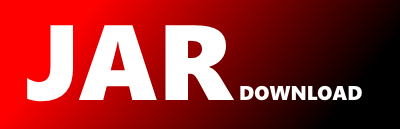
com.googlecode.cqengine.index.fallback.FallbackIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cqengine Show documentation
Show all versions of cqengine Show documentation
Collection Query Engine: NoSQL indexing and query engine for Java collections with ultra-low latency
/**
* Copyright 2012-2015 Niall Gallagher
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.cqengine.index.fallback;
import com.googlecode.cqengine.index.Index;
import com.googlecode.cqengine.query.Query;
import com.googlecode.cqengine.query.option.QueryOptions;
import com.googlecode.cqengine.query.simple.All;
import com.googlecode.cqengine.query.simple.None;
import com.googlecode.cqengine.resultset.filter.FilteringIterator;
import com.googlecode.cqengine.resultset.ResultSet;
import com.googlecode.cqengine.resultset.iterator.IteratorUtil;
import java.util.*;
/**
* A special index which when asked to retrieve data simply scans the underlying collection for matching objects.
* This index does not maintain any data structure of its own.
*
* This index supports all query types, because it it relies on the supplied query object itself
* to determine if objects in the collection match the query, by calling
* {@link Query#matches(Object, com.googlecode.cqengine.query.option.QueryOptions)}.
*
* The query engine automatically uses this fallback index when an attribute is referenced by a query,
* and no other index has been added for that attribute that supports the query.
*
* The time complexity of retrievals from this fallback index is usually O(n) - linear, proportional to the number of
* objects in the collection.
*
* @author Niall Gallagher
*/
public class FallbackIndex implements Index {
private static final int INDEX_RETRIEVAL_COST = Integer.MAX_VALUE;
private static final int INDEX_MERGE_COST = Integer.MAX_VALUE;
private Set collection = Collections.emptySet();
public FallbackIndex() {
}
/**
* {@inheritDoc}
*
* This index is mutable.
*
* @return true
*/
@Override
public boolean isMutable() {
return true;
}
/**
* {@inheritDoc}
*
* This implementation always returns true, as this index supports all types of query.
*
* @return true, this index supports all types of query
*/
@Override
public boolean supportsQuery(Query query) {
return true;
}
@Override
public boolean isQuantized() {
return false;
}
/**
* {@inheritDoc}
*/
@Override
public ResultSet retrieve(final Query query, final QueryOptions queryOptions) {
return new ResultSet() {
@Override
public Iterator iterator() {
if (query instanceof All) {
return IteratorUtil.wrapAsUnmodifiable(collection.iterator());
}
else if (query instanceof None) {
return Collections.emptyList().iterator();
}
else {
return new FilteringIterator(collection.iterator(), queryOptions) {
@Override
public boolean isValid(O object, QueryOptions queryOptions) {
return query.matches(object, queryOptions);
}
};
}
}
@Override
public boolean contains(O object) {
// Contains is based on objects contained in this *filtered* ResultSet, so delegate to iterator...
return IteratorUtil.iterableContains(this, object);
}
@Override
public int size() {
// Size is based on objects contained in this *filtered* ResultSet, so delegate to iterator...
return IteratorUtil.countElements(this);
}
@Override
public boolean matches(O object) {
return query.matches(object, queryOptions);
}
@Override
public int getRetrievalCost() {
return INDEX_RETRIEVAL_COST;
}
@Override
public int getMergeCost() {
return INDEX_MERGE_COST;
}
@Override
public void close() {
// No op.
}
@Override
public Query getQuery() {
return query;
}
@Override
public QueryOptions getQueryOptions() {
return queryOptions;
}
};
}
/**
* {@inheritDoc}
*
* In this implementation, does nothing.
*/
@Override
public boolean addAll(Collection objects, QueryOptions queryOptions) {
// No need to take any action
return false;
}
/**
* {@inheritDoc}
*
* In this implementation, does nothing.
*/
@Override
public boolean removeAll(Collection objects, QueryOptions queryOptions) {
// No need to take any action
return false;
}
/**
* {@inheritDoc}
*
* In this implementation, stores a reference to the supplied collection, which the
* {@link Index#retrieve(com.googlecode.cqengine.query.Query, com.googlecode.cqengine.query.option.QueryOptions)} method can subsequently iterate.
*/
@Override
public void init(Set collection, QueryOptions queryOptions) {
// Store the collection...
this.collection = collection;
}
/**
* {@inheritDoc}
* @param queryOptions
*/
@Override
public void clear(QueryOptions queryOptions) {
collection.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy