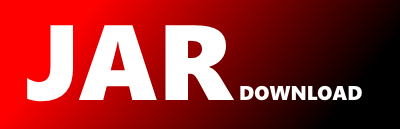
com.googlecode.cqengine.query.QueryFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cqengine Show documentation
Show all versions of cqengine Show documentation
Collection Query Engine: NoSQL indexing and query engine for Java collections with ultra-low latency
/**
* Copyright 2012-2015 Niall Gallagher
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.cqengine.query;
import com.googlecode.cqengine.IndexedCollection;
import com.googlecode.cqengine.attribute.Attribute;
import com.googlecode.cqengine.attribute.SimpleAttribute;
import com.googlecode.cqengine.query.option.*;
import com.googlecode.cqengine.query.simple.*;
import com.googlecode.cqengine.query.logical.And;
import com.googlecode.cqengine.query.logical.Not;
import com.googlecode.cqengine.query.logical.Or;
import com.googlecode.cqengine.query.option.AttributeOrder;
import java.util.*;
import java.util.regex.Pattern;
/**
* A static factory for creating {@link Query} objects and its descendants.
*
* @author Niall Gallagher
*/
public class QueryFactory {
/**
* Private constructor, not used.
*/
QueryFactory() {
}
/**
* Creates an {@link Equal} query which asserts that an attribute equals a certain value.
*
* @param attribute The attribute to which the query refers
* @param attributeValue The value to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link Equal} query
*/
public static Equal equal(Attribute attribute, A attributeValue) {
return new Equal(attribute, attributeValue);
}
/**
* Creates a {@link LessThan} query which asserts that an attribute is less than or equal to an upper bound
* (i.e. less than, inclusive).
*
* @param attribute The attribute to which the query refers
* @param attributeValue The upper bound to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return A {@link LessThan} query
*/
public static > LessThan lessThanOrEqualTo(Attribute attribute, A attributeValue) {
return new LessThan(attribute, attributeValue, true);
}
/**
* Creates a {@link LessThan} query which asserts that an attribute is less than (but not equal to) an upper
* bound (i.e. less than, exclusive).
*
* @param attribute The attribute to which the query refers
* @param attributeValue The upper bound to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return A {@link LessThan} query
*/
public static > LessThan lessThan(Attribute attribute, A attributeValue) {
return new LessThan(attribute, attributeValue, false);
}
/**
* Creates a {@link GreaterThan} query which asserts that an attribute is greater than or equal to a lower
* bound (i.e. greater than, inclusive).
*
* @param attribute The attribute to which the query refers
* @param attributeValue The lower bound to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return A {@link GreaterThan} query
*/
public static > GreaterThan greaterThanOrEqualTo(Attribute attribute, A attributeValue) {
return new GreaterThan(attribute, attributeValue, true);
}
/**
* Creates a {@link LessThan} query which asserts that an attribute is greater than (but not equal to) a lower
* bound (i.e. greater than, exclusive).
*
* @param attribute The attribute to which the query refers
* @param attributeValue The lower bound to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return A {@link GreaterThan} query
*/
public static > GreaterThan greaterThan(Attribute attribute, A attributeValue) {
return new GreaterThan(attribute, attributeValue, false);
}
/**
* Creates a {@link Between} query which asserts that an attribute is between a lower and an upper bound.
*
* @param attribute The attribute to which the query refers
* @param lowerValue The lower bound to be asserted by the query
* @param lowerInclusive Whether the lower bound is inclusive or not (true for "greater than or equal to")
* @param upperValue The upper bound to be asserted by the query
* @param upperInclusive Whether the upper bound is inclusive or not (true for "less than or equal to")
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return A {@link GreaterThan} query
*/
public static > Between between(Attribute attribute, A lowerValue, boolean lowerInclusive, A upperValue, boolean upperInclusive) {
return new Between(attribute, lowerValue, lowerInclusive, upperValue, upperInclusive);
}
/**
* Creates a {@link Between} query which asserts that an attribute is between a lower and an upper bound,
* inclusive.
*
* @param attribute The attribute to which the query refers
* @param lowerValue The lower bound to be asserted by the query
* @param upperValue The upper bound to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return A {@link GreaterThan} query
*/
public static > Between between(Attribute attribute, A lowerValue, A upperValue) {
return new Between(attribute, lowerValue, true, upperValue, true);
}
/**
* A shorthand way to create an {@link Or} query comprised of several {@link Equal} queries.
*
* @param attribute The attribute to which the query refers
* @param attributeValues The potential values for the {@link Equal} queries to be asserted by the {@link Or} query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link Or} query comprised of several {@link Equal} queries
*/
public static Or in(Attribute attribute, A... attributeValues) {
return in(attribute, Arrays.asList(attributeValues));
}
/**
* A shorthand way to create an {@link Or} query comprised of several {@link Equal} queries.
*
* Note that this can result in more efficient queries than several {@link Equal} queries "OR"ed together
* using other means.
*
* If the given attribute is a {@link SimpleAttribute}, this method will set a hint in the query to
* indicate that results for the child queries will inherently be disjoint and so will not require deduplication.
*
* @param attribute The attribute to which the query refers
* @param attributeValues The potential values for the {@link Equal} queries to be asserted by the {@link Or} query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link Or} query comprised of several {@link Equal} queries
*/
public static Or in(Attribute attribute, Collection attributeValues) {
List> equalStatements = new ArrayList>(attributeValues.size());
for (A attributeValue : attributeValues) {
Equal equalStatement = equal(attribute, attributeValue);
equalStatements.add(equalStatement);
}
return new Or(equalStatements, attribute instanceof SimpleAttribute);
}
/**
* Creates a {@link StringStartsWith} query which asserts that an attribute starts with a certain string fragment.
*
* @param attribute The attribute to which the query refers
* @param attributeValue The value to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link StringStartsWith} query
*/
public static StringStartsWith startsWith(Attribute attribute, A attributeValue) {
return new StringStartsWith(attribute, attributeValue);
}
/**
* Creates a {@link StringEndsWith} query which asserts that an attribute ends with a certain string fragment.
*
* @param attribute The attribute to which the query refers
* @param attributeValue The value to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link StringEndsWith} query
*/
public static StringEndsWith endsWith(Attribute attribute, A attributeValue) {
return new StringEndsWith(attribute, attributeValue);
}
/**
* Creates a {@link StringContains} query which asserts that an attribute contains with a certain string fragment.
*
* @param attribute The attribute to which the query refers
* @param attributeValue The value to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link StringContains} query
*/
public static StringContains contains(Attribute attribute, A attributeValue) {
return new StringContains(attribute, attributeValue);
}
/**
* Creates a {@link StringIsContainedIn} query which asserts that an attribute is contained in a certain string
* fragment.
*
* @param attribute The attribute to which the query refers
* @param attributeValue The value to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link StringStartsWith} query
*/
public static StringIsContainedIn isContainedIn(Attribute attribute, A attributeValue) {
return new StringIsContainedIn(attribute, attributeValue);
}
/**
* Creates a {@link StringMatchesRegex} query which asserts that an attribute's value matches a regular expression.
*
* To accelerate {@code matchesRegex(...)} queries, add a Standing Query Index on {@code matchesRegex(...)}.
*
* @param attribute The attribute to which the query refers
* @param regexPattern The regular expression pattern to be asserted by the query
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link StringStartsWith} query
*/
public static StringMatchesRegex matchesRegex(Attribute attribute, Pattern regexPattern) {
return new StringMatchesRegex(attribute, regexPattern);
}
/**
* Creates a {@link StringMatchesRegex} query which asserts that an attribute's value matches a regular expression.
*
* To accelerate {@code matchesRegex(...)} queries, add a Standing Query Index on {@code matchesRegex(...)}.
*
* @param attribute The attribute to which the query refers
* @param regex The regular expression to be asserted by the query (this will be compiled via {@link Pattern#compile(String)})
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link StringStartsWith} query
*/
public static StringMatchesRegex matchesRegex(Attribute attribute, String regex) {
return new StringMatchesRegex(attribute, Pattern.compile(regex));
}
/**
* Creates an {@link Has} query which asserts that an attribute has a value (is not null).
*
* Asserts that an attribute has a value (is not null).
*
* To accelerate {@code has(...)} queries, add a Standing Query Index on {@code has(...)}.
*
* To assert that an attribute does not have a value (is null), use not(has(...))
.
*
* To accelerate not(has(...))
queries, add a Standing Query Index on not(has(...))
.
*
* @param attribute The attribute to which the query refers
* @param The type of the attribute
* @param The type of the object containing the attribute
* @return An {@link Has} query
*/
public static Has has(Attribute attribute) {
return new Has(attribute);
}
/**
* Creates an {@link And} query, representing a logical AND on child queries, which when evaluated yields the
* set intersection of the result sets from child queries.
*
* @param queries The child queries to be connected via a logical AND
* @param The type of the object containing attributes to which child queries refer
* @return An {@link And} query, representing a logical AND on child queries
*/
public static And and(Query... queries) {
return new And(Arrays.asList(queries));
}
/**
* Creates an {@link And} query, representing a logical AND on child queries, which when evaluated yields the
* set intersection of the result sets from child queries.
*
* @param queries The child queries to be connected via a logical AND
* @param The type of the object containing attributes to which child queries refer
* @return An {@link And} query, representing a logical AND on child queries
*/
public static And and(Collection> queries) {
return new And(queries);
}
/**
* Creates an {@link Or} query, representing a logical OR on child queries, which when evaluated yields the
* set union of the result sets from child queries.
*
* @param queries The child queries to be connected via a logical OR
* @param The type of the object containing attributes to which child queries refer
* @return An {@link Or} query, representing a logical OR on child queries
*/
public static Or or(Query... queries) {
return new Or(Arrays.asList(queries));
}
/**
* Creates an {@link Or} query, representing a logical OR on child queries, which when evaluated yields the
* set union of the result sets from child queries.
*
* @param queries The child queries to be connected via a logical OR
* @param The type of the object containing attributes to which child queries refer
* @return An {@link Or} query, representing a logical OR on child queries
*/
public static Or or(Collection> queries) {
return new Or(queries);
}
/**
* Creates a {@link Not} query, representing a logical negation of a child query, which when evaluated
* yields the set complement of the result set from the child query.
*
* @param query The child query to be logically negated
* @param The type of the object containing attributes to which child queries refer
* @return A {@link Not} query, representing a logical negation of a child query
*/
public static Not not(Query query) {
return new Not(query);
}
/**
* Creates a query supporting the equivalent of SQL EXISTS
.
*
* Asserts that objects in a local {@code IndexedCollection} match objects in a foreign collection,
* based on a key attribute of local objects being equal to a key attribute of the foreign objects.
* This query can be performed on the local collection, supplying the foreign collection and the
* relevant attributes, as arguments to the query.
*
* This supports the SQL equivalent of:
*
* SELECT * From LocalCollection
* WHERE EXISTS (
* SELECT * FROM ForeignCollection
* WHERE LocalCollection.localAttribute = ForeignCollection.foreignAttribute
* )
*
*
* @param foreignCollection The collection of foreign objects
* @param localKeyAttribute An attribute of the local object
* @param foreignKeyAttribute An attribute of objects in the foreign collection
* @param The type of the local object
* @param The type of the foreign objects
* @param The type of the common attributes
* @return A query which checks if the local object matches any objects in the foreign collection based on the given
* key attributes being equal
*/
public static Query existsIn(final IndexedCollection foreignCollection, final Attribute localKeyAttribute, final Attribute foreignKeyAttribute) {
return new ExistsIn(foreignCollection, localKeyAttribute, foreignKeyAttribute);
}
/**
* Creates a query supporting the equivalent of SQL EXISTS
,
* with some additional restrictions on foreign objects.
*
* Asserts that objects in a local {@code IndexedCollection} match objects in a foreign collection,
* based on a key attribute of local objects being equal to a key attribute of the foreign objects,
* AND objects in the foreign collection matching some additional criteria.
* This query can be performed on the local collection, supplying the foreign collection and the
* relevant attributes, as arguments to the query.
*
* This supports the SQL equivalent of:
*
* SELECT * From LocalCollection
* WHERE EXISTS (
* SELECT * FROM ForeignCollection
* WHERE LocalCollection.localAttribute = ForeignCollection.foreignAttribute
* AND ([AND|OR|NOT](ForeignCollection.someOtherAttribute = x) ...)
* )
*
* @param foreignCollection The collection of foreign objects
* @param localKeyAttribute An attribute of the local object
* @param foreignKeyAttribute An attribute of objects in the foreign collection
* @param foreignRestrictions A query specifying additional restrictions on foreign objects
* @param The type of the local object
* @param The type of the foreign objects
* @param The type of the common attributes
* @return A query which checks if the local object matches any objects in the foreign collection based on the given
* key attributes being equal
*/
public static Query existsIn(final IndexedCollection foreignCollection, final Attribute localKeyAttribute, final Attribute foreignKeyAttribute, final Query foreignRestrictions) {
return new ExistsIn(foreignCollection, localKeyAttribute, foreignKeyAttribute, foreignRestrictions);
}
/**
* Creates a query which matches all objects in the collection.
*
* This is equivalent to a literal boolean 'true'.
*
* @param The type of the objects in the collection
* @return A query which matches all objects in the collection
*/
public static Query all(Class objectType) {
return new All(objectType);
}
/**
* Creates a query which matches no objects in the collection.
*
* This is equivalent to a literal boolean 'false'.
*
* @param The type of the objects in the collection
* @return A query which matches no objects in the collection
*/
public static Query none(Class objectType) {
return new None(objectType);
}
/**
* Creates an {@link OrderByOption} query option, encapsulating the given list of {@link AttributeOrder} objects
* which pair an attribute with a preference to sort results by that attribute in either ascending or descending
* order.
*
* @param attributeOrders The list of attribute orders by which objects should be sorted
* @param The type of the object containing the attributes
* @return An {@link OrderByOption} query option, requests results to be sorted in the given order
*/
public static OrderByOption orderBy(List> attributeOrders) {
return new OrderByOption(attributeOrders);
}
/**
* Creates an {@link OrderByOption} query option, encapsulating the given list of {@link AttributeOrder} objects
* which pair an attribute with a preference to sort results by that attribute in either ascending or descending
* order.
*
* @param attributeOrders The list of attribute orders by which objects should be sorted
* @param The type of the object containing the attributes
* @return An {@link OrderByOption} query option, requests results to be sorted in the given order
*/
public static OrderByOption orderBy(AttributeOrder... attributeOrders) {
return new OrderByOption(Arrays.asList(attributeOrders));
}
/**
* Creates an {@link AttributeOrder} object which pairs an attribute with a preference to sort results by that
* attribute in ascending order. These {@code AttributeOrder} objects can then be passed to the
* {@link #orderBy(com.googlecode.cqengine.query.option.AttributeOrder[])} method to create a query option which
* sorts results by the indicated attributes and ascending/descending preferences.
*
* @param attribute An attribute to sort by
* @param The type of the object containing the attributes
* @return An {@link AttributeOrder} object, encapsulating the attribute and a preference to sort results by it
* in ascending order
*/
public static AttributeOrder ascending(Attribute attribute) {
return new AttributeOrder(attribute, false);
}
/**
* Creates an {@link AttributeOrder} object which pairs an attribute with a preference to sort results by that
* attribute in descending order. These {@code AttributeOrder} objects can then be passed to the
* {@link #orderBy(com.googlecode.cqengine.query.option.AttributeOrder[])} method to create a query option which
* sorts results by the indicated attributes and ascending/descending preferences.
*
* @param attribute An attribute to sort by
* @param The type of the object containing the attributes
* @return An {@link AttributeOrder} object, encapsulating the attribute and a preference to sort results by it
* in descending order
*/
public static AttributeOrder descending(Attribute attribute) {
return new AttributeOrder(attribute, true);
}
/**
* Creates an {@link OrderingStrategyOption} query option, encapsulating a given {@link OrderingStrategy}, which
* when supplied to the query engine requests it to use the given strategy to order results.
*
* @param orderingStrategy The ordering strategy the query engine should use
* @return An {@link OrderingStrategyOption} query option
*/
public static OrderingStrategyOption orderingStrategy(OrderingStrategy orderingStrategy) {
return new OrderingStrategyOption(orderingStrategy);
}
/**
* Creates a {@link DeduplicationOption} query option, encapsulating a given {@link DeduplicationStrategy}, which
* when supplied to the query engine requests it to eliminate duplicates objects from the results returned using
* the strategy indicated.
*
* @param deduplicationStrategy The deduplication strategy the query engine should use
* @return A {@link DeduplicationOption} query option, requests duplicate objects to be eliminated from results
*/
public static DeduplicationOption deduplicate(DeduplicationStrategy deduplicationStrategy) {
return new DeduplicationOption(deduplicationStrategy);
}
/**
* Creates a {@link IsolationOption} query option, encapsulating a given {@link IsolationLevel}, which
* when supplied to the query engine requests that level of transaction isolation.
*
* @param isolationLevel The transaction isolation level to request
* @return An {@link IsolationOption} query option
*/
public static IsolationOption isolationLevel(IsolationLevel isolationLevel) {
return new IsolationOption(isolationLevel);
}
/**
* Creates an {@link ArgumentValidationOption} query option, encapsulating a given
* {@link ArgumentValidationStrategy}, which when supplied to the query engine requests that some argument
* validation may be disabled (or enabled) for performance or reliability reasons.
*
* @param strategy The argument validation strategy to request
* @return An {@link ArgumentValidationOption} query option
*/
public static ArgumentValidationOption argumentValidation(ArgumentValidationStrategy strategy) {
return new ArgumentValidationOption(strategy);
}
/**
* A convenience method to encapsulate several objects together as {@link com.googlecode.cqengine.query.option.QueryOptions},
* where the class of the object will become its key in the QueryOptions map.
*
* @param queryOptions The objects to encapsulate as QueryOptions
* @return A {@link QueryOptions} object
*/
public static QueryOptions queryOptions(Object... queryOptions) {
return queryOptions(Arrays.asList(queryOptions));
}
/**
* A convenience method to encapsulate a collection of objects as {@link com.googlecode.cqengine.query.option.QueryOptions},
* where the class of the object will become its key in the QueryOptions map.
*
* @param queryOptions The objects to encapsulate as QueryOptions
* @return A {@link QueryOptions} object
*/
public static QueryOptions queryOptions(Collection