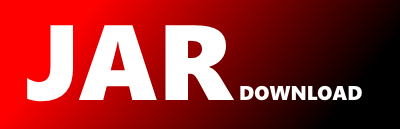
org.paukov.combinatorics.composition.package.html Maven / Gradle / Ivy
This package contains the integer composition generators.
A composition of an integer n is a way of writing n as the sum of a sequence
of (strictly) positive integers. This class generates the composition if a
positive integer value.
A composition of an integer n is a way of writing n as the sum of a sequence
of (strictly) positive integers. Two sequences that differ in the order of
their terms define different compositions of their sum, while they are
considered to define the same partition of that number.
The sixteen compositions of 5 are:
- 5
- 4+1
- 3+2
- 3+1+1
- 2+3
- 2+2+1
- 2+1+2
- 2+1+1+1
- 1+4
- 1+3+1
- 1+2+2
- 1+2+1+1
- 1+1+3
- 1+1+2+1
- 1+1+1+2
- 1+1+1+1+1.
Compare this with the seven partitions of 5:
- 5
- 4+1
- 3+2
- 3+1+1
- 2+2+1
- 2+1+1+1
- 1+1+1+1+1.
Example. Generate compositions all possible integer compositions of 5.
// Create an instance of the integer composition generator to generate all possible compositions of 5
Generator<Integer> gen = CombinatoricsFactory.createCompositionGenerator(5);
// Print the compositions
for (ICombinatoricsVector<Integer> p : gen) {
System.out.println(p);
}
And the result
CombinatoricsVector=([5], size=1)
CombinatoricsVector=([1, 4], size=2)
CombinatoricsVector=([2, 3], size=2)
CombinatoricsVector=([1, 1, 3], size=3)
CombinatoricsVector=([3, 2], size=2)
CombinatoricsVector=([1, 2, 2], size=3)
CombinatoricsVector=([2, 1, 2], size=3)
CombinatoricsVector=([1, 1, 1, 2], size=4)
CombinatoricsVector=([4, 1], size=2)
CombinatoricsVector=([1, 3, 1], size=3)
CombinatoricsVector=([2, 2, 1], size=3)
CombinatoricsVector=([1, 1, 2, 1], size=4)
CombinatoricsVector=([3, 1, 1], size=3)
CombinatoricsVector=([1, 2, 1, 1], size=4)
CombinatoricsVector=([2, 1, 1, 1], size=4)
CombinatoricsVector=([1, 1, 1, 1, 1], size=5)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy