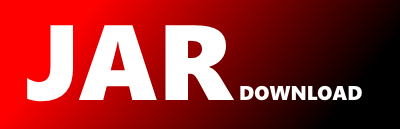
com.google.maps.fleetengine.v1.TripServiceClient Maven / Gradle / Ivy
Show all versions of google-maps-fleetengine Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.maps.fleetengine.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.maps.fleetengine.v1.stub.TripServiceStub;
import com.google.maps.fleetengine.v1.stub.TripServiceStubSettings;
import com.google.protobuf.Empty;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Trip management service.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* CreateTripRequest request =
* CreateTripRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setParent(TripName.of("[PROVIDER]", "[TRIP]").toString())
* .setTripId("tripId-865466336")
* .setTrip(Trip.newBuilder().build())
* .build();
* Trip response = tripServiceClient.createTrip(request);
* }
* }
*
* Note: close() needs to be called on the TripServiceClient object to clean up resources such as
* threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateTrip
* Creates a trip in the Fleet Engine and returns the new trip.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createTrip(CreateTripRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createTripCallable()
*
*
*
*
* GetTrip
* Get information about a single trip.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getTrip(GetTripRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getTripCallable()
*
*
*
*
* ReportBillableTrip
* Report billable trip usage.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* reportBillableTrip(ReportBillableTripRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* reportBillableTripCallable()
*
*
*
*
* SearchTrips
* Get all the trips for a specific vehicle.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* searchTrips(SearchTripsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* searchTripsPagedCallable()
*
searchTripsCallable()
*
*
*
*
* UpdateTrip
* Updates trip data.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateTrip(UpdateTripRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateTripCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of TripServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* TripServiceSettings tripServiceSettings =
* TripServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* TripServiceClient tripServiceClient = TripServiceClient.create(tripServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* TripServiceSettings tripServiceSettings =
* TripServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* TripServiceClient tripServiceClient = TripServiceClient.create(tripServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class TripServiceClient implements BackgroundResource {
private final TripServiceSettings settings;
private final TripServiceStub stub;
/** Constructs an instance of TripServiceClient with default settings. */
public static final TripServiceClient create() throws IOException {
return create(TripServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of TripServiceClient, using the given settings. The channels are created
* based on the settings passed in, or defaults for any settings that are not set.
*/
public static final TripServiceClient create(TripServiceSettings settings) throws IOException {
return new TripServiceClient(settings);
}
/**
* Constructs an instance of TripServiceClient, using the given stub for making calls. This is for
* advanced usage - prefer using create(TripServiceSettings).
*/
public static final TripServiceClient create(TripServiceStub stub) {
return new TripServiceClient(stub);
}
/**
* Constructs an instance of TripServiceClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected TripServiceClient(TripServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((TripServiceStubSettings) settings.getStubSettings()).createStub();
}
protected TripServiceClient(TripServiceStub stub) {
this.settings = null;
this.stub = stub;
}
public final TripServiceSettings getSettings() {
return settings;
}
public TripServiceStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a trip in the Fleet Engine and returns the new trip.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* CreateTripRequest request =
* CreateTripRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setParent(TripName.of("[PROVIDER]", "[TRIP]").toString())
* .setTripId("tripId-865466336")
* .setTrip(Trip.newBuilder().build())
* .build();
* Trip response = tripServiceClient.createTrip(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Trip createTrip(CreateTripRequest request) {
return createTripCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a trip in the Fleet Engine and returns the new trip.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* CreateTripRequest request =
* CreateTripRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setParent(TripName.of("[PROVIDER]", "[TRIP]").toString())
* .setTripId("tripId-865466336")
* .setTrip(Trip.newBuilder().build())
* .build();
* ApiFuture future = tripServiceClient.createTripCallable().futureCall(request);
* // Do something.
* Trip response = future.get();
* }
* }
*/
public final UnaryCallable createTripCallable() {
return stub.createTripCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get information about a single trip.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* GetTripRequest request =
* GetTripRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setName(TripName.of("[PROVIDER]", "[TRIP]").toString())
* .setView(TripView.forNumber(0))
* .setCurrentRouteSegmentVersion(Timestamp.newBuilder().build())
* .setRemainingWaypointsVersion(Timestamp.newBuilder().build())
* .setRouteFormatType(PolylineFormatType.forNumber(0))
* .setCurrentRouteSegmentTrafficVersion(Timestamp.newBuilder().build())
* .setRemainingWaypointsRouteVersion(Timestamp.newBuilder().build())
* .build();
* Trip response = tripServiceClient.getTrip(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Trip getTrip(GetTripRequest request) {
return getTripCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get information about a single trip.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* GetTripRequest request =
* GetTripRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setName(TripName.of("[PROVIDER]", "[TRIP]").toString())
* .setView(TripView.forNumber(0))
* .setCurrentRouteSegmentVersion(Timestamp.newBuilder().build())
* .setRemainingWaypointsVersion(Timestamp.newBuilder().build())
* .setRouteFormatType(PolylineFormatType.forNumber(0))
* .setCurrentRouteSegmentTrafficVersion(Timestamp.newBuilder().build())
* .setRemainingWaypointsRouteVersion(Timestamp.newBuilder().build())
* .build();
* ApiFuture future = tripServiceClient.getTripCallable().futureCall(request);
* // Do something.
* Trip response = future.get();
* }
* }
*/
public final UnaryCallable getTripCallable() {
return stub.getTripCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Report billable trip usage.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* ReportBillableTripRequest request =
* ReportBillableTripRequest.newBuilder()
* .setName("name3373707")
* .setCountryCode("countryCode-1477067101")
* .setPlatform(BillingPlatformIdentifier.forNumber(0))
* .addAllRelatedIds(new ArrayList())
* .build();
* tripServiceClient.reportBillableTrip(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void reportBillableTrip(ReportBillableTripRequest request) {
reportBillableTripCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Report billable trip usage.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* ReportBillableTripRequest request =
* ReportBillableTripRequest.newBuilder()
* .setName("name3373707")
* .setCountryCode("countryCode-1477067101")
* .setPlatform(BillingPlatformIdentifier.forNumber(0))
* .addAllRelatedIds(new ArrayList())
* .build();
* ApiFuture future = tripServiceClient.reportBillableTripCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable reportBillableTripCallable() {
return stub.reportBillableTripCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get all the trips for a specific vehicle.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* SearchTripsRequest request =
* SearchTripsRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setParent("parent-995424086")
* .setVehicleId("vehicleId-1984135833")
* .setActiveTripsOnly(true)
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setMinimumStaleness(Duration.newBuilder().build())
* .build();
* for (Trip element : tripServiceClient.searchTrips(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchTripsPagedResponse searchTrips(SearchTripsRequest request) {
return searchTripsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get all the trips for a specific vehicle.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* SearchTripsRequest request =
* SearchTripsRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setParent("parent-995424086")
* .setVehicleId("vehicleId-1984135833")
* .setActiveTripsOnly(true)
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setMinimumStaleness(Duration.newBuilder().build())
* .build();
* ApiFuture future = tripServiceClient.searchTripsPagedCallable().futureCall(request);
* // Do something.
* for (Trip element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
searchTripsPagedCallable() {
return stub.searchTripsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get all the trips for a specific vehicle.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* SearchTripsRequest request =
* SearchTripsRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setParent("parent-995424086")
* .setVehicleId("vehicleId-1984135833")
* .setActiveTripsOnly(true)
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setMinimumStaleness(Duration.newBuilder().build())
* .build();
* while (true) {
* SearchTripsResponse response = tripServiceClient.searchTripsCallable().call(request);
* for (Trip element : response.getTripsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable searchTripsCallable() {
return stub.searchTripsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates trip data.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* UpdateTripRequest request =
* UpdateTripRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setName("name3373707")
* .setTrip(Trip.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Trip response = tripServiceClient.updateTrip(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Trip updateTrip(UpdateTripRequest request) {
return updateTripCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates trip data.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TripServiceClient tripServiceClient = TripServiceClient.create()) {
* UpdateTripRequest request =
* UpdateTripRequest.newBuilder()
* .setHeader(RequestHeader.newBuilder().build())
* .setName("name3373707")
* .setTrip(Trip.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = tripServiceClient.updateTripCallable().futureCall(request);
* // Do something.
* Trip response = future.get();
* }
* }
*/
public final UnaryCallable updateTripCallable() {
return stub.updateTripCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class SearchTripsPagedResponse
extends AbstractPagedListResponse<
SearchTripsRequest,
SearchTripsResponse,
Trip,
SearchTripsPage,
SearchTripsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
SearchTripsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new SearchTripsPagedResponse(input), MoreExecutors.directExecutor());
}
private SearchTripsPagedResponse(SearchTripsPage page) {
super(page, SearchTripsFixedSizeCollection.createEmptyCollection());
}
}
public static class SearchTripsPage
extends AbstractPage {
private SearchTripsPage(
PageContext context,
SearchTripsResponse response) {
super(context, response);
}
private static SearchTripsPage createEmptyPage() {
return new SearchTripsPage(null, null);
}
@Override
protected SearchTripsPage createPage(
PageContext context,
SearchTripsResponse response) {
return new SearchTripsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class SearchTripsFixedSizeCollection
extends AbstractFixedSizeCollection<
SearchTripsRequest,
SearchTripsResponse,
Trip,
SearchTripsPage,
SearchTripsFixedSizeCollection> {
private SearchTripsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static SearchTripsFixedSizeCollection createEmptyCollection() {
return new SearchTripsFixedSizeCollection(null, 0);
}
@Override
protected SearchTripsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new SearchTripsFixedSizeCollection(pages, collectionSize);
}
}
}