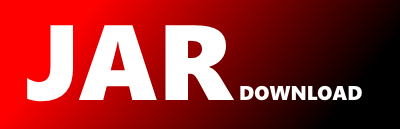
org.paukov.combinatorics.subsets.package.html Maven / Gradle / Ivy
This package contains the subsets generators.
A set A is a subset of a set B if A is "contained" inside B. A and B may
coincide. The relationship of one set being a subset of another is called
inclusion or sometimes containment.
Examples:
- The set (1, 2) is a proper subset of (1, 2, 3).
- Any set is a subset of itself, but not a proper subset.
- The empty set, denoted by (), is also a subset of any given set X.
All subsets of (1, 2, 3) are:
- ()
- (1)
- (2)
- (1, 2)
- (3)
- (1, 3)
- (2, 3)
- (1, 2, 3)
And code which generates all subsets of (one, two, three)
// Create an initial vector/set
ICombinatoricsVector<String> initialSet = CombinatoricsFactory.createVector(new String[] {
"one", "two", "three" });
// Create an instance of the subset generator
Generator<String> gen = CombinatoricsFactory.createSubSetGenerator(initialSet);
// Print the subsets
for (ICombinatoricsVector<String> subSet : gen) {
System.out.println(subSet);
}
And the result of all 8 possible subsets
CombinatoricsVector=([], size=0)
CombinatoricsVector=([one], size=1)
CombinatoricsVector=([two], size=1)
CombinatoricsVector=([one, two], size=2)
CombinatoricsVector=([three], size=1)
CombinatoricsVector=([one, three], size=2)
CombinatoricsVector=([two, three], size=2)
CombinatoricsVector=([one, two, three], size=3)
Version 2.0 of the combinatoricslib supports sets with duplicates. For
example, if the original vector contains duplicates like (a, b, a, c), then
the result will contain 14 subsets (instead of 16):
()
(a)
(b)
(a, b)
(a, a)
(b, a)
(a, b, a)
(c)
(a, c)
(b, c)
(a, b, c)
(a, a, c)
(b, a, c)
(a, b, a, c)
If you still would like to treat the set with duplicates as not identical,
you should create a generator and set the second parameter of the method
CombinatoricsFactory.createSubSetGenerator()
as false
. In this
case all 16 subsets will be generated.
Note. If the initial vector contains duplicates then the method
getNumberOfGeneratedObjects
won't be able to return the number
of the sub sets/lists. It will throw a runtime exception