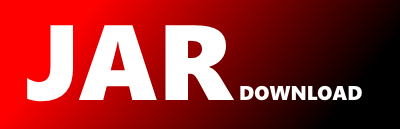
com.sri.ai.util.collect.NonDeterministicIterator Maven / Gradle / Ivy
Show all versions of aic-util Show documentation
/*
* Copyright (c) 2013, SRI International
* All rights reserved.
* Licensed under the The BSD 3-Clause License;
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://opensource.org/licenses/BSD-3-Clause
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* Neither the name of the aic-util nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
* INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT,
* STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED
* OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.sri.ai.util.collect;
import static com.sri.ai.util.Util.iterator;
import java.util.Iterator;
import java.util.Stack;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.sri.ai.util.Util;
import com.sri.ai.util.base.NullaryFunction;
/**
* An abstract iterator ranging over elements on the leaves of a virtual tree.
*
* The choice tree is either a information tree (containing an element of the iterator's range),
* or a non-information tree containing an iterator over sub-tree makers, which are thunks.
* These thunks are allowed to perform whatever bookkeeping necessary,
* and then return a sub-tree.
*
* @author braz
*/
@Beta
public class NonDeterministicIterator extends EZIterator {
private Stack>>> stack;
@SuppressWarnings("unchecked")
public NonDeterministicIterator(NullaryFunction> root) {
stack = new Stack<>();
stack.push(iterator(root));
}
public NonDeterministicIterator(LazyTree root) {
this(() -> root);
}
@Override
protected E calculateNext() {
E result = null;
while (result == null && !stack.isEmpty()) {
Iterator>> branchingPoint = stack.peek();
if (branchingPoint.hasNext()) {
NullaryFunction> branchMaker = branchingPoint.next();
LazyTree branchResult = branchMaker.apply();
Iterator>> subTreeMakers = branchResult.getSubTreeMakers();
if (!subTreeMakers.hasNext()) {
result = branchResult.getInformation();
}
else {
stack.push(subTreeMakers);
}
}
else {
stack.pop();
}
}
return result;
}
@SuppressWarnings("unchecked")
private static Iterator>> makeIterator(String prefix, int depth) {
if (depth == 2) {
return iterator(() -> new DefaultLazyTree(prefix));
}
Function>> fromStringToSubTreeMaker =
s -> () -> new DefaultLazyTree(makeIterator(prefix + s, depth + 1));
Iterator>> result =
FunctionIterator.make(iterator("a", "b", "c"), fromStringToSubTreeMaker);
return result;
}
public static void main(String[] args) {
DefaultLazyTree root = new DefaultLazyTree(makeIterator("info: ", 0));
NonDeterministicIterator it = new NonDeterministicIterator(root);
System.out.println(Util.join(it));
}
}