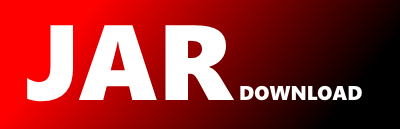
org.etlunit.cli.SetCmd Maven / Gradle / Ivy
package org.etlunit.cli;
import org.apache.maven.model.Model;
import org.apache.maven.model.io.DefaultModelReader;
import org.apache.maven.project.MavenProject;
import org.clamshellcli.api.Command;
import org.clamshellcli.api.Configurator;
import org.clamshellcli.api.Context;
import org.clamshellcli.api.IOConsole;
import org.etlunit.Configuration;
import org.etlunit.ETLTestVM;
import org.etlunit.feature.*;
import org.etlunit.maven.ETLUnitMojo;
import org.etlunit.util.MapList;
import javax.inject.Inject;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SetCmd implements Command
{
private static final String NAMESPACE = "syscmd";
public static final String ACTION_NAME = "set";
private static Map statelessOptions = new HashMap();
private MapList mapList;
public static void prepareVm(ETLTestVM vm)
{
vm.getRuntimeSupport().overrideRuntimeOptions(new ArrayList(statelessOptions.values()));
}
public Object execute(Context ctx)
{
IOConsole console = ctx.getIoConsole();
String[] inputLine = (String[]) ctx.getValue(Context.KEY_COMMAND_LINE_ARGS);
String featureName = null;
if (inputLine != null && inputLine.length == 1)
{
featureName = inputLine[0];
}
try
{
ETLTestVM vm = TestCmd.getEtlTestVM();
vm.addFeature(new AbstractFeature()
{
private String name = "mapListGrabber." + System.currentTimeMillis();
@Inject
public void receiveRuntimeSupport(MapList ml)
{
mapList = ml;
}
@Override
public String getFeatureName()
{
return name;
}
});
vm.installFeatures();
console.writeOutput("Overridden options:" + Configurator.VALUE_LINE_SEP);
for (Map.Entry optionEntry : statelessOptions.entrySet())
{
RuntimeOption option = optionEntry.getValue();
RuntimeOptionDescriptor descriptor = option.getDescriptor();
console.writeOutput("\t" + option.getName() + " - " + descriptor.getDescription() + Configurator.VALUE_LINE_SEP);
switch (descriptor.getOptionType())
{
case bool:
console.writeOutput("\t\tEnabled: " + option.isEnabled() + Configurator.VALUE_LINE_SEP);
break;
case integer:
console.writeOutput("\t\tValue: " + option.getIntegerValue() + Configurator.VALUE_LINE_SEP);
break;
case string:
console.writeOutput("\t\tValue: " + option.getStringValue() + Configurator.VALUE_LINE_SEP);
break;
}
}
Feature targetFeature = null;
if (featureName != null)
{
for (Feature feature : mapList.keySet())
{
if (feature.getFeatureName().equals(featureName))
{
targetFeature = feature;
}
}
if (targetFeature == null)
{
console.writeOutput("Invalid feature '" + featureName + "'" + Configurator.VALUE_LINE_SEP);
}
}
if (targetFeature == null)
{
console.writeOutput("Installed features:" + Configurator.VALUE_LINE_SEP);
int featureIndex = 0;
Map fmap = new HashMap();
for (Feature feature : mapList.keySet())
{
String featureKey = String.valueOf(featureIndex++);
fmap.put(featureKey, feature);
console.writeOutput("[" + featureKey + "] - " + feature.getFeatureName() + Configurator.VALUE_LINE_SEP);
}
console.writeOutput("[x] - cancel" + Configurator.VALUE_LINE_SEP);
while (targetFeature == null)
{
String res = console.readInput("Select a feature:").trim();
if (res.equalsIgnoreCase("x"))
{
return null;
}
targetFeature = fmap.get(res);
if (targetFeature == null)
{
console.writeOutput("Invalid selection - '" + res + "'" + Configurator.VALUE_LINE_SEP);
}
}
}
RuntimeOption ro = null;
for (Map.Entry> feature : mapList.entrySet())
{
Feature featureKey = feature.getKey();
String thisFeatureName = featureKey.getFeatureName();
if (targetFeature != featureKey)
{
continue;
}
console.writeOutput(thisFeatureName + Configurator.VALUE_LINE_SEP);
int roIndex = 0;
Map romap = new HashMap();
for (RuntimeOption option : feature.getValue())
{
String roKey = String.valueOf(roIndex++);
romap.put(roKey, option);
RuntimeOptionDescriptor descriptor = option.getDescriptor();
console.writeOutput("\t[" + roKey + "] - " + descriptor.getName() + " - " + descriptor.getDescription() + Configurator.VALUE_LINE_SEP);
switch (descriptor.getOptionType())
{
case bool:
console.writeOutput("\t\tEnabled: " + option.isEnabled() + Configurator.VALUE_LINE_SEP);
break;
case integer:
console.writeOutput("\t\tValue: " + option.getIntegerValue() + Configurator.VALUE_LINE_SEP);
break;
case string:
console.writeOutput("\t\tValue: " + option.getStringValue() + Configurator.VALUE_LINE_SEP);
break;
}
}
console.writeOutput("\t[x] - cancel" + Configurator.VALUE_LINE_SEP);
while (ro == null)
{
String res = console.readInput("Select an option:").trim();
if (res.equalsIgnoreCase("x"))
{
return null;
}
ro = romap.get(res);
if (ro == null)
{
console.writeOutput("Invalid selection - '" + res + "'" + Configurator.VALUE_LINE_SEP);
}
}
}
console.writeOutput("Updating - '" + ro.getName() + "'" + Configurator.VALUE_LINE_SEP);
// prompt for the new value
switch (ro.getDescriptor().getOptionType())
{
case bool:
boolean defBo = ro.getDescriptor().getDefaultBooleanValue();
statelessOptions.put(ro.getName(), option(new RuntimeOption(ro.getName(), getBool(console, defBo)), ro));
break;
case integer:
statelessOptions.put(ro.getName(), option(new RuntimeOption(ro.getName(), getInt(console, ro.getDescriptor().getDefaultIntegerValue())), ro));
break;
case string:
String in = getString(console, ro.getDescriptor().getDefaultStringValue());
statelessOptions.put(ro.getName(), option(new RuntimeOption(ro.getName(), in), ro));
break;
}
}
catch (Exception exc)
{
console.writeOutput(exc.toString() + Configurator.VALUE_LINE_SEP);
exc.printStackTrace(System.out);
}
return null;
}
private RuntimeOption option(RuntimeOption runtimeOption, RuntimeOption ro)
{
runtimeOption.setDescriptor(ro.getDescriptor());
runtimeOption.setFeature(ro.getFeature());
return runtimeOption;
}
private boolean getBool(IOConsole console, boolean defBo)
{
while (true)
{
String in = console.readInput("\tEnabled " + (defBo ? "Y/n" : "y/N")+ ": ").trim();
if (in.equalsIgnoreCase("Y"))
{
return true;
}
else if (in.equalsIgnoreCase("N"))
{
return false;
}
else if (in.equals(""))
{
return defBo;
}
console.writeOutput("Invalid boolean - '" + in + "' - please enter 'y' or 'n' or blank for the default" + Configurator.VALUE_LINE_SEP);
}
}
private String getString(IOConsole console, String defBo)
{
String in = console.readInput("\tValue [" + defBo + "]: ").trim();
if (in.equals(""))
{
return defBo;
}
return in;
}
private int getInt(IOConsole console, int def)
{
while (true)
{
String in = console.readInput("\tInteger Value [" + def + "]: ").trim();
if (in.equals(""))
{
return def;
}
try
{
return Integer.parseInt(in);
}
catch(NumberFormatException exc)
{
console.writeOutput("Invalid int - '" + in + "' - please enter a valid integer or blank for the default" + Configurator.VALUE_LINE_SEP);
}
}
}
public void plug(Context plug)
{
// no load-time setup needed
}
public Descriptor getDescriptor()
{
return new Descriptor()
{
public String getNamespace()
{
return NAMESPACE;
}
public String getName()
{
return ACTION_NAME;
}
public String getDescription()
{
return "Displays or sets options for system or for a feature";
}
public String getUsage()
{
return "Type [featureName]";
}
public Map getArguments()
{
Map map = new HashMap();
map.put("feature", "Optional feature name");
return map;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy