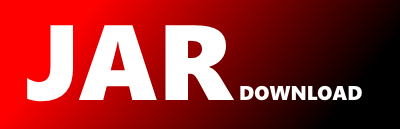
com.googlecode.bspi.ServiceIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of backport-spi Show documentation
Show all versions of backport-spi Show documentation
The backport-spi library is backport of Service Provider Interface to Java5 with extra features.
/*
* Copyright 2010 The backport-spi Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.bspi;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.NoSuchElementException;
/**
*
* @version $Id: ServiceIterator.java 4 2010-07-24 18:45:26Z simone.tripodi $
* @param
*/
final class ServiceIterator implements Iterator {
private static final Charset UTF_8 = Charset.forName("UTF-8");
private final Class service;
private final ClassLoader classLoader;
private final Enumeration serviceResources;
private final LinkedHashMap providers;
private Iterator pending = null;
private String nextName = null;
public ServiceIterator(Class service,
ClassLoader classLoader,
Enumeration serviceResources,
LinkedHashMap providers) {
this.service = service;
this.classLoader = classLoader;
this.serviceResources = serviceResources;
this.providers = providers;
}
public boolean hasNext() {
if (this.nextName != null) {
return true;
}
while ((this.pending == null) || !this.pending.hasNext()) {
if (!serviceResources.hasMoreElements()) {
return false;
}
this.pending = parseServiceFile(this.serviceResources.nextElement());
}
this.nextName = this.pending.next();
return true;
}
public S next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
String className = this.nextName;
this.nextName = null;
try {
S provider = this.service.cast(
Class.forName(className, true, this.classLoader).newInstance());
providers.put(className, provider);
return provider;
} catch (ClassNotFoundException e) {
throw new ServiceConfigurationError("Provider '"
+ className
+ "' not found", e);
} catch (ClassCastException e) {
throw new ServiceConfigurationError("Provider '"
+ className
+ "' is not assignable to Service '"
+ this.service.getName()
+ "'", e);
} catch (Throwable e) {
throw new ServiceConfigurationError("Provider '"
+ className
+ "' could not be instantiated", e);
}
}
public void remove() {
throw new UnsupportedOperationException();
}
private Iterator parseServiceFile(URL url) {
InputStream inputStream = null;
Reader reader = null;
try {
inputStream = url.openStream();
reader = new InputStreamReader(inputStream, UTF_8);
ServiceFileParser serviceFileParser = new ServiceFileParser(reader);
serviceFileParser.setProviders(this.providers);
serviceFileParser.parse();
return serviceFileParser.iterator();
} catch (Exception e) {
throw new ServiceConfigurationError("An error occurred while reading service resource '"
+ url
+ "' for service class '"
+ this.service.getName()
+ "'", e);
} finally {
closeQuietly(reader);
closeQuietly(inputStream);
}
}
private static void closeQuietly(Closeable closeable) {
if (closeable != null) {
try {
closeable.close();
} catch (IOException e) {
// close quietly
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy