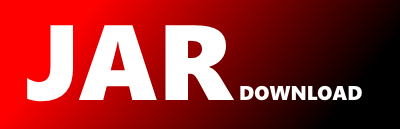
com.google.re2j.UnicodeTables Maven / Gradle / Ivy
// AUTOGENERATED by make_unicode_tables.awk from the output of
// go/src/pkg/unicode/maketables.go. Yes it's awful, but frankly
// it's quicker than porting 1300 more lines of Go.
package com.google.re2j;
import java.util.HashMap;
import java.util.Map;
class UnicodeTables {
static final int UpperCase = 0;
static final int LowerCase = 1;
static final int TitleCase = 2;
static final int UpperLower = 0x110000;
private static Map Categories() {
Map map = new HashMap();
map.put("Lm", Lm);
map.put("Ll", Ll);
map.put("C", C);
map.put("M", M);
map.put("L", L);
map.put("N", N);
map.put("P", P);
map.put("S", S);
map.put("Z", Z);
map.put("Me", Me);
map.put("Mc", Mc);
map.put("Mn", Mn);
map.put("Zl", Zl);
map.put("Zp", Zp);
map.put("Zs", Zs);
map.put("Cs", Cs);
map.put("Co", Co);
map.put("Cf", Cf);
map.put("Cc", Cc);
map.put("Po", Po);
map.put("Pi", Pi);
map.put("Pf", Pf);
map.put("Pe", Pe);
map.put("Pd", Pd);
map.put("Pc", Pc);
map.put("Ps", Ps);
map.put("Nd", Nd);
map.put("Nl", Nl);
map.put("No", No);
map.put("So", So);
map.put("Sm", Sm);
map.put("Sk", Sk);
map.put("Sc", Sc);
map.put("Lu", Lu);
map.put("Lt", Lt);
map.put("Lo", Lo);
return map;
}
private static final int[][] _Lm = make_Lm();
private static int[][] make_Lm() {
return new int[][] {
{0x02b0, 0x02c1, 1},
{0x02c6, 0x02d1, 1},
{0x02e0, 0x02e4, 1},
{0x02ec, 0x02ee, 2},
{0x0374, 0x037a, 6},
{0x0559, 0x0640, 231},
{0x06e5, 0x06e6, 1},
{0x07f4, 0x07f5, 1},
{0x07fa, 0x081a, 32},
{0x0824, 0x0828, 4},
{0x0971, 0x0e46, 1237},
{0x0ec6, 0x10fc, 566},
{0x17d7, 0x1843, 108},
{0x1aa7, 0x1c78, 465},
{0x1c79, 0x1c7d, 1},
{0x1d2c, 0x1d61, 1},
{0x1d78, 0x1d9b, 35},
{0x1d9c, 0x1dbf, 1},
{0x2071, 0x207f, 14},
{0x2090, 0x209c, 1},
{0x2c7d, 0x2d6f, 242},
{0x2e2f, 0x3005, 470},
{0x3031, 0x3035, 1},
{0x303b, 0x309d, 98},
{0x309e, 0x30fc, 94},
{0x30fd, 0x30fe, 1},
{0xa015, 0xa4f8, 1251},
{0xa4f9, 0xa4fd, 1},
{0xa60c, 0xa67f, 115},
{0xa717, 0xa71f, 1},
{0xa770, 0xa788, 24},
{0xa9cf, 0xaa70, 161},
{0xaadd, 0xff70, 21651},
{0xff9e, 0xff9f, 1},
};
}
private static final int[][] _Ll = make_Ll();
private static int[][] make_Ll() {
return new int[][] {
{0x0061, 0x007a, 1},
{0x00aa, 0x00b5, 11},
{0x00ba, 0x00df, 37},
{0x00e0, 0x00f6, 1},
{0x00f8, 0x00ff, 1},
{0x0101, 0x0137, 2},
{0x0138, 0x0148, 2},
{0x0149, 0x0177, 2},
{0x017a, 0x017e, 2},
{0x017f, 0x0180, 1},
{0x0183, 0x0185, 2},
{0x0188, 0x018c, 4},
{0x018d, 0x0192, 5},
{0x0195, 0x0199, 4},
{0x019a, 0x019b, 1},
{0x019e, 0x01a1, 3},
{0x01a3, 0x01a5, 2},
{0x01a8, 0x01aa, 2},
{0x01ab, 0x01ad, 2},
{0x01b0, 0x01b4, 4},
{0x01b6, 0x01b9, 3},
{0x01ba, 0x01bd, 3},
{0x01be, 0x01bf, 1},
{0x01c6, 0x01cc, 3},
{0x01ce, 0x01dc, 2},
{0x01dd, 0x01ef, 2},
{0x01f0, 0x01f3, 3},
{0x01f5, 0x01f9, 4},
{0x01fb, 0x0233, 2},
{0x0234, 0x0239, 1},
{0x023c, 0x023f, 3},
{0x0240, 0x0242, 2},
{0x0247, 0x024f, 2},
{0x0250, 0x0293, 1},
{0x0295, 0x02af, 1},
{0x0371, 0x0373, 2},
{0x0377, 0x037b, 4},
{0x037c, 0x037d, 1},
{0x0390, 0x03ac, 28},
{0x03ad, 0x03ce, 1},
{0x03d0, 0x03d1, 1},
{0x03d5, 0x03d7, 1},
{0x03d9, 0x03ef, 2},
{0x03f0, 0x03f3, 1},
{0x03f5, 0x03fb, 3},
{0x03fc, 0x0430, 52},
{0x0431, 0x045f, 1},
{0x0461, 0x0481, 2},
{0x048b, 0x04bf, 2},
{0x04c2, 0x04ce, 2},
{0x04cf, 0x0527, 2},
{0x0561, 0x0587, 1},
{0x1d00, 0x1d2b, 1},
{0x1d62, 0x1d77, 1},
{0x1d79, 0x1d9a, 1},
{0x1e01, 0x1e95, 2},
{0x1e96, 0x1e9d, 1},
{0x1e9f, 0x1eff, 2},
{0x1f00, 0x1f07, 1},
{0x1f10, 0x1f15, 1},
{0x1f20, 0x1f27, 1},
{0x1f30, 0x1f37, 1},
{0x1f40, 0x1f45, 1},
{0x1f50, 0x1f57, 1},
{0x1f60, 0x1f67, 1},
{0x1f70, 0x1f7d, 1},
{0x1f80, 0x1f87, 1},
{0x1f90, 0x1f97, 1},
{0x1fa0, 0x1fa7, 1},
{0x1fb0, 0x1fb4, 1},
{0x1fb6, 0x1fb7, 1},
{0x1fbe, 0x1fc2, 4},
{0x1fc3, 0x1fc4, 1},
{0x1fc6, 0x1fc7, 1},
{0x1fd0, 0x1fd3, 1},
{0x1fd6, 0x1fd7, 1},
{0x1fe0, 0x1fe7, 1},
{0x1ff2, 0x1ff4, 1},
{0x1ff6, 0x1ff7, 1},
{0x210a, 0x210e, 4},
{0x210f, 0x2113, 4},
{0x212f, 0x2139, 5},
{0x213c, 0x213d, 1},
{0x2146, 0x2149, 1},
{0x214e, 0x2184, 54},
{0x2c30, 0x2c5e, 1},
{0x2c61, 0x2c65, 4},
{0x2c66, 0x2c6c, 2},
{0x2c71, 0x2c73, 2},
{0x2c74, 0x2c76, 2},
{0x2c77, 0x2c7c, 1},
{0x2c81, 0x2ce3, 2},
{0x2ce4, 0x2cec, 8},
{0x2cee, 0x2d00, 18},
{0x2d01, 0x2d25, 1},
{0xa641, 0xa66d, 2},
{0xa681, 0xa697, 2},
{0xa723, 0xa72f, 2},
{0xa730, 0xa731, 1},
{0xa733, 0xa771, 2},
{0xa772, 0xa778, 1},
{0xa77a, 0xa77c, 2},
{0xa77f, 0xa787, 2},
{0xa78c, 0xa78e, 2},
{0xa791, 0xa7a1, 16},
{0xa7a3, 0xa7a9, 2},
{0xa7fa, 0xfb00, 21254},
{0xfb01, 0xfb06, 1},
{0xfb13, 0xfb17, 1},
{0xff41, 0xff5a, 1},
{0x10428, 0x1044f, 1},
{0x1d41a, 0x1d433, 1},
{0x1d44e, 0x1d454, 1},
{0x1d456, 0x1d467, 1},
{0x1d482, 0x1d49b, 1},
{0x1d4b6, 0x1d4b9, 1},
{0x1d4bb, 0x1d4bd, 2},
{0x1d4be, 0x1d4c3, 1},
{0x1d4c5, 0x1d4cf, 1},
{0x1d4ea, 0x1d503, 1},
{0x1d51e, 0x1d537, 1},
{0x1d552, 0x1d56b, 1},
{0x1d586, 0x1d59f, 1},
{0x1d5ba, 0x1d5d3, 1},
{0x1d5ee, 0x1d607, 1},
{0x1d622, 0x1d63b, 1},
{0x1d656, 0x1d66f, 1},
{0x1d68a, 0x1d6a5, 1},
{0x1d6c2, 0x1d6da, 1},
{0x1d6dc, 0x1d6e1, 1},
{0x1d6fc, 0x1d714, 1},
{0x1d716, 0x1d71b, 1},
{0x1d736, 0x1d74e, 1},
{0x1d750, 0x1d755, 1},
{0x1d770, 0x1d788, 1},
{0x1d78a, 0x1d78f, 1},
{0x1d7aa, 0x1d7c2, 1},
{0x1d7c4, 0x1d7c9, 1},
{0x1d7cb, 0x1d7cb, 1},
};
}
private static final int[][] _C = make_C();
private static int[][] make_C() {
return new int[][] {
{0x0001, 0x001f, 1},
{0x007f, 0x009f, 1},
{0x00ad, 0x0600, 1363},
{0x0601, 0x0603, 1},
{0x06dd, 0x070f, 50},
{0x17b4, 0x17b5, 1},
{0x200b, 0x200f, 1},
{0x202a, 0x202e, 1},
{0x2060, 0x2064, 1},
{0x206a, 0x206f, 1},
{0xd800, 0xf8ff, 1},
{0xfeff, 0xfff9, 250},
{0xfffa, 0xfffb, 1},
{0x110bd, 0x1d173, 49334},
{0x1d174, 0x1d17a, 1},
{0xe0001, 0xe0020, 31},
{0xe0021, 0xe007f, 1},
{0xf0000, 0xffffd, 1},
{0x100000, 0x10fffd, 1},
};
}
private static final int[][] _M = make_M();
private static int[][] make_M() {
return new int[][] {
{0x0300, 0x036f, 1},
{0x0483, 0x0489, 1},
{0x0591, 0x05bd, 1},
{0x05bf, 0x05c1, 2},
{0x05c2, 0x05c4, 2},
{0x05c5, 0x05c7, 2},
{0x0610, 0x061a, 1},
{0x064b, 0x065f, 1},
{0x0670, 0x06d6, 102},
{0x06d7, 0x06dc, 1},
{0x06df, 0x06e4, 1},
{0x06e7, 0x06e8, 1},
{0x06ea, 0x06ed, 1},
{0x0711, 0x0730, 31},
{0x0731, 0x074a, 1},
{0x07a6, 0x07b0, 1},
{0x07eb, 0x07f3, 1},
{0x0816, 0x0819, 1},
{0x081b, 0x0823, 1},
{0x0825, 0x0827, 1},
{0x0829, 0x082d, 1},
{0x0859, 0x085b, 1},
{0x0900, 0x0903, 1},
{0x093a, 0x093c, 1},
{0x093e, 0x094f, 1},
{0x0951, 0x0957, 1},
{0x0962, 0x0963, 1},
{0x0981, 0x0983, 1},
{0x09bc, 0x09be, 2},
{0x09bf, 0x09c4, 1},
{0x09c7, 0x09c8, 1},
{0x09cb, 0x09cd, 1},
{0x09d7, 0x09e2, 11},
{0x09e3, 0x0a01, 30},
{0x0a02, 0x0a03, 1},
{0x0a3c, 0x0a3e, 2},
{0x0a3f, 0x0a42, 1},
{0x0a47, 0x0a48, 1},
{0x0a4b, 0x0a4d, 1},
{0x0a51, 0x0a70, 31},
{0x0a71, 0x0a75, 4},
{0x0a81, 0x0a83, 1},
{0x0abc, 0x0abe, 2},
{0x0abf, 0x0ac5, 1},
{0x0ac7, 0x0ac9, 1},
{0x0acb, 0x0acd, 1},
{0x0ae2, 0x0ae3, 1},
{0x0b01, 0x0b03, 1},
{0x0b3c, 0x0b3e, 2},
{0x0b3f, 0x0b44, 1},
{0x0b47, 0x0b48, 1},
{0x0b4b, 0x0b4d, 1},
{0x0b56, 0x0b57, 1},
{0x0b62, 0x0b63, 1},
{0x0b82, 0x0bbe, 60},
{0x0bbf, 0x0bc2, 1},
{0x0bc6, 0x0bc8, 1},
{0x0bca, 0x0bcd, 1},
{0x0bd7, 0x0c01, 42},
{0x0c02, 0x0c03, 1},
{0x0c3e, 0x0c44, 1},
{0x0c46, 0x0c48, 1},
{0x0c4a, 0x0c4d, 1},
{0x0c55, 0x0c56, 1},
{0x0c62, 0x0c63, 1},
{0x0c82, 0x0c83, 1},
{0x0cbc, 0x0cbe, 2},
{0x0cbf, 0x0cc4, 1},
{0x0cc6, 0x0cc8, 1},
{0x0cca, 0x0ccd, 1},
{0x0cd5, 0x0cd6, 1},
{0x0ce2, 0x0ce3, 1},
{0x0d02, 0x0d03, 1},
{0x0d3e, 0x0d44, 1},
{0x0d46, 0x0d48, 1},
{0x0d4a, 0x0d4d, 1},
{0x0d57, 0x0d62, 11},
{0x0d63, 0x0d82, 31},
{0x0d83, 0x0dca, 71},
{0x0dcf, 0x0dd4, 1},
{0x0dd6, 0x0dd8, 2},
{0x0dd9, 0x0ddf, 1},
{0x0df2, 0x0df3, 1},
{0x0e31, 0x0e34, 3},
{0x0e35, 0x0e3a, 1},
{0x0e47, 0x0e4e, 1},
{0x0eb1, 0x0eb4, 3},
{0x0eb5, 0x0eb9, 1},
{0x0ebb, 0x0ebc, 1},
{0x0ec8, 0x0ecd, 1},
{0x0f18, 0x0f19, 1},
{0x0f35, 0x0f39, 2},
{0x0f3e, 0x0f3f, 1},
{0x0f71, 0x0f84, 1},
{0x0f86, 0x0f87, 1},
{0x0f8d, 0x0f97, 1},
{0x0f99, 0x0fbc, 1},
{0x0fc6, 0x102b, 101},
{0x102c, 0x103e, 1},
{0x1056, 0x1059, 1},
{0x105e, 0x1060, 1},
{0x1062, 0x1064, 1},
{0x1067, 0x106d, 1},
{0x1071, 0x1074, 1},
{0x1082, 0x108d, 1},
{0x108f, 0x109a, 11},
{0x109b, 0x109d, 1},
{0x135d, 0x135f, 1},
{0x1712, 0x1714, 1},
{0x1732, 0x1734, 1},
{0x1752, 0x1753, 1},
{0x1772, 0x1773, 1},
{0x17b6, 0x17d3, 1},
{0x17dd, 0x180b, 46},
{0x180c, 0x180d, 1},
{0x18a9, 0x1920, 119},
{0x1921, 0x192b, 1},
{0x1930, 0x193b, 1},
{0x19b0, 0x19c0, 1},
{0x19c8, 0x19c9, 1},
{0x1a17, 0x1a1b, 1},
{0x1a55, 0x1a5e, 1},
{0x1a60, 0x1a7c, 1},
{0x1a7f, 0x1b00, 129},
{0x1b01, 0x1b04, 1},
{0x1b34, 0x1b44, 1},
{0x1b6b, 0x1b73, 1},
{0x1b80, 0x1b82, 1},
{0x1ba1, 0x1baa, 1},
{0x1be6, 0x1bf3, 1},
{0x1c24, 0x1c37, 1},
{0x1cd0, 0x1cd2, 1},
{0x1cd4, 0x1ce8, 1},
{0x1ced, 0x1cf2, 5},
{0x1dc0, 0x1de6, 1},
{0x1dfc, 0x1dff, 1},
{0x20d0, 0x20f0, 1},
{0x2cef, 0x2cf1, 1},
{0x2d7f, 0x2de0, 97},
{0x2de1, 0x2dff, 1},
{0x302a, 0x302f, 1},
{0x3099, 0x309a, 1},
{0xa66f, 0xa672, 1},
{0xa67c, 0xa67d, 1},
{0xa6f0, 0xa6f1, 1},
{0xa802, 0xa806, 4},
{0xa80b, 0xa823, 24},
{0xa824, 0xa827, 1},
{0xa880, 0xa881, 1},
{0xa8b4, 0xa8c4, 1},
{0xa8e0, 0xa8f1, 1},
{0xa926, 0xa92d, 1},
{0xa947, 0xa953, 1},
{0xa980, 0xa983, 1},
{0xa9b3, 0xa9c0, 1},
{0xaa29, 0xaa36, 1},
{0xaa43, 0xaa4c, 9},
{0xaa4d, 0xaa7b, 46},
{0xaab0, 0xaab2, 2},
{0xaab3, 0xaab4, 1},
{0xaab7, 0xaab8, 1},
{0xaabe, 0xaabf, 1},
{0xaac1, 0xabe3, 290},
{0xabe4, 0xabea, 1},
{0xabec, 0xabed, 1},
{0xfb1e, 0xfe00, 738},
{0xfe01, 0xfe0f, 1},
{0xfe20, 0xfe26, 1},
{0x101fd, 0x10a01, 2052},
{0x10a02, 0x10a03, 1},
{0x10a05, 0x10a06, 1},
{0x10a0c, 0x10a0f, 1},
{0x10a38, 0x10a3a, 1},
{0x10a3f, 0x11000, 1473},
{0x11001, 0x11002, 1},
{0x11038, 0x11046, 1},
{0x11080, 0x11082, 1},
{0x110b0, 0x110ba, 1},
{0x1d165, 0x1d169, 1},
{0x1d16d, 0x1d172, 1},
{0x1d17b, 0x1d182, 1},
{0x1d185, 0x1d18b, 1},
{0x1d1aa, 0x1d1ad, 1},
{0x1d242, 0x1d244, 1},
{0xe0100, 0xe01ef, 1},
};
}
private static final int[][] _L = make_L();
private static int[][] make_L() {
return new int[][] {
{0x0041, 0x005a, 1},
{0x0061, 0x007a, 1},
{0x00aa, 0x00b5, 11},
{0x00ba, 0x00c0, 6},
{0x00c1, 0x00d6, 1},
{0x00d8, 0x00f6, 1},
{0x00f8, 0x02c1, 1},
{0x02c6, 0x02d1, 1},
{0x02e0, 0x02e4, 1},
{0x02ec, 0x02ee, 2},
{0x0370, 0x0374, 1},
{0x0376, 0x0377, 1},
{0x037a, 0x037d, 1},
{0x0386, 0x0388, 2},
{0x0389, 0x038a, 1},
{0x038c, 0x038e, 2},
{0x038f, 0x03a1, 1},
{0x03a3, 0x03f5, 1},
{0x03f7, 0x0481, 1},
{0x048a, 0x0527, 1},
{0x0531, 0x0556, 1},
{0x0559, 0x0561, 8},
{0x0562, 0x0587, 1},
{0x05d0, 0x05ea, 1},
{0x05f0, 0x05f2, 1},
{0x0620, 0x064a, 1},
{0x066e, 0x066f, 1},
{0x0671, 0x06d3, 1},
{0x06d5, 0x06e5, 16},
{0x06e6, 0x06ee, 8},
{0x06ef, 0x06fa, 11},
{0x06fb, 0x06fc, 1},
{0x06ff, 0x0710, 17},
{0x0712, 0x072f, 1},
{0x074d, 0x07a5, 1},
{0x07b1, 0x07ca, 25},
{0x07cb, 0x07ea, 1},
{0x07f4, 0x07f5, 1},
{0x07fa, 0x0800, 6},
{0x0801, 0x0815, 1},
{0x081a, 0x0824, 10},
{0x0828, 0x0840, 24},
{0x0841, 0x0858, 1},
{0x0904, 0x0939, 1},
{0x093d, 0x0950, 19},
{0x0958, 0x0961, 1},
{0x0971, 0x0977, 1},
{0x0979, 0x097f, 1},
{0x0985, 0x098c, 1},
{0x098f, 0x0990, 1},
{0x0993, 0x09a8, 1},
{0x09aa, 0x09b0, 1},
{0x09b2, 0x09b6, 4},
{0x09b7, 0x09b9, 1},
{0x09bd, 0x09ce, 17},
{0x09dc, 0x09dd, 1},
{0x09df, 0x09e1, 1},
{0x09f0, 0x09f1, 1},
{0x0a05, 0x0a0a, 1},
{0x0a0f, 0x0a10, 1},
{0x0a13, 0x0a28, 1},
{0x0a2a, 0x0a30, 1},
{0x0a32, 0x0a33, 1},
{0x0a35, 0x0a36, 1},
{0x0a38, 0x0a39, 1},
{0x0a59, 0x0a5c, 1},
{0x0a5e, 0x0a72, 20},
{0x0a73, 0x0a74, 1},
{0x0a85, 0x0a8d, 1},
{0x0a8f, 0x0a91, 1},
{0x0a93, 0x0aa8, 1},
{0x0aaa, 0x0ab0, 1},
{0x0ab2, 0x0ab3, 1},
{0x0ab5, 0x0ab9, 1},
{0x0abd, 0x0ad0, 19},
{0x0ae0, 0x0ae1, 1},
{0x0b05, 0x0b0c, 1},
{0x0b0f, 0x0b10, 1},
{0x0b13, 0x0b28, 1},
{0x0b2a, 0x0b30, 1},
{0x0b32, 0x0b33, 1},
{0x0b35, 0x0b39, 1},
{0x0b3d, 0x0b5c, 31},
{0x0b5d, 0x0b5f, 2},
{0x0b60, 0x0b61, 1},
{0x0b71, 0x0b83, 18},
{0x0b85, 0x0b8a, 1},
{0x0b8e, 0x0b90, 1},
{0x0b92, 0x0b95, 1},
{0x0b99, 0x0b9a, 1},
{0x0b9c, 0x0b9e, 2},
{0x0b9f, 0x0ba3, 4},
{0x0ba4, 0x0ba8, 4},
{0x0ba9, 0x0baa, 1},
{0x0bae, 0x0bb9, 1},
{0x0bd0, 0x0c05, 53},
{0x0c06, 0x0c0c, 1},
{0x0c0e, 0x0c10, 1},
{0x0c12, 0x0c28, 1},
{0x0c2a, 0x0c33, 1},
{0x0c35, 0x0c39, 1},
{0x0c3d, 0x0c58, 27},
{0x0c59, 0x0c60, 7},
{0x0c61, 0x0c85, 36},
{0x0c86, 0x0c8c, 1},
{0x0c8e, 0x0c90, 1},
{0x0c92, 0x0ca8, 1},
{0x0caa, 0x0cb3, 1},
{0x0cb5, 0x0cb9, 1},
{0x0cbd, 0x0cde, 33},
{0x0ce0, 0x0ce1, 1},
{0x0cf1, 0x0cf2, 1},
{0x0d05, 0x0d0c, 1},
{0x0d0e, 0x0d10, 1},
{0x0d12, 0x0d3a, 1},
{0x0d3d, 0x0d4e, 17},
{0x0d60, 0x0d61, 1},
{0x0d7a, 0x0d7f, 1},
{0x0d85, 0x0d96, 1},
{0x0d9a, 0x0db1, 1},
{0x0db3, 0x0dbb, 1},
{0x0dbd, 0x0dc0, 3},
{0x0dc1, 0x0dc6, 1},
{0x0e01, 0x0e30, 1},
{0x0e32, 0x0e33, 1},
{0x0e40, 0x0e46, 1},
{0x0e81, 0x0e82, 1},
{0x0e84, 0x0e87, 3},
{0x0e88, 0x0e8a, 2},
{0x0e8d, 0x0e94, 7},
{0x0e95, 0x0e97, 1},
{0x0e99, 0x0e9f, 1},
{0x0ea1, 0x0ea3, 1},
{0x0ea5, 0x0ea7, 2},
{0x0eaa, 0x0eab, 1},
{0x0ead, 0x0eb0, 1},
{0x0eb2, 0x0eb3, 1},
{0x0ebd, 0x0ec0, 3},
{0x0ec1, 0x0ec4, 1},
{0x0ec6, 0x0edc, 22},
{0x0edd, 0x0f00, 35},
{0x0f40, 0x0f47, 1},
{0x0f49, 0x0f6c, 1},
{0x0f88, 0x0f8c, 1},
{0x1000, 0x102a, 1},
{0x103f, 0x1050, 17},
{0x1051, 0x1055, 1},
{0x105a, 0x105d, 1},
{0x1061, 0x1065, 4},
{0x1066, 0x106e, 8},
{0x106f, 0x1070, 1},
{0x1075, 0x1081, 1},
{0x108e, 0x10a0, 18},
{0x10a1, 0x10c5, 1},
{0x10d0, 0x10fa, 1},
{0x10fc, 0x1100, 4},
{0x1101, 0x1248, 1},
{0x124a, 0x124d, 1},
{0x1250, 0x1256, 1},
{0x1258, 0x125a, 2},
{0x125b, 0x125d, 1},
{0x1260, 0x1288, 1},
{0x128a, 0x128d, 1},
{0x1290, 0x12b0, 1},
{0x12b2, 0x12b5, 1},
{0x12b8, 0x12be, 1},
{0x12c0, 0x12c2, 2},
{0x12c3, 0x12c5, 1},
{0x12c8, 0x12d6, 1},
{0x12d8, 0x1310, 1},
{0x1312, 0x1315, 1},
{0x1318, 0x135a, 1},
{0x1380, 0x138f, 1},
{0x13a0, 0x13f4, 1},
{0x1401, 0x166c, 1},
{0x166f, 0x167f, 1},
{0x1681, 0x169a, 1},
{0x16a0, 0x16ea, 1},
{0x1700, 0x170c, 1},
{0x170e, 0x1711, 1},
{0x1720, 0x1731, 1},
{0x1740, 0x1751, 1},
{0x1760, 0x176c, 1},
{0x176e, 0x1770, 1},
{0x1780, 0x17b3, 1},
{0x17d7, 0x17dc, 5},
{0x1820, 0x1877, 1},
{0x1880, 0x18a8, 1},
{0x18aa, 0x18b0, 6},
{0x18b1, 0x18f5, 1},
{0x1900, 0x191c, 1},
{0x1950, 0x196d, 1},
{0x1970, 0x1974, 1},
{0x1980, 0x19ab, 1},
{0x19c1, 0x19c7, 1},
{0x1a00, 0x1a16, 1},
{0x1a20, 0x1a54, 1},
{0x1aa7, 0x1b05, 94},
{0x1b06, 0x1b33, 1},
{0x1b45, 0x1b4b, 1},
{0x1b83, 0x1ba0, 1},
{0x1bae, 0x1baf, 1},
{0x1bc0, 0x1be5, 1},
{0x1c00, 0x1c23, 1},
{0x1c4d, 0x1c4f, 1},
{0x1c5a, 0x1c7d, 1},
{0x1ce9, 0x1cec, 1},
{0x1cee, 0x1cf1, 1},
{0x1d00, 0x1dbf, 1},
{0x1e00, 0x1f15, 1},
{0x1f18, 0x1f1d, 1},
{0x1f20, 0x1f45, 1},
{0x1f48, 0x1f4d, 1},
{0x1f50, 0x1f57, 1},
{0x1f59, 0x1f5f, 2},
{0x1f60, 0x1f7d, 1},
{0x1f80, 0x1fb4, 1},
{0x1fb6, 0x1fbc, 1},
{0x1fbe, 0x1fc2, 4},
{0x1fc3, 0x1fc4, 1},
{0x1fc6, 0x1fcc, 1},
{0x1fd0, 0x1fd3, 1},
{0x1fd6, 0x1fdb, 1},
{0x1fe0, 0x1fec, 1},
{0x1ff2, 0x1ff4, 1},
{0x1ff6, 0x1ffc, 1},
{0x2071, 0x207f, 14},
{0x2090, 0x209c, 1},
{0x2102, 0x2107, 5},
{0x210a, 0x2113, 1},
{0x2115, 0x2119, 4},
{0x211a, 0x211d, 1},
{0x2124, 0x212a, 2},
{0x212b, 0x212d, 1},
{0x212f, 0x2139, 1},
{0x213c, 0x213f, 1},
{0x2145, 0x2149, 1},
{0x214e, 0x2183, 53},
{0x2184, 0x2c00, 2684},
{0x2c01, 0x2c2e, 1},
{0x2c30, 0x2c5e, 1},
{0x2c60, 0x2ce4, 1},
{0x2ceb, 0x2cee, 1},
{0x2d00, 0x2d25, 1},
{0x2d30, 0x2d65, 1},
{0x2d6f, 0x2d80, 17},
{0x2d81, 0x2d96, 1},
{0x2da0, 0x2da6, 1},
{0x2da8, 0x2dae, 1},
{0x2db0, 0x2db6, 1},
{0x2db8, 0x2dbe, 1},
{0x2dc0, 0x2dc6, 1},
{0x2dc8, 0x2dce, 1},
{0x2dd0, 0x2dd6, 1},
{0x2dd8, 0x2dde, 1},
{0x2e2f, 0x3005, 470},
{0x3006, 0x3031, 43},
{0x3032, 0x3035, 1},
{0x303b, 0x303c, 1},
{0x3041, 0x3096, 1},
{0x309d, 0x309f, 1},
{0x30a1, 0x30fa, 1},
{0x30fc, 0x30ff, 1},
{0x3105, 0x312d, 1},
{0x3131, 0x318e, 1},
{0x31a0, 0x31ba, 1},
{0x31f0, 0x31ff, 1},
{0x3400, 0x4db5, 1},
{0x4e00, 0x9fcb, 1},
{0xa000, 0xa48c, 1},
{0xa4d0, 0xa4fd, 1},
{0xa500, 0xa60c, 1},
{0xa610, 0xa61f, 1},
{0xa62a, 0xa62b, 1},
{0xa640, 0xa66e, 1},
{0xa67f, 0xa697, 1},
{0xa6a0, 0xa6e5, 1},
{0xa717, 0xa71f, 1},
{0xa722, 0xa788, 1},
{0xa78b, 0xa78e, 1},
{0xa790, 0xa791, 1},
{0xa7a0, 0xa7a9, 1},
{0xa7fa, 0xa801, 1},
{0xa803, 0xa805, 1},
{0xa807, 0xa80a, 1},
{0xa80c, 0xa822, 1},
{0xa840, 0xa873, 1},
{0xa882, 0xa8b3, 1},
{0xa8f2, 0xa8f7, 1},
{0xa8fb, 0xa90a, 15},
{0xa90b, 0xa925, 1},
{0xa930, 0xa946, 1},
{0xa960, 0xa97c, 1},
{0xa984, 0xa9b2, 1},
{0xa9cf, 0xaa00, 49},
{0xaa01, 0xaa28, 1},
{0xaa40, 0xaa42, 1},
{0xaa44, 0xaa4b, 1},
{0xaa60, 0xaa76, 1},
{0xaa7a, 0xaa80, 6},
{0xaa81, 0xaaaf, 1},
{0xaab1, 0xaab5, 4},
{0xaab6, 0xaab9, 3},
{0xaaba, 0xaabd, 1},
{0xaac0, 0xaac2, 2},
{0xaadb, 0xaadd, 1},
{0xab01, 0xab06, 1},
{0xab09, 0xab0e, 1},
{0xab11, 0xab16, 1},
{0xab20, 0xab26, 1},
{0xab28, 0xab2e, 1},
{0xabc0, 0xabe2, 1},
{0xac00, 0xd7a3, 1},
{0xd7b0, 0xd7c6, 1},
{0xd7cb, 0xd7fb, 1},
{0xf900, 0xfa2d, 1},
{0xfa30, 0xfa6d, 1},
{0xfa70, 0xfad9, 1},
{0xfb00, 0xfb06, 1},
{0xfb13, 0xfb17, 1},
{0xfb1d, 0xfb1f, 2},
{0xfb20, 0xfb28, 1},
{0xfb2a, 0xfb36, 1},
{0xfb38, 0xfb3c, 1},
{0xfb3e, 0xfb40, 2},
{0xfb41, 0xfb43, 2},
{0xfb44, 0xfb46, 2},
{0xfb47, 0xfbb1, 1},
{0xfbd3, 0xfd3d, 1},
{0xfd50, 0xfd8f, 1},
{0xfd92, 0xfdc7, 1},
{0xfdf0, 0xfdfb, 1},
{0xfe70, 0xfe74, 1},
{0xfe76, 0xfefc, 1},
{0xff21, 0xff3a, 1},
{0xff41, 0xff5a, 1},
{0xff66, 0xffbe, 1},
{0xffc2, 0xffc7, 1},
{0xffca, 0xffcf, 1},
{0xffd2, 0xffd7, 1},
{0xffda, 0xffdc, 1},
{0x10000, 0x1000b, 1},
{0x1000d, 0x10026, 1},
{0x10028, 0x1003a, 1},
{0x1003c, 0x1003d, 1},
{0x1003f, 0x1004d, 1},
{0x10050, 0x1005d, 1},
{0x10080, 0x100fa, 1},
{0x10280, 0x1029c, 1},
{0x102a0, 0x102d0, 1},
{0x10300, 0x1031e, 1},
{0x10330, 0x10340, 1},
{0x10342, 0x10349, 1},
{0x10380, 0x1039d, 1},
{0x103a0, 0x103c3, 1},
{0x103c8, 0x103cf, 1},
{0x10400, 0x1049d, 1},
{0x10800, 0x10805, 1},
{0x10808, 0x1080a, 2},
{0x1080b, 0x10835, 1},
{0x10837, 0x10838, 1},
{0x1083c, 0x1083f, 3},
{0x10840, 0x10855, 1},
{0x10900, 0x10915, 1},
{0x10920, 0x10939, 1},
{0x10a00, 0x10a10, 16},
{0x10a11, 0x10a13, 1},
{0x10a15, 0x10a17, 1},
{0x10a19, 0x10a33, 1},
{0x10a60, 0x10a7c, 1},
{0x10b00, 0x10b35, 1},
{0x10b40, 0x10b55, 1},
{0x10b60, 0x10b72, 1},
{0x10c00, 0x10c48, 1},
{0x11003, 0x11037, 1},
{0x11083, 0x110af, 1},
{0x12000, 0x1236e, 1},
{0x13000, 0x1342e, 1},
{0x16800, 0x16a38, 1},
{0x1b000, 0x1b001, 1},
{0x1d400, 0x1d454, 1},
{0x1d456, 0x1d49c, 1},
{0x1d49e, 0x1d49f, 1},
{0x1d4a2, 0x1d4a5, 3},
{0x1d4a6, 0x1d4a9, 3},
{0x1d4aa, 0x1d4ac, 1},
{0x1d4ae, 0x1d4b9, 1},
{0x1d4bb, 0x1d4bd, 2},
{0x1d4be, 0x1d4c3, 1},
{0x1d4c5, 0x1d505, 1},
{0x1d507, 0x1d50a, 1},
{0x1d50d, 0x1d514, 1},
{0x1d516, 0x1d51c, 1},
{0x1d51e, 0x1d539, 1},
{0x1d53b, 0x1d53e, 1},
{0x1d540, 0x1d544, 1},
{0x1d546, 0x1d54a, 4},
{0x1d54b, 0x1d550, 1},
{0x1d552, 0x1d6a5, 1},
{0x1d6a8, 0x1d6c0, 1},
{0x1d6c2, 0x1d6da, 1},
{0x1d6dc, 0x1d6fa, 1},
{0x1d6fc, 0x1d714, 1},
{0x1d716, 0x1d734, 1},
{0x1d736, 0x1d74e, 1},
{0x1d750, 0x1d76e, 1},
{0x1d770, 0x1d788, 1},
{0x1d78a, 0x1d7a8, 1},
{0x1d7aa, 0x1d7c2, 1},
{0x1d7c4, 0x1d7cb, 1},
{0x20000, 0x2a6d6, 1},
{0x2a700, 0x2b734, 1},
{0x2b740, 0x2b81d, 1},
{0x2f800, 0x2fa1d, 1},
};
}
private static final int[][] _N = make_N();
private static int[][] make_N() {
return new int[][] {
{0x0030, 0x0039, 1},
{0x00b2, 0x00b3, 1},
{0x00b9, 0x00bc, 3},
{0x00bd, 0x00be, 1},
{0x0660, 0x0669, 1},
{0x06f0, 0x06f9, 1},
{0x07c0, 0x07c9, 1},
{0x0966, 0x096f, 1},
{0x09e6, 0x09ef, 1},
{0x09f4, 0x09f9, 1},
{0x0a66, 0x0a6f, 1},
{0x0ae6, 0x0aef, 1},
{0x0b66, 0x0b6f, 1},
{0x0b72, 0x0b77, 1},
{0x0be6, 0x0bf2, 1},
{0x0c66, 0x0c6f, 1},
{0x0c78, 0x0c7e, 1},
{0x0ce6, 0x0cef, 1},
{0x0d66, 0x0d75, 1},
{0x0e50, 0x0e59, 1},
{0x0ed0, 0x0ed9, 1},
{0x0f20, 0x0f33, 1},
{0x1040, 0x1049, 1},
{0x1090, 0x1099, 1},
{0x1369, 0x137c, 1},
{0x16ee, 0x16f0, 1},
{0x17e0, 0x17e9, 1},
{0x17f0, 0x17f9, 1},
{0x1810, 0x1819, 1},
{0x1946, 0x194f, 1},
{0x19d0, 0x19da, 1},
{0x1a80, 0x1a89, 1},
{0x1a90, 0x1a99, 1},
{0x1b50, 0x1b59, 1},
{0x1bb0, 0x1bb9, 1},
{0x1c40, 0x1c49, 1},
{0x1c50, 0x1c59, 1},
{0x2070, 0x2074, 4},
{0x2075, 0x2079, 1},
{0x2080, 0x2089, 1},
{0x2150, 0x2182, 1},
{0x2185, 0x2189, 1},
{0x2460, 0x249b, 1},
{0x24ea, 0x24ff, 1},
{0x2776, 0x2793, 1},
{0x2cfd, 0x3007, 778},
{0x3021, 0x3029, 1},
{0x3038, 0x303a, 1},
{0x3192, 0x3195, 1},
{0x3220, 0x3229, 1},
{0x3251, 0x325f, 1},
{0x3280, 0x3289, 1},
{0x32b1, 0x32bf, 1},
{0xa620, 0xa629, 1},
{0xa6e6, 0xa6ef, 1},
{0xa830, 0xa835, 1},
{0xa8d0, 0xa8d9, 1},
{0xa900, 0xa909, 1},
{0xa9d0, 0xa9d9, 1},
{0xaa50, 0xaa59, 1},
{0xabf0, 0xabf9, 1},
{0xff10, 0xff19, 1},
{0x10107, 0x10133, 1},
{0x10140, 0x10178, 1},
{0x1018a, 0x10320, 406},
{0x10321, 0x10323, 1},
{0x10341, 0x1034a, 9},
{0x103d1, 0x103d5, 1},
{0x104a0, 0x104a9, 1},
{0x10858, 0x1085f, 1},
{0x10916, 0x1091b, 1},
{0x10a40, 0x10a47, 1},
{0x10a7d, 0x10a7e, 1},
{0x10b58, 0x10b5f, 1},
{0x10b78, 0x10b7f, 1},
{0x10e60, 0x10e7e, 1},
{0x11052, 0x1106f, 1},
{0x12400, 0x12462, 1},
{0x1d360, 0x1d371, 1},
{0x1d7ce, 0x1d7ff, 1},
{0x1f100, 0x1f10a, 1},
};
}
private static final int[][] _P = make_P();
private static int[][] make_P() {
return new int[][] {
{0x0021, 0x0023, 1},
{0x0025, 0x002a, 1},
{0x002c, 0x002f, 1},
{0x003a, 0x003b, 1},
{0x003f, 0x0040, 1},
{0x005b, 0x005d, 1},
{0x005f, 0x007b, 28},
{0x007d, 0x00a1, 36},
{0x00ab, 0x00b7, 12},
{0x00bb, 0x00bf, 4},
{0x037e, 0x0387, 9},
{0x055a, 0x055f, 1},
{0x0589, 0x058a, 1},
{0x05be, 0x05c0, 2},
{0x05c3, 0x05c6, 3},
{0x05f3, 0x05f4, 1},
{0x0609, 0x060a, 1},
{0x060c, 0x060d, 1},
{0x061b, 0x061e, 3},
{0x061f, 0x066a, 75},
{0x066b, 0x066d, 1},
{0x06d4, 0x0700, 44},
{0x0701, 0x070d, 1},
{0x07f7, 0x07f9, 1},
{0x0830, 0x083e, 1},
{0x085e, 0x0964, 262},
{0x0965, 0x0970, 11},
{0x0df4, 0x0e4f, 91},
{0x0e5a, 0x0e5b, 1},
{0x0f04, 0x0f12, 1},
{0x0f3a, 0x0f3d, 1},
{0x0f85, 0x0fd0, 75},
{0x0fd1, 0x0fd4, 1},
{0x0fd9, 0x0fda, 1},
{0x104a, 0x104f, 1},
{0x10fb, 0x1361, 614},
{0x1362, 0x1368, 1},
{0x1400, 0x166d, 621},
{0x166e, 0x169b, 45},
{0x169c, 0x16eb, 79},
{0x16ec, 0x16ed, 1},
{0x1735, 0x1736, 1},
{0x17d4, 0x17d6, 1},
{0x17d8, 0x17da, 1},
{0x1800, 0x180a, 1},
{0x1944, 0x1945, 1},
{0x1a1e, 0x1a1f, 1},
{0x1aa0, 0x1aa6, 1},
{0x1aa8, 0x1aad, 1},
{0x1b5a, 0x1b60, 1},
{0x1bfc, 0x1bff, 1},
{0x1c3b, 0x1c3f, 1},
{0x1c7e, 0x1c7f, 1},
{0x1cd3, 0x2010, 829},
{0x2011, 0x2027, 1},
{0x2030, 0x2043, 1},
{0x2045, 0x2051, 1},
{0x2053, 0x205e, 1},
{0x207d, 0x207e, 1},
{0x208d, 0x208e, 1},
{0x2329, 0x232a, 1},
{0x2768, 0x2775, 1},
{0x27c5, 0x27c6, 1},
{0x27e6, 0x27ef, 1},
{0x2983, 0x2998, 1},
{0x29d8, 0x29db, 1},
{0x29fc, 0x29fd, 1},
{0x2cf9, 0x2cfc, 1},
{0x2cfe, 0x2cff, 1},
{0x2d70, 0x2e00, 144},
{0x2e01, 0x2e2e, 1},
{0x2e30, 0x2e31, 1},
{0x3001, 0x3003, 1},
{0x3008, 0x3011, 1},
{0x3014, 0x301f, 1},
{0x3030, 0x303d, 13},
{0x30a0, 0x30fb, 91},
{0xa4fe, 0xa4ff, 1},
{0xa60d, 0xa60f, 1},
{0xa673, 0xa67e, 11},
{0xa6f2, 0xa6f7, 1},
{0xa874, 0xa877, 1},
{0xa8ce, 0xa8cf, 1},
{0xa8f8, 0xa8fa, 1},
{0xa92e, 0xa92f, 1},
{0xa95f, 0xa9c1, 98},
{0xa9c2, 0xa9cd, 1},
{0xa9de, 0xa9df, 1},
{0xaa5c, 0xaa5f, 1},
{0xaade, 0xaadf, 1},
{0xabeb, 0xfd3e, 20819},
{0xfd3f, 0xfe10, 209},
{0xfe11, 0xfe19, 1},
{0xfe30, 0xfe52, 1},
{0xfe54, 0xfe61, 1},
{0xfe63, 0xfe68, 5},
{0xfe6a, 0xfe6b, 1},
{0xff01, 0xff03, 1},
{0xff05, 0xff0a, 1},
{0xff0c, 0xff0f, 1},
{0xff1a, 0xff1b, 1},
{0xff1f, 0xff20, 1},
{0xff3b, 0xff3d, 1},
{0xff3f, 0xff5b, 28},
{0xff5d, 0xff5f, 2},
{0xff60, 0xff65, 1},
{0x10100, 0x10101, 1},
{0x1039f, 0x103d0, 49},
{0x10857, 0x1091f, 200},
{0x1093f, 0x10a50, 273},
{0x10a51, 0x10a58, 1},
{0x10a7f, 0x10b39, 186},
{0x10b3a, 0x10b3f, 1},
{0x11047, 0x1104d, 1},
{0x110bb, 0x110bc, 1},
{0x110be, 0x110c1, 1},
{0x12470, 0x12473, 1},
};
}
private static final int[][] _S = make_S();
private static int[][] make_S() {
return new int[][] {
{0x0024, 0x002b, 7},
{0x003c, 0x003e, 1},
{0x005e, 0x0060, 2},
{0x007c, 0x007e, 2},
{0x00a2, 0x00a9, 1},
{0x00ac, 0x00ae, 2},
{0x00af, 0x00b1, 1},
{0x00b4, 0x00b8, 2},
{0x00d7, 0x00f7, 32},
{0x02c2, 0x02c5, 1},
{0x02d2, 0x02df, 1},
{0x02e5, 0x02eb, 1},
{0x02ed, 0x02ef, 2},
{0x02f0, 0x02ff, 1},
{0x0375, 0x0384, 15},
{0x0385, 0x03f6, 113},
{0x0482, 0x0606, 388},
{0x0607, 0x0608, 1},
{0x060b, 0x060e, 3},
{0x060f, 0x06de, 207},
{0x06e9, 0x06fd, 20},
{0x06fe, 0x07f6, 248},
{0x09f2, 0x09f3, 1},
{0x09fa, 0x09fb, 1},
{0x0af1, 0x0b70, 127},
{0x0bf3, 0x0bfa, 1},
{0x0c7f, 0x0d79, 250},
{0x0e3f, 0x0f01, 194},
{0x0f02, 0x0f03, 1},
{0x0f13, 0x0f17, 1},
{0x0f1a, 0x0f1f, 1},
{0x0f34, 0x0f38, 2},
{0x0fbe, 0x0fc5, 1},
{0x0fc7, 0x0fcc, 1},
{0x0fce, 0x0fcf, 1},
{0x0fd5, 0x0fd8, 1},
{0x109e, 0x109f, 1},
{0x1360, 0x1390, 48},
{0x1391, 0x1399, 1},
{0x17db, 0x1940, 357},
{0x19de, 0x19ff, 1},
{0x1b61, 0x1b6a, 1},
{0x1b74, 0x1b7c, 1},
{0x1fbd, 0x1fbf, 2},
{0x1fc0, 0x1fc1, 1},
{0x1fcd, 0x1fcf, 1},
{0x1fdd, 0x1fdf, 1},
{0x1fed, 0x1fef, 1},
{0x1ffd, 0x1ffe, 1},
{0x2044, 0x2052, 14},
{0x207a, 0x207c, 1},
{0x208a, 0x208c, 1},
{0x20a0, 0x20b9, 1},
{0x2100, 0x2101, 1},
{0x2103, 0x2106, 1},
{0x2108, 0x2109, 1},
{0x2114, 0x2116, 2},
{0x2117, 0x2118, 1},
{0x211e, 0x2123, 1},
{0x2125, 0x2129, 2},
{0x212e, 0x213a, 12},
{0x213b, 0x2140, 5},
{0x2141, 0x2144, 1},
{0x214a, 0x214d, 1},
{0x214f, 0x2190, 65},
{0x2191, 0x2328, 1},
{0x232b, 0x23f3, 1},
{0x2400, 0x2426, 1},
{0x2440, 0x244a, 1},
{0x249c, 0x24e9, 1},
{0x2500, 0x26ff, 1},
{0x2701, 0x2767, 1},
{0x2794, 0x27c4, 1},
{0x27c7, 0x27ca, 1},
{0x27cc, 0x27ce, 2},
{0x27cf, 0x27e5, 1},
{0x27f0, 0x2982, 1},
{0x2999, 0x29d7, 1},
{0x29dc, 0x29fb, 1},
{0x29fe, 0x2b4c, 1},
{0x2b50, 0x2b59, 1},
{0x2ce5, 0x2cea, 1},
{0x2e80, 0x2e99, 1},
{0x2e9b, 0x2ef3, 1},
{0x2f00, 0x2fd5, 1},
{0x2ff0, 0x2ffb, 1},
{0x3004, 0x3012, 14},
{0x3013, 0x3020, 13},
{0x3036, 0x3037, 1},
{0x303e, 0x303f, 1},
{0x309b, 0x309c, 1},
{0x3190, 0x3191, 1},
{0x3196, 0x319f, 1},
{0x31c0, 0x31e3, 1},
{0x3200, 0x321e, 1},
{0x322a, 0x3250, 1},
{0x3260, 0x327f, 1},
{0x328a, 0x32b0, 1},
{0x32c0, 0x32fe, 1},
{0x3300, 0x33ff, 1},
{0x4dc0, 0x4dff, 1},
{0xa490, 0xa4c6, 1},
{0xa700, 0xa716, 1},
{0xa720, 0xa721, 1},
{0xa789, 0xa78a, 1},
{0xa828, 0xa82b, 1},
{0xa836, 0xa839, 1},
{0xaa77, 0xaa79, 1},
{0xfb29, 0xfbb2, 137},
{0xfbb3, 0xfbc1, 1},
{0xfdfc, 0xfdfd, 1},
{0xfe62, 0xfe64, 2},
{0xfe65, 0xfe66, 1},
{0xfe69, 0xff04, 155},
{0xff0b, 0xff1c, 17},
{0xff1d, 0xff1e, 1},
{0xff3e, 0xff40, 2},
{0xff5c, 0xff5e, 2},
{0xffe0, 0xffe6, 1},
{0xffe8, 0xffee, 1},
{0xfffc, 0xfffd, 1},
{0x10102, 0x10137, 53},
{0x10138, 0x1013f, 1},
{0x10179, 0x10189, 1},
{0x10190, 0x1019b, 1},
{0x101d0, 0x101fc, 1},
{0x1d000, 0x1d0f5, 1},
{0x1d100, 0x1d126, 1},
{0x1d129, 0x1d164, 1},
{0x1d16a, 0x1d16c, 1},
{0x1d183, 0x1d184, 1},
{0x1d18c, 0x1d1a9, 1},
{0x1d1ae, 0x1d1dd, 1},
{0x1d200, 0x1d241, 1},
{0x1d245, 0x1d300, 187},
{0x1d301, 0x1d356, 1},
{0x1d6c1, 0x1d6db, 26},
{0x1d6fb, 0x1d715, 26},
{0x1d735, 0x1d74f, 26},
{0x1d76f, 0x1d789, 26},
{0x1d7a9, 0x1d7c3, 26},
{0x1f000, 0x1f02b, 1},
{0x1f030, 0x1f093, 1},
{0x1f0a0, 0x1f0ae, 1},
{0x1f0b1, 0x1f0be, 1},
{0x1f0c1, 0x1f0cf, 1},
{0x1f0d1, 0x1f0df, 1},
{0x1f110, 0x1f12e, 1},
{0x1f130, 0x1f169, 1},
{0x1f170, 0x1f19a, 1},
{0x1f1e6, 0x1f202, 1},
{0x1f210, 0x1f23a, 1},
{0x1f240, 0x1f248, 1},
{0x1f250, 0x1f251, 1},
{0x1f300, 0x1f320, 1},
{0x1f330, 0x1f335, 1},
{0x1f337, 0x1f37c, 1},
{0x1f380, 0x1f393, 1},
{0x1f3a0, 0x1f3c4, 1},
{0x1f3c6, 0x1f3ca, 1},
{0x1f3e0, 0x1f3f0, 1},
{0x1f400, 0x1f43e, 1},
{0x1f440, 0x1f442, 2},
{0x1f443, 0x1f4f7, 1},
{0x1f4f9, 0x1f4fc, 1},
{0x1f500, 0x1f53d, 1},
{0x1f550, 0x1f567, 1},
{0x1f5fb, 0x1f5ff, 1},
{0x1f601, 0x1f610, 1},
{0x1f612, 0x1f614, 1},
{0x1f616, 0x1f61c, 2},
{0x1f61d, 0x1f61e, 1},
{0x1f620, 0x1f625, 1},
{0x1f628, 0x1f62b, 1},
{0x1f62d, 0x1f630, 3},
{0x1f631, 0x1f633, 1},
{0x1f635, 0x1f640, 1},
{0x1f645, 0x1f64f, 1},
{0x1f680, 0x1f6c5, 1},
{0x1f700, 0x1f773, 1},
};
}
private static final int[][] _Z = make_Z();
private static int[][] make_Z() {
return new int[][] {
{0x0020, 0x00a0, 128},
{0x1680, 0x180e, 398},
{0x2000, 0x200a, 1},
{0x2028, 0x2029, 1},
{0x202f, 0x205f, 48},
{0x3000, 0x3000, 1},
};
}
private static final int[][] _Me = make_Me();
private static int[][] make_Me() {
return new int[][] {
{0x0488, 0x0489, 1},
{0x20dd, 0x20e0, 1},
{0x20e2, 0x20e4, 1},
{0xa670, 0xa672, 1},
};
}
private static final int[][] _Mc = make_Mc();
private static int[][] make_Mc() {
return new int[][] {
{0x0903, 0x093b, 56},
{0x093e, 0x0940, 1},
{0x0949, 0x094c, 1},
{0x094e, 0x094f, 1},
{0x0982, 0x0983, 1},
{0x09be, 0x09c0, 1},
{0x09c7, 0x09c8, 1},
{0x09cb, 0x09cc, 1},
{0x09d7, 0x0a03, 44},
{0x0a3e, 0x0a40, 1},
{0x0a83, 0x0abe, 59},
{0x0abf, 0x0ac0, 1},
{0x0ac9, 0x0acb, 2},
{0x0acc, 0x0b02, 54},
{0x0b03, 0x0b3e, 59},
{0x0b40, 0x0b47, 7},
{0x0b48, 0x0b4b, 3},
{0x0b4c, 0x0b57, 11},
{0x0bbe, 0x0bbf, 1},
{0x0bc1, 0x0bc2, 1},
{0x0bc6, 0x0bc8, 1},
{0x0bca, 0x0bcc, 1},
{0x0bd7, 0x0c01, 42},
{0x0c02, 0x0c03, 1},
{0x0c41, 0x0c44, 1},
{0x0c82, 0x0c83, 1},
{0x0cbe, 0x0cc0, 2},
{0x0cc1, 0x0cc4, 1},
{0x0cc7, 0x0cc8, 1},
{0x0cca, 0x0ccb, 1},
{0x0cd5, 0x0cd6, 1},
{0x0d02, 0x0d03, 1},
{0x0d3e, 0x0d40, 1},
{0x0d46, 0x0d48, 1},
{0x0d4a, 0x0d4c, 1},
{0x0d57, 0x0d82, 43},
{0x0d83, 0x0dcf, 76},
{0x0dd0, 0x0dd1, 1},
{0x0dd8, 0x0ddf, 1},
{0x0df2, 0x0df3, 1},
{0x0f3e, 0x0f3f, 1},
{0x0f7f, 0x102b, 172},
{0x102c, 0x1031, 5},
{0x1038, 0x103b, 3},
{0x103c, 0x1056, 26},
{0x1057, 0x1062, 11},
{0x1063, 0x1064, 1},
{0x1067, 0x106d, 1},
{0x1083, 0x1084, 1},
{0x1087, 0x108c, 1},
{0x108f, 0x109a, 11},
{0x109b, 0x109c, 1},
{0x17b6, 0x17be, 8},
{0x17bf, 0x17c5, 1},
{0x17c7, 0x17c8, 1},
{0x1923, 0x1926, 1},
{0x1929, 0x192b, 1},
{0x1930, 0x1931, 1},
{0x1933, 0x1938, 1},
{0x19b0, 0x19c0, 1},
{0x19c8, 0x19c9, 1},
{0x1a19, 0x1a1b, 1},
{0x1a55, 0x1a57, 2},
{0x1a61, 0x1a63, 2},
{0x1a64, 0x1a6d, 9},
{0x1a6e, 0x1a72, 1},
{0x1b04, 0x1b35, 49},
{0x1b3b, 0x1b3d, 2},
{0x1b3e, 0x1b41, 1},
{0x1b43, 0x1b44, 1},
{0x1b82, 0x1ba1, 31},
{0x1ba6, 0x1ba7, 1},
{0x1baa, 0x1be7, 61},
{0x1bea, 0x1bec, 1},
{0x1bee, 0x1bf2, 4},
{0x1bf3, 0x1c24, 49},
{0x1c25, 0x1c2b, 1},
{0x1c34, 0x1c35, 1},
{0x1ce1, 0x1cf2, 17},
{0xa823, 0xa824, 1},
{0xa827, 0xa880, 89},
{0xa881, 0xa8b4, 51},
{0xa8b5, 0xa8c3, 1},
{0xa952, 0xa953, 1},
{0xa983, 0xa9b4, 49},
{0xa9b5, 0xa9ba, 5},
{0xa9bb, 0xa9bd, 2},
{0xa9be, 0xa9c0, 1},
{0xaa2f, 0xaa30, 1},
{0xaa33, 0xaa34, 1},
{0xaa4d, 0xaa7b, 46},
{0xabe3, 0xabe4, 1},
{0xabe6, 0xabe7, 1},
{0xabe9, 0xabea, 1},
{0xabec, 0xabec, 1},
{0x11000, 0x11000, 1},
{0x11002, 0x11082, 128},
{0x110b0, 0x110b2, 1},
{0x110b7, 0x110b8, 1},
{0x1d165, 0x1d166, 1},
{0x1d16d, 0x1d172, 1},
};
}
private static final int[][] _Mn = make_Mn();
private static int[][] make_Mn() {
return new int[][] {
{0x0300, 0x036f, 1},
{0x0483, 0x0487, 1},
{0x0591, 0x05bd, 1},
{0x05bf, 0x05c1, 2},
{0x05c2, 0x05c4, 2},
{0x05c5, 0x05c7, 2},
{0x0610, 0x061a, 1},
{0x064b, 0x065f, 1},
{0x0670, 0x06d6, 102},
{0x06d7, 0x06dc, 1},
{0x06df, 0x06e4, 1},
{0x06e7, 0x06e8, 1},
{0x06ea, 0x06ed, 1},
{0x0711, 0x0730, 31},
{0x0731, 0x074a, 1},
{0x07a6, 0x07b0, 1},
{0x07eb, 0x07f3, 1},
{0x0816, 0x0819, 1},
{0x081b, 0x0823, 1},
{0x0825, 0x0827, 1},
{0x0829, 0x082d, 1},
{0x0859, 0x085b, 1},
{0x0900, 0x0902, 1},
{0x093a, 0x093c, 2},
{0x0941, 0x0948, 1},
{0x094d, 0x0951, 4},
{0x0952, 0x0957, 1},
{0x0962, 0x0963, 1},
{0x0981, 0x09bc, 59},
{0x09c1, 0x09c4, 1},
{0x09cd, 0x09e2, 21},
{0x09e3, 0x0a01, 30},
{0x0a02, 0x0a3c, 58},
{0x0a41, 0x0a42, 1},
{0x0a47, 0x0a48, 1},
{0x0a4b, 0x0a4d, 1},
{0x0a51, 0x0a70, 31},
{0x0a71, 0x0a75, 4},
{0x0a81, 0x0a82, 1},
{0x0abc, 0x0ac1, 5},
{0x0ac2, 0x0ac5, 1},
{0x0ac7, 0x0ac8, 1},
{0x0acd, 0x0ae2, 21},
{0x0ae3, 0x0b01, 30},
{0x0b3c, 0x0b3f, 3},
{0x0b41, 0x0b44, 1},
{0x0b4d, 0x0b56, 9},
{0x0b62, 0x0b63, 1},
{0x0b82, 0x0bc0, 62},
{0x0bcd, 0x0c3e, 113},
{0x0c3f, 0x0c40, 1},
{0x0c46, 0x0c48, 1},
{0x0c4a, 0x0c4d, 1},
{0x0c55, 0x0c56, 1},
{0x0c62, 0x0c63, 1},
{0x0cbc, 0x0cbf, 3},
{0x0cc6, 0x0ccc, 6},
{0x0ccd, 0x0ce2, 21},
{0x0ce3, 0x0d41, 94},
{0x0d42, 0x0d44, 1},
{0x0d4d, 0x0d62, 21},
{0x0d63, 0x0dca, 103},
{0x0dd2, 0x0dd4, 1},
{0x0dd6, 0x0e31, 91},
{0x0e34, 0x0e3a, 1},
{0x0e47, 0x0e4e, 1},
{0x0eb1, 0x0eb4, 3},
{0x0eb5, 0x0eb9, 1},
{0x0ebb, 0x0ebc, 1},
{0x0ec8, 0x0ecd, 1},
{0x0f18, 0x0f19, 1},
{0x0f35, 0x0f39, 2},
{0x0f71, 0x0f7e, 1},
{0x0f80, 0x0f84, 1},
{0x0f86, 0x0f87, 1},
{0x0f8d, 0x0f97, 1},
{0x0f99, 0x0fbc, 1},
{0x0fc6, 0x102d, 103},
{0x102e, 0x1030, 1},
{0x1032, 0x1037, 1},
{0x1039, 0x103a, 1},
{0x103d, 0x103e, 1},
{0x1058, 0x1059, 1},
{0x105e, 0x1060, 1},
{0x1071, 0x1074, 1},
{0x1082, 0x1085, 3},
{0x1086, 0x108d, 7},
{0x109d, 0x135d, 704},
{0x135e, 0x135f, 1},
{0x1712, 0x1714, 1},
{0x1732, 0x1734, 1},
{0x1752, 0x1753, 1},
{0x1772, 0x1773, 1},
{0x17b7, 0x17bd, 1},
{0x17c6, 0x17c9, 3},
{0x17ca, 0x17d3, 1},
{0x17dd, 0x180b, 46},
{0x180c, 0x180d, 1},
{0x18a9, 0x1920, 119},
{0x1921, 0x1922, 1},
{0x1927, 0x1928, 1},
{0x1932, 0x1939, 7},
{0x193a, 0x193b, 1},
{0x1a17, 0x1a18, 1},
{0x1a56, 0x1a58, 2},
{0x1a59, 0x1a5e, 1},
{0x1a60, 0x1a62, 2},
{0x1a65, 0x1a6c, 1},
{0x1a73, 0x1a7c, 1},
{0x1a7f, 0x1b00, 129},
{0x1b01, 0x1b03, 1},
{0x1b34, 0x1b36, 2},
{0x1b37, 0x1b3a, 1},
{0x1b3c, 0x1b42, 6},
{0x1b6b, 0x1b73, 1},
{0x1b80, 0x1b81, 1},
{0x1ba2, 0x1ba5, 1},
{0x1ba8, 0x1ba9, 1},
{0x1be6, 0x1be8, 2},
{0x1be9, 0x1bed, 4},
{0x1bef, 0x1bf1, 1},
{0x1c2c, 0x1c33, 1},
{0x1c36, 0x1c37, 1},
{0x1cd0, 0x1cd2, 1},
{0x1cd4, 0x1ce0, 1},
{0x1ce2, 0x1ce8, 1},
{0x1ced, 0x1dc0, 211},
{0x1dc1, 0x1de6, 1},
{0x1dfc, 0x1dff, 1},
{0x20d0, 0x20dc, 1},
{0x20e1, 0x20e5, 4},
{0x20e6, 0x20f0, 1},
{0x2cef, 0x2cf1, 1},
{0x2d7f, 0x2de0, 97},
{0x2de1, 0x2dff, 1},
{0x302a, 0x302f, 1},
{0x3099, 0x309a, 1},
{0xa66f, 0xa67c, 13},
{0xa67d, 0xa6f0, 115},
{0xa6f1, 0xa802, 273},
{0xa806, 0xa80b, 5},
{0xa825, 0xa826, 1},
{0xa8c4, 0xa8e0, 28},
{0xa8e1, 0xa8f1, 1},
{0xa926, 0xa92d, 1},
{0xa947, 0xa951, 1},
{0xa980, 0xa982, 1},
{0xa9b3, 0xa9b6, 3},
{0xa9b7, 0xa9b9, 1},
{0xa9bc, 0xaa29, 109},
{0xaa2a, 0xaa2e, 1},
{0xaa31, 0xaa32, 1},
{0xaa35, 0xaa36, 1},
{0xaa43, 0xaa4c, 9},
{0xaab0, 0xaab2, 2},
{0xaab3, 0xaab4, 1},
{0xaab7, 0xaab8, 1},
{0xaabe, 0xaabf, 1},
{0xaac1, 0xabe5, 292},
{0xabe8, 0xabed, 5},
{0xfb1e, 0xfe00, 738},
{0xfe01, 0xfe0f, 1},
{0xfe20, 0xfe26, 1},
{0x101fd, 0x10a01, 2052},
{0x10a02, 0x10a03, 1},
{0x10a05, 0x10a06, 1},
{0x10a0c, 0x10a0f, 1},
{0x10a38, 0x10a3a, 1},
{0x10a3f, 0x11001, 1474},
{0x11038, 0x11046, 1},
{0x11080, 0x11081, 1},
{0x110b3, 0x110b6, 1},
{0x110b9, 0x110ba, 1},
{0x1d167, 0x1d169, 1},
{0x1d17b, 0x1d182, 1},
{0x1d185, 0x1d18b, 1},
{0x1d1aa, 0x1d1ad, 1},
{0x1d242, 0x1d244, 1},
{0xe0100, 0xe01ef, 1},
};
}
private static final int[][] _Zl = make_Zl();
private static int[][] make_Zl() {
return new int[][] {
{0x2028, 0x2028, 1},
};
}
private static final int[][] _Zp = make_Zp();
private static int[][] make_Zp() {
return new int[][] {
{0x2029, 0x2029, 1},
};
}
private static final int[][] _Zs = make_Zs();
private static int[][] make_Zs() {
return new int[][] {
{0x0020, 0x00a0, 128},
{0x1680, 0x180e, 398},
{0x2000, 0x200a, 1},
{0x202f, 0x205f, 48},
{0x3000, 0x3000, 1},
};
}
private static final int[][] _Cs = make_Cs();
private static int[][] make_Cs() {
return new int[][] {
{0xd800, 0xdfff, 1},
};
}
private static final int[][] _Co = make_Co();
private static int[][] make_Co() {
return new int[][] {
{0xe000, 0xf8ff, 1},
{0xf0000, 0xffffd, 1},
{0x100000, 0x10fffd, 1},
};
}
private static final int[][] _Cf = make_Cf();
private static int[][] make_Cf() {
return new int[][] {
{0x00ad, 0x0600, 1363},
{0x0601, 0x0603, 1},
{0x06dd, 0x070f, 50},
{0x17b4, 0x17b5, 1},
{0x200b, 0x200f, 1},
{0x202a, 0x202e, 1},
{0x2060, 0x2064, 1},
{0x206a, 0x206f, 1},
{0xfeff, 0xfff9, 250},
{0xfffa, 0xfffb, 1},
{0x110bd, 0x1d173, 49334},
{0x1d174, 0x1d17a, 1},
{0xe0001, 0xe0020, 31},
{0xe0021, 0xe007f, 1},
};
}
private static final int[][] _Cc = make_Cc();
private static int[][] make_Cc() {
return new int[][] {
{0x0001, 0x001f, 1},
{0x007f, 0x009f, 1},
};
}
private static final int[][] _Po = make_Po();
private static int[][] make_Po() {
return new int[][] {
{0x0021, 0x0023, 1},
{0x0025, 0x0027, 1},
{0x002a, 0x002e, 2},
{0x002f, 0x003a, 11},
{0x003b, 0x003f, 4},
{0x0040, 0x005c, 28},
{0x00a1, 0x00b7, 22},
{0x00bf, 0x037e, 703},
{0x0387, 0x055a, 467},
{0x055b, 0x055f, 1},
{0x0589, 0x05c0, 55},
{0x05c3, 0x05c6, 3},
{0x05f3, 0x05f4, 1},
{0x0609, 0x060a, 1},
{0x060c, 0x060d, 1},
{0x061b, 0x061e, 3},
{0x061f, 0x066a, 75},
{0x066b, 0x066d, 1},
{0x06d4, 0x0700, 44},
{0x0701, 0x070d, 1},
{0x07f7, 0x07f9, 1},
{0x0830, 0x083e, 1},
{0x085e, 0x0964, 262},
{0x0965, 0x0970, 11},
{0x0df4, 0x0e4f, 91},
{0x0e5a, 0x0e5b, 1},
{0x0f04, 0x0f12, 1},
{0x0f85, 0x0fd0, 75},
{0x0fd1, 0x0fd4, 1},
{0x0fd9, 0x0fda, 1},
{0x104a, 0x104f, 1},
{0x10fb, 0x1361, 614},
{0x1362, 0x1368, 1},
{0x166d, 0x166e, 1},
{0x16eb, 0x16ed, 1},
{0x1735, 0x1736, 1},
{0x17d4, 0x17d6, 1},
{0x17d8, 0x17da, 1},
{0x1800, 0x1805, 1},
{0x1807, 0x180a, 1},
{0x1944, 0x1945, 1},
{0x1a1e, 0x1a1f, 1},
{0x1aa0, 0x1aa6, 1},
{0x1aa8, 0x1aad, 1},
{0x1b5a, 0x1b60, 1},
{0x1bfc, 0x1bff, 1},
{0x1c3b, 0x1c3f, 1},
{0x1c7e, 0x1c7f, 1},
{0x1cd3, 0x2016, 835},
{0x2017, 0x2020, 9},
{0x2021, 0x2027, 1},
{0x2030, 0x2038, 1},
{0x203b, 0x203e, 1},
{0x2041, 0x2043, 1},
{0x2047, 0x2051, 1},
{0x2053, 0x2055, 2},
{0x2056, 0x205e, 1},
{0x2cf9, 0x2cfc, 1},
{0x2cfe, 0x2cff, 1},
{0x2d70, 0x2e00, 144},
{0x2e01, 0x2e06, 5},
{0x2e07, 0x2e08, 1},
{0x2e0b, 0x2e0e, 3},
{0x2e0f, 0x2e16, 1},
{0x2e18, 0x2e19, 1},
{0x2e1b, 0x2e1e, 3},
{0x2e1f, 0x2e2a, 11},
{0x2e2b, 0x2e2e, 1},
{0x2e30, 0x2e31, 1},
{0x3001, 0x3003, 1},
{0x303d, 0x30fb, 190},
{0xa4fe, 0xa4ff, 1},
{0xa60d, 0xa60f, 1},
{0xa673, 0xa67e, 11},
{0xa6f2, 0xa6f7, 1},
{0xa874, 0xa877, 1},
{0xa8ce, 0xa8cf, 1},
{0xa8f8, 0xa8fa, 1},
{0xa92e, 0xa92f, 1},
{0xa95f, 0xa9c1, 98},
{0xa9c2, 0xa9cd, 1},
{0xa9de, 0xa9df, 1},
{0xaa5c, 0xaa5f, 1},
{0xaade, 0xaadf, 1},
{0xabeb, 0xfe10, 21029},
{0xfe11, 0xfe16, 1},
{0xfe19, 0xfe30, 23},
{0xfe45, 0xfe46, 1},
{0xfe49, 0xfe4c, 1},
{0xfe50, 0xfe52, 1},
{0xfe54, 0xfe57, 1},
{0xfe5f, 0xfe61, 1},
{0xfe68, 0xfe6a, 2},
{0xfe6b, 0xff01, 150},
{0xff02, 0xff03, 1},
{0xff05, 0xff07, 1},
{0xff0a, 0xff0e, 2},
{0xff0f, 0xff1a, 11},
{0xff1b, 0xff1f, 4},
{0xff20, 0xff3c, 28},
{0xff61, 0xff64, 3},
{0xff65, 0xff65, 1},
{0x10100, 0x10100, 1},
{0x10101, 0x1039f, 670},
{0x103d0, 0x10857, 1159},
{0x1091f, 0x1093f, 32},
{0x10a50, 0x10a58, 1},
{0x10a7f, 0x10b39, 186},
{0x10b3a, 0x10b3f, 1},
{0x11047, 0x1104d, 1},
{0x110bb, 0x110bc, 1},
{0x110be, 0x110c1, 1},
{0x12470, 0x12473, 1},
};
}
private static final int[][] _Pi = make_Pi();
private static int[][] make_Pi() {
return new int[][] {
{0x00ab, 0x2018, 8045},
{0x201b, 0x201c, 1},
{0x201f, 0x2039, 26},
{0x2e02, 0x2e04, 2},
{0x2e09, 0x2e0c, 3},
{0x2e1c, 0x2e20, 4},
};
}
private static final int[][] _Pf = make_Pf();
private static int[][] make_Pf() {
return new int[][] {
{0x00bb, 0x2019, 8030},
{0x201d, 0x203a, 29},
{0x2e03, 0x2e05, 2},
{0x2e0a, 0x2e0d, 3},
{0x2e1d, 0x2e21, 4},
};
}
private static final int[][] _Pe = make_Pe();
private static int[][] make_Pe() {
return new int[][] {
{0x0029, 0x005d, 52},
{0x007d, 0x0f3b, 3774},
{0x0f3d, 0x169c, 1887},
{0x2046, 0x207e, 56},
{0x208e, 0x232a, 668},
{0x2769, 0x2775, 2},
{0x27c6, 0x27e7, 33},
{0x27e9, 0x27ef, 2},
{0x2984, 0x2998, 2},
{0x29d9, 0x29db, 2},
{0x29fd, 0x2e23, 1062},
{0x2e25, 0x2e29, 2},
{0x3009, 0x3011, 2},
{0x3015, 0x301b, 2},
{0x301e, 0x301f, 1},
{0xfd3f, 0xfe18, 217},
{0xfe36, 0xfe44, 2},
{0xfe48, 0xfe5a, 18},
{0xfe5c, 0xfe5e, 2},
{0xff09, 0xff3d, 52},
{0xff5d, 0xff63, 3},
};
}
private static final int[][] _Pd = make_Pd();
private static int[][] make_Pd() {
return new int[][] {
{0x002d, 0x058a, 1373},
{0x05be, 0x1400, 3650},
{0x1806, 0x2010, 2058},
{0x2011, 0x2015, 1},
{0x2e17, 0x2e1a, 3},
{0x301c, 0x3030, 20},
{0x30a0, 0xfe31, 52625},
{0xfe32, 0xfe58, 38},
{0xfe63, 0xff0d, 170},
};
}
private static final int[][] _Pc = make_Pc();
private static int[][] make_Pc() {
return new int[][] {
{0x005f, 0x203f, 8160},
{0x2040, 0x2054, 20},
{0xfe33, 0xfe34, 1},
{0xfe4d, 0xfe4f, 1},
{0xff3f, 0xff3f, 1},
};
}
private static final int[][] _Ps = make_Ps();
private static int[][] make_Ps() {
return new int[][] {
{0x0028, 0x005b, 51},
{0x007b, 0x0f3a, 3775},
{0x0f3c, 0x169b, 1887},
{0x201a, 0x201e, 4},
{0x2045, 0x207d, 56},
{0x208d, 0x2329, 668},
{0x2768, 0x2774, 2},
{0x27c5, 0x27e6, 33},
{0x27e8, 0x27ee, 2},
{0x2983, 0x2997, 2},
{0x29d8, 0x29da, 2},
{0x29fc, 0x2e22, 1062},
{0x2e24, 0x2e28, 2},
{0x3008, 0x3010, 2},
{0x3014, 0x301a, 2},
{0x301d, 0xfd3e, 52513},
{0xfe17, 0xfe35, 30},
{0xfe37, 0xfe43, 2},
{0xfe47, 0xfe59, 18},
{0xfe5b, 0xfe5d, 2},
{0xff08, 0xff3b, 51},
{0xff5b, 0xff5f, 4},
{0xff62, 0xff62, 1},
};
}
private static final int[][] _Nd = make_Nd();
private static int[][] make_Nd() {
return new int[][] {
{0x0030, 0x0039, 1},
{0x0660, 0x0669, 1},
{0x06f0, 0x06f9, 1},
{0x07c0, 0x07c9, 1},
{0x0966, 0x096f, 1},
{0x09e6, 0x09ef, 1},
{0x0a66, 0x0a6f, 1},
{0x0ae6, 0x0aef, 1},
{0x0b66, 0x0b6f, 1},
{0x0be6, 0x0bef, 1},
{0x0c66, 0x0c6f, 1},
{0x0ce6, 0x0cef, 1},
{0x0d66, 0x0d6f, 1},
{0x0e50, 0x0e59, 1},
{0x0ed0, 0x0ed9, 1},
{0x0f20, 0x0f29, 1},
{0x1040, 0x1049, 1},
{0x1090, 0x1099, 1},
{0x17e0, 0x17e9, 1},
{0x1810, 0x1819, 1},
{0x1946, 0x194f, 1},
{0x19d0, 0x19d9, 1},
{0x1a80, 0x1a89, 1},
{0x1a90, 0x1a99, 1},
{0x1b50, 0x1b59, 1},
{0x1bb0, 0x1bb9, 1},
{0x1c40, 0x1c49, 1},
{0x1c50, 0x1c59, 1},
{0xa620, 0xa629, 1},
{0xa8d0, 0xa8d9, 1},
{0xa900, 0xa909, 1},
{0xa9d0, 0xa9d9, 1},
{0xaa50, 0xaa59, 1},
{0xabf0, 0xabf9, 1},
{0xff10, 0xff19, 1},
{0x104a0, 0x104a9, 1},
{0x11066, 0x1106f, 1},
{0x1d7ce, 0x1d7ff, 1},
};
}
private static final int[][] _Nl = make_Nl();
private static int[][] make_Nl() {
return new int[][] {
{0x16ee, 0x16f0, 1},
{0x2160, 0x2182, 1},
{0x2185, 0x2188, 1},
{0x3007, 0x3021, 26},
{0x3022, 0x3029, 1},
{0x3038, 0x303a, 1},
{0xa6e6, 0xa6ef, 1},
{0x10140, 0x10174, 1},
{0x10341, 0x1034a, 9},
{0x103d1, 0x103d5, 1},
{0x12400, 0x12462, 1},
};
}
private static final int[][] _No = make_No();
private static int[][] make_No() {
return new int[][] {
{0x00b2, 0x00b3, 1},
{0x00b9, 0x00bc, 3},
{0x00bd, 0x00be, 1},
{0x09f4, 0x09f9, 1},
{0x0b72, 0x0b77, 1},
{0x0bf0, 0x0bf2, 1},
{0x0c78, 0x0c7e, 1},
{0x0d70, 0x0d75, 1},
{0x0f2a, 0x0f33, 1},
{0x1369, 0x137c, 1},
{0x17f0, 0x17f9, 1},
{0x19da, 0x2070, 1686},
{0x2074, 0x2079, 1},
{0x2080, 0x2089, 1},
{0x2150, 0x215f, 1},
{0x2189, 0x2460, 727},
{0x2461, 0x249b, 1},
{0x24ea, 0x24ff, 1},
{0x2776, 0x2793, 1},
{0x2cfd, 0x3192, 1173},
{0x3193, 0x3195, 1},
{0x3220, 0x3229, 1},
{0x3251, 0x325f, 1},
{0x3280, 0x3289, 1},
{0x32b1, 0x32bf, 1},
{0xa830, 0xa835, 1},
{0x10107, 0x10133, 1},
{0x10175, 0x10178, 1},
{0x1018a, 0x10320, 406},
{0x10321, 0x10323, 1},
{0x10858, 0x1085f, 1},
{0x10916, 0x1091b, 1},
{0x10a40, 0x10a47, 1},
{0x10a7d, 0x10a7e, 1},
{0x10b58, 0x10b5f, 1},
{0x10b78, 0x10b7f, 1},
{0x10e60, 0x10e7e, 1},
{0x11052, 0x11065, 1},
{0x1d360, 0x1d371, 1},
{0x1f100, 0x1f10a, 1},
};
}
private static final int[][] _So = make_So();
private static int[][] make_So() {
return new int[][] {
{0x00a6, 0x00a7, 1},
{0x00a9, 0x00ae, 5},
{0x00b0, 0x00b6, 6},
{0x0482, 0x060e, 396},
{0x060f, 0x06de, 207},
{0x06e9, 0x06fd, 20},
{0x06fe, 0x07f6, 248},
{0x09fa, 0x0b70, 374},
{0x0bf3, 0x0bf8, 1},
{0x0bfa, 0x0c7f, 133},
{0x0d79, 0x0f01, 392},
{0x0f02, 0x0f03, 1},
{0x0f13, 0x0f17, 1},
{0x0f1a, 0x0f1f, 1},
{0x0f34, 0x0f38, 2},
{0x0fbe, 0x0fc5, 1},
{0x0fc7, 0x0fcc, 1},
{0x0fce, 0x0fcf, 1},
{0x0fd5, 0x0fd8, 1},
{0x109e, 0x109f, 1},
{0x1360, 0x1390, 48},
{0x1391, 0x1399, 1},
{0x1940, 0x19de, 158},
{0x19df, 0x19ff, 1},
{0x1b61, 0x1b6a, 1},
{0x1b74, 0x1b7c, 1},
{0x2100, 0x2101, 1},
{0x2103, 0x2106, 1},
{0x2108, 0x2109, 1},
{0x2114, 0x2116, 2},
{0x2117, 0x211e, 7},
{0x211f, 0x2123, 1},
{0x2125, 0x2129, 2},
{0x212e, 0x213a, 12},
{0x213b, 0x214a, 15},
{0x214c, 0x214d, 1},
{0x214f, 0x2195, 70},
{0x2196, 0x2199, 1},
{0x219c, 0x219f, 1},
{0x21a1, 0x21a2, 1},
{0x21a4, 0x21a5, 1},
{0x21a7, 0x21ad, 1},
{0x21af, 0x21cd, 1},
{0x21d0, 0x21d1, 1},
{0x21d3, 0x21d5, 2},
{0x21d6, 0x21f3, 1},
{0x2300, 0x2307, 1},
{0x230c, 0x231f, 1},
{0x2322, 0x2328, 1},
{0x232b, 0x237b, 1},
{0x237d, 0x239a, 1},
{0x23b4, 0x23db, 1},
{0x23e2, 0x23f3, 1},
{0x2400, 0x2426, 1},
{0x2440, 0x244a, 1},
{0x249c, 0x24e9, 1},
{0x2500, 0x25b6, 1},
{0x25b8, 0x25c0, 1},
{0x25c2, 0x25f7, 1},
{0x2600, 0x266e, 1},
{0x2670, 0x26ff, 1},
{0x2701, 0x2767, 1},
{0x2794, 0x27bf, 1},
{0x2800, 0x28ff, 1},
{0x2b00, 0x2b2f, 1},
{0x2b45, 0x2b46, 1},
{0x2b50, 0x2b59, 1},
{0x2ce5, 0x2cea, 1},
{0x2e80, 0x2e99, 1},
{0x2e9b, 0x2ef3, 1},
{0x2f00, 0x2fd5, 1},
{0x2ff0, 0x2ffb, 1},
{0x3004, 0x3012, 14},
{0x3013, 0x3020, 13},
{0x3036, 0x3037, 1},
{0x303e, 0x303f, 1},
{0x3190, 0x3191, 1},
{0x3196, 0x319f, 1},
{0x31c0, 0x31e3, 1},
{0x3200, 0x321e, 1},
{0x322a, 0x3250, 1},
{0x3260, 0x327f, 1},
{0x328a, 0x32b0, 1},
{0x32c0, 0x32fe, 1},
{0x3300, 0x33ff, 1},
{0x4dc0, 0x4dff, 1},
{0xa490, 0xa4c6, 1},
{0xa828, 0xa82b, 1},
{0xa836, 0xa837, 1},
{0xa839, 0xaa77, 574},
{0xaa78, 0xaa79, 1},
{0xfdfd, 0xffe4, 487},
{0xffe8, 0xffed, 5},
{0xffee, 0xfffc, 14},
{0xfffd, 0xfffd, 1},
{0x10102, 0x10102, 1},
{0x10137, 0x1013f, 1},
{0x10179, 0x10189, 1},
{0x10190, 0x1019b, 1},
{0x101d0, 0x101fc, 1},
{0x1d000, 0x1d0f5, 1},
{0x1d100, 0x1d126, 1},
{0x1d129, 0x1d164, 1},
{0x1d16a, 0x1d16c, 1},
{0x1d183, 0x1d184, 1},
{0x1d18c, 0x1d1a9, 1},
{0x1d1ae, 0x1d1dd, 1},
{0x1d200, 0x1d241, 1},
{0x1d245, 0x1d300, 187},
{0x1d301, 0x1d356, 1},
{0x1f000, 0x1f02b, 1},
{0x1f030, 0x1f093, 1},
{0x1f0a0, 0x1f0ae, 1},
{0x1f0b1, 0x1f0be, 1},
{0x1f0c1, 0x1f0cf, 1},
{0x1f0d1, 0x1f0df, 1},
{0x1f110, 0x1f12e, 1},
{0x1f130, 0x1f169, 1},
{0x1f170, 0x1f19a, 1},
{0x1f1e6, 0x1f202, 1},
{0x1f210, 0x1f23a, 1},
{0x1f240, 0x1f248, 1},
{0x1f250, 0x1f251, 1},
{0x1f300, 0x1f320, 1},
{0x1f330, 0x1f335, 1},
{0x1f337, 0x1f37c, 1},
{0x1f380, 0x1f393, 1},
{0x1f3a0, 0x1f3c4, 1},
{0x1f3c6, 0x1f3ca, 1},
{0x1f3e0, 0x1f3f0, 1},
{0x1f400, 0x1f43e, 1},
{0x1f440, 0x1f442, 2},
{0x1f443, 0x1f4f7, 1},
{0x1f4f9, 0x1f4fc, 1},
{0x1f500, 0x1f53d, 1},
{0x1f550, 0x1f567, 1},
{0x1f5fb, 0x1f5ff, 1},
{0x1f601, 0x1f610, 1},
{0x1f612, 0x1f614, 1},
{0x1f616, 0x1f61c, 2},
{0x1f61d, 0x1f61e, 1},
{0x1f620, 0x1f625, 1},
{0x1f628, 0x1f62b, 1},
{0x1f62d, 0x1f630, 3},
{0x1f631, 0x1f633, 1},
{0x1f635, 0x1f640, 1},
{0x1f645, 0x1f64f, 1},
{0x1f680, 0x1f6c5, 1},
{0x1f700, 0x1f773, 1},
};
}
private static final int[][] _Sm = make_Sm();
private static int[][] make_Sm() {
return new int[][] {
{0x002b, 0x003c, 17},
{0x003d, 0x003e, 1},
{0x007c, 0x007e, 2},
{0x00ac, 0x00b1, 5},
{0x00d7, 0x00f7, 32},
{0x03f6, 0x0606, 528},
{0x0607, 0x0608, 1},
{0x2044, 0x2052, 14},
{0x207a, 0x207c, 1},
{0x208a, 0x208c, 1},
{0x2118, 0x2140, 40},
{0x2141, 0x2144, 1},
{0x214b, 0x2190, 69},
{0x2191, 0x2194, 1},
{0x219a, 0x219b, 1},
{0x21a0, 0x21a6, 3},
{0x21ae, 0x21ce, 32},
{0x21cf, 0x21d2, 3},
{0x21d4, 0x21f4, 32},
{0x21f5, 0x22ff, 1},
{0x2308, 0x230b, 1},
{0x2320, 0x2321, 1},
{0x237c, 0x239b, 31},
{0x239c, 0x23b3, 1},
{0x23dc, 0x23e1, 1},
{0x25b7, 0x25c1, 10},
{0x25f8, 0x25ff, 1},
{0x266f, 0x27c0, 337},
{0x27c1, 0x27c4, 1},
{0x27c7, 0x27ca, 1},
{0x27cc, 0x27ce, 2},
{0x27cf, 0x27e5, 1},
{0x27f0, 0x27ff, 1},
{0x2900, 0x2982, 1},
{0x2999, 0x29d7, 1},
{0x29dc, 0x29fb, 1},
{0x29fe, 0x2aff, 1},
{0x2b30, 0x2b44, 1},
{0x2b47, 0x2b4c, 1},
{0xfb29, 0xfe62, 825},
{0xfe64, 0xfe66, 1},
{0xff0b, 0xff1c, 17},
{0xff1d, 0xff1e, 1},
{0xff5c, 0xff5e, 2},
{0xffe2, 0xffe9, 7},
{0xffea, 0xffec, 1},
{0x1d6c1, 0x1d6db, 26},
{0x1d6fb, 0x1d715, 26},
{0x1d735, 0x1d74f, 26},
{0x1d76f, 0x1d789, 26},
{0x1d7a9, 0x1d7c3, 26},
};
}
private static final int[][] _Sk = make_Sk();
private static int[][] make_Sk() {
return new int[][] {
{0x005e, 0x0060, 2},
{0x00a8, 0x00af, 7},
{0x00b4, 0x00b8, 4},
{0x02c2, 0x02c5, 1},
{0x02d2, 0x02df, 1},
{0x02e5, 0x02eb, 1},
{0x02ed, 0x02ef, 2},
{0x02f0, 0x02ff, 1},
{0x0375, 0x0384, 15},
{0x0385, 0x1fbd, 7224},
{0x1fbf, 0x1fc1, 1},
{0x1fcd, 0x1fcf, 1},
{0x1fdd, 0x1fdf, 1},
{0x1fed, 0x1fef, 1},
{0x1ffd, 0x1ffe, 1},
{0x309b, 0x309c, 1},
{0xa700, 0xa716, 1},
{0xa720, 0xa721, 1},
{0xa789, 0xa78a, 1},
{0xfbb2, 0xfbc1, 1},
{0xff3e, 0xff40, 2},
{0xffe3, 0xffe3, 1},
};
}
private static final int[][] _Sc = make_Sc();
private static int[][] make_Sc() {
return new int[][] {
{0x0024, 0x00a2, 126},
{0x00a3, 0x00a5, 1},
{0x060b, 0x09f2, 999},
{0x09f3, 0x09fb, 8},
{0x0af1, 0x0bf9, 264},
{0x0e3f, 0x17db, 2460},
{0x20a0, 0x20b9, 1},
{0xa838, 0xfdfc, 21956},
{0xfe69, 0xff04, 155},
{0xffe0, 0xffe1, 1},
{0xffe5, 0xffe6, 1},
};
}
private static final int[][] _Lu = make_Lu();
private static int[][] make_Lu() {
return new int[][] {
{0x0041, 0x005a, 1},
{0x00c0, 0x00d6, 1},
{0x00d8, 0x00de, 1},
{0x0100, 0x0136, 2},
{0x0139, 0x0147, 2},
{0x014a, 0x0178, 2},
{0x0179, 0x017d, 2},
{0x0181, 0x0182, 1},
{0x0184, 0x0186, 2},
{0x0187, 0x0189, 2},
{0x018a, 0x018b, 1},
{0x018e, 0x0191, 1},
{0x0193, 0x0194, 1},
{0x0196, 0x0198, 1},
{0x019c, 0x019d, 1},
{0x019f, 0x01a0, 1},
{0x01a2, 0x01a6, 2},
{0x01a7, 0x01a9, 2},
{0x01ac, 0x01ae, 2},
{0x01af, 0x01b1, 2},
{0x01b2, 0x01b3, 1},
{0x01b5, 0x01b7, 2},
{0x01b8, 0x01bc, 4},
{0x01c4, 0x01cd, 3},
{0x01cf, 0x01db, 2},
{0x01de, 0x01ee, 2},
{0x01f1, 0x01f4, 3},
{0x01f6, 0x01f8, 1},
{0x01fa, 0x0232, 2},
{0x023a, 0x023b, 1},
{0x023d, 0x023e, 1},
{0x0241, 0x0243, 2},
{0x0244, 0x0246, 1},
{0x0248, 0x024e, 2},
{0x0370, 0x0372, 2},
{0x0376, 0x0386, 16},
{0x0388, 0x038a, 1},
{0x038c, 0x038e, 2},
{0x038f, 0x0391, 2},
{0x0392, 0x03a1, 1},
{0x03a3, 0x03ab, 1},
{0x03cf, 0x03d2, 3},
{0x03d3, 0x03d4, 1},
{0x03d8, 0x03ee, 2},
{0x03f4, 0x03f7, 3},
{0x03f9, 0x03fa, 1},
{0x03fd, 0x042f, 1},
{0x0460, 0x0480, 2},
{0x048a, 0x04c0, 2},
{0x04c1, 0x04cd, 2},
{0x04d0, 0x0526, 2},
{0x0531, 0x0556, 1},
{0x10a0, 0x10c5, 1},
{0x1e00, 0x1e94, 2},
{0x1e9e, 0x1efe, 2},
{0x1f08, 0x1f0f, 1},
{0x1f18, 0x1f1d, 1},
{0x1f28, 0x1f2f, 1},
{0x1f38, 0x1f3f, 1},
{0x1f48, 0x1f4d, 1},
{0x1f59, 0x1f5f, 2},
{0x1f68, 0x1f6f, 1},
{0x1fb8, 0x1fbb, 1},
{0x1fc8, 0x1fcb, 1},
{0x1fd8, 0x1fdb, 1},
{0x1fe8, 0x1fec, 1},
{0x1ff8, 0x1ffb, 1},
{0x2102, 0x2107, 5},
{0x210b, 0x210d, 1},
{0x2110, 0x2112, 1},
{0x2115, 0x2119, 4},
{0x211a, 0x211d, 1},
{0x2124, 0x212a, 2},
{0x212b, 0x212d, 1},
{0x2130, 0x2133, 1},
{0x213e, 0x213f, 1},
{0x2145, 0x2183, 62},
{0x2c00, 0x2c2e, 1},
{0x2c60, 0x2c62, 2},
{0x2c63, 0x2c64, 1},
{0x2c67, 0x2c6d, 2},
{0x2c6e, 0x2c70, 1},
{0x2c72, 0x2c75, 3},
{0x2c7e, 0x2c80, 1},
{0x2c82, 0x2ce2, 2},
{0x2ceb, 0x2ced, 2},
{0xa640, 0xa66c, 2},
{0xa680, 0xa696, 2},
{0xa722, 0xa72e, 2},
{0xa732, 0xa76e, 2},
{0xa779, 0xa77d, 2},
{0xa77e, 0xa786, 2},
{0xa78b, 0xa78d, 2},
{0xa790, 0xa7a0, 16},
{0xa7a2, 0xa7a8, 2},
{0xff21, 0xff3a, 1},
{0x10400, 0x10427, 1},
{0x1d400, 0x1d419, 1},
{0x1d434, 0x1d44d, 1},
{0x1d468, 0x1d481, 1},
{0x1d49c, 0x1d49e, 2},
{0x1d49f, 0x1d4a5, 3},
{0x1d4a6, 0x1d4a9, 3},
{0x1d4aa, 0x1d4ac, 1},
{0x1d4ae, 0x1d4b5, 1},
{0x1d4d0, 0x1d4e9, 1},
{0x1d504, 0x1d505, 1},
{0x1d507, 0x1d50a, 1},
{0x1d50d, 0x1d514, 1},
{0x1d516, 0x1d51c, 1},
{0x1d538, 0x1d539, 1},
{0x1d53b, 0x1d53e, 1},
{0x1d540, 0x1d544, 1},
{0x1d546, 0x1d54a, 4},
{0x1d54b, 0x1d550, 1},
{0x1d56c, 0x1d585, 1},
{0x1d5a0, 0x1d5b9, 1},
{0x1d5d4, 0x1d5ed, 1},
{0x1d608, 0x1d621, 1},
{0x1d63c, 0x1d655, 1},
{0x1d670, 0x1d689, 1},
{0x1d6a8, 0x1d6c0, 1},
{0x1d6e2, 0x1d6fa, 1},
{0x1d71c, 0x1d734, 1},
{0x1d756, 0x1d76e, 1},
{0x1d790, 0x1d7a8, 1},
{0x1d7ca, 0x1d7ca, 1},
};
}
private static final int[][] _Lt = make_Lt();
private static int[][] make_Lt() {
return new int[][] {
{0x01c5, 0x01cb, 3},
{0x01f2, 0x1f88, 7574},
{0x1f89, 0x1f8f, 1},
{0x1f98, 0x1f9f, 1},
{0x1fa8, 0x1faf, 1},
{0x1fbc, 0x1fcc, 16},
{0x1ffc, 0x1ffc, 1},
};
}
private static final int[][] _Lo = make_Lo();
private static int[][] make_Lo() {
return new int[][] {
{0x01bb, 0x01c0, 5},
{0x01c1, 0x01c3, 1},
{0x0294, 0x05d0, 828},
{0x05d1, 0x05ea, 1},
{0x05f0, 0x05f2, 1},
{0x0620, 0x063f, 1},
{0x0641, 0x064a, 1},
{0x066e, 0x066f, 1},
{0x0671, 0x06d3, 1},
{0x06d5, 0x06ee, 25},
{0x06ef, 0x06fa, 11},
{0x06fb, 0x06fc, 1},
{0x06ff, 0x0710, 17},
{0x0712, 0x072f, 1},
{0x074d, 0x07a5, 1},
{0x07b1, 0x07ca, 25},
{0x07cb, 0x07ea, 1},
{0x0800, 0x0815, 1},
{0x0840, 0x0858, 1},
{0x0904, 0x0939, 1},
{0x093d, 0x0950, 19},
{0x0958, 0x0961, 1},
{0x0972, 0x0977, 1},
{0x0979, 0x097f, 1},
{0x0985, 0x098c, 1},
{0x098f, 0x0990, 1},
{0x0993, 0x09a8, 1},
{0x09aa, 0x09b0, 1},
{0x09b2, 0x09b6, 4},
{0x09b7, 0x09b9, 1},
{0x09bd, 0x09ce, 17},
{0x09dc, 0x09dd, 1},
{0x09df, 0x09e1, 1},
{0x09f0, 0x09f1, 1},
{0x0a05, 0x0a0a, 1},
{0x0a0f, 0x0a10, 1},
{0x0a13, 0x0a28, 1},
{0x0a2a, 0x0a30, 1},
{0x0a32, 0x0a33, 1},
{0x0a35, 0x0a36, 1},
{0x0a38, 0x0a39, 1},
{0x0a59, 0x0a5c, 1},
{0x0a5e, 0x0a72, 20},
{0x0a73, 0x0a74, 1},
{0x0a85, 0x0a8d, 1},
{0x0a8f, 0x0a91, 1},
{0x0a93, 0x0aa8, 1},
{0x0aaa, 0x0ab0, 1},
{0x0ab2, 0x0ab3, 1},
{0x0ab5, 0x0ab9, 1},
{0x0abd, 0x0ad0, 19},
{0x0ae0, 0x0ae1, 1},
{0x0b05, 0x0b0c, 1},
{0x0b0f, 0x0b10, 1},
{0x0b13, 0x0b28, 1},
{0x0b2a, 0x0b30, 1},
{0x0b32, 0x0b33, 1},
{0x0b35, 0x0b39, 1},
{0x0b3d, 0x0b5c, 31},
{0x0b5d, 0x0b5f, 2},
{0x0b60, 0x0b61, 1},
{0x0b71, 0x0b83, 18},
{0x0b85, 0x0b8a, 1},
{0x0b8e, 0x0b90, 1},
{0x0b92, 0x0b95, 1},
{0x0b99, 0x0b9a, 1},
{0x0b9c, 0x0b9e, 2},
{0x0b9f, 0x0ba3, 4},
{0x0ba4, 0x0ba8, 4},
{0x0ba9, 0x0baa, 1},
{0x0bae, 0x0bb9, 1},
{0x0bd0, 0x0c05, 53},
{0x0c06, 0x0c0c, 1},
{0x0c0e, 0x0c10, 1},
{0x0c12, 0x0c28, 1},
{0x0c2a, 0x0c33, 1},
{0x0c35, 0x0c39, 1},
{0x0c3d, 0x0c58, 27},
{0x0c59, 0x0c60, 7},
{0x0c61, 0x0c85, 36},
{0x0c86, 0x0c8c, 1},
{0x0c8e, 0x0c90, 1},
{0x0c92, 0x0ca8, 1},
{0x0caa, 0x0cb3, 1},
{0x0cb5, 0x0cb9, 1},
{0x0cbd, 0x0cde, 33},
{0x0ce0, 0x0ce1, 1},
{0x0cf1, 0x0cf2, 1},
{0x0d05, 0x0d0c, 1},
{0x0d0e, 0x0d10, 1},
{0x0d12, 0x0d3a, 1},
{0x0d3d, 0x0d4e, 17},
{0x0d60, 0x0d61, 1},
{0x0d7a, 0x0d7f, 1},
{0x0d85, 0x0d96, 1},
{0x0d9a, 0x0db1, 1},
{0x0db3, 0x0dbb, 1},
{0x0dbd, 0x0dc0, 3},
{0x0dc1, 0x0dc6, 1},
{0x0e01, 0x0e30, 1},
{0x0e32, 0x0e33, 1},
{0x0e40, 0x0e45, 1},
{0x0e81, 0x0e82, 1},
{0x0e84, 0x0e87, 3},
{0x0e88, 0x0e8a, 2},
{0x0e8d, 0x0e94, 7},
{0x0e95, 0x0e97, 1},
{0x0e99, 0x0e9f, 1},
{0x0ea1, 0x0ea3, 1},
{0x0ea5, 0x0ea7, 2},
{0x0eaa, 0x0eab, 1},
{0x0ead, 0x0eb0, 1},
{0x0eb2, 0x0eb3, 1},
{0x0ebd, 0x0ec0, 3},
{0x0ec1, 0x0ec4, 1},
{0x0edc, 0x0edd, 1},
{0x0f00, 0x0f40, 64},
{0x0f41, 0x0f47, 1},
{0x0f49, 0x0f6c, 1},
{0x0f88, 0x0f8c, 1},
{0x1000, 0x102a, 1},
{0x103f, 0x1050, 17},
{0x1051, 0x1055, 1},
{0x105a, 0x105d, 1},
{0x1061, 0x1065, 4},
{0x1066, 0x106e, 8},
{0x106f, 0x1070, 1},
{0x1075, 0x1081, 1},
{0x108e, 0x10d0, 66},
{0x10d1, 0x10fa, 1},
{0x1100, 0x1248, 1},
{0x124a, 0x124d, 1},
{0x1250, 0x1256, 1},
{0x1258, 0x125a, 2},
{0x125b, 0x125d, 1},
{0x1260, 0x1288, 1},
{0x128a, 0x128d, 1},
{0x1290, 0x12b0, 1},
{0x12b2, 0x12b5, 1},
{0x12b8, 0x12be, 1},
{0x12c0, 0x12c2, 2},
{0x12c3, 0x12c5, 1},
{0x12c8, 0x12d6, 1},
{0x12d8, 0x1310, 1},
{0x1312, 0x1315, 1},
{0x1318, 0x135a, 1},
{0x1380, 0x138f, 1},
{0x13a0, 0x13f4, 1},
{0x1401, 0x166c, 1},
{0x166f, 0x167f, 1},
{0x1681, 0x169a, 1},
{0x16a0, 0x16ea, 1},
{0x1700, 0x170c, 1},
{0x170e, 0x1711, 1},
{0x1720, 0x1731, 1},
{0x1740, 0x1751, 1},
{0x1760, 0x176c, 1},
{0x176e, 0x1770, 1},
{0x1780, 0x17b3, 1},
{0x17dc, 0x1820, 68},
{0x1821, 0x1842, 1},
{0x1844, 0x1877, 1},
{0x1880, 0x18a8, 1},
{0x18aa, 0x18b0, 6},
{0x18b1, 0x18f5, 1},
{0x1900, 0x191c, 1},
{0x1950, 0x196d, 1},
{0x1970, 0x1974, 1},
{0x1980, 0x19ab, 1},
{0x19c1, 0x19c7, 1},
{0x1a00, 0x1a16, 1},
{0x1a20, 0x1a54, 1},
{0x1b05, 0x1b33, 1},
{0x1b45, 0x1b4b, 1},
{0x1b83, 0x1ba0, 1},
{0x1bae, 0x1baf, 1},
{0x1bc0, 0x1be5, 1},
{0x1c00, 0x1c23, 1},
{0x1c4d, 0x1c4f, 1},
{0x1c5a, 0x1c77, 1},
{0x1ce9, 0x1cec, 1},
{0x1cee, 0x1cf1, 1},
{0x2135, 0x2138, 1},
{0x2d30, 0x2d65, 1},
{0x2d80, 0x2d96, 1},
{0x2da0, 0x2da6, 1},
{0x2da8, 0x2dae, 1},
{0x2db0, 0x2db6, 1},
{0x2db8, 0x2dbe, 1},
{0x2dc0, 0x2dc6, 1},
{0x2dc8, 0x2dce, 1},
{0x2dd0, 0x2dd6, 1},
{0x2dd8, 0x2dde, 1},
{0x3006, 0x303c, 54},
{0x3041, 0x3096, 1},
{0x309f, 0x30a1, 2},
{0x30a2, 0x30fa, 1},
{0x30ff, 0x3105, 6},
{0x3106, 0x312d, 1},
{0x3131, 0x318e, 1},
{0x31a0, 0x31ba, 1},
{0x31f0, 0x31ff, 1},
{0x3400, 0x4db5, 1},
{0x4e00, 0x9fcb, 1},
{0xa000, 0xa014, 1},
{0xa016, 0xa48c, 1},
{0xa4d0, 0xa4f7, 1},
{0xa500, 0xa60b, 1},
{0xa610, 0xa61f, 1},
{0xa62a, 0xa62b, 1},
{0xa66e, 0xa6a0, 50},
{0xa6a1, 0xa6e5, 1},
{0xa7fb, 0xa801, 1},
{0xa803, 0xa805, 1},
{0xa807, 0xa80a, 1},
{0xa80c, 0xa822, 1},
{0xa840, 0xa873, 1},
{0xa882, 0xa8b3, 1},
{0xa8f2, 0xa8f7, 1},
{0xa8fb, 0xa90a, 15},
{0xa90b, 0xa925, 1},
{0xa930, 0xa946, 1},
{0xa960, 0xa97c, 1},
{0xa984, 0xa9b2, 1},
{0xaa00, 0xaa28, 1},
{0xaa40, 0xaa42, 1},
{0xaa44, 0xaa4b, 1},
{0xaa60, 0xaa6f, 1},
{0xaa71, 0xaa76, 1},
{0xaa7a, 0xaa80, 6},
{0xaa81, 0xaaaf, 1},
{0xaab1, 0xaab5, 4},
{0xaab6, 0xaab9, 3},
{0xaaba, 0xaabd, 1},
{0xaac0, 0xaac2, 2},
{0xaadb, 0xaadc, 1},
{0xab01, 0xab06, 1},
{0xab09, 0xab0e, 1},
{0xab11, 0xab16, 1},
{0xab20, 0xab26, 1},
{0xab28, 0xab2e, 1},
{0xabc0, 0xabe2, 1},
{0xac00, 0xd7a3, 1},
{0xd7b0, 0xd7c6, 1},
{0xd7cb, 0xd7fb, 1},
{0xf900, 0xfa2d, 1},
{0xfa30, 0xfa6d, 1},
{0xfa70, 0xfad9, 1},
{0xfb1d, 0xfb1f, 2},
{0xfb20, 0xfb28, 1},
{0xfb2a, 0xfb36, 1},
{0xfb38, 0xfb3c, 1},
{0xfb3e, 0xfb40, 2},
{0xfb41, 0xfb43, 2},
{0xfb44, 0xfb46, 2},
{0xfb47, 0xfbb1, 1},
{0xfbd3, 0xfd3d, 1},
{0xfd50, 0xfd8f, 1},
{0xfd92, 0xfdc7, 1},
{0xfdf0, 0xfdfb, 1},
{0xfe70, 0xfe74, 1},
{0xfe76, 0xfefc, 1},
{0xff66, 0xff6f, 1},
{0xff71, 0xff9d, 1},
{0xffa0, 0xffbe, 1},
{0xffc2, 0xffc7, 1},
{0xffca, 0xffcf, 1},
{0xffd2, 0xffd7, 1},
{0xffda, 0xffdc, 1},
{0x10000, 0x1000b, 1},
{0x1000d, 0x10026, 1},
{0x10028, 0x1003a, 1},
{0x1003c, 0x1003d, 1},
{0x1003f, 0x1004d, 1},
{0x10050, 0x1005d, 1},
{0x10080, 0x100fa, 1},
{0x10280, 0x1029c, 1},
{0x102a0, 0x102d0, 1},
{0x10300, 0x1031e, 1},
{0x10330, 0x10340, 1},
{0x10342, 0x10349, 1},
{0x10380, 0x1039d, 1},
{0x103a0, 0x103c3, 1},
{0x103c8, 0x103cf, 1},
{0x10450, 0x1049d, 1},
{0x10800, 0x10805, 1},
{0x10808, 0x1080a, 2},
{0x1080b, 0x10835, 1},
{0x10837, 0x10838, 1},
{0x1083c, 0x1083f, 3},
{0x10840, 0x10855, 1},
{0x10900, 0x10915, 1},
{0x10920, 0x10939, 1},
{0x10a00, 0x10a10, 16},
{0x10a11, 0x10a13, 1},
{0x10a15, 0x10a17, 1},
{0x10a19, 0x10a33, 1},
{0x10a60, 0x10a7c, 1},
{0x10b00, 0x10b35, 1},
{0x10b40, 0x10b55, 1},
{0x10b60, 0x10b72, 1},
{0x10c00, 0x10c48, 1},
{0x11003, 0x11037, 1},
{0x11083, 0x110af, 1},
{0x12000, 0x1236e, 1},
{0x13000, 0x1342e, 1},
{0x16800, 0x16a38, 1},
{0x1b000, 0x1b001, 1},
{0x20000, 0x2a6d6, 1},
{0x2a700, 0x2b734, 1},
{0x2b740, 0x2b81d, 1},
{0x2f800, 0x2fa1d, 1},
};
}
static final int[][] Cc = _Cc;
static final int[][] Cf = _Cf;
static final int[][] Co = _Co;
static final int[][] Cs = _Cs;
static final int[][] Digit = _Nd;
static final int[][] Nd = _Nd;
static final int[][] Letter = _L;
static final int[][] L = _L;
static final int[][] Lm = _Lm;
static final int[][] Lo = _Lo;
static final int[][] Lower = _Ll;
static final int[][] Ll = _Ll;
static final int[][] Mark = _M;
static final int[][] M = _M;
static final int[][] Mc = _Mc;
static final int[][] Me = _Me;
static final int[][] Mn = _Mn;
static final int[][] Nl = _Nl;
static final int[][] No = _No;
static final int[][] Number = _N;
static final int[][] N = _N;
static final int[][] Other = _C;
static final int[][] C = _C;
static final int[][] Pc = _Pc;
static final int[][] Pd = _Pd;
static final int[][] Pe = _Pe;
static final int[][] Pf = _Pf;
static final int[][] Pi = _Pi;
static final int[][] Po = _Po;
static final int[][] Ps = _Ps;
static final int[][] Punct = _P;
static final int[][] P = _P;
static final int[][] Sc = _Sc;
static final int[][] Sk = _Sk;
static final int[][] Sm = _Sm;
static final int[][] So = _So;
static final int[][] Space = _Z;
static final int[][] Z = _Z;
static final int[][] Symbol = _S;
static final int[][] S = _S;
static final int[][] Title = _Lt;
static final int[][] Lt = _Lt;
static final int[][] Upper = _Lu;
static final int[][] Lu = _Lu;
static final int[][] Zl = _Zl;
static final int[][] Zp = _Zp;
static final int[][] Zs = _Zs;
private static Map Scripts() {
Map map = new HashMap();
map.put("Katakana", Katakana);
map.put("Malayalam", Malayalam);
map.put("Phags_Pa", Phags_Pa);
map.put("Inscriptional_Parthian", Inscriptional_Parthian);
map.put("Latin", Latin);
map.put("Inscriptional_Pahlavi", Inscriptional_Pahlavi);
map.put("Osmanya", Osmanya);
map.put("Khmer", Khmer);
map.put("Inherited", Inherited);
map.put("Telugu", Telugu);
map.put("Samaritan", Samaritan);
map.put("Bopomofo", Bopomofo);
map.put("Imperial_Aramaic", Imperial_Aramaic);
map.put("Kaithi", Kaithi);
map.put("Mandaic", Mandaic);
map.put("Old_South_Arabian", Old_South_Arabian);
map.put("Kayah_Li", Kayah_Li);
map.put("New_Tai_Lue", New_Tai_Lue);
map.put("Tai_Le", Tai_Le);
map.put("Kharoshthi", Kharoshthi);
map.put("Common", Common);
map.put("Kannada", Kannada);
map.put("Old_Turkic", Old_Turkic);
map.put("Tamil", Tamil);
map.put("Tagalog", Tagalog);
map.put("Brahmi", Brahmi);
map.put("Arabic", Arabic);
map.put("Tagbanwa", Tagbanwa);
map.put("Canadian_Aboriginal", Canadian_Aboriginal);
map.put("Tibetan", Tibetan);
map.put("Coptic", Coptic);
map.put("Hiragana", Hiragana);
map.put("Limbu", Limbu);
map.put("Egyptian_Hieroglyphs", Egyptian_Hieroglyphs);
map.put("Avestan", Avestan);
map.put("Myanmar", Myanmar);
map.put("Armenian", Armenian);
map.put("Sinhala", Sinhala);
map.put("Bengali", Bengali);
map.put("Greek", Greek);
map.put("Cham", Cham);
map.put("Hebrew", Hebrew);
map.put("Meetei_Mayek", Meetei_Mayek);
map.put("Saurashtra", Saurashtra);
map.put("Hangul", Hangul);
map.put("Runic", Runic);
map.put("Deseret", Deseret);
map.put("Lisu", Lisu);
map.put("Sundanese", Sundanese);
map.put("Glagolitic", Glagolitic);
map.put("Oriya", Oriya);
map.put("Buhid", Buhid);
map.put("Ethiopic", Ethiopic);
map.put("Javanese", Javanese);
map.put("Syloti_Nagri", Syloti_Nagri);
map.put("Vai", Vai);
map.put("Cherokee", Cherokee);
map.put("Ogham", Ogham);
map.put("Batak", Batak);
map.put("Syriac", Syriac);
map.put("Gurmukhi", Gurmukhi);
map.put("Tai_Tham", Tai_Tham);
map.put("Ol_Chiki", Ol_Chiki);
map.put("Mongolian", Mongolian);
map.put("Hanunoo", Hanunoo);
map.put("Cypriot", Cypriot);
map.put("Buginese", Buginese);
map.put("Bamum", Bamum);
map.put("Lepcha", Lepcha);
map.put("Thaana", Thaana);
map.put("Old_Persian", Old_Persian);
map.put("Cuneiform", Cuneiform);
map.put("Rejang", Rejang);
map.put("Georgian", Georgian);
map.put("Shavian", Shavian);
map.put("Lycian", Lycian);
map.put("Nko", Nko);
map.put("Yi", Yi);
map.put("Lao", Lao);
map.put("Linear_B", Linear_B);
map.put("Old_Italic", Old_Italic);
map.put("Tai_Viet", Tai_Viet);
map.put("Devanagari", Devanagari);
map.put("Lydian", Lydian);
map.put("Tifinagh", Tifinagh);
map.put("Ugaritic", Ugaritic);
map.put("Thai", Thai);
map.put("Cyrillic", Cyrillic);
map.put("Gujarati", Gujarati);
map.put("Carian", Carian);
map.put("Phoenician", Phoenician);
map.put("Balinese", Balinese);
map.put("Braille", Braille);
map.put("Han", Han);
map.put("Gothic", Gothic);
return map;
}
private static final int[][] _Katakana = make_Katakana();
private static int[][] make_Katakana() {
return new int[][] {
{0x30a1, 0x30fa, 1},
{0x30fd, 0x30ff, 1},
{0x31f0, 0x31ff, 1},
{0x32d0, 0x32fe, 1},
{0x3300, 0x3357, 1},
{0xff66, 0xff6f, 1},
{0xff71, 0xff9d, 1},
{0x1b000, 0x1b000, 1},
};
}
private static final int[][] _Malayalam = make_Malayalam();
private static int[][] make_Malayalam() {
return new int[][] {
{0x0d02, 0x0d03, 1},
{0x0d05, 0x0d0c, 1},
{0x0d0e, 0x0d10, 1},
{0x0d12, 0x0d3a, 1},
{0x0d3d, 0x0d44, 1},
{0x0d46, 0x0d48, 1},
{0x0d4a, 0x0d4e, 1},
{0x0d57, 0x0d57, 1},
{0x0d60, 0x0d63, 1},
{0x0d66, 0x0d75, 1},
{0x0d79, 0x0d7f, 1},
};
}
private static final int[][] _Phags_Pa = make_Phags_Pa();
private static int[][] make_Phags_Pa() {
return new int[][] {
{0xa840, 0xa877, 1},
};
}
private static final int[][] _Inscriptional_Parthian = make_Inscriptional_Parthian();
private static int[][] make_Inscriptional_Parthian() {
return new int[][] {
{0x10b40, 0x10b55, 1},
{0x10b58, 0x10b5f, 1},
};
}
private static final int[][] _Latin = make_Latin();
private static int[][] make_Latin() {
return new int[][] {
{0x0041, 0x005a, 1},
{0x0061, 0x007a, 1},
{0x00aa, 0x00aa, 1},
{0x00ba, 0x00ba, 1},
{0x00c0, 0x00d6, 1},
{0x00d8, 0x00f6, 1},
{0x00f8, 0x02b8, 1},
{0x02e0, 0x02e4, 1},
{0x1d00, 0x1d25, 1},
{0x1d2c, 0x1d5c, 1},
{0x1d62, 0x1d65, 1},
{0x1d6b, 0x1d77, 1},
{0x1d79, 0x1dbe, 1},
{0x1e00, 0x1eff, 1},
{0x2071, 0x2071, 1},
{0x207f, 0x207f, 1},
{0x2090, 0x209c, 1},
{0x212a, 0x212b, 1},
{0x2132, 0x2132, 1},
{0x214e, 0x214e, 1},
{0x2160, 0x2188, 1},
{0x2c60, 0x2c7f, 1},
{0xa722, 0xa787, 1},
{0xa78b, 0xa78e, 1},
{0xa790, 0xa791, 1},
{0xa7a0, 0xa7a9, 1},
{0xa7fa, 0xa7ff, 1},
{0xfb00, 0xfb06, 1},
{0xff21, 0xff3a, 1},
{0xff41, 0xff5a, 1},
};
}
private static final int[][] _Inscriptional_Pahlavi = make_Inscriptional_Pahlavi();
private static int[][] make_Inscriptional_Pahlavi() {
return new int[][] {
{0x10b60, 0x10b72, 1},
{0x10b78, 0x10b7f, 1},
};
}
private static final int[][] _Osmanya = make_Osmanya();
private static int[][] make_Osmanya() {
return new int[][] {
{0x10480, 0x1049d, 1},
{0x104a0, 0x104a9, 1},
};
}
private static final int[][] _Khmer = make_Khmer();
private static int[][] make_Khmer() {
return new int[][] {
{0x1780, 0x17dd, 1},
{0x17e0, 0x17e9, 1},
{0x17f0, 0x17f9, 1},
{0x19e0, 0x19ff, 1},
};
}
private static final int[][] _Inherited = make_Inherited();
private static int[][] make_Inherited() {
return new int[][] {
{0x0300, 0x036f, 1},
{0x0485, 0x0486, 1},
{0x064b, 0x0655, 1},
{0x065f, 0x065f, 1},
{0x0670, 0x0670, 1},
{0x0951, 0x0952, 1},
{0x1cd0, 0x1cd2, 1},
{0x1cd4, 0x1ce0, 1},
{0x1ce2, 0x1ce8, 1},
{0x1ced, 0x1ced, 1},
{0x1dc0, 0x1de6, 1},
{0x1dfc, 0x1dff, 1},
{0x200c, 0x200d, 1},
{0x20d0, 0x20f0, 1},
{0x302a, 0x302d, 1},
{0x3099, 0x309a, 1},
{0xfe00, 0xfe0f, 1},
{0xfe20, 0xfe26, 1},
{0x101fd, 0x101fd, 1},
{0x1d167, 0x1d169, 1},
{0x1d17b, 0x1d182, 1},
{0x1d185, 0x1d18b, 1},
{0x1d1aa, 0x1d1ad, 1},
{0xe0100, 0xe01ef, 1},
};
}
private static final int[][] _Telugu = make_Telugu();
private static int[][] make_Telugu() {
return new int[][] {
{0x0c01, 0x0c03, 1},
{0x0c05, 0x0c0c, 1},
{0x0c0e, 0x0c10, 1},
{0x0c12, 0x0c28, 1},
{0x0c2a, 0x0c33, 1},
{0x0c35, 0x0c39, 1},
{0x0c3d, 0x0c44, 1},
{0x0c46, 0x0c48, 1},
{0x0c4a, 0x0c4d, 1},
{0x0c55, 0x0c56, 1},
{0x0c58, 0x0c59, 1},
{0x0c60, 0x0c63, 1},
{0x0c66, 0x0c6f, 1},
{0x0c78, 0x0c7f, 1},
};
}
private static final int[][] _Samaritan = make_Samaritan();
private static int[][] make_Samaritan() {
return new int[][] {
{0x0800, 0x082d, 1},
{0x0830, 0x083e, 1},
};
}
private static final int[][] _Bopomofo = make_Bopomofo();
private static int[][] make_Bopomofo() {
return new int[][] {
{0x02ea, 0x02eb, 1},
{0x3105, 0x312d, 1},
{0x31a0, 0x31ba, 1},
};
}
private static final int[][] _Imperial_Aramaic = make_Imperial_Aramaic();
private static int[][] make_Imperial_Aramaic() {
return new int[][] {
{0x10840, 0x10855, 1},
{0x10857, 0x1085f, 1},
};
}
private static final int[][] _Kaithi = make_Kaithi();
private static int[][] make_Kaithi() {
return new int[][] {
{0x11080, 0x110c1, 1},
};
}
private static final int[][] _Mandaic = make_Mandaic();
private static int[][] make_Mandaic() {
return new int[][] {
{0x0840, 0x085b, 1},
{0x085e, 0x085e, 1},
};
}
private static final int[][] _Old_South_Arabian = make_Old_South_Arabian();
private static int[][] make_Old_South_Arabian() {
return new int[][] {
{0x10a60, 0x10a7f, 1},
};
}
private static final int[][] _Kayah_Li = make_Kayah_Li();
private static int[][] make_Kayah_Li() {
return new int[][] {
{0xa900, 0xa92f, 1},
};
}
private static final int[][] _New_Tai_Lue = make_New_Tai_Lue();
private static int[][] make_New_Tai_Lue() {
return new int[][] {
{0x1980, 0x19ab, 1},
{0x19b0, 0x19c9, 1},
{0x19d0, 0x19da, 1},
{0x19de, 0x19df, 1},
};
}
private static final int[][] _Tai_Le = make_Tai_Le();
private static int[][] make_Tai_Le() {
return new int[][] {
{0x1950, 0x196d, 1},
{0x1970, 0x1974, 1},
};
}
private static final int[][] _Kharoshthi = make_Kharoshthi();
private static int[][] make_Kharoshthi() {
return new int[][] {
{0x10a00, 0x10a03, 1},
{0x10a05, 0x10a06, 1},
{0x10a0c, 0x10a13, 1},
{0x10a15, 0x10a17, 1},
{0x10a19, 0x10a33, 1},
{0x10a38, 0x10a3a, 1},
{0x10a3f, 0x10a47, 1},
{0x10a50, 0x10a58, 1},
};
}
private static final int[][] _Common = make_Common();
private static int[][] make_Common() {
return new int[][] {
{0x0000, 0x0040, 1},
{0x005b, 0x0060, 1},
{0x007b, 0x00a9, 1},
{0x00ab, 0x00b9, 1},
{0x00bb, 0x00bf, 1},
{0x00d7, 0x00d7, 1},
{0x00f7, 0x00f7, 1},
{0x02b9, 0x02df, 1},
{0x02e5, 0x02e9, 1},
{0x02ec, 0x02ff, 1},
{0x0374, 0x0374, 1},
{0x037e, 0x037e, 1},
{0x0385, 0x0385, 1},
{0x0387, 0x0387, 1},
{0x0589, 0x0589, 1},
{0x060c, 0x060c, 1},
{0x061b, 0x061b, 1},
{0x061f, 0x061f, 1},
{0x0640, 0x0640, 1},
{0x0660, 0x0669, 1},
{0x06dd, 0x06dd, 1},
{0x0964, 0x0965, 1},
{0x0970, 0x0970, 1},
{0x0e3f, 0x0e3f, 1},
{0x0fd5, 0x0fd8, 1},
{0x10fb, 0x10fb, 1},
{0x16eb, 0x16ed, 1},
{0x1735, 0x1736, 1},
{0x1802, 0x1803, 1},
{0x1805, 0x1805, 1},
{0x1cd3, 0x1cd3, 1},
{0x1ce1, 0x1ce1, 1},
{0x1ce9, 0x1cec, 1},
{0x1cee, 0x1cf2, 1},
{0x2000, 0x200b, 1},
{0x200e, 0x2064, 1},
{0x206a, 0x2070, 1},
{0x2074, 0x207e, 1},
{0x2080, 0x208e, 1},
{0x20a0, 0x20b9, 1},
{0x2100, 0x2125, 1},
{0x2127, 0x2129, 1},
{0x212c, 0x2131, 1},
{0x2133, 0x214d, 1},
{0x214f, 0x215f, 1},
{0x2189, 0x2189, 1},
{0x2190, 0x23f3, 1},
{0x2400, 0x2426, 1},
{0x2440, 0x244a, 1},
{0x2460, 0x26ff, 1},
{0x2701, 0x27ca, 1},
{0x27cc, 0x27cc, 1},
{0x27ce, 0x27ff, 1},
{0x2900, 0x2b4c, 1},
{0x2b50, 0x2b59, 1},
{0x2e00, 0x2e31, 1},
{0x2ff0, 0x2ffb, 1},
{0x3000, 0x3004, 1},
{0x3006, 0x3006, 1},
{0x3008, 0x3020, 1},
{0x3030, 0x3037, 1},
{0x303c, 0x303f, 1},
{0x309b, 0x309c, 1},
{0x30a0, 0x30a0, 1},
{0x30fb, 0x30fc, 1},
{0x3190, 0x319f, 1},
{0x31c0, 0x31e3, 1},
{0x3220, 0x325f, 1},
{0x327f, 0x32cf, 1},
{0x3358, 0x33ff, 1},
{0x4dc0, 0x4dff, 1},
{0xa700, 0xa721, 1},
{0xa788, 0xa78a, 1},
{0xa830, 0xa839, 1},
{0xfd3e, 0xfd3f, 1},
{0xfdfd, 0xfdfd, 1},
{0xfe10, 0xfe19, 1},
{0xfe30, 0xfe52, 1},
{0xfe54, 0xfe66, 1},
{0xfe68, 0xfe6b, 1},
{0xfeff, 0xfeff, 1},
{0xff01, 0xff20, 1},
{0xff3b, 0xff40, 1},
{0xff5b, 0xff65, 1},
{0xff70, 0xff70, 1},
{0xff9e, 0xff9f, 1},
{0xffe0, 0xffe6, 1},
{0xffe8, 0xffee, 1},
{0xfff9, 0xfffd, 1},
{0x10100, 0x10102, 1},
{0x10107, 0x10133, 1},
{0x10137, 0x1013f, 1},
{0x10190, 0x1019b, 1},
{0x101d0, 0x101fc, 1},
{0x1d000, 0x1d0f5, 1},
{0x1d100, 0x1d126, 1},
{0x1d129, 0x1d166, 1},
{0x1d16a, 0x1d17a, 1},
{0x1d183, 0x1d184, 1},
{0x1d18c, 0x1d1a9, 1},
{0x1d1ae, 0x1d1dd, 1},
{0x1d300, 0x1d356, 1},
{0x1d360, 0x1d371, 1},
{0x1d400, 0x1d454, 1},
{0x1d456, 0x1d49c, 1},
{0x1d49e, 0x1d49f, 1},
{0x1d4a2, 0x1d4a2, 1},
{0x1d4a5, 0x1d4a6, 1},
{0x1d4a9, 0x1d4ac, 1},
{0x1d4ae, 0x1d4b9, 1},
{0x1d4bb, 0x1d4bb, 1},
{0x1d4bd, 0x1d4c3, 1},
{0x1d4c5, 0x1d505, 1},
{0x1d507, 0x1d50a, 1},
{0x1d50d, 0x1d514, 1},
{0x1d516, 0x1d51c, 1},
{0x1d51e, 0x1d539, 1},
{0x1d53b, 0x1d53e, 1},
{0x1d540, 0x1d544, 1},
{0x1d546, 0x1d546, 1},
{0x1d54a, 0x1d550, 1},
{0x1d552, 0x1d6a5, 1},
{0x1d6a8, 0x1d7cb, 1},
{0x1d7ce, 0x1d7ff, 1},
{0x1f000, 0x1f02b, 1},
{0x1f030, 0x1f093, 1},
{0x1f0a0, 0x1f0ae, 1},
{0x1f0b1, 0x1f0be, 1},
{0x1f0c1, 0x1f0cf, 1},
{0x1f0d1, 0x1f0df, 1},
{0x1f100, 0x1f10a, 1},
{0x1f110, 0x1f12e, 1},
{0x1f130, 0x1f169, 1},
{0x1f170, 0x1f19a, 1},
{0x1f1e6, 0x1f1ff, 1},
{0x1f201, 0x1f202, 1},
{0x1f210, 0x1f23a, 1},
{0x1f240, 0x1f248, 1},
{0x1f250, 0x1f251, 1},
{0x1f300, 0x1f320, 1},
{0x1f330, 0x1f335, 1},
{0x1f337, 0x1f37c, 1},
{0x1f380, 0x1f393, 1},
{0x1f3a0, 0x1f3c4, 1},
{0x1f3c6, 0x1f3ca, 1},
{0x1f3e0, 0x1f3f0, 1},
{0x1f400, 0x1f43e, 1},
{0x1f440, 0x1f440, 1},
{0x1f442, 0x1f4f7, 1},
{0x1f4f9, 0x1f4fc, 1},
{0x1f500, 0x1f53d, 1},
{0x1f550, 0x1f567, 1},
{0x1f5fb, 0x1f5ff, 1},
{0x1f601, 0x1f610, 1},
{0x1f612, 0x1f614, 1},
{0x1f616, 0x1f616, 1},
{0x1f618, 0x1f618, 1},
{0x1f61a, 0x1f61a, 1},
{0x1f61c, 0x1f61e, 1},
{0x1f620, 0x1f625, 1},
{0x1f628, 0x1f62b, 1},
{0x1f62d, 0x1f62d, 1},
{0x1f630, 0x1f633, 1},
{0x1f635, 0x1f640, 1},
{0x1f645, 0x1f64f, 1},
{0x1f680, 0x1f6c5, 1},
{0x1f700, 0x1f773, 1},
{0xe0001, 0xe0001, 1},
{0xe0020, 0xe007f, 1},
};
}
private static final int[][] _Kannada = make_Kannada();
private static int[][] make_Kannada() {
return new int[][] {
{0x0c82, 0x0c83, 1},
{0x0c85, 0x0c8c, 1},
{0x0c8e, 0x0c90, 1},
{0x0c92, 0x0ca8, 1},
{0x0caa, 0x0cb3, 1},
{0x0cb5, 0x0cb9, 1},
{0x0cbc, 0x0cc4, 1},
{0x0cc6, 0x0cc8, 1},
{0x0cca, 0x0ccd, 1},
{0x0cd5, 0x0cd6, 1},
{0x0cde, 0x0cde, 1},
{0x0ce0, 0x0ce3, 1},
{0x0ce6, 0x0cef, 1},
{0x0cf1, 0x0cf2, 1},
};
}
private static final int[][] _Old_Turkic = make_Old_Turkic();
private static int[][] make_Old_Turkic() {
return new int[][] {
{0x10c00, 0x10c48, 1},
};
}
private static final int[][] _Tamil = make_Tamil();
private static int[][] make_Tamil() {
return new int[][] {
{0x0b82, 0x0b83, 1},
{0x0b85, 0x0b8a, 1},
{0x0b8e, 0x0b90, 1},
{0x0b92, 0x0b95, 1},
{0x0b99, 0x0b9a, 1},
{0x0b9c, 0x0b9c, 1},
{0x0b9e, 0x0b9f, 1},
{0x0ba3, 0x0ba4, 1},
{0x0ba8, 0x0baa, 1},
{0x0bae, 0x0bb9, 1},
{0x0bbe, 0x0bc2, 1},
{0x0bc6, 0x0bc8, 1},
{0x0bca, 0x0bcd, 1},
{0x0bd0, 0x0bd0, 1},
{0x0bd7, 0x0bd7, 1},
{0x0be6, 0x0bfa, 1},
};
}
private static final int[][] _Tagalog = make_Tagalog();
private static int[][] make_Tagalog() {
return new int[][] {
{0x1700, 0x170c, 1},
{0x170e, 0x1714, 1},
};
}
private static final int[][] _Brahmi = make_Brahmi();
private static int[][] make_Brahmi() {
return new int[][] {
{0x11000, 0x1104d, 1},
{0x11052, 0x1106f, 1},
};
}
private static final int[][] _Arabic = make_Arabic();
private static int[][] make_Arabic() {
return new int[][] {
{0x0600, 0x0603, 1},
{0x0606, 0x060b, 1},
{0x060d, 0x061a, 1},
{0x061e, 0x061e, 1},
{0x0620, 0x063f, 1},
{0x0641, 0x064a, 1},
{0x0656, 0x065e, 1},
{0x066a, 0x066f, 1},
{0x0671, 0x06dc, 1},
{0x06de, 0x06ff, 1},
{0x0750, 0x077f, 1},
{0xfb50, 0xfbc1, 1},
{0xfbd3, 0xfd3d, 1},
{0xfd50, 0xfd8f, 1},
{0xfd92, 0xfdc7, 1},
{0xfdf0, 0xfdfc, 1},
{0xfe70, 0xfe74, 1},
{0xfe76, 0xfefc, 1},
{0x10e60, 0x10e7e, 1},
};
}
private static final int[][] _Tagbanwa = make_Tagbanwa();
private static int[][] make_Tagbanwa() {
return new int[][] {
{0x1760, 0x176c, 1},
{0x176e, 0x1770, 1},
{0x1772, 0x1773, 1},
};
}
private static final int[][] _Canadian_Aboriginal = make_Canadian_Aboriginal();
private static int[][] make_Canadian_Aboriginal() {
return new int[][] {
{0x1400, 0x167f, 1},
{0x18b0, 0x18f5, 1},
};
}
private static final int[][] _Tibetan = make_Tibetan();
private static int[][] make_Tibetan() {
return new int[][] {
{0x0f00, 0x0f47, 1},
{0x0f49, 0x0f6c, 1},
{0x0f71, 0x0f97, 1},
{0x0f99, 0x0fbc, 1},
{0x0fbe, 0x0fcc, 1},
{0x0fce, 0x0fd4, 1},
{0x0fd9, 0x0fda, 1},
};
}
private static final int[][] _Coptic = make_Coptic();
private static int[][] make_Coptic() {
return new int[][] {
{0x03e2, 0x03ef, 1},
{0x2c80, 0x2cf1, 1},
{0x2cf9, 0x2cff, 1},
};
}
private static final int[][] _Hiragana = make_Hiragana();
private static int[][] make_Hiragana() {
return new int[][] {
{0x3041, 0x3096, 1},
{0x309d, 0x309f, 1},
{0x1b001, 0x1b001, 1},
{0x1f200, 0x1f200, 1},
};
}
private static final int[][] _Limbu = make_Limbu();
private static int[][] make_Limbu() {
return new int[][] {
{0x1900, 0x191c, 1},
{0x1920, 0x192b, 1},
{0x1930, 0x193b, 1},
{0x1940, 0x1940, 1},
{0x1944, 0x194f, 1},
};
}
private static final int[][] _Egyptian_Hieroglyphs = make_Egyptian_Hieroglyphs();
private static int[][] make_Egyptian_Hieroglyphs() {
return new int[][] {
{0x13000, 0x1342e, 1},
};
}
private static final int[][] _Avestan = make_Avestan();
private static int[][] make_Avestan() {
return new int[][] {
{0x10b00, 0x10b35, 1},
{0x10b39, 0x10b3f, 1},
};
}
private static final int[][] _Myanmar = make_Myanmar();
private static int[][] make_Myanmar() {
return new int[][] {
{0x1000, 0x109f, 1},
{0xaa60, 0xaa7b, 1},
};
}
private static final int[][] _Armenian = make_Armenian();
private static int[][] make_Armenian() {
return new int[][] {
{0x0531, 0x0556, 1},
{0x0559, 0x055f, 1},
{0x0561, 0x0587, 1},
{0x058a, 0x058a, 1},
{0xfb13, 0xfb17, 1},
};
}
private static final int[][] _Sinhala = make_Sinhala();
private static int[][] make_Sinhala() {
return new int[][] {
{0x0d82, 0x0d83, 1},
{0x0d85, 0x0d96, 1},
{0x0d9a, 0x0db1, 1},
{0x0db3, 0x0dbb, 1},
{0x0dbd, 0x0dbd, 1},
{0x0dc0, 0x0dc6, 1},
{0x0dca, 0x0dca, 1},
{0x0dcf, 0x0dd4, 1},
{0x0dd6, 0x0dd6, 1},
{0x0dd8, 0x0ddf, 1},
{0x0df2, 0x0df4, 1},
};
}
private static final int[][] _Bengali = make_Bengali();
private static int[][] make_Bengali() {
return new int[][] {
{0x0981, 0x0983, 1},
{0x0985, 0x098c, 1},
{0x098f, 0x0990, 1},
{0x0993, 0x09a8, 1},
{0x09aa, 0x09b0, 1},
{0x09b2, 0x09b2, 1},
{0x09b6, 0x09b9, 1},
{0x09bc, 0x09c4, 1},
{0x09c7, 0x09c8, 1},
{0x09cb, 0x09ce, 1},
{0x09d7, 0x09d7, 1},
{0x09dc, 0x09dd, 1},
{0x09df, 0x09e3, 1},
{0x09e6, 0x09fb, 1},
};
}
private static final int[][] _Greek = make_Greek();
private static int[][] make_Greek() {
return new int[][] {
{0x0370, 0x0373, 1},
{0x0375, 0x0377, 1},
{0x037a, 0x037d, 1},
{0x0384, 0x0384, 1},
{0x0386, 0x0386, 1},
{0x0388, 0x038a, 1},
{0x038c, 0x038c, 1},
{0x038e, 0x03a1, 1},
{0x03a3, 0x03e1, 1},
{0x03f0, 0x03ff, 1},
{0x1d26, 0x1d2a, 1},
{0x1d5d, 0x1d61, 1},
{0x1d66, 0x1d6a, 1},
{0x1dbf, 0x1dbf, 1},
{0x1f00, 0x1f15, 1},
{0x1f18, 0x1f1d, 1},
{0x1f20, 0x1f45, 1},
{0x1f48, 0x1f4d, 1},
{0x1f50, 0x1f57, 1},
{0x1f59, 0x1f59, 1},
{0x1f5b, 0x1f5b, 1},
{0x1f5d, 0x1f5d, 1},
{0x1f5f, 0x1f7d, 1},
{0x1f80, 0x1fb4, 1},
{0x1fb6, 0x1fc4, 1},
{0x1fc6, 0x1fd3, 1},
{0x1fd6, 0x1fdb, 1},
{0x1fdd, 0x1fef, 1},
{0x1ff2, 0x1ff4, 1},
{0x1ff6, 0x1ffe, 1},
{0x2126, 0x2126, 1},
{0x10140, 0x1018a, 1},
{0x1d200, 0x1d245, 1},
};
}
private static final int[][] _Cham = make_Cham();
private static int[][] make_Cham() {
return new int[][] {
{0xaa00, 0xaa36, 1},
{0xaa40, 0xaa4d, 1},
{0xaa50, 0xaa59, 1},
{0xaa5c, 0xaa5f, 1},
};
}
private static final int[][] _Hebrew = make_Hebrew();
private static int[][] make_Hebrew() {
return new int[][] {
{0x0591, 0x05c7, 1},
{0x05d0, 0x05ea, 1},
{0x05f0, 0x05f4, 1},
{0xfb1d, 0xfb36, 1},
{0xfb38, 0xfb3c, 1},
{0xfb3e, 0xfb3e, 1},
{0xfb40, 0xfb41, 1},
{0xfb43, 0xfb44, 1},
{0xfb46, 0xfb4f, 1},
};
}
private static final int[][] _Meetei_Mayek = make_Meetei_Mayek();
private static int[][] make_Meetei_Mayek() {
return new int[][] {
{0xabc0, 0xabed, 1},
{0xabf0, 0xabf9, 1},
};
}
private static final int[][] _Saurashtra = make_Saurashtra();
private static int[][] make_Saurashtra() {
return new int[][] {
{0xa880, 0xa8c4, 1},
{0xa8ce, 0xa8d9, 1},
};
}
private static final int[][] _Hangul = make_Hangul();
private static int[][] make_Hangul() {
return new int[][] {
{0x1100, 0x11ff, 1},
{0x302e, 0x302f, 1},
{0x3131, 0x318e, 1},
{0x3200, 0x321e, 1},
{0x3260, 0x327e, 1},
{0xa960, 0xa97c, 1},
{0xac00, 0xd7a3, 1},
{0xd7b0, 0xd7c6, 1},
{0xd7cb, 0xd7fb, 1},
{0xffa0, 0xffbe, 1},
{0xffc2, 0xffc7, 1},
{0xffca, 0xffcf, 1},
{0xffd2, 0xffd7, 1},
{0xffda, 0xffdc, 1},
};
}
private static final int[][] _Runic = make_Runic();
private static int[][] make_Runic() {
return new int[][] {
{0x16a0, 0x16ea, 1},
{0x16ee, 0x16f0, 1},
};
}
private static final int[][] _Deseret = make_Deseret();
private static int[][] make_Deseret() {
return new int[][] {
{0x10400, 0x1044f, 1},
};
}
private static final int[][] _Lisu = make_Lisu();
private static int[][] make_Lisu() {
return new int[][] {
{0xa4d0, 0xa4ff, 1},
};
}
private static final int[][] _Sundanese = make_Sundanese();
private static int[][] make_Sundanese() {
return new int[][] {
{0x1b80, 0x1baa, 1},
{0x1bae, 0x1bb9, 1},
};
}
private static final int[][] _Glagolitic = make_Glagolitic();
private static int[][] make_Glagolitic() {
return new int[][] {
{0x2c00, 0x2c2e, 1},
{0x2c30, 0x2c5e, 1},
};
}
private static final int[][] _Oriya = make_Oriya();
private static int[][] make_Oriya() {
return new int[][] {
{0x0b01, 0x0b03, 1},
{0x0b05, 0x0b0c, 1},
{0x0b0f, 0x0b10, 1},
{0x0b13, 0x0b28, 1},
{0x0b2a, 0x0b30, 1},
{0x0b32, 0x0b33, 1},
{0x0b35, 0x0b39, 1},
{0x0b3c, 0x0b44, 1},
{0x0b47, 0x0b48, 1},
{0x0b4b, 0x0b4d, 1},
{0x0b56, 0x0b57, 1},
{0x0b5c, 0x0b5d, 1},
{0x0b5f, 0x0b63, 1},
{0x0b66, 0x0b77, 1},
};
}
private static final int[][] _Buhid = make_Buhid();
private static int[][] make_Buhid() {
return new int[][] {
{0x1740, 0x1753, 1},
};
}
private static final int[][] _Ethiopic = make_Ethiopic();
private static int[][] make_Ethiopic() {
return new int[][] {
{0x1200, 0x1248, 1},
{0x124a, 0x124d, 1},
{0x1250, 0x1256, 1},
{0x1258, 0x1258, 1},
{0x125a, 0x125d, 1},
{0x1260, 0x1288, 1},
{0x128a, 0x128d, 1},
{0x1290, 0x12b0, 1},
{0x12b2, 0x12b5, 1},
{0x12b8, 0x12be, 1},
{0x12c0, 0x12c0, 1},
{0x12c2, 0x12c5, 1},
{0x12c8, 0x12d6, 1},
{0x12d8, 0x1310, 1},
{0x1312, 0x1315, 1},
{0x1318, 0x135a, 1},
{0x135d, 0x137c, 1},
{0x1380, 0x1399, 1},
{0x2d80, 0x2d96, 1},
{0x2da0, 0x2da6, 1},
{0x2da8, 0x2dae, 1},
{0x2db0, 0x2db6, 1},
{0x2db8, 0x2dbe, 1},
{0x2dc0, 0x2dc6, 1},
{0x2dc8, 0x2dce, 1},
{0x2dd0, 0x2dd6, 1},
{0x2dd8, 0x2dde, 1},
{0xab01, 0xab06, 1},
{0xab09, 0xab0e, 1},
{0xab11, 0xab16, 1},
{0xab20, 0xab26, 1},
{0xab28, 0xab2e, 1},
};
}
private static final int[][] _Javanese = make_Javanese();
private static int[][] make_Javanese() {
return new int[][] {
{0xa980, 0xa9cd, 1},
{0xa9cf, 0xa9d9, 1},
{0xa9de, 0xa9df, 1},
};
}
private static final int[][] _Syloti_Nagri = make_Syloti_Nagri();
private static int[][] make_Syloti_Nagri() {
return new int[][] {
{0xa800, 0xa82b, 1},
};
}
private static final int[][] _Vai = make_Vai();
private static int[][] make_Vai() {
return new int[][] {
{0xa500, 0xa62b, 1},
};
}
private static final int[][] _Cherokee = make_Cherokee();
private static int[][] make_Cherokee() {
return new int[][] {
{0x13a0, 0x13f4, 1},
};
}
private static final int[][] _Ogham = make_Ogham();
private static int[][] make_Ogham() {
return new int[][] {
{0x1680, 0x169c, 1},
};
}
private static final int[][] _Batak = make_Batak();
private static int[][] make_Batak() {
return new int[][] {
{0x1bc0, 0x1bf3, 1},
{0x1bfc, 0x1bff, 1},
};
}
private static final int[][] _Syriac = make_Syriac();
private static int[][] make_Syriac() {
return new int[][] {
{0x0700, 0x070d, 1},
{0x070f, 0x074a, 1},
{0x074d, 0x074f, 1},
};
}
private static final int[][] _Gurmukhi = make_Gurmukhi();
private static int[][] make_Gurmukhi() {
return new int[][] {
{0x0a01, 0x0a03, 1},
{0x0a05, 0x0a0a, 1},
{0x0a0f, 0x0a10, 1},
{0x0a13, 0x0a28, 1},
{0x0a2a, 0x0a30, 1},
{0x0a32, 0x0a33, 1},
{0x0a35, 0x0a36, 1},
{0x0a38, 0x0a39, 1},
{0x0a3c, 0x0a3c, 1},
{0x0a3e, 0x0a42, 1},
{0x0a47, 0x0a48, 1},
{0x0a4b, 0x0a4d, 1},
{0x0a51, 0x0a51, 1},
{0x0a59, 0x0a5c, 1},
{0x0a5e, 0x0a5e, 1},
{0x0a66, 0x0a75, 1},
};
}
private static final int[][] _Tai_Tham = make_Tai_Tham();
private static int[][] make_Tai_Tham() {
return new int[][] {
{0x1a20, 0x1a5e, 1},
{0x1a60, 0x1a7c, 1},
{0x1a7f, 0x1a89, 1},
{0x1a90, 0x1a99, 1},
{0x1aa0, 0x1aad, 1},
};
}
private static final int[][] _Ol_Chiki = make_Ol_Chiki();
private static int[][] make_Ol_Chiki() {
return new int[][] {
{0x1c50, 0x1c7f, 1},
};
}
private static final int[][] _Mongolian = make_Mongolian();
private static int[][] make_Mongolian() {
return new int[][] {
{0x1800, 0x1801, 1},
{0x1804, 0x1804, 1},
{0x1806, 0x180e, 1},
{0x1810, 0x1819, 1},
{0x1820, 0x1877, 1},
{0x1880, 0x18aa, 1},
};
}
private static final int[][] _Hanunoo = make_Hanunoo();
private static int[][] make_Hanunoo() {
return new int[][] {
{0x1720, 0x1734, 1},
};
}
private static final int[][] _Cypriot = make_Cypriot();
private static int[][] make_Cypriot() {
return new int[][] {
{0x10800, 0x10805, 1},
{0x10808, 0x10808, 1},
{0x1080a, 0x10835, 1},
{0x10837, 0x10838, 1},
{0x1083c, 0x1083c, 1},
{0x1083f, 0x1083f, 1},
};
}
private static final int[][] _Buginese = make_Buginese();
private static int[][] make_Buginese() {
return new int[][] {
{0x1a00, 0x1a1b, 1},
{0x1a1e, 0x1a1f, 1},
};
}
private static final int[][] _Bamum = make_Bamum();
private static int[][] make_Bamum() {
return new int[][] {
{0xa6a0, 0xa6f7, 1},
{0x16800, 0x16a38, 1},
};
}
private static final int[][] _Lepcha = make_Lepcha();
private static int[][] make_Lepcha() {
return new int[][] {
{0x1c00, 0x1c37, 1},
{0x1c3b, 0x1c49, 1},
{0x1c4d, 0x1c4f, 1},
};
}
private static final int[][] _Thaana = make_Thaana();
private static int[][] make_Thaana() {
return new int[][] {
{0x0780, 0x07b1, 1},
};
}
private static final int[][] _Old_Persian = make_Old_Persian();
private static int[][] make_Old_Persian() {
return new int[][] {
{0x103a0, 0x103c3, 1},
{0x103c8, 0x103d5, 1},
};
}
private static final int[][] _Cuneiform = make_Cuneiform();
private static int[][] make_Cuneiform() {
return new int[][] {
{0x12000, 0x1236e, 1},
{0x12400, 0x12462, 1},
{0x12470, 0x12473, 1},
};
}
private static final int[][] _Rejang = make_Rejang();
private static int[][] make_Rejang() {
return new int[][] {
{0xa930, 0xa953, 1},
{0xa95f, 0xa95f, 1},
};
}
private static final int[][] _Georgian = make_Georgian();
private static int[][] make_Georgian() {
return new int[][] {
{0x10a0, 0x10c5, 1},
{0x10d0, 0x10fa, 1},
{0x10fc, 0x10fc, 1},
{0x2d00, 0x2d25, 1},
};
}
private static final int[][] _Shavian = make_Shavian();
private static int[][] make_Shavian() {
return new int[][] {
{0x10450, 0x1047f, 1},
};
}
private static final int[][] _Lycian = make_Lycian();
private static int[][] make_Lycian() {
return new int[][] {
{0x10280, 0x1029c, 1},
};
}
private static final int[][] _Nko = make_Nko();
private static int[][] make_Nko() {
return new int[][] {
{0x07c0, 0x07fa, 1},
};
}
private static final int[][] _Yi = make_Yi();
private static int[][] make_Yi() {
return new int[][] {
{0xa000, 0xa48c, 1},
{0xa490, 0xa4c6, 1},
};
}
private static final int[][] _Lao = make_Lao();
private static int[][] make_Lao() {
return new int[][] {
{0x0e81, 0x0e82, 1},
{0x0e84, 0x0e84, 1},
{0x0e87, 0x0e88, 1},
{0x0e8a, 0x0e8a, 1},
{0x0e8d, 0x0e8d, 1},
{0x0e94, 0x0e97, 1},
{0x0e99, 0x0e9f, 1},
{0x0ea1, 0x0ea3, 1},
{0x0ea5, 0x0ea5, 1},
{0x0ea7, 0x0ea7, 1},
{0x0eaa, 0x0eab, 1},
{0x0ead, 0x0eb9, 1},
{0x0ebb, 0x0ebd, 1},
{0x0ec0, 0x0ec4, 1},
{0x0ec6, 0x0ec6, 1},
{0x0ec8, 0x0ecd, 1},
{0x0ed0, 0x0ed9, 1},
{0x0edc, 0x0edd, 1},
};
}
private static final int[][] _Linear_B = make_Linear_B();
private static int[][] make_Linear_B() {
return new int[][] {
{0x10000, 0x1000b, 1},
{0x1000d, 0x10026, 1},
{0x10028, 0x1003a, 1},
{0x1003c, 0x1003d, 1},
{0x1003f, 0x1004d, 1},
{0x10050, 0x1005d, 1},
{0x10080, 0x100fa, 1},
};
}
private static final int[][] _Old_Italic = make_Old_Italic();
private static int[][] make_Old_Italic() {
return new int[][] {
{0x10300, 0x1031e, 1},
{0x10320, 0x10323, 1},
};
}
private static final int[][] _Tai_Viet = make_Tai_Viet();
private static int[][] make_Tai_Viet() {
return new int[][] {
{0xaa80, 0xaac2, 1},
{0xaadb, 0xaadf, 1},
};
}
private static final int[][] _Devanagari = make_Devanagari();
private static int[][] make_Devanagari() {
return new int[][] {
{0x0900, 0x0950, 1},
{0x0953, 0x0963, 1},
{0x0966, 0x096f, 1},
{0x0971, 0x0977, 1},
{0x0979, 0x097f, 1},
{0xa8e0, 0xa8fb, 1},
};
}
private static final int[][] _Lydian = make_Lydian();
private static int[][] make_Lydian() {
return new int[][] {
{0x10920, 0x10939, 1},
{0x1093f, 0x1093f, 1},
};
}
private static final int[][] _Tifinagh = make_Tifinagh();
private static int[][] make_Tifinagh() {
return new int[][] {
{0x2d30, 0x2d65, 1},
{0x2d6f, 0x2d70, 1},
{0x2d7f, 0x2d7f, 1},
};
}
private static final int[][] _Ugaritic = make_Ugaritic();
private static int[][] make_Ugaritic() {
return new int[][] {
{0x10380, 0x1039d, 1},
{0x1039f, 0x1039f, 1},
};
}
private static final int[][] _Thai = make_Thai();
private static int[][] make_Thai() {
return new int[][] {
{0x0e01, 0x0e3a, 1},
{0x0e40, 0x0e5b, 1},
};
}
private static final int[][] _Cyrillic = make_Cyrillic();
private static int[][] make_Cyrillic() {
return new int[][] {
{0x0400, 0x0484, 1},
{0x0487, 0x0527, 1},
{0x1d2b, 0x1d2b, 1},
{0x1d78, 0x1d78, 1},
{0x2de0, 0x2dff, 1},
{0xa640, 0xa673, 1},
{0xa67c, 0xa697, 1},
};
}
private static final int[][] _Gujarati = make_Gujarati();
private static int[][] make_Gujarati() {
return new int[][] {
{0x0a81, 0x0a83, 1},
{0x0a85, 0x0a8d, 1},
{0x0a8f, 0x0a91, 1},
{0x0a93, 0x0aa8, 1},
{0x0aaa, 0x0ab0, 1},
{0x0ab2, 0x0ab3, 1},
{0x0ab5, 0x0ab9, 1},
{0x0abc, 0x0ac5, 1},
{0x0ac7, 0x0ac9, 1},
{0x0acb, 0x0acd, 1},
{0x0ad0, 0x0ad0, 1},
{0x0ae0, 0x0ae3, 1},
{0x0ae6, 0x0aef, 1},
{0x0af1, 0x0af1, 1},
};
}
private static final int[][] _Carian = make_Carian();
private static int[][] make_Carian() {
return new int[][] {
{0x102a0, 0x102d0, 1},
};
}
private static final int[][] _Phoenician = make_Phoenician();
private static int[][] make_Phoenician() {
return new int[][] {
{0x10900, 0x1091b, 1},
{0x1091f, 0x1091f, 1},
};
}
private static final int[][] _Balinese = make_Balinese();
private static int[][] make_Balinese() {
return new int[][] {
{0x1b00, 0x1b4b, 1},
{0x1b50, 0x1b7c, 1},
};
}
private static final int[][] _Braille = make_Braille();
private static int[][] make_Braille() {
return new int[][] {
{0x2800, 0x28ff, 1},
};
}
private static final int[][] _Han = make_Han();
private static int[][] make_Han() {
return new int[][] {
{0x2e80, 0x2e99, 1},
{0x2e9b, 0x2ef3, 1},
{0x2f00, 0x2fd5, 1},
{0x3005, 0x3005, 1},
{0x3007, 0x3007, 1},
{0x3021, 0x3029, 1},
{0x3038, 0x303b, 1},
{0x3400, 0x4db5, 1},
{0x4e00, 0x9fcb, 1},
{0xf900, 0xfa2d, 1},
{0xfa30, 0xfa6d, 1},
{0xfa70, 0xfad9, 1},
{0x20000, 0x2a6d6, 1},
{0x2a700, 0x2b734, 1},
{0x2b740, 0x2b81d, 1},
{0x2f800, 0x2fa1d, 1},
};
}
private static final int[][] _Gothic = make_Gothic();
private static int[][] make_Gothic() {
return new int[][] {
{0x10330, 0x1034a, 1},
};
}
static final int[][] Arabic = _Arabic;
static final int[][] Armenian = _Armenian;
static final int[][] Avestan = _Avestan;
static final int[][] Balinese = _Balinese;
static final int[][] Bamum = _Bamum;
static final int[][] Batak = _Batak;
static final int[][] Bengali = _Bengali;
static final int[][] Bopomofo = _Bopomofo;
static final int[][] Brahmi = _Brahmi;
static final int[][] Braille = _Braille;
static final int[][] Buginese = _Buginese;
static final int[][] Buhid = _Buhid;
static final int[][] Canadian_Aboriginal = _Canadian_Aboriginal;
static final int[][] Carian = _Carian;
static final int[][] Cham = _Cham;
static final int[][] Cherokee = _Cherokee;
static final int[][] Common = _Common;
static final int[][] Coptic = _Coptic;
static final int[][] Cuneiform = _Cuneiform;
static final int[][] Cypriot = _Cypriot;
static final int[][] Cyrillic = _Cyrillic;
static final int[][] Deseret = _Deseret;
static final int[][] Devanagari = _Devanagari;
static final int[][] Egyptian_Hieroglyphs = _Egyptian_Hieroglyphs;
static final int[][] Ethiopic = _Ethiopic;
static final int[][] Georgian = _Georgian;
static final int[][] Glagolitic = _Glagolitic;
static final int[][] Gothic = _Gothic;
static final int[][] Greek = _Greek;
static final int[][] Gujarati = _Gujarati;
static final int[][] Gurmukhi = _Gurmukhi;
static final int[][] Han = _Han;
static final int[][] Hangul = _Hangul;
static final int[][] Hanunoo = _Hanunoo;
static final int[][] Hebrew = _Hebrew;
static final int[][] Hiragana = _Hiragana;
static final int[][] Imperial_Aramaic = _Imperial_Aramaic;
static final int[][] Inherited = _Inherited;
static final int[][] Inscriptional_Pahlavi = _Inscriptional_Pahlavi;
static final int[][] Inscriptional_Parthian = _Inscriptional_Parthian;
static final int[][] Javanese = _Javanese;
static final int[][] Kaithi = _Kaithi;
static final int[][] Kannada = _Kannada;
static final int[][] Katakana = _Katakana;
static final int[][] Kayah_Li = _Kayah_Li;
static final int[][] Kharoshthi = _Kharoshthi;
static final int[][] Khmer = _Khmer;
static final int[][] Lao = _Lao;
static final int[][] Latin = _Latin;
static final int[][] Lepcha = _Lepcha;
static final int[][] Limbu = _Limbu;
static final int[][] Linear_B = _Linear_B;
static final int[][] Lisu = _Lisu;
static final int[][] Lycian = _Lycian;
static final int[][] Lydian = _Lydian;
static final int[][] Malayalam = _Malayalam;
static final int[][] Mandaic = _Mandaic;
static final int[][] Meetei_Mayek = _Meetei_Mayek;
static final int[][] Mongolian = _Mongolian;
static final int[][] Myanmar = _Myanmar;
static final int[][] New_Tai_Lue = _New_Tai_Lue;
static final int[][] Nko = _Nko;
static final int[][] Ogham = _Ogham;
static final int[][] Ol_Chiki = _Ol_Chiki;
static final int[][] Old_Italic = _Old_Italic;
static final int[][] Old_Persian = _Old_Persian;
static final int[][] Old_South_Arabian = _Old_South_Arabian;
static final int[][] Old_Turkic = _Old_Turkic;
static final int[][] Oriya = _Oriya;
static final int[][] Osmanya = _Osmanya;
static final int[][] Phags_Pa = _Phags_Pa;
static final int[][] Phoenician = _Phoenician;
static final int[][] Rejang = _Rejang;
static final int[][] Runic = _Runic;
static final int[][] Samaritan = _Samaritan;
static final int[][] Saurashtra = _Saurashtra;
static final int[][] Shavian = _Shavian;
static final int[][] Sinhala = _Sinhala;
static final int[][] Sundanese = _Sundanese;
static final int[][] Syloti_Nagri = _Syloti_Nagri;
static final int[][] Syriac = _Syriac;
static final int[][] Tagalog = _Tagalog;
static final int[][] Tagbanwa = _Tagbanwa;
static final int[][] Tai_Le = _Tai_Le;
static final int[][] Tai_Tham = _Tai_Tham;
static final int[][] Tai_Viet = _Tai_Viet;
static final int[][] Tamil = _Tamil;
static final int[][] Telugu = _Telugu;
static final int[][] Thaana = _Thaana;
static final int[][] Thai = _Thai;
static final int[][] Tibetan = _Tibetan;
static final int[][] Tifinagh = _Tifinagh;
static final int[][] Ugaritic = _Ugaritic;
static final int[][] Vai = _Vai;
static final int[][] Yi = _Yi;
private static Map Properties() {
Map map = new HashMap();
map.put("Pattern_Syntax", Pattern_Syntax);
map.put("Other_ID_Start", Other_ID_Start);
map.put("Pattern_White_Space", Pattern_White_Space);
map.put("Other_Lowercase", Other_Lowercase);
map.put("Soft_Dotted", Soft_Dotted);
map.put("Hex_Digit", Hex_Digit);
map.put("ASCII_Hex_Digit", ASCII_Hex_Digit);
map.put("Deprecated", Deprecated);
map.put("Terminal_Punctuation", Terminal_Punctuation);
map.put("Quotation_Mark", Quotation_Mark);
map.put("Other_ID_Continue", Other_ID_Continue);
map.put("Bidi_Control", Bidi_Control);
map.put("Variation_Selector", Variation_Selector);
map.put("Noncharacter_Code_Point", Noncharacter_Code_Point);
map.put("Other_Math", Other_Math);
map.put("Unified_Ideograph", Unified_Ideograph);
map.put("Hyphen", Hyphen);
map.put("IDS_Binary_Operator", IDS_Binary_Operator);
map.put("Logical_Order_Exception", Logical_Order_Exception);
map.put("Radical", Radical);
map.put("Other_Uppercase", Other_Uppercase);
map.put("STerm", STerm);
map.put("Other_Alphabetic", Other_Alphabetic);
map.put("Diacritic", Diacritic);
map.put("Extender", Extender);
map.put("Join_Control", Join_Control);
map.put("Ideographic", Ideographic);
map.put("Dash", Dash);
map.put("IDS_Trinary_Operator", IDS_Trinary_Operator);
map.put("Other_Grapheme_Extend", Other_Grapheme_Extend);
map.put("Other_Default_Ignorable_Code_Point", Other_Default_Ignorable_Code_Point);
map.put("White_Space", White_Space);
return map;
}
private static final int[][] _Pattern_Syntax = make_Pattern_Syntax();
private static int[][] make_Pattern_Syntax() {
return new int[][] {
{0x0021, 0x002f, 1},
{0x003a, 0x0040, 1},
{0x005b, 0x005e, 1},
{0x0060, 0x0060, 1},
{0x007b, 0x007e, 1},
{0x00a1, 0x00a7, 1},
{0x00a9, 0x00a9, 1},
{0x00ab, 0x00ac, 1},
{0x00ae, 0x00ae, 1},
{0x00b0, 0x00b1, 1},
{0x00b6, 0x00b6, 1},
{0x00bb, 0x00bb, 1},
{0x00bf, 0x00bf, 1},
{0x00d7, 0x00d7, 1},
{0x00f7, 0x00f7, 1},
{0x2010, 0x2027, 1},
{0x2030, 0x203e, 1},
{0x2041, 0x2053, 1},
{0x2055, 0x205e, 1},
{0x2190, 0x245f, 1},
{0x2500, 0x2775, 1},
{0x2794, 0x2bff, 1},
{0x2e00, 0x2e7f, 1},
{0x3001, 0x3003, 1},
{0x3008, 0x3020, 1},
{0x3030, 0x3030, 1},
{0xfd3e, 0xfd3f, 1},
{0xfe45, 0xfe46, 1},
};
}
private static final int[][] _Other_ID_Start = make_Other_ID_Start();
private static int[][] make_Other_ID_Start() {
return new int[][] {
{0x2118, 0x2118, 1},
{0x212e, 0x212e, 1},
{0x309b, 0x309c, 1},
};
}
private static final int[][] _Pattern_White_Space = make_Pattern_White_Space();
private static int[][] make_Pattern_White_Space() {
return new int[][] {
{0x0009, 0x000d, 1},
{0x0020, 0x0020, 1},
{0x0085, 0x0085, 1},
{0x200e, 0x200f, 1},
{0x2028, 0x2029, 1},
};
}
private static final int[][] _Other_Lowercase = make_Other_Lowercase();
private static int[][] make_Other_Lowercase() {
return new int[][] {
{0x02b0, 0x02b8, 1},
{0x02c0, 0x02c1, 1},
{0x02e0, 0x02e4, 1},
{0x0345, 0x0345, 1},
{0x037a, 0x037a, 1},
{0x1d2c, 0x1d61, 1},
{0x1d78, 0x1d78, 1},
{0x1d9b, 0x1dbf, 1},
{0x2090, 0x2094, 1},
{0x2170, 0x217f, 1},
{0x24d0, 0x24e9, 1},
{0x2c7d, 0x2c7d, 1},
{0xa770, 0xa770, 1},
};
}
private static final int[][] _Soft_Dotted = make_Soft_Dotted();
private static int[][] make_Soft_Dotted() {
return new int[][] {
{0x0069, 0x006a, 1},
{0x012f, 0x012f, 1},
{0x0249, 0x0249, 1},
{0x0268, 0x0268, 1},
{0x029d, 0x029d, 1},
{0x02b2, 0x02b2, 1},
{0x03f3, 0x03f3, 1},
{0x0456, 0x0456, 1},
{0x0458, 0x0458, 1},
{0x1d62, 0x1d62, 1},
{0x1d96, 0x1d96, 1},
{0x1da4, 0x1da4, 1},
{0x1da8, 0x1da8, 1},
{0x1e2d, 0x1e2d, 1},
{0x1ecb, 0x1ecb, 1},
{0x2071, 0x2071, 1},
{0x2148, 0x2149, 1},
{0x2c7c, 0x2c7c, 1},
{0x1d422, 0x1d423, 1},
{0x1d456, 0x1d457, 1},
{0x1d48a, 0x1d48b, 1},
{0x1d4be, 0x1d4bf, 1},
{0x1d4f2, 0x1d4f3, 1},
{0x1d526, 0x1d527, 1},
{0x1d55a, 0x1d55b, 1},
{0x1d58e, 0x1d58f, 1},
{0x1d5c2, 0x1d5c3, 1},
{0x1d5f6, 0x1d5f7, 1},
{0x1d62a, 0x1d62b, 1},
{0x1d65e, 0x1d65f, 1},
{0x1d692, 0x1d693, 1},
};
}
private static final int[][] _Hex_Digit = make_Hex_Digit();
private static int[][] make_Hex_Digit() {
return new int[][] {
{0x0030, 0x0039, 1},
{0x0041, 0x0046, 1},
{0x0061, 0x0066, 1},
{0xff10, 0xff19, 1},
{0xff21, 0xff26, 1},
{0xff41, 0xff46, 1},
};
}
private static final int[][] _ASCII_Hex_Digit = make_ASCII_Hex_Digit();
private static int[][] make_ASCII_Hex_Digit() {
return new int[][] {
{0x0030, 0x0039, 1},
{0x0041, 0x0046, 1},
{0x0061, 0x0066, 1},
};
}
private static final int[][] _Deprecated = make_Deprecated();
private static int[][] make_Deprecated() {
return new int[][] {
{0x0149, 0x0149, 1},
{0x0673, 0x0673, 1},
{0x0f77, 0x0f77, 1},
{0x0f79, 0x0f79, 1},
{0x17a3, 0x17a4, 1},
{0x206a, 0x206f, 1},
{0x2329, 0x232a, 1},
{0xe0001, 0xe0001, 1},
{0xe0020, 0xe007f, 1},
};
}
private static final int[][] _Terminal_Punctuation = make_Terminal_Punctuation();
private static int[][] make_Terminal_Punctuation() {
return new int[][] {
{0x0021, 0x0021, 1},
{0x002c, 0x002c, 1},
{0x002e, 0x002e, 1},
{0x003a, 0x003b, 1},
{0x003f, 0x003f, 1},
{0x037e, 0x037e, 1},
{0x0387, 0x0387, 1},
{0x0589, 0x0589, 1},
{0x05c3, 0x05c3, 1},
{0x060c, 0x060c, 1},
{0x061b, 0x061b, 1},
{0x061f, 0x061f, 1},
{0x06d4, 0x06d4, 1},
{0x0700, 0x070a, 1},
{0x070c, 0x070c, 1},
{0x07f8, 0x07f9, 1},
{0x0830, 0x083e, 1},
{0x085e, 0x085e, 1},
{0x0964, 0x0965, 1},
{0x0e5a, 0x0e5b, 1},
{0x0f08, 0x0f08, 1},
{0x0f0d, 0x0f12, 1},
{0x104a, 0x104b, 1},
{0x1361, 0x1368, 1},
{0x166d, 0x166e, 1},
{0x16eb, 0x16ed, 1},
{0x17d4, 0x17d6, 1},
{0x17da, 0x17da, 1},
{0x1802, 0x1805, 1},
{0x1808, 0x1809, 1},
{0x1944, 0x1945, 1},
{0x1aa8, 0x1aab, 1},
{0x1b5a, 0x1b5b, 1},
{0x1b5d, 0x1b5f, 1},
{0x1c3b, 0x1c3f, 1},
{0x1c7e, 0x1c7f, 1},
{0x203c, 0x203d, 1},
{0x2047, 0x2049, 1},
{0x2e2e, 0x2e2e, 1},
{0x3001, 0x3002, 1},
{0xa4fe, 0xa4ff, 1},
{0xa60d, 0xa60f, 1},
{0xa6f3, 0xa6f7, 1},
{0xa876, 0xa877, 1},
{0xa8ce, 0xa8cf, 1},
{0xa92f, 0xa92f, 1},
{0xa9c7, 0xa9c9, 1},
{0xaa5d, 0xaa5f, 1},
{0xaadf, 0xaadf, 1},
{0xabeb, 0xabeb, 1},
{0xfe50, 0xfe52, 1},
{0xfe54, 0xfe57, 1},
{0xff01, 0xff01, 1},
{0xff0c, 0xff0c, 1},
{0xff0e, 0xff0e, 1},
{0xff1a, 0xff1b, 1},
{0xff1f, 0xff1f, 1},
{0xff61, 0xff61, 1},
{0xff64, 0xff64, 1},
{0x1039f, 0x1039f, 1},
{0x103d0, 0x103d0, 1},
{0x10857, 0x10857, 1},
{0x1091f, 0x1091f, 1},
{0x10b3a, 0x10b3f, 1},
{0x11047, 0x1104d, 1},
{0x110be, 0x110c1, 1},
{0x12470, 0x12473, 1},
};
}
private static final int[][] _Quotation_Mark = make_Quotation_Mark();
private static int[][] make_Quotation_Mark() {
return new int[][] {
{0x0022, 0x0022, 1},
{0x0027, 0x0027, 1},
{0x00ab, 0x00ab, 1},
{0x00bb, 0x00bb, 1},
{0x2018, 0x201f, 1},
{0x2039, 0x203a, 1},
{0x300c, 0x300f, 1},
{0x301d, 0x301f, 1},
{0xfe41, 0xfe44, 1},
{0xff02, 0xff02, 1},
{0xff07, 0xff07, 1},
{0xff62, 0xff63, 1},
};
}
private static final int[][] _Other_ID_Continue = make_Other_ID_Continue();
private static int[][] make_Other_ID_Continue() {
return new int[][] {
{0x00b7, 0x00b7, 1},
{0x0387, 0x0387, 1},
{0x1369, 0x1371, 1},
{0x19da, 0x19da, 1},
};
}
private static final int[][] _Bidi_Control = make_Bidi_Control();
private static int[][] make_Bidi_Control() {
return new int[][] {
{0x200e, 0x200f, 1},
{0x202a, 0x202e, 1},
};
}
private static final int[][] _Variation_Selector = make_Variation_Selector();
private static int[][] make_Variation_Selector() {
return new int[][] {
{0x180b, 0x180d, 1},
{0xfe00, 0xfe0f, 1},
{0xe0100, 0xe01ef, 1},
};
}
private static final int[][] _Noncharacter_Code_Point = make_Noncharacter_Code_Point();
private static int[][] make_Noncharacter_Code_Point() {
return new int[][] {
{0xfdd0, 0xfdef, 1},
{0xfffe, 0xffff, 1},
{0x1fffe, 0x1ffff, 1},
{0x2fffe, 0x2ffff, 1},
{0x3fffe, 0x3ffff, 1},
{0x4fffe, 0x4ffff, 1},
{0x5fffe, 0x5ffff, 1},
{0x6fffe, 0x6ffff, 1},
{0x7fffe, 0x7ffff, 1},
{0x8fffe, 0x8ffff, 1},
{0x9fffe, 0x9ffff, 1},
{0xafffe, 0xaffff, 1},
{0xbfffe, 0xbffff, 1},
{0xcfffe, 0xcffff, 1},
{0xdfffe, 0xdffff, 1},
{0xefffe, 0xeffff, 1},
{0xffffe, 0xfffff, 1},
{0x10fffe, 0x10ffff, 1},
};
}
private static final int[][] _Other_Math = make_Other_Math();
private static int[][] make_Other_Math() {
return new int[][] {
{0x005e, 0x005e, 1},
{0x03d0, 0x03d2, 1},
{0x03d5, 0x03d5, 1},
{0x03f0, 0x03f1, 1},
{0x03f4, 0x03f5, 1},
{0x2016, 0x2016, 1},
{0x2032, 0x2034, 1},
{0x2040, 0x2040, 1},
{0x2061, 0x2064, 1},
{0x207d, 0x207e, 1},
{0x208d, 0x208e, 1},
{0x20d0, 0x20dc, 1},
{0x20e1, 0x20e1, 1},
{0x20e5, 0x20e6, 1},
{0x20eb, 0x20ef, 1},
{0x2102, 0x2102, 1},
{0x2107, 0x2107, 1},
{0x210a, 0x2113, 1},
{0x2115, 0x2115, 1},
{0x2119, 0x211d, 1},
{0x2124, 0x2124, 1},
{0x2128, 0x2129, 1},
{0x212c, 0x212d, 1},
{0x212f, 0x2131, 1},
{0x2133, 0x2138, 1},
{0x213c, 0x213f, 1},
{0x2145, 0x2149, 1},
{0x2195, 0x2199, 1},
{0x219c, 0x219f, 1},
{0x21a1, 0x21a2, 1},
{0x21a4, 0x21a5, 1},
{0x21a7, 0x21a7, 1},
{0x21a9, 0x21ad, 1},
{0x21b0, 0x21b1, 1},
{0x21b6, 0x21b7, 1},
{0x21bc, 0x21cd, 1},
{0x21d0, 0x21d1, 1},
{0x21d3, 0x21d3, 1},
{0x21d5, 0x21db, 1},
{0x21dd, 0x21dd, 1},
{0x21e4, 0x21e5, 1},
{0x23b4, 0x23b5, 1},
{0x23b7, 0x23b7, 1},
{0x23d0, 0x23d0, 1},
{0x23e2, 0x23e2, 1},
{0x25a0, 0x25a1, 1},
{0x25ae, 0x25b6, 1},
{0x25bc, 0x25c0, 1},
{0x25c6, 0x25c7, 1},
{0x25ca, 0x25cb, 1},
{0x25cf, 0x25d3, 1},
{0x25e2, 0x25e2, 1},
{0x25e4, 0x25e4, 1},
{0x25e7, 0x25ec, 1},
{0x2605, 0x2606, 1},
{0x2640, 0x2640, 1},
{0x2642, 0x2642, 1},
{0x2660, 0x2663, 1},
{0x266d, 0x266e, 1},
{0x27c5, 0x27c6, 1},
{0x27e6, 0x27ef, 1},
{0x2983, 0x2998, 1},
{0x29d8, 0x29db, 1},
{0x29fc, 0x29fd, 1},
{0xfe61, 0xfe61, 1},
{0xfe63, 0xfe63, 1},
{0xfe68, 0xfe68, 1},
{0xff3c, 0xff3c, 1},
{0xff3e, 0xff3e, 1},
{0x1d400, 0x1d454, 1},
{0x1d456, 0x1d49c, 1},
{0x1d49e, 0x1d49f, 1},
{0x1d4a2, 0x1d4a2, 1},
{0x1d4a5, 0x1d4a6, 1},
{0x1d4a9, 0x1d4ac, 1},
{0x1d4ae, 0x1d4b9, 1},
{0x1d4bb, 0x1d4bb, 1},
{0x1d4bd, 0x1d4c3, 1},
{0x1d4c5, 0x1d505, 1},
{0x1d507, 0x1d50a, 1},
{0x1d50d, 0x1d514, 1},
{0x1d516, 0x1d51c, 1},
{0x1d51e, 0x1d539, 1},
{0x1d53b, 0x1d53e, 1},
{0x1d540, 0x1d544, 1},
{0x1d546, 0x1d546, 1},
{0x1d54a, 0x1d550, 1},
{0x1d552, 0x1d6a5, 1},
{0x1d6a8, 0x1d6c0, 1},
{0x1d6c2, 0x1d6da, 1},
{0x1d6dc, 0x1d6fa, 1},
{0x1d6fc, 0x1d714, 1},
{0x1d716, 0x1d734, 1},
{0x1d736, 0x1d74e, 1},
{0x1d750, 0x1d76e, 1},
{0x1d770, 0x1d788, 1},
{0x1d78a, 0x1d7a8, 1},
{0x1d7aa, 0x1d7c2, 1},
{0x1d7c4, 0x1d7cb, 1},
{0x1d7ce, 0x1d7ff, 1},
};
}
private static final int[][] _Unified_Ideograph = make_Unified_Ideograph();
private static int[][] make_Unified_Ideograph() {
return new int[][] {
{0x3400, 0x4db5, 1},
{0x4e00, 0x9fcb, 1},
{0xfa0e, 0xfa0f, 1},
{0xfa11, 0xfa11, 1},
{0xfa13, 0xfa14, 1},
{0xfa1f, 0xfa1f, 1},
{0xfa21, 0xfa21, 1},
{0xfa23, 0xfa24, 1},
{0xfa27, 0xfa29, 1},
{0x20000, 0x2a6d6, 1},
{0x2a700, 0x2b734, 1},
{0x2b740, 0x2b81d, 1},
};
}
private static final int[][] _Hyphen = make_Hyphen();
private static int[][] make_Hyphen() {
return new int[][] {
{0x002d, 0x002d, 1},
{0x00ad, 0x00ad, 1},
{0x058a, 0x058a, 1},
{0x1806, 0x1806, 1},
{0x2010, 0x2011, 1},
{0x2e17, 0x2e17, 1},
{0x30fb, 0x30fb, 1},
{0xfe63, 0xfe63, 1},
{0xff0d, 0xff0d, 1},
{0xff65, 0xff65, 1},
};
}
private static final int[][] _IDS_Binary_Operator = make_IDS_Binary_Operator();
private static int[][] make_IDS_Binary_Operator() {
return new int[][] {
{0x2ff0, 0x2ff1, 1},
{0x2ff4, 0x2ffb, 1},
};
}
private static final int[][] _Logical_Order_Exception = make_Logical_Order_Exception();
private static int[][] make_Logical_Order_Exception() {
return new int[][] {
{0x0e40, 0x0e44, 1},
{0x0ec0, 0x0ec4, 1},
{0xaab5, 0xaab6, 1},
{0xaab9, 0xaab9, 1},
{0xaabb, 0xaabc, 1},
};
}
private static final int[][] _Radical = make_Radical();
private static int[][] make_Radical() {
return new int[][] {
{0x2e80, 0x2e99, 1},
{0x2e9b, 0x2ef3, 1},
{0x2f00, 0x2fd5, 1},
};
}
private static final int[][] _Other_Uppercase = make_Other_Uppercase();
private static int[][] make_Other_Uppercase() {
return new int[][] {
{0x2160, 0x216f, 1},
{0x24b6, 0x24cf, 1},
};
}
private static final int[][] _STerm = make_STerm();
private static int[][] make_STerm() {
return new int[][] {
{0x0021, 0x0021, 1},
{0x002e, 0x002e, 1},
{0x003f, 0x003f, 1},
{0x055c, 0x055c, 1},
{0x055e, 0x055e, 1},
{0x0589, 0x0589, 1},
{0x061f, 0x061f, 1},
{0x06d4, 0x06d4, 1},
{0x0700, 0x0702, 1},
{0x07f9, 0x07f9, 1},
{0x0964, 0x0965, 1},
{0x104a, 0x104b, 1},
{0x1362, 0x1362, 1},
{0x1367, 0x1368, 1},
{0x166e, 0x166e, 1},
{0x1735, 0x1736, 1},
{0x1803, 0x1803, 1},
{0x1809, 0x1809, 1},
{0x1944, 0x1945, 1},
{0x1aa8, 0x1aab, 1},
{0x1b5a, 0x1b5b, 1},
{0x1b5e, 0x1b5f, 1},
{0x1c3b, 0x1c3c, 1},
{0x1c7e, 0x1c7f, 1},
{0x203c, 0x203d, 1},
{0x2047, 0x2049, 1},
{0x2e2e, 0x2e2e, 1},
{0x3002, 0x3002, 1},
{0xa4ff, 0xa4ff, 1},
{0xa60e, 0xa60f, 1},
{0xa6f3, 0xa6f3, 1},
{0xa6f7, 0xa6f7, 1},
{0xa876, 0xa877, 1},
{0xa8ce, 0xa8cf, 1},
{0xa92f, 0xa92f, 1},
{0xa9c8, 0xa9c9, 1},
{0xaa5d, 0xaa5f, 1},
{0xabeb, 0xabeb, 1},
{0xfe52, 0xfe52, 1},
{0xfe56, 0xfe57, 1},
{0xff01, 0xff01, 1},
{0xff0e, 0xff0e, 1},
{0xff1f, 0xff1f, 1},
{0xff61, 0xff61, 1},
{0x10a56, 0x10a57, 1},
{0x11047, 0x11048, 1},
{0x110be, 0x110c1, 1},
};
}
private static final int[][] _Other_Alphabetic = make_Other_Alphabetic();
private static int[][] make_Other_Alphabetic() {
return new int[][] {
{0x0345, 0x0345, 1},
{0x05b0, 0x05bd, 1},
{0x05bf, 0x05bf, 1},
{0x05c1, 0x05c2, 1},
{0x05c4, 0x05c5, 1},
{0x05c7, 0x05c7, 1},
{0x0610, 0x061a, 1},
{0x064b, 0x0657, 1},
{0x0659, 0x065f, 1},
{0x0670, 0x0670, 1},
{0x06d6, 0x06dc, 1},
{0x06e1, 0x06e4, 1},
{0x06e7, 0x06e8, 1},
{0x06ed, 0x06ed, 1},
{0x0711, 0x0711, 1},
{0x0730, 0x073f, 1},
{0x07a6, 0x07b0, 1},
{0x0816, 0x0817, 1},
{0x081b, 0x0823, 1},
{0x0825, 0x0827, 1},
{0x0829, 0x082c, 1},
{0x0900, 0x0903, 1},
{0x093a, 0x093b, 1},
{0x093e, 0x094c, 1},
{0x094e, 0x094f, 1},
{0x0955, 0x0957, 1},
{0x0962, 0x0963, 1},
{0x0981, 0x0983, 1},
{0x09be, 0x09c4, 1},
{0x09c7, 0x09c8, 1},
{0x09cb, 0x09cc, 1},
{0x09d7, 0x09d7, 1},
{0x09e2, 0x09e3, 1},
{0x0a01, 0x0a03, 1},
{0x0a3e, 0x0a42, 1},
{0x0a47, 0x0a48, 1},
{0x0a4b, 0x0a4c, 1},
{0x0a51, 0x0a51, 1},
{0x0a70, 0x0a71, 1},
{0x0a75, 0x0a75, 1},
{0x0a81, 0x0a83, 1},
{0x0abe, 0x0ac5, 1},
{0x0ac7, 0x0ac9, 1},
{0x0acb, 0x0acc, 1},
{0x0ae2, 0x0ae3, 1},
{0x0b01, 0x0b03, 1},
{0x0b3e, 0x0b44, 1},
{0x0b47, 0x0b48, 1},
{0x0b4b, 0x0b4c, 1},
{0x0b56, 0x0b57, 1},
{0x0b62, 0x0b63, 1},
{0x0b82, 0x0b82, 1},
{0x0bbe, 0x0bc2, 1},
{0x0bc6, 0x0bc8, 1},
{0x0bca, 0x0bcc, 1},
{0x0bd7, 0x0bd7, 1},
{0x0c01, 0x0c03, 1},
{0x0c3e, 0x0c44, 1},
{0x0c46, 0x0c48, 1},
{0x0c4a, 0x0c4c, 1},
{0x0c55, 0x0c56, 1},
{0x0c62, 0x0c63, 1},
{0x0c82, 0x0c83, 1},
{0x0cbe, 0x0cc4, 1},
{0x0cc6, 0x0cc8, 1},
{0x0cca, 0x0ccc, 1},
{0x0cd5, 0x0cd6, 1},
{0x0ce2, 0x0ce3, 1},
{0x0d02, 0x0d03, 1},
{0x0d3e, 0x0d44, 1},
{0x0d46, 0x0d48, 1},
{0x0d4a, 0x0d4c, 1},
{0x0d57, 0x0d57, 1},
{0x0d62, 0x0d63, 1},
{0x0d82, 0x0d83, 1},
{0x0dcf, 0x0dd4, 1},
{0x0dd6, 0x0dd6, 1},
{0x0dd8, 0x0ddf, 1},
{0x0df2, 0x0df3, 1},
{0x0e31, 0x0e31, 1},
{0x0e34, 0x0e3a, 1},
{0x0e4d, 0x0e4d, 1},
{0x0eb1, 0x0eb1, 1},
{0x0eb4, 0x0eb9, 1},
{0x0ebb, 0x0ebc, 1},
{0x0ecd, 0x0ecd, 1},
{0x0f71, 0x0f81, 1},
{0x0f8d, 0x0f97, 1},
{0x0f99, 0x0fbc, 1},
{0x102b, 0x1036, 1},
{0x1038, 0x1038, 1},
{0x103b, 0x103e, 1},
{0x1056, 0x1059, 1},
{0x105e, 0x1060, 1},
{0x1062, 0x1062, 1},
{0x1067, 0x1068, 1},
{0x1071, 0x1074, 1},
{0x1082, 0x1086, 1},
{0x109c, 0x109d, 1},
{0x135f, 0x135f, 1},
{0x1712, 0x1713, 1},
{0x1732, 0x1733, 1},
{0x1752, 0x1753, 1},
{0x1772, 0x1773, 1},
{0x17b6, 0x17c8, 1},
{0x18a9, 0x18a9, 1},
{0x1920, 0x192b, 1},
{0x1930, 0x1938, 1},
{0x19b0, 0x19c0, 1},
{0x19c8, 0x19c9, 1},
{0x1a17, 0x1a1b, 1},
{0x1a55, 0x1a5e, 1},
{0x1a61, 0x1a74, 1},
{0x1b00, 0x1b04, 1},
{0x1b35, 0x1b43, 1},
{0x1b80, 0x1b82, 1},
{0x1ba1, 0x1ba9, 1},
{0x1be7, 0x1bf1, 1},
{0x1c24, 0x1c35, 1},
{0x1cf2, 0x1cf2, 1},
{0x24b6, 0x24e9, 1},
{0x2de0, 0x2dff, 1},
{0xa823, 0xa827, 1},
{0xa880, 0xa881, 1},
{0xa8b4, 0xa8c3, 1},
{0xa926, 0xa92a, 1},
{0xa947, 0xa952, 1},
{0xa980, 0xa983, 1},
{0xa9b4, 0xa9bf, 1},
{0xaa29, 0xaa36, 1},
{0xaa43, 0xaa43, 1},
{0xaa4c, 0xaa4d, 1},
{0xaab0, 0xaab0, 1},
{0xaab2, 0xaab4, 1},
{0xaab7, 0xaab8, 1},
{0xaabe, 0xaabe, 1},
{0xabe3, 0xabea, 1},
{0xfb1e, 0xfb1e, 1},
{0x10a01, 0x10a03, 1},
{0x10a05, 0x10a06, 1},
{0x10a0c, 0x10a0f, 1},
{0x11000, 0x11002, 1},
{0x11038, 0x11045, 1},
{0x11082, 0x11082, 1},
{0x110b0, 0x110b8, 1},
};
}
private static final int[][] _Diacritic = make_Diacritic();
private static int[][] make_Diacritic() {
return new int[][] {
{0x005e, 0x005e, 1},
{0x0060, 0x0060, 1},
{0x00a8, 0x00a8, 1},
{0x00af, 0x00af, 1},
{0x00b4, 0x00b4, 1},
{0x00b7, 0x00b8, 1},
{0x02b0, 0x034e, 1},
{0x0350, 0x0357, 1},
{0x035d, 0x0362, 1},
{0x0374, 0x0375, 1},
{0x037a, 0x037a, 1},
{0x0384, 0x0385, 1},
{0x0483, 0x0487, 1},
{0x0559, 0x0559, 1},
{0x0591, 0x05a1, 1},
{0x05a3, 0x05bd, 1},
{0x05bf, 0x05bf, 1},
{0x05c1, 0x05c2, 1},
{0x05c4, 0x05c4, 1},
{0x064b, 0x0652, 1},
{0x0657, 0x0658, 1},
{0x06df, 0x06e0, 1},
{0x06e5, 0x06e6, 1},
{0x06ea, 0x06ec, 1},
{0x0730, 0x074a, 1},
{0x07a6, 0x07b0, 1},
{0x07eb, 0x07f5, 1},
{0x0818, 0x0819, 1},
{0x093c, 0x093c, 1},
{0x094d, 0x094d, 1},
{0x0951, 0x0954, 1},
{0x0971, 0x0971, 1},
{0x09bc, 0x09bc, 1},
{0x09cd, 0x09cd, 1},
{0x0a3c, 0x0a3c, 1},
{0x0a4d, 0x0a4d, 1},
{0x0abc, 0x0abc, 1},
{0x0acd, 0x0acd, 1},
{0x0b3c, 0x0b3c, 1},
{0x0b4d, 0x0b4d, 1},
{0x0bcd, 0x0bcd, 1},
{0x0c4d, 0x0c4d, 1},
{0x0cbc, 0x0cbc, 1},
{0x0ccd, 0x0ccd, 1},
{0x0d4d, 0x0d4d, 1},
{0x0dca, 0x0dca, 1},
{0x0e47, 0x0e4c, 1},
{0x0e4e, 0x0e4e, 1},
{0x0ec8, 0x0ecc, 1},
{0x0f18, 0x0f19, 1},
{0x0f35, 0x0f35, 1},
{0x0f37, 0x0f37, 1},
{0x0f39, 0x0f39, 1},
{0x0f3e, 0x0f3f, 1},
{0x0f82, 0x0f84, 1},
{0x0f86, 0x0f87, 1},
{0x0fc6, 0x0fc6, 1},
{0x1037, 0x1037, 1},
{0x1039, 0x103a, 1},
{0x1087, 0x108d, 1},
{0x108f, 0x108f, 1},
{0x109a, 0x109b, 1},
{0x17c9, 0x17d3, 1},
{0x17dd, 0x17dd, 1},
{0x1939, 0x193b, 1},
{0x1a75, 0x1a7c, 1},
{0x1a7f, 0x1a7f, 1},
{0x1b34, 0x1b34, 1},
{0x1b44, 0x1b44, 1},
{0x1b6b, 0x1b73, 1},
{0x1baa, 0x1baa, 1},
{0x1c36, 0x1c37, 1},
{0x1c78, 0x1c7d, 1},
{0x1cd0, 0x1ce8, 1},
{0x1ced, 0x1ced, 1},
{0x1d2c, 0x1d6a, 1},
{0x1dc4, 0x1dcf, 1},
{0x1dfd, 0x1dff, 1},
{0x1fbd, 0x1fbd, 1},
{0x1fbf, 0x1fc1, 1},
{0x1fcd, 0x1fcf, 1},
{0x1fdd, 0x1fdf, 1},
{0x1fed, 0x1fef, 1},
{0x1ffd, 0x1ffe, 1},
{0x2cef, 0x2cf1, 1},
{0x2e2f, 0x2e2f, 1},
{0x302a, 0x302f, 1},
{0x3099, 0x309c, 1},
{0x30fc, 0x30fc, 1},
{0xa66f, 0xa66f, 1},
{0xa67c, 0xa67d, 1},
{0xa67f, 0xa67f, 1},
{0xa6f0, 0xa6f1, 1},
{0xa717, 0xa721, 1},
{0xa788, 0xa788, 1},
{0xa8c4, 0xa8c4, 1},
{0xa8e0, 0xa8f1, 1},
{0xa92b, 0xa92e, 1},
{0xa953, 0xa953, 1},
{0xa9b3, 0xa9b3, 1},
{0xa9c0, 0xa9c0, 1},
{0xaa7b, 0xaa7b, 1},
{0xaabf, 0xaac2, 1},
{0xabec, 0xabed, 1},
{0xfb1e, 0xfb1e, 1},
{0xfe20, 0xfe26, 1},
{0xff3e, 0xff3e, 1},
{0xff40, 0xff40, 1},
{0xff70, 0xff70, 1},
{0xff9e, 0xff9f, 1},
{0xffe3, 0xffe3, 1},
{0x110b9, 0x110ba, 1},
{0x1d167, 0x1d169, 1},
{0x1d16d, 0x1d172, 1},
{0x1d17b, 0x1d182, 1},
{0x1d185, 0x1d18b, 1},
{0x1d1aa, 0x1d1ad, 1},
};
}
private static final int[][] _Extender = make_Extender();
private static int[][] make_Extender() {
return new int[][] {
{0x00b7, 0x00b7, 1},
{0x02d0, 0x02d1, 1},
{0x0640, 0x0640, 1},
{0x07fa, 0x07fa, 1},
{0x0e46, 0x0e46, 1},
{0x0ec6, 0x0ec6, 1},
{0x1843, 0x1843, 1},
{0x1aa7, 0x1aa7, 1},
{0x1c36, 0x1c36, 1},
{0x1c7b, 0x1c7b, 1},
{0x3005, 0x3005, 1},
{0x3031, 0x3035, 1},
{0x309d, 0x309e, 1},
{0x30fc, 0x30fe, 1},
{0xa015, 0xa015, 1},
{0xa60c, 0xa60c, 1},
{0xa9cf, 0xa9cf, 1},
{0xaa70, 0xaa70, 1},
{0xaadd, 0xaadd, 1},
{0xff70, 0xff70, 1},
};
}
private static final int[][] _Join_Control = make_Join_Control();
private static int[][] make_Join_Control() {
return new int[][] {
{0x200c, 0x200d, 1},
};
}
private static final int[][] _Ideographic = make_Ideographic();
private static int[][] make_Ideographic() {
return new int[][] {
{0x3006, 0x3007, 1},
{0x3021, 0x3029, 1},
{0x3038, 0x303a, 1},
{0x3400, 0x4db5, 1},
{0x4e00, 0x9fcb, 1},
{0xf900, 0xfa2d, 1},
{0xfa30, 0xfa6d, 1},
{0xfa70, 0xfad9, 1},
{0x20000, 0x2a6d6, 1},
{0x2a700, 0x2b734, 1},
{0x2b740, 0x2b81d, 1},
{0x2f800, 0x2fa1d, 1},
};
}
private static final int[][] _Dash = make_Dash();
private static int[][] make_Dash() {
return new int[][] {
{0x002d, 0x002d, 1},
{0x058a, 0x058a, 1},
{0x05be, 0x05be, 1},
{0x1400, 0x1400, 1},
{0x1806, 0x1806, 1},
{0x2010, 0x2015, 1},
{0x2053, 0x2053, 1},
{0x207b, 0x207b, 1},
{0x208b, 0x208b, 1},
{0x2212, 0x2212, 1},
{0x2e17, 0x2e17, 1},
{0x2e1a, 0x2e1a, 1},
{0x301c, 0x301c, 1},
{0x3030, 0x3030, 1},
{0x30a0, 0x30a0, 1},
{0xfe31, 0xfe32, 1},
{0xfe58, 0xfe58, 1},
{0xfe63, 0xfe63, 1},
{0xff0d, 0xff0d, 1},
};
}
private static final int[][] _IDS_Trinary_Operator = make_IDS_Trinary_Operator();
private static int[][] make_IDS_Trinary_Operator() {
return new int[][] {
{0x2ff2, 0x2ff3, 1},
};
}
private static final int[][] _Other_Grapheme_Extend = make_Other_Grapheme_Extend();
private static int[][] make_Other_Grapheme_Extend() {
return new int[][] {
{0x09be, 0x09be, 1},
{0x09d7, 0x09d7, 1},
{0x0b3e, 0x0b3e, 1},
{0x0b57, 0x0b57, 1},
{0x0bbe, 0x0bbe, 1},
{0x0bd7, 0x0bd7, 1},
{0x0cc2, 0x0cc2, 1},
{0x0cd5, 0x0cd6, 1},
{0x0d3e, 0x0d3e, 1},
{0x0d57, 0x0d57, 1},
{0x0dcf, 0x0dcf, 1},
{0x0ddf, 0x0ddf, 1},
{0x200c, 0x200d, 1},
{0xff9e, 0xff9f, 1},
{0x1d165, 0x1d165, 1},
{0x1d16e, 0x1d172, 1},
};
}
private static final int[][] _Other_Default_Ignorable_Code_Point = make_Other_Default_Ignorable_Code_Point();
private static int[][] make_Other_Default_Ignorable_Code_Point() {
return new int[][] {
{0x034f, 0x034f, 1},
{0x115f, 0x1160, 1},
{0x2065, 0x2069, 1},
{0x3164, 0x3164, 1},
{0xffa0, 0xffa0, 1},
{0xfff0, 0xfff8, 1},
{0xe0000, 0xe0000, 1},
{0xe0002, 0xe001f, 1},
{0xe0080, 0xe00ff, 1},
{0xe01f0, 0xe0fff, 1},
};
}
private static final int[][] _White_Space = make_White_Space();
private static int[][] make_White_Space() {
return new int[][] {
{0x0009, 0x000d, 1},
{0x0020, 0x0020, 1},
{0x0085, 0x0085, 1},
{0x00a0, 0x00a0, 1},
{0x1680, 0x1680, 1},
{0x180e, 0x180e, 1},
{0x2000, 0x200a, 1},
{0x2028, 0x2029, 1},
{0x202f, 0x202f, 1},
{0x205f, 0x205f, 1},
{0x3000, 0x3000, 1},
};
}
static final int[][] ASCII_Hex_Digit = _ASCII_Hex_Digit;
static final int[][] Bidi_Control = _Bidi_Control;
static final int[][] Dash = _Dash;
static final int[][] Deprecated = _Deprecated;
static final int[][] Diacritic = _Diacritic;
static final int[][] Extender = _Extender;
static final int[][] Hex_Digit = _Hex_Digit;
static final int[][] Hyphen = _Hyphen;
static final int[][] IDS_Binary_Operator = _IDS_Binary_Operator;
static final int[][] IDS_Trinary_Operator = _IDS_Trinary_Operator;
static final int[][] Ideographic = _Ideographic;
static final int[][] Join_Control = _Join_Control;
static final int[][] Logical_Order_Exception = _Logical_Order_Exception;
static final int[][] Noncharacter_Code_Point = _Noncharacter_Code_Point;
static final int[][] Other_Alphabetic = _Other_Alphabetic;
static final int[][] Other_Default_Ignorable_Code_Point = _Other_Default_Ignorable_Code_Point;
static final int[][] Other_Grapheme_Extend = _Other_Grapheme_Extend;
static final int[][] Other_ID_Continue = _Other_ID_Continue;
static final int[][] Other_ID_Start = _Other_ID_Start;
static final int[][] Other_Lowercase = _Other_Lowercase;
static final int[][] Other_Math = _Other_Math;
static final int[][] Other_Uppercase = _Other_Uppercase;
static final int[][] Pattern_Syntax = _Pattern_Syntax;
static final int[][] Pattern_White_Space = _Pattern_White_Space;
static final int[][] Quotation_Mark = _Quotation_Mark;
static final int[][] Radical = _Radical;
static final int[][] STerm = _STerm;
static final int[][] Soft_Dotted = _Soft_Dotted;
static final int[][] Terminal_Punctuation = _Terminal_Punctuation;
static final int[][] Unified_Ideograph = _Unified_Ideograph;
static final int[][] Variation_Selector = _Variation_Selector;
static final int[][] White_Space = _White_Space;
static final int[][] CASE_RANGES = {
{0x0041, 0x005A, 0, 32, 0},
{0x0061, 0x007A, -32, 0, -32},
{0x00B5, 0x00B5, 743, 0, 743},
{0x00C0, 0x00D6, 0, 32, 0},
{0x00D8, 0x00DE, 0, 32, 0},
{0x00E0, 0x00F6, -32, 0, -32},
{0x00F8, 0x00FE, -32, 0, -32},
{0x00FF, 0x00FF, 121, 0, 121},
{0x0100, 0x012F, UpperLower, UpperLower, UpperLower},
{0x0130, 0x0130, 0, -199, 0},
{0x0131, 0x0131, -232, 0, -232},
{0x0132, 0x0137, UpperLower, UpperLower, UpperLower},
{0x0139, 0x0148, UpperLower, UpperLower, UpperLower},
{0x014A, 0x0177, UpperLower, UpperLower, UpperLower},
{0x0178, 0x0178, 0, -121, 0},
{0x0179, 0x017E, UpperLower, UpperLower, UpperLower},
{0x017F, 0x017F, -300, 0, -300},
{0x0180, 0x0180, 195, 0, 195},
{0x0181, 0x0181, 0, 210, 0},
{0x0182, 0x0185, UpperLower, UpperLower, UpperLower},
{0x0186, 0x0186, 0, 206, 0},
{0x0187, 0x0188, UpperLower, UpperLower, UpperLower},
{0x0189, 0x018A, 0, 205, 0},
{0x018B, 0x018C, UpperLower, UpperLower, UpperLower},
{0x018E, 0x018E, 0, 79, 0},
{0x018F, 0x018F, 0, 202, 0},
{0x0190, 0x0190, 0, 203, 0},
{0x0191, 0x0192, UpperLower, UpperLower, UpperLower},
{0x0193, 0x0193, 0, 205, 0},
{0x0194, 0x0194, 0, 207, 0},
{0x0195, 0x0195, 97, 0, 97},
{0x0196, 0x0196, 0, 211, 0},
{0x0197, 0x0197, 0, 209, 0},
{0x0198, 0x0199, UpperLower, UpperLower, UpperLower},
{0x019A, 0x019A, 163, 0, 163},
{0x019C, 0x019C, 0, 211, 0},
{0x019D, 0x019D, 0, 213, 0},
{0x019E, 0x019E, 130, 0, 130},
{0x019F, 0x019F, 0, 214, 0},
{0x01A0, 0x01A5, UpperLower, UpperLower, UpperLower},
{0x01A6, 0x01A6, 0, 218, 0},
{0x01A7, 0x01A8, UpperLower, UpperLower, UpperLower},
{0x01A9, 0x01A9, 0, 218, 0},
{0x01AC, 0x01AD, UpperLower, UpperLower, UpperLower},
{0x01AE, 0x01AE, 0, 218, 0},
{0x01AF, 0x01B0, UpperLower, UpperLower, UpperLower},
{0x01B1, 0x01B2, 0, 217, 0},
{0x01B3, 0x01B6, UpperLower, UpperLower, UpperLower},
{0x01B7, 0x01B7, 0, 219, 0},
{0x01B8, 0x01B9, UpperLower, UpperLower, UpperLower},
{0x01BC, 0x01BD, UpperLower, UpperLower, UpperLower},
{0x01BF, 0x01BF, 56, 0, 56},
{0x01C4, 0x01C4, 0, 2, 1},
{0x01C5, 0x01C5, -1, 1, 0},
{0x01C6, 0x01C6, -2, 0, -1},
{0x01C7, 0x01C7, 0, 2, 1},
{0x01C8, 0x01C8, -1, 1, 0},
{0x01C9, 0x01C9, -2, 0, -1},
{0x01CA, 0x01CA, 0, 2, 1},
{0x01CB, 0x01CB, -1, 1, 0},
{0x01CC, 0x01CC, -2, 0, -1},
{0x01CD, 0x01DC, UpperLower, UpperLower, UpperLower},
{0x01DD, 0x01DD, -79, 0, -79},
{0x01DE, 0x01EF, UpperLower, UpperLower, UpperLower},
{0x01F1, 0x01F1, 0, 2, 1},
{0x01F2, 0x01F2, -1, 1, 0},
{0x01F3, 0x01F3, -2, 0, -1},
{0x01F4, 0x01F5, UpperLower, UpperLower, UpperLower},
{0x01F6, 0x01F6, 0, -97, 0},
{0x01F7, 0x01F7, 0, -56, 0},
{0x01F8, 0x021F, UpperLower, UpperLower, UpperLower},
{0x0220, 0x0220, 0, -130, 0},
{0x0222, 0x0233, UpperLower, UpperLower, UpperLower},
{0x023A, 0x023A, 0, 10795, 0},
{0x023B, 0x023C, UpperLower, UpperLower, UpperLower},
{0x023D, 0x023D, 0, -163, 0},
{0x023E, 0x023E, 0, 10792, 0},
{0x023F, 0x0240, 10815, 0, 10815},
{0x0241, 0x0242, UpperLower, UpperLower, UpperLower},
{0x0243, 0x0243, 0, -195, 0},
{0x0244, 0x0244, 0, 69, 0},
{0x0245, 0x0245, 0, 71, 0},
{0x0246, 0x024F, UpperLower, UpperLower, UpperLower},
{0x0250, 0x0250, 10783, 0, 10783},
{0x0251, 0x0251, 10780, 0, 10780},
{0x0252, 0x0252, 10782, 0, 10782},
{0x0253, 0x0253, -210, 0, -210},
{0x0254, 0x0254, -206, 0, -206},
{0x0256, 0x0257, -205, 0, -205},
{0x0259, 0x0259, -202, 0, -202},
{0x025B, 0x025B, -203, 0, -203},
{0x0260, 0x0260, -205, 0, -205},
{0x0263, 0x0263, -207, 0, -207},
{0x0265, 0x0265, 42280, 0, 42280},
{0x0268, 0x0268, -209, 0, -209},
{0x0269, 0x0269, -211, 0, -211},
{0x026B, 0x026B, 10743, 0, 10743},
{0x026F, 0x026F, -211, 0, -211},
{0x0271, 0x0271, 10749, 0, 10749},
{0x0272, 0x0272, -213, 0, -213},
{0x0275, 0x0275, -214, 0, -214},
{0x027D, 0x027D, 10727, 0, 10727},
{0x0280, 0x0280, -218, 0, -218},
{0x0283, 0x0283, -218, 0, -218},
{0x0288, 0x0288, -218, 0, -218},
{0x0289, 0x0289, -69, 0, -69},
{0x028A, 0x028B, -217, 0, -217},
{0x028C, 0x028C, -71, 0, -71},
{0x0292, 0x0292, -219, 0, -219},
{0x0345, 0x0345, 84, 0, 84},
{0x0370, 0x0373, UpperLower, UpperLower, UpperLower},
{0x0376, 0x0377, UpperLower, UpperLower, UpperLower},
{0x037B, 0x037D, 130, 0, 130},
{0x0386, 0x0386, 0, 38, 0},
{0x0388, 0x038A, 0, 37, 0},
{0x038C, 0x038C, 0, 64, 0},
{0x038E, 0x038F, 0, 63, 0},
{0x0391, 0x03A1, 0, 32, 0},
{0x03A3, 0x03AB, 0, 32, 0},
{0x03AC, 0x03AC, -38, 0, -38},
{0x03AD, 0x03AF, -37, 0, -37},
{0x03B1, 0x03C1, -32, 0, -32},
{0x03C2, 0x03C2, -31, 0, -31},
{0x03C3, 0x03CB, -32, 0, -32},
{0x03CC, 0x03CC, -64, 0, -64},
{0x03CD, 0x03CE, -63, 0, -63},
{0x03CF, 0x03CF, 0, 8, 0},
{0x03D0, 0x03D0, -62, 0, -62},
{0x03D1, 0x03D1, -57, 0, -57},
{0x03D5, 0x03D5, -47, 0, -47},
{0x03D6, 0x03D6, -54, 0, -54},
{0x03D7, 0x03D7, -8, 0, -8},
{0x03D8, 0x03EF, UpperLower, UpperLower, UpperLower},
{0x03F0, 0x03F0, -86, 0, -86},
{0x03F1, 0x03F1, -80, 0, -80},
{0x03F2, 0x03F2, 7, 0, 7},
{0x03F4, 0x03F4, 0, -60, 0},
{0x03F5, 0x03F5, -96, 0, -96},
{0x03F7, 0x03F8, UpperLower, UpperLower, UpperLower},
{0x03F9, 0x03F9, 0, -7, 0},
{0x03FA, 0x03FB, UpperLower, UpperLower, UpperLower},
{0x03FD, 0x03FF, 0, -130, 0},
{0x0400, 0x040F, 0, 80, 0},
{0x0410, 0x042F, 0, 32, 0},
{0x0430, 0x044F, -32, 0, -32},
{0x0450, 0x045F, -80, 0, -80},
{0x0460, 0x0481, UpperLower, UpperLower, UpperLower},
{0x048A, 0x04BF, UpperLower, UpperLower, UpperLower},
{0x04C0, 0x04C0, 0, 15, 0},
{0x04C1, 0x04CE, UpperLower, UpperLower, UpperLower},
{0x04CF, 0x04CF, -15, 0, -15},
{0x04D0, 0x0527, UpperLower, UpperLower, UpperLower},
{0x0531, 0x0556, 0, 48, 0},
{0x0561, 0x0586, -48, 0, -48},
{0x10A0, 0x10C5, 0, 7264, 0},
{0x1D79, 0x1D79, 35332, 0, 35332},
{0x1D7D, 0x1D7D, 3814, 0, 3814},
{0x1E00, 0x1E95, UpperLower, UpperLower, UpperLower},
{0x1E9B, 0x1E9B, -59, 0, -59},
{0x1E9E, 0x1E9E, 0, -7615, 0},
{0x1EA0, 0x1EFF, UpperLower, UpperLower, UpperLower},
{0x1F00, 0x1F07, 8, 0, 8},
{0x1F08, 0x1F0F, 0, -8, 0},
{0x1F10, 0x1F15, 8, 0, 8},
{0x1F18, 0x1F1D, 0, -8, 0},
{0x1F20, 0x1F27, 8, 0, 8},
{0x1F28, 0x1F2F, 0, -8, 0},
{0x1F30, 0x1F37, 8, 0, 8},
{0x1F38, 0x1F3F, 0, -8, 0},
{0x1F40, 0x1F45, 8, 0, 8},
{0x1F48, 0x1F4D, 0, -8, 0},
{0x1F51, 0x1F51, 8, 0, 8},
{0x1F53, 0x1F53, 8, 0, 8},
{0x1F55, 0x1F55, 8, 0, 8},
{0x1F57, 0x1F57, 8, 0, 8},
{0x1F59, 0x1F59, 0, -8, 0},
{0x1F5B, 0x1F5B, 0, -8, 0},
{0x1F5D, 0x1F5D, 0, -8, 0},
{0x1F5F, 0x1F5F, 0, -8, 0},
{0x1F60, 0x1F67, 8, 0, 8},
{0x1F68, 0x1F6F, 0, -8, 0},
{0x1F70, 0x1F71, 74, 0, 74},
{0x1F72, 0x1F75, 86, 0, 86},
{0x1F76, 0x1F77, 100, 0, 100},
{0x1F78, 0x1F79, 128, 0, 128},
{0x1F7A, 0x1F7B, 112, 0, 112},
{0x1F7C, 0x1F7D, 126, 0, 126},
{0x1F80, 0x1F87, 8, 0, 8},
{0x1F88, 0x1F8F, 0, -8, 0},
{0x1F90, 0x1F97, 8, 0, 8},
{0x1F98, 0x1F9F, 0, -8, 0},
{0x1FA0, 0x1FA7, 8, 0, 8},
{0x1FA8, 0x1FAF, 0, -8, 0},
{0x1FB0, 0x1FB1, 8, 0, 8},
{0x1FB3, 0x1FB3, 9, 0, 9},
{0x1FB8, 0x1FB9, 0, -8, 0},
{0x1FBA, 0x1FBB, 0, -74, 0},
{0x1FBC, 0x1FBC, 0, -9, 0},
{0x1FBE, 0x1FBE, -7205, 0, -7205},
{0x1FC3, 0x1FC3, 9, 0, 9},
{0x1FC8, 0x1FCB, 0, -86, 0},
{0x1FCC, 0x1FCC, 0, -9, 0},
{0x1FD0, 0x1FD1, 8, 0, 8},
{0x1FD8, 0x1FD9, 0, -8, 0},
{0x1FDA, 0x1FDB, 0, -100, 0},
{0x1FE0, 0x1FE1, 8, 0, 8},
{0x1FE5, 0x1FE5, 7, 0, 7},
{0x1FE8, 0x1FE9, 0, -8, 0},
{0x1FEA, 0x1FEB, 0, -112, 0},
{0x1FEC, 0x1FEC, 0, -7, 0},
{0x1FF3, 0x1FF3, 9, 0, 9},
{0x1FF8, 0x1FF9, 0, -128, 0},
{0x1FFA, 0x1FFB, 0, -126, 0},
{0x1FFC, 0x1FFC, 0, -9, 0},
{0x2126, 0x2126, 0, -7517, 0},
{0x212A, 0x212A, 0, -8383, 0},
{0x212B, 0x212B, 0, -8262, 0},
{0x2132, 0x2132, 0, 28, 0},
{0x214E, 0x214E, -28, 0, -28},
{0x2160, 0x216F, 0, 16, 0},
{0x2170, 0x217F, -16, 0, -16},
{0x2183, 0x2184, UpperLower, UpperLower, UpperLower},
{0x24B6, 0x24CF, 0, 26, 0},
{0x24D0, 0x24E9, -26, 0, -26},
{0x2C00, 0x2C2E, 0, 48, 0},
{0x2C30, 0x2C5E, -48, 0, -48},
{0x2C60, 0x2C61, UpperLower, UpperLower, UpperLower},
{0x2C62, 0x2C62, 0, -10743, 0},
{0x2C63, 0x2C63, 0, -3814, 0},
{0x2C64, 0x2C64, 0, -10727, 0},
{0x2C65, 0x2C65, -10795, 0, -10795},
{0x2C66, 0x2C66, -10792, 0, -10792},
{0x2C67, 0x2C6C, UpperLower, UpperLower, UpperLower},
{0x2C6D, 0x2C6D, 0, -10780, 0},
{0x2C6E, 0x2C6E, 0, -10749, 0},
{0x2C6F, 0x2C6F, 0, -10783, 0},
{0x2C70, 0x2C70, 0, -10782, 0},
{0x2C72, 0x2C73, UpperLower, UpperLower, UpperLower},
{0x2C75, 0x2C76, UpperLower, UpperLower, UpperLower},
{0x2C7E, 0x2C7F, 0, -10815, 0},
{0x2C80, 0x2CE3, UpperLower, UpperLower, UpperLower},
{0x2CEB, 0x2CEE, UpperLower, UpperLower, UpperLower},
{0x2D00, 0x2D25, -7264, 0, -7264},
{0xA640, 0xA66D, UpperLower, UpperLower, UpperLower},
{0xA680, 0xA697, UpperLower, UpperLower, UpperLower},
{0xA722, 0xA72F, UpperLower, UpperLower, UpperLower},
{0xA732, 0xA76F, UpperLower, UpperLower, UpperLower},
{0xA779, 0xA77C, UpperLower, UpperLower, UpperLower},
{0xA77D, 0xA77D, 0, -35332, 0},
{0xA77E, 0xA787, UpperLower, UpperLower, UpperLower},
{0xA78B, 0xA78C, UpperLower, UpperLower, UpperLower},
{0xA78D, 0xA78D, 0, -42280, 0},
{0xA790, 0xA791, UpperLower, UpperLower, UpperLower},
{0xA7A0, 0xA7A9, UpperLower, UpperLower, UpperLower},
{0xFF21, 0xFF3A, 0, 32, 0},
{0xFF41, 0xFF5A, -32, 0, -32},
{0x10400, 0x10427, 0, 40, 0},
{0x10428, 0x1044F, -40, 0, -40},
};
static final int[][] CASE_ORBIT = {
{0x004B, 0x006B},
{0x0053, 0x0073},
{0x006B, 0x212A},
{0x0073, 0x017F},
{0x00B5, 0x039C},
{0x00C5, 0x00E5},
{0x00DF, 0x1E9E},
{0x00E5, 0x212B},
{0x0130, 0x0130},
{0x0131, 0x0131},
{0x017F, 0x0053},
{0x01C4, 0x01C5},
{0x01C5, 0x01C6},
{0x01C6, 0x01C4},
{0x01C7, 0x01C8},
{0x01C8, 0x01C9},
{0x01C9, 0x01C7},
{0x01CA, 0x01CB},
{0x01CB, 0x01CC},
{0x01CC, 0x01CA},
{0x01F1, 0x01F2},
{0x01F2, 0x01F3},
{0x01F3, 0x01F1},
{0x0345, 0x0399},
{0x0392, 0x03B2},
{0x0395, 0x03B5},
{0x0398, 0x03B8},
{0x0399, 0x03B9},
{0x039A, 0x03BA},
{0x039C, 0x03BC},
{0x03A0, 0x03C0},
{0x03A1, 0x03C1},
{0x03A3, 0x03C2},
{0x03A6, 0x03C6},
{0x03A9, 0x03C9},
{0x03B2, 0x03D0},
{0x03B5, 0x03F5},
{0x03B8, 0x03D1},
{0x03B9, 0x1FBE},
{0x03BA, 0x03F0},
{0x03BC, 0x00B5},
{0x03C0, 0x03D6},
{0x03C1, 0x03F1},
{0x03C2, 0x03C3},
{0x03C3, 0x03A3},
{0x03C6, 0x03D5},
{0x03C9, 0x2126},
{0x03D0, 0x0392},
{0x03D1, 0x03F4},
{0x03D5, 0x03A6},
{0x03D6, 0x03A0},
{0x03F0, 0x039A},
{0x03F1, 0x03A1},
{0x03F4, 0x0398},
{0x03F5, 0x0395},
{0x1E60, 0x1E61},
{0x1E61, 0x1E9B},
{0x1E9B, 0x1E60},
{0x1E9E, 0x00DF},
{0x1FBE, 0x0345},
{0x2126, 0x03A9},
{0x212A, 0x004B},
{0x212B, 0x00C5},
};
private static Map FoldCategory() {
Map map = new HashMap();
map.put("Ll", foldLl);
map.put("Inherited", foldInherited);
map.put("M", foldM);
map.put("L", foldL);
map.put("Mn", foldMn);
map.put("Common", foldCommon);
map.put("Greek", foldGreek);
map.put("Lu", foldLu);
map.put("Lt", foldLt);
return map;
}
private static final int[][] foldLl = makefoldLl();
private static int[][] makefoldLl() {
return new int[][] {
{0x0041, 0x005a, 1},
{0x00c0, 0x00d6, 1},
{0x00d8, 0x00de, 1},
{0x0100, 0x012e, 2},
{0x0132, 0x0136, 2},
{0x0139, 0x0147, 2},
{0x014a, 0x0178, 2},
{0x0179, 0x017d, 2},
{0x0181, 0x0182, 1},
{0x0184, 0x0186, 2},
{0x0187, 0x0189, 2},
{0x018a, 0x018b, 1},
{0x018e, 0x0191, 1},
{0x0193, 0x0194, 1},
{0x0196, 0x0198, 1},
{0x019c, 0x019d, 1},
{0x019f, 0x01a0, 1},
{0x01a2, 0x01a6, 2},
{0x01a7, 0x01a9, 2},
{0x01ac, 0x01ae, 2},
{0x01af, 0x01b1, 2},
{0x01b2, 0x01b3, 1},
{0x01b5, 0x01b7, 2},
{0x01b8, 0x01bc, 4},
{0x01c4, 0x01c5, 1},
{0x01c7, 0x01c8, 1},
{0x01ca, 0x01cb, 1},
{0x01cd, 0x01db, 2},
{0x01de, 0x01ee, 2},
{0x01f1, 0x01f2, 1},
{0x01f4, 0x01f6, 2},
{0x01f7, 0x01f8, 1},
{0x01fa, 0x0232, 2},
{0x023a, 0x023b, 1},
{0x023d, 0x023e, 1},
{0x0241, 0x0243, 2},
{0x0244, 0x0246, 1},
{0x0248, 0x024e, 2},
{0x0345, 0x0370, 43},
{0x0372, 0x0376, 4},
{0x0386, 0x0388, 2},
{0x0389, 0x038a, 1},
{0x038c, 0x038e, 2},
{0x038f, 0x0391, 2},
{0x0392, 0x03a1, 1},
{0x03a3, 0x03ab, 1},
{0x03cf, 0x03d8, 9},
{0x03da, 0x03ee, 2},
{0x03f4, 0x03f7, 3},
{0x03f9, 0x03fa, 1},
{0x03fd, 0x042f, 1},
{0x0460, 0x0480, 2},
{0x048a, 0x04c0, 2},
{0x04c1, 0x04cd, 2},
{0x04d0, 0x0526, 2},
{0x0531, 0x0556, 1},
{0x10a0, 0x10c5, 1},
{0x1e00, 0x1e94, 2},
{0x1e9e, 0x1efe, 2},
{0x1f08, 0x1f0f, 1},
{0x1f18, 0x1f1d, 1},
{0x1f28, 0x1f2f, 1},
{0x1f38, 0x1f3f, 1},
{0x1f48, 0x1f4d, 1},
{0x1f59, 0x1f5f, 2},
{0x1f68, 0x1f6f, 1},
{0x1f88, 0x1f8f, 1},
{0x1f98, 0x1f9f, 1},
{0x1fa8, 0x1faf, 1},
{0x1fb8, 0x1fbc, 1},
{0x1fc8, 0x1fcc, 1},
{0x1fd8, 0x1fdb, 1},
{0x1fe8, 0x1fec, 1},
{0x1ff8, 0x1ffc, 1},
{0x2126, 0x212a, 4},
{0x212b, 0x2132, 7},
{0x2183, 0x2c00, 2685},
{0x2c01, 0x2c2e, 1},
{0x2c60, 0x2c62, 2},
{0x2c63, 0x2c64, 1},
{0x2c67, 0x2c6d, 2},
{0x2c6e, 0x2c70, 1},
{0x2c72, 0x2c75, 3},
{0x2c7e, 0x2c80, 1},
{0x2c82, 0x2ce2, 2},
{0x2ceb, 0x2ced, 2},
{0xa640, 0xa66c, 2},
{0xa680, 0xa696, 2},
{0xa722, 0xa72e, 2},
{0xa732, 0xa76e, 2},
{0xa779, 0xa77d, 2},
{0xa77e, 0xa786, 2},
{0xa78b, 0xa78d, 2},
{0xa790, 0xa7a0, 16},
{0xa7a2, 0xa7a8, 2},
{0xff21, 0xff3a, 1},
{0x10400, 0x10427, 1},
};
}
private static final int[][] foldInherited = makefoldInherited();
private static int[][] makefoldInherited() {
return new int[][] {
{0x0399, 0x03b9, 32},
{0x1fbe, 0x1fbe, 1},
};
}
private static final int[][] foldM = makefoldM();
private static int[][] makefoldM() {
return new int[][] {
{0x0399, 0x03b9, 32},
{0x1fbe, 0x1fbe, 1},
};
}
private static final int[][] foldL = makefoldL();
private static int[][] makefoldL() {
return new int[][] {
{0x0345, 0x0345, 1},
};
}
private static final int[][] foldMn = makefoldMn();
private static int[][] makefoldMn() {
return new int[][] {
{0x0399, 0x03b9, 32},
{0x1fbe, 0x1fbe, 1},
};
}
private static final int[][] foldCommon = makefoldCommon();
private static int[][] makefoldCommon() {
return new int[][] {
{0x039c, 0x03bc, 32},
};
}
private static final int[][] foldGreek = makefoldGreek();
private static int[][] makefoldGreek() {
return new int[][] {
{0x00b5, 0x0345, 656},
};
}
private static final int[][] foldLu = makefoldLu();
private static int[][] makefoldLu() {
return new int[][] {
{0x0061, 0x007a, 1},
{0x00b5, 0x00df, 42},
{0x00e0, 0x00f6, 1},
{0x00f8, 0x00ff, 1},
{0x0101, 0x012f, 2},
{0x0133, 0x0137, 2},
{0x013a, 0x0148, 2},
{0x014b, 0x0177, 2},
{0x017a, 0x017e, 2},
{0x017f, 0x0180, 1},
{0x0183, 0x0185, 2},
{0x0188, 0x018c, 4},
{0x0192, 0x0195, 3},
{0x0199, 0x019a, 1},
{0x019e, 0x01a1, 3},
{0x01a3, 0x01a5, 2},
{0x01a8, 0x01ad, 5},
{0x01b0, 0x01b4, 4},
{0x01b6, 0x01b9, 3},
{0x01bd, 0x01bf, 2},
{0x01c5, 0x01c6, 1},
{0x01c8, 0x01c9, 1},
{0x01cb, 0x01cc, 1},
{0x01ce, 0x01dc, 2},
{0x01dd, 0x01ef, 2},
{0x01f2, 0x01f3, 1},
{0x01f5, 0x01f9, 4},
{0x01fb, 0x021f, 2},
{0x0223, 0x0233, 2},
{0x023c, 0x023f, 3},
{0x0240, 0x0242, 2},
{0x0247, 0x024f, 2},
{0x0250, 0x0254, 1},
{0x0256, 0x0257, 1},
{0x0259, 0x025b, 2},
{0x0260, 0x0263, 3},
{0x0265, 0x0268, 3},
{0x0269, 0x026b, 2},
{0x026f, 0x0271, 2},
{0x0272, 0x0275, 3},
{0x027d, 0x0283, 3},
{0x0288, 0x028c, 1},
{0x0292, 0x0345, 179},
{0x0371, 0x0373, 2},
{0x0377, 0x037b, 4},
{0x037c, 0x037d, 1},
{0x03ac, 0x03af, 1},
{0x03b1, 0x03ce, 1},
{0x03d0, 0x03d1, 1},
{0x03d5, 0x03d7, 1},
{0x03d9, 0x03ef, 2},
{0x03f0, 0x03f2, 1},
{0x03f5, 0x03fb, 3},
{0x0430, 0x045f, 1},
{0x0461, 0x0481, 2},
{0x048b, 0x04bf, 2},
{0x04c2, 0x04ce, 2},
{0x04cf, 0x0527, 2},
{0x0561, 0x0586, 1},
{0x1d79, 0x1d7d, 4},
{0x1e01, 0x1e95, 2},
{0x1e9b, 0x1ea1, 6},
{0x1ea3, 0x1eff, 2},
{0x1f00, 0x1f07, 1},
{0x1f10, 0x1f15, 1},
{0x1f20, 0x1f27, 1},
{0x1f30, 0x1f37, 1},
{0x1f40, 0x1f45, 1},
{0x1f51, 0x1f57, 2},
{0x1f60, 0x1f67, 1},
{0x1f70, 0x1f7d, 1},
{0x1fb0, 0x1fb1, 1},
{0x1fbe, 0x1fd0, 18},
{0x1fd1, 0x1fe0, 15},
{0x1fe1, 0x1fe5, 4},
{0x214e, 0x2184, 54},
{0x2c30, 0x2c5e, 1},
{0x2c61, 0x2c65, 4},
{0x2c66, 0x2c6c, 2},
{0x2c73, 0x2c76, 3},
{0x2c81, 0x2ce3, 2},
{0x2cec, 0x2cee, 2},
{0x2d00, 0x2d25, 1},
{0xa641, 0xa66d, 2},
{0xa681, 0xa697, 2},
{0xa723, 0xa72f, 2},
{0xa733, 0xa76f, 2},
{0xa77a, 0xa77c, 2},
{0xa77f, 0xa787, 2},
{0xa78c, 0xa791, 5},
{0xa7a1, 0xa7a9, 2},
{0xff41, 0xff5a, 1},
{0x10428, 0x1044f, 1},
};
}
private static final int[][] foldLt = makefoldLt();
private static int[][] makefoldLt() {
return new int[][] {
{0x01c4, 0x01c6, 2},
{0x01c7, 0x01c9, 2},
{0x01ca, 0x01cc, 2},
{0x01f1, 0x01f3, 2},
{0x1f80, 0x1f87, 1},
{0x1f90, 0x1f97, 1},
{0x1fa0, 0x1fa7, 1},
{0x1fb3, 0x1fc3, 16},
{0x1ff3, 0x1ff3, 1},
};
}
private static Map FoldScript() {
return new HashMap();
}
static final Map CATEGORIES = Categories();
static final Map SCRIPTS = Scripts();
static final Map PROPERTIES = Properties();
static final Map FOLD_CATEGORIES = FoldCategory();
static final Map FOLD_SCRIPT = FoldScript();
// Fold orbit bytes: 63 pairs, 252 bytes
private UnicodeTables() {} // uninstantiable
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy