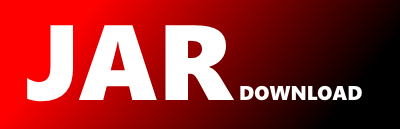
com.cedarsolutions.client.gwt.custom.table.SwitchableSelectionModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cedar-common-gwt Show documentation
Show all versions of cedar-common-gwt Show documentation
Utility code for use with GWT and Mvp4g.
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* C E D A R
* S O L U T I O N S "Software done right."
* S O F T W A R E
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (c) 2013 Kenneth J. Pronovici.
* All rights reserved.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Apache License, Version 2.0.
* See LICENSE for more information about the licensing terms.
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Author : Kenneth J. Pronovici
* Language : Java 6
* Project : Common Java Functionality
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package com.cedarsolutions.client.gwt.custom.table;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import com.google.gwt.view.client.ProvidesKey;
import com.google.gwt.view.client.SelectionChangeEvent;
import com.google.gwt.view.client.SelectionModel.AbstractSelectionModel;
import com.google.gwt.view.client.SetSelectionModel;
/**
* Selection model that allows switching the selection type, for use with DataTable.
*
*
* Code Source
*
*
*
* This code was initially copied from GWT under the terms of its license, and
* was later modified to allow both single and multiple selection types.
*
*
*
*
*
*
* Source:
* {@link com.google.gwt.view.client.MultiSelectionModel}
*
*
* Version:
* GWT 2.5.1
*
*
* Date:
* December, 2013
*
*
*
*
*
* @param Type of the associated table.
* @author Kenneth J. Pronovici
*/
public class SwitchableSelectionModel extends AbstractSelectionModel implements SetSelectionModel {
private SelectionType selectionType;
private Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy