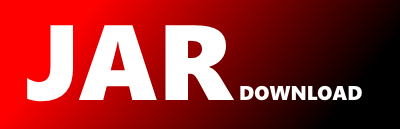
com.google.sitebricks.persist.EntityQuery Maven / Gradle / Ivy
package com.google.sitebricks.persist;
import java.util.List;
/**
* @author [email protected] (Dhanji R. Prasanna)
*/
public interface EntityQuery {
Clause where(E field, FieldMatcher matcher);
public static interface Clause {
Clause and(E field, FieldMatcher matcher);
EntityQuery or();
List list();
List list(int limit);
List list(int offset, int limit);
/**
* Delete all matching persistent objects from the underlying datastore. Useful
* for bulk deletions, only datastores that support this natively will work.
*/
void remove();
}
public static class FieldMatcher {
public final Kind kind;
public final E low, high;
public final double threshold;
protected FieldMatcher(Kind kind, E value) {
this(kind, value, null, -1.0);
}
protected FieldMatcher(Kind kind, E low, E high) {
this(kind, low, high, -1.0);
}
protected FieldMatcher(Kind kind, E low, E high, double threshold) {
this.kind = kind;
this.low = low;
this.high = high;
this.threshold = threshold;
}
public static FieldMatcher is(T t) {
return new FieldMatcher(Kind.IS, t);
}
public static FieldMatcher not(T t) {
return new FieldMatcher(Kind.NOT, t);
}
public static FieldMatcher like(String t) {
return new FieldMatcher(Kind.LIKE, t);
}
/**
* Adds fuzzy query constraint on the given string matched against a fuzziness threshold.
* For example:
*
* similarTo("cafe", 1.0);
*
*
* May return "cate", "café", "late" etc., depending on the threshold. The threshold is an
* abstract specifier, which the underlying datastore may choose to incorporate into its
* algorithm or ignore completely.
*
* A value of 0 of the threshold indicates an exact match, whereas 1.0 indicates an maximally
* expansive, fuzzy match.
*
*/
public static FieldMatcher similarTo(String t, double threshold) {
if (threshold > 1.0 || threshold < 0.0)
throw new IllegalArgumentException("Similarity threshold must be between [0 - 1]");
return new FieldMatcher(Kind.SIMILAR_TO, t, null, threshold);
}
public static FieldMatcher between(T t1, T t2) {
return new FieldMatcher(Kind.BETWEEN, t1, t2);
}
public static FieldMatcher above(T t) {
return new FieldMatcher(Kind.ABOVE, t);
}
public static FieldMatcher below(T t) {
return new FieldMatcher(Kind.BELOW, t);
}
public static FieldMatcher aboveIncluding(T t) {
return new FieldMatcher(Kind.ABOVE_INCLUDING, t);
}
public static FieldMatcher belowIncluding(T t) {
return new FieldMatcher(Kind.BELOW_INCLUDING, t);
}
public static enum Kind {
IS, NOT, LIKE, SIMILAR_TO, ABOVE, BELOW, ABOVE_INCLUDING, BELOW_INCLUDING, BETWEEN,
CUSTOM,
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy