return getLoadLimitsMap();
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public java.util.Map
getLoadLimitsMap() {
return internalGetLoadLimits().getMap();
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public /* nullable */ com.google.maps.routeoptimization.v1.Vehicle.LoadLimit
getLoadLimitsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetLoadLimits().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit getLoadLimitsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetLoadLimits().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int COST_PER_HOUR_FIELD_NUMBER = 16;
private double costPerHour_ = 0D;
/**
*
*
*
* Vehicle costs: all costs add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
* Cost per hour of the vehicle route. This cost is applied to the total time
* taken by the route, and includes travel time, waiting time, and visit time.
* Using `cost_per_hour` instead of just `cost_per_traveled_hour` may result
* in additional latency.
*
*
* double cost_per_hour = 16;
*
* @return The costPerHour.
*/
@java.lang.Override
public double getCostPerHour() {
return costPerHour_;
}
public static final int COST_PER_TRAVELED_HOUR_FIELD_NUMBER = 17;
private double costPerTraveledHour_ = 0D;
/**
*
*
*
* Cost per traveled hour of the vehicle route. This cost is applied only to
* travel time taken by the route (i.e., that reported in
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]),
* and excludes waiting time and visit time.
*
*
* double cost_per_traveled_hour = 17;
*
* @return The costPerTraveledHour.
*/
@java.lang.Override
public double getCostPerTraveledHour() {
return costPerTraveledHour_;
}
public static final int COST_PER_KILOMETER_FIELD_NUMBER = 18;
private double costPerKilometer_ = 0D;
/**
*
*
*
* Cost per kilometer of the vehicle route. This cost is applied to the
* distance reported in the
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* and does not apply to any distance implicitly traveled from the
* `arrival_location` to the `departure_location` of a single `VisitRequest`.
*
*
* double cost_per_kilometer = 18;
*
* @return The costPerKilometer.
*/
@java.lang.Override
public double getCostPerKilometer() {
return costPerKilometer_;
}
public static final int FIXED_COST_FIELD_NUMBER = 19;
private double fixedCost_ = 0D;
/**
*
*
*
* Fixed cost applied if this vehicle is used to handle a shipment.
*
*
* double fixed_cost = 19;
*
* @return The fixedCost.
*/
@java.lang.Override
public double getFixedCost() {
return fixedCost_;
}
public static final int USED_IF_ROUTE_IS_EMPTY_FIELD_NUMBER = 20;
private boolean usedIfRouteIsEmpty_ = false;
/**
*
*
*
* This field only applies to vehicles when their route does not serve any
* shipments. It indicates if the vehicle should be considered as used or not
* in this case.
*
* If true, the vehicle goes from its start to its end location even if it
* doesn't serve any shipments, and time and distance costs resulting from its
* start --> end travel are taken into account.
*
* Otherwise, it doesn't travel from its start to its end location, and no
* `break_rule` or delay (from `TransitionAttributes`) are scheduled for this
* vehicle. In this case, the vehicle's `ShipmentRoute` doesn't contain any
* information except for the vehicle index and label.
*
*
* bool used_if_route_is_empty = 20;
*
* @return The usedIfRouteIsEmpty.
*/
@java.lang.Override
public boolean getUsedIfRouteIsEmpty() {
return usedIfRouteIsEmpty_;
}
public static final int ROUTE_DURATION_LIMIT_FIELD_NUMBER = 21;
private com.google.maps.routeoptimization.v1.Vehicle.DurationLimit routeDurationLimit_;
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
* @return Whether the routeDurationLimit field is set.
*/
@java.lang.Override
public boolean hasRouteDurationLimit() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
* @return The routeDurationLimit.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit getRouteDurationLimit() {
return routeDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: routeDurationLimit_;
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder
getRouteDurationLimitOrBuilder() {
return routeDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: routeDurationLimit_;
}
public static final int TRAVEL_DURATION_LIMIT_FIELD_NUMBER = 22;
private com.google.maps.routeoptimization.v1.Vehicle.DurationLimit travelDurationLimit_;
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*
* @return Whether the travelDurationLimit field is set.
*/
@java.lang.Override
public boolean hasTravelDurationLimit() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*
* @return The travelDurationLimit.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit getTravelDurationLimit() {
return travelDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: travelDurationLimit_;
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder
getTravelDurationLimitOrBuilder() {
return travelDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: travelDurationLimit_;
}
public static final int ROUTE_DISTANCE_LIMIT_FIELD_NUMBER = 23;
private com.google.maps.routeoptimization.v1.DistanceLimit routeDistanceLimit_;
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*
* @return Whether the routeDistanceLimit field is set.
*/
@java.lang.Override
public boolean hasRouteDistanceLimit() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*
* @return The routeDistanceLimit.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.DistanceLimit getRouteDistanceLimit() {
return routeDistanceLimit_ == null
? com.google.maps.routeoptimization.v1.DistanceLimit.getDefaultInstance()
: routeDistanceLimit_;
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.DistanceLimitOrBuilder
getRouteDistanceLimitOrBuilder() {
return routeDistanceLimit_ == null
? com.google.maps.routeoptimization.v1.DistanceLimit.getDefaultInstance()
: routeDistanceLimit_;
}
public static final int EXTRA_VISIT_DURATION_FOR_VISIT_TYPE_FIELD_NUMBER = 24;
private static final class ExtraVisitDurationForVisitTypeDefaultEntryHolder {
static final com.google.protobuf.MapEntry
defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_ExtraVisitDurationForVisitTypeEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.protobuf.Duration.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField
extraVisitDurationForVisitType_;
private com.google.protobuf.MapField
internalGetExtraVisitDurationForVisitType() {
if (extraVisitDurationForVisitType_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ExtraVisitDurationForVisitTypeDefaultEntryHolder.defaultEntry);
}
return extraVisitDurationForVisitType_;
}
public int getExtraVisitDurationForVisitTypeCount() {
return internalGetExtraVisitDurationForVisitType().getMap().size();
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public boolean containsExtraVisitDurationForVisitType(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetExtraVisitDurationForVisitType().getMap().containsKey(key);
}
/** Use {@link #getExtraVisitDurationForVisitTypeMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getExtraVisitDurationForVisitType() {
return getExtraVisitDurationForVisitTypeMap();
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public java.util.Map
getExtraVisitDurationForVisitTypeMap() {
return internalGetExtraVisitDurationForVisitType().getMap();
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public /* nullable */ com.google.protobuf.Duration getExtraVisitDurationForVisitTypeOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Duration defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetExtraVisitDurationForVisitType().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public com.google.protobuf.Duration getExtraVisitDurationForVisitTypeOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetExtraVisitDurationForVisitType().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int BREAK_RULE_FIELD_NUMBER = 25;
private com.google.maps.routeoptimization.v1.BreakRule breakRule_;
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*
* @return Whether the breakRule field is set.
*/
@java.lang.Override
public boolean hasBreakRule() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*
* @return The breakRule.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.BreakRule getBreakRule() {
return breakRule_ == null
? com.google.maps.routeoptimization.v1.BreakRule.getDefaultInstance()
: breakRule_;
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.BreakRuleOrBuilder getBreakRuleOrBuilder() {
return breakRule_ == null
? com.google.maps.routeoptimization.v1.BreakRule.getDefaultInstance()
: breakRule_;
}
public static final int LABEL_FIELD_NUMBER = 27;
@SuppressWarnings("serial")
private volatile java.lang.Object label_ = "";
/**
*
*
*
* Specifies a label for this vehicle. This label is reported in the response
* as the `vehicle_label` of the corresponding
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
*
* string label = 27;
*
* @return The label.
*/
@java.lang.Override
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
label_ = s;
return s;
}
}
/**
*
*
*
* Specifies a label for this vehicle. This label is reported in the response
* as the `vehicle_label` of the corresponding
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
*
* string label = 27;
*
* @return The bytes for label.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IGNORE_FIELD_NUMBER = 28;
private boolean ignore_ = false;
/**
*
*
*
* If true, `used_if_route_is_empty` must be false, and this vehicle will
* remain unused.
*
* If a shipment is performed by an ignored vehicle in
* `injected_first_solution_routes`, it is skipped in the first solution but
* is free to be performed in the response.
*
* If a shipment is performed by an ignored vehicle in
* `injected_solution_constraint` and any related pickup/delivery is
* constrained to remain on the vehicle (i.e., not relaxed to level
* `RELAX_ALL_AFTER_THRESHOLD`), it is skipped in the response.
* If a shipment has a non-empty `allowed_vehicle_indices` field and all of
* the allowed vehicles are ignored, it is skipped in the response.
*
*
* bool ignore = 28;
*
* @return The ignore.
*/
@java.lang.Override
public boolean getIgnore() {
return ignore_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (travelMode_
!= com.google.maps.routeoptimization.v1.Vehicle.TravelMode.TRAVEL_MODE_UNSPECIFIED
.getNumber()) {
output.writeEnum(1, travelMode_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(2, getRouteModifiers());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getStartLocation());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(4, getStartWaypoint());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(5, getEndLocation());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(6, getEndWaypoint());
}
for (int i = 0; i < startTags_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, startTags_.getRaw(i));
}
for (int i = 0; i < endTags_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, endTags_.getRaw(i));
}
for (int i = 0; i < startTimeWindows_.size(); i++) {
output.writeMessage(9, startTimeWindows_.get(i));
}
for (int i = 0; i < endTimeWindows_.size(); i++) {
output.writeMessage(10, endTimeWindows_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeDouble(11, travelDurationMultiple_);
}
if (unloadingPolicy_
!= com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy.UNLOADING_POLICY_UNSPECIFIED
.getNumber()) {
output.writeEnum(12, unloadingPolicy_);
}
if (java.lang.Double.doubleToRawLongBits(costPerHour_) != 0) {
output.writeDouble(16, costPerHour_);
}
if (java.lang.Double.doubleToRawLongBits(costPerTraveledHour_) != 0) {
output.writeDouble(17, costPerTraveledHour_);
}
if (java.lang.Double.doubleToRawLongBits(costPerKilometer_) != 0) {
output.writeDouble(18, costPerKilometer_);
}
if (java.lang.Double.doubleToRawLongBits(fixedCost_) != 0) {
output.writeDouble(19, fixedCost_);
}
if (usedIfRouteIsEmpty_ != false) {
output.writeBool(20, usedIfRouteIsEmpty_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(21, getRouteDurationLimit());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(22, getTravelDurationLimit());
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(23, getRouteDistanceLimit());
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output,
internalGetExtraVisitDurationForVisitType(),
ExtraVisitDurationForVisitTypeDefaultEntryHolder.defaultEntry,
24);
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(25, getBreakRule());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(label_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 27, label_);
}
if (ignore_ != false) {
output.writeBool(28, ignore_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetLoadLimits(), LoadLimitsDefaultEntryHolder.defaultEntry, 30);
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(displayName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 32, displayName_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (travelMode_
!= com.google.maps.routeoptimization.v1.Vehicle.TravelMode.TRAVEL_MODE_UNSPECIFIED
.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(1, travelMode_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(2, getRouteModifiers());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getStartLocation());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getStartWaypoint());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(5, getEndLocation());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getEndWaypoint());
}
{
int dataSize = 0;
for (int i = 0; i < startTags_.size(); i++) {
dataSize += computeStringSizeNoTag(startTags_.getRaw(i));
}
size += dataSize;
size += 1 * getStartTagsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < endTags_.size(); i++) {
dataSize += computeStringSizeNoTag(endTags_.getRaw(i));
}
size += dataSize;
size += 1 * getEndTagsList().size();
}
for (int i = 0; i < startTimeWindows_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(9, startTimeWindows_.get(i));
}
for (int i = 0; i < endTimeWindows_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(10, endTimeWindows_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(11, travelDurationMultiple_);
}
if (unloadingPolicy_
!= com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy.UNLOADING_POLICY_UNSPECIFIED
.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(12, unloadingPolicy_);
}
if (java.lang.Double.doubleToRawLongBits(costPerHour_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(16, costPerHour_);
}
if (java.lang.Double.doubleToRawLongBits(costPerTraveledHour_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(17, costPerTraveledHour_);
}
if (java.lang.Double.doubleToRawLongBits(costPerKilometer_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(18, costPerKilometer_);
}
if (java.lang.Double.doubleToRawLongBits(fixedCost_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(19, fixedCost_);
}
if (usedIfRouteIsEmpty_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(20, usedIfRouteIsEmpty_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(21, getRouteDurationLimit());
}
if (((bitField0_ & 0x00000080) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(22, getTravelDurationLimit());
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(23, getRouteDistanceLimit());
}
for (java.util.Map.Entry entry :
internalGetExtraVisitDurationForVisitType().getMap().entrySet()) {
com.google.protobuf.MapEntry
extraVisitDurationForVisitType__ =
ExtraVisitDurationForVisitTypeDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
24, extraVisitDurationForVisitType__);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(25, getBreakRule());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(label_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(27, label_);
}
if (ignore_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(28, ignore_);
}
for (java.util.Map.Entry<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
entry : internalGetLoadLimits().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
loadLimits__ =
LoadLimitsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(30, loadLimits__);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(displayName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(32, displayName_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.maps.routeoptimization.v1.Vehicle)) {
return super.equals(obj);
}
com.google.maps.routeoptimization.v1.Vehicle other =
(com.google.maps.routeoptimization.v1.Vehicle) obj;
if (!getDisplayName().equals(other.getDisplayName())) return false;
if (travelMode_ != other.travelMode_) return false;
if (hasRouteModifiers() != other.hasRouteModifiers()) return false;
if (hasRouteModifiers()) {
if (!getRouteModifiers().equals(other.getRouteModifiers())) return false;
}
if (hasStartLocation() != other.hasStartLocation()) return false;
if (hasStartLocation()) {
if (!getStartLocation().equals(other.getStartLocation())) return false;
}
if (hasStartWaypoint() != other.hasStartWaypoint()) return false;
if (hasStartWaypoint()) {
if (!getStartWaypoint().equals(other.getStartWaypoint())) return false;
}
if (hasEndLocation() != other.hasEndLocation()) return false;
if (hasEndLocation()) {
if (!getEndLocation().equals(other.getEndLocation())) return false;
}
if (hasEndWaypoint() != other.hasEndWaypoint()) return false;
if (hasEndWaypoint()) {
if (!getEndWaypoint().equals(other.getEndWaypoint())) return false;
}
if (!getStartTagsList().equals(other.getStartTagsList())) return false;
if (!getEndTagsList().equals(other.getEndTagsList())) return false;
if (!getStartTimeWindowsList().equals(other.getStartTimeWindowsList())) return false;
if (!getEndTimeWindowsList().equals(other.getEndTimeWindowsList())) return false;
if (hasTravelDurationMultiple() != other.hasTravelDurationMultiple()) return false;
if (hasTravelDurationMultiple()) {
if (java.lang.Double.doubleToLongBits(getTravelDurationMultiple())
!= java.lang.Double.doubleToLongBits(other.getTravelDurationMultiple())) return false;
}
if (unloadingPolicy_ != other.unloadingPolicy_) return false;
if (!internalGetLoadLimits().equals(other.internalGetLoadLimits())) return false;
if (java.lang.Double.doubleToLongBits(getCostPerHour())
!= java.lang.Double.doubleToLongBits(other.getCostPerHour())) return false;
if (java.lang.Double.doubleToLongBits(getCostPerTraveledHour())
!= java.lang.Double.doubleToLongBits(other.getCostPerTraveledHour())) return false;
if (java.lang.Double.doubleToLongBits(getCostPerKilometer())
!= java.lang.Double.doubleToLongBits(other.getCostPerKilometer())) return false;
if (java.lang.Double.doubleToLongBits(getFixedCost())
!= java.lang.Double.doubleToLongBits(other.getFixedCost())) return false;
if (getUsedIfRouteIsEmpty() != other.getUsedIfRouteIsEmpty()) return false;
if (hasRouteDurationLimit() != other.hasRouteDurationLimit()) return false;
if (hasRouteDurationLimit()) {
if (!getRouteDurationLimit().equals(other.getRouteDurationLimit())) return false;
}
if (hasTravelDurationLimit() != other.hasTravelDurationLimit()) return false;
if (hasTravelDurationLimit()) {
if (!getTravelDurationLimit().equals(other.getTravelDurationLimit())) return false;
}
if (hasRouteDistanceLimit() != other.hasRouteDistanceLimit()) return false;
if (hasRouteDistanceLimit()) {
if (!getRouteDistanceLimit().equals(other.getRouteDistanceLimit())) return false;
}
if (!internalGetExtraVisitDurationForVisitType()
.equals(other.internalGetExtraVisitDurationForVisitType())) return false;
if (hasBreakRule() != other.hasBreakRule()) return false;
if (hasBreakRule()) {
if (!getBreakRule().equals(other.getBreakRule())) return false;
}
if (!getLabel().equals(other.getLabel())) return false;
if (getIgnore() != other.getIgnore()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DISPLAY_NAME_FIELD_NUMBER;
hash = (53 * hash) + getDisplayName().hashCode();
hash = (37 * hash) + TRAVEL_MODE_FIELD_NUMBER;
hash = (53 * hash) + travelMode_;
if (hasRouteModifiers()) {
hash = (37 * hash) + ROUTE_MODIFIERS_FIELD_NUMBER;
hash = (53 * hash) + getRouteModifiers().hashCode();
}
if (hasStartLocation()) {
hash = (37 * hash) + START_LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getStartLocation().hashCode();
}
if (hasStartWaypoint()) {
hash = (37 * hash) + START_WAYPOINT_FIELD_NUMBER;
hash = (53 * hash) + getStartWaypoint().hashCode();
}
if (hasEndLocation()) {
hash = (37 * hash) + END_LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getEndLocation().hashCode();
}
if (hasEndWaypoint()) {
hash = (37 * hash) + END_WAYPOINT_FIELD_NUMBER;
hash = (53 * hash) + getEndWaypoint().hashCode();
}
if (getStartTagsCount() > 0) {
hash = (37 * hash) + START_TAGS_FIELD_NUMBER;
hash = (53 * hash) + getStartTagsList().hashCode();
}
if (getEndTagsCount() > 0) {
hash = (37 * hash) + END_TAGS_FIELD_NUMBER;
hash = (53 * hash) + getEndTagsList().hashCode();
}
if (getStartTimeWindowsCount() > 0) {
hash = (37 * hash) + START_TIME_WINDOWS_FIELD_NUMBER;
hash = (53 * hash) + getStartTimeWindowsList().hashCode();
}
if (getEndTimeWindowsCount() > 0) {
hash = (37 * hash) + END_TIME_WINDOWS_FIELD_NUMBER;
hash = (53 * hash) + getEndTimeWindowsList().hashCode();
}
if (hasTravelDurationMultiple()) {
hash = (37 * hash) + TRAVEL_DURATION_MULTIPLE_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTravelDurationMultiple()));
}
hash = (37 * hash) + UNLOADING_POLICY_FIELD_NUMBER;
hash = (53 * hash) + unloadingPolicy_;
if (!internalGetLoadLimits().getMap().isEmpty()) {
hash = (37 * hash) + LOAD_LIMITS_FIELD_NUMBER;
hash = (53 * hash) + internalGetLoadLimits().hashCode();
}
hash = (37 * hash) + COST_PER_HOUR_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCostPerHour()));
hash = (37 * hash) + COST_PER_TRAVELED_HOUR_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCostPerTraveledHour()));
hash = (37 * hash) + COST_PER_KILOMETER_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCostPerKilometer()));
hash = (37 * hash) + FIXED_COST_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getFixedCost()));
hash = (37 * hash) + USED_IF_ROUTE_IS_EMPTY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getUsedIfRouteIsEmpty());
if (hasRouteDurationLimit()) {
hash = (37 * hash) + ROUTE_DURATION_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getRouteDurationLimit().hashCode();
}
if (hasTravelDurationLimit()) {
hash = (37 * hash) + TRAVEL_DURATION_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getTravelDurationLimit().hashCode();
}
if (hasRouteDistanceLimit()) {
hash = (37 * hash) + ROUTE_DISTANCE_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getRouteDistanceLimit().hashCode();
}
if (!internalGetExtraVisitDurationForVisitType().getMap().isEmpty()) {
hash = (37 * hash) + EXTRA_VISIT_DURATION_FOR_VISIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + internalGetExtraVisitDurationForVisitType().hashCode();
}
if (hasBreakRule()) {
hash = (37 * hash) + BREAK_RULE_FIELD_NUMBER;
hash = (53 * hash) + getBreakRule().hashCode();
}
hash = (37 * hash) + LABEL_FIELD_NUMBER;
hash = (53 * hash) + getLabel().hashCode();
hash = (37 * hash) + IGNORE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getIgnore());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.maps.routeoptimization.v1.Vehicle parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.maps.routeoptimization.v1.Vehicle prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Models a vehicle in a shipment problem. Solving a shipment problem will
* build a route starting from `start_location` and ending at `end_location`
* for this vehicle. A route is a sequence of visits (see `ShipmentRoute`).
*
*
* Protobuf type {@code google.maps.routeoptimization.v1.Vehicle}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.maps.routeoptimization.v1.Vehicle)
com.google.maps.routeoptimization.v1.VehicleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 30:
return internalGetLoadLimits();
case 24:
return internalGetExtraVisitDurationForVisitType();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 30:
return internalGetMutableLoadLimits();
case 24:
return internalGetMutableExtraVisitDurationForVisitType();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.maps.routeoptimization.v1.Vehicle.class,
com.google.maps.routeoptimization.v1.Vehicle.Builder.class);
}
// Construct using com.google.maps.routeoptimization.v1.Vehicle.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getRouteModifiersFieldBuilder();
getStartLocationFieldBuilder();
getStartWaypointFieldBuilder();
getEndLocationFieldBuilder();
getEndWaypointFieldBuilder();
getStartTimeWindowsFieldBuilder();
getEndTimeWindowsFieldBuilder();
getRouteDurationLimitFieldBuilder();
getTravelDurationLimitFieldBuilder();
getRouteDistanceLimitFieldBuilder();
getBreakRuleFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
displayName_ = "";
travelMode_ = 0;
routeModifiers_ = null;
if (routeModifiersBuilder_ != null) {
routeModifiersBuilder_.dispose();
routeModifiersBuilder_ = null;
}
startLocation_ = null;
if (startLocationBuilder_ != null) {
startLocationBuilder_.dispose();
startLocationBuilder_ = null;
}
startWaypoint_ = null;
if (startWaypointBuilder_ != null) {
startWaypointBuilder_.dispose();
startWaypointBuilder_ = null;
}
endLocation_ = null;
if (endLocationBuilder_ != null) {
endLocationBuilder_.dispose();
endLocationBuilder_ = null;
}
endWaypoint_ = null;
if (endWaypointBuilder_ != null) {
endWaypointBuilder_.dispose();
endWaypointBuilder_ = null;
}
startTags_ = com.google.protobuf.LazyStringArrayList.emptyList();
endTags_ = com.google.protobuf.LazyStringArrayList.emptyList();
if (startTimeWindowsBuilder_ == null) {
startTimeWindows_ = java.util.Collections.emptyList();
} else {
startTimeWindows_ = null;
startTimeWindowsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (endTimeWindowsBuilder_ == null) {
endTimeWindows_ = java.util.Collections.emptyList();
} else {
endTimeWindows_ = null;
endTimeWindowsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
travelDurationMultiple_ = 0D;
unloadingPolicy_ = 0;
internalGetMutableLoadLimits().clear();
costPerHour_ = 0D;
costPerTraveledHour_ = 0D;
costPerKilometer_ = 0D;
fixedCost_ = 0D;
usedIfRouteIsEmpty_ = false;
routeDurationLimit_ = null;
if (routeDurationLimitBuilder_ != null) {
routeDurationLimitBuilder_.dispose();
routeDurationLimitBuilder_ = null;
}
travelDurationLimit_ = null;
if (travelDurationLimitBuilder_ != null) {
travelDurationLimitBuilder_.dispose();
travelDurationLimitBuilder_ = null;
}
routeDistanceLimit_ = null;
if (routeDistanceLimitBuilder_ != null) {
routeDistanceLimitBuilder_.dispose();
routeDistanceLimitBuilder_ = null;
}
internalGetMutableExtraVisitDurationForVisitType().clear();
breakRule_ = null;
if (breakRuleBuilder_ != null) {
breakRuleBuilder_.dispose();
breakRuleBuilder_ = null;
}
label_ = "";
ignore_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.maps.routeoptimization.v1.RouteOptimizationServiceProto
.internal_static_google_maps_routeoptimization_v1_Vehicle_descriptor;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle getDefaultInstanceForType() {
return com.google.maps.routeoptimization.v1.Vehicle.getDefaultInstance();
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle build() {
com.google.maps.routeoptimization.v1.Vehicle result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle buildPartial() {
com.google.maps.routeoptimization.v1.Vehicle result =
new com.google.maps.routeoptimization.v1.Vehicle(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.maps.routeoptimization.v1.Vehicle result) {
if (startTimeWindowsBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)) {
startTimeWindows_ = java.util.Collections.unmodifiableList(startTimeWindows_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.startTimeWindows_ = startTimeWindows_;
} else {
result.startTimeWindows_ = startTimeWindowsBuilder_.build();
}
if (endTimeWindowsBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)) {
endTimeWindows_ = java.util.Collections.unmodifiableList(endTimeWindows_);
bitField0_ = (bitField0_ & ~0x00000400);
}
result.endTimeWindows_ = endTimeWindows_;
} else {
result.endTimeWindows_ = endTimeWindowsBuilder_.build();
}
}
private void buildPartial0(com.google.maps.routeoptimization.v1.Vehicle result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.displayName_ = displayName_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.travelMode_ = travelMode_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.routeModifiers_ =
routeModifiersBuilder_ == null ? routeModifiers_ : routeModifiersBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.startLocation_ =
startLocationBuilder_ == null ? startLocation_ : startLocationBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.startWaypoint_ =
startWaypointBuilder_ == null ? startWaypoint_ : startWaypointBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.endLocation_ =
endLocationBuilder_ == null ? endLocation_ : endLocationBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.endWaypoint_ =
endWaypointBuilder_ == null ? endWaypoint_ : endWaypointBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
startTags_.makeImmutable();
result.startTags_ = startTags_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
endTags_.makeImmutable();
result.endTags_ = endTags_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.travelDurationMultiple_ = travelDurationMultiple_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.unloadingPolicy_ = unloadingPolicy_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.loadLimits_ =
internalGetLoadLimits().build(LoadLimitsDefaultEntryHolder.defaultEntry);
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.costPerHour_ = costPerHour_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.costPerTraveledHour_ = costPerTraveledHour_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.costPerKilometer_ = costPerKilometer_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.fixedCost_ = fixedCost_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.usedIfRouteIsEmpty_ = usedIfRouteIsEmpty_;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.routeDurationLimit_ =
routeDurationLimitBuilder_ == null
? routeDurationLimit_
: routeDurationLimitBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.travelDurationLimit_ =
travelDurationLimitBuilder_ == null
? travelDurationLimit_
: travelDurationLimitBuilder_.build();
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.routeDistanceLimit_ =
routeDistanceLimitBuilder_ == null
? routeDistanceLimit_
: routeDistanceLimitBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.extraVisitDurationForVisitType_ =
internalGetExtraVisitDurationForVisitType()
.build(ExtraVisitDurationForVisitTypeDefaultEntryHolder.defaultEntry);
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.breakRule_ = breakRuleBuilder_ == null ? breakRule_ : breakRuleBuilder_.build();
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.label_ = label_;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.ignore_ = ignore_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.maps.routeoptimization.v1.Vehicle) {
return mergeFrom((com.google.maps.routeoptimization.v1.Vehicle) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.maps.routeoptimization.v1.Vehicle other) {
if (other == com.google.maps.routeoptimization.v1.Vehicle.getDefaultInstance()) return this;
if (!other.getDisplayName().isEmpty()) {
displayName_ = other.displayName_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.travelMode_ != 0) {
setTravelModeValue(other.getTravelModeValue());
}
if (other.hasRouteModifiers()) {
mergeRouteModifiers(other.getRouteModifiers());
}
if (other.hasStartLocation()) {
mergeStartLocation(other.getStartLocation());
}
if (other.hasStartWaypoint()) {
mergeStartWaypoint(other.getStartWaypoint());
}
if (other.hasEndLocation()) {
mergeEndLocation(other.getEndLocation());
}
if (other.hasEndWaypoint()) {
mergeEndWaypoint(other.getEndWaypoint());
}
if (!other.startTags_.isEmpty()) {
if (startTags_.isEmpty()) {
startTags_ = other.startTags_;
bitField0_ |= 0x00000080;
} else {
ensureStartTagsIsMutable();
startTags_.addAll(other.startTags_);
}
onChanged();
}
if (!other.endTags_.isEmpty()) {
if (endTags_.isEmpty()) {
endTags_ = other.endTags_;
bitField0_ |= 0x00000100;
} else {
ensureEndTagsIsMutable();
endTags_.addAll(other.endTags_);
}
onChanged();
}
if (startTimeWindowsBuilder_ == null) {
if (!other.startTimeWindows_.isEmpty()) {
if (startTimeWindows_.isEmpty()) {
startTimeWindows_ = other.startTimeWindows_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureStartTimeWindowsIsMutable();
startTimeWindows_.addAll(other.startTimeWindows_);
}
onChanged();
}
} else {
if (!other.startTimeWindows_.isEmpty()) {
if (startTimeWindowsBuilder_.isEmpty()) {
startTimeWindowsBuilder_.dispose();
startTimeWindowsBuilder_ = null;
startTimeWindows_ = other.startTimeWindows_;
bitField0_ = (bitField0_ & ~0x00000200);
startTimeWindowsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getStartTimeWindowsFieldBuilder()
: null;
} else {
startTimeWindowsBuilder_.addAllMessages(other.startTimeWindows_);
}
}
}
if (endTimeWindowsBuilder_ == null) {
if (!other.endTimeWindows_.isEmpty()) {
if (endTimeWindows_.isEmpty()) {
endTimeWindows_ = other.endTimeWindows_;
bitField0_ = (bitField0_ & ~0x00000400);
} else {
ensureEndTimeWindowsIsMutable();
endTimeWindows_.addAll(other.endTimeWindows_);
}
onChanged();
}
} else {
if (!other.endTimeWindows_.isEmpty()) {
if (endTimeWindowsBuilder_.isEmpty()) {
endTimeWindowsBuilder_.dispose();
endTimeWindowsBuilder_ = null;
endTimeWindows_ = other.endTimeWindows_;
bitField0_ = (bitField0_ & ~0x00000400);
endTimeWindowsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getEndTimeWindowsFieldBuilder()
: null;
} else {
endTimeWindowsBuilder_.addAllMessages(other.endTimeWindows_);
}
}
}
if (other.hasTravelDurationMultiple()) {
setTravelDurationMultiple(other.getTravelDurationMultiple());
}
if (other.unloadingPolicy_ != 0) {
setUnloadingPolicyValue(other.getUnloadingPolicyValue());
}
internalGetMutableLoadLimits().mergeFrom(other.internalGetLoadLimits());
bitField0_ |= 0x00002000;
if (other.getCostPerHour() != 0D) {
setCostPerHour(other.getCostPerHour());
}
if (other.getCostPerTraveledHour() != 0D) {
setCostPerTraveledHour(other.getCostPerTraveledHour());
}
if (other.getCostPerKilometer() != 0D) {
setCostPerKilometer(other.getCostPerKilometer());
}
if (other.getFixedCost() != 0D) {
setFixedCost(other.getFixedCost());
}
if (other.getUsedIfRouteIsEmpty() != false) {
setUsedIfRouteIsEmpty(other.getUsedIfRouteIsEmpty());
}
if (other.hasRouteDurationLimit()) {
mergeRouteDurationLimit(other.getRouteDurationLimit());
}
if (other.hasTravelDurationLimit()) {
mergeTravelDurationLimit(other.getTravelDurationLimit());
}
if (other.hasRouteDistanceLimit()) {
mergeRouteDistanceLimit(other.getRouteDistanceLimit());
}
internalGetMutableExtraVisitDurationForVisitType()
.mergeFrom(other.internalGetExtraVisitDurationForVisitType());
bitField0_ |= 0x00400000;
if (other.hasBreakRule()) {
mergeBreakRule(other.getBreakRule());
}
if (!other.getLabel().isEmpty()) {
label_ = other.label_;
bitField0_ |= 0x01000000;
onChanged();
}
if (other.getIgnore() != false) {
setIgnore(other.getIgnore());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
travelMode_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 8
case 18:
{
input.readMessage(getRouteModifiersFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 18
case 26:
{
input.readMessage(getStartLocationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 26
case 34:
{
input.readMessage(getStartWaypointFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 34
case 42:
{
input.readMessage(getEndLocationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 42
case 50:
{
input.readMessage(getEndWaypointFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 50
case 58:
{
java.lang.String s = input.readStringRequireUtf8();
ensureStartTagsIsMutable();
startTags_.add(s);
break;
} // case 58
case 66:
{
java.lang.String s = input.readStringRequireUtf8();
ensureEndTagsIsMutable();
endTags_.add(s);
break;
} // case 66
case 74:
{
com.google.maps.routeoptimization.v1.TimeWindow m =
input.readMessage(
com.google.maps.routeoptimization.v1.TimeWindow.parser(),
extensionRegistry);
if (startTimeWindowsBuilder_ == null) {
ensureStartTimeWindowsIsMutable();
startTimeWindows_.add(m);
} else {
startTimeWindowsBuilder_.addMessage(m);
}
break;
} // case 74
case 82:
{
com.google.maps.routeoptimization.v1.TimeWindow m =
input.readMessage(
com.google.maps.routeoptimization.v1.TimeWindow.parser(),
extensionRegistry);
if (endTimeWindowsBuilder_ == null) {
ensureEndTimeWindowsIsMutable();
endTimeWindows_.add(m);
} else {
endTimeWindowsBuilder_.addMessage(m);
}
break;
} // case 82
case 89:
{
travelDurationMultiple_ = input.readDouble();
bitField0_ |= 0x00000800;
break;
} // case 89
case 96:
{
unloadingPolicy_ = input.readEnum();
bitField0_ |= 0x00001000;
break;
} // case 96
case 129:
{
costPerHour_ = input.readDouble();
bitField0_ |= 0x00004000;
break;
} // case 129
case 137:
{
costPerTraveledHour_ = input.readDouble();
bitField0_ |= 0x00008000;
break;
} // case 137
case 145:
{
costPerKilometer_ = input.readDouble();
bitField0_ |= 0x00010000;
break;
} // case 145
case 153:
{
fixedCost_ = input.readDouble();
bitField0_ |= 0x00020000;
break;
} // case 153
case 160:
{
usedIfRouteIsEmpty_ = input.readBool();
bitField0_ |= 0x00040000;
break;
} // case 160
case 170:
{
input.readMessage(
getRouteDurationLimitFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00080000;
break;
} // case 170
case 178:
{
input.readMessage(
getTravelDurationLimitFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00100000;
break;
} // case 178
case 186:
{
input.readMessage(
getRouteDistanceLimitFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00200000;
break;
} // case 186
case 194:
{
com.google.protobuf.MapEntry
extraVisitDurationForVisitType__ =
input.readMessage(
ExtraVisitDurationForVisitTypeDefaultEntryHolder.defaultEntry
.getParserForType(),
extensionRegistry);
internalGetMutableExtraVisitDurationForVisitType()
.ensureBuilderMap()
.put(
extraVisitDurationForVisitType__.getKey(),
extraVisitDurationForVisitType__.getValue());
bitField0_ |= 0x00400000;
break;
} // case 194
case 202:
{
input.readMessage(getBreakRuleFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00800000;
break;
} // case 202
case 218:
{
label_ = input.readStringRequireUtf8();
bitField0_ |= 0x01000000;
break;
} // case 218
case 224:
{
ignore_ = input.readBool();
bitField0_ |= 0x02000000;
break;
} // case 224
case 242:
{
com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
loadLimits__ =
input.readMessage(
LoadLimitsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableLoadLimits()
.ensureBuilderMap()
.put(loadLimits__.getKey(), loadLimits__.getValue());
bitField0_ |= 0x00002000;
break;
} // case 242
case 258:
{
displayName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 258
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object displayName_ = "";
/**
*
*
*
* The user-defined display name of the vehicle.
* It can be up to 63 characters long and may use UTF-8 characters.
*
*
* string display_name = 32;
*
* @return The displayName.
*/
public java.lang.String getDisplayName() {
java.lang.Object ref = displayName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
displayName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The user-defined display name of the vehicle.
* It can be up to 63 characters long and may use UTF-8 characters.
*
*
* string display_name = 32;
*
* @return The bytes for displayName.
*/
public com.google.protobuf.ByteString getDisplayNameBytes() {
java.lang.Object ref = displayName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
displayName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The user-defined display name of the vehicle.
* It can be up to 63 characters long and may use UTF-8 characters.
*
*
* string display_name = 32;
*
* @param value The displayName to set.
* @return This builder for chaining.
*/
public Builder setDisplayName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
displayName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The user-defined display name of the vehicle.
* It can be up to 63 characters long and may use UTF-8 characters.
*
*
* string display_name = 32;
*
* @return This builder for chaining.
*/
public Builder clearDisplayName() {
displayName_ = getDefaultInstance().getDisplayName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The user-defined display name of the vehicle.
* It can be up to 63 characters long and may use UTF-8 characters.
*
*
* string display_name = 32;
*
* @param value The bytes for displayName to set.
* @return This builder for chaining.
*/
public Builder setDisplayNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
displayName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int travelMode_ = 0;
/**
*
*
*
* The travel mode which affects the roads usable by the vehicle and its
* speed. See also `travel_duration_multiple`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.TravelMode travel_mode = 1;
*
* @return The enum numeric value on the wire for travelMode.
*/
@java.lang.Override
public int getTravelModeValue() {
return travelMode_;
}
/**
*
*
*
* The travel mode which affects the roads usable by the vehicle and its
* speed. See also `travel_duration_multiple`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.TravelMode travel_mode = 1;
*
* @param value The enum numeric value on the wire for travelMode to set.
* @return This builder for chaining.
*/
public Builder setTravelModeValue(int value) {
travelMode_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The travel mode which affects the roads usable by the vehicle and its
* speed. See also `travel_duration_multiple`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.TravelMode travel_mode = 1;
*
* @return The travelMode.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.TravelMode getTravelMode() {
com.google.maps.routeoptimization.v1.Vehicle.TravelMode result =
com.google.maps.routeoptimization.v1.Vehicle.TravelMode.forNumber(travelMode_);
return result == null
? com.google.maps.routeoptimization.v1.Vehicle.TravelMode.UNRECOGNIZED
: result;
}
/**
*
*
*
* The travel mode which affects the roads usable by the vehicle and its
* speed. See also `travel_duration_multiple`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.TravelMode travel_mode = 1;
*
* @param value The travelMode to set.
* @return This builder for chaining.
*/
public Builder setTravelMode(com.google.maps.routeoptimization.v1.Vehicle.TravelMode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
travelMode_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* The travel mode which affects the roads usable by the vehicle and its
* speed. See also `travel_duration_multiple`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.TravelMode travel_mode = 1;
*
* @return This builder for chaining.
*/
public Builder clearTravelMode() {
bitField0_ = (bitField0_ & ~0x00000002);
travelMode_ = 0;
onChanged();
return this;
}
private com.google.maps.routeoptimization.v1.RouteModifiers routeModifiers_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.RouteModifiers,
com.google.maps.routeoptimization.v1.RouteModifiers.Builder,
com.google.maps.routeoptimization.v1.RouteModifiersOrBuilder>
routeModifiersBuilder_;
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*
* @return Whether the routeModifiers field is set.
*/
public boolean hasRouteModifiers() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*
* @return The routeModifiers.
*/
public com.google.maps.routeoptimization.v1.RouteModifiers getRouteModifiers() {
if (routeModifiersBuilder_ == null) {
return routeModifiers_ == null
? com.google.maps.routeoptimization.v1.RouteModifiers.getDefaultInstance()
: routeModifiers_;
} else {
return routeModifiersBuilder_.getMessage();
}
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
public Builder setRouteModifiers(com.google.maps.routeoptimization.v1.RouteModifiers value) {
if (routeModifiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
routeModifiers_ = value;
} else {
routeModifiersBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
public Builder setRouteModifiers(
com.google.maps.routeoptimization.v1.RouteModifiers.Builder builderForValue) {
if (routeModifiersBuilder_ == null) {
routeModifiers_ = builderForValue.build();
} else {
routeModifiersBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
public Builder mergeRouteModifiers(com.google.maps.routeoptimization.v1.RouteModifiers value) {
if (routeModifiersBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& routeModifiers_ != null
&& routeModifiers_
!= com.google.maps.routeoptimization.v1.RouteModifiers.getDefaultInstance()) {
getRouteModifiersBuilder().mergeFrom(value);
} else {
routeModifiers_ = value;
}
} else {
routeModifiersBuilder_.mergeFrom(value);
}
if (routeModifiers_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
public Builder clearRouteModifiers() {
bitField0_ = (bitField0_ & ~0x00000004);
routeModifiers_ = null;
if (routeModifiersBuilder_ != null) {
routeModifiersBuilder_.dispose();
routeModifiersBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
public com.google.maps.routeoptimization.v1.RouteModifiers.Builder getRouteModifiersBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getRouteModifiersFieldBuilder().getBuilder();
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
public com.google.maps.routeoptimization.v1.RouteModifiersOrBuilder
getRouteModifiersOrBuilder() {
if (routeModifiersBuilder_ != null) {
return routeModifiersBuilder_.getMessageOrBuilder();
} else {
return routeModifiers_ == null
? com.google.maps.routeoptimization.v1.RouteModifiers.getDefaultInstance()
: routeModifiers_;
}
}
/**
*
*
*
* A set of conditions to satisfy that affect the way routes are calculated
* for the given vehicle.
*
*
* .google.maps.routeoptimization.v1.RouteModifiers route_modifiers = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.RouteModifiers,
com.google.maps.routeoptimization.v1.RouteModifiers.Builder,
com.google.maps.routeoptimization.v1.RouteModifiersOrBuilder>
getRouteModifiersFieldBuilder() {
if (routeModifiersBuilder_ == null) {
routeModifiersBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.RouteModifiers,
com.google.maps.routeoptimization.v1.RouteModifiers.Builder,
com.google.maps.routeoptimization.v1.RouteModifiersOrBuilder>(
getRouteModifiers(), getParentForChildren(), isClean());
routeModifiers_ = null;
}
return routeModifiersBuilder_;
}
private com.google.type.LatLng startLocation_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.type.LatLng, com.google.type.LatLng.Builder, com.google.type.LatLngOrBuilder>
startLocationBuilder_;
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*
* @return Whether the startLocation field is set.
*/
public boolean hasStartLocation() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*
* @return The startLocation.
*/
public com.google.type.LatLng getStartLocation() {
if (startLocationBuilder_ == null) {
return startLocation_ == null
? com.google.type.LatLng.getDefaultInstance()
: startLocation_;
} else {
return startLocationBuilder_.getMessage();
}
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
public Builder setStartLocation(com.google.type.LatLng value) {
if (startLocationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startLocation_ = value;
} else {
startLocationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
public Builder setStartLocation(com.google.type.LatLng.Builder builderForValue) {
if (startLocationBuilder_ == null) {
startLocation_ = builderForValue.build();
} else {
startLocationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
public Builder mergeStartLocation(com.google.type.LatLng value) {
if (startLocationBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& startLocation_ != null
&& startLocation_ != com.google.type.LatLng.getDefaultInstance()) {
getStartLocationBuilder().mergeFrom(value);
} else {
startLocation_ = value;
}
} else {
startLocationBuilder_.mergeFrom(value);
}
if (startLocation_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
public Builder clearStartLocation() {
bitField0_ = (bitField0_ & ~0x00000008);
startLocation_ = null;
if (startLocationBuilder_ != null) {
startLocationBuilder_.dispose();
startLocationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
public com.google.type.LatLng.Builder getStartLocationBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getStartLocationFieldBuilder().getBuilder();
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
public com.google.type.LatLngOrBuilder getStartLocationOrBuilder() {
if (startLocationBuilder_ != null) {
return startLocationBuilder_.getMessageOrBuilder();
} else {
return startLocation_ == null
? com.google.type.LatLng.getDefaultInstance()
: startLocation_;
}
}
/**
*
*
*
* Geographic location where the vehicle starts before picking up any
* shipments. If not specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_location`
* must not be specified.
*
*
* .google.type.LatLng start_location = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.type.LatLng, com.google.type.LatLng.Builder, com.google.type.LatLngOrBuilder>
getStartLocationFieldBuilder() {
if (startLocationBuilder_ == null) {
startLocationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.type.LatLng,
com.google.type.LatLng.Builder,
com.google.type.LatLngOrBuilder>(
getStartLocation(), getParentForChildren(), isClean());
startLocation_ = null;
}
return startLocationBuilder_;
}
private com.google.maps.routeoptimization.v1.Waypoint startWaypoint_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Waypoint,
com.google.maps.routeoptimization.v1.Waypoint.Builder,
com.google.maps.routeoptimization.v1.WaypointOrBuilder>
startWaypointBuilder_;
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*
* @return Whether the startWaypoint field is set.
*/
public boolean hasStartWaypoint() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*
* @return The startWaypoint.
*/
public com.google.maps.routeoptimization.v1.Waypoint getStartWaypoint() {
if (startWaypointBuilder_ == null) {
return startWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: startWaypoint_;
} else {
return startWaypointBuilder_.getMessage();
}
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
public Builder setStartWaypoint(com.google.maps.routeoptimization.v1.Waypoint value) {
if (startWaypointBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startWaypoint_ = value;
} else {
startWaypointBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
public Builder setStartWaypoint(
com.google.maps.routeoptimization.v1.Waypoint.Builder builderForValue) {
if (startWaypointBuilder_ == null) {
startWaypoint_ = builderForValue.build();
} else {
startWaypointBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
public Builder mergeStartWaypoint(com.google.maps.routeoptimization.v1.Waypoint value) {
if (startWaypointBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)
&& startWaypoint_ != null
&& startWaypoint_
!= com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()) {
getStartWaypointBuilder().mergeFrom(value);
} else {
startWaypoint_ = value;
}
} else {
startWaypointBuilder_.mergeFrom(value);
}
if (startWaypoint_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
public Builder clearStartWaypoint() {
bitField0_ = (bitField0_ & ~0x00000010);
startWaypoint_ = null;
if (startWaypointBuilder_ != null) {
startWaypointBuilder_.dispose();
startWaypointBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
public com.google.maps.routeoptimization.v1.Waypoint.Builder getStartWaypointBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getStartWaypointFieldBuilder().getBuilder();
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
public com.google.maps.routeoptimization.v1.WaypointOrBuilder getStartWaypointOrBuilder() {
if (startWaypointBuilder_ != null) {
return startWaypointBuilder_.getMessageOrBuilder();
} else {
return startWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: startWaypoint_;
}
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle starts before
* picking up any shipments. If neither `start_waypoint` nor `start_location`
* is specified, the vehicle starts at its first pickup.
* If the shipment model has duration and distance matrices, `start_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint start_waypoint = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Waypoint,
com.google.maps.routeoptimization.v1.Waypoint.Builder,
com.google.maps.routeoptimization.v1.WaypointOrBuilder>
getStartWaypointFieldBuilder() {
if (startWaypointBuilder_ == null) {
startWaypointBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Waypoint,
com.google.maps.routeoptimization.v1.Waypoint.Builder,
com.google.maps.routeoptimization.v1.WaypointOrBuilder>(
getStartWaypoint(), getParentForChildren(), isClean());
startWaypoint_ = null;
}
return startWaypointBuilder_;
}
private com.google.type.LatLng endLocation_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.type.LatLng, com.google.type.LatLng.Builder, com.google.type.LatLngOrBuilder>
endLocationBuilder_;
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*
* @return Whether the endLocation field is set.
*/
public boolean hasEndLocation() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*
* @return The endLocation.
*/
public com.google.type.LatLng getEndLocation() {
if (endLocationBuilder_ == null) {
return endLocation_ == null ? com.google.type.LatLng.getDefaultInstance() : endLocation_;
} else {
return endLocationBuilder_.getMessage();
}
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
public Builder setEndLocation(com.google.type.LatLng value) {
if (endLocationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endLocation_ = value;
} else {
endLocationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
public Builder setEndLocation(com.google.type.LatLng.Builder builderForValue) {
if (endLocationBuilder_ == null) {
endLocation_ = builderForValue.build();
} else {
endLocationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
public Builder mergeEndLocation(com.google.type.LatLng value) {
if (endLocationBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& endLocation_ != null
&& endLocation_ != com.google.type.LatLng.getDefaultInstance()) {
getEndLocationBuilder().mergeFrom(value);
} else {
endLocation_ = value;
}
} else {
endLocationBuilder_.mergeFrom(value);
}
if (endLocation_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
public Builder clearEndLocation() {
bitField0_ = (bitField0_ & ~0x00000020);
endLocation_ = null;
if (endLocationBuilder_ != null) {
endLocationBuilder_.dispose();
endLocationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
public com.google.type.LatLng.Builder getEndLocationBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getEndLocationFieldBuilder().getBuilder();
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
public com.google.type.LatLngOrBuilder getEndLocationOrBuilder() {
if (endLocationBuilder_ != null) {
return endLocationBuilder_.getMessageOrBuilder();
} else {
return endLocation_ == null ? com.google.type.LatLng.getDefaultInstance() : endLocation_;
}
}
/**
*
*
*
* Geographic location where the vehicle ends after it has completed its last
* `VisitRequest`. If not specified the vehicle's `ShipmentRoute` ends
* immediately when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_location`
* must not be specified.
*
*
* .google.type.LatLng end_location = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.type.LatLng, com.google.type.LatLng.Builder, com.google.type.LatLngOrBuilder>
getEndLocationFieldBuilder() {
if (endLocationBuilder_ == null) {
endLocationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.type.LatLng,
com.google.type.LatLng.Builder,
com.google.type.LatLngOrBuilder>(
getEndLocation(), getParentForChildren(), isClean());
endLocation_ = null;
}
return endLocationBuilder_;
}
private com.google.maps.routeoptimization.v1.Waypoint endWaypoint_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Waypoint,
com.google.maps.routeoptimization.v1.Waypoint.Builder,
com.google.maps.routeoptimization.v1.WaypointOrBuilder>
endWaypointBuilder_;
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*
* @return Whether the endWaypoint field is set.
*/
public boolean hasEndWaypoint() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*
* @return The endWaypoint.
*/
public com.google.maps.routeoptimization.v1.Waypoint getEndWaypoint() {
if (endWaypointBuilder_ == null) {
return endWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: endWaypoint_;
} else {
return endWaypointBuilder_.getMessage();
}
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
public Builder setEndWaypoint(com.google.maps.routeoptimization.v1.Waypoint value) {
if (endWaypointBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endWaypoint_ = value;
} else {
endWaypointBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
public Builder setEndWaypoint(
com.google.maps.routeoptimization.v1.Waypoint.Builder builderForValue) {
if (endWaypointBuilder_ == null) {
endWaypoint_ = builderForValue.build();
} else {
endWaypointBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
public Builder mergeEndWaypoint(com.google.maps.routeoptimization.v1.Waypoint value) {
if (endWaypointBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)
&& endWaypoint_ != null
&& endWaypoint_ != com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()) {
getEndWaypointBuilder().mergeFrom(value);
} else {
endWaypoint_ = value;
}
} else {
endWaypointBuilder_.mergeFrom(value);
}
if (endWaypoint_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
public Builder clearEndWaypoint() {
bitField0_ = (bitField0_ & ~0x00000040);
endWaypoint_ = null;
if (endWaypointBuilder_ != null) {
endWaypointBuilder_.dispose();
endWaypointBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
public com.google.maps.routeoptimization.v1.Waypoint.Builder getEndWaypointBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getEndWaypointFieldBuilder().getBuilder();
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
public com.google.maps.routeoptimization.v1.WaypointOrBuilder getEndWaypointOrBuilder() {
if (endWaypointBuilder_ != null) {
return endWaypointBuilder_.getMessageOrBuilder();
} else {
return endWaypoint_ == null
? com.google.maps.routeoptimization.v1.Waypoint.getDefaultInstance()
: endWaypoint_;
}
}
/**
*
*
*
* Waypoint representing a geographic location where the vehicle ends after
* it has completed its last `VisitRequest`. If neither `end_waypoint` nor
* `end_location` is specified, the vehicle's `ShipmentRoute` ends immediately
* when it completes its last `VisitRequest`.
* If the shipment model has duration and distance matrices, `end_waypoint`
* must not be specified.
*
*
* .google.maps.routeoptimization.v1.Waypoint end_waypoint = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Waypoint,
com.google.maps.routeoptimization.v1.Waypoint.Builder,
com.google.maps.routeoptimization.v1.WaypointOrBuilder>
getEndWaypointFieldBuilder() {
if (endWaypointBuilder_ == null) {
endWaypointBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Waypoint,
com.google.maps.routeoptimization.v1.Waypoint.Builder,
com.google.maps.routeoptimization.v1.WaypointOrBuilder>(
getEndWaypoint(), getParentForChildren(), isClean());
endWaypoint_ = null;
}
return endWaypointBuilder_;
}
private com.google.protobuf.LazyStringArrayList startTags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureStartTagsIsMutable() {
if (!startTags_.isModifiable()) {
startTags_ = new com.google.protobuf.LazyStringArrayList(startTags_);
}
bitField0_ |= 0x00000080;
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @return A list containing the startTags.
*/
public com.google.protobuf.ProtocolStringList getStartTagsList() {
startTags_.makeImmutable();
return startTags_;
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @return The count of startTags.
*/
public int getStartTagsCount() {
return startTags_.size();
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param index The index of the element to return.
* @return The startTags at the given index.
*/
public java.lang.String getStartTags(int index) {
return startTags_.get(index);
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param index The index of the value to return.
* @return The bytes of the startTags at the given index.
*/
public com.google.protobuf.ByteString getStartTagsBytes(int index) {
return startTags_.getByteString(index);
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param index The index to set the value at.
* @param value The startTags to set.
* @return This builder for chaining.
*/
public Builder setStartTags(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStartTagsIsMutable();
startTags_.set(index, value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param value The startTags to add.
* @return This builder for chaining.
*/
public Builder addStartTags(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStartTagsIsMutable();
startTags_.add(value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param values The startTags to add.
* @return This builder for chaining.
*/
public Builder addAllStartTags(java.lang.Iterable values) {
ensureStartTagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, startTags_);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @return This builder for chaining.
*/
public Builder clearStartTags() {
startTags_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the start of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string start_tags = 7;
*
* @param value The bytes of the startTags to add.
* @return This builder for chaining.
*/
public Builder addStartTagsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureStartTagsIsMutable();
startTags_.add(value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList endTags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureEndTagsIsMutable() {
if (!endTags_.isModifiable()) {
endTags_ = new com.google.protobuf.LazyStringArrayList(endTags_);
}
bitField0_ |= 0x00000100;
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @return A list containing the endTags.
*/
public com.google.protobuf.ProtocolStringList getEndTagsList() {
endTags_.makeImmutable();
return endTags_;
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @return The count of endTags.
*/
public int getEndTagsCount() {
return endTags_.size();
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param index The index of the element to return.
* @return The endTags at the given index.
*/
public java.lang.String getEndTags(int index) {
return endTags_.get(index);
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param index The index of the value to return.
* @return The bytes of the endTags at the given index.
*/
public com.google.protobuf.ByteString getEndTagsBytes(int index) {
return endTags_.getByteString(index);
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param index The index to set the value at.
* @param value The endTags to set.
* @return This builder for chaining.
*/
public Builder setEndTags(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureEndTagsIsMutable();
endTags_.set(index, value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param value The endTags to add.
* @return This builder for chaining.
*/
public Builder addEndTags(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureEndTagsIsMutable();
endTags_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param values The endTags to add.
* @return This builder for chaining.
*/
public Builder addAllEndTags(java.lang.Iterable values) {
ensureEndTagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, endTags_);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @return This builder for chaining.
*/
public Builder clearEndTags() {
endTags_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
;
onChanged();
return this;
}
/**
*
*
*
* Specifies tags attached to the end of the vehicle's route.
*
* Empty or duplicate strings are not allowed.
*
*
* repeated string end_tags = 8;
*
* @param value The bytes of the endTags to add.
* @return This builder for chaining.
*/
public Builder addEndTagsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureEndTagsIsMutable();
endTags_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private java.util.List startTimeWindows_ =
java.util.Collections.emptyList();
private void ensureStartTimeWindowsIsMutable() {
if (!((bitField0_ & 0x00000200) != 0)) {
startTimeWindows_ =
new java.util.ArrayList(
startTimeWindows_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.TimeWindow,
com.google.maps.routeoptimization.v1.TimeWindow.Builder,
com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
startTimeWindowsBuilder_;
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public java.util.List
getStartTimeWindowsList() {
if (startTimeWindowsBuilder_ == null) {
return java.util.Collections.unmodifiableList(startTimeWindows_);
} else {
return startTimeWindowsBuilder_.getMessageList();
}
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public int getStartTimeWindowsCount() {
if (startTimeWindowsBuilder_ == null) {
return startTimeWindows_.size();
} else {
return startTimeWindowsBuilder_.getCount();
}
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public com.google.maps.routeoptimization.v1.TimeWindow getStartTimeWindows(int index) {
if (startTimeWindowsBuilder_ == null) {
return startTimeWindows_.get(index);
} else {
return startTimeWindowsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder setStartTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow value) {
if (startTimeWindowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStartTimeWindowsIsMutable();
startTimeWindows_.set(index, value);
onChanged();
} else {
startTimeWindowsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder setStartTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow.Builder builderForValue) {
if (startTimeWindowsBuilder_ == null) {
ensureStartTimeWindowsIsMutable();
startTimeWindows_.set(index, builderForValue.build());
onChanged();
} else {
startTimeWindowsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder addStartTimeWindows(com.google.maps.routeoptimization.v1.TimeWindow value) {
if (startTimeWindowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStartTimeWindowsIsMutable();
startTimeWindows_.add(value);
onChanged();
} else {
startTimeWindowsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder addStartTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow value) {
if (startTimeWindowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStartTimeWindowsIsMutable();
startTimeWindows_.add(index, value);
onChanged();
} else {
startTimeWindowsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder addStartTimeWindows(
com.google.maps.routeoptimization.v1.TimeWindow.Builder builderForValue) {
if (startTimeWindowsBuilder_ == null) {
ensureStartTimeWindowsIsMutable();
startTimeWindows_.add(builderForValue.build());
onChanged();
} else {
startTimeWindowsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder addStartTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow.Builder builderForValue) {
if (startTimeWindowsBuilder_ == null) {
ensureStartTimeWindowsIsMutable();
startTimeWindows_.add(index, builderForValue.build());
onChanged();
} else {
startTimeWindowsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder addAllStartTimeWindows(
java.lang.Iterable extends com.google.maps.routeoptimization.v1.TimeWindow> values) {
if (startTimeWindowsBuilder_ == null) {
ensureStartTimeWindowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, startTimeWindows_);
onChanged();
} else {
startTimeWindowsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder clearStartTimeWindows() {
if (startTimeWindowsBuilder_ == null) {
startTimeWindows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
startTimeWindowsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public Builder removeStartTimeWindows(int index) {
if (startTimeWindowsBuilder_ == null) {
ensureStartTimeWindowsIsMutable();
startTimeWindows_.remove(index);
onChanged();
} else {
startTimeWindowsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public com.google.maps.routeoptimization.v1.TimeWindow.Builder getStartTimeWindowsBuilder(
int index) {
return getStartTimeWindowsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public com.google.maps.routeoptimization.v1.TimeWindowOrBuilder getStartTimeWindowsOrBuilder(
int index) {
if (startTimeWindowsBuilder_ == null) {
return startTimeWindows_.get(index);
} else {
return startTimeWindowsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public java.util.List extends com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
getStartTimeWindowsOrBuilderList() {
if (startTimeWindowsBuilder_ != null) {
return startTimeWindowsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(startTimeWindows_);
}
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public com.google.maps.routeoptimization.v1.TimeWindow.Builder addStartTimeWindowsBuilder() {
return getStartTimeWindowsFieldBuilder()
.addBuilder(com.google.maps.routeoptimization.v1.TimeWindow.getDefaultInstance());
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public com.google.maps.routeoptimization.v1.TimeWindow.Builder addStartTimeWindowsBuilder(
int index) {
return getStartTimeWindowsFieldBuilder()
.addBuilder(index, com.google.maps.routeoptimization.v1.TimeWindow.getDefaultInstance());
}
/**
*
*
*
* Time windows during which the vehicle may depart its start location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow start_time_windows = 9;
*/
public java.util.List
getStartTimeWindowsBuilderList() {
return getStartTimeWindowsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.TimeWindow,
com.google.maps.routeoptimization.v1.TimeWindow.Builder,
com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
getStartTimeWindowsFieldBuilder() {
if (startTimeWindowsBuilder_ == null) {
startTimeWindowsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.TimeWindow,
com.google.maps.routeoptimization.v1.TimeWindow.Builder,
com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>(
startTimeWindows_,
((bitField0_ & 0x00000200) != 0),
getParentForChildren(),
isClean());
startTimeWindows_ = null;
}
return startTimeWindowsBuilder_;
}
private java.util.List endTimeWindows_ =
java.util.Collections.emptyList();
private void ensureEndTimeWindowsIsMutable() {
if (!((bitField0_ & 0x00000400) != 0)) {
endTimeWindows_ =
new java.util.ArrayList(
endTimeWindows_);
bitField0_ |= 0x00000400;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.TimeWindow,
com.google.maps.routeoptimization.v1.TimeWindow.Builder,
com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
endTimeWindowsBuilder_;
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public java.util.List getEndTimeWindowsList() {
if (endTimeWindowsBuilder_ == null) {
return java.util.Collections.unmodifiableList(endTimeWindows_);
} else {
return endTimeWindowsBuilder_.getMessageList();
}
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public int getEndTimeWindowsCount() {
if (endTimeWindowsBuilder_ == null) {
return endTimeWindows_.size();
} else {
return endTimeWindowsBuilder_.getCount();
}
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public com.google.maps.routeoptimization.v1.TimeWindow getEndTimeWindows(int index) {
if (endTimeWindowsBuilder_ == null) {
return endTimeWindows_.get(index);
} else {
return endTimeWindowsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder setEndTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow value) {
if (endTimeWindowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEndTimeWindowsIsMutable();
endTimeWindows_.set(index, value);
onChanged();
} else {
endTimeWindowsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder setEndTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow.Builder builderForValue) {
if (endTimeWindowsBuilder_ == null) {
ensureEndTimeWindowsIsMutable();
endTimeWindows_.set(index, builderForValue.build());
onChanged();
} else {
endTimeWindowsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder addEndTimeWindows(com.google.maps.routeoptimization.v1.TimeWindow value) {
if (endTimeWindowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEndTimeWindowsIsMutable();
endTimeWindows_.add(value);
onChanged();
} else {
endTimeWindowsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder addEndTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow value) {
if (endTimeWindowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEndTimeWindowsIsMutable();
endTimeWindows_.add(index, value);
onChanged();
} else {
endTimeWindowsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder addEndTimeWindows(
com.google.maps.routeoptimization.v1.TimeWindow.Builder builderForValue) {
if (endTimeWindowsBuilder_ == null) {
ensureEndTimeWindowsIsMutable();
endTimeWindows_.add(builderForValue.build());
onChanged();
} else {
endTimeWindowsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder addEndTimeWindows(
int index, com.google.maps.routeoptimization.v1.TimeWindow.Builder builderForValue) {
if (endTimeWindowsBuilder_ == null) {
ensureEndTimeWindowsIsMutable();
endTimeWindows_.add(index, builderForValue.build());
onChanged();
} else {
endTimeWindowsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder addAllEndTimeWindows(
java.lang.Iterable extends com.google.maps.routeoptimization.v1.TimeWindow> values) {
if (endTimeWindowsBuilder_ == null) {
ensureEndTimeWindowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, endTimeWindows_);
onChanged();
} else {
endTimeWindowsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder clearEndTimeWindows() {
if (endTimeWindowsBuilder_ == null) {
endTimeWindows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
} else {
endTimeWindowsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public Builder removeEndTimeWindows(int index) {
if (endTimeWindowsBuilder_ == null) {
ensureEndTimeWindowsIsMutable();
endTimeWindows_.remove(index);
onChanged();
} else {
endTimeWindowsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public com.google.maps.routeoptimization.v1.TimeWindow.Builder getEndTimeWindowsBuilder(
int index) {
return getEndTimeWindowsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public com.google.maps.routeoptimization.v1.TimeWindowOrBuilder getEndTimeWindowsOrBuilder(
int index) {
if (endTimeWindowsBuilder_ == null) {
return endTimeWindows_.get(index);
} else {
return endTimeWindowsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public java.util.List extends com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
getEndTimeWindowsOrBuilderList() {
if (endTimeWindowsBuilder_ != null) {
return endTimeWindowsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(endTimeWindows_);
}
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public com.google.maps.routeoptimization.v1.TimeWindow.Builder addEndTimeWindowsBuilder() {
return getEndTimeWindowsFieldBuilder()
.addBuilder(com.google.maps.routeoptimization.v1.TimeWindow.getDefaultInstance());
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public com.google.maps.routeoptimization.v1.TimeWindow.Builder addEndTimeWindowsBuilder(
int index) {
return getEndTimeWindowsFieldBuilder()
.addBuilder(index, com.google.maps.routeoptimization.v1.TimeWindow.getDefaultInstance());
}
/**
*
*
*
* Time windows during which the vehicle may arrive at its end location.
* They must be within the global time limits (see
* [ShipmentModel.global_*][google.maps.routeoptimization.v1.ShipmentModel.global_start_time]
* fields). If unspecified, there is no limitation besides those global time
* limits.
*
* Time windows belonging to the same repeated field must be disjoint, i.e. no
* time window can overlap with or be adjacent to another, and they must be in
* chronological order.
*
* `cost_per_hour_after_soft_end_time` and `soft_end_time` can only be set if
* there is a single time window.
*
*
* repeated .google.maps.routeoptimization.v1.TimeWindow end_time_windows = 10;
*/
public java.util.List
getEndTimeWindowsBuilderList() {
return getEndTimeWindowsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.TimeWindow,
com.google.maps.routeoptimization.v1.TimeWindow.Builder,
com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>
getEndTimeWindowsFieldBuilder() {
if (endTimeWindowsBuilder_ == null) {
endTimeWindowsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.maps.routeoptimization.v1.TimeWindow,
com.google.maps.routeoptimization.v1.TimeWindow.Builder,
com.google.maps.routeoptimization.v1.TimeWindowOrBuilder>(
endTimeWindows_,
((bitField0_ & 0x00000400) != 0),
getParentForChildren(),
isClean());
endTimeWindows_ = null;
}
return endTimeWindowsBuilder_;
}
private double travelDurationMultiple_;
/**
*
*
*
* Specifies a multiplicative factor that can be used to increase or decrease
* travel times of this vehicle. For example, setting this to 2.0 means
* that this vehicle is slower and has travel times that are twice what they
* are for standard vehicles. This multiple does not affect visit durations.
* It does affect cost if `cost_per_hour` or `cost_per_traveled_hour` are
* specified. This must be in the range [0.001, 1000.0]. If unset, the vehicle
* is standard, and this multiple is considered 1.0.
*
* WARNING: Travel times will be rounded to the nearest second after this
* multiple is applied but before performing any numerical operations, thus,
* a small multiple may result in a loss of precision.
*
* See also `extra_visit_duration_for_visit_type` below.
*
*
* optional double travel_duration_multiple = 11;
*
* @return Whether the travelDurationMultiple field is set.
*/
@java.lang.Override
public boolean hasTravelDurationMultiple() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Specifies a multiplicative factor that can be used to increase or decrease
* travel times of this vehicle. For example, setting this to 2.0 means
* that this vehicle is slower and has travel times that are twice what they
* are for standard vehicles. This multiple does not affect visit durations.
* It does affect cost if `cost_per_hour` or `cost_per_traveled_hour` are
* specified. This must be in the range [0.001, 1000.0]. If unset, the vehicle
* is standard, and this multiple is considered 1.0.
*
* WARNING: Travel times will be rounded to the nearest second after this
* multiple is applied but before performing any numerical operations, thus,
* a small multiple may result in a loss of precision.
*
* See also `extra_visit_duration_for_visit_type` below.
*
*
* optional double travel_duration_multiple = 11;
*
* @return The travelDurationMultiple.
*/
@java.lang.Override
public double getTravelDurationMultiple() {
return travelDurationMultiple_;
}
/**
*
*
*
* Specifies a multiplicative factor that can be used to increase or decrease
* travel times of this vehicle. For example, setting this to 2.0 means
* that this vehicle is slower and has travel times that are twice what they
* are for standard vehicles. This multiple does not affect visit durations.
* It does affect cost if `cost_per_hour` or `cost_per_traveled_hour` are
* specified. This must be in the range [0.001, 1000.0]. If unset, the vehicle
* is standard, and this multiple is considered 1.0.
*
* WARNING: Travel times will be rounded to the nearest second after this
* multiple is applied but before performing any numerical operations, thus,
* a small multiple may result in a loss of precision.
*
* See also `extra_visit_duration_for_visit_type` below.
*
*
* optional double travel_duration_multiple = 11;
*
* @param value The travelDurationMultiple to set.
* @return This builder for chaining.
*/
public Builder setTravelDurationMultiple(double value) {
travelDurationMultiple_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* Specifies a multiplicative factor that can be used to increase or decrease
* travel times of this vehicle. For example, setting this to 2.0 means
* that this vehicle is slower and has travel times that are twice what they
* are for standard vehicles. This multiple does not affect visit durations.
* It does affect cost if `cost_per_hour` or `cost_per_traveled_hour` are
* specified. This must be in the range [0.001, 1000.0]. If unset, the vehicle
* is standard, and this multiple is considered 1.0.
*
* WARNING: Travel times will be rounded to the nearest second after this
* multiple is applied but before performing any numerical operations, thus,
* a small multiple may result in a loss of precision.
*
* See also `extra_visit_duration_for_visit_type` below.
*
*
* optional double travel_duration_multiple = 11;
*
* @return This builder for chaining.
*/
public Builder clearTravelDurationMultiple() {
bitField0_ = (bitField0_ & ~0x00000800);
travelDurationMultiple_ = 0D;
onChanged();
return this;
}
private int unloadingPolicy_ = 0;
/**
*
*
*
* Unloading policy enforced on the vehicle.
*
*
* .google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy unloading_policy = 12;
*
* @return The enum numeric value on the wire for unloadingPolicy.
*/
@java.lang.Override
public int getUnloadingPolicyValue() {
return unloadingPolicy_;
}
/**
*
*
*
* Unloading policy enforced on the vehicle.
*
*
* .google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy unloading_policy = 12;
*
* @param value The enum numeric value on the wire for unloadingPolicy to set.
* @return This builder for chaining.
*/
public Builder setUnloadingPolicyValue(int value) {
unloadingPolicy_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Unloading policy enforced on the vehicle.
*
*
* .google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy unloading_policy = 12;
*
* @return The unloadingPolicy.
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy getUnloadingPolicy() {
com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy result =
com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy.forNumber(unloadingPolicy_);
return result == null
? com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy.UNRECOGNIZED
: result;
}
/**
*
*
*
* Unloading policy enforced on the vehicle.
*
*
* .google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy unloading_policy = 12;
*
* @param value The unloadingPolicy to set.
* @return This builder for chaining.
*/
public Builder setUnloadingPolicy(
com.google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
unloadingPolicy_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Unloading policy enforced on the vehicle.
*
*
* .google.maps.routeoptimization.v1.Vehicle.UnloadingPolicy unloading_policy = 12;
*
* @return This builder for chaining.
*/
public Builder clearUnloadingPolicy() {
bitField0_ = (bitField0_ & ~0x00001000);
unloadingPolicy_ = 0;
onChanged();
return this;
}
private static final class LoadLimitsConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit> {
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit build(
com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder val) {
if (val instanceof com.google.maps.routeoptimization.v1.Vehicle.LoadLimit) {
return (com.google.maps.routeoptimization.v1.Vehicle.LoadLimit) val;
}
return ((com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
defaultEntry() {
return LoadLimitsDefaultEntryHolder.defaultEntry;
}
};
private static final LoadLimitsConverter loadLimitsConverter = new LoadLimitsConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder>
loadLimits_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder>
internalGetLoadLimits() {
if (loadLimits_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(loadLimitsConverter);
}
return loadLimits_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit,
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder>
internalGetMutableLoadLimits() {
if (loadLimits_ == null) {
loadLimits_ = new com.google.protobuf.MapFieldBuilder<>(loadLimitsConverter);
}
bitField0_ |= 0x00002000;
onChanged();
return loadLimits_;
}
public int getLoadLimitsCount() {
return internalGetLoadLimits().ensureBuilderMap().size();
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public boolean containsLoadLimits(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLoadLimits().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getLoadLimitsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getLoadLimits() {
return getLoadLimitsMap();
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public java.util.Map
getLoadLimitsMap() {
return internalGetLoadLimits().getImmutableMap();
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public /* nullable */ com.google.maps.routeoptimization.v1.Vehicle.LoadLimit
getLoadLimitsOrDefault(
java.lang.String key,
/* nullable */
com.google.maps.routeoptimization.v1.Vehicle.LoadLimit defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder>
map = internalGetMutableLoadLimits().ensureBuilderMap();
return map.containsKey(key) ? loadLimitsConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit getLoadLimitsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder>
map = internalGetMutableLoadLimits().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return loadLimitsConverter.build(map.get(key));
}
public Builder clearLoadLimits() {
bitField0_ = (bitField0_ & ~0x00002000);
internalGetMutableLoadLimits().clear();
return this;
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
public Builder removeLoadLimits(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableLoadLimits().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map
getMutableLoadLimits() {
bitField0_ |= 0x00002000;
return internalGetMutableLoadLimits().ensureMessageMap();
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
public Builder putLoadLimits(
java.lang.String key, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableLoadLimits().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00002000;
return this;
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
public Builder putAllLoadLimits(
java.util.Map
values) {
for (java.util.Map.Entry<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimit>
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableLoadLimits().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00002000;
return this;
}
/**
*
*
*
* Capacities of the vehicle (weight, volume, # of pallets for example).
* The keys in the map are the identifiers of the type of load, consistent
* with the keys of the
* [Shipment.load_demands][google.maps.routeoptimization.v1.Shipment.load_demands]
* field. If a given key is absent from this map, the corresponding capacity
* is considered to be limitless.
*
*
*
* map<string, .google.maps.routeoptimization.v1.Vehicle.LoadLimit> load_limits = 30;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder
putLoadLimitsBuilderIfAbsent(java.lang.String key) {
java.util.Map<
java.lang.String, com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder>
builderMap = internalGetMutableLoadLimits().ensureBuilderMap();
com.google.maps.routeoptimization.v1.Vehicle.LoadLimitOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.maps.routeoptimization.v1.Vehicle.LoadLimit) {
entry = ((com.google.maps.routeoptimization.v1.Vehicle.LoadLimit) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.maps.routeoptimization.v1.Vehicle.LoadLimit.Builder) entry;
}
private double costPerHour_;
/**
*
*
*
* Vehicle costs: all costs add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
* Cost per hour of the vehicle route. This cost is applied to the total time
* taken by the route, and includes travel time, waiting time, and visit time.
* Using `cost_per_hour` instead of just `cost_per_traveled_hour` may result
* in additional latency.
*
*
* double cost_per_hour = 16;
*
* @return The costPerHour.
*/
@java.lang.Override
public double getCostPerHour() {
return costPerHour_;
}
/**
*
*
*
* Vehicle costs: all costs add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
* Cost per hour of the vehicle route. This cost is applied to the total time
* taken by the route, and includes travel time, waiting time, and visit time.
* Using `cost_per_hour` instead of just `cost_per_traveled_hour` may result
* in additional latency.
*
*
* double cost_per_hour = 16;
*
* @param value The costPerHour to set.
* @return This builder for chaining.
*/
public Builder setCostPerHour(double value) {
costPerHour_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Vehicle costs: all costs add up and must be in the same unit as
* [Shipment.penalty_cost][google.maps.routeoptimization.v1.Shipment.penalty_cost].
*
* Cost per hour of the vehicle route. This cost is applied to the total time
* taken by the route, and includes travel time, waiting time, and visit time.
* Using `cost_per_hour` instead of just `cost_per_traveled_hour` may result
* in additional latency.
*
*
* double cost_per_hour = 16;
*
* @return This builder for chaining.
*/
public Builder clearCostPerHour() {
bitField0_ = (bitField0_ & ~0x00004000);
costPerHour_ = 0D;
onChanged();
return this;
}
private double costPerTraveledHour_;
/**
*
*
*
* Cost per traveled hour of the vehicle route. This cost is applied only to
* travel time taken by the route (i.e., that reported in
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]),
* and excludes waiting time and visit time.
*
*
* double cost_per_traveled_hour = 17;
*
* @return The costPerTraveledHour.
*/
@java.lang.Override
public double getCostPerTraveledHour() {
return costPerTraveledHour_;
}
/**
*
*
*
* Cost per traveled hour of the vehicle route. This cost is applied only to
* travel time taken by the route (i.e., that reported in
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]),
* and excludes waiting time and visit time.
*
*
* double cost_per_traveled_hour = 17;
*
* @param value The costPerTraveledHour to set.
* @return This builder for chaining.
*/
public Builder setCostPerTraveledHour(double value) {
costPerTraveledHour_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Cost per traveled hour of the vehicle route. This cost is applied only to
* travel time taken by the route (i.e., that reported in
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]),
* and excludes waiting time and visit time.
*
*
* double cost_per_traveled_hour = 17;
*
* @return This builder for chaining.
*/
public Builder clearCostPerTraveledHour() {
bitField0_ = (bitField0_ & ~0x00008000);
costPerTraveledHour_ = 0D;
onChanged();
return this;
}
private double costPerKilometer_;
/**
*
*
*
* Cost per kilometer of the vehicle route. This cost is applied to the
* distance reported in the
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* and does not apply to any distance implicitly traveled from the
* `arrival_location` to the `departure_location` of a single `VisitRequest`.
*
*
* double cost_per_kilometer = 18;
*
* @return The costPerKilometer.
*/
@java.lang.Override
public double getCostPerKilometer() {
return costPerKilometer_;
}
/**
*
*
*
* Cost per kilometer of the vehicle route. This cost is applied to the
* distance reported in the
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* and does not apply to any distance implicitly traveled from the
* `arrival_location` to the `departure_location` of a single `VisitRequest`.
*
*
* double cost_per_kilometer = 18;
*
* @param value The costPerKilometer to set.
* @return This builder for chaining.
*/
public Builder setCostPerKilometer(double value) {
costPerKilometer_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* Cost per kilometer of the vehicle route. This cost is applied to the
* distance reported in the
* [ShipmentRoute.transitions][google.maps.routeoptimization.v1.ShipmentRoute.transitions]
* and does not apply to any distance implicitly traveled from the
* `arrival_location` to the `departure_location` of a single `VisitRequest`.
*
*
* double cost_per_kilometer = 18;
*
* @return This builder for chaining.
*/
public Builder clearCostPerKilometer() {
bitField0_ = (bitField0_ & ~0x00010000);
costPerKilometer_ = 0D;
onChanged();
return this;
}
private double fixedCost_;
/**
*
*
*
* Fixed cost applied if this vehicle is used to handle a shipment.
*
*
* double fixed_cost = 19;
*
* @return The fixedCost.
*/
@java.lang.Override
public double getFixedCost() {
return fixedCost_;
}
/**
*
*
*
* Fixed cost applied if this vehicle is used to handle a shipment.
*
*
* double fixed_cost = 19;
*
* @param value The fixedCost to set.
* @return This builder for chaining.
*/
public Builder setFixedCost(double value) {
fixedCost_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* Fixed cost applied if this vehicle is used to handle a shipment.
*
*
* double fixed_cost = 19;
*
* @return This builder for chaining.
*/
public Builder clearFixedCost() {
bitField0_ = (bitField0_ & ~0x00020000);
fixedCost_ = 0D;
onChanged();
return this;
}
private boolean usedIfRouteIsEmpty_;
/**
*
*
*
* This field only applies to vehicles when their route does not serve any
* shipments. It indicates if the vehicle should be considered as used or not
* in this case.
*
* If true, the vehicle goes from its start to its end location even if it
* doesn't serve any shipments, and time and distance costs resulting from its
* start --> end travel are taken into account.
*
* Otherwise, it doesn't travel from its start to its end location, and no
* `break_rule` or delay (from `TransitionAttributes`) are scheduled for this
* vehicle. In this case, the vehicle's `ShipmentRoute` doesn't contain any
* information except for the vehicle index and label.
*
*
* bool used_if_route_is_empty = 20;
*
* @return The usedIfRouteIsEmpty.
*/
@java.lang.Override
public boolean getUsedIfRouteIsEmpty() {
return usedIfRouteIsEmpty_;
}
/**
*
*
*
* This field only applies to vehicles when their route does not serve any
* shipments. It indicates if the vehicle should be considered as used or not
* in this case.
*
* If true, the vehicle goes from its start to its end location even if it
* doesn't serve any shipments, and time and distance costs resulting from its
* start --> end travel are taken into account.
*
* Otherwise, it doesn't travel from its start to its end location, and no
* `break_rule` or delay (from `TransitionAttributes`) are scheduled for this
* vehicle. In this case, the vehicle's `ShipmentRoute` doesn't contain any
* information except for the vehicle index and label.
*
*
* bool used_if_route_is_empty = 20;
*
* @param value The usedIfRouteIsEmpty to set.
* @return This builder for chaining.
*/
public Builder setUsedIfRouteIsEmpty(boolean value) {
usedIfRouteIsEmpty_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* This field only applies to vehicles when their route does not serve any
* shipments. It indicates if the vehicle should be considered as used or not
* in this case.
*
* If true, the vehicle goes from its start to its end location even if it
* doesn't serve any shipments, and time and distance costs resulting from its
* start --> end travel are taken into account.
*
* Otherwise, it doesn't travel from its start to its end location, and no
* `break_rule` or delay (from `TransitionAttributes`) are scheduled for this
* vehicle. In this case, the vehicle's `ShipmentRoute` doesn't contain any
* information except for the vehicle index and label.
*
*
* bool used_if_route_is_empty = 20;
*
* @return This builder for chaining.
*/
public Builder clearUsedIfRouteIsEmpty() {
bitField0_ = (bitField0_ & ~0x00040000);
usedIfRouteIsEmpty_ = false;
onChanged();
return this;
}
private com.google.maps.routeoptimization.v1.Vehicle.DurationLimit routeDurationLimit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder>
routeDurationLimitBuilder_;
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*
* @return Whether the routeDurationLimit field is set.
*/
public boolean hasRouteDurationLimit() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*
* @return The routeDurationLimit.
*/
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit getRouteDurationLimit() {
if (routeDurationLimitBuilder_ == null) {
return routeDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: routeDurationLimit_;
} else {
return routeDurationLimitBuilder_.getMessage();
}
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*/
public Builder setRouteDurationLimit(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit value) {
if (routeDurationLimitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
routeDurationLimit_ = value;
} else {
routeDurationLimitBuilder_.setMessage(value);
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*/
public Builder setRouteDurationLimit(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder builderForValue) {
if (routeDurationLimitBuilder_ == null) {
routeDurationLimit_ = builderForValue.build();
} else {
routeDurationLimitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*/
public Builder mergeRouteDurationLimit(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit value) {
if (routeDurationLimitBuilder_ == null) {
if (((bitField0_ & 0x00080000) != 0)
&& routeDurationLimit_ != null
&& routeDurationLimit_
!= com.google.maps.routeoptimization.v1.Vehicle.DurationLimit
.getDefaultInstance()) {
getRouteDurationLimitBuilder().mergeFrom(value);
} else {
routeDurationLimit_ = value;
}
} else {
routeDurationLimitBuilder_.mergeFrom(value);
}
if (routeDurationLimit_ != null) {
bitField0_ |= 0x00080000;
onChanged();
}
return this;
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*/
public Builder clearRouteDurationLimit() {
bitField0_ = (bitField0_ & ~0x00080000);
routeDurationLimit_ = null;
if (routeDurationLimitBuilder_ != null) {
routeDurationLimitBuilder_.dispose();
routeDurationLimitBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder
getRouteDurationLimitBuilder() {
bitField0_ |= 0x00080000;
onChanged();
return getRouteDurationLimitFieldBuilder().getBuilder();
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder
getRouteDurationLimitOrBuilder() {
if (routeDurationLimitBuilder_ != null) {
return routeDurationLimitBuilder_.getMessageOrBuilder();
} else {
return routeDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: routeDurationLimit_;
}
}
/**
*
*
*
* Limit applied to the total duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route duration of a vehicle is the
* difference between its `vehicle_end_time` and `vehicle_start_time`.
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit route_duration_limit = 21;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder>
getRouteDurationLimitFieldBuilder() {
if (routeDurationLimitBuilder_ == null) {
routeDurationLimitBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder>(
getRouteDurationLimit(), getParentForChildren(), isClean());
routeDurationLimit_ = null;
}
return routeDurationLimitBuilder_;
}
private com.google.maps.routeoptimization.v1.Vehicle.DurationLimit travelDurationLimit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder>
travelDurationLimitBuilder_;
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*
* @return Whether the travelDurationLimit field is set.
*/
public boolean hasTravelDurationLimit() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*
* @return The travelDurationLimit.
*/
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit getTravelDurationLimit() {
if (travelDurationLimitBuilder_ == null) {
return travelDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: travelDurationLimit_;
} else {
return travelDurationLimitBuilder_.getMessage();
}
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
public Builder setTravelDurationLimit(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit value) {
if (travelDurationLimitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
travelDurationLimit_ = value;
} else {
travelDurationLimitBuilder_.setMessage(value);
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
public Builder setTravelDurationLimit(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder builderForValue) {
if (travelDurationLimitBuilder_ == null) {
travelDurationLimit_ = builderForValue.build();
} else {
travelDurationLimitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
public Builder mergeTravelDurationLimit(
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit value) {
if (travelDurationLimitBuilder_ == null) {
if (((bitField0_ & 0x00100000) != 0)
&& travelDurationLimit_ != null
&& travelDurationLimit_
!= com.google.maps.routeoptimization.v1.Vehicle.DurationLimit
.getDefaultInstance()) {
getTravelDurationLimitBuilder().mergeFrom(value);
} else {
travelDurationLimit_ = value;
}
} else {
travelDurationLimitBuilder_.mergeFrom(value);
}
if (travelDurationLimit_ != null) {
bitField0_ |= 0x00100000;
onChanged();
}
return this;
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
public Builder clearTravelDurationLimit() {
bitField0_ = (bitField0_ & ~0x00100000);
travelDurationLimit_ = null;
if (travelDurationLimitBuilder_ != null) {
travelDurationLimitBuilder_.dispose();
travelDurationLimitBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder
getTravelDurationLimitBuilder() {
bitField0_ |= 0x00100000;
onChanged();
return getTravelDurationLimitFieldBuilder().getBuilder();
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
public com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder
getTravelDurationLimitOrBuilder() {
if (travelDurationLimitBuilder_ != null) {
return travelDurationLimitBuilder_.getMessageOrBuilder();
} else {
return travelDurationLimit_ == null
? com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.getDefaultInstance()
: travelDurationLimit_;
}
}
/**
*
*
*
* Limit applied to the travel duration of the vehicle's route. In a given
* `OptimizeToursResponse`, the route travel duration is the sum of all its
* [transitions.travel_duration][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_duration].
*
*
* .google.maps.routeoptimization.v1.Vehicle.DurationLimit travel_duration_limit = 22;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder>
getTravelDurationLimitFieldBuilder() {
if (travelDurationLimitBuilder_ == null) {
travelDurationLimitBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimit.Builder,
com.google.maps.routeoptimization.v1.Vehicle.DurationLimitOrBuilder>(
getTravelDurationLimit(), getParentForChildren(), isClean());
travelDurationLimit_ = null;
}
return travelDurationLimitBuilder_;
}
private com.google.maps.routeoptimization.v1.DistanceLimit routeDistanceLimit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.DistanceLimit,
com.google.maps.routeoptimization.v1.DistanceLimit.Builder,
com.google.maps.routeoptimization.v1.DistanceLimitOrBuilder>
routeDistanceLimitBuilder_;
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*
* @return Whether the routeDistanceLimit field is set.
*/
public boolean hasRouteDistanceLimit() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*
* @return The routeDistanceLimit.
*/
public com.google.maps.routeoptimization.v1.DistanceLimit getRouteDistanceLimit() {
if (routeDistanceLimitBuilder_ == null) {
return routeDistanceLimit_ == null
? com.google.maps.routeoptimization.v1.DistanceLimit.getDefaultInstance()
: routeDistanceLimit_;
} else {
return routeDistanceLimitBuilder_.getMessage();
}
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
public Builder setRouteDistanceLimit(com.google.maps.routeoptimization.v1.DistanceLimit value) {
if (routeDistanceLimitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
routeDistanceLimit_ = value;
} else {
routeDistanceLimitBuilder_.setMessage(value);
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
public Builder setRouteDistanceLimit(
com.google.maps.routeoptimization.v1.DistanceLimit.Builder builderForValue) {
if (routeDistanceLimitBuilder_ == null) {
routeDistanceLimit_ = builderForValue.build();
} else {
routeDistanceLimitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
public Builder mergeRouteDistanceLimit(
com.google.maps.routeoptimization.v1.DistanceLimit value) {
if (routeDistanceLimitBuilder_ == null) {
if (((bitField0_ & 0x00200000) != 0)
&& routeDistanceLimit_ != null
&& routeDistanceLimit_
!= com.google.maps.routeoptimization.v1.DistanceLimit.getDefaultInstance()) {
getRouteDistanceLimitBuilder().mergeFrom(value);
} else {
routeDistanceLimit_ = value;
}
} else {
routeDistanceLimitBuilder_.mergeFrom(value);
}
if (routeDistanceLimit_ != null) {
bitField0_ |= 0x00200000;
onChanged();
}
return this;
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
public Builder clearRouteDistanceLimit() {
bitField0_ = (bitField0_ & ~0x00200000);
routeDistanceLimit_ = null;
if (routeDistanceLimitBuilder_ != null) {
routeDistanceLimitBuilder_.dispose();
routeDistanceLimitBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
public com.google.maps.routeoptimization.v1.DistanceLimit.Builder
getRouteDistanceLimitBuilder() {
bitField0_ |= 0x00200000;
onChanged();
return getRouteDistanceLimitFieldBuilder().getBuilder();
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
public com.google.maps.routeoptimization.v1.DistanceLimitOrBuilder
getRouteDistanceLimitOrBuilder() {
if (routeDistanceLimitBuilder_ != null) {
return routeDistanceLimitBuilder_.getMessageOrBuilder();
} else {
return routeDistanceLimit_ == null
? com.google.maps.routeoptimization.v1.DistanceLimit.getDefaultInstance()
: routeDistanceLimit_;
}
}
/**
*
*
*
* Limit applied to the total distance of the vehicle's route. In a given
* `OptimizeToursResponse`, the route distance is the sum of all its
* [transitions.travel_distance_meters][google.maps.routeoptimization.v1.ShipmentRoute.Transition.travel_distance_meters].
*
*
* .google.maps.routeoptimization.v1.DistanceLimit route_distance_limit = 23;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.DistanceLimit,
com.google.maps.routeoptimization.v1.DistanceLimit.Builder,
com.google.maps.routeoptimization.v1.DistanceLimitOrBuilder>
getRouteDistanceLimitFieldBuilder() {
if (routeDistanceLimitBuilder_ == null) {
routeDistanceLimitBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.DistanceLimit,
com.google.maps.routeoptimization.v1.DistanceLimit.Builder,
com.google.maps.routeoptimization.v1.DistanceLimitOrBuilder>(
getRouteDistanceLimit(), getParentForChildren(), isClean());
routeDistanceLimit_ = null;
}
return routeDistanceLimitBuilder_;
}
private static final class ExtraVisitDurationForVisitTypeConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String, com.google.protobuf.DurationOrBuilder, com.google.protobuf.Duration> {
@java.lang.Override
public com.google.protobuf.Duration build(com.google.protobuf.DurationOrBuilder val) {
if (val instanceof com.google.protobuf.Duration) {
return (com.google.protobuf.Duration) val;
}
return ((com.google.protobuf.Duration.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry
defaultEntry() {
return ExtraVisitDurationForVisitTypeDefaultEntryHolder.defaultEntry;
}
};
private static final ExtraVisitDurationForVisitTypeConverter
extraVisitDurationForVisitTypeConverter = new ExtraVisitDurationForVisitTypeConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.protobuf.DurationOrBuilder,
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder>
extraVisitDurationForVisitType_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.protobuf.DurationOrBuilder,
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder>
internalGetExtraVisitDurationForVisitType() {
if (extraVisitDurationForVisitType_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(extraVisitDurationForVisitTypeConverter);
}
return extraVisitDurationForVisitType_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.protobuf.DurationOrBuilder,
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder>
internalGetMutableExtraVisitDurationForVisitType() {
if (extraVisitDurationForVisitType_ == null) {
extraVisitDurationForVisitType_ =
new com.google.protobuf.MapFieldBuilder<>(extraVisitDurationForVisitTypeConverter);
}
bitField0_ |= 0x00400000;
onChanged();
return extraVisitDurationForVisitType_;
}
public int getExtraVisitDurationForVisitTypeCount() {
return internalGetExtraVisitDurationForVisitType().ensureBuilderMap().size();
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public boolean containsExtraVisitDurationForVisitType(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetExtraVisitDurationForVisitType().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getExtraVisitDurationForVisitTypeMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getExtraVisitDurationForVisitType() {
return getExtraVisitDurationForVisitTypeMap();
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public java.util.Map
getExtraVisitDurationForVisitTypeMap() {
return internalGetExtraVisitDurationForVisitType().getImmutableMap();
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public /* nullable */ com.google.protobuf.Duration getExtraVisitDurationForVisitTypeOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Duration defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetMutableExtraVisitDurationForVisitType().ensureBuilderMap();
return map.containsKey(key)
? extraVisitDurationForVisitTypeConverter.build(map.get(key))
: defaultValue;
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
@java.lang.Override
public com.google.protobuf.Duration getExtraVisitDurationForVisitTypeOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetMutableExtraVisitDurationForVisitType().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return extraVisitDurationForVisitTypeConverter.build(map.get(key));
}
public Builder clearExtraVisitDurationForVisitType() {
bitField0_ = (bitField0_ & ~0x00400000);
internalGetMutableExtraVisitDurationForVisitType().clear();
return this;
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
public Builder removeExtraVisitDurationForVisitType(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableExtraVisitDurationForVisitType().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map
getMutableExtraVisitDurationForVisitType() {
bitField0_ |= 0x00400000;
return internalGetMutableExtraVisitDurationForVisitType().ensureMessageMap();
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
public Builder putExtraVisitDurationForVisitType(
java.lang.String key, com.google.protobuf.Duration value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableExtraVisitDurationForVisitType().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00400000;
return this;
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
public Builder putAllExtraVisitDurationForVisitType(
java.util.Map values) {
for (java.util.Map.Entry e :
values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableExtraVisitDurationForVisitType().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00400000;
return this;
}
/**
*
*
*
* Specifies a map from visit_types strings to durations. The duration is time
* in addition to
* [VisitRequest.duration][google.maps.routeoptimization.v1.Shipment.VisitRequest.duration]
* to be taken at visits with the specified `visit_types`. This extra visit
* duration adds cost if `cost_per_hour` is specified. Keys (i.e.
* `visit_types`) cannot be empty strings.
*
* If a visit request has multiple types, a duration will be added for each
* type in the map.
*
*
* map<string, .google.protobuf.Duration> extra_visit_duration_for_visit_type = 24;
*
*/
public com.google.protobuf.Duration.Builder putExtraVisitDurationForVisitTypeBuilderIfAbsent(
java.lang.String key) {
java.util.Map builderMap =
internalGetMutableExtraVisitDurationForVisitType().ensureBuilderMap();
com.google.protobuf.DurationOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.protobuf.Duration.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.protobuf.Duration) {
entry = ((com.google.protobuf.Duration) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.protobuf.Duration.Builder) entry;
}
private com.google.maps.routeoptimization.v1.BreakRule breakRule_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.BreakRule,
com.google.maps.routeoptimization.v1.BreakRule.Builder,
com.google.maps.routeoptimization.v1.BreakRuleOrBuilder>
breakRuleBuilder_;
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*
* @return Whether the breakRule field is set.
*/
public boolean hasBreakRule() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*
* @return The breakRule.
*/
public com.google.maps.routeoptimization.v1.BreakRule getBreakRule() {
if (breakRuleBuilder_ == null) {
return breakRule_ == null
? com.google.maps.routeoptimization.v1.BreakRule.getDefaultInstance()
: breakRule_;
} else {
return breakRuleBuilder_.getMessage();
}
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
public Builder setBreakRule(com.google.maps.routeoptimization.v1.BreakRule value) {
if (breakRuleBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
breakRule_ = value;
} else {
breakRuleBuilder_.setMessage(value);
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
public Builder setBreakRule(
com.google.maps.routeoptimization.v1.BreakRule.Builder builderForValue) {
if (breakRuleBuilder_ == null) {
breakRule_ = builderForValue.build();
} else {
breakRuleBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
public Builder mergeBreakRule(com.google.maps.routeoptimization.v1.BreakRule value) {
if (breakRuleBuilder_ == null) {
if (((bitField0_ & 0x00800000) != 0)
&& breakRule_ != null
&& breakRule_ != com.google.maps.routeoptimization.v1.BreakRule.getDefaultInstance()) {
getBreakRuleBuilder().mergeFrom(value);
} else {
breakRule_ = value;
}
} else {
breakRuleBuilder_.mergeFrom(value);
}
if (breakRule_ != null) {
bitField0_ |= 0x00800000;
onChanged();
}
return this;
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
public Builder clearBreakRule() {
bitField0_ = (bitField0_ & ~0x00800000);
breakRule_ = null;
if (breakRuleBuilder_ != null) {
breakRuleBuilder_.dispose();
breakRuleBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
public com.google.maps.routeoptimization.v1.BreakRule.Builder getBreakRuleBuilder() {
bitField0_ |= 0x00800000;
onChanged();
return getBreakRuleFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
public com.google.maps.routeoptimization.v1.BreakRuleOrBuilder getBreakRuleOrBuilder() {
if (breakRuleBuilder_ != null) {
return breakRuleBuilder_.getMessageOrBuilder();
} else {
return breakRule_ == null
? com.google.maps.routeoptimization.v1.BreakRule.getDefaultInstance()
: breakRule_;
}
}
/**
*
*
*
* Describes the break schedule to be enforced on this vehicle.
* If empty, no breaks will be scheduled for this vehicle.
*
*
* .google.maps.routeoptimization.v1.BreakRule break_rule = 25;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.BreakRule,
com.google.maps.routeoptimization.v1.BreakRule.Builder,
com.google.maps.routeoptimization.v1.BreakRuleOrBuilder>
getBreakRuleFieldBuilder() {
if (breakRuleBuilder_ == null) {
breakRuleBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.maps.routeoptimization.v1.BreakRule,
com.google.maps.routeoptimization.v1.BreakRule.Builder,
com.google.maps.routeoptimization.v1.BreakRuleOrBuilder>(
getBreakRule(), getParentForChildren(), isClean());
breakRule_ = null;
}
return breakRuleBuilder_;
}
private java.lang.Object label_ = "";
/**
*
*
*
* Specifies a label for this vehicle. This label is reported in the response
* as the `vehicle_label` of the corresponding
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
*
* string label = 27;
*
* @return The label.
*/
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
label_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies a label for this vehicle. This label is reported in the response
* as the `vehicle_label` of the corresponding
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
*
* string label = 27;
*
* @return The bytes for label.
*/
public com.google.protobuf.ByteString getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies a label for this vehicle. This label is reported in the response
* as the `vehicle_label` of the corresponding
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
*
* string label = 27;
*
* @param value The label to set.
* @return This builder for chaining.
*/
public Builder setLabel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
label_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
*
*
* Specifies a label for this vehicle. This label is reported in the response
* as the `vehicle_label` of the corresponding
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
*
* string label = 27;
*
* @return This builder for chaining.
*/
public Builder clearLabel() {
label_ = getDefaultInstance().getLabel();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
return this;
}
/**
*
*
*
* Specifies a label for this vehicle. This label is reported in the response
* as the `vehicle_label` of the corresponding
* [ShipmentRoute][google.maps.routeoptimization.v1.ShipmentRoute].
*
*
* string label = 27;
*
* @param value The bytes for label to set.
* @return This builder for chaining.
*/
public Builder setLabelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
label_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
private boolean ignore_;
/**
*
*
*
* If true, `used_if_route_is_empty` must be false, and this vehicle will
* remain unused.
*
* If a shipment is performed by an ignored vehicle in
* `injected_first_solution_routes`, it is skipped in the first solution but
* is free to be performed in the response.
*
* If a shipment is performed by an ignored vehicle in
* `injected_solution_constraint` and any related pickup/delivery is
* constrained to remain on the vehicle (i.e., not relaxed to level
* `RELAX_ALL_AFTER_THRESHOLD`), it is skipped in the response.
* If a shipment has a non-empty `allowed_vehicle_indices` field and all of
* the allowed vehicles are ignored, it is skipped in the response.
*
*
* bool ignore = 28;
*
* @return The ignore.
*/
@java.lang.Override
public boolean getIgnore() {
return ignore_;
}
/**
*
*
*
* If true, `used_if_route_is_empty` must be false, and this vehicle will
* remain unused.
*
* If a shipment is performed by an ignored vehicle in
* `injected_first_solution_routes`, it is skipped in the first solution but
* is free to be performed in the response.
*
* If a shipment is performed by an ignored vehicle in
* `injected_solution_constraint` and any related pickup/delivery is
* constrained to remain on the vehicle (i.e., not relaxed to level
* `RELAX_ALL_AFTER_THRESHOLD`), it is skipped in the response.
* If a shipment has a non-empty `allowed_vehicle_indices` field and all of
* the allowed vehicles are ignored, it is skipped in the response.
*
*
* bool ignore = 28;
*
* @param value The ignore to set.
* @return This builder for chaining.
*/
public Builder setIgnore(boolean value) {
ignore_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
*
*
* If true, `used_if_route_is_empty` must be false, and this vehicle will
* remain unused.
*
* If a shipment is performed by an ignored vehicle in
* `injected_first_solution_routes`, it is skipped in the first solution but
* is free to be performed in the response.
*
* If a shipment is performed by an ignored vehicle in
* `injected_solution_constraint` and any related pickup/delivery is
* constrained to remain on the vehicle (i.e., not relaxed to level
* `RELAX_ALL_AFTER_THRESHOLD`), it is skipped in the response.
* If a shipment has a non-empty `allowed_vehicle_indices` field and all of
* the allowed vehicles are ignored, it is skipped in the response.
*
*
* bool ignore = 28;
*
* @return This builder for chaining.
*/
public Builder clearIgnore() {
bitField0_ = (bitField0_ & ~0x02000000);
ignore_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.maps.routeoptimization.v1.Vehicle)
}
// @@protoc_insertion_point(class_scope:google.maps.routeoptimization.v1.Vehicle)
private static final com.google.maps.routeoptimization.v1.Vehicle DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.maps.routeoptimization.v1.Vehicle();
}
public static com.google.maps.routeoptimization.v1.Vehicle getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Vehicle parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.maps.routeoptimization.v1.Vehicle getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy