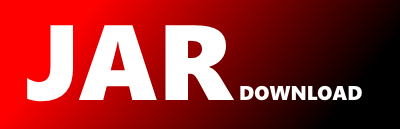
com.google.shopping.merchant.accounts.v1beta.ServiceOrBuilder Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/shopping/merchant/accounts/v1beta/shippingsettings.proto
// Protobuf Java Version: 3.25.5
package com.google.shopping.merchant.accounts.v1beta;
public interface ServiceOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.shopping.merchant.accounts.v1beta.Service)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the serviceName field is set.
*/
boolean hasServiceName();
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The serviceName.
*/
java.lang.String getServiceName();
/**
*
*
*
* Required. Free-form name of the service. Must be unique within target
* account.
*
*
* optional string service_name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for serviceName.
*/
com.google.protobuf.ByteString getServiceNameBytes();
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the active field is set.
*/
boolean hasActive();
/**
*
*
*
* Required. A boolean exposing the active status of the shipping service.
*
*
* optional bool active = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The active.
*/
boolean getActive();
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return A list containing the deliveryCountries.
*/
java.util.List getDeliveryCountriesList();
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return The count of deliveryCountries.
*/
int getDeliveryCountriesCount();
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @param index The index of the element to return.
* @return The deliveryCountries at the given index.
*/
java.lang.String getDeliveryCountries(int index);
/**
*
*
*
* Required. The CLDR territory code of the countries to which the service
* applies.
*
*
* repeated string delivery_countries = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @param index The index of the value to return.
* @return The bytes of the deliveryCountries at the given index.
*/
com.google.protobuf.ByteString getDeliveryCountriesBytes(int index);
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return Whether the currencyCode field is set.
*/
boolean hasCurrencyCode();
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return The currencyCode.
*/
java.lang.String getCurrencyCode();
/**
*
*
*
* The CLDR code of the currency to which this service applies. Must match
* that of the prices in rate groups.
*
*
* optional string currency_code = 4;
*
* @return The bytes for currencyCode.
*/
com.google.protobuf.ByteString getCurrencyCodeBytes();
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the deliveryTime field is set.
*/
boolean hasDeliveryTime();
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The deliveryTime.
*/
com.google.shopping.merchant.accounts.v1beta.DeliveryTime getDeliveryTime();
/**
*
*
*
* Required. Time spent in various aspects from order to the delivery of the
* product.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.DeliveryTime delivery_time = 5 [(.google.api.field_behavior) = REQUIRED];
*
*/
com.google.shopping.merchant.accounts.v1beta.DeliveryTimeOrBuilder getDeliveryTimeOrBuilder();
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List getRateGroupsList();
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.shopping.merchant.accounts.v1beta.RateGroup getRateGroups(int index);
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getRateGroupsCount();
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List extends com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder>
getRateGroupsOrBuilderList();
/**
*
*
*
* Optional. Shipping rate group definitions. Only the last one is allowed to
* have an empty `applicable_shipping_labels`, which means "everything else".
* The other `applicable_shipping_labels` must not overlap.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.RateGroup rate_groups = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.shopping.merchant.accounts.v1beta.RateGroupOrBuilder getRateGroupsOrBuilder(int index);
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return Whether the shipmentType field is set.
*/
boolean hasShipmentType();
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return The enum numeric value on the wire for shipmentType.
*/
int getShipmentTypeValue();
/**
*
*
*
* Type of locations this service ships orders to.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.ShipmentType shipment_type = 7;
*
*
* @return The shipmentType.
*/
com.google.shopping.merchant.accounts.v1beta.Service.ShipmentType getShipmentType();
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*
* @return Whether the minimumOrderValue field is set.
*/
boolean hasMinimumOrderValue();
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*
* @return The minimumOrderValue.
*/
com.google.shopping.type.Price getMinimumOrderValue();
/**
*
*
*
* Minimum order value for this service. If set, indicates that customers
* will have to spend at least this amount.
* All prices within a service must have the same currency.
* Cannot be set together with minimum_order_value_table.
*
*
* optional .google.shopping.type.Price minimum_order_value = 8;
*/
com.google.shopping.type.PriceOrBuilder getMinimumOrderValueOrBuilder();
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*
* @return Whether the minimumOrderValueTable field is set.
*/
boolean hasMinimumOrderValueTable();
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*
* @return The minimumOrderValueTable.
*/
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable getMinimumOrderValueTable();
/**
*
*
*
* Table of per store minimum order values for the pickup fulfillment type.
* Cannot be set together with minimum_order_value.
*
*
*
* optional .google.shopping.merchant.accounts.v1beta.MinimumOrderValueTable minimum_order_value_table = 9;
*
*/
com.google.shopping.merchant.accounts.v1beta.MinimumOrderValueTableOrBuilder
getMinimumOrderValueTableOrBuilder();
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*
* @return Whether the storeConfig field is set.
*/
boolean hasStoreConfig();
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*
* @return The storeConfig.
*/
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfig getStoreConfig();
/**
*
*
*
* A list of stores your products are delivered from.
* This is only valid for the local delivery shipment type.
*
*
* optional .google.shopping.merchant.accounts.v1beta.Service.StoreConfig store_config = 10;
*
*/
com.google.shopping.merchant.accounts.v1beta.Service.StoreConfigOrBuilder
getStoreConfigOrBuilder();
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List
getLoyaltyProgramsList();
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram getLoyaltyPrograms(int index);
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getLoyaltyProgramsCount();
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List<
? extends com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder>
getLoyaltyProgramsOrBuilderList();
/**
*
*
*
* Optional. Loyalty programs that this shipping service is limited to.
*
*
*
* repeated .google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgram loyalty_programs = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.shopping.merchant.accounts.v1beta.Service.LoyaltyProgramOrBuilder
getLoyaltyProgramsOrBuilder(int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy