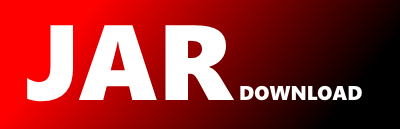
org.paukov.combinatorics.permutations.package.html Maven / Gradle / Ivy
This package contains the generators for the permutations with and without repetitions.
Simple permutations
A permutation is an ordering of a set in the context of all possible orderings. For example, the set
containing the first three digits, 123, has
six permutations: 123, 132, 213, 231, 312, and 321.
This is an example of the permutations of 3 string items (apple, orange, cherry):
// Create the initial vector of 3 elements (apple, orange, cherry)
ICombinatoricsVector<String> originalVector = CombinatoricsFactory.createVector(new String[] { "apple", "orange", "cherry" });
// Create the permutation generator by calling the appropriate method of the Factory class
Generator<String> gen = CombinatoricsFactory.createPermutationGenerator(originalVector);
// Print the result
for (ICombinatoricsVector<String> perm : gen)
System.out.println(perm);
And the result
CombinatoricsVector=([apple, orange, cherry], size=3)
CombinatoricsVector=([apple, cherry, orange], size=3)
CombinatoricsVector=([cherry, apple, orange], size=3)
CombinatoricsVector=([cherry, orange, apple], size=3)
CombinatoricsVector=([orange, cherry, apple], size=3)
CombinatoricsVector=([orange, apple, cherry], size=3)
Permutations with repetitions
The permutation may have more elements than slots. For example, the three
possible permutation of 12 in three slots are: 111, 211, 121, 221, 112, 212,
122, and 222.
Let's generate all possible permutations with repetitions of 3 elements from
the set of apple and orange:
// Create the initial vector of 2 elements (apple, orange)
ICombinatoricsVector<String> originalVector = Factory.createVector(new String[] { "apple", "orange" });
// Create the generator by calling the appropriate method in the Factory class.
// Set the second parameter as 3, since we will generate 3-elemets permutations
Generator<String> gen = Factory.createPermutationWithRepetitionGenerator(originalVector, 3);
// Print the result
for (ICombinatoricsVector<String> perm : gen)
System.out.println( perm );
And the result
CombinatoricsVector=([apple, apple, apple], size=3)
CombinatoricsVector=([orange, apple, apple], size=3)
CombinatoricsVector=([apple, orange, apple], size=3)
CombinatoricsVector=([orange, orange, apple], size=3)
CombinatoricsVector=([apple, apple, orange], size=3)
CombinatoricsVector=([orange, apple, orange], size=3)
CombinatoricsVector=([apple, orange, orange], size=3)
CombinatoricsVector=([orange, orange, orange], size=3)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy