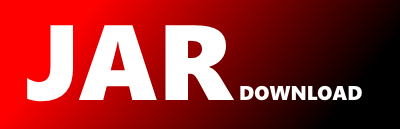
com.google.maps.clients.mapsengine.geojson.GeometryCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapsengine-api-java-wrapper Show documentation
Show all versions of mapsengine-api-java-wrapper Show documentation
Provides some extra sugar for the machine-generated Java library for Google Maps Engine. https://developers.google.com/api-client-library/java/apis/mapsengine/v1
package com.google.maps.clients.mapsengine.geojson;
import com.google.api.services.mapsengine.model.Feature;
import com.google.api.services.mapsengine.model.GeoJsonGeometry;
import com.google.api.services.mapsengine.model.GeoJsonGeometryCollection;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* A collection of {@link Geometry}s.
*/
public class GeometryCollection extends Geometry {
private final List geometries;
/**
* Creates a GeometryCollection from the provided Geometries
* @param geometries The ordered list of geometries
*/
public GeometryCollection(List geometries) {
this.geometries = geometries;
}
/**
* Converts the provided Feature into a typed GeometryCollection, discarding properties. Throws
* an exception if the feature is not a geometry collection.
* @param feature the Feature returned by the API
* @throws IllegalArgumentException when feature is not a geometry collection
*/
public GeometryCollection(Feature feature) {
GeoJsonGeometry geometry = feature.getGeometry();
if (!(geometry instanceof GeoJsonGeometryCollection)) {
throw new IllegalArgumentException("Feature is not a GeometryCollection: " +
geometry.getType());
}
GeoJsonGeometryCollection geometryCollection = (GeoJsonGeometryCollection) geometry;
List jsonGeometries = geometryCollection.getGeometries();
geometries = new ArrayList(jsonGeometries.size());
for (GeoJsonGeometry jsonGeometry : jsonGeometries) {
geometries.add(Geometry.fromGeoJson(jsonGeometry));
}
}
/**
* Returns a Feature that can be used by the Maps Engine API.
*
* @param properties The properties to attach to the feature
* @return an API-compatible Feature object
*/
@Override
public Feature asFeature(Map properties) {
if (properties == null) {
throw new IllegalArgumentException("Properties are required, even if empty");
}
List geoJsonGeometries = new ArrayList(geometries.size());
for (Geometry geometry : geometries) {
geoJsonGeometries.add(geometry.asFeature(properties).getGeometry());
}
GeoJsonGeometryCollection geoJsonCollection = new GeoJsonGeometryCollection();
geoJsonCollection.setGeometries(geoJsonGeometries);
Feature feature = new Feature();
feature.setType(FEATURE_TYPE);
feature.setGeometry(geoJsonCollection);
feature.setProperties(properties);
return feature;
}
/** Retrieves the list of geometries */
public List getGeometries() {
return geometries;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy