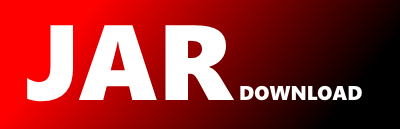
aima.core.util.datastructure.Table Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aima-core Show documentation
Show all versions of aima-core Show documentation
AIMA-Java Core Algorithms from the book Artificial Intelligence a Modern Approach 3rd Ed.
package aima.core.util.datastructure;
import java.util.Hashtable;
import java.util.List;
/**
* @author Ravi Mohan
* @author Mike Stampone
*/
public class Table {
private List rowHeaders;
private List columnHeaders;
private Hashtable> rows;
/**
* Constructs a Table with the specified row and column headers.
*
* @param rowHeaders
* a list of row headers
* @param columnHeaders
* a list of column headers
*/
public Table(List rowHeaders,
List columnHeaders) {
this.rowHeaders = rowHeaders;
this.columnHeaders = columnHeaders;
this.rows = new Hashtable>();
for (RowHeaderType rowHeader : rowHeaders) {
rows.put(rowHeader, new Hashtable());
}
}
/**
* Maps the specified row and column to the specified value in the table.
* Neither the row nor the column nor the value can be null
* The value can be retrieved by calling the get
method with a
* row and column that is equal to the original row and column.
*
* @param r
* the table row
* @param c
* the table column
* @param v
* the value
*
* @throws NullPointerException
* if the row, column, or value is null
.
*/
public void set(RowHeaderType r, ColumnHeaderType c, ValueType v) {
rows.get(r).put(c, v);
}
/**
* Returns the value to which the specified row and column is mapped in this
* table.
*
* @param r
* a row in the table
* @param c
* a column in the table
*
* @return the value to which the row and column is mapped in this table;
* null
if the row and column is not mapped to any
* values in this table.
*
* @throws NullPointerException
* if the row or column is null
.
*/
public ValueType get(RowHeaderType r, ColumnHeaderType c) {
Hashtable rowValues = rows.get(r);
return rowValues == null ? null : rowValues.get(c);
}
@Override
public String toString() {
StringBuffer buf = new StringBuffer();
for (RowHeaderType r : rowHeaders) {
for (ColumnHeaderType c : columnHeaders) {
buf.append(get(r, c).toString());
buf.append(" ");
}
buf.append("\n");
}
return buf.toString();
}
class Row {
private Hashtable cells;
public Row() {
this.cells = new Hashtable();
}
public Hashtable cells() {
return this.cells;
}
}
class Cell {
private ValueHeaderType value;
public Cell() {
value = null;
}
public Cell(ValueHeaderType value) {
this.value = value;
}
public void set(ValueHeaderType value) {
this.value = value;
}
public ValueHeaderType value() {
return value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy