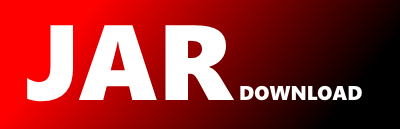
com.googlecode.blaisemath.firestarter.property.EnumObjectEditor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of firestarter Show documentation
Show all versions of firestarter Show documentation
Provides editors for various Java bean object types, and a generic, customizable
PropertySheet.
package com.googlecode.blaisemath.firestarter.property;
/*
* #%L
* Firestarter
* --
* Copyright (C) 2009 - 2022 Elisha Peterson
* --
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.googlecode.blaisemath.firestarter.editor.MPanelEditorSupport;
import java.awt.BorderLayout;
import java.awt.Insets;
import java.awt.Window;
import java.awt.event.ItemEvent;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
/**
* Implements a customized editor for components that do not have a default
* assigned editor, but have an associated "getInstance" method, with argument taking
* an enum value, such that each enum returns a value of the object. When the enum value is
* selected in a ComboBox, the underlying value is updated.
*
* @author Elisha Peterson
*/
public final class EnumObjectEditor extends MPanelEditorSupport {
private static final Logger LOG = Logger.getLogger(EnumObjectEditor.class.getName());
/** Method used to retrieve new instances. */
private Method instanceMethod;
/** Options for selecting different object types */
private JComboBox
© 2015 - 2025 Weber Informatics LLC | Privacy Policy