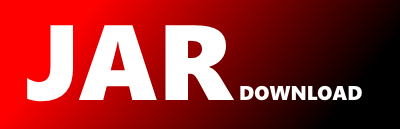
com.google.maps.routing.v2.RouteLegOrBuilder Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/maps/routing/v2/route.proto
// Protobuf Java Version: 3.25.3
package com.google.maps.routing.v2;
public interface RouteLegOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routing.v2.RouteLeg)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The travel distance of the route leg, in meters.
*
*
* int32 distance_meters = 1;
*
* @return The distanceMeters.
*/
int getDistanceMeters();
/**
*
*
*
* The length of time needed to navigate the leg. If the `route_preference`
* is set to `TRAFFIC_UNAWARE`, then this value is the same as
* `static_duration`. If the `route_preference` is either `TRAFFIC_AWARE` or
* `TRAFFIC_AWARE_OPTIMAL`, then this value is calculated taking traffic
* conditions into account.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return Whether the duration field is set.
*/
boolean hasDuration();
/**
*
*
*
* The length of time needed to navigate the leg. If the `route_preference`
* is set to `TRAFFIC_UNAWARE`, then this value is the same as
* `static_duration`. If the `route_preference` is either `TRAFFIC_AWARE` or
* `TRAFFIC_AWARE_OPTIMAL`, then this value is calculated taking traffic
* conditions into account.
*
*
* .google.protobuf.Duration duration = 2;
*
* @return The duration.
*/
com.google.protobuf.Duration getDuration();
/**
*
*
*
* The length of time needed to navigate the leg. If the `route_preference`
* is set to `TRAFFIC_UNAWARE`, then this value is the same as
* `static_duration`. If the `route_preference` is either `TRAFFIC_AWARE` or
* `TRAFFIC_AWARE_OPTIMAL`, then this value is calculated taking traffic
* conditions into account.
*
*
* .google.protobuf.Duration duration = 2;
*/
com.google.protobuf.DurationOrBuilder getDurationOrBuilder();
/**
*
*
*
* The duration of travel through the leg, calculated without taking
* traffic conditions into consideration.
*
*
* .google.protobuf.Duration static_duration = 3;
*
* @return Whether the staticDuration field is set.
*/
boolean hasStaticDuration();
/**
*
*
*
* The duration of travel through the leg, calculated without taking
* traffic conditions into consideration.
*
*
* .google.protobuf.Duration static_duration = 3;
*
* @return The staticDuration.
*/
com.google.protobuf.Duration getStaticDuration();
/**
*
*
*
* The duration of travel through the leg, calculated without taking
* traffic conditions into consideration.
*
*
* .google.protobuf.Duration static_duration = 3;
*/
com.google.protobuf.DurationOrBuilder getStaticDurationOrBuilder();
/**
*
*
*
* The overall polyline for this leg that includes each `step`'s
* polyline.
*
*
* .google.maps.routing.v2.Polyline polyline = 4;
*
* @return Whether the polyline field is set.
*/
boolean hasPolyline();
/**
*
*
*
* The overall polyline for this leg that includes each `step`'s
* polyline.
*
*
* .google.maps.routing.v2.Polyline polyline = 4;
*
* @return The polyline.
*/
com.google.maps.routing.v2.Polyline getPolyline();
/**
*
*
*
* The overall polyline for this leg that includes each `step`'s
* polyline.
*
*
* .google.maps.routing.v2.Polyline polyline = 4;
*/
com.google.maps.routing.v2.PolylineOrBuilder getPolylineOrBuilder();
/**
*
*
*
* The start location of this leg. This location might be different from the
* provided `origin`. For example, when the provided `origin` is not near a
* road, this is a point on the road.
*
*
* .google.maps.routing.v2.Location start_location = 5;
*
* @return Whether the startLocation field is set.
*/
boolean hasStartLocation();
/**
*
*
*
* The start location of this leg. This location might be different from the
* provided `origin`. For example, when the provided `origin` is not near a
* road, this is a point on the road.
*
*
* .google.maps.routing.v2.Location start_location = 5;
*
* @return The startLocation.
*/
com.google.maps.routing.v2.Location getStartLocation();
/**
*
*
*
* The start location of this leg. This location might be different from the
* provided `origin`. For example, when the provided `origin` is not near a
* road, this is a point on the road.
*
*
* .google.maps.routing.v2.Location start_location = 5;
*/
com.google.maps.routing.v2.LocationOrBuilder getStartLocationOrBuilder();
/**
*
*
*
* The end location of this leg. This location might be different from the
* provided `destination`. For example, when the provided `destination` is not
* near a road, this is a point on the road.
*
*
* .google.maps.routing.v2.Location end_location = 6;
*
* @return Whether the endLocation field is set.
*/
boolean hasEndLocation();
/**
*
*
*
* The end location of this leg. This location might be different from the
* provided `destination`. For example, when the provided `destination` is not
* near a road, this is a point on the road.
*
*
* .google.maps.routing.v2.Location end_location = 6;
*
* @return The endLocation.
*/
com.google.maps.routing.v2.Location getEndLocation();
/**
*
*
*
* The end location of this leg. This location might be different from the
* provided `destination`. For example, when the provided `destination` is not
* near a road, this is a point on the road.
*
*
* .google.maps.routing.v2.Location end_location = 6;
*/
com.google.maps.routing.v2.LocationOrBuilder getEndLocationOrBuilder();
/**
*
*
*
* An array of steps denoting segments within this leg. Each step represents
* one navigation instruction.
*
*
* repeated .google.maps.routing.v2.RouteLegStep steps = 7;
*/
java.util.List getStepsList();
/**
*
*
*
* An array of steps denoting segments within this leg. Each step represents
* one navigation instruction.
*
*
* repeated .google.maps.routing.v2.RouteLegStep steps = 7;
*/
com.google.maps.routing.v2.RouteLegStep getSteps(int index);
/**
*
*
*
* An array of steps denoting segments within this leg. Each step represents
* one navigation instruction.
*
*
* repeated .google.maps.routing.v2.RouteLegStep steps = 7;
*/
int getStepsCount();
/**
*
*
*
* An array of steps denoting segments within this leg. Each step represents
* one navigation instruction.
*
*
* repeated .google.maps.routing.v2.RouteLegStep steps = 7;
*/
java.util.List extends com.google.maps.routing.v2.RouteLegStepOrBuilder>
getStepsOrBuilderList();
/**
*
*
*
* An array of steps denoting segments within this leg. Each step represents
* one navigation instruction.
*
*
* repeated .google.maps.routing.v2.RouteLegStep steps = 7;
*/
com.google.maps.routing.v2.RouteLegStepOrBuilder getStepsOrBuilder(int index);
/**
*
*
*
* Contains the additional information that the user should be informed
* about, such as possible traffic zone restrictions, on a route leg.
*
*
* .google.maps.routing.v2.RouteLegTravelAdvisory travel_advisory = 8;
*
* @return Whether the travelAdvisory field is set.
*/
boolean hasTravelAdvisory();
/**
*
*
*
* Contains the additional information that the user should be informed
* about, such as possible traffic zone restrictions, on a route leg.
*
*
* .google.maps.routing.v2.RouteLegTravelAdvisory travel_advisory = 8;
*
* @return The travelAdvisory.
*/
com.google.maps.routing.v2.RouteLegTravelAdvisory getTravelAdvisory();
/**
*
*
*
* Contains the additional information that the user should be informed
* about, such as possible traffic zone restrictions, on a route leg.
*
*
* .google.maps.routing.v2.RouteLegTravelAdvisory travel_advisory = 8;
*/
com.google.maps.routing.v2.RouteLegTravelAdvisoryOrBuilder getTravelAdvisoryOrBuilder();
/**
*
*
*
* Text representations of properties of the `RouteLeg`.
*
*
* .google.maps.routing.v2.RouteLeg.RouteLegLocalizedValues localized_values = 9;
*
* @return Whether the localizedValues field is set.
*/
boolean hasLocalizedValues();
/**
*
*
*
* Text representations of properties of the `RouteLeg`.
*
*
* .google.maps.routing.v2.RouteLeg.RouteLegLocalizedValues localized_values = 9;
*
* @return The localizedValues.
*/
com.google.maps.routing.v2.RouteLeg.RouteLegLocalizedValues getLocalizedValues();
/**
*
*
*
* Text representations of properties of the `RouteLeg`.
*
*
* .google.maps.routing.v2.RouteLeg.RouteLegLocalizedValues localized_values = 9;
*/
com.google.maps.routing.v2.RouteLeg.RouteLegLocalizedValuesOrBuilder
getLocalizedValuesOrBuilder();
/**
*
*
*
* Overview information about the steps in this `RouteLeg`. This field is only
* populated for TRANSIT routes.
*
*
* .google.maps.routing.v2.RouteLeg.StepsOverview steps_overview = 10;
*
* @return Whether the stepsOverview field is set.
*/
boolean hasStepsOverview();
/**
*
*
*
* Overview information about the steps in this `RouteLeg`. This field is only
* populated for TRANSIT routes.
*
*
* .google.maps.routing.v2.RouteLeg.StepsOverview steps_overview = 10;
*
* @return The stepsOverview.
*/
com.google.maps.routing.v2.RouteLeg.StepsOverview getStepsOverview();
/**
*
*
*
* Overview information about the steps in this `RouteLeg`. This field is only
* populated for TRANSIT routes.
*
*
* .google.maps.routing.v2.RouteLeg.StepsOverview steps_overview = 10;
*/
com.google.maps.routing.v2.RouteLeg.StepsOverviewOrBuilder getStepsOverviewOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy