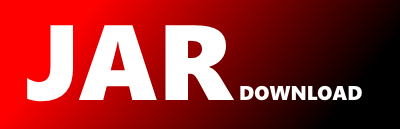
com.google.shopping.merchant.accounts.v1beta.DeliveryTime Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/shopping/merchant/accounts/v1beta/shippingsettings.proto
// Protobuf Java Version: 3.25.5
package com.google.shopping.merchant.accounts.v1beta;
/**
*
*
*
* Time spent in various aspects from order to the delivery of the product.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.DeliveryTime}
*/
public final class DeliveryTime extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.shopping.merchant.accounts.v1beta.DeliveryTime)
DeliveryTimeOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeliveryTime.newBuilder() to construct.
private DeliveryTime(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeliveryTime() {
warehouseBasedDeliveryTimes_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new DeliveryTime();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_DeliveryTime_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_DeliveryTime_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.class,
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.Builder.class);
}
private int bitField0_;
public static final int MIN_TRANSIT_DAYS_FIELD_NUMBER = 1;
private int minTransitDays_ = 0;
/**
*
*
*
* Minimum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery.
* Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` must be set, but not both.
*
*
* optional int32 min_transit_days = 1;
*
* @return Whether the minTransitDays field is set.
*/
@java.lang.Override
public boolean hasMinTransitDays() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Minimum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery.
* Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` must be set, but not both.
*
*
* optional int32 min_transit_days = 1;
*
* @return The minTransitDays.
*/
@java.lang.Override
public int getMinTransitDays() {
return minTransitDays_;
}
public static final int MAX_TRANSIT_DAYS_FIELD_NUMBER = 2;
private int maxTransitDays_ = 0;
/**
*
*
*
* Maximum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery. Must be greater than or equal
* to `min_transit_days`.
*
*
* optional int32 max_transit_days = 2;
*
* @return Whether the maxTransitDays field is set.
*/
@java.lang.Override
public boolean hasMaxTransitDays() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Maximum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery. Must be greater than or equal
* to `min_transit_days`.
*
*
* optional int32 max_transit_days = 2;
*
* @return The maxTransitDays.
*/
@java.lang.Override
public int getMaxTransitDays() {
return maxTransitDays_;
}
public static final int CUTOFF_TIME_FIELD_NUMBER = 3;
private com.google.shopping.merchant.accounts.v1beta.CutoffTime cutoffTime_;
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*
* @return Whether the cutoffTime field is set.
*/
@java.lang.Override
public boolean hasCutoffTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*
* @return The cutoffTime.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.CutoffTime getCutoffTime() {
return cutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.CutoffTime.getDefaultInstance()
: cutoffTime_;
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.CutoffTimeOrBuilder getCutoffTimeOrBuilder() {
return cutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.CutoffTime.getDefaultInstance()
: cutoffTime_;
}
public static final int MIN_HANDLING_DAYS_FIELD_NUMBER = 4;
private int minHandlingDays_ = 0;
/**
*
*
*
* Minimum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 min_handling_days = 4;
*
* @return Whether the minHandlingDays field is set.
*/
@java.lang.Override
public boolean hasMinHandlingDays() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Minimum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 min_handling_days = 4;
*
* @return The minHandlingDays.
*/
@java.lang.Override
public int getMinHandlingDays() {
return minHandlingDays_;
}
public static final int MAX_HANDLING_DAYS_FIELD_NUMBER = 5;
private int maxHandlingDays_ = 0;
/**
*
*
*
* Maximum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* Must be greater than or equal to `min_handling_days`.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 max_handling_days = 5;
*
* @return Whether the maxHandlingDays field is set.
*/
@java.lang.Override
public boolean hasMaxHandlingDays() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Maximum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* Must be greater than or equal to `min_handling_days`.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 max_handling_days = 5;
*
* @return The maxHandlingDays.
*/
@java.lang.Override
public int getMaxHandlingDays() {
return maxHandlingDays_;
}
public static final int TRANSIT_TIME_TABLE_FIELD_NUMBER = 6;
private com.google.shopping.merchant.accounts.v1beta.TransitTable transitTimeTable_;
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*
* @return Whether the transitTimeTable field is set.
*/
@java.lang.Override
public boolean hasTransitTimeTable() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*
* @return The transitTimeTable.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.TransitTable getTransitTimeTable() {
return transitTimeTable_ == null
? com.google.shopping.merchant.accounts.v1beta.TransitTable.getDefaultInstance()
: transitTimeTable_;
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.TransitTableOrBuilder
getTransitTimeTableOrBuilder() {
return transitTimeTable_ == null
? com.google.shopping.merchant.accounts.v1beta.TransitTable.getDefaultInstance()
: transitTimeTable_;
}
public static final int HANDLING_BUSINESS_DAY_CONFIG_FIELD_NUMBER = 7;
private com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig handlingBusinessDayConfig_;
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*
* @return Whether the handlingBusinessDayConfig field is set.
*/
@java.lang.Override
public boolean hasHandlingBusinessDayConfig() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*
* @return The handlingBusinessDayConfig.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
getHandlingBusinessDayConfig() {
return handlingBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: handlingBusinessDayConfig_;
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder
getHandlingBusinessDayConfigOrBuilder() {
return handlingBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: handlingBusinessDayConfig_;
}
public static final int TRANSIT_BUSINESS_DAY_CONFIG_FIELD_NUMBER = 8;
private com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig transitBusinessDayConfig_;
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*
* @return Whether the transitBusinessDayConfig field is set.
*/
@java.lang.Override
public boolean hasTransitBusinessDayConfig() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*
* @return The transitBusinessDayConfig.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
getTransitBusinessDayConfig() {
return transitBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: transitBusinessDayConfig_;
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder
getTransitBusinessDayConfigOrBuilder() {
return transitBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: transitBusinessDayConfig_;
}
public static final int WAREHOUSE_BASED_DELIVERY_TIMES_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private java.util.List
warehouseBasedDeliveryTimes_;
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List
getWarehouseBasedDeliveryTimesList() {
return warehouseBasedDeliveryTimes_;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List<
? extends
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTimeOrBuilder>
getWarehouseBasedDeliveryTimesOrBuilderList() {
return warehouseBasedDeliveryTimes_;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public int getWarehouseBasedDeliveryTimesCount() {
return warehouseBasedDeliveryTimes_.size();
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime
getWarehouseBasedDeliveryTimes(int index) {
return warehouseBasedDeliveryTimes_.get(index);
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTimeOrBuilder
getWarehouseBasedDeliveryTimesOrBuilder(int index) {
return warehouseBasedDeliveryTimes_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, minTransitDays_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, maxTransitDays_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(3, getCutoffTime());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(4, minHandlingDays_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(5, maxHandlingDays_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(6, getTransitTimeTable());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(7, getHandlingBusinessDayConfig());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(8, getTransitBusinessDayConfig());
}
for (int i = 0; i < warehouseBasedDeliveryTimes_.size(); i++) {
output.writeMessage(9, warehouseBasedDeliveryTimes_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(1, minTransitDays_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(2, maxTransitDays_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getCutoffTime());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(4, minHandlingDays_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(5, maxHandlingDays_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getTransitTimeTable());
}
if (((bitField0_ & 0x00000040) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
7, getHandlingBusinessDayConfig());
}
if (((bitField0_ & 0x00000080) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
8, getTransitBusinessDayConfig());
}
for (int i = 0; i < warehouseBasedDeliveryTimes_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
9, warehouseBasedDeliveryTimes_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.shopping.merchant.accounts.v1beta.DeliveryTime)) {
return super.equals(obj);
}
com.google.shopping.merchant.accounts.v1beta.DeliveryTime other =
(com.google.shopping.merchant.accounts.v1beta.DeliveryTime) obj;
if (hasMinTransitDays() != other.hasMinTransitDays()) return false;
if (hasMinTransitDays()) {
if (getMinTransitDays() != other.getMinTransitDays()) return false;
}
if (hasMaxTransitDays() != other.hasMaxTransitDays()) return false;
if (hasMaxTransitDays()) {
if (getMaxTransitDays() != other.getMaxTransitDays()) return false;
}
if (hasCutoffTime() != other.hasCutoffTime()) return false;
if (hasCutoffTime()) {
if (!getCutoffTime().equals(other.getCutoffTime())) return false;
}
if (hasMinHandlingDays() != other.hasMinHandlingDays()) return false;
if (hasMinHandlingDays()) {
if (getMinHandlingDays() != other.getMinHandlingDays()) return false;
}
if (hasMaxHandlingDays() != other.hasMaxHandlingDays()) return false;
if (hasMaxHandlingDays()) {
if (getMaxHandlingDays() != other.getMaxHandlingDays()) return false;
}
if (hasTransitTimeTable() != other.hasTransitTimeTable()) return false;
if (hasTransitTimeTable()) {
if (!getTransitTimeTable().equals(other.getTransitTimeTable())) return false;
}
if (hasHandlingBusinessDayConfig() != other.hasHandlingBusinessDayConfig()) return false;
if (hasHandlingBusinessDayConfig()) {
if (!getHandlingBusinessDayConfig().equals(other.getHandlingBusinessDayConfig()))
return false;
}
if (hasTransitBusinessDayConfig() != other.hasTransitBusinessDayConfig()) return false;
if (hasTransitBusinessDayConfig()) {
if (!getTransitBusinessDayConfig().equals(other.getTransitBusinessDayConfig())) return false;
}
if (!getWarehouseBasedDeliveryTimesList().equals(other.getWarehouseBasedDeliveryTimesList()))
return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMinTransitDays()) {
hash = (37 * hash) + MIN_TRANSIT_DAYS_FIELD_NUMBER;
hash = (53 * hash) + getMinTransitDays();
}
if (hasMaxTransitDays()) {
hash = (37 * hash) + MAX_TRANSIT_DAYS_FIELD_NUMBER;
hash = (53 * hash) + getMaxTransitDays();
}
if (hasCutoffTime()) {
hash = (37 * hash) + CUTOFF_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCutoffTime().hashCode();
}
if (hasMinHandlingDays()) {
hash = (37 * hash) + MIN_HANDLING_DAYS_FIELD_NUMBER;
hash = (53 * hash) + getMinHandlingDays();
}
if (hasMaxHandlingDays()) {
hash = (37 * hash) + MAX_HANDLING_DAYS_FIELD_NUMBER;
hash = (53 * hash) + getMaxHandlingDays();
}
if (hasTransitTimeTable()) {
hash = (37 * hash) + TRANSIT_TIME_TABLE_FIELD_NUMBER;
hash = (53 * hash) + getTransitTimeTable().hashCode();
}
if (hasHandlingBusinessDayConfig()) {
hash = (37 * hash) + HANDLING_BUSINESS_DAY_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getHandlingBusinessDayConfig().hashCode();
}
if (hasTransitBusinessDayConfig()) {
hash = (37 * hash) + TRANSIT_BUSINESS_DAY_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getTransitBusinessDayConfig().hashCode();
}
if (getWarehouseBasedDeliveryTimesCount() > 0) {
hash = (37 * hash) + WAREHOUSE_BASED_DELIVERY_TIMES_FIELD_NUMBER;
hash = (53 * hash) + getWarehouseBasedDeliveryTimesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.shopping.merchant.accounts.v1beta.DeliveryTime prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Time spent in various aspects from order to the delivery of the product.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.DeliveryTime}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.shopping.merchant.accounts.v1beta.DeliveryTime)
com.google.shopping.merchant.accounts.v1beta.DeliveryTimeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_DeliveryTime_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_DeliveryTime_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.class,
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.Builder.class);
}
// Construct using com.google.shopping.merchant.accounts.v1beta.DeliveryTime.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getCutoffTimeFieldBuilder();
getTransitTimeTableFieldBuilder();
getHandlingBusinessDayConfigFieldBuilder();
getTransitBusinessDayConfigFieldBuilder();
getWarehouseBasedDeliveryTimesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
minTransitDays_ = 0;
maxTransitDays_ = 0;
cutoffTime_ = null;
if (cutoffTimeBuilder_ != null) {
cutoffTimeBuilder_.dispose();
cutoffTimeBuilder_ = null;
}
minHandlingDays_ = 0;
maxHandlingDays_ = 0;
transitTimeTable_ = null;
if (transitTimeTableBuilder_ != null) {
transitTimeTableBuilder_.dispose();
transitTimeTableBuilder_ = null;
}
handlingBusinessDayConfig_ = null;
if (handlingBusinessDayConfigBuilder_ != null) {
handlingBusinessDayConfigBuilder_.dispose();
handlingBusinessDayConfigBuilder_ = null;
}
transitBusinessDayConfig_ = null;
if (transitBusinessDayConfigBuilder_ != null) {
transitBusinessDayConfigBuilder_.dispose();
transitBusinessDayConfigBuilder_ = null;
}
if (warehouseBasedDeliveryTimesBuilder_ == null) {
warehouseBasedDeliveryTimes_ = java.util.Collections.emptyList();
} else {
warehouseBasedDeliveryTimes_ = null;
warehouseBasedDeliveryTimesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_DeliveryTime_descriptor;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.DeliveryTime getDefaultInstanceForType() {
return com.google.shopping.merchant.accounts.v1beta.DeliveryTime.getDefaultInstance();
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.DeliveryTime build() {
com.google.shopping.merchant.accounts.v1beta.DeliveryTime result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.DeliveryTime buildPartial() {
com.google.shopping.merchant.accounts.v1beta.DeliveryTime result =
new com.google.shopping.merchant.accounts.v1beta.DeliveryTime(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.shopping.merchant.accounts.v1beta.DeliveryTime result) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
warehouseBasedDeliveryTimes_ =
java.util.Collections.unmodifiableList(warehouseBasedDeliveryTimes_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.warehouseBasedDeliveryTimes_ = warehouseBasedDeliveryTimes_;
} else {
result.warehouseBasedDeliveryTimes_ = warehouseBasedDeliveryTimesBuilder_.build();
}
}
private void buildPartial0(com.google.shopping.merchant.accounts.v1beta.DeliveryTime result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.minTransitDays_ = minTransitDays_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.maxTransitDays_ = maxTransitDays_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.cutoffTime_ = cutoffTimeBuilder_ == null ? cutoffTime_ : cutoffTimeBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.minHandlingDays_ = minHandlingDays_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.maxHandlingDays_ = maxHandlingDays_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.transitTimeTable_ =
transitTimeTableBuilder_ == null ? transitTimeTable_ : transitTimeTableBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.handlingBusinessDayConfig_ =
handlingBusinessDayConfigBuilder_ == null
? handlingBusinessDayConfig_
: handlingBusinessDayConfigBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.transitBusinessDayConfig_ =
transitBusinessDayConfigBuilder_ == null
? transitBusinessDayConfig_
: transitBusinessDayConfigBuilder_.build();
to_bitField0_ |= 0x00000080;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.shopping.merchant.accounts.v1beta.DeliveryTime) {
return mergeFrom((com.google.shopping.merchant.accounts.v1beta.DeliveryTime) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.shopping.merchant.accounts.v1beta.DeliveryTime other) {
if (other == com.google.shopping.merchant.accounts.v1beta.DeliveryTime.getDefaultInstance())
return this;
if (other.hasMinTransitDays()) {
setMinTransitDays(other.getMinTransitDays());
}
if (other.hasMaxTransitDays()) {
setMaxTransitDays(other.getMaxTransitDays());
}
if (other.hasCutoffTime()) {
mergeCutoffTime(other.getCutoffTime());
}
if (other.hasMinHandlingDays()) {
setMinHandlingDays(other.getMinHandlingDays());
}
if (other.hasMaxHandlingDays()) {
setMaxHandlingDays(other.getMaxHandlingDays());
}
if (other.hasTransitTimeTable()) {
mergeTransitTimeTable(other.getTransitTimeTable());
}
if (other.hasHandlingBusinessDayConfig()) {
mergeHandlingBusinessDayConfig(other.getHandlingBusinessDayConfig());
}
if (other.hasTransitBusinessDayConfig()) {
mergeTransitBusinessDayConfig(other.getTransitBusinessDayConfig());
}
if (warehouseBasedDeliveryTimesBuilder_ == null) {
if (!other.warehouseBasedDeliveryTimes_.isEmpty()) {
if (warehouseBasedDeliveryTimes_.isEmpty()) {
warehouseBasedDeliveryTimes_ = other.warehouseBasedDeliveryTimes_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.addAll(other.warehouseBasedDeliveryTimes_);
}
onChanged();
}
} else {
if (!other.warehouseBasedDeliveryTimes_.isEmpty()) {
if (warehouseBasedDeliveryTimesBuilder_.isEmpty()) {
warehouseBasedDeliveryTimesBuilder_.dispose();
warehouseBasedDeliveryTimesBuilder_ = null;
warehouseBasedDeliveryTimes_ = other.warehouseBasedDeliveryTimes_;
bitField0_ = (bitField0_ & ~0x00000100);
warehouseBasedDeliveryTimesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getWarehouseBasedDeliveryTimesFieldBuilder()
: null;
} else {
warehouseBasedDeliveryTimesBuilder_.addAllMessages(other.warehouseBasedDeliveryTimes_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
minTransitDays_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16:
{
maxTransitDays_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26:
{
input.readMessage(getCutoffTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 32:
{
minHandlingDays_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40:
{
maxHandlingDays_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50:
{
input.readMessage(
getTransitTimeTableFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 58:
{
input.readMessage(
getHandlingBusinessDayConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 58
case 66:
{
input.readMessage(
getTransitBusinessDayConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 74:
{
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime m =
input.readMessage(
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime
.parser(),
extensionRegistry);
if (warehouseBasedDeliveryTimesBuilder_ == null) {
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.add(m);
} else {
warehouseBasedDeliveryTimesBuilder_.addMessage(m);
}
break;
} // case 74
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int minTransitDays_;
/**
*
*
*
* Minimum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery.
* Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` must be set, but not both.
*
*
* optional int32 min_transit_days = 1;
*
* @return Whether the minTransitDays field is set.
*/
@java.lang.Override
public boolean hasMinTransitDays() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Minimum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery.
* Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` must be set, but not both.
*
*
* optional int32 min_transit_days = 1;
*
* @return The minTransitDays.
*/
@java.lang.Override
public int getMinTransitDays() {
return minTransitDays_;
}
/**
*
*
*
* Minimum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery.
* Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` must be set, but not both.
*
*
* optional int32 min_transit_days = 1;
*
* @param value The minTransitDays to set.
* @return This builder for chaining.
*/
public Builder setMinTransitDays(int value) {
minTransitDays_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Minimum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery.
* Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` must be set, but not both.
*
*
* optional int32 min_transit_days = 1;
*
* @return This builder for chaining.
*/
public Builder clearMinTransitDays() {
bitField0_ = (bitField0_ & ~0x00000001);
minTransitDays_ = 0;
onChanged();
return this;
}
private int maxTransitDays_;
/**
*
*
*
* Maximum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery. Must be greater than or equal
* to `min_transit_days`.
*
*
* optional int32 max_transit_days = 2;
*
* @return Whether the maxTransitDays field is set.
*/
@java.lang.Override
public boolean hasMaxTransitDays() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Maximum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery. Must be greater than or equal
* to `min_transit_days`.
*
*
* optional int32 max_transit_days = 2;
*
* @return The maxTransitDays.
*/
@java.lang.Override
public int getMaxTransitDays() {
return maxTransitDays_;
}
/**
*
*
*
* Maximum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery. Must be greater than or equal
* to `min_transit_days`.
*
*
* optional int32 max_transit_days = 2;
*
* @param value The maxTransitDays to set.
* @return This builder for chaining.
*/
public Builder setMaxTransitDays(int value) {
maxTransitDays_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Maximum number of business days that is spent in transit. 0 means same
* day delivery, 1 means next day delivery. Must be greater than or equal
* to `min_transit_days`.
*
*
* optional int32 max_transit_days = 2;
*
* @return This builder for chaining.
*/
public Builder clearMaxTransitDays() {
bitField0_ = (bitField0_ & ~0x00000002);
maxTransitDays_ = 0;
onChanged();
return this;
}
private com.google.shopping.merchant.accounts.v1beta.CutoffTime cutoffTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.CutoffTime,
com.google.shopping.merchant.accounts.v1beta.CutoffTime.Builder,
com.google.shopping.merchant.accounts.v1beta.CutoffTimeOrBuilder>
cutoffTimeBuilder_;
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*
* @return Whether the cutoffTime field is set.
*/
public boolean hasCutoffTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*
* @return The cutoffTime.
*/
public com.google.shopping.merchant.accounts.v1beta.CutoffTime getCutoffTime() {
if (cutoffTimeBuilder_ == null) {
return cutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.CutoffTime.getDefaultInstance()
: cutoffTime_;
} else {
return cutoffTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
public Builder setCutoffTime(com.google.shopping.merchant.accounts.v1beta.CutoffTime value) {
if (cutoffTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cutoffTime_ = value;
} else {
cutoffTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
public Builder setCutoffTime(
com.google.shopping.merchant.accounts.v1beta.CutoffTime.Builder builderForValue) {
if (cutoffTimeBuilder_ == null) {
cutoffTime_ = builderForValue.build();
} else {
cutoffTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
public Builder mergeCutoffTime(com.google.shopping.merchant.accounts.v1beta.CutoffTime value) {
if (cutoffTimeBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& cutoffTime_ != null
&& cutoffTime_
!= com.google.shopping.merchant.accounts.v1beta.CutoffTime.getDefaultInstance()) {
getCutoffTimeBuilder().mergeFrom(value);
} else {
cutoffTime_ = value;
}
} else {
cutoffTimeBuilder_.mergeFrom(value);
}
if (cutoffTime_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
public Builder clearCutoffTime() {
bitField0_ = (bitField0_ & ~0x00000004);
cutoffTime_ = null;
if (cutoffTimeBuilder_ != null) {
cutoffTimeBuilder_.dispose();
cutoffTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
public com.google.shopping.merchant.accounts.v1beta.CutoffTime.Builder getCutoffTimeBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getCutoffTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
public com.google.shopping.merchant.accounts.v1beta.CutoffTimeOrBuilder
getCutoffTimeOrBuilder() {
if (cutoffTimeBuilder_ != null) {
return cutoffTimeBuilder_.getMessageOrBuilder();
} else {
return cutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.CutoffTime.getDefaultInstance()
: cutoffTime_;
}
}
/**
*
*
*
* Business days cutoff time definition.
* If not configured the cutoff time will be defaulted to 8AM PST.
*
*
* optional .google.shopping.merchant.accounts.v1beta.CutoffTime cutoff_time = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.CutoffTime,
com.google.shopping.merchant.accounts.v1beta.CutoffTime.Builder,
com.google.shopping.merchant.accounts.v1beta.CutoffTimeOrBuilder>
getCutoffTimeFieldBuilder() {
if (cutoffTimeBuilder_ == null) {
cutoffTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.CutoffTime,
com.google.shopping.merchant.accounts.v1beta.CutoffTime.Builder,
com.google.shopping.merchant.accounts.v1beta.CutoffTimeOrBuilder>(
getCutoffTime(), getParentForChildren(), isClean());
cutoffTime_ = null;
}
return cutoffTimeBuilder_;
}
private int minHandlingDays_;
/**
*
*
*
* Minimum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 min_handling_days = 4;
*
* @return Whether the minHandlingDays field is set.
*/
@java.lang.Override
public boolean hasMinHandlingDays() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Minimum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 min_handling_days = 4;
*
* @return The minHandlingDays.
*/
@java.lang.Override
public int getMinHandlingDays() {
return minHandlingDays_;
}
/**
*
*
*
* Minimum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 min_handling_days = 4;
*
* @param value The minHandlingDays to set.
* @return This builder for chaining.
*/
public Builder setMinHandlingDays(int value) {
minHandlingDays_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Minimum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 min_handling_days = 4;
*
* @return This builder for chaining.
*/
public Builder clearMinHandlingDays() {
bitField0_ = (bitField0_ & ~0x00000008);
minHandlingDays_ = 0;
onChanged();
return this;
}
private int maxHandlingDays_;
/**
*
*
*
* Maximum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* Must be greater than or equal to `min_handling_days`.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 max_handling_days = 5;
*
* @return Whether the maxHandlingDays field is set.
*/
@java.lang.Override
public boolean hasMaxHandlingDays() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Maximum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* Must be greater than or equal to `min_handling_days`.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 max_handling_days = 5;
*
* @return The maxHandlingDays.
*/
@java.lang.Override
public int getMaxHandlingDays() {
return maxHandlingDays_;
}
/**
*
*
*
* Maximum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* Must be greater than or equal to `min_handling_days`.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 max_handling_days = 5;
*
* @param value The maxHandlingDays to set.
* @return This builder for chaining.
*/
public Builder setMaxHandlingDays(int value) {
maxHandlingDays_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Maximum number of business days spent before an order is shipped.
* 0 means same day shipped, 1 means next day shipped.
* Must be greater than or equal to `min_handling_days`.
* 'min_handling_days' and 'max_handling_days' should be either set or not set
* at the same time.
*
*
* optional int32 max_handling_days = 5;
*
* @return This builder for chaining.
*/
public Builder clearMaxHandlingDays() {
bitField0_ = (bitField0_ & ~0x00000010);
maxHandlingDays_ = 0;
onChanged();
return this;
}
private com.google.shopping.merchant.accounts.v1beta.TransitTable transitTimeTable_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.TransitTable,
com.google.shopping.merchant.accounts.v1beta.TransitTable.Builder,
com.google.shopping.merchant.accounts.v1beta.TransitTableOrBuilder>
transitTimeTableBuilder_;
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*
* @return Whether the transitTimeTable field is set.
*/
public boolean hasTransitTimeTable() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*
* @return The transitTimeTable.
*/
public com.google.shopping.merchant.accounts.v1beta.TransitTable getTransitTimeTable() {
if (transitTimeTableBuilder_ == null) {
return transitTimeTable_ == null
? com.google.shopping.merchant.accounts.v1beta.TransitTable.getDefaultInstance()
: transitTimeTable_;
} else {
return transitTimeTableBuilder_.getMessage();
}
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
public Builder setTransitTimeTable(
com.google.shopping.merchant.accounts.v1beta.TransitTable value) {
if (transitTimeTableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
transitTimeTable_ = value;
} else {
transitTimeTableBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
public Builder setTransitTimeTable(
com.google.shopping.merchant.accounts.v1beta.TransitTable.Builder builderForValue) {
if (transitTimeTableBuilder_ == null) {
transitTimeTable_ = builderForValue.build();
} else {
transitTimeTableBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
public Builder mergeTransitTimeTable(
com.google.shopping.merchant.accounts.v1beta.TransitTable value) {
if (transitTimeTableBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& transitTimeTable_ != null
&& transitTimeTable_
!= com.google.shopping.merchant.accounts.v1beta.TransitTable.getDefaultInstance()) {
getTransitTimeTableBuilder().mergeFrom(value);
} else {
transitTimeTable_ = value;
}
} else {
transitTimeTableBuilder_.mergeFrom(value);
}
if (transitTimeTable_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
public Builder clearTransitTimeTable() {
bitField0_ = (bitField0_ & ~0x00000020);
transitTimeTable_ = null;
if (transitTimeTableBuilder_ != null) {
transitTimeTableBuilder_.dispose();
transitTimeTableBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
public com.google.shopping.merchant.accounts.v1beta.TransitTable.Builder
getTransitTimeTableBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getTransitTimeTableFieldBuilder().getBuilder();
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
public com.google.shopping.merchant.accounts.v1beta.TransitTableOrBuilder
getTransitTimeTableOrBuilder() {
if (transitTimeTableBuilder_ != null) {
return transitTimeTableBuilder_.getMessageOrBuilder();
} else {
return transitTimeTable_ == null
? com.google.shopping.merchant.accounts.v1beta.TransitTable.getDefaultInstance()
: transitTimeTable_;
}
}
/**
*
*
*
* Transit time table, number of business days spent in transit based on row
* and column dimensions. Either `min_transit_days`, `max_transit_days` or
* `transit_time_table` can be set, but not both.
*
*
* optional .google.shopping.merchant.accounts.v1beta.TransitTable transit_time_table = 6;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.TransitTable,
com.google.shopping.merchant.accounts.v1beta.TransitTable.Builder,
com.google.shopping.merchant.accounts.v1beta.TransitTableOrBuilder>
getTransitTimeTableFieldBuilder() {
if (transitTimeTableBuilder_ == null) {
transitTimeTableBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.TransitTable,
com.google.shopping.merchant.accounts.v1beta.TransitTable.Builder,
com.google.shopping.merchant.accounts.v1beta.TransitTableOrBuilder>(
getTransitTimeTable(), getParentForChildren(), isClean());
transitTimeTable_ = null;
}
return transitTimeTableBuilder_;
}
private com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
handlingBusinessDayConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder>
handlingBusinessDayConfigBuilder_;
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*
* @return Whether the handlingBusinessDayConfig field is set.
*/
public boolean hasHandlingBusinessDayConfig() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*
* @return The handlingBusinessDayConfig.
*/
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
getHandlingBusinessDayConfig() {
if (handlingBusinessDayConfigBuilder_ == null) {
return handlingBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: handlingBusinessDayConfig_;
} else {
return handlingBusinessDayConfigBuilder_.getMessage();
}
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
public Builder setHandlingBusinessDayConfig(
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig value) {
if (handlingBusinessDayConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
handlingBusinessDayConfig_ = value;
} else {
handlingBusinessDayConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
public Builder setHandlingBusinessDayConfig(
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder builderForValue) {
if (handlingBusinessDayConfigBuilder_ == null) {
handlingBusinessDayConfig_ = builderForValue.build();
} else {
handlingBusinessDayConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
public Builder mergeHandlingBusinessDayConfig(
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig value) {
if (handlingBusinessDayConfigBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)
&& handlingBusinessDayConfig_ != null
&& handlingBusinessDayConfig_
!= com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
.getDefaultInstance()) {
getHandlingBusinessDayConfigBuilder().mergeFrom(value);
} else {
handlingBusinessDayConfig_ = value;
}
} else {
handlingBusinessDayConfigBuilder_.mergeFrom(value);
}
if (handlingBusinessDayConfig_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
public Builder clearHandlingBusinessDayConfig() {
bitField0_ = (bitField0_ & ~0x00000040);
handlingBusinessDayConfig_ = null;
if (handlingBusinessDayConfigBuilder_ != null) {
handlingBusinessDayConfigBuilder_.dispose();
handlingBusinessDayConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder
getHandlingBusinessDayConfigBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getHandlingBusinessDayConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder
getHandlingBusinessDayConfigOrBuilder() {
if (handlingBusinessDayConfigBuilder_ != null) {
return handlingBusinessDayConfigBuilder_.getMessageOrBuilder();
} else {
return handlingBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: handlingBusinessDayConfig_;
}
}
/**
*
*
*
* The business days during which orders can be handled.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig handling_business_day_config = 7;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder>
getHandlingBusinessDayConfigFieldBuilder() {
if (handlingBusinessDayConfigBuilder_ == null) {
handlingBusinessDayConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder>(
getHandlingBusinessDayConfig(), getParentForChildren(), isClean());
handlingBusinessDayConfig_ = null;
}
return handlingBusinessDayConfigBuilder_;
}
private com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
transitBusinessDayConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder>
transitBusinessDayConfigBuilder_;
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*
* @return Whether the transitBusinessDayConfig field is set.
*/
public boolean hasTransitBusinessDayConfig() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*
* @return The transitBusinessDayConfig.
*/
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
getTransitBusinessDayConfig() {
if (transitBusinessDayConfigBuilder_ == null) {
return transitBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: transitBusinessDayConfig_;
} else {
return transitBusinessDayConfigBuilder_.getMessage();
}
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
public Builder setTransitBusinessDayConfig(
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig value) {
if (transitBusinessDayConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
transitBusinessDayConfig_ = value;
} else {
transitBusinessDayConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
public Builder setTransitBusinessDayConfig(
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder builderForValue) {
if (transitBusinessDayConfigBuilder_ == null) {
transitBusinessDayConfig_ = builderForValue.build();
} else {
transitBusinessDayConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
public Builder mergeTransitBusinessDayConfig(
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig value) {
if (transitBusinessDayConfigBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& transitBusinessDayConfig_ != null
&& transitBusinessDayConfig_
!= com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig
.getDefaultInstance()) {
getTransitBusinessDayConfigBuilder().mergeFrom(value);
} else {
transitBusinessDayConfig_ = value;
}
} else {
transitBusinessDayConfigBuilder_.mergeFrom(value);
}
if (transitBusinessDayConfig_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
public Builder clearTransitBusinessDayConfig() {
bitField0_ = (bitField0_ & ~0x00000080);
transitBusinessDayConfig_ = null;
if (transitBusinessDayConfigBuilder_ != null) {
transitBusinessDayConfigBuilder_.dispose();
transitBusinessDayConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder
getTransitBusinessDayConfigBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getTransitBusinessDayConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
public com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder
getTransitBusinessDayConfigOrBuilder() {
if (transitBusinessDayConfigBuilder_ != null) {
return transitBusinessDayConfigBuilder_.getMessageOrBuilder();
} else {
return transitBusinessDayConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.getDefaultInstance()
: transitBusinessDayConfig_;
}
}
/**
*
*
*
* The business days during which orders can be in-transit.
* If not provided, Monday to Friday business days will be assumed.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.BusinessDayConfig transit_business_day_config = 8;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder>
getTransitBusinessDayConfigFieldBuilder() {
if (transitBusinessDayConfigBuilder_ == null) {
transitBusinessDayConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.BusinessDayConfigOrBuilder>(
getTransitBusinessDayConfig(), getParentForChildren(), isClean());
transitBusinessDayConfig_ = null;
}
return transitBusinessDayConfigBuilder_;
}
private java.util.List
warehouseBasedDeliveryTimes_ = java.util.Collections.emptyList();
private void ensureWarehouseBasedDeliveryTimesIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
warehouseBasedDeliveryTimes_ =
new java.util.ArrayList<
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime>(
warehouseBasedDeliveryTimes_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTimeOrBuilder>
warehouseBasedDeliveryTimesBuilder_;
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getWarehouseBasedDeliveryTimesList() {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
return java.util.Collections.unmodifiableList(warehouseBasedDeliveryTimes_);
} else {
return warehouseBasedDeliveryTimesBuilder_.getMessageList();
}
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public int getWarehouseBasedDeliveryTimesCount() {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
return warehouseBasedDeliveryTimes_.size();
} else {
return warehouseBasedDeliveryTimesBuilder_.getCount();
}
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime
getWarehouseBasedDeliveryTimes(int index) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
return warehouseBasedDeliveryTimes_.get(index);
} else {
return warehouseBasedDeliveryTimesBuilder_.getMessage(index);
}
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setWarehouseBasedDeliveryTimes(
int index, com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime value) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.set(index, value);
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setWarehouseBasedDeliveryTimes(
int index,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder
builderForValue) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.set(index, builderForValue.build());
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addWarehouseBasedDeliveryTimes(
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime value) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.add(value);
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addWarehouseBasedDeliveryTimes(
int index, com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime value) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.add(index, value);
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addWarehouseBasedDeliveryTimes(
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder
builderForValue) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.add(builderForValue.build());
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addWarehouseBasedDeliveryTimes(
int index,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder
builderForValue) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.add(index, builderForValue.build());
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAllWarehouseBasedDeliveryTimes(
java.lang.Iterable<
? extends com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime>
values) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
ensureWarehouseBasedDeliveryTimesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, warehouseBasedDeliveryTimes_);
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearWarehouseBasedDeliveryTimes() {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
warehouseBasedDeliveryTimes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder removeWarehouseBasedDeliveryTimes(int index) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
ensureWarehouseBasedDeliveryTimesIsMutable();
warehouseBasedDeliveryTimes_.remove(index);
onChanged();
} else {
warehouseBasedDeliveryTimesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder
getWarehouseBasedDeliveryTimesBuilder(int index) {
return getWarehouseBasedDeliveryTimesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTimeOrBuilder
getWarehouseBasedDeliveryTimesOrBuilder(int index) {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
return warehouseBasedDeliveryTimes_.get(index);
} else {
return warehouseBasedDeliveryTimesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List<
? extends
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTimeOrBuilder>
getWarehouseBasedDeliveryTimesOrBuilderList() {
if (warehouseBasedDeliveryTimesBuilder_ != null) {
return warehouseBasedDeliveryTimesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(warehouseBasedDeliveryTimes_);
}
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder
addWarehouseBasedDeliveryTimesBuilder() {
return getWarehouseBasedDeliveryTimesFieldBuilder()
.addBuilder(
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime
.getDefaultInstance());
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder
addWarehouseBasedDeliveryTimesBuilder(int index) {
return getWarehouseBasedDeliveryTimesFieldBuilder()
.addBuilder(
index,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime
.getDefaultInstance());
}
/**
*
*
*
* Optional. Indicates that the delivery time should be calculated per
* warehouse (shipping origin location) based on the settings of the selected
* carrier. When set, no other transit time related field in [delivery
* time][[google.shopping.content.bundles.ShippingSetting.DeliveryTime] should
* be set.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime warehouse_based_delivery_times = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List<
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder>
getWarehouseBasedDeliveryTimesBuilderList() {
return getWarehouseBasedDeliveryTimesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTimeOrBuilder>
getWarehouseBasedDeliveryTimesFieldBuilder() {
if (warehouseBasedDeliveryTimesBuilder_ == null) {
warehouseBasedDeliveryTimesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTime.Builder,
com.google.shopping.merchant.accounts.v1beta.WarehouseBasedDeliveryTimeOrBuilder>(
warehouseBasedDeliveryTimes_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
warehouseBasedDeliveryTimes_ = null;
}
return warehouseBasedDeliveryTimesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.shopping.merchant.accounts.v1beta.DeliveryTime)
}
// @@protoc_insertion_point(class_scope:google.shopping.merchant.accounts.v1beta.DeliveryTime)
private static final com.google.shopping.merchant.accounts.v1beta.DeliveryTime DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.shopping.merchant.accounts.v1beta.DeliveryTime();
}
public static com.google.shopping.merchant.accounts.v1beta.DeliveryTime getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeliveryTime parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.DeliveryTime getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy