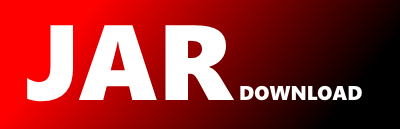
com.google.maps.routing.v2.RouteTravelAdvisoryOrBuilder Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/maps/routing/v2/route.proto
// Protobuf Java Version: 3.25.3
package com.google.maps.routing.v2;
public interface RouteTravelAdvisoryOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.maps.routing.v2.RouteTravelAdvisory)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Contains information about tolls on the route. This field is only populated
* if tolls are expected on the route. If this field is set, but the
* `estimatedPrice` subfield is not populated, then the route contains tolls,
* but the estimated price is unknown. If this field is not set, then there
* are no tolls expected on the route.
*
*
* .google.maps.routing.v2.TollInfo toll_info = 2;
*
* @return Whether the tollInfo field is set.
*/
boolean hasTollInfo();
/**
*
*
*
* Contains information about tolls on the route. This field is only populated
* if tolls are expected on the route. If this field is set, but the
* `estimatedPrice` subfield is not populated, then the route contains tolls,
* but the estimated price is unknown. If this field is not set, then there
* are no tolls expected on the route.
*
*
* .google.maps.routing.v2.TollInfo toll_info = 2;
*
* @return The tollInfo.
*/
com.google.maps.routing.v2.TollInfo getTollInfo();
/**
*
*
*
* Contains information about tolls on the route. This field is only populated
* if tolls are expected on the route. If this field is set, but the
* `estimatedPrice` subfield is not populated, then the route contains tolls,
* but the estimated price is unknown. If this field is not set, then there
* are no tolls expected on the route.
*
*
* .google.maps.routing.v2.TollInfo toll_info = 2;
*/
com.google.maps.routing.v2.TollInfoOrBuilder getTollInfoOrBuilder();
/**
*
*
*
* Speed reading intervals detailing traffic density. Applicable in case of
* `TRAFFIC_AWARE` and `TRAFFIC_AWARE_OPTIMAL` routing preferences.
* The intervals cover the entire polyline of the route without overlap.
* The start point of a specified interval is the same as the end point of the
* preceding interval.
*
* Example:
*
* polyline: A ---- B ---- C ---- D ---- E ---- F ---- G
* speed_reading_intervals: [A,C), [C,D), [D,G).
*
*
* repeated .google.maps.routing.v2.SpeedReadingInterval speed_reading_intervals = 3;
*/
java.util.List getSpeedReadingIntervalsList();
/**
*
*
*
* Speed reading intervals detailing traffic density. Applicable in case of
* `TRAFFIC_AWARE` and `TRAFFIC_AWARE_OPTIMAL` routing preferences.
* The intervals cover the entire polyline of the route without overlap.
* The start point of a specified interval is the same as the end point of the
* preceding interval.
*
* Example:
*
* polyline: A ---- B ---- C ---- D ---- E ---- F ---- G
* speed_reading_intervals: [A,C), [C,D), [D,G).
*
*
* repeated .google.maps.routing.v2.SpeedReadingInterval speed_reading_intervals = 3;
*/
com.google.maps.routing.v2.SpeedReadingInterval getSpeedReadingIntervals(int index);
/**
*
*
*
* Speed reading intervals detailing traffic density. Applicable in case of
* `TRAFFIC_AWARE` and `TRAFFIC_AWARE_OPTIMAL` routing preferences.
* The intervals cover the entire polyline of the route without overlap.
* The start point of a specified interval is the same as the end point of the
* preceding interval.
*
* Example:
*
* polyline: A ---- B ---- C ---- D ---- E ---- F ---- G
* speed_reading_intervals: [A,C), [C,D), [D,G).
*
*
* repeated .google.maps.routing.v2.SpeedReadingInterval speed_reading_intervals = 3;
*/
int getSpeedReadingIntervalsCount();
/**
*
*
*
* Speed reading intervals detailing traffic density. Applicable in case of
* `TRAFFIC_AWARE` and `TRAFFIC_AWARE_OPTIMAL` routing preferences.
* The intervals cover the entire polyline of the route without overlap.
* The start point of a specified interval is the same as the end point of the
* preceding interval.
*
* Example:
*
* polyline: A ---- B ---- C ---- D ---- E ---- F ---- G
* speed_reading_intervals: [A,C), [C,D), [D,G).
*
*
* repeated .google.maps.routing.v2.SpeedReadingInterval speed_reading_intervals = 3;
*/
java.util.List extends com.google.maps.routing.v2.SpeedReadingIntervalOrBuilder>
getSpeedReadingIntervalsOrBuilderList();
/**
*
*
*
* Speed reading intervals detailing traffic density. Applicable in case of
* `TRAFFIC_AWARE` and `TRAFFIC_AWARE_OPTIMAL` routing preferences.
* The intervals cover the entire polyline of the route without overlap.
* The start point of a specified interval is the same as the end point of the
* preceding interval.
*
* Example:
*
* polyline: A ---- B ---- C ---- D ---- E ---- F ---- G
* speed_reading_intervals: [A,C), [C,D), [D,G).
*
*
* repeated .google.maps.routing.v2.SpeedReadingInterval speed_reading_intervals = 3;
*/
com.google.maps.routing.v2.SpeedReadingIntervalOrBuilder getSpeedReadingIntervalsOrBuilder(
int index);
/**
*
*
*
* The predicted fuel consumption in microliters.
*
*
* int64 fuel_consumption_microliters = 5;
*
* @return The fuelConsumptionMicroliters.
*/
long getFuelConsumptionMicroliters();
/**
*
*
*
* Returned route may have restrictions that are not suitable for requested
* travel mode or route modifiers.
*
*
* bool route_restrictions_partially_ignored = 6;
*
* @return The routeRestrictionsPartiallyIgnored.
*/
boolean getRouteRestrictionsPartiallyIgnored();
/**
*
*
*
* If present, contains the total fare or ticket costs on this route
* This property is only returned for `TRANSIT` requests and only
* for routes where fare information is available for all transit steps.
*
*
* .google.type.Money transit_fare = 7;
*
* @return Whether the transitFare field is set.
*/
boolean hasTransitFare();
/**
*
*
*
* If present, contains the total fare or ticket costs on this route
* This property is only returned for `TRANSIT` requests and only
* for routes where fare information is available for all transit steps.
*
*
* .google.type.Money transit_fare = 7;
*
* @return The transitFare.
*/
com.google.type.Money getTransitFare();
/**
*
*
*
* If present, contains the total fare or ticket costs on this route
* This property is only returned for `TRANSIT` requests and only
* for routes where fare information is available for all transit steps.
*
*
* .google.type.Money transit_fare = 7;
*/
com.google.type.MoneyOrBuilder getTransitFareOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy