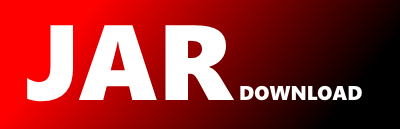
com.sri.ai.util.collect.CopyOnWriteMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aic-util Show documentation
Show all versions of aic-util Show documentation
SRI International's AIC Utility Library (for Java 1.6+)
package com.sri.ai.util.collect;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
/**
* A {@link Map} implementation that directs methods to another map,
* which is either a map received at construction, or a copy of it
* to a new {@link HashMap} made upon the first writing (or potentially writing} operation.
*/
public class CopyOnWriteMap implements Map {
private Map base;
private boolean ownsBase;
public CopyOnWriteMap(Map base) {
this.base = base;
this.ownsBase = false;
}
private void copy() {
if ( ! ownsBase) {
base = new HashMap(base);
ownsBase = true;
}
}
@Override
public void clear() {
base = new HashMap();
}
@Override
public boolean containsKey(Object arg0) {
return base.containsKey(arg0);
}
@Override
public boolean containsValue(Object arg0) {
return base.containsValue(arg0);
}
@Override
public Set> entrySet() {
copy();
return base.entrySet();
}
@Override
public V get(Object arg0) {
return base.get(arg0);
}
@Override
public boolean isEmpty() {
return base.isEmpty();
}
@Override
public Set keySet() {
copy();
return base.keySet();
}
@Override
public V put(K arg0, V arg1) {
copy();
return base.put(arg0, arg1);
}
@Override
public void putAll(Map extends K, ? extends V> arg0) {
copy();
base.putAll(arg0);
}
@Override
public V remove(Object arg0) {
V result = null;
Map newBase = new HashMap();
for (Map.Entry entry : base.entrySet()) {
if (entry.getKey().equals(arg0)) {
result = entry.getValue();
}
else {
newBase.put(entry.getKey(), entry.getValue());
}
}
base = newBase;
return result;
}
@Override
public int size() {
return base.size();
}
@Override
public Collection values() {
copy();
return base.values();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy