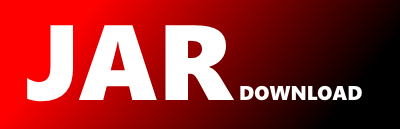
com.cedarsolutions.junit.gwt.classloader.GwtResourceCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cedar-common-gwttestutil Show documentation
Show all versions of cedar-common-gwttestutil Show documentation
Utility code for use in testing GWT, including a stubbed test runner using Mockito.
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* C E D A R
* S O L U T I O N S "Software done right."
* S O F T W A R E
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (c) 2013-2014 Kenneth J. Pronovici.
* All rights reserved.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Apache License, Version 2.0.
* See LICENSE for more information about the licensing terms.
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Author : Kenneth J. Pronovici
* Language : Java 6
* Project : Common Java Functionality
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package com.cedarsolutions.junit.gwt.classloader;
import static org.mockito.Mockito.mock;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.mockito.Mockito;
import org.mockito.invocation.InvocationOnMock;
import org.mockito.stubbing.Answer;
import com.google.gwt.i18n.client.Constants;
import com.google.gwt.i18n.client.Constants.DefaultBooleanValue;
import com.google.gwt.i18n.client.Constants.DefaultDoubleValue;
import com.google.gwt.i18n.client.Constants.DefaultFloatValue;
import com.google.gwt.i18n.client.Constants.DefaultIntValue;
import com.google.gwt.i18n.client.Constants.DefaultStringArrayValue;
import com.google.gwt.i18n.client.Constants.DefaultStringMapValue;
import com.google.gwt.i18n.client.Constants.DefaultStringValue;
import com.google.gwt.i18n.client.ConstantsWithLookup;
import com.google.gwt.i18n.client.Messages;
import com.google.gwt.i18n.client.Messages.DefaultMessage;
/**
* Creates mocked versions of objects for stubbed GWT client tests.
*
*
* This class creates all of its resources as Mockito mocks. There is also
* some additional logic layered on top to handle certain particular types of
* interfaces and classes, like Constants or Messages.
*
*
*
* This class was derived in part from source code in gwt-test-utils. See
* README.credits for more information.
*
*
*
* This whole thing has sort of a hack-ish feel. There's no simple way to
* emulate GWT's behavior, and I can basically only make it work by trial-
* and-error.
*
*
* @author Kenneth J. Pronovici
*/
@SuppressWarnings("unchecked")
public class GwtResourceCreator {
/** Create a mocked resource for a particular class. */
public static synchronized T create(Class> clazz) {
if (ConstantsWithLookup.class.isAssignableFrom(clazz)) {
// ConstantsWithLookup extends Constants, so do this first
return (T) mock(clazz, new ConstantsWithLookupAnswer(clazz));
} else if (Constants.class.isAssignableFrom(clazz)) {
return (T) mock(clazz, new ConstantsAnswer());
} else if (Messages.class.isAssignableFrom(clazz)) {
return (T) mock(clazz, new MessagesAnswer());
} else {
return (T) mock(clazz);
}
}
/** Uses reflection to return proper values for mocks that implement ConstantsWithLookup. */
public static class ConstantsWithLookupAnswer implements Answer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy