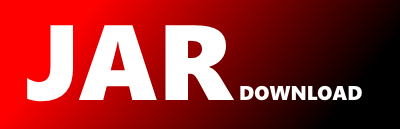
com.googlecode.blaisemath.graphics.UpdatingGraphicComposite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blaise-graphics Show documentation
Show all versions of blaise-graphics Show documentation
Scene graph and style library using Java2D graphics.
package com.googlecode.blaisemath.graphics;
/*
* #%L
* blaise-graphics
* --
* Copyright (C) 2009 - 2024 Elisha Peterson
* --
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.google.common.annotations.Beta;
import com.google.common.collect.BiMap;
import com.google.common.collect.HashBiMap;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import org.checkerframework.checker.nullness.qual.Nullable;
import java.awt.geom.Rectangle2D;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import static java.util.Objects.requireNonNull;
/**
* Encapsulates a set of graphics as a composite, along with elements used
* to create/update the graphics.
*
* @author Elisha Peterson
* @param type of object represented by the composite
*/
@Beta
public class UpdatingGraphicComposite {
/** Contains the graphic elements */
private final GraphicComposite composite = new GraphicComposite<>();
/** Cache of source objects and their locations */
private final Map bounds = Maps.newLinkedHashMap();
/** Index for the graphics, based on source object */
private final BiMap> index = HashBiMap.create();
/** Creates/updates the graphics */
private GraphicUpdater updater;
public UpdatingGraphicComposite(GraphicUpdater updater) {
this.updater = requireNonNull(updater);
}
public static UpdatingGraphicComposite create(GraphicUpdater updater) {
return new UpdatingGraphicComposite<>(updater);
}
//region PROPERTIES
public GraphicComposite getGraphic() {
return composite;
}
public GraphicUpdater getUpdater() {
return updater;
}
public void setUpdater(GraphicUpdater gr) {
this.updater = gr;
}
public void setObjects(Iterable data, Function locMap) {
Collection cData = data instanceof Collection ? (Collection) data : Lists.newArrayList(data);
bounds.keySet().retainAll(cData);
Set> toRemove = Sets.newHashSet(composite.getGraphics());
for (T t : data) {
bounds.put(t, locMap.apply(t));
if (index.containsKey(t)) {
toRemove.remove(index.get(t));
}
}
composite.removeGraphics(toRemove);
index.keySet().retainAll(cData);
updateItemGraphics();
}
private void updateItemGraphics() {
for (T obj : bounds.keySet()) {
Graphic existing = index.get(obj);
Rectangle2D loc = bounds.get(obj);
if (loc == null && existing != null) {
composite.removeGraphic(existing);
index.remove(obj);
} else if (loc != null) {
Graphic gfc = updater.update(obj, loc, existing);
if (existing == null) {
index.put(obj, gfc);
composite.addGraphic(gfc);
}
}
}
}
//endregion
//region LOOKUPS
public T objectOf(Graphic gfc) {
return index.inverse().get(gfc);
}
public Graphic graphicOf(T obj) {
return index.get(obj);
}
//endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy