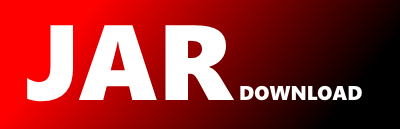
aima.core.probability.CategoricalDistribution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aima-core Show documentation
Show all versions of aima-core Show documentation
AIMA-Java Core Algorithms from the book Artificial Intelligence a Modern Approach 3rd Ed.
package aima.core.probability;
import java.util.Map;
import aima.core.probability.proposition.AssignmentProposition;
/**
* Artificial Intelligence A Modern Approach (3rd Edition): page 487.
*
* A probability distribution for discrete random variables with a finite set of
* values.
* Note: This definition corresponds to that given in AIMA3e pg. 487, for
* a Probability Distribution.
*
* @see Probability Distribution
*
* @author Ciaran O'Reilly
*/
public interface CategoricalDistribution extends ProbabilityMass {
/**
* Interface to be implemented by an object/algorithm that wishes to iterate
* over the possible assignments for the random variables comprising this
* categorical distribution.
*
* @see CategoricalDistribution#iterateOver(Iterator)
* @see CategoricalDistribution#iterateOver(Iterator,
* AssignmentProposition...)
*/
public interface Iterator {
/**
* Called for each possible assignment for the Random Variables
* comprising this CategoricalDistribution.
*
* @param possibleAssignment
* a possible assignment, ω, of variable/value pairs.
* @param probability
* the probability associated with ω
*/
void iterate(Map possibleAssignment,
double probability);
}
/**
* Note: Do not modify the double[] returned by this method directly.
* Instead use setValue() as this method is intended to be for read only
* purposes.
*
* @return the double[] used to represent the CategoricalDistribution.
*
* @see CategoricalDistribution#setValue(int, double)
*/
double[] getValues();
/**
* Set the value at a specified index within the distribution.
*
* @param idx
* @param value
*/
void setValue(int idx, double value);
/**
*
* @return the summation of all of the elements within the Distribution.
*/
double getSum();
/**
* Normalize the values comprising this distribution.
*
* @return this instance with its values normalized.
*/
CategoricalDistribution normalize();
/**
* Retrieve the index into the CategoricalDistribution for the provided set
* of values for the random variables comprising the Distribution.
*
* @param values
* an ordered set of values for the random variables comprising
* the Distribution (Note: the order must match the order
* of the random variables describing the distribution)
* @return the index within the Distribution for the values specified.
*
* @see CategoricalDistribution#getValues()
* @see ProbabilityDistribution#getFor()
*/
int getIndex(Object... values);
/**
* Get the marginal probability for the provided variables from this
* Distribution creating a new Distribution of the remaining variables with
* their values updated with the summed out random variables.
*
* see: AIMA3e page 492.
*
*
* @param vars
* the random variables to marginalize/sum out.
* @return a new Distribution containing any remaining random variables not
* summed out and a new set of values updated with the summed out
* values.
*/
CategoricalDistribution marginal(RandomVariable... vars);
/**
* Divide the dividend (this) CategoricalDistribution by the divisor to
* create a new CategoricalDistribution representing the quotient. The
* variables comprising the divisor distribution must be a subset of the
* dividend. However, ordering of variables does not matter as the quotient
* contains the same variables as the dividend and the internal
* implementation logic should handle iterating through the two
* distributions correctly, irrespective of the order of their variables.
*
* @param divisor
* @return a new Distribution representing the quotient of the dividend
* (this) divided by the divisor.
* @throws IllegalArgumentException
* if the variables of the divisor distribution are not a subset
* of the dividend.
*/
CategoricalDistribution divideBy(CategoricalDistribution divisor);
/**
* Multiplication of this Distribution by a given multiplier, creating a new
* Distribution representing the product of the two. Note: Is
* equivalent to pointwise product calculation on factors.
*
* see: AIMA3e Figure 14.10 page 527.
*
* Note: Default Distribution multiplication is not commutative. The reason
* is because the order of the variables comprising a Distribution dictate
* the ordering of the values for that distribution. For example (the
* General case of Baye's rule, AIMA3e pg. 496), using this API method:
*
* P(Y | X) = (P(X | Y)P(Y))/P(X)
*
* is NOT true, due to multiplication of distributions not being
* commutative. However:
*
* P(Y | X) = (P(Y)P(X | Y))/P(X)
*
* is true, using this API.
*
* The default order of the variable of the Distribution returned is the
* order of the variables as they are seen, as read from the left to right
* term, for e.g.:
*
* P(Y)P(X | Y)
*
* would give a Distribution of the following form:
* Y, X
*
* i.e. an ordered union of the variables from the two distributions.
* To override the default order of the product use multiplyByPOS().
*
* @param multiplier
*
* @return a new Distribution representing the product of this and the
* passed in multiplier. The order of the variables comprising the
* product distribution is the ordered union of the left term (this)
* and the right term (multiplier).
*
* @see CategoricalDistribution#multiplyByPOS(CategoricalDistribution,
* RandomVariable...)
*/
CategoricalDistribution multiplyBy(CategoricalDistribution multiplier);
/**
* Multiplication - Product Order Specified (POS). Note: Is
* equivalent to pointwise product calculation.
*
* see: AIMA3e Figure 14.10 page 527.
*
* Multiplication of this Distribution by a given multiplier, creating a new
* Distribution representing the product of the two. The order of the
* variables comprising the product will match those specified. For example
* (the General case of Baye's rule, AIMA3e pg. 496), using this API method:
*
* P(Y | X) = (P(X | Y)P(Y), [Y, X])/P(X)
*
* is true when the correct product order is specified.
*
* @param multiplier
* @param prodVarOrder
* the order the variables comprising the product are to be in.
*
* @return a new Distribution representing the product of this and the
* passed in multiplier. The order of the variables comprising the
* product distribution is the order specified.
*
* @see CategoricalDistribution#multiplyBy(CategoricalDistribution)
*/
CategoricalDistribution multiplyByPOS(CategoricalDistribution multiplier,
RandomVariable... prodVarOrder);
/**
* Iterate over all the possible value assignments for the Random Variables
* comprising this CategoricalDistribution.
*
* @param cdi
* the CategoricalDistribution Iterator to iterate.
*/
void iterateOver(Iterator cdi);
/**
* Iterate over all possible values assignments for the Random Variables
* comprising this CategoricalDistribution that are not in the fixed set of
* values. This allows you to iterate over a subset of possible
* combinations.
*
* @param cdi
* the CategoricalDistribution Iterator to iterate
* @param fixedValues
* Fixed values for a subset of the Random Variables comprising
* this CategoricalDistribution.
*/
void iterateOver(Iterator cdi, AssignmentProposition... fixedValues);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy