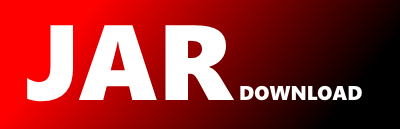
aima.core.search.csp.CSP Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aima-core Show documentation
Show all versions of aima-core Show documentation
AIMA-Java Core Algorithms from the book Artificial Intelligence a Modern Approach 3rd Ed.
package aima.core.search.csp;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Hashtable;
import java.util.List;
/**
* Artificial Intelligence A Modern Approach (3rd Ed.): Section 6.1, Page 202.
*
* A constraint satisfaction problem or CSP consists of three components, X, D,
* and C:
*
* - X is a set of variables, {X1, ... ,Xn}.
* - D is a set of domains, {D1, ... ,Dn}, one for each variable.
* - C is a set of constraints that specify allowable combinations of values.
*
*
* @author Ruediger Lunde
*/
public class CSP {
private List variables;
private List domains;
private List constraints;
/** Lookup, which maps a variable to its index in the list of variables. */
private Hashtable varIndexHash;
/**
* Constraint network. Maps variables to those constraints in which they
* participate.
*/
private Hashtable> cnet;
/** Creates a new CSP. */
public CSP() {
variables = new ArrayList();
domains = new ArrayList();
constraints = new ArrayList();
varIndexHash = new Hashtable();
cnet = new Hashtable>();
}
/** Creates a new CSP. */
public CSP(List vars) {
this();
for (Variable v : vars)
addVariable(v);
}
protected void addVariable(Variable var) {
Domain emptyDomain = new Domain(Collections.emptyList());
variables.add(var);
domains.add(emptyDomain);
varIndexHash.put(var, variables.size()-1);
cnet.put(var, new ArrayList());
}
public List getVariables() {
return Collections.unmodifiableList(variables);
}
public int indexOf(Variable var) {
return varIndexHash.get(var);
}
public Domain getDomain(Variable var) {
return domains.get(varIndexHash.get(var));
}
public void setDomain(Variable var, Domain domain) {
domains.set(indexOf(var), domain);
}
/**
* Replaces the domain of the specified variable by new domain, which
* contains all values of the old domain except the specified value.
*/
public void removeValueFromDomain(Variable var, Object value) {
Domain currDomain = getDomain(var);
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy