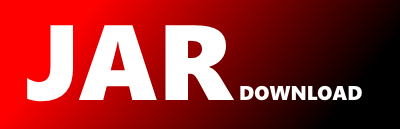
com.google.maps.fleetengine.v1.VehicleServiceSettings Maven / Gradle / Ivy
Show all versions of google-maps-fleetengine Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.maps.fleetengine.v1;
import static com.google.maps.fleetengine.v1.VehicleServiceClient.ListVehiclesPagedResponse;
import com.google.api.core.ApiFunction;
import com.google.api.gax.core.GoogleCredentialsProvider;
import com.google.api.gax.core.InstantiatingExecutorProvider;
import com.google.api.gax.grpc.InstantiatingGrpcChannelProvider;
import com.google.api.gax.rpc.ApiClientHeaderProvider;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.ClientSettings;
import com.google.api.gax.rpc.PagedCallSettings;
import com.google.api.gax.rpc.TransportChannelProvider;
import com.google.api.gax.rpc.UnaryCallSettings;
import com.google.maps.fleetengine.v1.stub.VehicleServiceStubSettings;
import java.io.IOException;
import java.util.List;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Settings class to configure an instance of {@link VehicleServiceClient}.
*
* The default instance has everything set to sensible defaults:
*
*
* - The default service address (fleetengine.googleapis.com) and default port (443) are used.
*
- Credentials are acquired automatically through Application Default Credentials.
*
- Retries are configured for idempotent methods but not for non-idempotent methods.
*
*
* The builder of this class is recursive, so contained classes are themselves builders. When
* build() is called, the tree of builders is called to create the complete settings object.
*
*
For example, to set the
* [RetrySettings](https://cloud.google.com/java/docs/reference/gax/latest/com.google.api.gax.retrying.RetrySettings)
* of createVehicle:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* VehicleServiceSettings.Builder vehicleServiceSettingsBuilder =
* VehicleServiceSettings.newBuilder();
* vehicleServiceSettingsBuilder
* .createVehicleSettings()
* .setRetrySettings(
* vehicleServiceSettingsBuilder
* .createVehicleSettings()
* .getRetrySettings()
* .toBuilder()
* .setInitialRetryDelayDuration(Duration.ofSeconds(1))
* .setInitialRpcTimeoutDuration(Duration.ofSeconds(5))
* .setMaxAttempts(5)
* .setMaxRetryDelayDuration(Duration.ofSeconds(30))
* .setMaxRpcTimeoutDuration(Duration.ofSeconds(60))
* .setRetryDelayMultiplier(1.3)
* .setRpcTimeoutMultiplier(1.5)
* .setTotalTimeoutDuration(Duration.ofSeconds(300))
* .build());
* VehicleServiceSettings vehicleServiceSettings = vehicleServiceSettingsBuilder.build();
* }
*
* Please refer to the [Client Side Retry
* Guide](https://github.com/googleapis/google-cloud-java/blob/main/docs/client_retries.md) for
* additional support in setting retries.
*/
@Generated("by gapic-generator-java")
public class VehicleServiceSettings extends ClientSettings {
/** Returns the object with the settings used for calls to createVehicle. */
public UnaryCallSettings createVehicleSettings() {
return ((VehicleServiceStubSettings) getStubSettings()).createVehicleSettings();
}
/** Returns the object with the settings used for calls to getVehicle. */
public UnaryCallSettings getVehicleSettings() {
return ((VehicleServiceStubSettings) getStubSettings()).getVehicleSettings();
}
/** Returns the object with the settings used for calls to updateVehicle. */
public UnaryCallSettings updateVehicleSettings() {
return ((VehicleServiceStubSettings) getStubSettings()).updateVehicleSettings();
}
/** Returns the object with the settings used for calls to updateVehicleAttributes. */
public UnaryCallSettings
updateVehicleAttributesSettings() {
return ((VehicleServiceStubSettings) getStubSettings()).updateVehicleAttributesSettings();
}
/** Returns the object with the settings used for calls to listVehicles. */
public PagedCallSettings
listVehiclesSettings() {
return ((VehicleServiceStubSettings) getStubSettings()).listVehiclesSettings();
}
/** Returns the object with the settings used for calls to searchVehicles. */
public UnaryCallSettings searchVehiclesSettings() {
return ((VehicleServiceStubSettings) getStubSettings()).searchVehiclesSettings();
}
public static final VehicleServiceSettings create(VehicleServiceStubSettings stub)
throws IOException {
return new VehicleServiceSettings.Builder(stub.toBuilder()).build();
}
/** Returns a builder for the default ExecutorProvider for this service. */
public static InstantiatingExecutorProvider.Builder defaultExecutorProviderBuilder() {
return VehicleServiceStubSettings.defaultExecutorProviderBuilder();
}
/** Returns the default service endpoint. */
public static String getDefaultEndpoint() {
return VehicleServiceStubSettings.getDefaultEndpoint();
}
/** Returns the default service scopes. */
public static List getDefaultServiceScopes() {
return VehicleServiceStubSettings.getDefaultServiceScopes();
}
/** Returns a builder for the default credentials for this service. */
public static GoogleCredentialsProvider.Builder defaultCredentialsProviderBuilder() {
return VehicleServiceStubSettings.defaultCredentialsProviderBuilder();
}
/** Returns a builder for the default ChannelProvider for this service. */
public static InstantiatingGrpcChannelProvider.Builder defaultGrpcTransportProviderBuilder() {
return VehicleServiceStubSettings.defaultGrpcTransportProviderBuilder();
}
public static TransportChannelProvider defaultTransportChannelProvider() {
return VehicleServiceStubSettings.defaultTransportChannelProvider();
}
public static ApiClientHeaderProvider.Builder defaultApiClientHeaderProviderBuilder() {
return VehicleServiceStubSettings.defaultApiClientHeaderProviderBuilder();
}
/** Returns a new builder for this class. */
public static Builder newBuilder() {
return Builder.createDefault();
}
/** Returns a new builder for this class. */
public static Builder newBuilder(ClientContext clientContext) {
return new Builder(clientContext);
}
/** Returns a builder containing all the values of this settings class. */
public Builder toBuilder() {
return new Builder(this);
}
protected VehicleServiceSettings(Builder settingsBuilder) throws IOException {
super(settingsBuilder);
}
/** Builder for VehicleServiceSettings. */
public static class Builder extends ClientSettings.Builder {
protected Builder() throws IOException {
this(((ClientContext) null));
}
protected Builder(ClientContext clientContext) {
super(VehicleServiceStubSettings.newBuilder(clientContext));
}
protected Builder(VehicleServiceSettings settings) {
super(settings.getStubSettings().toBuilder());
}
protected Builder(VehicleServiceStubSettings.Builder stubSettings) {
super(stubSettings);
}
private static Builder createDefault() {
return new Builder(VehicleServiceStubSettings.newBuilder());
}
public VehicleServiceStubSettings.Builder getStubSettingsBuilder() {
return ((VehicleServiceStubSettings.Builder) getStubSettings());
}
/**
* Applies the given settings updater function to all of the unary API methods in this service.
*
* Note: This method does not support applying settings to streaming methods.
*/
public Builder applyToAllUnaryMethods(
ApiFunction, Void> settingsUpdater) {
super.applyToAllUnaryMethods(
getStubSettingsBuilder().unaryMethodSettingsBuilders(), settingsUpdater);
return this;
}
/** Returns the builder for the settings used for calls to createVehicle. */
public UnaryCallSettings.Builder createVehicleSettings() {
return getStubSettingsBuilder().createVehicleSettings();
}
/** Returns the builder for the settings used for calls to getVehicle. */
public UnaryCallSettings.Builder getVehicleSettings() {
return getStubSettingsBuilder().getVehicleSettings();
}
/** Returns the builder for the settings used for calls to updateVehicle. */
public UnaryCallSettings.Builder updateVehicleSettings() {
return getStubSettingsBuilder().updateVehicleSettings();
}
/** Returns the builder for the settings used for calls to updateVehicleAttributes. */
public UnaryCallSettings.Builder<
UpdateVehicleAttributesRequest, UpdateVehicleAttributesResponse>
updateVehicleAttributesSettings() {
return getStubSettingsBuilder().updateVehicleAttributesSettings();
}
/** Returns the builder for the settings used for calls to listVehicles. */
public PagedCallSettings.Builder<
ListVehiclesRequest, ListVehiclesResponse, ListVehiclesPagedResponse>
listVehiclesSettings() {
return getStubSettingsBuilder().listVehiclesSettings();
}
/** Returns the builder for the settings used for calls to searchVehicles. */
public UnaryCallSettings.Builder
searchVehiclesSettings() {
return getStubSettingsBuilder().searchVehiclesSettings();
}
@Override
public VehicleServiceSettings build() throws IOException {
return new VehicleServiceSettings(this);
}
}
}