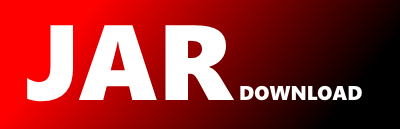
com.sri.ai.grinder.sgdpllt.library.indexexpression.IndexExpressions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aic-expresso Show documentation
Show all versions of aic-expresso Show documentation
SRI International's AIC Symbolic Manipulation and Evaluation Library (for Java 1.8+)
The newest version!
package com.sri.ai.grinder.sgdpllt.library.indexexpression;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.google.common.base.Function;
import com.google.common.base.Predicate;
import com.sri.ai.expresso.api.Expression;
import com.sri.ai.expresso.api.IndexExpressionsSet;
import com.sri.ai.expresso.api.QuantifiedExpression;
import com.sri.ai.expresso.api.SyntaxTree;
import com.sri.ai.expresso.core.ExtensionalIndexExpressionsSet;
import com.sri.ai.expresso.helper.Expressions;
import com.sri.ai.grinder.api.Registry;
import com.sri.ai.grinder.helper.GrinderUtil;
import com.sri.ai.grinder.sgdpllt.api.Context;
import com.sri.ai.grinder.sgdpllt.library.FunctorConstants;
import com.sri.ai.util.Util;
import com.sri.ai.util.base.Null;
import com.sri.ai.util.base.Pair;
/**
* Provides multiple utility methods for manipulating index expressions.
* An index is either a symbol or a function application of a function other than "in".
* An index expression is either an index, or a function application of "in" to an index and a set (known as "type" of the index expression).
* An index expression is meant to be used as an index declaration in expressions like
* intensional sets, lambda expressions, and quantified expressions.
* Examples are
*
* - The
X
in the expression there exists X : p(X)
* - The
X in Type
in the expression there exists X in Type : p(X)
* - The
q(X)
in the expression there exists q(X) : p(q(X))
* - The
q(X) in {1,2,3}
in the expression there exists q(X) in {1,2,3} : p(q(X))
* - The
q(X) in {1,2,3}
in the expression { (on q(X) in {1,2,3}) q(X) + 2 | q(X) != 2 }
*
*
* @author braz
*
*/
public class IndexExpressions {
public static LinkedHashMap getIndexToTypeMapWithDefaultNull(IndexExpressionsSet indexExpressions) {
List indexExpressionsList = ((ExtensionalIndexExpressionsSet) indexExpressions).getList();
LinkedHashMap result =
Expressions.getRelationalMap(
indexExpressionsList,
Expressions.makeSymbol("in"),
new Null());
return result;
}
public static ExtensionalIndexExpressionsSet makeExtensionalIndexExpressionsSetFromSymbolsAndTypesStrings(String...symbolsAndTypes) {
Expression[] symbolsAndTypesExpressions = GrinderUtil.makeListOfSymbolsAndTypesExpressionsFromSymbolsAndTypesStrings(symbolsAndTypes);
List indexExpressions = GrinderUtil.makeIndexExpressionsFromSymbolsAndTypes(symbolsAndTypesExpressions);
ExtensionalIndexExpressionsSet result = new ExtensionalIndexExpressionsSet(indexExpressions);
return result;
}
public static LinkedHashMap
getIndexToTypeMapWithDefaultTypeOfIndex(IndexExpressionsSet indexExpressions) {
List indexExpressionsList = ((ExtensionalIndexExpressionsSet) indexExpressions).getList();
LinkedHashMap result =
Expressions.getRelationalMap(
indexExpressionsList,
Expressions.makeSymbol("in"),
new TypeOfIndexInIndexExpression());
return result;
}
public static Expression copyIndexExpressionWithNewIndex(Expression indexExpression, Expression newIndex) {
if (newIndex != IndexExpressions.getIndex(indexExpression)) {
Expression result;
if (indexExpression.hasFunctor("in")) {
result = Expressions.apply("in", newIndex, indexExpression.get(1));
}
else {
result = newIndex;
}
return result;
}
return indexExpression;
}
public static boolean indexExpressionsContainIndex(IndexExpressionsSet indexExpressions, Expression index) {
List indexExpressionsList = ((ExtensionalIndexExpressionsSet) indexExpressions).getList();
boolean result = Util.findFirst(indexExpressionsList, new HasIndex(index)) != null;
return result;
}
public static List getIndexExpressionsWithType(IndexExpressionsSet indexExpressions) {
List indexExpressionsList = ((ExtensionalIndexExpressionsSet) indexExpressions).getList();
List result = Util.replaceElementsNonDestructively(indexExpressionsList, IndexExpressions.getMakeIndexExpressionWithType());
return result;
}
public static Function getMakeIndexExpressionWithType() {
return new Function() {
@Override
public Expression apply(Expression indexExpression) {
if (indexExpression.hasFunctor("in")) {
return indexExpression;
}
return Expressions.apply(
"in",
indexExpression,
Expressions.makeExpressionOnSyntaxTreeWithLabelAndSubTrees("type", indexExpression));
}
};
}
private static class TypeOfIndexInIndexExpression implements Function {
@Override
public Expression apply(Expression expression) {
return Expressions.makeExpressionOnSyntaxTreeWithLabelAndSubTrees("type", expression);
}
}
/**
* Indicates whether a received index expression's index is in a given collection.
* @author braz
*/
public static class IndexExpressionHasIndexIn implements Predicate {
private Collection indices;
public IndexExpressionHasIndexIn(Collection indices) {
super();
this.indices = indices;
}
@Override
public boolean apply(Expression expression) {
boolean result = indices.contains(getIndex(expression));
return result;
}
}
public static class GetIndex implements Function {
@Override
public Expression apply(Expression indexExpression) {
return getIndex(indexExpression);
}
}
public static GetIndex GET_INDEX = new GetIndex();
public static class IsIndexIn implements Predicate {
private IndexExpressionsSet indexExpressions;
public IsIndexIn(IndexExpressionsSet indexExpressions) {
super();
this.indexExpressions = indexExpressions;
}
@Override
public boolean apply(Expression possibleIndex) {
List indexExpressionsList = ((ExtensionalIndexExpressionsSet) indexExpressions).getList();
boolean result = Util.thereExists(indexExpressionsList, new HasIndex(possibleIndex));
return result;
}
}
public static class HasIndex implements Predicate {
private Expression index;
public HasIndex(Expression index) {
this.index = index;
}
@Override
public boolean apply(Expression indexExpression) {
if (getIndex(indexExpression).equals(index)) {
return true;
}
return false;
}
}
/**
* Makes an index expression for variable and type (the latter being possibly null
indicating unknown type).
*/
public static Expression makeIndexExpression(Expression index, Expression type) {
Expression result = type == null? index : Expressions.apply("in", index, type);
return result;
}
public static Expression replaceOrAddType(Expression indexExpression, Expression newType) {
Pair indexAndType = getIndexAndDomain(indexExpression);
Expression result;
if (newType.equals(indexAndType.second)) {
result = indexExpression;
}
else {
result = makeIndexExpression(indexAndType.first, newType);
}
return result;
}
public static Expression replaceArgument(Expression indexExpression, int argumentIndex, Expression newArgument) {
Pair indexAndType = getIndexAndDomain(indexExpression);
Expression index = indexAndType.first;
Expression type = indexAndType.second;
Expression argument = index.get(argumentIndex);
Expression result;
if (newArgument.equals(argument)) {
result = indexExpression;
}
else {
Expression newIndex = index.set(argumentIndex, newArgument);
result = makeIndexExpression(newIndex, type);
}
return result;
}
public static Pair getIndexAndDomain(Expression indexExpression) {
boolean bothIndexAndDomain = indexExpression.hasFunctor("in") && indexExpression.numberOfArguments() == 2;
Expression index;
Expression indexDomain;
if (bothIndexAndDomain) {
index = indexExpression.get(0);
indexDomain = indexExpression.get(1);
}
else {
index = indexExpression;
indexDomain = type(index);
}
return new Pair(index, indexDomain);
}
public static Expression type(Expression expression) {
return Expressions.makeExpressionOnSyntaxTreeWithLabelAndSubTrees("type", expression);
}
public static Expression getIndex(Expression indexExpression) {
if (indexExpression.hasFunctor("in")) {
return indexExpression.get(0);
}
return indexExpression;
}
public static Expression getType(Expression indexExpression) {
Pair indexAndDomain = getIndexAndDomain(indexExpression);
return indexAndDomain.second;
}
public static void addSubTreeWithIndexAndBasePathPlusArgumentIndex(
Expression syntaxTree, int subTreeIndex, List basePath,
List>> result) {
SyntaxTree subTree = syntaxTree.getSyntaxTree().getSubTree(subTreeIndex);
List subExpressionPath = new LinkedList(basePath);
subExpressionPath.add(subTreeIndex);
result.add(new Pair>(subTree, subExpressionPath));
}
/** Return a list of indexes from a list of index expressions. */
public static List getIndices(IndexExpressionsSet indexExpressions) {
List indexExpressionsList = ((ExtensionalIndexExpressionsSet) indexExpressions).getList();
List result = Util.mapIntoList(indexExpressionsList, new GetIndex());
return result;
}
/** Return a set of indexes from a set of index expressions. */
public static Set getIndices(Set indexExpressions) {
Set result = Util.mapIntoSet(indexExpressions, new GetIndex());
return result;
}
public static ExtensionalIndexExpressionsSet getIndexExpressionsFromSymbolsAndTypes(Map variablesAndDomains) {
List indexExpressions = new LinkedList();
for (Map.Entry entry : variablesAndDomains.entrySet()) {
if (entry.getValue() == null) {
indexExpressions.add(entry.getKey());
}
else {
indexExpressions.add(Expressions.apply(FunctorConstants.IN, entry.getKey(), entry.getValue()));
}
}
return new ExtensionalIndexExpressionsSet(indexExpressions);
}
public static Expression getIndexExpressionForVariableFromcontextualSymbolsAndTypes(Expression expression, Context context) {
Expression type = context.getTypeExpressionOfRegisteredSymbol(expression);
Expression indexExpression = makeIndexExpression(expression, type);
return indexExpression;
}
public static Expression renameSymbol(Expression indexExpression, Expression symbol, Expression newSymbol, Registry registry) {
Expression result;
Expression index = getIndex(indexExpression);
if (indexExpression.hasFunctor(FunctorConstants.IN)) {
Expression type = getType(indexExpression);
Expression newIndex = index.replaceSymbol(symbol, newSymbol, registry);
Expression newType = type.replaceSymbol(symbol, newSymbol, registry);
if (newIndex != index || newType != type) {
result = makeIndexExpression(newIndex, newType);
}
else {
result = indexExpression;
}
}
else {
result = index.replaceSymbol(symbol, newSymbol, registry);
}
return result;
}
public static LinkedHashMap getIndexToTypeMapWithDefaultTypeOfIndex(Expression quantifiedExpression) {
IndexExpressionsSet indexExpressions = ((QuantifiedExpression) quantifiedExpression).getIndexExpressions();
return getIndexToTypeMapWithDefaultTypeOfIndex(indexExpressions);
}
public static LinkedHashMap getIndexToTypeMapWithDefaultNull(Expression quantifiedExpression) {
IndexExpressionsSet indexExpressions = ((QuantifiedExpression) quantifiedExpression).getIndexExpressions();
return getIndexToTypeMapWithDefaultNull(indexExpressions);
}
public static Collection getIndexDomainsOfQuantifiedExpression(Expression quantifiedExpression) {
return getIndexToTypeMapWithDefaultTypeOfIndex(quantifiedExpression).values();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy