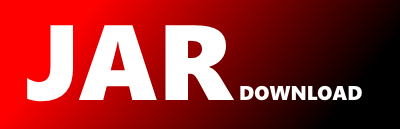
com.google.shopping.merchant.accounts.v1beta.Service Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/shopping/merchant/accounts/v1beta/shippingsettings.proto
// Protobuf Java Version: 3.25.5
package com.google.shopping.merchant.accounts.v1beta;
/**
*
*
*
* Shipping service.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.Service}
*/
public final class Service extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.shopping.merchant.accounts.v1beta.Service)
ServiceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Service.newBuilder() to construct.
private Service(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Service() {
serviceName_ = "";
deliveryCountries_ = com.google.protobuf.LazyStringArrayList.emptyList();
currencyCode_ = "";
rateGroups_ = java.util.Collections.emptyList();
shipmentType_ = 0;
loyaltyPrograms_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Service();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.class,
com.google.shopping.merchant.accounts.v1beta.Service.Builder.class);
}
/**
*
*
*
* Shipment type of shipping service.
*
*
* Protobuf enum {@code google.shopping.merchant.accounts.v1beta.Service.ShipmentType}
*/
public enum ShipmentType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* This service did not specify shipment type.
*
*
* SHIPMENT_TYPE_UNSPECIFIED = 0;
*/
SHIPMENT_TYPE_UNSPECIFIED(0),
/**
*
*
*
* This service ships orders to an address chosen by the customer.
*
*
* DELIVERY = 1;
*/
DELIVERY(1),
/**
*
*
*
* This service ships orders to an address chosen by the customer.
* The order is shipped from a local store near by.
*
*
* LOCAL_DELIVERY = 2;
*/
LOCAL_DELIVERY(2),
/**
*
*
*
* This service ships orders to an address chosen by the customer.
* The order is shipped from a collection point.
*
*
* COLLECTION_POINT = 3;
*/
COLLECTION_POINT(3),
UNRECOGNIZED(-1),
;
/**
*
*
*
* This service did not specify shipment type.
*
*
* SHIPMENT_TYPE_UNSPECIFIED = 0;
*/
public static final int SHIPMENT_TYPE_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* This service ships orders to an address chosen by the customer.
*
*
* DELIVERY = 1;
*/
public static final int DELIVERY_VALUE = 1;
/**
*
*
*
* This service ships orders to an address chosen by the customer.
* The order is shipped from a local store near by.
*
*
* LOCAL_DELIVERY = 2;
*/
public static final int LOCAL_DELIVERY_VALUE = 2;
/**
*
*
*
* This service ships orders to an address chosen by the customer.
* The order is shipped from a collection point.
*
*
* COLLECTION_POINT = 3;
*/
public static final int COLLECTION_POINT_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ShipmentType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ShipmentType forNumber(int value) {
switch (value) {
case 0:
return SHIPMENT_TYPE_UNSPECIFIED;
case 1:
return DELIVERY;
case 2:
return LOCAL_DELIVERY;
case 3:
return COLLECTION_POINT;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ShipmentType findValueByNumber(int number) {
return ShipmentType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.Service.getDescriptor()
.getEnumTypes()
.get(0);
}
private static final ShipmentType[] VALUES = values();
public static ShipmentType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ShipmentType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.shopping.merchant.accounts.v1beta.Service.ShipmentType)
}
public interface StoreConfigOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.shopping.merchant.accounts.v1beta.Service.StoreConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return Whether the storeServiceType field is set.
*/
boolean hasStoreServiceType();
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return The enum numeric value on the wire for storeServiceType.
*/
int getStoreServiceTypeValue();
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return The storeServiceType.
*/
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType
getStoreServiceType();
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return A list containing the storeCodes.
*/
java.util.List getStoreCodesList();
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The count of storeCodes.
*/
int getStoreCodesCount();
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the element to return.
* @return The storeCodes at the given index.
*/
java.lang.String getStoreCodes(int index);
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the value to return.
* @return The bytes of the storeCodes at the given index.
*/
com.google.protobuf.ByteString getStoreCodesBytes(int index);
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*
* @return Whether the cutoffConfig field is set.
*/
boolean hasCutoffConfig();
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*
* @return The cutoffConfig.
*/
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig getCutoffConfig();
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfigOrBuilder
getCutoffConfigOrBuilder();
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
* @return Whether the serviceRadius field is set.
*/
boolean hasServiceRadius();
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
* @return The serviceRadius.
*/
com.google.shopping.merchant.accounts.v1beta.Distance getServiceRadius();
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*/
com.google.shopping.merchant.accounts.v1beta.DistanceOrBuilder getServiceRadiusOrBuilder();
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.Service.StoreConfig}
*/
public static final class StoreConfig extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.shopping.merchant.accounts.v1beta.Service.StoreConfig)
StoreConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use StoreConfig.newBuilder() to construct.
private StoreConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StoreConfig() {
storeServiceType_ = 0;
storeCodes_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new StoreConfig();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.class,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.Builder.class);
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by the
* merchant provide local delivery.
*
*
* Protobuf enum {@code
* google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType}
*/
public enum StoreServiceType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* Did not specify store service type.
*
*
* STORE_SERVICE_TYPE_UNSPECIFIED = 0;
*/
STORE_SERVICE_TYPE_UNSPECIFIED(0),
/**
*
*
*
* Indicates whether all stores, current and future, listed by this
* merchant provide local delivery.
*
*
* ALL_STORES = 1;
*/
ALL_STORES(1),
/**
*
*
*
* Indicates that only the stores listed in `store_codes` are eligible
* for local delivery.
*
*
* SELECTED_STORES = 2;
*/
SELECTED_STORES(2),
UNRECOGNIZED(-1),
;
/**
*
*
*
* Did not specify store service type.
*
*
* STORE_SERVICE_TYPE_UNSPECIFIED = 0;
*/
public static final int STORE_SERVICE_TYPE_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* Indicates whether all stores, current and future, listed by this
* merchant provide local delivery.
*
*
* ALL_STORES = 1;
*/
public static final int ALL_STORES_VALUE = 1;
/**
*
*
*
* Indicates that only the stores listed in `store_codes` are eligible
* for local delivery.
*
*
* SELECTED_STORES = 2;
*/
public static final int SELECTED_STORES_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StoreServiceType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static StoreServiceType forNumber(int value) {
switch (value) {
case 0:
return STORE_SERVICE_TYPE_UNSPECIFIED;
case 1:
return ALL_STORES;
case 2:
return SELECTED_STORES;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public StoreServiceType findValueByNumber(int number) {
return StoreServiceType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.getDescriptor()
.getEnumTypes()
.get(0);
}
private static final StoreServiceType[] VALUES = values();
public static StoreServiceType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private StoreServiceType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType)
}
public interface CutoffConfigOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*
* @return Whether the localCutoffTime field is set.
*/
boolean hasLocalCutoffTime();
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*
* @return The localCutoffTime.
*/
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime
getLocalCutoffTime();
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTimeOrBuilder
getLocalCutoffTimeOrBuilder();
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @return Whether the storeCloseOffsetHours field is set.
*/
boolean hasStoreCloseOffsetHours();
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @return The storeCloseOffsetHours.
*/
long getStoreCloseOffsetHours();
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @return Whether the noDeliveryPostCutoff field is set.
*/
boolean hasNoDeliveryPostCutoff();
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @return The noDeliveryPostCutoff.
*/
boolean getNoDeliveryPostCutoff();
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
* Protobuf type {@code
* google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig}
*/
public static final class CutoffConfig extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig)
CutoffConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use CutoffConfig.newBuilder() to construct.
private CutoffConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CutoffConfig() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new CutoffConfig();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.class,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.Builder.class);
}
public interface LocalCutoffTimeOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @return Whether the hour field is set.
*/
boolean hasHour();
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @return The hour.
*/
long getHour();
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @return Whether the minute field is set.
*/
boolean hasMinute();
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @return The minute.
*/
long getMinute();
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
* Protobuf type {@code
* google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime}
*/
public static final class LocalCutoffTime extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime)
LocalCutoffTimeOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocalCutoffTime.newBuilder() to construct.
private LocalCutoffTime(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocalCutoffTime() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new LocalCutoffTime();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_LocalCutoffTime_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_LocalCutoffTime_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.class,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.Builder.class);
}
private int bitField0_;
public static final int HOUR_FIELD_NUMBER = 1;
private long hour_ = 0L;
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @return Whether the hour field is set.
*/
@java.lang.Override
public boolean hasHour() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @return The hour.
*/
@java.lang.Override
public long getHour() {
return hour_;
}
public static final int MINUTE_FIELD_NUMBER = 2;
private long minute_ = 0L;
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @return Whether the minute field is set.
*/
@java.lang.Override
public boolean hasMinute() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @return The minute.
*/
@java.lang.Override
public long getMinute() {
return minute_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, hour_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, minute_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(1, hour_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(2, minute_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj
instanceof
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime)) {
return super.equals(obj);
}
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
other =
(com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime)
obj;
if (hasHour() != other.hasHour()) return false;
if (hasHour()) {
if (getHour() != other.getHour()) return false;
}
if (hasMinute() != other.hasMinute()) return false;
if (hasMinute()) {
if (getMinute() != other.getMinute()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHour()) {
hash = (37 * hash) + HOUR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getHour());
}
if (hasMinute()) {
hash = (37 * hash) + MINUTE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getMinute());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
* Protobuf type {@code
* google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime)
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTimeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_LocalCutoffTime_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_LocalCutoffTime_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.class,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.Builder.class);
}
// Construct using
// com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
hour_ = 0L;
minute_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_LocalCutoffTime_descriptor;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
getDefaultInstanceForType() {
return com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.getDefaultInstance();
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
build() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
buildPartial() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
result =
new com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.CutoffConfig.LocalCutoffTime(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.hour_ = hour_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.minute_ = minute_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other
instanceof
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime) {
return mergeFrom(
(com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime)
other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
other) {
if (other
== com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.getDefaultInstance()) return this;
if (other.hasHour()) {
setHour(other.getHour());
}
if (other.hasMinute()) {
setMinute(other.getMinute());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
hour_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16:
{
minute_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long hour_;
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @return Whether the hour field is set.
*/
@java.lang.Override
public boolean hasHour() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @return The hour.
*/
@java.lang.Override
public long getHour() {
return hour_;
}
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @param value The hour to set.
* @return This builder for chaining.
*/
public Builder setHour(long value) {
hour_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Hour local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 hour = 1;
*
* @return This builder for chaining.
*/
public Builder clearHour() {
bitField0_ = (bitField0_ & ~0x00000001);
hour_ = 0L;
onChanged();
return this;
}
private long minute_;
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @return Whether the minute field is set.
*/
@java.lang.Override
public boolean hasMinute() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @return The minute.
*/
@java.lang.Override
public long getMinute() {
return minute_;
}
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @param value The minute to set.
* @return This builder for chaining.
*/
public Builder setMinute(long value) {
minute_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Minute local delivery orders must be placed by to process the same
* day.
*
*
* optional int64 minute = 2;
*
* @return This builder for chaining.
*/
public Builder clearMinute() {
bitField0_ = (bitField0_ & ~0x00000002);
minute_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime)
}
// @@protoc_insertion_point(class_scope:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime)
private static final com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.CutoffConfig.LocalCutoffTime
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE =
new com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime();
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LocalCutoffTime parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int LOCAL_CUTOFF_TIME_FIELD_NUMBER = 1;
private com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
localCutoffTime_;
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*
* @return Whether the localCutoffTime field is set.
*/
@java.lang.Override
public boolean hasLocalCutoffTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*
* @return The localCutoffTime.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
getLocalCutoffTime() {
return localCutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.getDefaultInstance()
: localCutoffTime_;
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTimeOrBuilder
getLocalCutoffTimeOrBuilder() {
return localCutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.getDefaultInstance()
: localCutoffTime_;
}
public static final int STORE_CLOSE_OFFSET_HOURS_FIELD_NUMBER = 2;
private long storeCloseOffsetHours_ = 0L;
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @return Whether the storeCloseOffsetHours field is set.
*/
@java.lang.Override
public boolean hasStoreCloseOffsetHours() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @return The storeCloseOffsetHours.
*/
@java.lang.Override
public long getStoreCloseOffsetHours() {
return storeCloseOffsetHours_;
}
public static final int NO_DELIVERY_POST_CUTOFF_FIELD_NUMBER = 3;
private boolean noDeliveryPostCutoff_ = false;
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @return Whether the noDeliveryPostCutoff field is set.
*/
@java.lang.Override
public boolean hasNoDeliveryPostCutoff() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @return The noDeliveryPostCutoff.
*/
@java.lang.Override
public boolean getNoDeliveryPostCutoff() {
return noDeliveryPostCutoff_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getLocalCutoffTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, storeCloseOffsetHours_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(3, noDeliveryPostCutoff_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getLocalCutoffTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(2, storeCloseOffsetHours_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(3, noDeliveryPostCutoff_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj
instanceof
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig)) {
return super.equals(obj);
}
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig other =
(com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig) obj;
if (hasLocalCutoffTime() != other.hasLocalCutoffTime()) return false;
if (hasLocalCutoffTime()) {
if (!getLocalCutoffTime().equals(other.getLocalCutoffTime())) return false;
}
if (hasStoreCloseOffsetHours() != other.hasStoreCloseOffsetHours()) return false;
if (hasStoreCloseOffsetHours()) {
if (getStoreCloseOffsetHours() != other.getStoreCloseOffsetHours()) return false;
}
if (hasNoDeliveryPostCutoff() != other.hasNoDeliveryPostCutoff()) return false;
if (hasNoDeliveryPostCutoff()) {
if (getNoDeliveryPostCutoff() != other.getNoDeliveryPostCutoff()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLocalCutoffTime()) {
hash = (37 * hash) + LOCAL_CUTOFF_TIME_FIELD_NUMBER;
hash = (53 * hash) + getLocalCutoffTime().hashCode();
}
if (hasStoreCloseOffsetHours()) {
hash = (37 * hash) + STORE_CLOSE_OFFSET_HOURS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getStoreCloseOffsetHours());
}
if (hasNoDeliveryPostCutoff()) {
hash = (37 * hash) + NO_DELIVERY_POST_CUTOFF_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getNoDeliveryPostCutoff());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
* Protobuf type {@code
* google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig)
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.class,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.Builder.class);
}
// Construct using
// com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getLocalCutoffTimeFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
localCutoffTime_ = null;
if (localCutoffTimeBuilder_ != null) {
localCutoffTimeBuilder_.dispose();
localCutoffTimeBuilder_ = null;
}
storeCloseOffsetHours_ = 0L;
noDeliveryPostCutoff_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_CutoffConfig_descriptor;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
getDefaultInstanceForType() {
return com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.getDefaultInstance();
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
build() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig result =
buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
buildPartial() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig result =
new com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig(
this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.localCutoffTime_ =
localCutoffTimeBuilder_ == null
? localCutoffTime_
: localCutoffTimeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.storeCloseOffsetHours_ = storeCloseOffsetHours_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.noDeliveryPostCutoff_ = noDeliveryPostCutoff_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other
instanceof
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig) {
return mergeFrom(
(com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig)
other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig other) {
if (other
== com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.getDefaultInstance()) return this;
if (other.hasLocalCutoffTime()) {
mergeLocalCutoffTime(other.getLocalCutoffTime());
}
if (other.hasStoreCloseOffsetHours()) {
setStoreCloseOffsetHours(other.getStoreCloseOffsetHours());
}
if (other.hasNoDeliveryPostCutoff()) {
setNoDeliveryPostCutoff(other.getNoDeliveryPostCutoff());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(
getLocalCutoffTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16:
{
storeCloseOffsetHours_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24:
{
noDeliveryPostCutoff_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
localCutoffTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTimeOrBuilder>
localCutoffTimeBuilder_;
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*
* @return Whether the localCutoffTime field is set.
*/
public boolean hasLocalCutoffTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*
* @return The localCutoffTime.
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
getLocalCutoffTime() {
if (localCutoffTimeBuilder_ == null) {
return localCutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.getDefaultInstance()
: localCutoffTime_;
} else {
return localCutoffTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
public Builder setLocalCutoffTime(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
value) {
if (localCutoffTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localCutoffTime_ = value;
} else {
localCutoffTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
public Builder setLocalCutoffTime(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.Builder
builderForValue) {
if (localCutoffTimeBuilder_ == null) {
localCutoffTime_ = builderForValue.build();
} else {
localCutoffTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
public Builder mergeLocalCutoffTime(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime
value) {
if (localCutoffTimeBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& localCutoffTime_ != null
&& localCutoffTime_
!= com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.getDefaultInstance()) {
getLocalCutoffTimeBuilder().mergeFrom(value);
} else {
localCutoffTime_ = value;
}
} else {
localCutoffTimeBuilder_.mergeFrom(value);
}
if (localCutoffTime_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
public Builder clearLocalCutoffTime() {
bitField0_ = (bitField0_ & ~0x00000001);
localCutoffTime_ = null;
if (localCutoffTimeBuilder_ != null) {
localCutoffTimeBuilder_.dispose();
localCutoffTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.Builder
getLocalCutoffTimeBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getLocalCutoffTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTimeOrBuilder
getLocalCutoffTimeOrBuilder() {
if (localCutoffTimeBuilder_ != null) {
return localCutoffTimeBuilder_.getMessageOrBuilder();
} else {
return localCutoffTime_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.getDefaultInstance()
: localCutoffTime_;
}
}
/**
*
*
*
* Time that local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.LocalCutoffTime local_cutoff_time = 1;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTimeOrBuilder>
getLocalCutoffTimeFieldBuilder() {
if (localCutoffTimeBuilder_ == null) {
localCutoffTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTime.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.LocalCutoffTimeOrBuilder>(
getLocalCutoffTime(), getParentForChildren(), isClean());
localCutoffTime_ = null;
}
return localCutoffTimeBuilder_;
}
private long storeCloseOffsetHours_;
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @return Whether the storeCloseOffsetHours field is set.
*/
@java.lang.Override
public boolean hasStoreCloseOffsetHours() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @return The storeCloseOffsetHours.
*/
@java.lang.Override
public long getStoreCloseOffsetHours() {
return storeCloseOffsetHours_;
}
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @param value The storeCloseOffsetHours to set.
* @return This builder for chaining.
*/
public Builder setStoreCloseOffsetHours(long value) {
storeCloseOffsetHours_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Only valid with local delivery fulfillment. Represents cutoff time
* as the number of hours before store closing. Mutually exclusive
* with `local_cutoff_time`.
*
*
* optional int64 store_close_offset_hours = 2;
*
* @return This builder for chaining.
*/
public Builder clearStoreCloseOffsetHours() {
bitField0_ = (bitField0_ & ~0x00000002);
storeCloseOffsetHours_ = 0L;
onChanged();
return this;
}
private boolean noDeliveryPostCutoff_;
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @return Whether the noDeliveryPostCutoff field is set.
*/
@java.lang.Override
public boolean hasNoDeliveryPostCutoff() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @return The noDeliveryPostCutoff.
*/
@java.lang.Override
public boolean getNoDeliveryPostCutoff() {
return noDeliveryPostCutoff_;
}
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @param value The noDeliveryPostCutoff to set.
* @return This builder for chaining.
*/
public Builder setNoDeliveryPostCutoff(boolean value) {
noDeliveryPostCutoff_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Merchants can opt-out of showing n+1 day local delivery when they have
* a shipping service configured to n day local delivery. For example, if
* the shipping service defines same-day delivery, and it's past the
* cut-off, setting this field to `true` results in the calculated
* shipping service rate returning `NO_DELIVERY_POST_CUTOFF`. In the
* same example, setting this field to `false` results in the calculated
* shipping time being one day. This is only for local delivery.
*
*
* optional bool no_delivery_post_cutoff = 3;
*
* @return This builder for chaining.
*/
public Builder clearNoDeliveryPostCutoff() {
bitField0_ = (bitField0_ & ~0x00000004);
noDeliveryPostCutoff_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig)
}
// @@protoc_insertion_point(class_scope:google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig)
private static final com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.CutoffConfig
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE =
new com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig();
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CutoffConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int STORE_SERVICE_TYPE_FIELD_NUMBER = 1;
private int storeServiceType_ = 0;
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return Whether the storeServiceType field is set.
*/
@java.lang.Override
public boolean hasStoreServiceType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return The enum numeric value on the wire for storeServiceType.
*/
@java.lang.Override
public int getStoreServiceTypeValue() {
return storeServiceType_;
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return The storeServiceType.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType
getStoreServiceType() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType result =
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType
.forNumber(storeServiceType_);
return result == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType
.UNRECOGNIZED
: result;
}
public static final int STORE_CODES_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList storeCodes_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return A list containing the storeCodes.
*/
public com.google.protobuf.ProtocolStringList getStoreCodesList() {
return storeCodes_;
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The count of storeCodes.
*/
public int getStoreCodesCount() {
return storeCodes_.size();
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the element to return.
* @return The storeCodes at the given index.
*/
public java.lang.String getStoreCodes(int index) {
return storeCodes_.get(index);
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the value to return.
* @return The bytes of the storeCodes at the given index.
*/
public com.google.protobuf.ByteString getStoreCodesBytes(int index) {
return storeCodes_.getByteString(index);
}
public static final int CUTOFF_CONFIG_FIELD_NUMBER = 3;
private com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
cutoffConfig_;
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*
* @return Whether the cutoffConfig field is set.
*/
@java.lang.Override
public boolean hasCutoffConfig() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*
* @return The cutoffConfig.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
getCutoffConfig() {
return cutoffConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.getDefaultInstance()
: cutoffConfig_;
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfigOrBuilder
getCutoffConfigOrBuilder() {
return cutoffConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.getDefaultInstance()
: cutoffConfig_;
}
public static final int SERVICE_RADIUS_FIELD_NUMBER = 4;
private com.google.shopping.merchant.accounts.v1beta.Distance serviceRadius_;
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
* @return Whether the serviceRadius field is set.
*/
@java.lang.Override
public boolean hasServiceRadius() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
* @return The serviceRadius.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Distance getServiceRadius() {
return serviceRadius_ == null
? com.google.shopping.merchant.accounts.v1beta.Distance.getDefaultInstance()
: serviceRadius_;
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.DistanceOrBuilder
getServiceRadiusOrBuilder() {
return serviceRadius_ == null
? com.google.shopping.merchant.accounts.v1beta.Distance.getDefaultInstance()
: serviceRadius_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, storeServiceType_);
}
for (int i = 0; i < storeCodes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, storeCodes_.getRaw(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getCutoffConfig());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(4, getServiceRadius());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(1, storeServiceType_);
}
{
int dataSize = 0;
for (int i = 0; i < storeCodes_.size(); i++) {
dataSize += computeStringSizeNoTag(storeCodes_.getRaw(i));
}
size += dataSize;
size += 1 * getStoreCodesList().size();
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getCutoffConfig());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getServiceRadius());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig)) {
return super.equals(obj);
}
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig other =
(com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig) obj;
if (hasStoreServiceType() != other.hasStoreServiceType()) return false;
if (hasStoreServiceType()) {
if (storeServiceType_ != other.storeServiceType_) return false;
}
if (!getStoreCodesList().equals(other.getStoreCodesList())) return false;
if (hasCutoffConfig() != other.hasCutoffConfig()) return false;
if (hasCutoffConfig()) {
if (!getCutoffConfig().equals(other.getCutoffConfig())) return false;
}
if (hasServiceRadius() != other.hasServiceRadius()) return false;
if (hasServiceRadius()) {
if (!getServiceRadius().equals(other.getServiceRadius())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStoreServiceType()) {
hash = (37 * hash) + STORE_SERVICE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + storeServiceType_;
}
if (getStoreCodesCount() > 0) {
hash = (37 * hash) + STORE_CODES_FIELD_NUMBER;
hash = (53 * hash) + getStoreCodesList().hashCode();
}
if (hasCutoffConfig()) {
hash = (37 * hash) + CUTOFF_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getCutoffConfig().hashCode();
}
if (hasServiceRadius()) {
hash = (37 * hash) + SERVICE_RADIUS_FIELD_NUMBER;
hash = (53 * hash) + getServiceRadius().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.Service.StoreConfig}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.shopping.merchant.accounts.v1beta.Service.StoreConfig)
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.class,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.Builder.class);
}
// Construct using
// com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getCutoffConfigFieldBuilder();
getServiceRadiusFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
storeServiceType_ = 0;
storeCodes_ = com.google.protobuf.LazyStringArrayList.emptyList();
cutoffConfig_ = null;
if (cutoffConfigBuilder_ != null) {
cutoffConfigBuilder_.dispose();
cutoffConfigBuilder_ = null;
}
serviceRadius_ = null;
if (serviceRadiusBuilder_ != null) {
serviceRadiusBuilder_.dispose();
serviceRadiusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_StoreConfig_descriptor;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
getDefaultInstanceForType() {
return com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.getDefaultInstance();
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig build() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig buildPartial() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig result =
new com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.storeServiceType_ = storeServiceType_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
storeCodes_.makeImmutable();
result.storeCodes_ = storeCodes_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.cutoffConfig_ =
cutoffConfigBuilder_ == null ? cutoffConfig_ : cutoffConfigBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.serviceRadius_ =
serviceRadiusBuilder_ == null ? serviceRadius_ : serviceRadiusBuilder_.build();
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig) {
return mergeFrom(
(com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig other) {
if (other
== com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.getDefaultInstance()) return this;
if (other.hasStoreServiceType()) {
setStoreServiceType(other.getStoreServiceType());
}
if (!other.storeCodes_.isEmpty()) {
if (storeCodes_.isEmpty()) {
storeCodes_ = other.storeCodes_;
bitField0_ |= 0x00000002;
} else {
ensureStoreCodesIsMutable();
storeCodes_.addAll(other.storeCodes_);
}
onChanged();
}
if (other.hasCutoffConfig()) {
mergeCutoffConfig(other.getCutoffConfig());
}
if (other.hasServiceRadius()) {
mergeServiceRadius(other.getServiceRadius());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
storeServiceType_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18:
{
java.lang.String s = input.readStringRequireUtf8();
ensureStoreCodesIsMutable();
storeCodes_.add(s);
break;
} // case 18
case 26:
{
input.readMessage(getCutoffConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34:
{
input.readMessage(getServiceRadiusFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int storeServiceType_ = 0;
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return Whether the storeServiceType field is set.
*/
@java.lang.Override
public boolean hasStoreServiceType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return The enum numeric value on the wire for storeServiceType.
*/
@java.lang.Override
public int getStoreServiceTypeValue() {
return storeServiceType_;
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @param value The enum numeric value on the wire for storeServiceType to set.
* @return This builder for chaining.
*/
public Builder setStoreServiceTypeValue(int value) {
storeServiceType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return The storeServiceType.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType
getStoreServiceType() {
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType result =
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType
.forNumber(storeServiceType_);
return result == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType
.UNRECOGNIZED
: result;
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @param value The storeServiceType to set.
* @return This builder for chaining.
*/
public Builder setStoreServiceType(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
storeServiceType_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Indicates whether all stores, or selected stores, listed by this
* merchant provide local delivery.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.StoreServiceType store_service_type = 1;
*
*
* @return This builder for chaining.
*/
public Builder clearStoreServiceType() {
bitField0_ = (bitField0_ & ~0x00000001);
storeServiceType_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList storeCodes_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureStoreCodesIsMutable() {
if (!storeCodes_.isModifiable()) {
storeCodes_ = new com.google.protobuf.LazyStringArrayList(storeCodes_);
}
bitField0_ |= 0x00000002;
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return A list containing the storeCodes.
*/
public com.google.protobuf.ProtocolStringList getStoreCodesList() {
storeCodes_.makeImmutable();
return storeCodes_;
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The count of storeCodes.
*/
public int getStoreCodesCount() {
return storeCodes_.size();
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the element to return.
* @return The storeCodes at the given index.
*/
public java.lang.String getStoreCodes(int index) {
return storeCodes_.get(index);
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the value to return.
* @return The bytes of the storeCodes at the given index.
*/
public com.google.protobuf.ByteString getStoreCodesBytes(int index) {
return storeCodes_.getByteString(index);
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index to set the value at.
* @param value The storeCodes to set.
* @return This builder for chaining.
*/
public Builder setStoreCodes(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStoreCodesIsMutable();
storeCodes_.set(index, value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The storeCodes to add.
* @return This builder for chaining.
*/
public Builder addStoreCodes(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStoreCodesIsMutable();
storeCodes_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param values The storeCodes to add.
* @return This builder for chaining.
*/
public Builder addAllStoreCodes(java.lang.Iterable values) {
ensureStoreCodesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, storeCodes_);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return This builder for chaining.
*/
public Builder clearStoreCodes() {
storeCodes_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of store codes that provide local delivery.
* If empty, then `all_stores` must be true.
*
*
* repeated string store_codes = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The bytes of the storeCodes to add.
* @return This builder for chaining.
*/
public Builder addStoreCodesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureStoreCodesIsMutable();
storeCodes_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
cutoffConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.CutoffConfigOrBuilder>
cutoffConfigBuilder_;
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*
* @return Whether the cutoffConfig field is set.
*/
public boolean hasCutoffConfig() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*
* @return The cutoffConfig.
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
getCutoffConfig() {
if (cutoffConfigBuilder_ == null) {
return cutoffConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.getDefaultInstance()
: cutoffConfig_;
} else {
return cutoffConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
public Builder setCutoffConfig(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig value) {
if (cutoffConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cutoffConfig_ = value;
} else {
cutoffConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
public Builder setCutoffConfig(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.Builder
builderForValue) {
if (cutoffConfigBuilder_ == null) {
cutoffConfig_ = builderForValue.build();
} else {
cutoffConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
public Builder mergeCutoffConfig(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig value) {
if (cutoffConfigBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& cutoffConfig_ != null
&& cutoffConfig_
!= com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.getDefaultInstance()) {
getCutoffConfigBuilder().mergeFrom(value);
} else {
cutoffConfig_ = value;
}
} else {
cutoffConfigBuilder_.mergeFrom(value);
}
if (cutoffConfig_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
public Builder clearCutoffConfig() {
bitField0_ = (bitField0_ & ~0x00000004);
cutoffConfig_ = null;
if (cutoffConfigBuilder_ != null) {
cutoffConfigBuilder_.dispose();
cutoffConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.Builder
getCutoffConfigBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getCutoffConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfigOrBuilder
getCutoffConfigOrBuilder() {
if (cutoffConfigBuilder_ != null) {
return cutoffConfigBuilder_.getMessageOrBuilder();
} else {
return cutoffConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.getDefaultInstance()
: cutoffConfig_;
}
}
/**
*
*
*
* Configs related to local delivery ends for the day.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig cutoff_config = 3;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.CutoffConfigOrBuilder>
getCutoffConfigFieldBuilder() {
if (cutoffConfigBuilder_ == null) {
cutoffConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.CutoffConfig
.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.CutoffConfigOrBuilder>(getCutoffConfig(), getParentForChildren(), isClean());
cutoffConfig_ = null;
}
return cutoffConfigBuilder_;
}
private com.google.shopping.merchant.accounts.v1beta.Distance serviceRadius_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Distance,
com.google.shopping.merchant.accounts.v1beta.Distance.Builder,
com.google.shopping.merchant.accounts.v1beta.DistanceOrBuilder>
serviceRadiusBuilder_;
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*
* @return Whether the serviceRadius field is set.
*/
public boolean hasServiceRadius() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*
* @return The serviceRadius.
*/
public com.google.shopping.merchant.accounts.v1beta.Distance getServiceRadius() {
if (serviceRadiusBuilder_ == null) {
return serviceRadius_ == null
? com.google.shopping.merchant.accounts.v1beta.Distance.getDefaultInstance()
: serviceRadius_;
} else {
return serviceRadiusBuilder_.getMessage();
}
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*/
public Builder setServiceRadius(com.google.shopping.merchant.accounts.v1beta.Distance value) {
if (serviceRadiusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
serviceRadius_ = value;
} else {
serviceRadiusBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*/
public Builder setServiceRadius(
com.google.shopping.merchant.accounts.v1beta.Distance.Builder builderForValue) {
if (serviceRadiusBuilder_ == null) {
serviceRadius_ = builderForValue.build();
} else {
serviceRadiusBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*/
public Builder mergeServiceRadius(
com.google.shopping.merchant.accounts.v1beta.Distance value) {
if (serviceRadiusBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& serviceRadius_ != null
&& serviceRadius_
!= com.google.shopping.merchant.accounts.v1beta.Distance.getDefaultInstance()) {
getServiceRadiusBuilder().mergeFrom(value);
} else {
serviceRadius_ = value;
}
} else {
serviceRadiusBuilder_.mergeFrom(value);
}
if (serviceRadius_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*/
public Builder clearServiceRadius() {
bitField0_ = (bitField0_ & ~0x00000008);
serviceRadius_ = null;
if (serviceRadiusBuilder_ != null) {
serviceRadiusBuilder_.dispose();
serviceRadiusBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*/
public com.google.shopping.merchant.accounts.v1beta.Distance.Builder
getServiceRadiusBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getServiceRadiusFieldBuilder().getBuilder();
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*/
public com.google.shopping.merchant.accounts.v1beta.DistanceOrBuilder
getServiceRadiusOrBuilder() {
if (serviceRadiusBuilder_ != null) {
return serviceRadiusBuilder_.getMessageOrBuilder();
} else {
return serviceRadius_ == null
? com.google.shopping.merchant.accounts.v1beta.Distance.getDefaultInstance()
: serviceRadius_;
}
}
/**
*
*
*
* Maximum delivery radius.
* This is only required for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Distance service_radius = 4;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Distance,
com.google.shopping.merchant.accounts.v1beta.Distance.Builder,
com.google.shopping.merchant.accounts.v1beta.DistanceOrBuilder>
getServiceRadiusFieldBuilder() {
if (serviceRadiusBuilder_ == null) {
serviceRadiusBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Distance,
com.google.shopping.merchant.accounts.v1beta.Distance.Builder,
com.google.shopping.merchant.accounts.v1beta.DistanceOrBuilder>(
getServiceRadius(), getParentForChildren(), isClean());
serviceRadius_ = null;
}
return serviceRadiusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.shopping.merchant.accounts.v1beta.Service.StoreConfig)
}
// @@protoc_insertion_point(class_scope:google.shopping.merchant.accounts.v1beta.Service.StoreConfig)
private static final com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig();
}
public static com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StoreConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LoyaltyProgramOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return Whether the programLabel field is set.
*/
boolean hasProgramLabel();
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return The programLabel.
*/
java.lang.String getProgramLabel();
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return The bytes for programLabel.
*/
com.google.protobuf.ByteString getProgramLabelBytes();
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers>
getLoyaltyProgramTiersList();
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
getLoyaltyProgramTiers(int index);
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getLoyaltyProgramTiersCount();
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List<
? extends
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder>
getLoyaltyProgramTiersOrBuilderList();
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiersOrBuilder
getLoyaltyProgramTiersOrBuilder(int index);
}
/**
*
*
*
* [Loyalty program](https://support.google.com/merchants/answer/12922446)
* provided by a merchant.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram}
*/
public static final class LoyaltyProgram extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram)
LoyaltyProgramOrBuilder {
private static final long serialVersionUID = 0L;
// Use LoyaltyProgram.newBuilder() to construct.
private LoyaltyProgram(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LoyaltyProgram() {
programLabel_ = "";
loyaltyProgramTiers_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new LoyaltyProgram();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.class,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder.class);
}
public interface LoyaltyProgramTiersOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return Whether the tierLabel field is set.
*/
boolean hasTierLabel();
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return The tierLabel.
*/
java.lang.String getTierLabel();
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return The bytes for tierLabel.
*/
com.google.protobuf.ByteString getTierLabelBytes();
}
/**
*
*
*
* Subset of a merchants loyalty program.
*
*
* Protobuf type {@code
* google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers}
*/
public static final class LoyaltyProgramTiers extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers)
LoyaltyProgramTiersOrBuilder {
private static final long serialVersionUID = 0L;
// Use LoyaltyProgramTiers.newBuilder() to construct.
private LoyaltyProgramTiers(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LoyaltyProgramTiers() {
tierLabel_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new LoyaltyProgramTiers();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_LoyaltyProgramTiers_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_LoyaltyProgramTiers_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.class,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.Builder.class);
}
private int bitField0_;
public static final int TIER_LABEL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object tierLabel_ = "";
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return Whether the tierLabel field is set.
*/
@java.lang.Override
public boolean hasTierLabel() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return The tierLabel.
*/
@java.lang.Override
public java.lang.String getTierLabel() {
java.lang.Object ref = tierLabel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tierLabel_ = s;
return s;
}
}
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return The bytes for tierLabel.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTierLabelBytes() {
java.lang.Object ref = tierLabel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
tierLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, tierLabel_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, tierLabel_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj
instanceof
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers)) {
return super.equals(obj);
}
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
other =
(com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers)
obj;
if (hasTierLabel() != other.hasTierLabel()) return false;
if (hasTierLabel()) {
if (!getTierLabel().equals(other.getTierLabel())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTierLabel()) {
hash = (37 * hash) + TIER_LABEL_FIELD_NUMBER;
hash = (53 * hash) + getTierLabel().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Subset of a merchants loyalty program.
*
*
* Protobuf type {@code
* google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers)
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_LoyaltyProgramTiers_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_LoyaltyProgramTiers_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.class,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.Builder.class);
}
// Construct using
// com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
tierLabel_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_LoyaltyProgramTiers_descriptor;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
getDefaultInstanceForType() {
return com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.getDefaultInstance();
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
build() {
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
buildPartial() {
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
result =
new com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.tierLabel_ = tierLabel_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other
instanceof
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers) {
return mergeFrom(
(com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers)
other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
other) {
if (other
== com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.getDefaultInstance()) return this;
if (other.hasTierLabel()) {
tierLabel_ = other.tierLabel_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
tierLabel_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object tierLabel_ = "";
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return Whether the tierLabel field is set.
*/
public boolean hasTierLabel() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return The tierLabel.
*/
public java.lang.String getTierLabel() {
java.lang.Object ref = tierLabel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tierLabel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return The bytes for tierLabel.
*/
public com.google.protobuf.ByteString getTierLabelBytes() {
java.lang.Object ref = tierLabel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
tierLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @param value The tierLabel to set.
* @return This builder for chaining.
*/
public Builder setTierLabel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tierLabel_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @return This builder for chaining.
*/
public Builder clearTierLabel() {
tierLabel_ = getDefaultInstance().getTierLabel();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The tier label [tier_label] sub-attribute differentiates offer level
* benefits between each tier. This value is also set in your program
* settings in Merchant Center, and is required for data source changes
* even if your loyalty program only has 1 tier.
*
*
* optional string tier_label = 1;
*
* @param value The bytes for tierLabel to set.
* @return This builder for chaining.
*/
public Builder setTierLabelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tierLabel_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers)
}
// @@protoc_insertion_point(class_scope:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers)
private static final com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE =
new com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers();
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LoyaltyProgramTiers parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int PROGRAM_LABEL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object programLabel_ = "";
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return Whether the programLabel field is set.
*/
@java.lang.Override
public boolean hasProgramLabel() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return The programLabel.
*/
@java.lang.Override
public java.lang.String getProgramLabel() {
java.lang.Object ref = programLabel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
programLabel_ = s;
return s;
}
}
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return The bytes for programLabel.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProgramLabelBytes() {
java.lang.Object ref = programLabel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
programLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOYALTY_PROGRAM_TIERS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers>
loyaltyProgramTiers_;
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers>
getLoyaltyProgramTiersList() {
return loyaltyProgramTiers_;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List<
? extends
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder>
getLoyaltyProgramTiersOrBuilderList() {
return loyaltyProgramTiers_;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public int getLoyaltyProgramTiersCount() {
return loyaltyProgramTiers_.size();
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
getLoyaltyProgramTiers(int index) {
return loyaltyProgramTiers_.get(index);
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder
getLoyaltyProgramTiersOrBuilder(int index) {
return loyaltyProgramTiers_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, programLabel_);
}
for (int i = 0; i < loyaltyProgramTiers_.size(); i++) {
output.writeMessage(2, loyaltyProgramTiers_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, programLabel_);
}
for (int i = 0; i < loyaltyProgramTiers_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
2, loyaltyProgramTiers_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram)) {
return super.equals(obj);
}
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram other =
(com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram) obj;
if (hasProgramLabel() != other.hasProgramLabel()) return false;
if (hasProgramLabel()) {
if (!getProgramLabel().equals(other.getProgramLabel())) return false;
}
if (!getLoyaltyProgramTiersList().equals(other.getLoyaltyProgramTiersList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasProgramLabel()) {
hash = (37 * hash) + PROGRAM_LABEL_FIELD_NUMBER;
hash = (53 * hash) + getProgramLabel().hashCode();
}
if (getLoyaltyProgramTiersCount() > 0) {
hash = (37 * hash) + LOYALTY_PROGRAM_TIERS_FIELD_NUMBER;
hash = (53 * hash) + getLoyaltyProgramTiersList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* [Loyalty program](https://support.google.com/merchants/answer/12922446)
* provided by a merchant.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram)
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.class,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder.class);
}
// Construct using
// com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
programLabel_ = "";
if (loyaltyProgramTiersBuilder_ == null) {
loyaltyProgramTiers_ = java.util.Collections.emptyList();
} else {
loyaltyProgramTiers_ = null;
loyaltyProgramTiersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_LoyaltyProgram_descriptor;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
getDefaultInstanceForType() {
return com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.getDefaultInstance();
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram build() {
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram buildPartial() {
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram result =
new com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram result) {
if (loyaltyProgramTiersBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
loyaltyProgramTiers_ = java.util.Collections.unmodifiableList(loyaltyProgramTiers_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.loyaltyProgramTiers_ = loyaltyProgramTiers_;
} else {
result.loyaltyProgramTiers_ = loyaltyProgramTiersBuilder_.build();
}
}
private void buildPartial0(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.programLabel_ = programLabel_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram) {
return mergeFrom(
(com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram other) {
if (other
== com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.getDefaultInstance()) return this;
if (other.hasProgramLabel()) {
programLabel_ = other.programLabel_;
bitField0_ |= 0x00000001;
onChanged();
}
if (loyaltyProgramTiersBuilder_ == null) {
if (!other.loyaltyProgramTiers_.isEmpty()) {
if (loyaltyProgramTiers_.isEmpty()) {
loyaltyProgramTiers_ = other.loyaltyProgramTiers_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.addAll(other.loyaltyProgramTiers_);
}
onChanged();
}
} else {
if (!other.loyaltyProgramTiers_.isEmpty()) {
if (loyaltyProgramTiersBuilder_.isEmpty()) {
loyaltyProgramTiersBuilder_.dispose();
loyaltyProgramTiersBuilder_ = null;
loyaltyProgramTiers_ = other.loyaltyProgramTiers_;
bitField0_ = (bitField0_ & ~0x00000002);
loyaltyProgramTiersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getLoyaltyProgramTiersFieldBuilder()
: null;
} else {
loyaltyProgramTiersBuilder_.addAllMessages(other.loyaltyProgramTiers_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
programLabel_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers
m =
input.readMessage(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.parser(),
extensionRegistry);
if (loyaltyProgramTiersBuilder_ == null) {
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.add(m);
} else {
loyaltyProgramTiersBuilder_.addMessage(m);
}
break;
} // case 18
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object programLabel_ = "";
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return Whether the programLabel field is set.
*/
public boolean hasProgramLabel() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return The programLabel.
*/
public java.lang.String getProgramLabel() {
java.lang.Object ref = programLabel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
programLabel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return The bytes for programLabel.
*/
public com.google.protobuf.ByteString getProgramLabelBytes() {
java.lang.Object ref = programLabel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
programLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @param value The programLabel to set.
* @return This builder for chaining.
*/
public Builder setProgramLabel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
programLabel_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @return This builder for chaining.
*/
public Builder clearProgramLabel() {
programLabel_ = getDefaultInstance().getProgramLabel();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* This is the loyalty program label set in your loyalty program settings in
* Merchant Center. This sub-attribute allows Google to map your loyalty
* program to eligible offers.
*
*
* optional string program_label = 1;
*
* @param value The bytes for programLabel to set.
* @return This builder for chaining.
*/
public Builder setProgramLabelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
programLabel_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.util.List<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers>
loyaltyProgramTiers_ = java.util.Collections.emptyList();
private void ensureLoyaltyProgramTiersIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
loyaltyProgramTiers_ =
new java.util.ArrayList<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers>(loyaltyProgramTiers_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder>
loyaltyProgramTiersBuilder_;
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers>
getLoyaltyProgramTiersList() {
if (loyaltyProgramTiersBuilder_ == null) {
return java.util.Collections.unmodifiableList(loyaltyProgramTiers_);
} else {
return loyaltyProgramTiersBuilder_.getMessageList();
}
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public int getLoyaltyProgramTiersCount() {
if (loyaltyProgramTiersBuilder_ == null) {
return loyaltyProgramTiers_.size();
} else {
return loyaltyProgramTiersBuilder_.getCount();
}
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
getLoyaltyProgramTiers(int index) {
if (loyaltyProgramTiersBuilder_ == null) {
return loyaltyProgramTiers_.get(index);
} else {
return loyaltyProgramTiersBuilder_.getMessage(index);
}
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setLoyaltyProgramTiers(
int index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
value) {
if (loyaltyProgramTiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.set(index, value);
onChanged();
} else {
loyaltyProgramTiersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setLoyaltyProgramTiers(
int index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
.Builder
builderForValue) {
if (loyaltyProgramTiersBuilder_ == null) {
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.set(index, builderForValue.build());
onChanged();
} else {
loyaltyProgramTiersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyProgramTiers(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
value) {
if (loyaltyProgramTiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.add(value);
onChanged();
} else {
loyaltyProgramTiersBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyProgramTiers(
int index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
value) {
if (loyaltyProgramTiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.add(index, value);
onChanged();
} else {
loyaltyProgramTiersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyProgramTiers(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
.Builder
builderForValue) {
if (loyaltyProgramTiersBuilder_ == null) {
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.add(builderForValue.build());
onChanged();
} else {
loyaltyProgramTiersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyProgramTiers(
int index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
.Builder
builderForValue) {
if (loyaltyProgramTiersBuilder_ == null) {
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.add(index, builderForValue.build());
onChanged();
} else {
loyaltyProgramTiersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAllLoyaltyProgramTiers(
java.lang.Iterable<
? extends
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers>
values) {
if (loyaltyProgramTiersBuilder_ == null) {
ensureLoyaltyProgramTiersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, loyaltyProgramTiers_);
onChanged();
} else {
loyaltyProgramTiersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearLoyaltyProgramTiers() {
if (loyaltyProgramTiersBuilder_ == null) {
loyaltyProgramTiers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
loyaltyProgramTiersBuilder_.clear();
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder removeLoyaltyProgramTiers(int index) {
if (loyaltyProgramTiersBuilder_ == null) {
ensureLoyaltyProgramTiersIsMutable();
loyaltyProgramTiers_.remove(index);
onChanged();
} else {
loyaltyProgramTiersBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
.Builder
getLoyaltyProgramTiersBuilder(int index) {
return getLoyaltyProgramTiersFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder
getLoyaltyProgramTiersOrBuilder(int index) {
if (loyaltyProgramTiersBuilder_ == null) {
return loyaltyProgramTiers_.get(index);
} else {
return loyaltyProgramTiersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List<
? extends
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder>
getLoyaltyProgramTiersOrBuilderList() {
if (loyaltyProgramTiersBuilder_ != null) {
return loyaltyProgramTiersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(loyaltyProgramTiers_);
}
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
.Builder
addLoyaltyProgramTiersBuilder() {
return getLoyaltyProgramTiersFieldBuilder()
.addBuilder(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.getDefaultInstance());
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers
.Builder
addLoyaltyProgramTiersBuilder(int index) {
return getLoyaltyProgramTiersFieldBuilder()
.addBuilder(
index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.getDefaultInstance());
}
/**
*
*
*
* Optional. Loyalty program tier of this shipping service.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.LoyaltyProgramTiers loyalty_program_tiers = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.Builder>
getLoyaltyProgramTiersBuilderList() {
return getLoyaltyProgramTiersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder>
getLoyaltyProgramTiersFieldBuilder() {
if (loyaltyProgramTiersBuilder_ == null) {
loyaltyProgramTiersBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiers.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.LoyaltyProgramTiersOrBuilder>(
loyaltyProgramTiers_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
loyaltyProgramTiers_ = null;
}
return loyaltyProgramTiersBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram)
}
// @@protoc_insertion_point(class_scope:google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram)
private static final com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram();
}
public static com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LoyaltyProgram parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int SERVICE_NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object serviceName_ = "";
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the serviceName field is set.
*/
@java.lang.Override
public boolean hasServiceName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The serviceName.
*/
@java.lang.Override
public java.lang.String getServiceName() {
java.lang.Object ref = serviceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serviceName_ = s;
return s;
}
}
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for serviceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getServiceNameBytes() {
java.lang.Object ref = serviceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
serviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACTIVE_FIELD_NUMBER = 2;
private boolean active_ = false;
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the active field is set.
*/
@java.lang.Override
public boolean hasActive() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The active.
*/
@java.lang.Override
public boolean getActive() {
return active_;
}
public static final int DELIVERY_COUNTRIES_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList deliveryCountries_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return A list containing the deliveryCountries.
*/
public com.google.protobuf.ProtocolStringList getDeliveryCountriesList() {
return deliveryCountries_;
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return The count of deliveryCountries.
*/
public int getDeliveryCountriesCount() {
return deliveryCountries_.size();
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @param index The index of the element to return.
* @return The deliveryCountries at the given index.
*/
public java.lang.String getDeliveryCountries(int index) {
return deliveryCountries_.get(index);
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @param index The index of the value to return.
* @return The bytes of the deliveryCountries at the given index.
*/
public com.google.protobuf.ByteString getDeliveryCountriesBytes(int index) {
return deliveryCountries_.getByteString(index);
}
public static final int CURRENCY_CODE_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object currencyCode_ = "";
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return Whether the currencyCode field is set.
*/
@java.lang.Override
public boolean hasCurrencyCode() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return The currencyCode.
*/
@java.lang.Override
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
}
}
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return The bytes for currencyCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DELIVERY_TIME_FIELD_NUMBER = 5;
private com.google.shopping.merchant.accounts.v1beta.DeliveryTime deliveryTime_;
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the deliveryTime field is set.
*/
@java.lang.Override
public boolean hasDeliveryTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The deliveryTime.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.DeliveryTime getDeliveryTime() {
return deliveryTime_ == null
? com.google.shopping.merchant.accounts.v1beta.DeliveryTime.getDefaultInstance()
: deliveryTime_;
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.DeliveryTimeOrBuilder
getDeliveryTimeOrBuilder() {
return deliveryTime_ == null
? com.google.shopping.merchant.accounts.v1beta.DeliveryTime.getDefaultInstance()
: deliveryTime_;
}
public static final int RATE_GROUPS_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List rateGroups_;
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List
getRateGroupsList() {
return rateGroups_;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List extends com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder>
getRateGroupsOrBuilderList() {
return rateGroups_;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public int getRateGroupsCount() {
return rateGroups_.size();
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.RateGroup getRateGroups(int index) {
return rateGroups_.get(index);
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder getRateGroupsOrBuilder(
int index) {
return rateGroups_.get(index);
}
public static final int SHIPMENT_TYPE_FIELD_NUMBER = 7;
private int shipmentType_ = 0;
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return Whether the shipmentType field is set.
*/
@java.lang.Override
public boolean hasShipmentType() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return The enum numeric value on the wire for shipmentType.
*/
@java.lang.Override
public int getShipmentTypeValue() {
return shipmentType_;
}
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return The shipmentType.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType getShipmentType() {
com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType result =
com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType.forNumber(shipmentType_);
return result == null
? com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType.UNRECOGNIZED
: result;
}
public static final int MINIMUM_ORDER_VALUE_FIELD_NUMBER = 8;
private com.google.shopping.type.Price minimumOrderValue_;
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*
* @return Whether the minimumOrderValue field is set.
*/
@java.lang.Override
public boolean hasMinimumOrderValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*
* @return The minimumOrderValue.
*/
@java.lang.Override
public com.google.shopping.type.Price getMinimumOrderValue() {
return minimumOrderValue_ == null
? com.google.shopping.type.Price.getDefaultInstance()
: minimumOrderValue_;
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
@java.lang.Override
public com.google.shopping.type.PriceOrBuilder getMinimumOrderValueOrBuilder() {
return minimumOrderValue_ == null
? com.google.shopping.type.Price.getDefaultInstance()
: minimumOrderValue_;
}
public static final int MINIMUM_ORDER_VALUE_TABLE_FIELD_NUMBER = 9;
private com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable
minimumOrderValueTable_;
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*
* @return Whether the minimumOrderValueTable field is set.
*/
@java.lang.Override
public boolean hasMinimumOrderValueTable() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*
* @return The minimumOrderValueTable.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable
getMinimumOrderValueTable() {
return minimumOrderValueTable_ == null
? com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable.getDefaultInstance()
: minimumOrderValueTable_;
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTableOrBuilder
getMinimumOrderValueTableOrBuilder() {
return minimumOrderValueTable_ == null
? com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable.getDefaultInstance()
: minimumOrderValueTable_;
}
public static final int STORE_CONFIG_FIELD_NUMBER = 10;
private com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig storeConfig_;
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*
* @return Whether the storeConfig field is set.
*/
@java.lang.Override
public boolean hasStoreConfig() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*
* @return The storeConfig.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig getStoreConfig() {
return storeConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.getDefaultInstance()
: storeConfig_;
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfigOrBuilder
getStoreConfigOrBuilder() {
return storeConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.getDefaultInstance()
: storeConfig_;
}
public static final int LOYALTY_PROGRAMS_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private java.util.List
loyaltyPrograms_;
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List
getLoyaltyProgramsList() {
return loyaltyPrograms_;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List<
? extends com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder>
getLoyaltyProgramsOrBuilderList() {
return loyaltyPrograms_;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public int getLoyaltyProgramsCount() {
return loyaltyPrograms_.size();
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram getLoyaltyPrograms(
int index) {
return loyaltyPrograms_.get(index);
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder
getLoyaltyProgramsOrBuilder(int index) {
return loyaltyPrograms_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, serviceName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(2, active_);
}
for (int i = 0; i < deliveryCountries_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, deliveryCountries_.getRaw(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, currencyCode_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(5, getDeliveryTime());
}
for (int i = 0; i < rateGroups_.size(); i++) {
output.writeMessage(6, rateGroups_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeEnum(7, shipmentType_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(8, getMinimumOrderValue());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(9, getMinimumOrderValueTable());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(10, getStoreConfig());
}
for (int i = 0; i < loyaltyPrograms_.size(); i++) {
output.writeMessage(11, loyaltyPrograms_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, serviceName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(2, active_);
}
{
int dataSize = 0;
for (int i = 0; i < deliveryCountries_.size(); i++) {
dataSize += computeStringSizeNoTag(deliveryCountries_.getRaw(i));
}
size += dataSize;
size += 1 * getDeliveryCountriesList().size();
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, currencyCode_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(5, getDeliveryTime());
}
for (int i = 0; i < rateGroups_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, rateGroups_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(7, shipmentType_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(8, getMinimumOrderValue());
}
if (((bitField0_ & 0x00000040) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(9, getMinimumOrderValueTable());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(10, getStoreConfig());
}
for (int i = 0; i < loyaltyPrograms_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(11, loyaltyPrograms_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.shopping.merchant.accounts.v1beta.Service)) {
return super.equals(obj);
}
com.google.shopping.merchant.accounts.v1beta.Service other =
(com.google.shopping.merchant.accounts.v1beta.Service) obj;
if (hasServiceName() != other.hasServiceName()) return false;
if (hasServiceName()) {
if (!getServiceName().equals(other.getServiceName())) return false;
}
if (hasActive() != other.hasActive()) return false;
if (hasActive()) {
if (getActive() != other.getActive()) return false;
}
if (!getDeliveryCountriesList().equals(other.getDeliveryCountriesList())) return false;
if (hasCurrencyCode() != other.hasCurrencyCode()) return false;
if (hasCurrencyCode()) {
if (!getCurrencyCode().equals(other.getCurrencyCode())) return false;
}
if (hasDeliveryTime() != other.hasDeliveryTime()) return false;
if (hasDeliveryTime()) {
if (!getDeliveryTime().equals(other.getDeliveryTime())) return false;
}
if (!getRateGroupsList().equals(other.getRateGroupsList())) return false;
if (hasShipmentType() != other.hasShipmentType()) return false;
if (hasShipmentType()) {
if (shipmentType_ != other.shipmentType_) return false;
}
if (hasMinimumOrderValue() != other.hasMinimumOrderValue()) return false;
if (hasMinimumOrderValue()) {
if (!getMinimumOrderValue().equals(other.getMinimumOrderValue())) return false;
}
if (hasMinimumOrderValueTable() != other.hasMinimumOrderValueTable()) return false;
if (hasMinimumOrderValueTable()) {
if (!getMinimumOrderValueTable().equals(other.getMinimumOrderValueTable())) return false;
}
if (hasStoreConfig() != other.hasStoreConfig()) return false;
if (hasStoreConfig()) {
if (!getStoreConfig().equals(other.getStoreConfig())) return false;
}
if (!getLoyaltyProgramsList().equals(other.getLoyaltyProgramsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasServiceName()) {
hash = (37 * hash) + SERVICE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getServiceName().hashCode();
}
if (hasActive()) {
hash = (37 * hash) + ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getActive());
}
if (getDeliveryCountriesCount() > 0) {
hash = (37 * hash) + DELIVERY_COUNTRIES_FIELD_NUMBER;
hash = (53 * hash) + getDeliveryCountriesList().hashCode();
}
if (hasCurrencyCode()) {
hash = (37 * hash) + CURRENCY_CODE_FIELD_NUMBER;
hash = (53 * hash) + getCurrencyCode().hashCode();
}
if (hasDeliveryTime()) {
hash = (37 * hash) + DELIVERY_TIME_FIELD_NUMBER;
hash = (53 * hash) + getDeliveryTime().hashCode();
}
if (getRateGroupsCount() > 0) {
hash = (37 * hash) + RATE_GROUPS_FIELD_NUMBER;
hash = (53 * hash) + getRateGroupsList().hashCode();
}
if (hasShipmentType()) {
hash = (37 * hash) + SHIPMENT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + shipmentType_;
}
if (hasMinimumOrderValue()) {
hash = (37 * hash) + MINIMUM_ORDER_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getMinimumOrderValue().hashCode();
}
if (hasMinimumOrderValueTable()) {
hash = (37 * hash) + MINIMUM_ORDER_VALUE_TABLE_FIELD_NUMBER;
hash = (53 * hash) + getMinimumOrderValueTable().hashCode();
}
if (hasStoreConfig()) {
hash = (37 * hash) + STORE_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getStoreConfig().hashCode();
}
if (getLoyaltyProgramsCount() > 0) {
hash = (37 * hash) + LOYALTY_PROGRAMS_FIELD_NUMBER;
hash = (53 * hash) + getLoyaltyProgramsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.shopping.merchant.accounts.v1beta.Service parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.shopping.merchant.accounts.v1beta.Service prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Shipping service.
*
*
* Protobuf type {@code google.shopping.merchant.accounts.v1beta.Service}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.shopping.merchant.accounts.v1beta.Service)
com.google.shopping.merchant.accounts.v1beta.ServiceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.shopping.merchant.accounts.v1beta.Service.class,
com.google.shopping.merchant.accounts.v1beta.Service.Builder.class);
}
// Construct using com.google.shopping.merchant.accounts.v1beta.Service.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getDeliveryTimeFieldBuilder();
getRateGroupsFieldBuilder();
getMinimumOrderValueFieldBuilder();
getMinimumOrderValueTableFieldBuilder();
getStoreConfigFieldBuilder();
getLoyaltyProgramsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
serviceName_ = "";
active_ = false;
deliveryCountries_ = com.google.protobuf.LazyStringArrayList.emptyList();
currencyCode_ = "";
deliveryTime_ = null;
if (deliveryTimeBuilder_ != null) {
deliveryTimeBuilder_.dispose();
deliveryTimeBuilder_ = null;
}
if (rateGroupsBuilder_ == null) {
rateGroups_ = java.util.Collections.emptyList();
} else {
rateGroups_ = null;
rateGroupsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
shipmentType_ = 0;
minimumOrderValue_ = null;
if (minimumOrderValueBuilder_ != null) {
minimumOrderValueBuilder_.dispose();
minimumOrderValueBuilder_ = null;
}
minimumOrderValueTable_ = null;
if (minimumOrderValueTableBuilder_ != null) {
minimumOrderValueTableBuilder_.dispose();
minimumOrderValueTableBuilder_ = null;
}
storeConfig_ = null;
if (storeConfigBuilder_ != null) {
storeConfigBuilder_.dispose();
storeConfigBuilder_ = null;
}
if (loyaltyProgramsBuilder_ == null) {
loyaltyPrograms_ = java.util.Collections.emptyList();
} else {
loyaltyPrograms_ = null;
loyaltyProgramsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.shopping.merchant.accounts.v1beta.ShippingSettingsProto
.internal_static_google_shopping_merchant_accounts_v1beta_Service_descriptor;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service getDefaultInstanceForType() {
return com.google.shopping.merchant.accounts.v1beta.Service.getDefaultInstance();
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service build() {
com.google.shopping.merchant.accounts.v1beta.Service result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service buildPartial() {
com.google.shopping.merchant.accounts.v1beta.Service result =
new com.google.shopping.merchant.accounts.v1beta.Service(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.shopping.merchant.accounts.v1beta.Service result) {
if (rateGroupsBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
rateGroups_ = java.util.Collections.unmodifiableList(rateGroups_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.rateGroups_ = rateGroups_;
} else {
result.rateGroups_ = rateGroupsBuilder_.build();
}
if (loyaltyProgramsBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)) {
loyaltyPrograms_ = java.util.Collections.unmodifiableList(loyaltyPrograms_);
bitField0_ = (bitField0_ & ~0x00000400);
}
result.loyaltyPrograms_ = loyaltyPrograms_;
} else {
result.loyaltyPrograms_ = loyaltyProgramsBuilder_.build();
}
}
private void buildPartial0(com.google.shopping.merchant.accounts.v1beta.Service result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.serviceName_ = serviceName_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.active_ = active_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
deliveryCountries_.makeImmutable();
result.deliveryCountries_ = deliveryCountries_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.currencyCode_ = currencyCode_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.deliveryTime_ =
deliveryTimeBuilder_ == null ? deliveryTime_ : deliveryTimeBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.shipmentType_ = shipmentType_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.minimumOrderValue_ =
minimumOrderValueBuilder_ == null
? minimumOrderValue_
: minimumOrderValueBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.minimumOrderValueTable_ =
minimumOrderValueTableBuilder_ == null
? minimumOrderValueTable_
: minimumOrderValueTableBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.storeConfig_ =
storeConfigBuilder_ == null ? storeConfig_ : storeConfigBuilder_.build();
to_bitField0_ |= 0x00000080;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.shopping.merchant.accounts.v1beta.Service) {
return mergeFrom((com.google.shopping.merchant.accounts.v1beta.Service) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.shopping.merchant.accounts.v1beta.Service other) {
if (other == com.google.shopping.merchant.accounts.v1beta.Service.getDefaultInstance())
return this;
if (other.hasServiceName()) {
serviceName_ = other.serviceName_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasActive()) {
setActive(other.getActive());
}
if (!other.deliveryCountries_.isEmpty()) {
if (deliveryCountries_.isEmpty()) {
deliveryCountries_ = other.deliveryCountries_;
bitField0_ |= 0x00000004;
} else {
ensureDeliveryCountriesIsMutable();
deliveryCountries_.addAll(other.deliveryCountries_);
}
onChanged();
}
if (other.hasCurrencyCode()) {
currencyCode_ = other.currencyCode_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasDeliveryTime()) {
mergeDeliveryTime(other.getDeliveryTime());
}
if (rateGroupsBuilder_ == null) {
if (!other.rateGroups_.isEmpty()) {
if (rateGroups_.isEmpty()) {
rateGroups_ = other.rateGroups_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureRateGroupsIsMutable();
rateGroups_.addAll(other.rateGroups_);
}
onChanged();
}
} else {
if (!other.rateGroups_.isEmpty()) {
if (rateGroupsBuilder_.isEmpty()) {
rateGroupsBuilder_.dispose();
rateGroupsBuilder_ = null;
rateGroups_ = other.rateGroups_;
bitField0_ = (bitField0_ & ~0x00000020);
rateGroupsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getRateGroupsFieldBuilder()
: null;
} else {
rateGroupsBuilder_.addAllMessages(other.rateGroups_);
}
}
}
if (other.hasShipmentType()) {
setShipmentType(other.getShipmentType());
}
if (other.hasMinimumOrderValue()) {
mergeMinimumOrderValue(other.getMinimumOrderValue());
}
if (other.hasMinimumOrderValueTable()) {
mergeMinimumOrderValueTable(other.getMinimumOrderValueTable());
}
if (other.hasStoreConfig()) {
mergeStoreConfig(other.getStoreConfig());
}
if (loyaltyProgramsBuilder_ == null) {
if (!other.loyaltyPrograms_.isEmpty()) {
if (loyaltyPrograms_.isEmpty()) {
loyaltyPrograms_ = other.loyaltyPrograms_;
bitField0_ = (bitField0_ & ~0x00000400);
} else {
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.addAll(other.loyaltyPrograms_);
}
onChanged();
}
} else {
if (!other.loyaltyPrograms_.isEmpty()) {
if (loyaltyProgramsBuilder_.isEmpty()) {
loyaltyProgramsBuilder_.dispose();
loyaltyProgramsBuilder_ = null;
loyaltyPrograms_ = other.loyaltyPrograms_;
bitField0_ = (bitField0_ & ~0x00000400);
loyaltyProgramsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getLoyaltyProgramsFieldBuilder()
: null;
} else {
loyaltyProgramsBuilder_.addAllMessages(other.loyaltyPrograms_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
serviceName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16:
{
active_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26:
{
java.lang.String s = input.readStringRequireUtf8();
ensureDeliveryCountriesIsMutable();
deliveryCountries_.add(s);
break;
} // case 26
case 34:
{
currencyCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42:
{
input.readMessage(getDeliveryTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
case 50:
{
com.google.shopping.merchant.accounts.v1beta.RateGroup m =
input.readMessage(
com.google.shopping.merchant.accounts.v1beta.RateGroup.parser(),
extensionRegistry);
if (rateGroupsBuilder_ == null) {
ensureRateGroupsIsMutable();
rateGroups_.add(m);
} else {
rateGroupsBuilder_.addMessage(m);
}
break;
} // case 50
case 56:
{
shipmentType_ = input.readEnum();
bitField0_ |= 0x00000040;
break;
} // case 56
case 66:
{
input.readMessage(
getMinimumOrderValueFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 74:
{
input.readMessage(
getMinimumOrderValueTableFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 74
case 82:
{
input.readMessage(getStoreConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 82
case 90:
{
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram m =
input.readMessage(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.parser(),
extensionRegistry);
if (loyaltyProgramsBuilder_ == null) {
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.add(m);
} else {
loyaltyProgramsBuilder_.addMessage(m);
}
break;
} // case 90
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object serviceName_ = "";
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the serviceName field is set.
*/
public boolean hasServiceName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The serviceName.
*/
public java.lang.String getServiceName() {
java.lang.Object ref = serviceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serviceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for serviceName.
*/
public com.google.protobuf.ByteString getServiceNameBytes() {
java.lang.Object ref = serviceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
serviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The serviceName to set.
* @return This builder for chaining.
*/
public Builder setServiceName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
serviceName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return This builder for chaining.
*/
public Builder clearServiceName() {
serviceName_ = getDefaultInstance().getServiceName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The bytes for serviceName to set.
* @return This builder for chaining.
*/
public Builder setServiceNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
serviceName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private boolean active_;
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the active field is set.
*/
@java.lang.Override
public boolean hasActive() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The active.
*/
@java.lang.Override
public boolean getActive() {
return active_;
}
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The active to set.
* @return This builder for chaining.
*/
public Builder setActive(boolean value) {
active_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return This builder for chaining.
*/
public Builder clearActive() {
bitField0_ = (bitField0_ & ~0x00000002);
active_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList deliveryCountries_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureDeliveryCountriesIsMutable() {
if (!deliveryCountries_.isModifiable()) {
deliveryCountries_ = new com.google.protobuf.LazyStringArrayList(deliveryCountries_);
}
bitField0_ |= 0x00000004;
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return A list containing the deliveryCountries.
*/
public com.google.protobuf.ProtocolStringList getDeliveryCountriesList() {
deliveryCountries_.makeImmutable();
return deliveryCountries_;
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The count of deliveryCountries.
*/
public int getDeliveryCountriesCount() {
return deliveryCountries_.size();
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param index The index of the element to return.
* @return The deliveryCountries at the given index.
*/
public java.lang.String getDeliveryCountries(int index) {
return deliveryCountries_.get(index);
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param index The index of the value to return.
* @return The bytes of the deliveryCountries at the given index.
*/
public com.google.protobuf.ByteString getDeliveryCountriesBytes(int index) {
return deliveryCountries_.getByteString(index);
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param index The index to set the value at.
* @param value The deliveryCountries to set.
* @return This builder for chaining.
*/
public Builder setDeliveryCountries(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeliveryCountriesIsMutable();
deliveryCountries_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param value The deliveryCountries to add.
* @return This builder for chaining.
*/
public Builder addDeliveryCountries(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeliveryCountriesIsMutable();
deliveryCountries_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param values The deliveryCountries to add.
* @return This builder for chaining.
*/
public Builder addAllDeliveryCountries(java.lang.Iterable values) {
ensureDeliveryCountriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, deliveryCountries_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return This builder for chaining.
*/
public Builder clearDeliveryCountries() {
deliveryCountries_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
;
onChanged();
return this;
}
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param value The bytes of the deliveryCountries to add.
* @return This builder for chaining.
*/
public Builder addDeliveryCountriesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureDeliveryCountriesIsMutable();
deliveryCountries_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object currencyCode_ = "";
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return Whether the currencyCode field is set.
*/
public boolean hasCurrencyCode() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return The currencyCode.
*/
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return The bytes for currencyCode.
*/
public com.google.protobuf.ByteString getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @param value The currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCode(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
currencyCode_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return This builder for chaining.
*/
public Builder clearCurrencyCode() {
currencyCode_ = getDefaultInstance().getCurrencyCode();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @param value The bytes for currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCodeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
currencyCode_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private com.google.shopping.merchant.accounts.v1beta.DeliveryTime deliveryTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.DeliveryTime,
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.Builder,
com.google.shopping.merchant.accounts.v1beta.DeliveryTimeOrBuilder>
deliveryTimeBuilder_;
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the deliveryTime field is set.
*/
public boolean hasDeliveryTime() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The deliveryTime.
*/
public com.google.shopping.merchant.accounts.v1beta.DeliveryTime getDeliveryTime() {
if (deliveryTimeBuilder_ == null) {
return deliveryTime_ == null
? com.google.shopping.merchant.accounts.v1beta.DeliveryTime.getDefaultInstance()
: deliveryTime_;
} else {
return deliveryTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setDeliveryTime(
com.google.shopping.merchant.accounts.v1beta.DeliveryTime value) {
if (deliveryTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deliveryTime_ = value;
} else {
deliveryTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setDeliveryTime(
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.Builder builderForValue) {
if (deliveryTimeBuilder_ == null) {
deliveryTime_ = builderForValue.build();
} else {
deliveryTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder mergeDeliveryTime(
com.google.shopping.merchant.accounts.v1beta.DeliveryTime value) {
if (deliveryTimeBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)
&& deliveryTime_ != null
&& deliveryTime_
!= com.google.shopping.merchant.accounts.v1beta.DeliveryTime.getDefaultInstance()) {
getDeliveryTimeBuilder().mergeFrom(value);
} else {
deliveryTime_ = value;
}
} else {
deliveryTimeBuilder_.mergeFrom(value);
}
if (deliveryTime_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder clearDeliveryTime() {
bitField0_ = (bitField0_ & ~0x00000010);
deliveryTime_ = null;
if (deliveryTimeBuilder_ != null) {
deliveryTimeBuilder_.dispose();
deliveryTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.shopping.merchant.accounts.v1beta.DeliveryTime.Builder
getDeliveryTimeBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getDeliveryTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.shopping.merchant.accounts.v1beta.DeliveryTimeOrBuilder
getDeliveryTimeOrBuilder() {
if (deliveryTimeBuilder_ != null) {
return deliveryTimeBuilder_.getMessageOrBuilder();
} else {
return deliveryTime_ == null
? com.google.shopping.merchant.accounts.v1beta.DeliveryTime.getDefaultInstance()
: deliveryTime_;
}
}
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.DeliveryTime,
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.Builder,
com.google.shopping.merchant.accounts.v1beta.DeliveryTimeOrBuilder>
getDeliveryTimeFieldBuilder() {
if (deliveryTimeBuilder_ == null) {
deliveryTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.DeliveryTime,
com.google.shopping.merchant.accounts.v1beta.DeliveryTime.Builder,
com.google.shopping.merchant.accounts.v1beta.DeliveryTimeOrBuilder>(
getDeliveryTime(), getParentForChildren(), isClean());
deliveryTime_ = null;
}
return deliveryTimeBuilder_;
}
private java.util.List rateGroups_ =
java.util.Collections.emptyList();
private void ensureRateGroupsIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
rateGroups_ =
new java.util.ArrayList(
rateGroups_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.RateGroup,
com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder,
com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder>
rateGroupsBuilder_;
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getRateGroupsList() {
if (rateGroupsBuilder_ == null) {
return java.util.Collections.unmodifiableList(rateGroups_);
} else {
return rateGroupsBuilder_.getMessageList();
}
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public int getRateGroupsCount() {
if (rateGroupsBuilder_ == null) {
return rateGroups_.size();
} else {
return rateGroupsBuilder_.getCount();
}
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.RateGroup getRateGroups(int index) {
if (rateGroupsBuilder_ == null) {
return rateGroups_.get(index);
} else {
return rateGroupsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setRateGroups(
int index, com.google.shopping.merchant.accounts.v1beta.RateGroup value) {
if (rateGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRateGroupsIsMutable();
rateGroups_.set(index, value);
onChanged();
} else {
rateGroupsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setRateGroups(
int index, com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder builderForValue) {
if (rateGroupsBuilder_ == null) {
ensureRateGroupsIsMutable();
rateGroups_.set(index, builderForValue.build());
onChanged();
} else {
rateGroupsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addRateGroups(com.google.shopping.merchant.accounts.v1beta.RateGroup value) {
if (rateGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRateGroupsIsMutable();
rateGroups_.add(value);
onChanged();
} else {
rateGroupsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addRateGroups(
int index, com.google.shopping.merchant.accounts.v1beta.RateGroup value) {
if (rateGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRateGroupsIsMutable();
rateGroups_.add(index, value);
onChanged();
} else {
rateGroupsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addRateGroups(
com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder builderForValue) {
if (rateGroupsBuilder_ == null) {
ensureRateGroupsIsMutable();
rateGroups_.add(builderForValue.build());
onChanged();
} else {
rateGroupsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addRateGroups(
int index, com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder builderForValue) {
if (rateGroupsBuilder_ == null) {
ensureRateGroupsIsMutable();
rateGroups_.add(index, builderForValue.build());
onChanged();
} else {
rateGroupsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAllRateGroups(
java.lang.Iterable extends com.google.shopping.merchant.accounts.v1beta.RateGroup>
values) {
if (rateGroupsBuilder_ == null) {
ensureRateGroupsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, rateGroups_);
onChanged();
} else {
rateGroupsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearRateGroups() {
if (rateGroupsBuilder_ == null) {
rateGroups_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
rateGroupsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder removeRateGroups(int index) {
if (rateGroupsBuilder_ == null) {
ensureRateGroupsIsMutable();
rateGroups_.remove(index);
onChanged();
} else {
rateGroupsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder getRateGroupsBuilder(
int index) {
return getRateGroupsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder getRateGroupsOrBuilder(
int index) {
if (rateGroupsBuilder_ == null) {
return rateGroups_.get(index);
} else {
return rateGroupsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List extends com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder>
getRateGroupsOrBuilderList() {
if (rateGroupsBuilder_ != null) {
return rateGroupsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rateGroups_);
}
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder addRateGroupsBuilder() {
return getRateGroupsFieldBuilder()
.addBuilder(com.google.shopping.merchant.accounts.v1beta.RateGroup.getDefaultInstance());
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder addRateGroupsBuilder(
int index) {
return getRateGroupsFieldBuilder()
.addBuilder(
index, com.google.shopping.merchant.accounts.v1beta.RateGroup.getDefaultInstance());
}
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getRateGroupsBuilderList() {
return getRateGroupsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.RateGroup,
com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder,
com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder>
getRateGroupsFieldBuilder() {
if (rateGroupsBuilder_ == null) {
rateGroupsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.RateGroup,
com.google.shopping.merchant.accounts.v1beta.RateGroup.Builder,
com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder>(
rateGroups_, ((bitField0_ & 0x00000020) != 0), getParentForChildren(), isClean());
rateGroups_ = null;
}
return rateGroupsBuilder_;
}
private int shipmentType_ = 0;
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return Whether the shipmentType field is set.
*/
@java.lang.Override
public boolean hasShipmentType() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return The enum numeric value on the wire for shipmentType.
*/
@java.lang.Override
public int getShipmentTypeValue() {
return shipmentType_;
}
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @param value The enum numeric value on the wire for shipmentType to set.
* @return This builder for chaining.
*/
public Builder setShipmentTypeValue(int value) {
shipmentType_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return The shipmentType.
*/
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType getShipmentType() {
com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType result =
com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType.forNumber(
shipmentType_);
return result == null
? com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType.UNRECOGNIZED
: result;
}
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @param value The shipmentType to set.
* @return This builder for chaining.
*/
public Builder setShipmentType(
com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
shipmentType_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return This builder for chaining.
*/
public Builder clearShipmentType() {
bitField0_ = (bitField0_ & ~0x00000040);
shipmentType_ = 0;
onChanged();
return this;
}
private com.google.shopping.type.Price minimumOrderValue_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.type.Price,
com.google.shopping.type.Price.Builder,
com.google.shopping.type.PriceOrBuilder>
minimumOrderValueBuilder_;
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*
* @return Whether the minimumOrderValue field is set.
*/
public boolean hasMinimumOrderValue() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*
* @return The minimumOrderValue.
*/
public com.google.shopping.type.Price getMinimumOrderValue() {
if (minimumOrderValueBuilder_ == null) {
return minimumOrderValue_ == null
? com.google.shopping.type.Price.getDefaultInstance()
: minimumOrderValue_;
} else {
return minimumOrderValueBuilder_.getMessage();
}
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
public Builder setMinimumOrderValue(com.google.shopping.type.Price value) {
if (minimumOrderValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
minimumOrderValue_ = value;
} else {
minimumOrderValueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
public Builder setMinimumOrderValue(com.google.shopping.type.Price.Builder builderForValue) {
if (minimumOrderValueBuilder_ == null) {
minimumOrderValue_ = builderForValue.build();
} else {
minimumOrderValueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
public Builder mergeMinimumOrderValue(com.google.shopping.type.Price value) {
if (minimumOrderValueBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& minimumOrderValue_ != null
&& minimumOrderValue_ != com.google.shopping.type.Price.getDefaultInstance()) {
getMinimumOrderValueBuilder().mergeFrom(value);
} else {
minimumOrderValue_ = value;
}
} else {
minimumOrderValueBuilder_.mergeFrom(value);
}
if (minimumOrderValue_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
public Builder clearMinimumOrderValue() {
bitField0_ = (bitField0_ & ~0x00000080);
minimumOrderValue_ = null;
if (minimumOrderValueBuilder_ != null) {
minimumOrderValueBuilder_.dispose();
minimumOrderValueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
public com.google.shopping.type.Price.Builder getMinimumOrderValueBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getMinimumOrderValueFieldBuilder().getBuilder();
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
public com.google.shopping.type.PriceOrBuilder getMinimumOrderValueOrBuilder() {
if (minimumOrderValueBuilder_ != null) {
return minimumOrderValueBuilder_.getMessageOrBuilder();
} else {
return minimumOrderValue_ == null
? com.google.shopping.type.Price.getDefaultInstance()
: minimumOrderValue_;
}
}
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.type.Price,
com.google.shopping.type.Price.Builder,
com.google.shopping.type.PriceOrBuilder>
getMinimumOrderValueFieldBuilder() {
if (minimumOrderValueBuilder_ == null) {
minimumOrderValueBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.type.Price,
com.google.shopping.type.Price.Builder,
com.google.shopping.type.PriceOrBuilder>(
getMinimumOrderValue(), getParentForChildren(), isClean());
minimumOrderValue_ = null;
}
return minimumOrderValueBuilder_;
}
private com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable
minimumOrderValueTable_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable,
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable.Builder,
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTableOrBuilder>
minimumOrderValueTableBuilder_;
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*
* @return Whether the minimumOrderValueTable field is set.
*/
public boolean hasMinimumOrderValueTable() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*
* @return The minimumOrderValueTable.
*/
public com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable
getMinimumOrderValueTable() {
if (minimumOrderValueTableBuilder_ == null) {
return minimumOrderValueTable_ == null
? com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable
.getDefaultInstance()
: minimumOrderValueTable_;
} else {
return minimumOrderValueTableBuilder_.getMessage();
}
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
public Builder setMinimumOrderValueTable(
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable value) {
if (minimumOrderValueTableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
minimumOrderValueTable_ = value;
} else {
minimumOrderValueTableBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
public Builder setMinimumOrderValueTable(
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable.Builder
builderForValue) {
if (minimumOrderValueTableBuilder_ == null) {
minimumOrderValueTable_ = builderForValue.build();
} else {
minimumOrderValueTableBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
public Builder mergeMinimumOrderValueTable(
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable value) {
if (minimumOrderValueTableBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)
&& minimumOrderValueTable_ != null
&& minimumOrderValueTable_
!= com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable
.getDefaultInstance()) {
getMinimumOrderValueTableBuilder().mergeFrom(value);
} else {
minimumOrderValueTable_ = value;
}
} else {
minimumOrderValueTableBuilder_.mergeFrom(value);
}
if (minimumOrderValueTable_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
public Builder clearMinimumOrderValueTable() {
bitField0_ = (bitField0_ & ~0x00000100);
minimumOrderValueTable_ = null;
if (minimumOrderValueTableBuilder_ != null) {
minimumOrderValueTableBuilder_.dispose();
minimumOrderValueTableBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
public com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable.Builder
getMinimumOrderValueTableBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getMinimumOrderValueTableFieldBuilder().getBuilder();
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
public com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTableOrBuilder
getMinimumOrderValueTableOrBuilder() {
if (minimumOrderValueTableBuilder_ != null) {
return minimumOrderValueTableBuilder_.getMessageOrBuilder();
} else {
return minimumOrderValueTable_ == null
? com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable
.getDefaultInstance()
: minimumOrderValueTable_;
}
}
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable,
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable.Builder,
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTableOrBuilder>
getMinimumOrderValueTableFieldBuilder() {
if (minimumOrderValueTableBuilder_ == null) {
minimumOrderValueTableBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable,
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable.Builder,
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTableOrBuilder>(
getMinimumOrderValueTable(), getParentForChildren(), isClean());
minimumOrderValueTable_ = null;
}
return minimumOrderValueTableBuilder_;
}
private com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig storeConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfigOrBuilder>
storeConfigBuilder_;
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*
* @return Whether the storeConfig field is set.
*/
public boolean hasStoreConfig() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*
* @return The storeConfig.
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig getStoreConfig() {
if (storeConfigBuilder_ == null) {
return storeConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.getDefaultInstance()
: storeConfig_;
} else {
return storeConfigBuilder_.getMessage();
}
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
public Builder setStoreConfig(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig value) {
if (storeConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
storeConfig_ = value;
} else {
storeConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
public Builder setStoreConfig(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.Builder builderForValue) {
if (storeConfigBuilder_ == null) {
storeConfig_ = builderForValue.build();
} else {
storeConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
public Builder mergeStoreConfig(
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig value) {
if (storeConfigBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)
&& storeConfig_ != null
&& storeConfig_
!= com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig
.getDefaultInstance()) {
getStoreConfigBuilder().mergeFrom(value);
} else {
storeConfig_ = value;
}
} else {
storeConfigBuilder_.mergeFrom(value);
}
if (storeConfig_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
public Builder clearStoreConfig() {
bitField0_ = (bitField0_ & ~0x00000200);
storeConfig_ = null;
if (storeConfigBuilder_ != null) {
storeConfigBuilder_.dispose();
storeConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.Builder
getStoreConfigBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getStoreConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.StoreConfigOrBuilder
getStoreConfigOrBuilder() {
if (storeConfigBuilder_ != null) {
return storeConfigBuilder_.getMessageOrBuilder();
} else {
return storeConfig_ == null
? com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.getDefaultInstance()
: storeConfig_;
}
}
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfigOrBuilder>
getStoreConfigFieldBuilder() {
if (storeConfigBuilder_ == null) {
storeConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfigOrBuilder>(
getStoreConfig(), getParentForChildren(), isClean());
storeConfig_ = null;
}
return storeConfigBuilder_;
}
private java.util.List
loyaltyPrograms_ = java.util.Collections.emptyList();
private void ensureLoyaltyProgramsIsMutable() {
if (!((bitField0_ & 0x00000400) != 0)) {
loyaltyPrograms_ =
new java.util.ArrayList<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram>(
loyaltyPrograms_);
bitField0_ |= 0x00000400;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder>
loyaltyProgramsBuilder_;
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getLoyaltyProgramsList() {
if (loyaltyProgramsBuilder_ == null) {
return java.util.Collections.unmodifiableList(loyaltyPrograms_);
} else {
return loyaltyProgramsBuilder_.getMessageList();
}
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public int getLoyaltyProgramsCount() {
if (loyaltyProgramsBuilder_ == null) {
return loyaltyPrograms_.size();
} else {
return loyaltyProgramsBuilder_.getCount();
}
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram getLoyaltyPrograms(
int index) {
if (loyaltyProgramsBuilder_ == null) {
return loyaltyPrograms_.get(index);
} else {
return loyaltyProgramsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setLoyaltyPrograms(
int index, com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram value) {
if (loyaltyProgramsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.set(index, value);
onChanged();
} else {
loyaltyProgramsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setLoyaltyPrograms(
int index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder
builderForValue) {
if (loyaltyProgramsBuilder_ == null) {
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.set(index, builderForValue.build());
onChanged();
} else {
loyaltyProgramsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyPrograms(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram value) {
if (loyaltyProgramsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.add(value);
onChanged();
} else {
loyaltyProgramsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyPrograms(
int index, com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram value) {
if (loyaltyProgramsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.add(index, value);
onChanged();
} else {
loyaltyProgramsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyPrograms(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder
builderForValue) {
if (loyaltyProgramsBuilder_ == null) {
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.add(builderForValue.build());
onChanged();
} else {
loyaltyProgramsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addLoyaltyPrograms(
int index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder
builderForValue) {
if (loyaltyProgramsBuilder_ == null) {
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.add(index, builderForValue.build());
onChanged();
} else {
loyaltyProgramsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAllLoyaltyPrograms(
java.lang.Iterable<
? extends com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram>
values) {
if (loyaltyProgramsBuilder_ == null) {
ensureLoyaltyProgramsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, loyaltyPrograms_);
onChanged();
} else {
loyaltyProgramsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearLoyaltyPrograms() {
if (loyaltyProgramsBuilder_ == null) {
loyaltyPrograms_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
} else {
loyaltyProgramsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder removeLoyaltyPrograms(int index) {
if (loyaltyProgramsBuilder_ == null) {
ensureLoyaltyProgramsIsMutable();
loyaltyPrograms_.remove(index);
onChanged();
} else {
loyaltyProgramsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder
getLoyaltyProgramsBuilder(int index) {
return getLoyaltyProgramsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder
getLoyaltyProgramsOrBuilder(int index) {
if (loyaltyProgramsBuilder_ == null) {
return loyaltyPrograms_.get(index);
} else {
return loyaltyProgramsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List<
? extends com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder>
getLoyaltyProgramsOrBuilderList() {
if (loyaltyProgramsBuilder_ != null) {
return loyaltyProgramsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(loyaltyPrograms_);
}
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder
addLoyaltyProgramsBuilder() {
return getLoyaltyProgramsFieldBuilder()
.addBuilder(
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.getDefaultInstance());
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder
addLoyaltyProgramsBuilder(int index) {
return getLoyaltyProgramsFieldBuilder()
.addBuilder(
index,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram
.getDefaultInstance());
}
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder>
getLoyaltyProgramsBuilderList() {
return getLoyaltyProgramsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder>
getLoyaltyProgramsFieldBuilder() {
if (loyaltyProgramsBuilder_ == null) {
loyaltyProgramsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram.Builder,
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder>(
loyaltyPrograms_,
((bitField0_ & 0x00000400) != 0),
getParentForChildren(),
isClean());
loyaltyPrograms_ = null;
}
return loyaltyProgramsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.shopping.merchant.accounts.v1beta.Service)
}
// @@protoc_insertion_point(class_scope:google.shopping.merchant.accounts.v1beta.Service)
private static final com.google.shopping.merchant.accounts.v1beta.Service DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.shopping.merchant.accounts.v1beta.Service();
}
public static com.google.shopping.merchant.accounts.v1beta.Service getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Service parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.shopping.merchant.accounts.v1beta.Service getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy